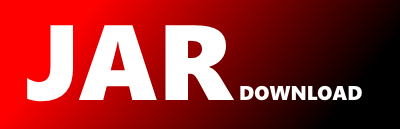
tech.picnic.errorprone.refasterrules.TimeRulesRecipes Maven / Gradle / Ivy
Show all versions of error-prone-contrib Show documentation
package tech.picnic.errorprone.refasterrules;
import org.openrewrite.ExecutionContext;
import org.openrewrite.Preconditions;
import org.openrewrite.Recipe;
import org.openrewrite.TreeVisitor;
import org.openrewrite.internal.lang.NonNullApi;
import org.openrewrite.java.JavaParser;
import org.openrewrite.java.JavaTemplate;
import org.openrewrite.java.JavaVisitor;
import org.openrewrite.java.search.*;
import org.openrewrite.java.template.Primitive;
import org.openrewrite.java.template.function.*;
import org.openrewrite.java.template.internal.AbstractRefasterJavaVisitor;
import org.openrewrite.java.tree.*;
import javax.annotation.Generated;
import java.util.*;
import static org.openrewrite.java.template.internal.AbstractRefasterJavaVisitor.EmbeddingOption.*;
/**
* OpenRewrite recipes created for Refaster template {@code tech.picnic.errorprone.refasterrules.TimeRules}.
*/
@SuppressWarnings("all")
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public class TimeRulesRecipes extends Recipe {
/**
* Instantiates a new instance.
*/
public TimeRulesRecipes() {}
@Override
public String getDisplayName() {
return "Refaster rules related to expressions dealing with time";
}
@Override
public String getDescription() {
return "Refaster template recipes for `tech.picnic.errorprone.refasterrules.TimeRules`. [Source](https://error-prone.picnic.tech/refasterrules/TimeRules).";
}
@Override
public List getRecipeList() {
return Arrays.asList(
new ClockInstantRecipe(),
new UtcConstantRecipe(),
new LocalDateOfInstantRecipe(),
new LocalDateTimeOfInstantRecipe(),
new LocalTimeOfInstantRecipe(),
new OffsetDateTimeOfInstantRecipe(),
new InstantAtOffsetRecipe(),
new OffsetTimeOfInstantRecipe(),
new InstantAtZoneRecipe(),
new UtcClockRecipe(),
new EpochInstantRecipe(),
new InstantIsBeforeRecipe(),
new InstantIsAfterRecipe(),
new LocalTimeMinRecipe(),
new LocalDateAtStartOfDayRecipe(),
new ChronoLocalDateIsBeforeRecipe(),
new ChronoLocalDateIsAfterRecipe(),
new ChronoLocalDateTimeIsBeforeRecipe(),
new ChronoLocalDateTimeIsAfterRecipe(),
new ChronoZonedDateTimeIsBeforeRecipe(),
new ChronoZonedDateTimeIsAfterRecipe(),
new OffsetDateTimeIsBeforeRecipe(),
new OffsetDateTimeIsAfterRecipe(),
new ZeroDurationRecipe(),
new DurationOfDaysRecipe(),
new DurationOfHoursRecipe(),
new DurationOfMillisRecipe(),
new DurationOfMinutesRecipe(),
new DurationOfNanosRecipe(),
new DurationOfSecondsRecipe(),
new DurationBetweenInstantsRecipe(),
new DurationBetweenOffsetDateTimesRecipe(),
new DurationIsZeroRecipe(),
new ZeroPeriodRecipe(),
new LocalDatePlusDaysRecipe(),
new LocalDatePlusWeeksRecipe(),
new LocalDatePlusMonthsRecipe(),
new LocalDatePlusYearsRecipe(),
new LocalDateMinusDaysRecipe(),
new LocalDateMinusWeeksRecipe(),
new LocalDateMinusMonthsRecipe(),
new LocalDateMinusYearsRecipe(),
new LocalTimePlusNanosRecipe(),
new LocalTimePlusSecondsRecipe(),
new LocalTimePlusMinutesRecipe(),
new LocalTimePlusHoursRecipe(),
new LocalTimeMinusNanosRecipe(),
new LocalTimeMinusSecondsRecipe(),
new LocalTimeMinusMinutesRecipe(),
new LocalTimeMinusHoursRecipe(),
new OffsetTimePlusNanosRecipe(),
new OffsetTimePlusSecondsRecipe(),
new OffsetTimePlusMinutesRecipe(),
new OffsetTimePlusHoursRecipe(),
new OffsetTimeMinusNanosRecipe(),
new OffsetTimeMinusSecondsRecipe(),
new OffsetTimeMinusMinutesRecipe(),
new OffsetTimeMinusHoursRecipe(),
new LocalDateTimePlusNanosRecipe(),
new LocalDateTimePlusSecondsRecipe(),
new LocalDateTimePlusMinutesRecipe(),
new LocalDateTimePlusHoursRecipe(),
new LocalDateTimePlusDaysRecipe(),
new LocalDateTimePlusWeeksRecipe(),
new LocalDateTimePlusMonthsRecipe(),
new LocalDateTimePlusYearsRecipe(),
new LocalDateTimeMinusNanosRecipe(),
new LocalDateTimeMinusSecondsRecipe(),
new LocalDateTimeMinusMinutesRecipe(),
new LocalDateTimeMinusHoursRecipe(),
new LocalDateTimeMinusDaysRecipe(),
new LocalDateTimeMinusWeeksRecipe(),
new LocalDateTimeMinusMonthsRecipe(),
new LocalDateTimeMinusYearsRecipe(),
new OffsetDateTimePlusNanosRecipe(),
new OffsetDateTimePlusSecondsRecipe(),
new OffsetDateTimePlusMinutesRecipe(),
new OffsetDateTimePlusHoursRecipe(),
new OffsetDateTimePlusDaysRecipe(),
new OffsetDateTimePlusWeeksRecipe(),
new OffsetDateTimePlusMonthsRecipe(),
new OffsetDateTimePlusYearsRecipe(),
new OffsetDateTimeMinusNanosRecipe(),
new OffsetDateTimeMinusSecondsRecipe(),
new OffsetDateTimeMinusMinutesRecipe(),
new OffsetDateTimeMinusHoursRecipe(),
new OffsetDateTimeMinusDaysRecipe(),
new OffsetDateTimeMinusWeeksRecipe(),
new OffsetDateTimeMinusMonthsRecipe(),
new OffsetDateTimeMinusYearsRecipe(),
new ZonedDateTimePlusNanosRecipe(),
new ZonedDateTimePlusSecondsRecipe(),
new ZonedDateTimePlusMinutesRecipe(),
new ZonedDateTimePlusHoursRecipe(),
new ZonedDateTimePlusDaysRecipe(),
new ZonedDateTimePlusWeeksRecipe(),
new ZonedDateTimePlusMonthsRecipe(),
new ZonedDateTimePlusYearsRecipe(),
new ZonedDateTimeMinusNanosRecipe(),
new ZonedDateTimeMinusSecondsRecipe(),
new ZonedDateTimeMinusMinutesRecipe(),
new ZonedDateTimeMinusHoursRecipe(),
new ZonedDateTimeMinusDaysRecipe(),
new ZonedDateTimeMinusWeeksRecipe(),
new ZonedDateTimeMinusMonthsRecipe(),
new ZonedDateTimeMinusYearsRecipe()
);
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.ClockInstant}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class ClockInstantRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public ClockInstantRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `Clock#instant()` over `Instant#now(Clock)`, as it is more concise and more \"OOP-py\"";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class ClockInstant {\n \n @BeforeTemplate\n Instant before(Clock clock) {\n return Instant.now(clock);\n }\n \n @AfterTemplate\n Instant after(Clock clock) {\n return clock.instant();\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("java.time.Instant.now(#{clock:any(java.time.Clock)})")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{clock:any(java.time.Clock)}.instant()")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.Instant", true),
new UsesType<>("java.time.Clock", true),
new UsesMethod<>("java.time.Instant now(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.UtcConstant}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class UtcConstantRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public UtcConstantRecipe() {}
@Override
public String getDisplayName() {
return "Use `ZoneOffset#UTC` when possible";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class UtcConstant {\n \n @BeforeTemplate\n ZoneId before() {\n return Refaster.anyOf(ZoneId.of(\"GMT\"), ZoneId.of(\"UTC\"), ZoneId.of(\"+0\"), ZoneId.of(\"-0\"), UTC.normalized(), ZoneId.from(UTC));\n }\n \n @AfterTemplate\n ZoneOffset after() {\n return UTC;\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before$0 = JavaTemplate
.builder("java.time.ZoneId.of(\"GMT\")")
.build();
final JavaTemplate before$1 = JavaTemplate
.builder("java.time.ZoneId.of(\"UTC\")")
.build();
final JavaTemplate before$2 = JavaTemplate
.builder("java.time.ZoneId.of(\"+0\")")
.build();
final JavaTemplate before$3 = JavaTemplate
.builder("java.time.ZoneId.of(\"-0\")")
.build();
final JavaTemplate before$4 = JavaTemplate
.builder("java.time.ZoneOffset.UTC.normalized()")
.build();
final JavaTemplate before$5 = JavaTemplate
.builder("java.time.ZoneId.from(java.time.ZoneOffset.UTC)")
.build();
final JavaTemplate after = JavaTemplate
.builder("java.time.ZoneOffset.UTC")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before$0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.ZoneId");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace()),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before$1.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.ZoneId");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace()),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before$2.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.ZoneId");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace()),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before$3.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.ZoneId");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace()),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before$4.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.ZoneId");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace()),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before$5.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.ZoneId");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace()),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.ZoneId", true),
Preconditions.or(
new UsesMethod<>("java.time.ZoneId of(..)"),
new UsesMethod<>("java.time.ZoneId of(..)"),
new UsesMethod<>("java.time.ZoneId of(..)"),
new UsesMethod<>("java.time.ZoneId of(..)"),
new UsesMethod<>("java.time.ZoneOffset normalized(..)"),
new UsesMethod<>("java.time.ZoneId from(..)")
)
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.LocalDateOfInstant}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class LocalDateOfInstantRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public LocalDateOfInstantRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `LocalDate#ofInstant(Instant, ZoneId)` over more indirect alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class LocalDateOfInstant {\n \n @BeforeTemplate\n LocalDate before(Instant instant, ZoneId zoneId) {\n return Refaster.anyOf(instant.atZone(zoneId).toLocalDate(), LocalDateTime.ofInstant(instant, zoneId).toLocalDate(), OffsetDateTime.ofInstant(instant, zoneId).toLocalDate());\n }\n \n @BeforeTemplate\n LocalDate before(Instant instant, ZoneOffset zoneId) {\n return instant.atOffset(zoneId).toLocalDate();\n }\n \n @AfterTemplate\n LocalDate after(Instant instant, ZoneId zoneId) {\n return LocalDate.ofInstant(instant, zoneId);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before$0 = JavaTemplate
.builder("#{instant:any(java.time.Instant)}.atZone(#{zoneId:any(java.time.ZoneId)}).toLocalDate()")
.build();
final JavaTemplate before$1 = JavaTemplate
.builder("java.time.LocalDateTime.ofInstant(#{instant:any(java.time.Instant)}, #{zoneId:any(java.time.ZoneId)}).toLocalDate()")
.build();
final JavaTemplate before$2 = JavaTemplate
.builder("java.time.OffsetDateTime.ofInstant(#{instant:any(java.time.Instant)}, #{zoneId:any(java.time.ZoneId)}).toLocalDate()")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{instant:any(java.time.Instant)}.atOffset(#{zoneId:any(java.time.ZoneOffset)}).toLocalDate()")
.build();
final JavaTemplate after = JavaTemplate
.builder("java.time.LocalDate.ofInstant(#{instant:any(java.time.Instant)}, #{zoneId:any(java.time.ZoneId)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before$0.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before$1.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.LocalDateTime");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before$2.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.OffsetDateTime");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.ZoneOffset");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.LocalDate", true),
new UsesType<>("java.time.Instant", true),
Preconditions.or(
Preconditions.and(
new UsesType<>("java.time.ZoneId", true),
new UsesMethod<>("java.time.ZonedDateTime toLocalDate(..)"),
new UsesMethod<>("java.time.Instant atZone(..)")
),
Preconditions.and(
new UsesType<>("java.time.LocalDateTime", true),
new UsesType<>("java.time.ZoneId", true),
new UsesMethod<>("java.time.LocalDateTime toLocalDate(..)"),
new UsesMethod<>("java.time.LocalDateTime ofInstant(..)")
),
Preconditions.and(
new UsesType<>("java.time.OffsetDateTime", true),
new UsesType<>("java.time.ZoneId", true),
new UsesMethod<>("java.time.OffsetDateTime toLocalDate(..)"),
new UsesMethod<>("java.time.OffsetDateTime ofInstant(..)")
),
Preconditions.and(
new UsesType<>("java.time.ZoneOffset", true),
new UsesMethod<>("java.time.OffsetDateTime toLocalDate(..)"),
new UsesMethod<>("java.time.Instant atOffset(..)")
)
)
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.LocalDateTimeOfInstant}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class LocalDateTimeOfInstantRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public LocalDateTimeOfInstantRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `LocalDateTime#ofInstant(Instant, ZoneId)` over more indirect alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class LocalDateTimeOfInstant {\n \n @BeforeTemplate\n LocalDateTime before(Instant instant, ZoneId zoneId) {\n return Refaster.anyOf(instant.atZone(zoneId).toLocalDateTime(), OffsetDateTime.ofInstant(instant, zoneId).toLocalDateTime());\n }\n \n @BeforeTemplate\n LocalDateTime before(Instant instant, ZoneOffset zoneId) {\n return instant.atOffset(zoneId).toLocalDateTime();\n }\n \n @AfterTemplate\n LocalDateTime after(Instant instant, ZoneId zoneId) {\n return LocalDateTime.ofInstant(instant, zoneId);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before$0 = JavaTemplate
.builder("#{instant:any(java.time.Instant)}.atZone(#{zoneId:any(java.time.ZoneId)}).toLocalDateTime()")
.build();
final JavaTemplate before$1 = JavaTemplate
.builder("java.time.OffsetDateTime.ofInstant(#{instant:any(java.time.Instant)}, #{zoneId:any(java.time.ZoneId)}).toLocalDateTime()")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{instant:any(java.time.Instant)}.atOffset(#{zoneId:any(java.time.ZoneOffset)}).toLocalDateTime()")
.build();
final JavaTemplate after = JavaTemplate
.builder("java.time.LocalDateTime.ofInstant(#{instant:any(java.time.Instant)}, #{zoneId:any(java.time.ZoneId)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before$0.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before$1.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.OffsetDateTime");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.ZoneOffset");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.LocalDateTime", true),
new UsesType<>("java.time.Instant", true),
Preconditions.or(
Preconditions.and(
new UsesType<>("java.time.ZoneId", true),
new UsesMethod<>("java.time.ZonedDateTime toLocalDateTime(..)"),
new UsesMethod<>("java.time.Instant atZone(..)")
),
Preconditions.and(
new UsesType<>("java.time.OffsetDateTime", true),
new UsesType<>("java.time.ZoneId", true),
new UsesMethod<>("java.time.OffsetDateTime toLocalDateTime(..)"),
new UsesMethod<>("java.time.OffsetDateTime ofInstant(..)")
),
Preconditions.and(
new UsesType<>("java.time.ZoneOffset", true),
new UsesMethod<>("java.time.OffsetDateTime toLocalDateTime(..)"),
new UsesMethod<>("java.time.Instant atOffset(..)")
)
)
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.LocalTimeOfInstant}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class LocalTimeOfInstantRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public LocalTimeOfInstantRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `LocalTime#ofInstant(Instant, ZoneId)` over more indirect alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class LocalTimeOfInstant {\n \n @BeforeTemplate\n LocalTime before(Instant instant, ZoneId zoneId) {\n return Refaster.anyOf(instant.atZone(zoneId).toLocalTime(), LocalDateTime.ofInstant(instant, zoneId).toLocalTime(), OffsetDateTime.ofInstant(instant, zoneId).toLocalTime(), OffsetTime.ofInstant(instant, zoneId).toLocalTime());\n }\n \n @BeforeTemplate\n LocalTime before(Instant instant, ZoneOffset zoneId) {\n return instant.atOffset(zoneId).toLocalTime();\n }\n \n @AfterTemplate\n LocalTime after(Instant instant, ZoneId zoneId) {\n return LocalTime.ofInstant(instant, zoneId);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before$0 = JavaTemplate
.builder("#{instant:any(java.time.Instant)}.atZone(#{zoneId:any(java.time.ZoneId)}).toLocalTime()")
.build();
final JavaTemplate before$1 = JavaTemplate
.builder("java.time.LocalDateTime.ofInstant(#{instant:any(java.time.Instant)}, #{zoneId:any(java.time.ZoneId)}).toLocalTime()")
.build();
final JavaTemplate before$2 = JavaTemplate
.builder("java.time.OffsetDateTime.ofInstant(#{instant:any(java.time.Instant)}, #{zoneId:any(java.time.ZoneId)}).toLocalTime()")
.build();
final JavaTemplate before$3 = JavaTemplate
.builder("java.time.OffsetTime.ofInstant(#{instant:any(java.time.Instant)}, #{zoneId:any(java.time.ZoneId)}).toLocalTime()")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{instant:any(java.time.Instant)}.atOffset(#{zoneId:any(java.time.ZoneOffset)}).toLocalTime()")
.build();
final JavaTemplate after = JavaTemplate
.builder("java.time.LocalTime.ofInstant(#{instant:any(java.time.Instant)}, #{zoneId:any(java.time.ZoneId)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before$0.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before$1.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.LocalDateTime");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before$2.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.OffsetDateTime");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before$3.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.OffsetTime");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.ZoneOffset");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.LocalTime", true),
new UsesType<>("java.time.Instant", true),
Preconditions.or(
Preconditions.and(
new UsesType<>("java.time.ZoneId", true),
new UsesMethod<>("java.time.ZonedDateTime toLocalTime(..)"),
new UsesMethod<>("java.time.Instant atZone(..)")
),
Preconditions.and(
new UsesType<>("java.time.LocalDateTime", true),
new UsesType<>("java.time.ZoneId", true),
new UsesMethod<>("java.time.LocalDateTime toLocalTime(..)"),
new UsesMethod<>("java.time.LocalDateTime ofInstant(..)")
),
Preconditions.and(
new UsesType<>("java.time.OffsetDateTime", true),
new UsesType<>("java.time.ZoneId", true),
new UsesMethod<>("java.time.OffsetDateTime toLocalTime(..)"),
new UsesMethod<>("java.time.OffsetDateTime ofInstant(..)")
),
Preconditions.and(
new UsesType<>("java.time.OffsetTime", true),
new UsesType<>("java.time.ZoneId", true),
new UsesMethod<>("java.time.OffsetTime toLocalTime(..)"),
new UsesMethod<>("java.time.OffsetTime ofInstant(..)")
),
Preconditions.and(
new UsesType<>("java.time.ZoneOffset", true),
new UsesMethod<>("java.time.OffsetDateTime toLocalTime(..)"),
new UsesMethod<>("java.time.Instant atOffset(..)")
)
)
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.OffsetDateTimeOfInstant}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class OffsetDateTimeOfInstantRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public OffsetDateTimeOfInstantRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `OffsetDateTime#ofInstant(Instant, ZoneId)` over more indirect alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class OffsetDateTimeOfInstant {\n \n @BeforeTemplate\n OffsetDateTime before(Instant instant, ZoneId zoneId) {\n return instant.atZone(zoneId).toOffsetDateTime();\n }\n \n @AfterTemplate\n OffsetDateTime after(Instant instant, ZoneId zoneId) {\n return OffsetDateTime.ofInstant(instant, zoneId);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{instant:any(java.time.Instant)}.atZone(#{zoneId:any(java.time.ZoneId)}).toOffsetDateTime()")
.build();
final JavaTemplate after = JavaTemplate
.builder("java.time.OffsetDateTime.ofInstant(#{instant:any(java.time.Instant)}, #{zoneId:any(java.time.ZoneId)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.OffsetDateTime", true),
new UsesType<>("java.time.Instant", true),
new UsesType<>("java.time.ZoneId", true),
new UsesMethod<>("java.time.ZonedDateTime toOffsetDateTime(..)"),
new UsesMethod<>("java.time.Instant atZone(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.InstantAtOffset}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class InstantAtOffsetRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public InstantAtOffsetRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `Instant#atOffset(ZoneOffset)` over more verbose alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class InstantAtOffset {\n \n @BeforeTemplate\n OffsetDateTime before(Instant instant, ZoneOffset zoneOffset) {\n return OffsetDateTime.ofInstant(instant, zoneOffset);\n }\n \n @AfterTemplate\n OffsetDateTime after(Instant instant, ZoneOffset zoneOffset) {\n return instant.atOffset(zoneOffset);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("java.time.OffsetDateTime.ofInstant(#{instant:any(java.time.Instant)}, #{zoneOffset:any(java.time.ZoneOffset)})")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{instant:any(java.time.Instant)}.atOffset(#{zoneOffset:any(java.time.ZoneOffset)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.OffsetDateTime", true),
new UsesType<>("java.time.Instant", true),
new UsesType<>("java.time.ZoneOffset", true),
new UsesMethod<>("java.time.OffsetDateTime ofInstant(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.OffsetTimeOfInstant}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class OffsetTimeOfInstantRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public OffsetTimeOfInstantRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `OffsetTime#ofInstant(Instant, ZoneId)` over more indirect alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class OffsetTimeOfInstant {\n \n @BeforeTemplate\n OffsetTime before(Instant instant, ZoneId zoneId) {\n return OffsetDateTime.ofInstant(instant, zoneId).toOffsetTime();\n }\n \n @BeforeTemplate\n OffsetTime before(Instant instant, ZoneOffset zoneId) {\n return instant.atOffset(zoneId).toOffsetTime();\n }\n \n @AfterTemplate\n OffsetTime after(Instant instant, ZoneId zoneId) {\n return OffsetTime.ofInstant(instant, zoneId);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("java.time.OffsetDateTime.ofInstant(#{instant:any(java.time.Instant)}, #{zoneId:any(java.time.ZoneId)}).toOffsetTime()")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{instant:any(java.time.Instant)}.atOffset(#{zoneId:any(java.time.ZoneOffset)}).toOffsetTime()")
.build();
final JavaTemplate after = JavaTemplate
.builder("java.time.OffsetTime.ofInstant(#{instant:any(java.time.Instant)}, #{zoneId:any(java.time.ZoneId)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.OffsetDateTime");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.ZoneOffset");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.OffsetTime", true),
new UsesType<>("java.time.Instant", true),
new UsesType<>("java.time.ZoneOffset", true),
new UsesMethod<>("java.time.OffsetDateTime toOffsetTime(..)"),
new UsesMethod<>("java.time.Instant atOffset(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.InstantAtZone}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class InstantAtZoneRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public InstantAtZoneRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `Instant#atZone(ZoneId)` over more verbose alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class InstantAtZone {\n \n @BeforeTemplate\n ZonedDateTime before(Instant instant, ZoneId zoneId) {\n return ZonedDateTime.ofInstant(instant, zoneId);\n }\n \n @AfterTemplate\n ZonedDateTime after(Instant instant, ZoneId zoneId) {\n return instant.atZone(zoneId);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("java.time.ZonedDateTime.ofInstant(#{instant:any(java.time.Instant)}, #{zoneId:any(java.time.ZoneId)})")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{instant:any(java.time.Instant)}.atZone(#{zoneId:any(java.time.ZoneId)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.ZonedDateTime", true),
new UsesType<>("java.time.Instant", true),
new UsesType<>("java.time.ZoneId", true),
new UsesMethod<>("java.time.ZonedDateTime ofInstant(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.UtcClock}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class UtcClockRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public UtcClockRecipe() {}
@Override
public String getDisplayName() {
return "Use `Clock#systemUTC()` when possible";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class UtcClock {\n \n @BeforeTemplate\n @SuppressWarnings(value = \"TimeZoneUsage\")\n Clock before() {\n return Clock.system(UTC);\n }\n \n @AfterTemplate\n @SuppressWarnings(value = \"TimeZoneUsage\")\n Clock after() {\n return Clock.systemUTC();\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("java.time.Clock.system(java.time.ZoneOffset.UTC)")
.build();
final JavaTemplate after = JavaTemplate
.builder("java.time.Clock.systemUTC()")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.ZoneOffset.UTC");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace()),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.Clock", true),
new UsesMethod<>("java.time.Clock system(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.EpochInstant}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class EpochInstantRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public EpochInstantRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `Instant#EPOCH` over alternative representations";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class EpochInstant {\n \n @BeforeTemplate\n Instant before() {\n return Refaster.anyOf(Instant.ofEpochMilli(0), Instant.ofEpochSecond(0), Instant.ofEpochSecond(0, 0));\n }\n \n @AfterTemplate\n Instant after() {\n return Instant.EPOCH;\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before$0 = JavaTemplate
.builder("java.time.Instant.ofEpochMilli(0)")
.build();
final JavaTemplate before$1 = JavaTemplate
.builder("java.time.Instant.ofEpochSecond(0)")
.build();
final JavaTemplate before$2 = JavaTemplate
.builder("java.time.Instant.ofEpochSecond(0, 0)")
.build();
final JavaTemplate after = JavaTemplate
.builder("java.time.Instant.EPOCH")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before$0.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace()),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before$1.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace()),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before$2.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace()),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.Instant", true),
Preconditions.or(
new UsesMethod<>("java.time.Instant ofEpochMilli(..)"),
new UsesMethod<>("java.time.Instant ofEpochSecond(..)"),
new UsesMethod<>("java.time.Instant ofEpochSecond(..)")
)
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.InstantIsBefore}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class InstantIsBeforeRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public InstantIsBeforeRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `Instant#isBefore(Instant)` over explicit comparison, as it yields more readable code";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class InstantIsBefore {\n \n @BeforeTemplate\n boolean before(Instant a, Instant b) {\n return a.compareTo(b) < 0;\n }\n \n @AfterTemplate\n @AlsoNegation\n boolean after(Instant a, Instant b) {\n return a.isBefore(b);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{a:any(java.time.Instant)}.compareTo(#{b:any(java.time.Instant)}) < 0")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{a:any(java.time.Instant)}.isBefore(#{b:any(java.time.Instant)})")
.build();
@Override
public J visitBinary(J.Binary elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES, SIMPLIFY_BOOLEANS
);
}
return super.visitBinary(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.Instant", true),
new UsesMethod<>("java.time.Instant compareTo(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.InstantIsAfter}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class InstantIsAfterRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public InstantIsAfterRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `Instant#isBefore(Instant)` over explicit comparison, as it yields more readable code";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class InstantIsAfter {\n \n @BeforeTemplate\n boolean before(Instant a, Instant b) {\n return a.compareTo(b) > 0;\n }\n \n @AfterTemplate\n @AlsoNegation\n boolean after(Instant a, Instant b) {\n return a.isAfter(b);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{a:any(java.time.Instant)}.compareTo(#{b:any(java.time.Instant)}) > 0")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{a:any(java.time.Instant)}.isAfter(#{b:any(java.time.Instant)})")
.build();
@Override
public J visitBinary(J.Binary elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES, SIMPLIFY_BOOLEANS
);
}
return super.visitBinary(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.Instant", true),
new UsesMethod<>("java.time.Instant compareTo(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.LocalTimeMin}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class LocalTimeMinRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public LocalTimeMinRecipe() {}
@Override
public String getDisplayName() {
return "Prefer the `LocalTime#MIN` over alternative representations";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class LocalTimeMin {\n \n @BeforeTemplate\n LocalTime before() {\n return Refaster.anyOf(LocalTime.MIDNIGHT, LocalTime.of(0, 0), LocalTime.of(0, 0, 0), LocalTime.of(0, 0, 0, 0), LocalTime.ofNanoOfDay(0), LocalTime.ofSecondOfDay(0));\n }\n \n @AfterTemplate\n LocalTime after() {\n return LocalTime.MIN;\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before$0 = JavaTemplate
.builder("java.time.LocalTime.MIDNIGHT")
.build();
final JavaTemplate before$1 = JavaTemplate
.builder("java.time.LocalTime.of(0, 0)")
.build();
final JavaTemplate before$2 = JavaTemplate
.builder("java.time.LocalTime.of(0, 0, 0)")
.build();
final JavaTemplate before$3 = JavaTemplate
.builder("java.time.LocalTime.of(0, 0, 0, 0)")
.build();
final JavaTemplate before$4 = JavaTemplate
.builder("java.time.LocalTime.ofNanoOfDay(0)")
.build();
final JavaTemplate before$5 = JavaTemplate
.builder("java.time.LocalTime.ofSecondOfDay(0)")
.build();
final JavaTemplate after = JavaTemplate
.builder("java.time.LocalTime.MIN")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before$0.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace()),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before$1.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace()),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before$2.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace()),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before$3.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace()),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before$4.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace()),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before$5.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace()),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.LocalTime", true),
Preconditions.or(
new UsesMethod<>("java.time.LocalTime of(..)"),
new UsesMethod<>("java.time.LocalTime of(..)"),
new UsesMethod<>("java.time.LocalTime of(..)"),
new UsesMethod<>("java.time.LocalTime ofNanoOfDay(..)"),
new UsesMethod<>("java.time.LocalTime ofSecondOfDay(..)")
)
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.LocalDateAtStartOfDay}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class LocalDateAtStartOfDayRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public LocalDateAtStartOfDayRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `LocalDate#atStartOfDay()` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class LocalDateAtStartOfDay {\n \n @BeforeTemplate\n LocalDateTime before(LocalDate localDate) {\n return localDate.atTime(LocalTime.MIN);\n }\n \n @AfterTemplate\n LocalDateTime after(LocalDate localDate) {\n return localDate.atStartOfDay();\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{localDate:any(java.time.LocalDate)}.atTime(java.time.LocalTime.MIN)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{localDate:any(java.time.LocalDate)}.atStartOfDay()")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.LocalTime");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.LocalTime", true),
new UsesType<>("java.time.LocalDateTime", true),
new UsesType<>("java.time.LocalDate", true),
new UsesMethod<>("java.time.LocalDate atTime(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.ChronoLocalDateIsBefore}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class ChronoLocalDateIsBeforeRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public ChronoLocalDateIsBeforeRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `ChronoLocalDate#isBefore(ChronoLocalDate)` over explicit comparison, as it yields more readable code";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class ChronoLocalDateIsBefore {\n \n @BeforeTemplate\n boolean before(ChronoLocalDate a, ChronoLocalDate b) {\n return a.compareTo(b) < 0;\n }\n \n @AfterTemplate\n @AlsoNegation\n boolean after(ChronoLocalDate a, ChronoLocalDate b) {\n return a.isBefore(b);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{a:any(java.time.chrono.ChronoLocalDate)}.compareTo(#{b:any(java.time.chrono.ChronoLocalDate)}) < 0")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{a:any(java.time.chrono.ChronoLocalDate)}.isBefore(#{b:any(java.time.chrono.ChronoLocalDate)})")
.build();
@Override
public J visitBinary(J.Binary elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES, SIMPLIFY_BOOLEANS
);
}
return super.visitBinary(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.chrono.ChronoLocalDate", true),
new UsesMethod<>("java.time.chrono.ChronoLocalDate compareTo(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.ChronoLocalDateIsAfter}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class ChronoLocalDateIsAfterRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public ChronoLocalDateIsAfterRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `ChronoLocalDate#isBefore(ChronoLocalDate)` over explicit comparison, as it yields more readable code";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class ChronoLocalDateIsAfter {\n \n @BeforeTemplate\n boolean before(ChronoLocalDate a, ChronoLocalDate b) {\n return a.compareTo(b) > 0;\n }\n \n @AfterTemplate\n @AlsoNegation\n boolean after(ChronoLocalDate a, ChronoLocalDate b) {\n return a.isAfter(b);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{a:any(java.time.chrono.ChronoLocalDate)}.compareTo(#{b:any(java.time.chrono.ChronoLocalDate)}) > 0")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{a:any(java.time.chrono.ChronoLocalDate)}.isAfter(#{b:any(java.time.chrono.ChronoLocalDate)})")
.build();
@Override
public J visitBinary(J.Binary elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES, SIMPLIFY_BOOLEANS
);
}
return super.visitBinary(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.chrono.ChronoLocalDate", true),
new UsesMethod<>("java.time.chrono.ChronoLocalDate compareTo(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.ChronoLocalDateTimeIsBefore}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class ChronoLocalDateTimeIsBeforeRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public ChronoLocalDateTimeIsBeforeRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `ChronoLocalDateTime#isBefore(ChronoLocalDateTime)` over explicit comparison, as it yields more readable code";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class ChronoLocalDateTimeIsBefore {\n \n @BeforeTemplate\n boolean before(ChronoLocalDateTime> a, ChronoLocalDateTime> b) {\n return a.compareTo(b) < 0;\n }\n \n @AfterTemplate\n @AlsoNegation\n boolean after(ChronoLocalDateTime> a, ChronoLocalDateTime> b) {\n return a.isBefore(b);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{a:any(java.time.chrono.ChronoLocalDateTime>)}.compareTo(#{b:any(java.time.chrono.ChronoLocalDateTime>)}) < 0")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{a:any(java.time.chrono.ChronoLocalDateTime>)}.isBefore(#{b:any(java.time.chrono.ChronoLocalDateTime>)})")
.build();
@Override
public J visitBinary(J.Binary elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES, SIMPLIFY_BOOLEANS
);
}
return super.visitBinary(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.chrono.ChronoLocalDateTime", true),
new UsesMethod<>("java.time.chrono.ChronoLocalDateTime compareTo(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.ChronoLocalDateTimeIsAfter}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class ChronoLocalDateTimeIsAfterRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public ChronoLocalDateTimeIsAfterRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `ChronoLocalDateTime#isBefore(ChronoLocalDateTime)` over explicit comparison, as it yields more readable code";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class ChronoLocalDateTimeIsAfter {\n \n @BeforeTemplate\n boolean before(ChronoLocalDateTime> a, ChronoLocalDateTime> b) {\n return a.compareTo(b) > 0;\n }\n \n @AfterTemplate\n @AlsoNegation\n boolean after(ChronoLocalDateTime> a, ChronoLocalDateTime> b) {\n return a.isAfter(b);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{a:any(java.time.chrono.ChronoLocalDateTime>)}.compareTo(#{b:any(java.time.chrono.ChronoLocalDateTime>)}) > 0")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{a:any(java.time.chrono.ChronoLocalDateTime>)}.isAfter(#{b:any(java.time.chrono.ChronoLocalDateTime>)})")
.build();
@Override
public J visitBinary(J.Binary elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES, SIMPLIFY_BOOLEANS
);
}
return super.visitBinary(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.chrono.ChronoLocalDateTime", true),
new UsesMethod<>("java.time.chrono.ChronoLocalDateTime compareTo(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.ChronoZonedDateTimeIsBefore}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class ChronoZonedDateTimeIsBeforeRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public ChronoZonedDateTimeIsBeforeRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `ChronoZonedDateTime#isBefore(ChronoZonedDateTime)` over explicit comparison, as it yields more readable code";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class ChronoZonedDateTimeIsBefore {\n \n @BeforeTemplate\n boolean before(ChronoZonedDateTime> a, ChronoZonedDateTime> b) {\n return a.compareTo(b) < 0;\n }\n \n @AfterTemplate\n @AlsoNegation\n boolean after(ChronoZonedDateTime> a, ChronoZonedDateTime> b) {\n return a.isBefore(b);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{a:any(java.time.chrono.ChronoZonedDateTime>)}.compareTo(#{b:any(java.time.chrono.ChronoZonedDateTime>)}) < 0")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{a:any(java.time.chrono.ChronoZonedDateTime>)}.isBefore(#{b:any(java.time.chrono.ChronoZonedDateTime>)})")
.build();
@Override
public J visitBinary(J.Binary elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES, SIMPLIFY_BOOLEANS
);
}
return super.visitBinary(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.chrono.ChronoZonedDateTime", true),
new UsesMethod<>("java.time.chrono.ChronoZonedDateTime compareTo(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.ChronoZonedDateTimeIsAfter}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class ChronoZonedDateTimeIsAfterRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public ChronoZonedDateTimeIsAfterRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `ChronoZonedDateTime#isBefore(ChronoZonedDateTime)` over explicit comparison, as it yields more readable code";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class ChronoZonedDateTimeIsAfter {\n \n @BeforeTemplate\n boolean before(ChronoZonedDateTime> a, ChronoZonedDateTime> b) {\n return a.compareTo(b) > 0;\n }\n \n @AfterTemplate\n @AlsoNegation\n boolean after(ChronoZonedDateTime> a, ChronoZonedDateTime> b) {\n return a.isAfter(b);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{a:any(java.time.chrono.ChronoZonedDateTime>)}.compareTo(#{b:any(java.time.chrono.ChronoZonedDateTime>)}) > 0")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{a:any(java.time.chrono.ChronoZonedDateTime>)}.isAfter(#{b:any(java.time.chrono.ChronoZonedDateTime>)})")
.build();
@Override
public J visitBinary(J.Binary elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES, SIMPLIFY_BOOLEANS
);
}
return super.visitBinary(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.chrono.ChronoZonedDateTime", true),
new UsesMethod<>("java.time.chrono.ChronoZonedDateTime compareTo(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.OffsetDateTimeIsBefore}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class OffsetDateTimeIsBeforeRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public OffsetDateTimeIsBeforeRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `OffsetDateTime#isBefore(OffsetDateTime)` over explicit comparison, as it yields more readable code";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class OffsetDateTimeIsBefore {\n \n @BeforeTemplate\n boolean before(OffsetDateTime a, OffsetDateTime b) {\n return a.compareTo(b) < 0;\n }\n \n @AfterTemplate\n @AlsoNegation\n boolean after(OffsetDateTime a, OffsetDateTime b) {\n return a.isBefore(b);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{a:any(java.time.OffsetDateTime)}.compareTo(#{b:any(java.time.OffsetDateTime)}) < 0")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{a:any(java.time.OffsetDateTime)}.isBefore(#{b:any(java.time.OffsetDateTime)})")
.build();
@Override
public J visitBinary(J.Binary elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES, SIMPLIFY_BOOLEANS
);
}
return super.visitBinary(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.OffsetDateTime", true),
new UsesMethod<>("java.time.OffsetDateTime compareTo(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.OffsetDateTimeIsAfter}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class OffsetDateTimeIsAfterRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public OffsetDateTimeIsAfterRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `OffsetDateTime#isBefore(OffsetDateTime)` over explicit comparison, as it yields more readable code";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class OffsetDateTimeIsAfter {\n \n @BeforeTemplate\n boolean before(OffsetDateTime a, OffsetDateTime b) {\n return a.compareTo(b) > 0;\n }\n \n @AfterTemplate\n @AlsoNegation\n boolean after(OffsetDateTime a, OffsetDateTime b) {\n return a.isAfter(b);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{a:any(java.time.OffsetDateTime)}.compareTo(#{b:any(java.time.OffsetDateTime)}) > 0")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{a:any(java.time.OffsetDateTime)}.isAfter(#{b:any(java.time.OffsetDateTime)})")
.build();
@Override
public J visitBinary(J.Binary elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES, SIMPLIFY_BOOLEANS
);
}
return super.visitBinary(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.OffsetDateTime", true),
new UsesMethod<>("java.time.OffsetDateTime compareTo(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.ZeroDuration}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class ZeroDurationRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public ZeroDurationRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `TimeRules.ZeroDuration`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class ZeroDuration {\n \n @BeforeTemplate\n Duration before(TemporalUnit temporalUnit) {\n return Refaster.anyOf(Duration.ofNanos(0), Duration.ofMillis(0), Duration.ofSeconds(0), Duration.ofSeconds(0, 0), Duration.ofMinutes(0), Duration.ofHours(0), Duration.ofDays(0), Duration.of(0, temporalUnit));\n }\n \n @AfterTemplate\n Duration after() {\n return Duration.ZERO;\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before$0 = JavaTemplate
.builder("java.time.Duration.ofNanos(0)")
.build();
final JavaTemplate before$1 = JavaTemplate
.builder("java.time.Duration.ofMillis(0)")
.build();
final JavaTemplate before$2 = JavaTemplate
.builder("java.time.Duration.ofSeconds(0)")
.build();
final JavaTemplate before$3 = JavaTemplate
.builder("java.time.Duration.ofSeconds(0, 0)")
.build();
final JavaTemplate before$4 = JavaTemplate
.builder("java.time.Duration.ofMinutes(0)")
.build();
final JavaTemplate before$5 = JavaTemplate
.builder("java.time.Duration.ofHours(0)")
.build();
final JavaTemplate before$6 = JavaTemplate
.builder("java.time.Duration.ofDays(0)")
.build();
final JavaTemplate before$7 = JavaTemplate
.builder("java.time.Duration.of(0, #{temporalUnit:any(java.time.temporal.TemporalUnit)})")
.build();
final JavaTemplate after = JavaTemplate
.builder("java.time.Duration.ZERO")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before$0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.TemporalUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace()),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before$1.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.TemporalUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace()),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before$2.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.TemporalUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace()),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before$3.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.TemporalUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace()),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before$4.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.TemporalUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace()),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before$5.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.TemporalUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace()),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before$6.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.TemporalUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace()),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before$7.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.TemporalUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace()),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.Duration", true),
new UsesType<>("java.time.temporal.TemporalUnit", true),
Preconditions.or(
new UsesMethod<>("java.time.Duration ofNanos(..)"),
new UsesMethod<>("java.time.Duration ofMillis(..)"),
new UsesMethod<>("java.time.Duration ofSeconds(..)"),
new UsesMethod<>("java.time.Duration ofSeconds(..)"),
new UsesMethod<>("java.time.Duration ofMinutes(..)"),
new UsesMethod<>("java.time.Duration ofHours(..)"),
new UsesMethod<>("java.time.Duration ofDays(..)"),
new UsesMethod<>("java.time.Duration of(..)")
)
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.DurationOfDays}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class DurationOfDaysRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public DurationOfDaysRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `Duration#ofDays(long)` over alternative representations";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class DurationOfDays {\n \n @BeforeTemplate\n Duration before(long amount) {\n return Duration.of(amount, ChronoUnit.DAYS);\n }\n \n @AfterTemplate\n Duration after(long amount) {\n return Duration.ofDays(amount);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("java.time.Duration.of(#{amount:any(long)}, java.time.temporal.ChronoUnit.DAYS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("java.time.Duration.ofDays(#{amount:any(long)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.Duration", true),
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesMethod<>("java.time.Duration of(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.DurationOfHours}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class DurationOfHoursRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public DurationOfHoursRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `Duration#ofHours(long)` over alternative representations";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class DurationOfHours {\n \n @BeforeTemplate\n Duration before(long amount) {\n return Duration.of(amount, ChronoUnit.HOURS);\n }\n \n @AfterTemplate\n Duration after(long amount) {\n return Duration.ofHours(amount);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("java.time.Duration.of(#{amount:any(long)}, java.time.temporal.ChronoUnit.HOURS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("java.time.Duration.ofHours(#{amount:any(long)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.Duration", true),
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesMethod<>("java.time.Duration of(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.DurationOfMillis}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class DurationOfMillisRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public DurationOfMillisRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `Duration#ofMillis(long)` over alternative representations";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class DurationOfMillis {\n \n @BeforeTemplate\n Duration before(long amount) {\n return Duration.of(amount, ChronoUnit.MILLIS);\n }\n \n @AfterTemplate\n Duration after(long amount) {\n return Duration.ofMillis(amount);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("java.time.Duration.of(#{amount:any(long)}, java.time.temporal.ChronoUnit.MILLIS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("java.time.Duration.ofMillis(#{amount:any(long)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.Duration", true),
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesMethod<>("java.time.Duration of(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.DurationOfMinutes}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class DurationOfMinutesRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public DurationOfMinutesRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `Duration#ofMinutes(long)` over alternative representations";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class DurationOfMinutes {\n \n @BeforeTemplate\n Duration before(long amount) {\n return Duration.of(amount, ChronoUnit.MINUTES);\n }\n \n @AfterTemplate\n Duration after(long amount) {\n return Duration.ofMinutes(amount);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("java.time.Duration.of(#{amount:any(long)}, java.time.temporal.ChronoUnit.MINUTES)")
.build();
final JavaTemplate after = JavaTemplate
.builder("java.time.Duration.ofMinutes(#{amount:any(long)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.Duration", true),
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesMethod<>("java.time.Duration of(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.DurationOfNanos}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class DurationOfNanosRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public DurationOfNanosRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `Duration#ofNanos(long)` over alternative representations";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class DurationOfNanos {\n \n @BeforeTemplate\n Duration before(long amount) {\n return Duration.of(amount, ChronoUnit.NANOS);\n }\n \n @AfterTemplate\n Duration after(long amount) {\n return Duration.ofNanos(amount);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("java.time.Duration.of(#{amount:any(long)}, java.time.temporal.ChronoUnit.NANOS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("java.time.Duration.ofNanos(#{amount:any(long)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.Duration", true),
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesMethod<>("java.time.Duration of(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.DurationOfSeconds}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class DurationOfSecondsRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public DurationOfSecondsRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `Duration#ofSeconds(long)` over alternative representations";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class DurationOfSeconds {\n \n @BeforeTemplate\n Duration before(long amount) {\n return Duration.of(amount, ChronoUnit.SECONDS);\n }\n \n @AfterTemplate\n Duration after(long amount) {\n return Duration.ofSeconds(amount);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("java.time.Duration.of(#{amount:any(long)}, java.time.temporal.ChronoUnit.SECONDS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("java.time.Duration.ofSeconds(#{amount:any(long)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.Duration", true),
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesMethod<>("java.time.Duration of(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.DurationBetweenInstants}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class DurationBetweenInstantsRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public DurationBetweenInstantsRecipe() {}
@Override
public String getDisplayName() {
return "Don't unnecessarily convert to and from milliseconds. (This way nanosecond precision is retained.) Warning: this rewrite rule increases precision!";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class DurationBetweenInstants {\n \n @BeforeTemplate\n Duration before(Instant a, Instant b) {\n return Duration.ofMillis(b.toEpochMilli() - a.toEpochMilli());\n }\n \n @AfterTemplate\n Duration after(Instant a, Instant b) {\n return Duration.between(a, b);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("java.time.Duration.ofMillis(#{b:any(java.time.Instant)}.toEpochMilli() - #{a:any(java.time.Instant)}.toEpochMilli())")
.build();
final JavaTemplate after = JavaTemplate
.builder("java.time.Duration.between(#{a:any(java.time.Instant)}, #{b:any(java.time.Instant)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(1), matcher.parameter(0)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.Duration", true),
new UsesType<>("java.time.Instant", true),
new UsesMethod<>("java.time.Duration ofMillis(..)"),
new UsesMethod<>("java.time.Instant toEpochMilli(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.DurationBetweenOffsetDateTimes}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class DurationBetweenOffsetDateTimesRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public DurationBetweenOffsetDateTimesRecipe() {}
@Override
public String getDisplayName() {
return "Don't unnecessarily convert to and from milliseconds. (This way nanosecond precision is retained.) Warning: this rewrite rule increases precision!";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class DurationBetweenOffsetDateTimes {\n \n @BeforeTemplate\n Duration before(OffsetDateTime a, OffsetDateTime b) {\n return Refaster.anyOf(Duration.between(a.toInstant(), b.toInstant()), Duration.ofSeconds(b.toEpochSecond() - a.toEpochSecond()));\n }\n \n @AfterTemplate\n Duration after(OffsetDateTime a, OffsetDateTime b) {\n return Duration.between(a, b);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before$0 = JavaTemplate
.builder("java.time.Duration.between(#{a:any(java.time.OffsetDateTime)}.toInstant(), #{b:any(java.time.OffsetDateTime)}.toInstant())")
.build();
final JavaTemplate before$1 = JavaTemplate
.builder("java.time.Duration.ofSeconds(#{b:any(java.time.OffsetDateTime)}.toEpochSecond() - #{a:any(java.time.OffsetDateTime)}.toEpochSecond())")
.build();
final JavaTemplate after = JavaTemplate
.builder("java.time.Duration.between(#{a:any(java.time.OffsetDateTime)}, #{b:any(java.time.OffsetDateTime)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before$0.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before$1.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.Duration", true),
new UsesType<>("java.time.OffsetDateTime", true),
Preconditions.or(
Preconditions.and(
new UsesMethod<>("java.time.Duration between(..)"),
new UsesMethod<>("java.time.OffsetDateTime toInstant(..)")
),
Preconditions.and(
new UsesMethod<>("java.time.Duration ofSeconds(..)"),
new UsesMethod<>("java.time.OffsetDateTime toEpochSecond(..)")
)
)
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.DurationIsZero}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class DurationIsZeroRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public DurationIsZeroRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `Duration#isZero()` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class DurationIsZero {\n \n @BeforeTemplate\n boolean before(Duration duration) {\n return Refaster.anyOf(duration.equals(Duration.ZERO), Duration.ZERO.equals(duration));\n }\n \n @AfterTemplate\n boolean after(Duration duration) {\n return duration.isZero();\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before$0 = JavaTemplate
.builder("#{duration:any(java.time.Duration)}.equals(java.time.Duration.ZERO)")
.build();
final JavaTemplate before$1 = JavaTemplate
.builder("java.time.Duration.ZERO.equals(#{duration:any(java.time.Duration)})")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{duration:any(java.time.Duration)}.isZero()")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before$0.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0)),
getCursor(),
ctx,
SHORTEN_NAMES, SIMPLIFY_BOOLEANS
);
}
if ((matcher = before$1.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0)),
getCursor(),
ctx,
SHORTEN_NAMES, SIMPLIFY_BOOLEANS
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.Duration", true),
new UsesMethod<>("java.time.Duration equals(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.ZeroPeriod}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class ZeroPeriodRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public ZeroPeriodRecipe() {}
@Override
public String getDisplayName() {
return "Refaster template `TimeRules.ZeroPeriod`";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class ZeroPeriod {\n \n @BeforeTemplate\n Period before() {\n return Refaster.anyOf(Period.ofDays(0), Period.ofWeeks(0), Period.ofMonths(0), Period.ofYears(0), Period.of(0, 0, 0));\n }\n \n @AfterTemplate\n Period after() {\n return Period.ZERO;\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before$0 = JavaTemplate
.builder("java.time.Period.ofDays(0)")
.build();
final JavaTemplate before$1 = JavaTemplate
.builder("java.time.Period.ofWeeks(0)")
.build();
final JavaTemplate before$2 = JavaTemplate
.builder("java.time.Period.ofMonths(0)")
.build();
final JavaTemplate before$3 = JavaTemplate
.builder("java.time.Period.ofYears(0)")
.build();
final JavaTemplate before$4 = JavaTemplate
.builder("java.time.Period.of(0, 0, 0)")
.build();
final JavaTemplate after = JavaTemplate
.builder("java.time.Period.ZERO")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before$0.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace()),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before$1.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace()),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before$2.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace()),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before$3.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace()),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before$4.matcher(getCursor())).find()) {
return embed(
after.apply(getCursor(), elem.getCoordinates().replace()),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.Period", true),
Preconditions.or(
new UsesMethod<>("java.time.Period ofDays(..)"),
new UsesMethod<>("java.time.Period ofWeeks(..)"),
new UsesMethod<>("java.time.Period ofMonths(..)"),
new UsesMethod<>("java.time.Period ofYears(..)"),
new UsesMethod<>("java.time.Period of(..)")
)
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.LocalDatePlusDays}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class LocalDatePlusDaysRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public LocalDatePlusDaysRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `LocalDate#plusDays(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class LocalDatePlusDays {\n \n @BeforeTemplate\n LocalDate before(LocalDate localDate, int days) {\n return localDate.plus(Period.ofDays(days));\n }\n \n @BeforeTemplate\n LocalDate before(LocalDate localDate, long days) {\n return localDate.plus(days, ChronoUnit.DAYS);\n }\n \n @AfterTemplate\n LocalDate after(LocalDate localDate, int days) {\n return localDate.plusDays(days);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{localDate:any(java.time.LocalDate)}.plus(java.time.Period.ofDays(#{days:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{localDate:any(java.time.LocalDate)}.plus(#{days:any(long)}, java.time.temporal.ChronoUnit.DAYS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{localDate:any(java.time.LocalDate)}.plusDays(#{days:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Period");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.LocalDate", true),
new UsesMethod<>("java.time.LocalDate plus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.LocalDatePlusWeeks}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class LocalDatePlusWeeksRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public LocalDatePlusWeeksRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `LocalDate#plusWeeks(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class LocalDatePlusWeeks {\n \n @BeforeTemplate\n LocalDate before(LocalDate localDate, int weeks) {\n return localDate.plus(Period.ofWeeks(weeks));\n }\n \n @BeforeTemplate\n LocalDate before(LocalDate localDate, long weeks) {\n return localDate.plus(weeks, ChronoUnit.WEEKS);\n }\n \n @AfterTemplate\n LocalDate after(LocalDate localDate, int weeks) {\n return localDate.plusWeeks(weeks);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{localDate:any(java.time.LocalDate)}.plus(java.time.Period.ofWeeks(#{weeks:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{localDate:any(java.time.LocalDate)}.plus(#{weeks:any(long)}, java.time.temporal.ChronoUnit.WEEKS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{localDate:any(java.time.LocalDate)}.plusWeeks(#{weeks:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Period");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.LocalDate", true),
new UsesMethod<>("java.time.LocalDate plus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.LocalDatePlusMonths}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class LocalDatePlusMonthsRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public LocalDatePlusMonthsRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `LocalDate#plusMonths(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class LocalDatePlusMonths {\n \n @BeforeTemplate\n LocalDate before(LocalDate localDate, int months) {\n return localDate.plus(Period.ofMonths(months));\n }\n \n @BeforeTemplate\n LocalDate before(LocalDate localDate, long months) {\n return localDate.plus(months, ChronoUnit.MONTHS);\n }\n \n @AfterTemplate\n LocalDate after(LocalDate localDate, int months) {\n return localDate.plusMonths(months);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{localDate:any(java.time.LocalDate)}.plus(java.time.Period.ofMonths(#{months:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{localDate:any(java.time.LocalDate)}.plus(#{months:any(long)}, java.time.temporal.ChronoUnit.MONTHS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{localDate:any(java.time.LocalDate)}.plusMonths(#{months:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Period");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.LocalDate", true),
new UsesMethod<>("java.time.LocalDate plus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.LocalDatePlusYears}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class LocalDatePlusYearsRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public LocalDatePlusYearsRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `LocalDate#plusYears(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class LocalDatePlusYears {\n \n @BeforeTemplate\n LocalDate before(LocalDate localDate, int years) {\n return localDate.plus(Period.ofYears(years));\n }\n \n @BeforeTemplate\n LocalDate before(LocalDate localDate, long years) {\n return localDate.plus(years, ChronoUnit.YEARS);\n }\n \n @AfterTemplate\n LocalDate after(LocalDate localDate, int years) {\n return localDate.plusYears(years);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{localDate:any(java.time.LocalDate)}.plus(java.time.Period.ofYears(#{years:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{localDate:any(java.time.LocalDate)}.plus(#{years:any(long)}, java.time.temporal.ChronoUnit.YEARS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{localDate:any(java.time.LocalDate)}.plusYears(#{years:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Period");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.LocalDate", true),
new UsesMethod<>("java.time.LocalDate plus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.LocalDateMinusDays}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class LocalDateMinusDaysRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public LocalDateMinusDaysRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `LocalDate#minusDays(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class LocalDateMinusDays {\n \n @BeforeTemplate\n LocalDate before(LocalDate localDate, int days) {\n return localDate.minus(Period.ofDays(days));\n }\n \n @BeforeTemplate\n LocalDate before(LocalDate localDate, long days) {\n return localDate.minus(days, ChronoUnit.DAYS);\n }\n \n @AfterTemplate\n LocalDate after(LocalDate localDate, int days) {\n return localDate.minusDays(days);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{localDate:any(java.time.LocalDate)}.minus(java.time.Period.ofDays(#{days:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{localDate:any(java.time.LocalDate)}.minus(#{days:any(long)}, java.time.temporal.ChronoUnit.DAYS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{localDate:any(java.time.LocalDate)}.minusDays(#{days:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Period");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.LocalDate", true),
new UsesMethod<>("java.time.LocalDate minus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.LocalDateMinusWeeks}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class LocalDateMinusWeeksRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public LocalDateMinusWeeksRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `LocalDate#minusWeeks(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class LocalDateMinusWeeks {\n \n @BeforeTemplate\n LocalDate before(LocalDate localDate, int weeks) {\n return localDate.minus(Period.ofWeeks(weeks));\n }\n \n @BeforeTemplate\n LocalDate before(LocalDate localDate, long weeks) {\n return localDate.minus(weeks, ChronoUnit.WEEKS);\n }\n \n @AfterTemplate\n LocalDate after(LocalDate localDate, int weeks) {\n return localDate.minusWeeks(weeks);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{localDate:any(java.time.LocalDate)}.minus(java.time.Period.ofWeeks(#{weeks:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{localDate:any(java.time.LocalDate)}.minus(#{weeks:any(long)}, java.time.temporal.ChronoUnit.WEEKS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{localDate:any(java.time.LocalDate)}.minusWeeks(#{weeks:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Period");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.LocalDate", true),
new UsesMethod<>("java.time.LocalDate minus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.LocalDateMinusMonths}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class LocalDateMinusMonthsRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public LocalDateMinusMonthsRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `LocalDate#minusMonths(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class LocalDateMinusMonths {\n \n @BeforeTemplate\n LocalDate before(LocalDate localDate, int months) {\n return localDate.minus(Period.ofMonths(months));\n }\n \n @BeforeTemplate\n LocalDate before(LocalDate localDate, long months) {\n return localDate.minus(months, ChronoUnit.MONTHS);\n }\n \n @AfterTemplate\n LocalDate after(LocalDate localDate, int months) {\n return localDate.minusMonths(months);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{localDate:any(java.time.LocalDate)}.minus(java.time.Period.ofMonths(#{months:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{localDate:any(java.time.LocalDate)}.minus(#{months:any(long)}, java.time.temporal.ChronoUnit.MONTHS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{localDate:any(java.time.LocalDate)}.minusMonths(#{months:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Period");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.LocalDate", true),
new UsesMethod<>("java.time.LocalDate minus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.LocalDateMinusYears}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class LocalDateMinusYearsRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public LocalDateMinusYearsRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `LocalDate#minusYears(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class LocalDateMinusYears {\n \n @BeforeTemplate\n LocalDate before(LocalDate localDate, int years) {\n return localDate.minus(Period.ofYears(years));\n }\n \n @BeforeTemplate\n LocalDate before(LocalDate localDate, long years) {\n return localDate.minus(years, ChronoUnit.YEARS);\n }\n \n @AfterTemplate\n LocalDate after(LocalDate localDate, int years) {\n return localDate.minusYears(years);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{localDate:any(java.time.LocalDate)}.minus(java.time.Period.ofYears(#{years:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{localDate:any(java.time.LocalDate)}.minus(#{years:any(long)}, java.time.temporal.ChronoUnit.YEARS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{localDate:any(java.time.LocalDate)}.minusYears(#{years:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Period");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.LocalDate", true),
new UsesMethod<>("java.time.LocalDate minus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.LocalTimePlusNanos}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class LocalTimePlusNanosRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public LocalTimePlusNanosRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `LocalTime#plusNanos(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class LocalTimePlusNanos {\n \n @BeforeTemplate\n LocalTime before(LocalTime localTime, int nanos) {\n return localTime.plus(Duration.ofNanos(nanos));\n }\n \n @BeforeTemplate\n LocalTime before(LocalTime localTime, long nanos) {\n return localTime.plus(nanos, ChronoUnit.NANOS);\n }\n \n @AfterTemplate\n LocalTime after(LocalTime localTime, int nanos) {\n return localTime.plusNanos(nanos);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{localTime:any(java.time.LocalTime)}.plus(java.time.Duration.ofNanos(#{nanos:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{localTime:any(java.time.LocalTime)}.plus(#{nanos:any(long)}, java.time.temporal.ChronoUnit.NANOS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{localTime:any(java.time.LocalTime)}.plusNanos(#{nanos:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.LocalTime", true),
new UsesMethod<>("java.time.LocalTime plus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.LocalTimePlusSeconds}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class LocalTimePlusSecondsRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public LocalTimePlusSecondsRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `LocalTime#plusSeconds(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class LocalTimePlusSeconds {\n \n @BeforeTemplate\n LocalTime before(LocalTime localTime, int seconds) {\n return localTime.plus(Duration.ofSeconds(seconds));\n }\n \n @BeforeTemplate\n LocalTime before(LocalTime localTime, long seconds) {\n return localTime.plus(seconds, ChronoUnit.SECONDS);\n }\n \n @AfterTemplate\n LocalTime after(LocalTime localTime, int seconds) {\n return localTime.plusSeconds(seconds);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{localTime:any(java.time.LocalTime)}.plus(java.time.Duration.ofSeconds(#{seconds:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{localTime:any(java.time.LocalTime)}.plus(#{seconds:any(long)}, java.time.temporal.ChronoUnit.SECONDS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{localTime:any(java.time.LocalTime)}.plusSeconds(#{seconds:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.LocalTime", true),
new UsesMethod<>("java.time.LocalTime plus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.LocalTimePlusMinutes}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class LocalTimePlusMinutesRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public LocalTimePlusMinutesRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `LocalTime#plusMinutes(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class LocalTimePlusMinutes {\n \n @BeforeTemplate\n LocalTime before(LocalTime localTime, int minutes) {\n return localTime.plus(Duration.ofMinutes(minutes));\n }\n \n @BeforeTemplate\n LocalTime before(LocalTime localTime, long minutes) {\n return localTime.plus(minutes, ChronoUnit.MINUTES);\n }\n \n @AfterTemplate\n LocalTime after(LocalTime localTime, int minutes) {\n return localTime.plusMinutes(minutes);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{localTime:any(java.time.LocalTime)}.plus(java.time.Duration.ofMinutes(#{minutes:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{localTime:any(java.time.LocalTime)}.plus(#{minutes:any(long)}, java.time.temporal.ChronoUnit.MINUTES)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{localTime:any(java.time.LocalTime)}.plusMinutes(#{minutes:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.LocalTime", true),
new UsesMethod<>("java.time.LocalTime plus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.LocalTimePlusHours}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class LocalTimePlusHoursRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public LocalTimePlusHoursRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `LocalTime#plusHours(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class LocalTimePlusHours {\n \n @BeforeTemplate\n LocalTime before(LocalTime localTime, int hours) {\n return localTime.plus(Duration.ofHours(hours));\n }\n \n @BeforeTemplate\n LocalTime before(LocalTime localTime, long hours) {\n return localTime.plus(hours, ChronoUnit.HOURS);\n }\n \n @AfterTemplate\n LocalTime after(LocalTime localTime, int hours) {\n return localTime.plusHours(hours);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{localTime:any(java.time.LocalTime)}.plus(java.time.Duration.ofHours(#{hours:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{localTime:any(java.time.LocalTime)}.plus(#{hours:any(long)}, java.time.temporal.ChronoUnit.HOURS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{localTime:any(java.time.LocalTime)}.plusHours(#{hours:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.LocalTime", true),
new UsesMethod<>("java.time.LocalTime plus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.LocalTimeMinusNanos}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class LocalTimeMinusNanosRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public LocalTimeMinusNanosRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `LocalTime#minusNanos(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class LocalTimeMinusNanos {\n \n @BeforeTemplate\n LocalTime before(LocalTime localTime, int nanos) {\n return localTime.minus(Duration.ofNanos(nanos));\n }\n \n @BeforeTemplate\n LocalTime before(LocalTime localTime, long nanos) {\n return localTime.minus(nanos, ChronoUnit.NANOS);\n }\n \n @AfterTemplate\n LocalTime after(LocalTime localTime, int nanos) {\n return localTime.minusNanos(nanos);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{localTime:any(java.time.LocalTime)}.minus(java.time.Duration.ofNanos(#{nanos:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{localTime:any(java.time.LocalTime)}.minus(#{nanos:any(long)}, java.time.temporal.ChronoUnit.NANOS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{localTime:any(java.time.LocalTime)}.minusNanos(#{nanos:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.LocalTime", true),
new UsesMethod<>("java.time.LocalTime minus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.LocalTimeMinusSeconds}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class LocalTimeMinusSecondsRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public LocalTimeMinusSecondsRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `LocalTime#minusSeconds(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class LocalTimeMinusSeconds {\n \n @BeforeTemplate\n LocalTime before(LocalTime localTime, int seconds) {\n return localTime.minus(Duration.ofSeconds(seconds));\n }\n \n @BeforeTemplate\n LocalTime before(LocalTime localTime, long seconds) {\n return localTime.minus(seconds, ChronoUnit.SECONDS);\n }\n \n @AfterTemplate\n LocalTime after(LocalTime localTime, int seconds) {\n return localTime.minusSeconds(seconds);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{localTime:any(java.time.LocalTime)}.minus(java.time.Duration.ofSeconds(#{seconds:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{localTime:any(java.time.LocalTime)}.minus(#{seconds:any(long)}, java.time.temporal.ChronoUnit.SECONDS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{localTime:any(java.time.LocalTime)}.minusSeconds(#{seconds:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.LocalTime", true),
new UsesMethod<>("java.time.LocalTime minus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.LocalTimeMinusMinutes}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class LocalTimeMinusMinutesRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public LocalTimeMinusMinutesRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `LocalTime#minusMinutes(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class LocalTimeMinusMinutes {\n \n @BeforeTemplate\n LocalTime before(LocalTime localTime, int minutes) {\n return localTime.minus(Duration.ofMinutes(minutes));\n }\n \n @BeforeTemplate\n LocalTime before(LocalTime localTime, long minutes) {\n return localTime.minus(minutes, ChronoUnit.MINUTES);\n }\n \n @AfterTemplate\n LocalTime after(LocalTime localTime, int minutes) {\n return localTime.minusMinutes(minutes);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{localTime:any(java.time.LocalTime)}.minus(java.time.Duration.ofMinutes(#{minutes:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{localTime:any(java.time.LocalTime)}.minus(#{minutes:any(long)}, java.time.temporal.ChronoUnit.MINUTES)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{localTime:any(java.time.LocalTime)}.minusMinutes(#{minutes:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.LocalTime", true),
new UsesMethod<>("java.time.LocalTime minus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.LocalTimeMinusHours}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class LocalTimeMinusHoursRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public LocalTimeMinusHoursRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `LocalTime#minusHours(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class LocalTimeMinusHours {\n \n @BeforeTemplate\n LocalTime before(LocalTime localTime, int hours) {\n return localTime.minus(Duration.ofHours(hours));\n }\n \n @BeforeTemplate\n LocalTime before(LocalTime localTime, long hours) {\n return localTime.minus(hours, ChronoUnit.HOURS);\n }\n \n @AfterTemplate\n LocalTime after(LocalTime localTime, int hours) {\n return localTime.minusHours(hours);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{localTime:any(java.time.LocalTime)}.minus(java.time.Duration.ofHours(#{hours:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{localTime:any(java.time.LocalTime)}.minus(#{hours:any(long)}, java.time.temporal.ChronoUnit.HOURS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{localTime:any(java.time.LocalTime)}.minusHours(#{hours:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.LocalTime", true),
new UsesMethod<>("java.time.LocalTime minus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.OffsetTimePlusNanos}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class OffsetTimePlusNanosRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public OffsetTimePlusNanosRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `OffsetTime#plusNanos(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class OffsetTimePlusNanos {\n \n @BeforeTemplate\n OffsetTime before(OffsetTime offsetTime, int nanos) {\n return offsetTime.plus(Duration.ofNanos(nanos));\n }\n \n @BeforeTemplate\n OffsetTime before(OffsetTime offsetTime, long nanos) {\n return offsetTime.plus(nanos, ChronoUnit.NANOS);\n }\n \n @AfterTemplate\n OffsetTime after(OffsetTime offsetTime, int nanos) {\n return offsetTime.plusNanos(nanos);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{offsetTime:any(java.time.OffsetTime)}.plus(java.time.Duration.ofNanos(#{nanos:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{offsetTime:any(java.time.OffsetTime)}.plus(#{nanos:any(long)}, java.time.temporal.ChronoUnit.NANOS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{offsetTime:any(java.time.OffsetTime)}.plusNanos(#{nanos:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.OffsetTime", true),
new UsesMethod<>("java.time.OffsetTime plus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.OffsetTimePlusSeconds}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class OffsetTimePlusSecondsRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public OffsetTimePlusSecondsRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `OffsetTime#plusSeconds(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class OffsetTimePlusSeconds {\n \n @BeforeTemplate\n OffsetTime before(OffsetTime offsetTime, int seconds) {\n return offsetTime.plus(Duration.ofSeconds(seconds));\n }\n \n @BeforeTemplate\n OffsetTime before(OffsetTime offsetTime, long seconds) {\n return offsetTime.plus(seconds, ChronoUnit.SECONDS);\n }\n \n @AfterTemplate\n OffsetTime after(OffsetTime offsetTime, int seconds) {\n return offsetTime.plusSeconds(seconds);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{offsetTime:any(java.time.OffsetTime)}.plus(java.time.Duration.ofSeconds(#{seconds:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{offsetTime:any(java.time.OffsetTime)}.plus(#{seconds:any(long)}, java.time.temporal.ChronoUnit.SECONDS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{offsetTime:any(java.time.OffsetTime)}.plusSeconds(#{seconds:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.OffsetTime", true),
new UsesMethod<>("java.time.OffsetTime plus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.OffsetTimePlusMinutes}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class OffsetTimePlusMinutesRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public OffsetTimePlusMinutesRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `OffsetTime#plusMinutes(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class OffsetTimePlusMinutes {\n \n @BeforeTemplate\n OffsetTime before(OffsetTime offsetTime, int minutes) {\n return offsetTime.plus(Duration.ofMinutes(minutes));\n }\n \n @BeforeTemplate\n OffsetTime before(OffsetTime offsetTime, long minutes) {\n return offsetTime.plus(minutes, ChronoUnit.MINUTES);\n }\n \n @AfterTemplate\n OffsetTime after(OffsetTime offsetTime, int minutes) {\n return offsetTime.plusMinutes(minutes);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{offsetTime:any(java.time.OffsetTime)}.plus(java.time.Duration.ofMinutes(#{minutes:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{offsetTime:any(java.time.OffsetTime)}.plus(#{minutes:any(long)}, java.time.temporal.ChronoUnit.MINUTES)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{offsetTime:any(java.time.OffsetTime)}.plusMinutes(#{minutes:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.OffsetTime", true),
new UsesMethod<>("java.time.OffsetTime plus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.OffsetTimePlusHours}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class OffsetTimePlusHoursRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public OffsetTimePlusHoursRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `OffsetTime#plusHours(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class OffsetTimePlusHours {\n \n @BeforeTemplate\n OffsetTime before(OffsetTime offsetTime, int hours) {\n return offsetTime.plus(Duration.ofHours(hours));\n }\n \n @BeforeTemplate\n OffsetTime before(OffsetTime offsetTime, long hours) {\n return offsetTime.plus(hours, ChronoUnit.HOURS);\n }\n \n @AfterTemplate\n OffsetTime after(OffsetTime offsetTime, int hours) {\n return offsetTime.plusHours(hours);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{offsetTime:any(java.time.OffsetTime)}.plus(java.time.Duration.ofHours(#{hours:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{offsetTime:any(java.time.OffsetTime)}.plus(#{hours:any(long)}, java.time.temporal.ChronoUnit.HOURS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{offsetTime:any(java.time.OffsetTime)}.plusHours(#{hours:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.OffsetTime", true),
new UsesMethod<>("java.time.OffsetTime plus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.OffsetTimeMinusNanos}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class OffsetTimeMinusNanosRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public OffsetTimeMinusNanosRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `OffsetTime#minusNanos(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class OffsetTimeMinusNanos {\n \n @BeforeTemplate\n OffsetTime before(OffsetTime offsetTime, int nanos) {\n return offsetTime.minus(Duration.ofNanos(nanos));\n }\n \n @BeforeTemplate\n OffsetTime before(OffsetTime offsetTime, long nanos) {\n return offsetTime.minus(nanos, ChronoUnit.NANOS);\n }\n \n @AfterTemplate\n OffsetTime after(OffsetTime offsetTime, int nanos) {\n return offsetTime.minusNanos(nanos);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{offsetTime:any(java.time.OffsetTime)}.minus(java.time.Duration.ofNanos(#{nanos:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{offsetTime:any(java.time.OffsetTime)}.minus(#{nanos:any(long)}, java.time.temporal.ChronoUnit.NANOS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{offsetTime:any(java.time.OffsetTime)}.minusNanos(#{nanos:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.OffsetTime", true),
new UsesMethod<>("java.time.OffsetTime minus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.OffsetTimeMinusSeconds}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class OffsetTimeMinusSecondsRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public OffsetTimeMinusSecondsRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `OffsetTime#minusSeconds(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class OffsetTimeMinusSeconds {\n \n @BeforeTemplate\n OffsetTime before(OffsetTime offsetTime, int seconds) {\n return offsetTime.minus(Duration.ofSeconds(seconds));\n }\n \n @BeforeTemplate\n OffsetTime before(OffsetTime offsetTime, long seconds) {\n return offsetTime.minus(seconds, ChronoUnit.SECONDS);\n }\n \n @AfterTemplate\n OffsetTime after(OffsetTime offsetTime, int seconds) {\n return offsetTime.minusSeconds(seconds);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{offsetTime:any(java.time.OffsetTime)}.minus(java.time.Duration.ofSeconds(#{seconds:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{offsetTime:any(java.time.OffsetTime)}.minus(#{seconds:any(long)}, java.time.temporal.ChronoUnit.SECONDS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{offsetTime:any(java.time.OffsetTime)}.minusSeconds(#{seconds:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.OffsetTime", true),
new UsesMethod<>("java.time.OffsetTime minus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.OffsetTimeMinusMinutes}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class OffsetTimeMinusMinutesRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public OffsetTimeMinusMinutesRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `OffsetTime#minusMinutes(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class OffsetTimeMinusMinutes {\n \n @BeforeTemplate\n OffsetTime before(OffsetTime offsetTime, int minutes) {\n return offsetTime.minus(Duration.ofMinutes(minutes));\n }\n \n @BeforeTemplate\n OffsetTime before(OffsetTime offsetTime, long minutes) {\n return offsetTime.minus(minutes, ChronoUnit.MINUTES);\n }\n \n @AfterTemplate\n OffsetTime after(OffsetTime offsetTime, int minutes) {\n return offsetTime.minusMinutes(minutes);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{offsetTime:any(java.time.OffsetTime)}.minus(java.time.Duration.ofMinutes(#{minutes:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{offsetTime:any(java.time.OffsetTime)}.minus(#{minutes:any(long)}, java.time.temporal.ChronoUnit.MINUTES)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{offsetTime:any(java.time.OffsetTime)}.minusMinutes(#{minutes:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.OffsetTime", true),
new UsesMethod<>("java.time.OffsetTime minus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.OffsetTimeMinusHours}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class OffsetTimeMinusHoursRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public OffsetTimeMinusHoursRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `OffsetTime#minusHours(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class OffsetTimeMinusHours {\n \n @BeforeTemplate\n OffsetTime before(OffsetTime offsetTime, int hours) {\n return offsetTime.minus(Duration.ofHours(hours));\n }\n \n @BeforeTemplate\n OffsetTime before(OffsetTime offsetTime, long hours) {\n return offsetTime.minus(hours, ChronoUnit.HOURS);\n }\n \n @AfterTemplate\n OffsetTime after(OffsetTime offsetTime, int hours) {\n return offsetTime.minusHours(hours);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{offsetTime:any(java.time.OffsetTime)}.minus(java.time.Duration.ofHours(#{hours:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{offsetTime:any(java.time.OffsetTime)}.minus(#{hours:any(long)}, java.time.temporal.ChronoUnit.HOURS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{offsetTime:any(java.time.OffsetTime)}.minusHours(#{hours:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.OffsetTime", true),
new UsesMethod<>("java.time.OffsetTime minus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.LocalDateTimePlusNanos}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class LocalDateTimePlusNanosRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public LocalDateTimePlusNanosRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `LocalDateTime#plusNanos(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class LocalDateTimePlusNanos {\n \n @BeforeTemplate\n LocalDateTime before(LocalDateTime localDateTime, int nanos) {\n return localDateTime.plus(Duration.ofNanos(nanos));\n }\n \n @BeforeTemplate\n LocalDateTime before(LocalDateTime localDateTime, long nanos) {\n return localDateTime.plus(nanos, ChronoUnit.NANOS);\n }\n \n @AfterTemplate\n LocalDateTime after(LocalDateTime localDateTime, int nanos) {\n return localDateTime.plusNanos(nanos);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.plus(java.time.Duration.ofNanos(#{nanos:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.plus(#{nanos:any(long)}, java.time.temporal.ChronoUnit.NANOS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.plusNanos(#{nanos:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.LocalDateTime", true),
new UsesMethod<>("java.time.LocalDateTime plus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.LocalDateTimePlusSeconds}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class LocalDateTimePlusSecondsRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public LocalDateTimePlusSecondsRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `LocalDateTime#plusSeconds(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class LocalDateTimePlusSeconds {\n \n @BeforeTemplate\n LocalDateTime before(LocalDateTime localDateTime, int seconds) {\n return localDateTime.plus(Duration.ofSeconds(seconds));\n }\n \n @BeforeTemplate\n LocalDateTime before(LocalDateTime localDateTime, long seconds) {\n return localDateTime.plus(seconds, ChronoUnit.SECONDS);\n }\n \n @AfterTemplate\n LocalDateTime after(LocalDateTime localDateTime, int seconds) {\n return localDateTime.plusSeconds(seconds);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.plus(java.time.Duration.ofSeconds(#{seconds:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.plus(#{seconds:any(long)}, java.time.temporal.ChronoUnit.SECONDS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.plusSeconds(#{seconds:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.LocalDateTime", true),
new UsesMethod<>("java.time.LocalDateTime plus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.LocalDateTimePlusMinutes}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class LocalDateTimePlusMinutesRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public LocalDateTimePlusMinutesRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `LocalDateTime#plusMinutes(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class LocalDateTimePlusMinutes {\n \n @BeforeTemplate\n LocalDateTime before(LocalDateTime localDateTime, int minutes) {\n return localDateTime.plus(Duration.ofMinutes(minutes));\n }\n \n @BeforeTemplate\n LocalDateTime before(LocalDateTime localDateTime, long minutes) {\n return localDateTime.plus(minutes, ChronoUnit.MINUTES);\n }\n \n @AfterTemplate\n LocalDateTime after(LocalDateTime localDateTime, int minutes) {\n return localDateTime.plusMinutes(minutes);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.plus(java.time.Duration.ofMinutes(#{minutes:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.plus(#{minutes:any(long)}, java.time.temporal.ChronoUnit.MINUTES)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.plusMinutes(#{minutes:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.LocalDateTime", true),
new UsesMethod<>("java.time.LocalDateTime plus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.LocalDateTimePlusHours}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class LocalDateTimePlusHoursRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public LocalDateTimePlusHoursRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `LocalDateTime#plusHours(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class LocalDateTimePlusHours {\n \n @BeforeTemplate\n LocalDateTime before(LocalDateTime localDateTime, int hours) {\n return localDateTime.plus(Duration.ofHours(hours));\n }\n \n @BeforeTemplate\n LocalDateTime before(LocalDateTime localDateTime, long hours) {\n return localDateTime.plus(hours, ChronoUnit.HOURS);\n }\n \n @AfterTemplate\n LocalDateTime after(LocalDateTime localDateTime, int hours) {\n return localDateTime.plusHours(hours);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.plus(java.time.Duration.ofHours(#{hours:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.plus(#{hours:any(long)}, java.time.temporal.ChronoUnit.HOURS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.plusHours(#{hours:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.LocalDateTime", true),
new UsesMethod<>("java.time.LocalDateTime plus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.LocalDateTimePlusDays}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class LocalDateTimePlusDaysRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public LocalDateTimePlusDaysRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `LocalDateTime#plusDays(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class LocalDateTimePlusDays {\n \n @BeforeTemplate\n LocalDateTime before(LocalDateTime localDateTime, int days) {\n return localDateTime.plus(Period.ofDays(days));\n }\n \n @BeforeTemplate\n LocalDateTime before(LocalDateTime localDateTime, long days) {\n return localDateTime.plus(days, ChronoUnit.DAYS);\n }\n \n @AfterTemplate\n LocalDateTime after(LocalDateTime localDateTime, int days) {\n return localDateTime.plusDays(days);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.plus(java.time.Period.ofDays(#{days:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.plus(#{days:any(long)}, java.time.temporal.ChronoUnit.DAYS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.plusDays(#{days:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Period");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.LocalDateTime", true),
new UsesMethod<>("java.time.LocalDateTime plus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.LocalDateTimePlusWeeks}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class LocalDateTimePlusWeeksRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public LocalDateTimePlusWeeksRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `LocalDateTime#plusWeeks(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class LocalDateTimePlusWeeks {\n \n @BeforeTemplate\n LocalDateTime before(LocalDateTime localDateTime, int weeks) {\n return localDateTime.plus(Period.ofWeeks(weeks));\n }\n \n @BeforeTemplate\n LocalDateTime before(LocalDateTime localDateTime, long weeks) {\n return localDateTime.plus(weeks, ChronoUnit.WEEKS);\n }\n \n @AfterTemplate\n LocalDateTime after(LocalDateTime localDateTime, int weeks) {\n return localDateTime.plusWeeks(weeks);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.plus(java.time.Period.ofWeeks(#{weeks:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.plus(#{weeks:any(long)}, java.time.temporal.ChronoUnit.WEEKS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.plusWeeks(#{weeks:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Period");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.LocalDateTime", true),
new UsesMethod<>("java.time.LocalDateTime plus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.LocalDateTimePlusMonths}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class LocalDateTimePlusMonthsRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public LocalDateTimePlusMonthsRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `LocalDateTime#plusMonths(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class LocalDateTimePlusMonths {\n \n @BeforeTemplate\n LocalDateTime before(LocalDateTime localDateTime, int months) {\n return localDateTime.plus(Period.ofMonths(months));\n }\n \n @BeforeTemplate\n LocalDateTime before(LocalDateTime localDateTime, long months) {\n return localDateTime.plus(months, ChronoUnit.MONTHS);\n }\n \n @AfterTemplate\n LocalDateTime after(LocalDateTime localDateTime, int months) {\n return localDateTime.plusMonths(months);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.plus(java.time.Period.ofMonths(#{months:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.plus(#{months:any(long)}, java.time.temporal.ChronoUnit.MONTHS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.plusMonths(#{months:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Period");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.LocalDateTime", true),
new UsesMethod<>("java.time.LocalDateTime plus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.LocalDateTimePlusYears}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class LocalDateTimePlusYearsRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public LocalDateTimePlusYearsRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `LocalDateTime#plusYears(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class LocalDateTimePlusYears {\n \n @BeforeTemplate\n LocalDateTime before(LocalDateTime localDateTime, int years) {\n return localDateTime.plus(Period.ofYears(years));\n }\n \n @BeforeTemplate\n LocalDateTime before(LocalDateTime localDateTime, long years) {\n return localDateTime.plus(years, ChronoUnit.YEARS);\n }\n \n @AfterTemplate\n LocalDateTime after(LocalDateTime localDateTime, int years) {\n return localDateTime.plusYears(years);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.plus(java.time.Period.ofYears(#{years:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.plus(#{years:any(long)}, java.time.temporal.ChronoUnit.YEARS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.plusYears(#{years:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Period");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.LocalDateTime", true),
new UsesMethod<>("java.time.LocalDateTime plus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.LocalDateTimeMinusNanos}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class LocalDateTimeMinusNanosRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public LocalDateTimeMinusNanosRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `LocalDateTime#minusNanos(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class LocalDateTimeMinusNanos {\n \n @BeforeTemplate\n LocalDateTime before(LocalDateTime localDateTime, int nanos) {\n return localDateTime.minus(Duration.ofNanos(nanos));\n }\n \n @BeforeTemplate\n LocalDateTime before(LocalDateTime localDateTime, long nanos) {\n return localDateTime.minus(nanos, ChronoUnit.NANOS);\n }\n \n @AfterTemplate\n LocalDateTime after(LocalDateTime localDateTime, int nanos) {\n return localDateTime.minusNanos(nanos);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.minus(java.time.Duration.ofNanos(#{nanos:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.minus(#{nanos:any(long)}, java.time.temporal.ChronoUnit.NANOS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.minusNanos(#{nanos:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.LocalDateTime", true),
new UsesMethod<>("java.time.LocalDateTime minus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.LocalDateTimeMinusSeconds}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class LocalDateTimeMinusSecondsRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public LocalDateTimeMinusSecondsRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `LocalDateTime#minusSeconds(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class LocalDateTimeMinusSeconds {\n \n @BeforeTemplate\n LocalDateTime before(LocalDateTime localDateTime, int seconds) {\n return localDateTime.minus(Duration.ofSeconds(seconds));\n }\n \n @BeforeTemplate\n LocalDateTime before(LocalDateTime localDateTime, long seconds) {\n return localDateTime.minus(seconds, ChronoUnit.SECONDS);\n }\n \n @AfterTemplate\n LocalDateTime after(LocalDateTime localDateTime, int seconds) {\n return localDateTime.minusSeconds(seconds);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.minus(java.time.Duration.ofSeconds(#{seconds:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.minus(#{seconds:any(long)}, java.time.temporal.ChronoUnit.SECONDS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.minusSeconds(#{seconds:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.LocalDateTime", true),
new UsesMethod<>("java.time.LocalDateTime minus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.LocalDateTimeMinusMinutes}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class LocalDateTimeMinusMinutesRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public LocalDateTimeMinusMinutesRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `LocalDateTime#minusMinutes(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class LocalDateTimeMinusMinutes {\n \n @BeforeTemplate\n LocalDateTime before(LocalDateTime localDateTime, int minutes) {\n return localDateTime.minus(Duration.ofMinutes(minutes));\n }\n \n @BeforeTemplate\n LocalDateTime before(LocalDateTime localDateTime, long minutes) {\n return localDateTime.minus(minutes, ChronoUnit.MINUTES);\n }\n \n @AfterTemplate\n LocalDateTime after(LocalDateTime localDateTime, int minutes) {\n return localDateTime.minusMinutes(minutes);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.minus(java.time.Duration.ofMinutes(#{minutes:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.minus(#{minutes:any(long)}, java.time.temporal.ChronoUnit.MINUTES)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.minusMinutes(#{minutes:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.LocalDateTime", true),
new UsesMethod<>("java.time.LocalDateTime minus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.LocalDateTimeMinusHours}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class LocalDateTimeMinusHoursRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public LocalDateTimeMinusHoursRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `LocalDateTime#minusHours(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class LocalDateTimeMinusHours {\n \n @BeforeTemplate\n LocalDateTime before(LocalDateTime localDateTime, int hours) {\n return localDateTime.minus(Duration.ofHours(hours));\n }\n \n @BeforeTemplate\n LocalDateTime before(LocalDateTime localDateTime, long hours) {\n return localDateTime.minus(hours, ChronoUnit.HOURS);\n }\n \n @AfterTemplate\n LocalDateTime after(LocalDateTime localDateTime, int hours) {\n return localDateTime.minusHours(hours);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.minus(java.time.Duration.ofHours(#{hours:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.minus(#{hours:any(long)}, java.time.temporal.ChronoUnit.HOURS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.minusHours(#{hours:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.LocalDateTime", true),
new UsesMethod<>("java.time.LocalDateTime minus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.LocalDateTimeMinusDays}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class LocalDateTimeMinusDaysRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public LocalDateTimeMinusDaysRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `LocalDateTime#minusDays(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class LocalDateTimeMinusDays {\n \n @BeforeTemplate\n LocalDateTime before(LocalDateTime localDateTime, int days) {\n return localDateTime.minus(Period.ofDays(days));\n }\n \n @BeforeTemplate\n LocalDateTime before(LocalDateTime localDateTime, long days) {\n return localDateTime.minus(days, ChronoUnit.DAYS);\n }\n \n @AfterTemplate\n LocalDateTime after(LocalDateTime localDateTime, int days) {\n return localDateTime.minusDays(days);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.minus(java.time.Period.ofDays(#{days:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.minus(#{days:any(long)}, java.time.temporal.ChronoUnit.DAYS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.minusDays(#{days:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Period");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.LocalDateTime", true),
new UsesMethod<>("java.time.LocalDateTime minus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.LocalDateTimeMinusWeeks}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class LocalDateTimeMinusWeeksRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public LocalDateTimeMinusWeeksRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `LocalDateTime#minusWeeks(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class LocalDateTimeMinusWeeks {\n \n @BeforeTemplate\n LocalDateTime before(LocalDateTime localDateTime, int weeks) {\n return localDateTime.minus(Period.ofWeeks(weeks));\n }\n \n @BeforeTemplate\n LocalDateTime before(LocalDateTime localDateTime, long weeks) {\n return localDateTime.minus(weeks, ChronoUnit.WEEKS);\n }\n \n @AfterTemplate\n LocalDateTime after(LocalDateTime localDateTime, int weeks) {\n return localDateTime.minusWeeks(weeks);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.minus(java.time.Period.ofWeeks(#{weeks:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.minus(#{weeks:any(long)}, java.time.temporal.ChronoUnit.WEEKS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.minusWeeks(#{weeks:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Period");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.LocalDateTime", true),
new UsesMethod<>("java.time.LocalDateTime minus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.LocalDateTimeMinusMonths}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class LocalDateTimeMinusMonthsRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public LocalDateTimeMinusMonthsRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `LocalDateTime#minusMonths(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class LocalDateTimeMinusMonths {\n \n @BeforeTemplate\n LocalDateTime before(LocalDateTime localDateTime, int months) {\n return localDateTime.minus(Period.ofMonths(months));\n }\n \n @BeforeTemplate\n LocalDateTime before(LocalDateTime localDateTime, long months) {\n return localDateTime.minus(months, ChronoUnit.MONTHS);\n }\n \n @AfterTemplate\n LocalDateTime after(LocalDateTime localDateTime, int months) {\n return localDateTime.minusMonths(months);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.minus(java.time.Period.ofMonths(#{months:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.minus(#{months:any(long)}, java.time.temporal.ChronoUnit.MONTHS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.minusMonths(#{months:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Period");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.LocalDateTime", true),
new UsesMethod<>("java.time.LocalDateTime minus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.LocalDateTimeMinusYears}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class LocalDateTimeMinusYearsRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public LocalDateTimeMinusYearsRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `LocalDateTime#minusYears(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class LocalDateTimeMinusYears {\n \n @BeforeTemplate\n LocalDateTime before(LocalDateTime localDateTime, int years) {\n return localDateTime.minus(Period.ofYears(years));\n }\n \n @BeforeTemplate\n LocalDateTime before(LocalDateTime localDateTime, long years) {\n return localDateTime.minus(years, ChronoUnit.YEARS);\n }\n \n @AfterTemplate\n LocalDateTime after(LocalDateTime localDateTime, int years) {\n return localDateTime.minusYears(years);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.minus(java.time.Period.ofYears(#{years:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.minus(#{years:any(long)}, java.time.temporal.ChronoUnit.YEARS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{localDateTime:any(java.time.LocalDateTime)}.minusYears(#{years:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Period");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.LocalDateTime", true),
new UsesMethod<>("java.time.LocalDateTime minus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.OffsetDateTimePlusNanos}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class OffsetDateTimePlusNanosRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public OffsetDateTimePlusNanosRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `OffsetDateTime#plusNanos(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class OffsetDateTimePlusNanos {\n \n @BeforeTemplate\n OffsetDateTime before(OffsetDateTime offsetDateTime, int nanos) {\n return offsetDateTime.plus(Duration.ofNanos(nanos));\n }\n \n @BeforeTemplate\n OffsetDateTime before(OffsetDateTime offsetDateTime, long nanos) {\n return offsetDateTime.plus(nanos, ChronoUnit.NANOS);\n }\n \n @AfterTemplate\n OffsetDateTime after(OffsetDateTime offsetDateTime, int nanos) {\n return offsetDateTime.plusNanos(nanos);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.plus(java.time.Duration.ofNanos(#{nanos:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.plus(#{nanos:any(long)}, java.time.temporal.ChronoUnit.NANOS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.plusNanos(#{nanos:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.OffsetDateTime", true),
new UsesMethod<>("java.time.OffsetDateTime plus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.OffsetDateTimePlusSeconds}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class OffsetDateTimePlusSecondsRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public OffsetDateTimePlusSecondsRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `OffsetDateTime#plusSeconds(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class OffsetDateTimePlusSeconds {\n \n @BeforeTemplate\n OffsetDateTime before(OffsetDateTime offsetDateTime, int seconds) {\n return offsetDateTime.plus(Duration.ofSeconds(seconds));\n }\n \n @BeforeTemplate\n OffsetDateTime before(OffsetDateTime offsetDateTime, long seconds) {\n return offsetDateTime.plus(seconds, ChronoUnit.SECONDS);\n }\n \n @AfterTemplate\n OffsetDateTime after(OffsetDateTime offsetDateTime, int seconds) {\n return offsetDateTime.plusSeconds(seconds);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.plus(java.time.Duration.ofSeconds(#{seconds:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.plus(#{seconds:any(long)}, java.time.temporal.ChronoUnit.SECONDS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.plusSeconds(#{seconds:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.OffsetDateTime", true),
new UsesMethod<>("java.time.OffsetDateTime plus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.OffsetDateTimePlusMinutes}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class OffsetDateTimePlusMinutesRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public OffsetDateTimePlusMinutesRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `OffsetDateTime#plusMinutes(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class OffsetDateTimePlusMinutes {\n \n @BeforeTemplate\n OffsetDateTime before(OffsetDateTime offsetDateTime, int minutes) {\n return offsetDateTime.plus(Duration.ofMinutes(minutes));\n }\n \n @BeforeTemplate\n OffsetDateTime before(OffsetDateTime offsetDateTime, long minutes) {\n return offsetDateTime.plus(minutes, ChronoUnit.MINUTES);\n }\n \n @AfterTemplate\n OffsetDateTime after(OffsetDateTime offsetDateTime, int minutes) {\n return offsetDateTime.plusMinutes(minutes);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.plus(java.time.Duration.ofMinutes(#{minutes:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.plus(#{minutes:any(long)}, java.time.temporal.ChronoUnit.MINUTES)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.plusMinutes(#{minutes:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.OffsetDateTime", true),
new UsesMethod<>("java.time.OffsetDateTime plus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.OffsetDateTimePlusHours}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class OffsetDateTimePlusHoursRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public OffsetDateTimePlusHoursRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `OffsetDateTime#plusHours(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class OffsetDateTimePlusHours {\n \n @BeforeTemplate\n OffsetDateTime before(OffsetDateTime offsetDateTime, int hours) {\n return offsetDateTime.plus(Duration.ofHours(hours));\n }\n \n @BeforeTemplate\n OffsetDateTime before(OffsetDateTime offsetDateTime, long hours) {\n return offsetDateTime.plus(hours, ChronoUnit.HOURS);\n }\n \n @AfterTemplate\n OffsetDateTime after(OffsetDateTime offsetDateTime, int hours) {\n return offsetDateTime.plusHours(hours);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.plus(java.time.Duration.ofHours(#{hours:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.plus(#{hours:any(long)}, java.time.temporal.ChronoUnit.HOURS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.plusHours(#{hours:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.OffsetDateTime", true),
new UsesMethod<>("java.time.OffsetDateTime plus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.OffsetDateTimePlusDays}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class OffsetDateTimePlusDaysRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public OffsetDateTimePlusDaysRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `OffsetDateTime#plusDays(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class OffsetDateTimePlusDays {\n \n @BeforeTemplate\n OffsetDateTime before(OffsetDateTime offsetDateTime, int days) {\n return offsetDateTime.plus(Period.ofDays(days));\n }\n \n @BeforeTemplate\n OffsetDateTime before(OffsetDateTime offsetDateTime, long days) {\n return offsetDateTime.plus(days, ChronoUnit.DAYS);\n }\n \n @AfterTemplate\n OffsetDateTime after(OffsetDateTime offsetDateTime, int days) {\n return offsetDateTime.plusDays(days);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.plus(java.time.Period.ofDays(#{days:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.plus(#{days:any(long)}, java.time.temporal.ChronoUnit.DAYS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.plusDays(#{days:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Period");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.OffsetDateTime", true),
new UsesMethod<>("java.time.OffsetDateTime plus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.OffsetDateTimePlusWeeks}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class OffsetDateTimePlusWeeksRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public OffsetDateTimePlusWeeksRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `OffsetDateTime#plusWeeks(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class OffsetDateTimePlusWeeks {\n \n @BeforeTemplate\n OffsetDateTime before(OffsetDateTime offsetDateTime, int weeks) {\n return offsetDateTime.plus(Period.ofWeeks(weeks));\n }\n \n @BeforeTemplate\n OffsetDateTime before(OffsetDateTime offsetDateTime, long weeks) {\n return offsetDateTime.plus(weeks, ChronoUnit.WEEKS);\n }\n \n @AfterTemplate\n OffsetDateTime after(OffsetDateTime offsetDateTime, int weeks) {\n return offsetDateTime.plusWeeks(weeks);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.plus(java.time.Period.ofWeeks(#{weeks:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.plus(#{weeks:any(long)}, java.time.temporal.ChronoUnit.WEEKS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.plusWeeks(#{weeks:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Period");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.OffsetDateTime", true),
new UsesMethod<>("java.time.OffsetDateTime plus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.OffsetDateTimePlusMonths}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class OffsetDateTimePlusMonthsRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public OffsetDateTimePlusMonthsRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `OffsetDateTime#plusMonths(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class OffsetDateTimePlusMonths {\n \n @BeforeTemplate\n OffsetDateTime before(OffsetDateTime offsetDateTime, int months) {\n return offsetDateTime.plus(Period.ofMonths(months));\n }\n \n @BeforeTemplate\n OffsetDateTime before(OffsetDateTime offsetDateTime, long months) {\n return offsetDateTime.plus(months, ChronoUnit.MONTHS);\n }\n \n @AfterTemplate\n OffsetDateTime after(OffsetDateTime offsetDateTime, int months) {\n return offsetDateTime.plusMonths(months);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.plus(java.time.Period.ofMonths(#{months:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.plus(#{months:any(long)}, java.time.temporal.ChronoUnit.MONTHS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.plusMonths(#{months:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Period");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.OffsetDateTime", true),
new UsesMethod<>("java.time.OffsetDateTime plus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.OffsetDateTimePlusYears}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class OffsetDateTimePlusYearsRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public OffsetDateTimePlusYearsRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `OffsetDateTime#plusYears(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class OffsetDateTimePlusYears {\n \n @BeforeTemplate\n OffsetDateTime before(OffsetDateTime offsetDateTime, int years) {\n return offsetDateTime.plus(Period.ofYears(years));\n }\n \n @BeforeTemplate\n OffsetDateTime before(OffsetDateTime offsetDateTime, long years) {\n return offsetDateTime.plus(years, ChronoUnit.YEARS);\n }\n \n @AfterTemplate\n OffsetDateTime after(OffsetDateTime offsetDateTime, int years) {\n return offsetDateTime.plusYears(years);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.plus(java.time.Period.ofYears(#{years:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.plus(#{years:any(long)}, java.time.temporal.ChronoUnit.YEARS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.plusYears(#{years:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Period");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.OffsetDateTime", true),
new UsesMethod<>("java.time.OffsetDateTime plus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.OffsetDateTimeMinusNanos}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class OffsetDateTimeMinusNanosRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public OffsetDateTimeMinusNanosRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `OffsetDateTime#minusNanos(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class OffsetDateTimeMinusNanos {\n \n @BeforeTemplate\n OffsetDateTime before(OffsetDateTime offsetDateTime, int nanos) {\n return offsetDateTime.minus(Duration.ofNanos(nanos));\n }\n \n @BeforeTemplate\n OffsetDateTime before(OffsetDateTime offsetDateTime, long nanos) {\n return offsetDateTime.minus(nanos, ChronoUnit.NANOS);\n }\n \n @AfterTemplate\n OffsetDateTime after(OffsetDateTime offsetDateTime, int nanos) {\n return offsetDateTime.minusNanos(nanos);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.minus(java.time.Duration.ofNanos(#{nanos:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.minus(#{nanos:any(long)}, java.time.temporal.ChronoUnit.NANOS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.minusNanos(#{nanos:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.OffsetDateTime", true),
new UsesMethod<>("java.time.OffsetDateTime minus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.OffsetDateTimeMinusSeconds}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class OffsetDateTimeMinusSecondsRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public OffsetDateTimeMinusSecondsRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `OffsetDateTime#minusSeconds(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class OffsetDateTimeMinusSeconds {\n \n @BeforeTemplate\n OffsetDateTime before(OffsetDateTime offsetDateTime, int seconds) {\n return offsetDateTime.minus(Duration.ofSeconds(seconds));\n }\n \n @BeforeTemplate\n OffsetDateTime before(OffsetDateTime offsetDateTime, long seconds) {\n return offsetDateTime.minus(seconds, ChronoUnit.SECONDS);\n }\n \n @AfterTemplate\n OffsetDateTime after(OffsetDateTime offsetDateTime, int seconds) {\n return offsetDateTime.minusSeconds(seconds);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.minus(java.time.Duration.ofSeconds(#{seconds:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.minus(#{seconds:any(long)}, java.time.temporal.ChronoUnit.SECONDS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.minusSeconds(#{seconds:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.OffsetDateTime", true),
new UsesMethod<>("java.time.OffsetDateTime minus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.OffsetDateTimeMinusMinutes}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class OffsetDateTimeMinusMinutesRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public OffsetDateTimeMinusMinutesRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `OffsetDateTime#minusMinutes(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class OffsetDateTimeMinusMinutes {\n \n @BeforeTemplate\n OffsetDateTime before(OffsetDateTime offsetDateTime, int minutes) {\n return offsetDateTime.minus(Duration.ofMinutes(minutes));\n }\n \n @BeforeTemplate\n OffsetDateTime before(OffsetDateTime offsetDateTime, long minutes) {\n return offsetDateTime.minus(minutes, ChronoUnit.MINUTES);\n }\n \n @AfterTemplate\n OffsetDateTime after(OffsetDateTime offsetDateTime, int minutes) {\n return offsetDateTime.minusMinutes(minutes);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.minus(java.time.Duration.ofMinutes(#{minutes:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.minus(#{minutes:any(long)}, java.time.temporal.ChronoUnit.MINUTES)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.minusMinutes(#{minutes:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.OffsetDateTime", true),
new UsesMethod<>("java.time.OffsetDateTime minus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.OffsetDateTimeMinusHours}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class OffsetDateTimeMinusHoursRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public OffsetDateTimeMinusHoursRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `OffsetDateTime#minusHours(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class OffsetDateTimeMinusHours {\n \n @BeforeTemplate\n OffsetDateTime before(OffsetDateTime offsetDateTime, int hours) {\n return offsetDateTime.minus(Duration.ofHours(hours));\n }\n \n @BeforeTemplate\n OffsetDateTime before(OffsetDateTime offsetDateTime, long hours) {\n return offsetDateTime.minus(hours, ChronoUnit.HOURS);\n }\n \n @AfterTemplate\n OffsetDateTime after(OffsetDateTime offsetDateTime, int hours) {\n return offsetDateTime.minusHours(hours);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.minus(java.time.Duration.ofHours(#{hours:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.minus(#{hours:any(long)}, java.time.temporal.ChronoUnit.HOURS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.minusHours(#{hours:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.OffsetDateTime", true),
new UsesMethod<>("java.time.OffsetDateTime minus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.OffsetDateTimeMinusDays}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class OffsetDateTimeMinusDaysRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public OffsetDateTimeMinusDaysRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `OffsetDateTime#minusDays(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class OffsetDateTimeMinusDays {\n \n @BeforeTemplate\n OffsetDateTime before(OffsetDateTime offsetDateTime, int days) {\n return offsetDateTime.minus(Period.ofDays(days));\n }\n \n @BeforeTemplate\n OffsetDateTime before(OffsetDateTime offsetDateTime, long days) {\n return offsetDateTime.minus(days, ChronoUnit.DAYS);\n }\n \n @AfterTemplate\n OffsetDateTime after(OffsetDateTime offsetDateTime, int days) {\n return offsetDateTime.minusDays(days);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.minus(java.time.Period.ofDays(#{days:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.minus(#{days:any(long)}, java.time.temporal.ChronoUnit.DAYS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.minusDays(#{days:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Period");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.OffsetDateTime", true),
new UsesMethod<>("java.time.OffsetDateTime minus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.OffsetDateTimeMinusWeeks}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class OffsetDateTimeMinusWeeksRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public OffsetDateTimeMinusWeeksRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `OffsetDateTime#minusWeeks(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class OffsetDateTimeMinusWeeks {\n \n @BeforeTemplate\n OffsetDateTime before(OffsetDateTime offsetDateTime, int weeks) {\n return offsetDateTime.minus(Period.ofWeeks(weeks));\n }\n \n @BeforeTemplate\n OffsetDateTime before(OffsetDateTime offsetDateTime, long weeks) {\n return offsetDateTime.minus(weeks, ChronoUnit.WEEKS);\n }\n \n @AfterTemplate\n OffsetDateTime after(OffsetDateTime offsetDateTime, int weeks) {\n return offsetDateTime.minusWeeks(weeks);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.minus(java.time.Period.ofWeeks(#{weeks:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.minus(#{weeks:any(long)}, java.time.temporal.ChronoUnit.WEEKS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.minusWeeks(#{weeks:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Period");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.OffsetDateTime", true),
new UsesMethod<>("java.time.OffsetDateTime minus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.OffsetDateTimeMinusMonths}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class OffsetDateTimeMinusMonthsRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public OffsetDateTimeMinusMonthsRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `OffsetDateTime#minusMonths(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class OffsetDateTimeMinusMonths {\n \n @BeforeTemplate\n OffsetDateTime before(OffsetDateTime offsetDateTime, int months) {\n return offsetDateTime.minus(Period.ofMonths(months));\n }\n \n @BeforeTemplate\n OffsetDateTime before(OffsetDateTime offsetDateTime, long months) {\n return offsetDateTime.minus(months, ChronoUnit.MONTHS);\n }\n \n @AfterTemplate\n OffsetDateTime after(OffsetDateTime offsetDateTime, int months) {\n return offsetDateTime.minusMonths(months);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.minus(java.time.Period.ofMonths(#{months:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.minus(#{months:any(long)}, java.time.temporal.ChronoUnit.MONTHS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.minusMonths(#{months:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Period");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.OffsetDateTime", true),
new UsesMethod<>("java.time.OffsetDateTime minus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.OffsetDateTimeMinusYears}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class OffsetDateTimeMinusYearsRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public OffsetDateTimeMinusYearsRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `OffsetDateTime#minusYears(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class OffsetDateTimeMinusYears {\n \n @BeforeTemplate\n OffsetDateTime before(OffsetDateTime offsetDateTime, int years) {\n return offsetDateTime.minus(Period.ofYears(years));\n }\n \n @BeforeTemplate\n OffsetDateTime before(OffsetDateTime offsetDateTime, long years) {\n return offsetDateTime.minus(years, ChronoUnit.YEARS);\n }\n \n @AfterTemplate\n OffsetDateTime after(OffsetDateTime offsetDateTime, int years) {\n return offsetDateTime.minusYears(years);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.minus(java.time.Period.ofYears(#{years:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.minus(#{years:any(long)}, java.time.temporal.ChronoUnit.YEARS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{offsetDateTime:any(java.time.OffsetDateTime)}.minusYears(#{years:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Period");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.OffsetDateTime", true),
new UsesMethod<>("java.time.OffsetDateTime minus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.ZonedDateTimePlusNanos}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class ZonedDateTimePlusNanosRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public ZonedDateTimePlusNanosRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `ZonedDateTime#plusNanos(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class ZonedDateTimePlusNanos {\n \n @BeforeTemplate\n ZonedDateTime before(ZonedDateTime zonedDateTime, int nanos) {\n return zonedDateTime.plus(Duration.ofNanos(nanos));\n }\n \n @BeforeTemplate\n ZonedDateTime before(ZonedDateTime zonedDateTime, long nanos) {\n return zonedDateTime.plus(nanos, ChronoUnit.NANOS);\n }\n \n @AfterTemplate\n ZonedDateTime after(ZonedDateTime zonedDateTime, int nanos) {\n return zonedDateTime.plusNanos(nanos);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.plus(java.time.Duration.ofNanos(#{nanos:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.plus(#{nanos:any(long)}, java.time.temporal.ChronoUnit.NANOS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.plusNanos(#{nanos:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.ZonedDateTime", true),
new UsesMethod<>("java.time.ZonedDateTime plus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.ZonedDateTimePlusSeconds}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class ZonedDateTimePlusSecondsRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public ZonedDateTimePlusSecondsRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `ZonedDateTime#plusSeconds(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class ZonedDateTimePlusSeconds {\n \n @BeforeTemplate\n ZonedDateTime before(ZonedDateTime zonedDateTime, int seconds) {\n return zonedDateTime.plus(Duration.ofSeconds(seconds));\n }\n \n @BeforeTemplate\n ZonedDateTime before(ZonedDateTime zonedDateTime, long seconds) {\n return zonedDateTime.plus(seconds, ChronoUnit.SECONDS);\n }\n \n @AfterTemplate\n ZonedDateTime after(ZonedDateTime zonedDateTime, int seconds) {\n return zonedDateTime.plusSeconds(seconds);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.plus(java.time.Duration.ofSeconds(#{seconds:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.plus(#{seconds:any(long)}, java.time.temporal.ChronoUnit.SECONDS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.plusSeconds(#{seconds:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.ZonedDateTime", true),
new UsesMethod<>("java.time.ZonedDateTime plus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.ZonedDateTimePlusMinutes}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class ZonedDateTimePlusMinutesRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public ZonedDateTimePlusMinutesRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `ZonedDateTime#plusMinutes(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class ZonedDateTimePlusMinutes {\n \n @BeforeTemplate\n ZonedDateTime before(ZonedDateTime zonedDateTime, int minutes) {\n return zonedDateTime.plus(Duration.ofMinutes(minutes));\n }\n \n @BeforeTemplate\n ZonedDateTime before(ZonedDateTime zonedDateTime, long minutes) {\n return zonedDateTime.plus(minutes, ChronoUnit.MINUTES);\n }\n \n @AfterTemplate\n ZonedDateTime after(ZonedDateTime zonedDateTime, int minutes) {\n return zonedDateTime.plusMinutes(minutes);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.plus(java.time.Duration.ofMinutes(#{minutes:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.plus(#{minutes:any(long)}, java.time.temporal.ChronoUnit.MINUTES)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.plusMinutes(#{minutes:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.ZonedDateTime", true),
new UsesMethod<>("java.time.ZonedDateTime plus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.ZonedDateTimePlusHours}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class ZonedDateTimePlusHoursRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public ZonedDateTimePlusHoursRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `ZonedDateTime#plusHours(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class ZonedDateTimePlusHours {\n \n @BeforeTemplate\n ZonedDateTime before(ZonedDateTime zonedDateTime, int hours) {\n return zonedDateTime.plus(Duration.ofHours(hours));\n }\n \n @BeforeTemplate\n ZonedDateTime before(ZonedDateTime zonedDateTime, long hours) {\n return zonedDateTime.plus(hours, ChronoUnit.HOURS);\n }\n \n @AfterTemplate\n ZonedDateTime after(ZonedDateTime zonedDateTime, int hours) {\n return zonedDateTime.plusHours(hours);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.plus(java.time.Duration.ofHours(#{hours:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.plus(#{hours:any(long)}, java.time.temporal.ChronoUnit.HOURS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.plusHours(#{hours:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.ZonedDateTime", true),
new UsesMethod<>("java.time.ZonedDateTime plus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.ZonedDateTimePlusDays}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class ZonedDateTimePlusDaysRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public ZonedDateTimePlusDaysRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `ZonedDateTime#plusDays(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class ZonedDateTimePlusDays {\n \n @BeforeTemplate\n ZonedDateTime before(ZonedDateTime zonedDateTime, int days) {\n return zonedDateTime.plus(Period.ofDays(days));\n }\n \n @BeforeTemplate\n ZonedDateTime before(ZonedDateTime zonedDateTime, long days) {\n return zonedDateTime.plus(days, ChronoUnit.DAYS);\n }\n \n @AfterTemplate\n ZonedDateTime after(ZonedDateTime zonedDateTime, int days) {\n return zonedDateTime.plusDays(days);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.plus(java.time.Period.ofDays(#{days:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.plus(#{days:any(long)}, java.time.temporal.ChronoUnit.DAYS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.plusDays(#{days:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Period");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.ZonedDateTime", true),
new UsesMethod<>("java.time.ZonedDateTime plus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.ZonedDateTimePlusWeeks}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class ZonedDateTimePlusWeeksRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public ZonedDateTimePlusWeeksRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `ZonedDateTime#plusWeeks(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class ZonedDateTimePlusWeeks {\n \n @BeforeTemplate\n ZonedDateTime before(ZonedDateTime zonedDateTime, int weeks) {\n return zonedDateTime.plus(Period.ofWeeks(weeks));\n }\n \n @BeforeTemplate\n ZonedDateTime before(ZonedDateTime zonedDateTime, long weeks) {\n return zonedDateTime.plus(weeks, ChronoUnit.WEEKS);\n }\n \n @AfterTemplate\n ZonedDateTime after(ZonedDateTime zonedDateTime, int weeks) {\n return zonedDateTime.plusWeeks(weeks);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.plus(java.time.Period.ofWeeks(#{weeks:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.plus(#{weeks:any(long)}, java.time.temporal.ChronoUnit.WEEKS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.plusWeeks(#{weeks:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Period");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.ZonedDateTime", true),
new UsesMethod<>("java.time.ZonedDateTime plus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.ZonedDateTimePlusMonths}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class ZonedDateTimePlusMonthsRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public ZonedDateTimePlusMonthsRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `ZonedDateTime#plusMonths(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class ZonedDateTimePlusMonths {\n \n @BeforeTemplate\n ZonedDateTime before(ZonedDateTime zonedDateTime, int months) {\n return zonedDateTime.plus(Period.ofMonths(months));\n }\n \n @BeforeTemplate\n ZonedDateTime before(ZonedDateTime zonedDateTime, long months) {\n return zonedDateTime.plus(months, ChronoUnit.MONTHS);\n }\n \n @AfterTemplate\n ZonedDateTime after(ZonedDateTime zonedDateTime, int months) {\n return zonedDateTime.plusMonths(months);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.plus(java.time.Period.ofMonths(#{months:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.plus(#{months:any(long)}, java.time.temporal.ChronoUnit.MONTHS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.plusMonths(#{months:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Period");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.ZonedDateTime", true),
new UsesMethod<>("java.time.ZonedDateTime plus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.ZonedDateTimePlusYears}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class ZonedDateTimePlusYearsRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public ZonedDateTimePlusYearsRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `ZonedDateTime#plusYears(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class ZonedDateTimePlusYears {\n \n @BeforeTemplate\n ZonedDateTime before(ZonedDateTime zonedDateTime, int years) {\n return zonedDateTime.plus(Period.ofYears(years));\n }\n \n @BeforeTemplate\n ZonedDateTime before(ZonedDateTime zonedDateTime, long years) {\n return zonedDateTime.plus(years, ChronoUnit.YEARS);\n }\n \n @AfterTemplate\n ZonedDateTime after(ZonedDateTime zonedDateTime, int years) {\n return zonedDateTime.plusYears(years);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.plus(java.time.Period.ofYears(#{years:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.plus(#{years:any(long)}, java.time.temporal.ChronoUnit.YEARS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.plusYears(#{years:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Period");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.ZonedDateTime", true),
new UsesMethod<>("java.time.ZonedDateTime plus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.ZonedDateTimeMinusNanos}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class ZonedDateTimeMinusNanosRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public ZonedDateTimeMinusNanosRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `ZonedDateTime#minusNanos(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class ZonedDateTimeMinusNanos {\n \n @BeforeTemplate\n ZonedDateTime before(ZonedDateTime zonedDateTime, int nanos) {\n return zonedDateTime.minus(Duration.ofNanos(nanos));\n }\n \n @BeforeTemplate\n ZonedDateTime before(ZonedDateTime zonedDateTime, long nanos) {\n return zonedDateTime.minus(nanos, ChronoUnit.NANOS);\n }\n \n @AfterTemplate\n ZonedDateTime after(ZonedDateTime zonedDateTime, int nanos) {\n return zonedDateTime.minusNanos(nanos);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.minus(java.time.Duration.ofNanos(#{nanos:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.minus(#{nanos:any(long)}, java.time.temporal.ChronoUnit.NANOS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.minusNanos(#{nanos:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.ZonedDateTime", true),
new UsesMethod<>("java.time.ZonedDateTime minus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.ZonedDateTimeMinusSeconds}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class ZonedDateTimeMinusSecondsRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public ZonedDateTimeMinusSecondsRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `ZonedDateTime#minusSeconds(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class ZonedDateTimeMinusSeconds {\n \n @BeforeTemplate\n ZonedDateTime before(ZonedDateTime zonedDateTime, int seconds) {\n return zonedDateTime.minus(Duration.ofSeconds(seconds));\n }\n \n @BeforeTemplate\n ZonedDateTime before(ZonedDateTime zonedDateTime, long seconds) {\n return zonedDateTime.minus(seconds, ChronoUnit.SECONDS);\n }\n \n @AfterTemplate\n ZonedDateTime after(ZonedDateTime zonedDateTime, int seconds) {\n return zonedDateTime.minusSeconds(seconds);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.minus(java.time.Duration.ofSeconds(#{seconds:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.minus(#{seconds:any(long)}, java.time.temporal.ChronoUnit.SECONDS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.minusSeconds(#{seconds:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.ZonedDateTime", true),
new UsesMethod<>("java.time.ZonedDateTime minus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.ZonedDateTimeMinusMinutes}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class ZonedDateTimeMinusMinutesRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public ZonedDateTimeMinusMinutesRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `ZonedDateTime#minusMinutes(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class ZonedDateTimeMinusMinutes {\n \n @BeforeTemplate\n ZonedDateTime before(ZonedDateTime zonedDateTime, int minutes) {\n return zonedDateTime.minus(Duration.ofMinutes(minutes));\n }\n \n @BeforeTemplate\n ZonedDateTime before(ZonedDateTime zonedDateTime, long minutes) {\n return zonedDateTime.minus(minutes, ChronoUnit.MINUTES);\n }\n \n @AfterTemplate\n ZonedDateTime after(ZonedDateTime zonedDateTime, int minutes) {\n return zonedDateTime.minusMinutes(minutes);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.minus(java.time.Duration.ofMinutes(#{minutes:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.minus(#{minutes:any(long)}, java.time.temporal.ChronoUnit.MINUTES)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.minusMinutes(#{minutes:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.ZonedDateTime", true),
new UsesMethod<>("java.time.ZonedDateTime minus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.ZonedDateTimeMinusHours}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class ZonedDateTimeMinusHoursRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public ZonedDateTimeMinusHoursRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `ZonedDateTime#minusHours(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class ZonedDateTimeMinusHours {\n \n @BeforeTemplate\n ZonedDateTime before(ZonedDateTime zonedDateTime, int hours) {\n return zonedDateTime.minus(Duration.ofHours(hours));\n }\n \n @BeforeTemplate\n ZonedDateTime before(ZonedDateTime zonedDateTime, long hours) {\n return zonedDateTime.minus(hours, ChronoUnit.HOURS);\n }\n \n @AfterTemplate\n ZonedDateTime after(ZonedDateTime zonedDateTime, int hours) {\n return zonedDateTime.minusHours(hours);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.minus(java.time.Duration.ofHours(#{hours:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.minus(#{hours:any(long)}, java.time.temporal.ChronoUnit.HOURS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.minusHours(#{hours:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Duration");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.ZonedDateTime", true),
new UsesMethod<>("java.time.ZonedDateTime minus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.ZonedDateTimeMinusDays}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class ZonedDateTimeMinusDaysRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public ZonedDateTimeMinusDaysRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `ZonedDateTime#minusDays(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class ZonedDateTimeMinusDays {\n \n @BeforeTemplate\n ZonedDateTime before(ZonedDateTime zonedDateTime, int days) {\n return zonedDateTime.minus(Period.ofDays(days));\n }\n \n @BeforeTemplate\n ZonedDateTime before(ZonedDateTime zonedDateTime, long days) {\n return zonedDateTime.minus(days, ChronoUnit.DAYS);\n }\n \n @AfterTemplate\n ZonedDateTime after(ZonedDateTime zonedDateTime, int days) {\n return zonedDateTime.minusDays(days);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.minus(java.time.Period.ofDays(#{days:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.minus(#{days:any(long)}, java.time.temporal.ChronoUnit.DAYS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.minusDays(#{days:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Period");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.ZonedDateTime", true),
new UsesMethod<>("java.time.ZonedDateTime minus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.ZonedDateTimeMinusWeeks}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class ZonedDateTimeMinusWeeksRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public ZonedDateTimeMinusWeeksRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `ZonedDateTime#minusWeeks(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class ZonedDateTimeMinusWeeks {\n \n @BeforeTemplate\n ZonedDateTime before(ZonedDateTime zonedDateTime, int weeks) {\n return zonedDateTime.minus(Period.ofWeeks(weeks));\n }\n \n @BeforeTemplate\n ZonedDateTime before(ZonedDateTime zonedDateTime, long weeks) {\n return zonedDateTime.minus(weeks, ChronoUnit.WEEKS);\n }\n \n @AfterTemplate\n ZonedDateTime after(ZonedDateTime zonedDateTime, int weeks) {\n return zonedDateTime.minusWeeks(weeks);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.minus(java.time.Period.ofWeeks(#{weeks:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.minus(#{weeks:any(long)}, java.time.temporal.ChronoUnit.WEEKS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.minusWeeks(#{weeks:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Period");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.ZonedDateTime", true),
new UsesMethod<>("java.time.ZonedDateTime minus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.ZonedDateTimeMinusMonths}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class ZonedDateTimeMinusMonthsRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public ZonedDateTimeMinusMonthsRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `ZonedDateTime#minusMonths(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class ZonedDateTimeMinusMonths {\n \n @BeforeTemplate\n ZonedDateTime before(ZonedDateTime zonedDateTime, int months) {\n return zonedDateTime.minus(Period.ofMonths(months));\n }\n \n @BeforeTemplate\n ZonedDateTime before(ZonedDateTime zonedDateTime, long months) {\n return zonedDateTime.minus(months, ChronoUnit.MONTHS);\n }\n \n @AfterTemplate\n ZonedDateTime after(ZonedDateTime zonedDateTime, int months) {\n return zonedDateTime.minusMonths(months);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.minus(java.time.Period.ofMonths(#{months:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.minus(#{months:any(long)}, java.time.temporal.ChronoUnit.MONTHS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.minusMonths(#{months:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Period");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.ZonedDateTime", true),
new UsesMethod<>("java.time.ZonedDateTime minus(..)")
),
javaVisitor
);
}
}
/**
* OpenRewrite recipe created for Refaster template {@code TimeRules.ZonedDateTimeMinusYears}.
*/
@SuppressWarnings("all")
@NonNullApi
@Generated("org.openrewrite.java.template.processor.RefasterTemplateProcessor")
public static class ZonedDateTimeMinusYearsRecipe extends Recipe {
/**
* Instantiates a new instance.
*/
public ZonedDateTimeMinusYearsRecipe() {}
@Override
public String getDisplayName() {
return "Prefer `ZonedDateTime#minusYears(long)` over more contrived alternatives";
}
@Override
public String getDescription() {
return "Recipe created for the following Refaster template:\n```java\nstatic final class ZonedDateTimeMinusYears {\n \n @BeforeTemplate\n ZonedDateTime before(ZonedDateTime zonedDateTime, int years) {\n return zonedDateTime.minus(Period.ofYears(years));\n }\n \n @BeforeTemplate\n ZonedDateTime before(ZonedDateTime zonedDateTime, long years) {\n return zonedDateTime.minus(years, ChronoUnit.YEARS);\n }\n \n @AfterTemplate\n ZonedDateTime after(ZonedDateTime zonedDateTime, int years) {\n return zonedDateTime.minusYears(years);\n }\n}\n```\n.";
}
@Override
public TreeVisitor, ExecutionContext> getVisitor() {
JavaVisitor javaVisitor = new AbstractRefasterJavaVisitor() {
final JavaTemplate before = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.minus(java.time.Period.ofYears(#{years:any(int)}))")
.build();
final JavaTemplate before0 = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.minus(#{years:any(long)}, java.time.temporal.ChronoUnit.YEARS)")
.build();
final JavaTemplate after = JavaTemplate
.builder("#{zonedDateTime:any(java.time.ZonedDateTime)}.minusYears(#{years:any(int)})")
.build();
@Override
public J visitMethodInvocation(J.MethodInvocation elem, ExecutionContext ctx) {
JavaTemplate.Matcher matcher;
if ((matcher = before.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.Period");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
if ((matcher = before0.matcher(getCursor())).find()) {
maybeRemoveImport("java.time.temporal.ChronoUnit");
return embed(
after.apply(getCursor(), elem.getCoordinates().replace(), matcher.parameter(0), matcher.parameter(1)),
getCursor(),
ctx,
SHORTEN_NAMES
);
}
return super.visitMethodInvocation(elem, ctx);
}
};
return Preconditions.check(
Preconditions.and(
new UsesType<>("java.time.temporal.ChronoUnit", true),
new UsesType<>("java.time.ZonedDateTime", true),
new UsesMethod<>("java.time.ZonedDateTime minus(..)")
),
javaVisitor
);
}
}
}