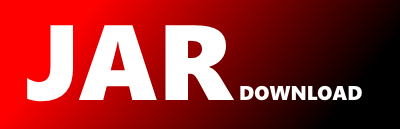
tech.tablesaw.api.ml.classification.Knn Maven / Gradle / Ivy
package tech.tablesaw.api.ml.classification;
import com.google.common.base.Preconditions;
import smile.classification.KNN;
import tech.tablesaw.api.BooleanColumn;
import tech.tablesaw.api.CategoryColumn;
import tech.tablesaw.api.IntColumn;
import tech.tablesaw.api.NumericColumn;
import tech.tablesaw.api.ShortColumn;
import tech.tablesaw.util.DoubleArrays;
import java.util.SortedSet;
import java.util.TreeSet;
/**
*
*/
public class Knn extends AbstractClassifier {
private final KNN classifierModel;
private Knn(KNN classifierModel) {
this.classifierModel = classifierModel;
}
public static Knn learn(int k, ShortColumn labels, NumericColumn... predictors) {
KNN classifierModel = KNN.learn(DoubleArrays.to2dArray(predictors), labels.toIntArray(), k);
return new Knn(classifierModel);
}
public static Knn learn(int k, IntColumn labels, NumericColumn... predictors) {
KNN classifierModel = KNN.learn(DoubleArrays.to2dArray(predictors), labels.data().toIntArray(), k);
return new Knn(classifierModel);
}
public static Knn learn(int k, BooleanColumn labels, NumericColumn... predictors) {
KNN classifierModel = KNN.learn(DoubleArrays.to2dArray(predictors), labels.toIntArray(), k);
return new Knn(classifierModel);
}
public static Knn learn(int k, CategoryColumn labels, NumericColumn... predictors) {
KNN classifierModel = KNN.learn(DoubleArrays.to2dArray(predictors), labels.data().toIntArray(), k);
return new Knn(classifierModel);
}
public int predict(double[] data) {
return classifierModel.predict(data);
}
public ConfusionMatrix predictMatrix(ShortColumn labels, NumericColumn... predictors) {
Preconditions.checkArgument(predictors.length > 0);
SortedSet
© 2015 - 2025 Weber Informatics LLC | Privacy Policy