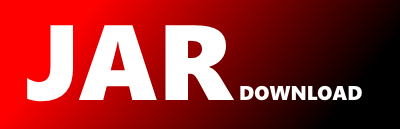
tech.uom.lib.assertj.assertions.AbstractQuantityAssert Maven / Gradle / Ivy
package tech.uom.lib.assertj.assertions;
import javax.measure.Quantity;
import org.assertj.core.api.AbstractObjectAssert;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link Quantity} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractQuantityAssert, A extends Quantity> extends AbstractObjectAssert {
/**
* Creates a new {@link AbstractQuantityAssert}
to make assertions on actual Quantity.
* @param actual the Quantity we want to make assertions on.
*/
protected AbstractQuantityAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual Quantity's unit is equal to the given one.
* @param unit the given unit to compare the actual Quantity's unit to.
* @return this assertion object.
* @throws AssertionError - if the actual Quantity's unit is not equal to the given one.
*/
public S hasUnit(javax.measure.Unit unit) {
// check that actual Quantity we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting unit of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
javax.measure.Unit actualUnit = actual.getUnit();
if (!Objects.areEqual(actualUnit, unit)) {
failWithMessage(assertjErrorMessage, actual, unit, actualUnit);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual Quantity's value is equal to the given one.
* @param value the given value to compare the actual Quantity's value to.
* @return this assertion object.
* @throws AssertionError - if the actual Quantity's value is not equal to the given one.
*/
public S hasValue(Number value) {
// check that actual Quantity we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting value of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
Number actualValue = actual.getValue();
if (!Objects.areEqual(actualValue, value)) {
failWithMessage(assertjErrorMessage, actual, value, actualValue);
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy