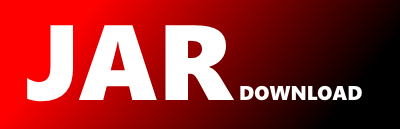
emu.grasscutter.server.packet.send.PacketPlayerChatNotify Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of grasscutter Show documentation
Show all versions of grasscutter Show documentation
A server software reimplementation for an anime game.
The newest version!
package emu.grasscutter.server.packet.send;
import emu.grasscutter.game.player.Player;
import emu.grasscutter.net.packet.BasePacket;
import emu.grasscutter.net.packet.PacketOpcodes;
import emu.grasscutter.net.proto.ChatInfoOuterClass.ChatInfo;
import emu.grasscutter.net.proto.PlayerChatNotifyOuterClass.PlayerChatNotify;
import emu.grasscutter.net.proto.SystemHintOuterClass.SystemHint;
public class PacketPlayerChatNotify extends BasePacket {
public PacketPlayerChatNotify(Player sender, int channelId, String message) {
super(PacketOpcodes.PlayerChatNotify);
ChatInfo info = ChatInfo.newBuilder()
.setTime((int) (System.currentTimeMillis() / 1000))
.setUid(sender.getUid())
.setText(message)
.build();
PlayerChatNotify proto = PlayerChatNotify.newBuilder()
.setChannelId(channelId)
.setChatInfo(info)
.build();
this.setData(proto);
}
public PacketPlayerChatNotify(Player sender, int channelId, int emote) {
super(PacketOpcodes.PlayerChatNotify);
ChatInfo info = ChatInfo.newBuilder()
.setTime((int) (System.currentTimeMillis() / 1000))
.setUid(sender.getUid())
.setIcon(emote)
.build();
PlayerChatNotify proto = PlayerChatNotify.newBuilder()
.setChannelId(channelId)
.setChatInfo(info)
.build();
this.setData(proto);
}
public PacketPlayerChatNotify(Player sender, int channelId, ChatInfo.SystemHint systemHint) {
super(PacketOpcodes.PlayerChatNotify);
ChatInfo info = ChatInfo.newBuilder()
.setTime((int) (System.currentTimeMillis() / 1000))
.setUid(sender.getUid())
.setSystemHint(systemHint)
.build();
PlayerChatNotify proto = PlayerChatNotify.newBuilder()
.setChannelId(channelId)
.setChatInfo(info)
.build();
this.setData(proto);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy