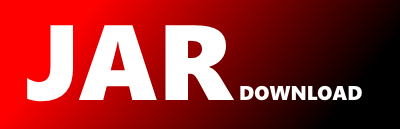
tech.ydb.yoj.databind.converter.ValueConverter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yoj-databind Show documentation
Show all versions of yoj-databind Show documentation
Core data-binding logic used by YOJ (YDB ORM for Java) to convert
between Java objects and database rows (or anything representable by
a Java Map, really).
The newest version!
package tech.ydb.yoj.databind.converter;
import lombok.NonNull;
import tech.ydb.yoj.ExperimentalApi;
import tech.ydb.yoj.databind.schema.Schema.JavaField;
/**
* Custom conversion logic between database column values ({@code }) and Java field values ({@code }).
* A good citizen {@code ValueConverter} must:
*
* - Have a no-args public constructor that does not perform any CPU- or I/O-intensive operations.
* - Be thread-safe and reentrant. {@code ValueConverter} might be created and called from any thread:
* it is possible for {@code toColumn()} method to be called while the same instance's {@code toJava()}
* method is running in a different thread, and vice versa. It it therefore highly
* recommended for the conversion to be a pure function.
* - Be effectively stateless: changes to the internal state of the {@code ValueConverter} must not affect
* the result of the conversions.
* - Never acquire scarce or heavy system resources, because YOJ might create a {@code ValueConverter}
* at any time, and there is no way to dispose of a created {@code ValueConverter}.
*
*
* @param Java field value type
* @param Database column value type. Must not be the same type as {@code }. Must be {@link Comparable}.
*/
@ExperimentalApi(issue = "https://github.com/ydb-platform/yoj-project/issues/24")
public interface ValueConverter> {
/**
* Converts a field value to a {@link tech.ydb.yoj.databind.FieldValueType database column value} supported by YOJ.
*
* @param field schema field
* @param v field value, guaranteed to not be {@code null}
* @return database column value corresponding to the Java field value, must not be {@code null}
*
* @see #toJava(JavaField, Comparable)
*/
@NonNull
C toColumn(@NonNull JavaField field, @NonNull J v);
/**
* Converts a database column value to a Java field value.
*
* @param field schema field
* @param c database column value, guaranteed to not be {@code null}
* @return Java field value corresponding to the database column value, must not be {@code null}
*
* @see #toColumn(JavaField, Object)
*/
@NonNull
J toJava(@NonNull JavaField field, @NonNull C c);
/**
* Represents "no custom converter is defined" for {@link tech.ydb.yoj.databind.CustomValueType @CustomValueType}
* annotation inside a {@link tech.ydb.yoj.databind.schema.Column @Column} annotation.
* Non-instantiable, every method including the constructor throws {@link UnsupportedOperationException}.
*/
final class NoConverter implements ValueConverter {
private NoConverter() {
throw new UnsupportedOperationException("Not instantiable");
}
@Override
public @NonNull Boolean toColumn(@NonNull JavaField field, @NonNull Void v) {
throw new UnsupportedOperationException();
}
@Override
public @NonNull Void toJava(@NonNull JavaField field, @NonNull Boolean unused) {
throw new UnsupportedOperationException();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy