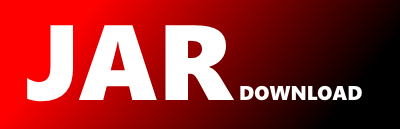
tech.ydb.yoj.databind.expression.values.TimestampFieldValue Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yoj-databind Show documentation
Show all versions of yoj-databind Show documentation
Core data-binding logic used by YOJ (YDB ORM for Java) to convert
between Java objects and database rows (or anything representable by
a Java Map, really).
The newest version!
package tech.ydb.yoj.databind.expression.values;
import lombok.NonNull;
import tech.ydb.yoj.databind.FieldValueType;
import tech.ydb.yoj.databind.expression.IllegalExpressionException.FieldTypeError.TimestampFieldExpected;
import tech.ydb.yoj.databind.expression.IllegalExpressionException.FieldTypeError.TimestampToIntegerInexact;
import java.lang.reflect.Type;
import java.time.Instant;
import java.util.Optional;
import static java.lang.String.format;
import static tech.ydb.yoj.databind.expression.values.FieldValue.ValidationResult.invalidFieldValue;
import static tech.ydb.yoj.databind.expression.values.FieldValue.ValidationResult.validFieldValue;
public record TimestampFieldValue(@NonNull Instant timestamp) implements FieldValue {
@Override
public Optional> getComparableByType(Type fieldType, FieldValueType valueType) {
return switch (valueType) {
case TIMESTAMP -> Optional.of(timestamp);
case INTEGER -> Optional.of(timestamp.toEpochMilli());
default -> Optional.empty();
};
}
@Override
public ValidationResult isValidValueOfType(Type fieldType, FieldValueType valueType) {
return switch (valueType) {
case TIMESTAMP -> validFieldValue();
case INTEGER -> isValidInteger()
? validFieldValue()
: invalidFieldValue(TimestampToIntegerInexact::new, p -> format("Timestamp value is too large for integer field \"%s\"", p));
default -> invalidFieldValue(TimestampFieldExpected::new, p -> format("Specified a timestamp value for non-timestamp field \"%s\"", p));
};
}
private boolean isValidInteger() {
try {
long ignore = timestamp.toEpochMilli();
return true;
} catch (ArithmeticException e) {
return false;
}
}
@Override
public String toString() {
return "#" + timestamp + "#";
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy