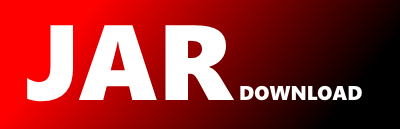
tech.ydb.yoj.databind.expression.visitor.RemoveIf Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of yoj-databind Show documentation
Show all versions of yoj-databind Show documentation
Core data-binding logic used by YOJ (YDB ORM for Java) to convert
between Java objects and database rows (or anything representable by
a Java Map, really).
package tech.ydb.yoj.databind.expression.visitor;
import lombok.NonNull;
import lombok.RequiredArgsConstructor;
import tech.ydb.yoj.databind.expression.FilterExpression;
import tech.ydb.yoj.databind.expression.LeafExpression;
import java.util.List;
import java.util.Objects;
import java.util.function.Predicate;
import static java.util.stream.Collectors.toList;
import static tech.ydb.yoj.databind.expression.FilterBuilder.and;
import static tech.ydb.yoj.databind.expression.FilterBuilder.not;
import static tech.ydb.yoj.databind.expression.FilterBuilder.or;
@RequiredArgsConstructor
public final class RemoveIf extends FilterExpression.Visitor.Simple> {
@NonNull
private final Predicate> predicate;
@Override
protected FilterExpression visitLeaf(@NonNull LeafExpression leaf) {
return predicate.test(leaf) ? null : leaf;
}
@Override
protected FilterExpression visitComposite(@NonNull FilterExpression composite) {
List> filtered = composite.stream()
.map(e -> e.visit(this))
.filter(Objects::nonNull)
.collect(toList());
if (filtered.isEmpty()) {
return null;
}
return switch (composite.getType()) {
case OR -> or(filtered);
case AND -> and(filtered);
case NOT -> not(filtered.get(0));
default -> throw new UnsupportedOperationException("Unknown composite expression:" + composite);
};
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy