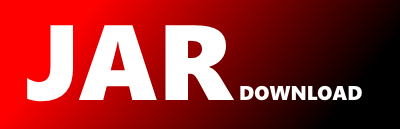
tech.ytsaurus.ysontree.YTreeMapNodeImpl Maven / Gradle / Ivy
package tech.ytsaurus.ysontree;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import java.util.Optional;
import java.util.Set;
import javax.annotation.Nullable;
public class YTreeMapNodeImpl extends YTreeNodeImpl implements YTreeMapNode {
private final Map map = new HashMap<>();
public YTreeMapNodeImpl(Map data, @Nullable Map attributes) {
super(attributes);
map.putAll(data);
}
public YTreeMapNodeImpl(@Nullable Map attributes) {
this(Collections.emptyMap(), attributes);
}
@Override
public Map asMap() {
return map;
}
@Override
public boolean containsKey(String key) {
return map.containsKey(key);
}
@Override
public void clear() {
map.clear();
}
@Override
public Optional remove(String key) {
return Optional.ofNullable(map.remove(key));
}
@Override
public Optional put(String key, YTreeNode value) {
return Optional.ofNullable(map.put(key, value));
}
@Override
public void putAll(Map extends String, ? extends YTreeNode> data) {
map.putAll(data);
}
@Override
public Iterator> iterator() {
return map.entrySet().iterator();
}
@Override
public int size() {
return map.size();
}
@Override
public Set keys() {
return map.keySet();
}
@Override
public Collection values() {
return map.values();
}
@Override
public Optional get(String key) {
return Optional.ofNullable(map.get(key));
}
@Override
public int hashCode() {
return hashCodeBase() * 4243 + map.hashCode();
}
@Override
public boolean equals(Object another) {
if (this == another) {
return true;
}
if (another == null || !(another instanceof YTreeMapNode)) {
return false;
}
YTreeMapNode node = (YTreeMapNode) another;
return map.equals(node.asMap()) && equalsBase(node);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy