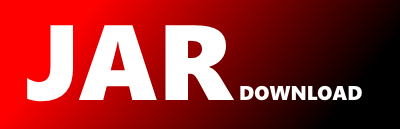
tech.ytsaurus.client.TableAttachmentReader Maven / Gradle / Ivy
package tech.ytsaurus.client;
import java.nio.ByteBuffer;
import java.util.Arrays;
import java.util.List;
import javax.annotation.Nullable;
import NYT.NChunkClient.NProto.DataStatistics.TDataStatistics;
import com.google.protobuf.Message;
import tech.ytsaurus.client.rows.EntitySkiffSerializer;
import tech.ytsaurus.client.rows.WireRowDeserializer;
import tech.ytsaurus.core.tables.TableSchema;
import tech.ytsaurus.ysontree.YTreeNode;
public interface TableAttachmentReader {
@Nullable
List parse(@Nullable byte[] attachments) throws Exception;
@Nullable
List parse(@Nullable byte[] attachments, int offset, int length) throws Exception;
long getTotalRowCount();
@Nullable
TDataStatistics getDataStatistics();
@Nullable
TableSchema getCurrentReadSchema();
/**
* @deprecated don't use it explicitly
*/
@Deprecated
static TableAttachmentReader wireProtocol(WireRowDeserializer deserializer) {
return new TableAttachmentWireProtocolReader<>(deserializer);
}
static TableAttachmentReader byPass() {
return new TableAttachmentByPassReader();
}
static TableAttachmentReader byteBuffer() {
return new TableAttachmentByteBufferReader();
}
static TableAttachmentReader ysonBinary() {
return new TableAttachmentYsonReader();
}
static TableAttachmentReader skiff(EntitySkiffSerializer serializer) {
return new TableAttachmentSkiffReader<>(serializer);
}
static TableAttachmentReader protobuf(Message.Builder messageBuilder) {
return new TableAttachmentProtobufReader<>(messageBuilder);
}
}
class TableAttachmentByPassReader implements TableAttachmentReader {
@Override
public List parse(@Nullable byte[] attachments) {
if (attachments == null) {
return null;
} else {
return Arrays.asList(attachments);
}
}
@Override
public List parse(@Nullable byte[] attachments, int offset, int length) {
if (attachments == null) {
return null;
} else {
if (offset == 0 && length == attachments.length) {
return List.of(attachments);
} else {
return List.of(Arrays.copyOfRange(attachments, offset, length));
}
}
}
@Override
public long getTotalRowCount() {
return 0;
}
@Override
public TDataStatistics getDataStatistics() {
return null;
}
@Nullable
@Override
public TableSchema getCurrentReadSchema() {
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy