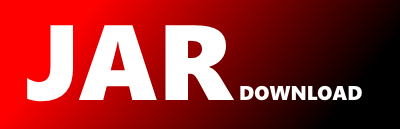
tech.ytsaurus.client.request.ModifyRowsRequest Maven / Gradle / Ivy
package tech.ytsaurus.client.request;
import java.util.AbstractList;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import javax.annotation.Nullable;
import tech.ytsaurus.client.ApiServiceUtil;
import tech.ytsaurus.client.SerializationResolver;
import tech.ytsaurus.client.rows.UnversionedRow;
import tech.ytsaurus.client.rows.UnversionedRowSerializer;
import tech.ytsaurus.client.rows.UnversionedValue;
import tech.ytsaurus.client.rows.WireProtocolWriter;
import tech.ytsaurus.core.tables.TableSchema;
import tech.ytsaurus.rpcproxy.ERowModificationType;
/**
* Row modification request that uses {@link UnversionedRow} as table row representation
*
* @see UnversionedRow
*/
public class ModifyRowsRequest extends PreparableModifyRowsRequest {
private final List rows;
private final List unconvertedRows;
public ModifyRowsRequest(BuilderBase> builder) {
super(builder);
this.rows = new ArrayList<>(builder.rows);
this.unconvertedRows = new ArrayList<>(builder.unconvertedRows);
}
public ModifyRowsRequest(String path, TableSchema schema) {
this(builder().setPath(path).setSchema(schema));
}
public static Builder builder() {
return new Builder();
}
public List getRows() {
return Collections.unmodifiableList(rows);
}
@Override
public void convertValues(SerializationResolver serializationResolver) {
for (BuilderBase.RowMeta meta : unconvertedRows) {
List> values;
switch (meta.type) {
case INSERT: {
values = meta.values != null
? meta.values
: mapToValues(Objects.requireNonNull(meta.map), schema.getColumnsCount());
if (values.size() != schema.getColumns().size()) {
throw new IllegalArgumentException(
"Number of insert columns must match number of schema columns");
}
break;
}
case UPDATE: {
values = meta.values != null
? meta.values
: mapToValues(Objects.requireNonNull(meta.map), schema.getColumnsCount());
if (values.size() <= schema.getKeyColumnsCount()
|| values.size() > schema.getColumns().size()) {
throw new IllegalArgumentException(
"Number of update columns must be more than the number of key columns");
}
break;
}
case DELETE: {
values = meta.values != null
? meta.values
: mapToValues(Objects.requireNonNull(meta.map), schema.getKeyColumnsCount());
if (values.size() != schema.getKeyColumnsCount()) {
throw new IllegalArgumentException(
"Number of delete columns must match number of key columns");
}
break;
}
default: {
throw new IllegalArgumentException("unknown modification type");
}
}
rows.add(convertValuesToRow(values, meta.skipMissingValues, meta.aggregate, serializationResolver));
}
this.unconvertedRows.clear();
}
private UnversionedRow convertValuesToRow(
List> values, boolean skipMissingValues, boolean aggregate, SerializationResolver serializationResolver) {
if (values.size() < schema.getKeyColumnsCount()) {
throw new IllegalArgumentException(
"Number of values must be more than or equal to the number of key columns");
}
List row = new ArrayList<>(values.size());
ApiServiceUtil.convertKeyColumns(row, schema, values, serializationResolver);
ApiServiceUtil.convertValueColumns(row, schema, values, skipMissingValues, aggregate, serializationResolver);
return new UnversionedRow(row);
}
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy