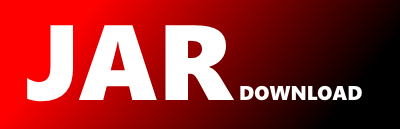
tofu.bi.BiContext.scala Maven / Gradle / Ivy
Go to download
Kiwi is a utility library. We really like Google's Guava, and also use Apache Commons.
But if they don't have something we need, and we think it is useful, this is where we put it.
Please wait ...