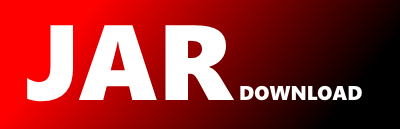
tk.hongkailiu.test.app.tree.BinaryTree Maven / Gradle / Ivy
The newest version!
package tk.hongkailiu.test.app.tree;
import java.util.ArrayList;
import java.util.LinkedList;
import java.util.List;
public class BinaryTree extends Tree {
private BinaryTree left;
private BinaryTree right;
public BinaryTree(E value) {
super(value);
if (this.children == null) {
this.children = new ArrayList>(2);
}
this.children.add(null);
this.children.add(null);
validate();
}
public BinaryTree(E value, BinaryTree left, BinaryTree right) {
super(value);
this.left = left;
this.right = right;
if (this.children == null) {
this.children = new ArrayList>(2);
}
this.children.add(left);
this.children.add(right);
validate();
}
@Override public List> getChildren() {
throw new IllegalArgumentException("not supported method: use getters of left and right");
}
@Override public void setChildren(List> children) {
throw new IllegalArgumentException("not supported method: use setters of left and right");
}
public BinaryTree getLeft() {
return left;
}
public void setLeft(BinaryTree left) {
this.left = left;
this.children.clear();
this.children.add(left);
this.children.add(right);
validate();
}
public BinaryTree getRight() {
return right;
}
public void setRight(BinaryTree right) {
this.right = right;
this.children.clear();
this.children.add(left);
this.children.add(right);
validate();
}
protected void validate() {
if (this.children != null) {
if (this.children.size() != 2) {
throw new IllegalArgumentException("exact 2 children in binary tree");
}
if (this.left != null) {
left.validate();
}
if (this.right != null) {
right.validate();
}
}
}
public E getValue() {
return value;
}
public void setValue(E value) {
this.value = value;
}
public List depthFirstTraversalInOrder() {
List list = new LinkedList();
if (left != null) {
list.addAll(left.depthFirstTraversalInOrder());
}
list.add(value);
if (right != null) {
list.addAll(right.depthFirstTraversalInOrder());
}
return list;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy