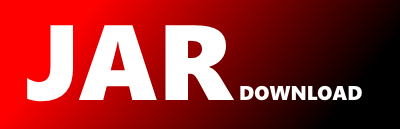
tk.labyrinth.misc4j.collectoin.CollectorUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jaap Show documentation
Show all versions of jaap Show documentation
Java Advanced Annotation Processing
package tk.labyrinth.misc4j.collectoin;
import java.util.ArrayList;
import java.util.List;
import java.util.stream.Collector;
import java.util.stream.Collectors;
public class CollectorUtils {
/**
* @param Element
*
* @return non-null
*/
public static Collector findOnly() {
return findOnly(false, false);
}
/**
* @param allowEmpty whether to fail or not if Stream is empty
* @param Element
*
* @return non-null
*/
public static Collector findOnly(boolean allowEmpty) {
return findOnly(allowEmpty, false);
}
/**
* @param allowEmpty whether to fail or not if Stream is empty
* @param allowNull whether to fail or not if only Element is null
* @param Element
*
* @return non-null
*/
public static Collector findOnly(boolean allowEmpty, boolean allowNull) {
return Collectors.collectingAndThen(Collectors.toList(), list -> {
if (list.size() > 1) {
throw new IllegalArgumentException("Require only element: " + list);
}
E result;
if (!list.isEmpty()) {
result = list.get(0);
if (result == null && !allowNull) {
throw new IllegalArgumentException("Require non-null element: " + list);
}
} else {
if (!allowEmpty) {
throw new IllegalArgumentException("Require non-empty");
} else {
result = null;
}
}
return result;
});
}
/**
* Unlike {@link Collectors#toList()} this Collector guarantees to use {@link ArrayList}.
*
* @param Element
*
* @return non-null
*/
public static Collector> toArrayList() {
return Collectors.toCollection(ArrayList::new);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy