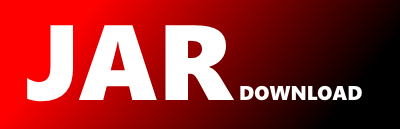
cvc5-cvc5-1.2.0.include.cvc5.cvc5_kind.h Maven / Gradle / Ivy
The newest version!
/******************************************************************************
* Top contributors (to current version):
* Aina Niemetz, Gereon Kremer, Andrew Reynolds
*
* This file is part of the cvc5 project.
*
* Copyright (c) 2009-2024 by the authors listed in the file AUTHORS
* in the top-level source directory and their institutional affiliations.
* All rights reserved. See the file COPYING in the top-level source
* directory for licensing information.
* ****************************************************************************
*
* The term kinds of the cvc5 C++ and C APIs.
*/
#if (!defined(CVC5_API_USE_C_ENUMS) && !defined(CVC5__API__CVC5_CPP_KIND_H)) \
|| (defined(CVC5_API_USE_C_ENUMS) && !defined(CVC5__API__CVC5_C_KIND_H))
#ifdef CVC5_API_USE_C_ENUMS
#include
#include
#undef ENUM
#define ENUM(name) Cvc5##name
#else
#include
#include
#include
namespace cvc5 {
#undef ENUM
#define ENUM(name) class name
#undef EVALUE
#define EVALUE(name) name
#endif
/* -------------------------------------------------------------------------- */
/* Kind */
/* -------------------------------------------------------------------------- */
#ifdef CVC5_API_USE_C_ENUMS
#undef EVALUE
#define EVALUE(name) CVC5_KIND_##name
#endif
// clang-format off
/**
* The kind of a cvc5 Term.
*
* \internal
*
* Note that the API type `cvc5::Kind` roughly corresponds to
* `cvc5::internal::Kind`, but is a different type. It hides internal kinds
* that should not be exported to the API, and maps all kinds that we want to
* export to its corresponding internal kinds. The underlying type of
* `cvc5::Kind` must be signed (to enable range checks for validity). The size
* of this type depends on the size of `cvc5::internal::Kind`
* (`NodeValue::NBITS_KIND`, currently 10 bits, see expr/node_value.h).
*/
enum ENUM(Kind)
{
/**
* Internal kind.
*
* This kind serves as an abstraction for internal kinds that are not exposed
* via the API but may appear in terms returned by API functions, e.g.,
* when querying the simplified form of a term.
*
* \rst
* .. note:: Should never be created via the API.
* \endrst
*/
EVALUE(INTERNAL_KIND = -2),
/**
* Undefined kind.
*
* \rst
* .. note:: Should never be exposed or created via the API.
* \endrst
*/
EVALUE(UNDEFINED_KIND = -1),
/**
* Null kind.
*
* The kind of a null term (Term::Term()).
*
* \rst
* .. note:: May not be explicitly created via API functions other than
* :cpp:func:`Term::Term()`.
* \endrst
*/
EVALUE(NULL_TERM),
/* Builtin --------------------------------------------------------------- */
/**
* The value of an uninterpreted constant.
*
* \rst
* .. note:: May be returned as the result of an API call, but terms of this
* kind may not be created explicitly via the API and may not
* appear in assertions.
* \endrst
*/
EVALUE(UNINTERPRETED_SORT_VALUE),
/**
* Equality, chainable.
*
* - Arity: ``n > 1``
*
* - ``1..n:`` Terms of the same Sort
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(EQUAL),
/**
* Disequality.
*
* - Arity: ``n > 1``
*
* - ``1..n:`` Terms of the same Sort
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(DISTINCT),
/**
* Free constant symbol.
*
* - Create Term of this Kind with:
*
* - Solver::mkConst(const Sort&, const std::string&) const
* - Solver::mkConst(const Sort&) const
*
* \rst
* .. note:: Not permitted in bindings (e.g., :cpp:enumerator:`FORALL`,
* :cpp:enumerator:`EXISTS`).
* \endrst
*/
EVALUE(CONSTANT),
/**
* (Bound) variable.
*
* - Create Term of this Kind with:
*
* - Solver::mkVar(const Sort&, const std::string&) const
*
* \rst
* .. note:: Only permitted in bindings and in lambda and quantifier bodies.
* \endrst
*/
EVALUE(VARIABLE),
/**
* A Skolem.
*
* \rst
* .. note:: Represents an internally generated term. Information on the
* skolem is available via the calls `Solver::getSkolemId` and
* `Solver::getSkolemIndices`.
* \endrst
*/
EVALUE(SKOLEM),
/**
* Symbolic expression.
*
* - Arity: ``n > 0``
*
* - ``1..n:`` Terms with same sorts
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*
* \rst
* .. warning:: This kind is experimental and may be changed or removed in
* future versions.
* \endrst
*/
EVALUE(SEXPR),
/**
* Lambda expression.
*
* - Arity: ``2``
*
* - ``1:`` Term of kind :cpp:enumerator:`VARIABLE_LIST`
* - ``2:`` Term of any Sort (the body of the lambda)
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(LAMBDA),
/**
* Witness.
*
* The syntax of a witness term is similar to a quantified formula except that
* only one variable is allowed.
* For example, the term
* \rst
* .. code:: smtlib
*
* (witness ((x S)) F)
*
* returns an element :math:`x` of Sort :math:`S` and asserts formula
* :math:`F`.
*
* The witness operator behaves like the description operator
* (see https://planetmath.org/hilbertsvarepsilonoperator) if there is
* no :math:`x` that satisfies :math:`F`. But if such :math:`x` exists, the
* witness operator does not enforce the following axiom which ensures
* uniqueness up to logical equivalence:
*
* .. math::
*
* \forall x. F \equiv G \Rightarrow witness~x. F = witness~x. G
*
* For example, if there are two elements of Sort :math:`S` that satisfy
* formula :math:`F`, then the following formula is satisfiable:
*
* .. code:: smtlib
*
* (distinct
* (witness ((x Int)) F)
* (witness ((x Int)) F))
*
* \endrst
*
* - Arity: ``3``
*
* - ``1:`` Term of kind :cpp:enumerator:`VARIABLE_LIST`
* - ``2:`` Term of Sort Bool (the body of the witness)
* - ``3:`` (optional) Term of kind :cpp:enumerator:`INST_PATTERN_LIST`
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*
* \rst
* .. note::
*
* This kind is primarily used internally, but may be returned in
* models (e.g., for arithmetic terms in non-linear queries). However,
* it is not supported by the parser. Moreover, the user of the API
* should be cautious when using this operator. In general, all witness
* terms ``(witness ((x Int)) F)`` should be such that ``(exists ((x Int))
* F)`` is a valid formula. If this is not the case, then the semantics
* in formulas that use witness terms may be unintuitive. For example,
* the following formula is unsatisfiable:
* ``(or (= (witness ((x Int)) false) 0) (not (= (witness ((x Int))
* false) 0))``, whereas notice that ``(or (= z 0) (not (= z 0)))`` is
* true for any :math:`z`.
* \endrst
*/
EVALUE(WITNESS),
/* Boolean --------------------------------------------------------------- */
/**
* Boolean constant.
*
* - Create Term of this Kind with:
*
* - Solver::mkTrue() const
* - Solver::mkFalse() const
* - Solver::mkBoolean(bool) const
*/
EVALUE(CONST_BOOLEAN),
/**
* Logical negation.
*
* - Arity: ``1``
*
* - ``1:`` Term of Sort Bool
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(NOT),
/**
* Logical conjunction.
*
* - Arity: ``n > 1``
*
* - ``1..n:`` Terms of Sort Bool
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(AND),
/**
* Logical implication.
*
* - Arity: ``n > 1``
*
* - ``1..n:`` Terms of Sort Bool
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(IMPLIES),
/**
* Logical disjunction.
*
* - Arity: ``n > 1``
*
* - ``1..n:`` Terms of Sort Bool
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(OR),
/**
* Logical exclusive disjunction, left associative.
*
* - Arity: ``n > 1``
*
* - ``1..n:`` Terms of Sort Bool
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(XOR),
/**
* If-then-else.
*
* - Arity: ``3``
*
* - ``1:`` Term of Sort Bool
* - ``2:`` The 'then' term, Term of any Sort
* - ``3:`` The 'else' term, Term of the same sort as second argument
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(ITE),
/* UF -------------------------------------------------------------------- */
/**
* Application of an uninterpreted function.
*
* - Arity: ``n > 1``
*
* - ``1:`` Function Term
* - ``2..n:`` Function argument instantiation Terms of any first-class Sort
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(APPLY_UF),
/**
* Cardinality constraint on uninterpreted sort.
*
* \rst
* Interpreted as a predicate that is true when the cardinality of
* uinterpreted Sort :math:`S` is less than or equal to an upper bound.
* \endrst
*
* - Create Term of this Kind with:
*
* - Solver::mkCardinalityConstraint(const Sort&, uint32_t) const
*
* \rst
* .. warning:: This kind is experimental and may be changed or removed in
* future versions.
* \endrst
*/
EVALUE(CARDINALITY_CONSTRAINT),
/**
* Higher-order applicative encoding of function application, left
* associative.
*
* - Arity: ``n = 2``
*
* - ``1:`` Function Term
* - ``2:`` Argument Term of the domain Sort of the function
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(HO_APPLY),
/* Arithmetic ------------------------------------------------------------ */
/**
* Arithmetic addition.
*
* - Arity: ``n > 1``
*
* - ``1..n:`` Terms of Sort Int or Real (sorts must match)
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(ADD),
/**
* Arithmetic multiplication.
*
* - Arity: ``n > 1``
*
* - ``1..n:`` Terms of Sort Int or Real (sorts must match)
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(MULT),
/**
* Integer and.
*
* \rst
* Operator for bit-wise ``AND`` over integers, parameterized by a (positive)
* bit-width :math:`k`.
*
* .. code:: smtlib
*
* ((_ iand k) i_1 i_2)
*
* is equivalent to
*
* .. code:: smtlib
*
* ((_ iand k) i_1 i_2)
* (bv2int (bvand ((_ int2bv k) i_1) ((_ int2bv k) i_2)))
*
* for all integers ``i_1``, ``i_2``.
*
* - Arity: ``2``
*
* - ``1..2:`` Terms of Sort Int
*
* - Indices: ``1``
*
* - ``1:`` Bit-width :math:`k`
* \endrst
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(IAND),
/**
* Power of two.
*
* Operator for raising ``2`` to a non-negative integer power.
*
* - Arity: ``1``
*
* - ``1:`` Term of Sort Int
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(POW2),
/**
* Arithmetic subtraction, left associative.
*
* - Arity: ``n > 1``
*
* - ``1..n:`` Terms of Sort Int or Real (sorts must match)
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(SUB),
/**
* Arithmetic negation.
*
* - Arity: ``1``
*
* - ``1:`` Term of Sort Int or Real
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(NEG),
/**
* Real division, division by 0 undefined, left associative.
*
* - Arity: ``n > 1``
*
* - ``1..n:`` Terms of Sort Real
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(DIVISION),
/**
* Real division, division by 0 defined to be 0, left associative.
*
* - Arity: ``n > 1``
*
* - ``1..n:`` Terms of Sort Real
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*
* \rst
* .. warning:: This kind is experimental and may be changed or removed in
* future versions.
* \endrst
*/
EVALUE(DIVISION_TOTAL),
/**
* Integer division, division by 0 undefined, left associative.
*
* - Arity: ``n > 1``
*
* - ``1..n:`` Terms of Sort Int
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(INTS_DIVISION),
/**
* Integer division, division by 0 defined to be 0, left associative.
*
* - Arity: ``n > 1``
*
* - ``1..n:`` Terms of Sort Int
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*
* \rst
* .. warning:: This kind is experimental and may be changed or removed in
* future versions.
* \endrst
*/
EVALUE(INTS_DIVISION_TOTAL),
/**
* Integer modulus, modulus by 0 undefined.
*
* - Arity: ``2``
*
* - ``1:`` Term of Sort Int
* - ``2:`` Term of Sort Int
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(INTS_MODULUS),
/**
* Integer modulus, t modulus by 0 defined to be t.
*
* - Arity: ``2``
*
* - ``1:`` Term of Sort Int
* - ``2:`` Term of Sort Int
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*
* \rst
* .. warning:: This kind is experimental and may be changed or removed in
* future versions.
* \endrst
*/
EVALUE(INTS_MODULUS_TOTAL),
/**
* Absolute value.
*
* - Arity: ``1``
*
* - ``1:`` Term of Sort Int or Real
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(ABS),
/**
* Arithmetic power.
*
* - Arity: ``2``
*
* - ``1..2:`` Term of Sort Int or Real (sorts must match)
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(POW),
/**
* Exponential function.
*
* - Arity: ``1``
*
* - ``1:`` Term of Sort Real
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(EXPONENTIAL),
/**
* Sine function.
*
* - Arity: ``1``
*
* - ``1:`` Term of Sort Real
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(SINE),
/**
* Cosine function.
*
* - Arity: ``1``
*
* - ``1:`` Term of Sort Real
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(COSINE),
/**
* Tangent function.
*
* - Arity: ``1``
*
* - ``1:`` Term of Sort Real
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(TANGENT),
/**
* Cosecant function.
*
* - Arity: ``1``
*
* - ``1:`` Term of Sort Real
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(COSECANT),
/**
* Secant function.
*
* - Arity: ``1``
*
* - ``1:`` Term of Sort Real
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(SECANT),
/**
* Cotangent function.
*
* - Arity: ``1``
*
* - ``1:`` Term of Sort Real
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(COTANGENT),
/**
* Arc sine function.
*
* - Arity: ``1``
*
* - ``1:`` Term of Sort Real
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(ARCSINE),
/**
* Arc cosine function.
*
* - Arity: ``1``
*
* - ``1:`` Term of Sort Real
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(ARCCOSINE),
/**
* Arc tangent function.
*
* - Arity: ``1``
*
* - ``1:`` Term of Sort Real
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(ARCTANGENT),
/**
* Arc cosecant function.
*
* - Arity: ``1``
*
* - ``1:`` Term of Sort Real
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(ARCCOSECANT),
/**
* Arc secant function.
*
* - Arity: ``1``
*
* - ``1:`` Term of Sort Real
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(ARCSECANT),
/**
* Arc cotangent function.
*
* - Arity: ``1``
*
* - ``1:`` Term of Sort Real
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(ARCCOTANGENT),
/**
* Square root.
*
* If the argument `x` is non-negative, then this returns a non-negative value
* `y` such that `y * y = x`.
*
* - Arity: ``1``
*
* - ``1:`` Term of Sort Real
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(SQRT),
/**
* \rst
* Operator for the divisibility-by-:math:`k` predicate.
*
* - Arity: ``1``
*
* - ``1:`` Term of Sort Int
*
* - Indices: ``1``
*
* - ``1:`` The integer :math:`k` to divide by.
* \endrst
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(DIVISIBLE),
/**
* Arbitrary-precision rational constant.
*
* - Create Term of this Kind with:
*
* - Solver::mkReal(const std::string&) const
* - Solver::mkReal(int64_t) const
* - Solver::mkReal(int64_t, int64_t) const
*/
EVALUE(CONST_RATIONAL),
/**
* Arbitrary-precision integer constant.
*
* - Create Term of this Kind with:
*
* - Solver::mkInteger(const std::string&) const
* - Solver::mkInteger(int64_t) const
*/
EVALUE(CONST_INTEGER),
/**
* Less than, chainable.
*
* - Arity: ``n > 1``
*
* - ``1..n:`` Terms of Sort Int or Real (sorts must match)
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(LT),
/**
* Less than or equal, chainable.
*
* - Arity: ``n > 1``
*
* - ``1..n:`` Terms of Sort Int or Real (sorts must match)
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(LEQ),
/**
* Greater than, chainable.
*
* - Arity: ``n > 1``
*
* - ``1..n:`` Terms of Sort Int or Real (sorts must match)
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(GT),
/**
* Greater than or equal, chainable.
*
* - Arity: ``n > 1``
*
* - ``1..n:`` Terms of Sort Int or Real (sorts must match)
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(GEQ),
/**
* Is-integer predicate.
*
* - Arity: ``1``
*
* - ``1:`` Term of Sort Int or Real
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(IS_INTEGER),
/**
* Convert Term of sort Int or Real to Int via the floor function.
*
* - Arity: ``1``
*
* - ``1:`` Term of Sort Int or Real
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(TO_INTEGER),
/**
* Convert Term of Sort Int or Real to Real.
*
* - Arity: ``1``
*
* - ``1:`` Term of Sort Int or Real
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(TO_REAL),
/**
* Pi constant.
*
* - Create Term of this Kind with:
*
* - Solver::mkPi() const
*
* \rst
* .. note:: :cpp:enumerator:`PI` is considered a special symbol of Sort
* Real, but is not a Real value, i.e.,
* :cpp:func:`Term::isRealValue()` will return ``false``.
* \endrst
*/
EVALUE(PI),
/* BV -------------------------------------------------------------------- */
/**
* Fixed-size bit-vector constant.
*
* - Create Term of this Kind with:
*
* - Solver::mkBitVector(uint32_t, uint64_t) const
* - Solver::mkBitVector(uint32_t, const std::string&, uint32_t) const
*/
EVALUE(CONST_BITVECTOR),
/**
* Concatenation of two or more bit-vectors.
*
* - Arity: ``n > 1``
*
* - ``1..n:`` Terms of bit-vector Sort (sorts must match)
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(BITVECTOR_CONCAT),
/**
* Bit-wise and.
*
* - Arity: ``n > 1``
*
* - ``1..n:`` Terms of bit-vector Sort (sorts must match)
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(BITVECTOR_AND),
/**
* Bit-wise or.
*
* - Arity: ``n > 1``
*
* - ``1..n:`` Terms of bit-vector Sort (sorts must match)
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(BITVECTOR_OR),
/**
* Bit-wise xor.
*
* - Arity: ``n > 1``
*
* - ``1..n:`` Terms of bit-vector Sort (sorts must match)
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(BITVECTOR_XOR),
/**
* Bit-wise negation.
*
* - Arity: ``1``
*
* - ``1:`` Term of bit-vector Sort
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(BITVECTOR_NOT),
/**
* Bit-wise nand.
*
* - Arity: ``2``
*
* - ``1..2:`` Terms of bit-vector Sort (sorts must match)
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(BITVECTOR_NAND),
/**
* Bit-wise nor.
*
* - Arity: ``2``
*
* - ``1..2:`` Terms of bit-vector Sort (sorts must match)
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(BITVECTOR_NOR),
/**
* Bit-wise xnor, left associative.
*
* - Arity: ``2``
*
* - ``1..2:`` Terms of bit-vector Sort (sorts must match)
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(BITVECTOR_XNOR),
/**
* Equality comparison (returns bit-vector of size ``1``).
*
* - Arity: ``2``
*
* - ``1..2:`` Terms of bit-vector Sort (sorts must match)
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(BITVECTOR_COMP),
/**
* Multiplication of two or more bit-vectors.
*
* - Arity: ``n > 1``
*
* - ``1..n:`` Terms of bit-vector Sort (sorts must match)
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(BITVECTOR_MULT),
/**
* Addition of two or more bit-vectors.
*
* - Arity: ``n > 1``
*
* - ``1..n:`` Terms of bit-vector Sort (sorts must match)
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(BITVECTOR_ADD),
/**
* Subtraction of two bit-vectors.
*
* - Arity: ``n > 1``
*
* - ``1..n:`` Terms of bit-vector Sort (sorts must match)
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(BITVECTOR_SUB),
/**
* Negation of a bit-vector (two's complement).
*
* - Arity: ``1``
*
* - ``1:`` Term of bit-vector Sort
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(BITVECTOR_NEG),
/**
* Unsigned bit-vector division.
*
* Truncates towards ``0``. If the divisor is zero, the result is all ones.
*
* - Arity: ``2``
*
* - ``1..2:`` Terms of bit-vector Sort (sorts must match)
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(BITVECTOR_UDIV),
/**
* Unsigned bit-vector remainder.
*
* Remainder from unsigned bit-vector division. If the modulus is zero, the
* result is the dividend.
*
* - Arity: ``2``
*
* - ``1..2:`` Terms of bit-vector Sort (sorts must match)
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(BITVECTOR_UREM),
/**
* Signed bit-vector division.
*
* Two's complement signed division of two bit-vectors. If the divisor is
* zero and the dividend is positive, the result is all ones. If the divisor
* is zero and the dividend is negative, the result is one.
*
* - Arity: ``2``
*
* - ``1..2:`` Terms of bit-vector Sort (sorts must match)
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(BITVECTOR_SDIV),
/**
* Signed bit-vector remainder (sign follows dividend).
*
* Two's complement signed remainder of two bit-vectors where the sign
* follows the dividend. If the modulus is zero, the result is the dividend.
*
* - Arity: ``2``
*
* - ``1..2:`` Terms of bit-vector Sort (sorts must match)
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(BITVECTOR_SREM),
/**
* Signed bit-vector remainder (sign follows divisor).
*
* Two's complement signed remainder where the sign follows the divisor. If
* the modulus is zero, the result is the dividend.
*
* - Arity: ``2``
*
* - ``1..2:`` Terms of bit-vector Sort (sorts must match)
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(BITVECTOR_SMOD),
/**
* Bit-vector shift left.
*
* - Arity: ``2``
*
* - ``1..2:`` Terms of bit-vector Sort (sorts must match)
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(BITVECTOR_SHL),
/**
* Bit-vector logical shift right.
*
* - Arity: ``2``
*
* - ``1..2:`` Terms of bit-vector Sort (sorts must match)
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(BITVECTOR_LSHR),
/**
* Bit-vector arithmetic shift right.
*
* - Arity: ``2``
*
* - ``1..2:`` Terms of bit-vector Sort (sorts must match)
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(BITVECTOR_ASHR),
/**
* Bit-vector unsigned less than.
*
* - Arity: ``2``
*
* - ``1..2:`` Terms of bit-vector Sort (sorts must match)
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(BITVECTOR_ULT),
/**
* Bit-vector unsigned less than or equal.
*
* - Arity: ``2``
*
* - ``1..2:`` Terms of bit-vector Sort (sorts must match)
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(BITVECTOR_ULE),
/**
* Bit-vector unsigned greater than.
*
* - Arity: ``2``
*
* - ``1..2:`` Terms of bit-vector Sort (sorts must match)
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(BITVECTOR_UGT),
/**
* Bit-vector unsigned greater than or equal.
*
* - Arity: ``2``
*
* - ``1..2:`` Terms of bit-vector Sort (sorts must match)
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(BITVECTOR_UGE),
/**
* Bit-vector signed less than.
*
* - Arity: ``2``
*
* - ``1..2:`` Terms of bit-vector Sort (sorts must match)
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(BITVECTOR_SLT),
/**
* Bit-vector signed less than or equal.
*
* - Arity: ``2``
*
* - ``1..2:`` Terms of bit-vector Sort (sorts must match)
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(BITVECTOR_SLE),
/**
* Bit-vector signed greater than.
*
* - Arity: ``2``
*
* - ``1..2:`` Terms of bit-vector Sort (sorts must match)
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(BITVECTOR_SGT),
/**
* Bit-vector signed greater than or equal.
*
* - Arity: ``2``
*
* - ``1..2:`` Terms of bit-vector Sort (sorts must match)
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(BITVECTOR_SGE),
/**
* Bit-vector unsigned less than returning a bit-vector of size 1.
*
* - Arity: ``2``
*
* - ``1..2:`` Terms of bit-vector Sort (sorts must match)
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(BITVECTOR_ULTBV),
/**
* Bit-vector signed less than returning a bit-vector of size ``1``.
*
* - Arity: ``2``
*
* - ``1..2:`` Terms of bit-vector Sort (sorts must match)
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(BITVECTOR_SLTBV),
/**
* Bit-vector if-then-else.
*
* Same semantics as regular ITE, but condition is bit-vector of size ``1``.
*
* - Arity: ``3``
*
* - ``1:`` Term of bit-vector Sort of size `1`
* - ``1..3:`` Terms of bit-vector sort (sorts must match)
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(BITVECTOR_ITE),
/**
* Bit-vector redor.
*
* - Arity: ``1``
*
* - ``1:`` Term of bit-vector Sort
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector&) const
*
* - Create Op of this kind with:
*
* - Solver::mkOp(Kind, const std::vector&) const
*/
EVALUE(BITVECTOR_REDOR),
/**
* Bit-vector redand.
*
* - Arity: ``1``
*
* - ``1:`` Term of bit-vector Sort
*
* - Create Term of this Kind with:
*
* - Solver::mkTerm(Kind, const std::vector&) const
* - Solver::mkTerm(const Op&, const std::vector