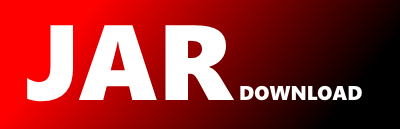
cvc5-cvc5-1.2.0.src.theory.mkrewriter Maven / Gradle / Ivy
The newest version!
#!/usr/bin/env bash
#
# mkrewriter
# Morgan Deters for cvc5
# Copyright (c) 2010-2013 The cvc5 Project
#
# The purpose of this script is to create rewriter_tables.h from a template
# and a list of theory kinds.
#
# Invocation:
#
# mkrewriter template-file theory-kind-files...
#
# Output is to standard out.
#
copyright=2010-2022
cat <&2
exit 1
fi
# this script doesn't care about the theory class information, but
# makes does make sure it's there
seen_theory=true
if [ "$1" = THEORY_BUILTIN ]; then
if $seen_theory_builtin; then
echo "$kf:$lineno: error: \"builtin\" theory redefined" >&2
exit 1
fi
seen_theory_builtin=true
elif [ -z "$1" -o -z "$2" -o -z "$3" ]; then
echo "$kf:$lineno: error: \"theory\" directive missing class or header argument" >&2
exit 1
elif ! expr "$2" : '\(::*\)' >/dev/null; then
echo "$kf:$lineno: warning: theory class \`$2' isn't fully-qualified (e.g., ::cvc5::internal::theory::foo)" >&2
elif ! expr "$2" : '\(::cvc5::internal::theory::*\)' >/dev/null; then
echo "$kf:$lineno: warning: theory class not under ::cvc5::internal::theory namespace" >&2
fi
theory_id="$1"
}
function alternate {
# alternate ID name T header
lineno=${BASH_LINENO[0]}
if $seen_theory; then
echo "$kf:$lineno: error: multiple theories defined in one file !?" >&2
exit 1
fi
seen_theory=true
seen_endtheory=true
}
function properties {
# properties prop*
lineno=${BASH_LINENO[0]}
check_theory_seen
}
function endtheory {
# endtheory
lineno=${BASH_LINENO[0]}
check_theory_seen
seen_endtheory=true
}
function enumerator {
# enumerator KIND enumerator-class header
lineno=${BASH_LINENO[0]}
check_theory_seen
}
function typechecker {
# typechecker header
lineno=${BASH_LINENO[0]}
check_theory_seen
}
function typerule {
# typerule OPERATOR typechecking-class
lineno=${BASH_LINENO[0]}
check_theory_seen
}
function construle {
# construle OPERATOR isconst-checking-class
lineno=${BASH_LINENO[0]}
check_theory_seen
}
function rewriter {
# rewriter class header
class="$1"
header="$2"
rewriter_includes="${rewriter_includes}#include \"$header\"
"
pre_rewrite_attribute_ids="${pre_rewrite_attribute_ids} preids.push_back(expr::attr::AttributeManager::getAttributeId(RewriteAttibute<${theory_id}>::pre_rewrite()));
"
post_rewrite_attribute_ids="${post_rewrite_attribute_ids} postids.push_back(expr::attr::AttributeManager::getAttributeId(RewriteAttibute<${theory_id}>::post_rewrite()));
"
pre_rewrite_get_cache="${pre_rewrite_get_cache} case ${theory_id}: return RewriteAttibute<${theory_id}>::getPreRewriteCache(node);
"
pre_rewrite_set_cache="${pre_rewrite_set_cache} case ${theory_id}: return RewriteAttibute<${theory_id}>::setPreRewriteCache(node, cache);
"
post_rewrite_get_cache="${post_rewrite_get_cache} case ${theory_id}: return RewriteAttibute<${theory_id}>::getPostRewriteCache(node);
"
post_rewrite_set_cache="${post_rewrite_set_cache} case ${theory_id}: return RewriteAttibute<${theory_id}>::setPostRewriteCache(node, cache);
"
lineno=${BASH_LINENO[0]}
check_theory_seen
}
function sort {
# sort TYPE cardinality [well-founded ground-term header | not-well-founded] ["comment"]
lineno=${BASH_LINENO[0]}
check_theory_seen
}
function cardinality {
# cardinality TYPE cardinality-computer [header]
lineno=${BASH_LINENO[0]}
check_theory_seen
}
function well-founded {
# well-founded TYPE wellfoundedness-computer [header]
lineno=${BASH_LINENO[0]}
check_theory_seen
}
function variable {
# variable K ["comment"]
lineno=${BASH_LINENO[0]}
check_theory_seen
}
function operator {
# operator K #children ["comment"]
lineno=${BASH_LINENO[0]}
check_theory_seen
}
function parameterized {
# parameterized K1 K2 #children ["comment"]
lineno=${BASH_LINENO[0]}
check_theory_seen
}
function constant {
# constant K T Hasher header ["comment"]
lineno=${BASH_LINENO[0]}
check_theory_seen
}
function nullaryoperator {
# nullaryoperator K ["comment"]
lineno=${BASH_LINENO[0]}
check_theory_seen
}
function check_theory_seen {
if $seen_endtheory; then
echo "$kf:$lineno: error: command after \"endtheory\" declaration (endtheory has to be last)" >&2
exit 1
fi
if ! $seen_theory; then
echo "$kf:$lineno: error: no \"theory\" declaration found (it has to be first)" >&2
exit 1
fi
}
function check_builtin_theory_seen {
if ! $seen_theory_builtin; then
echo "$me: warning: no declaration for the builtin theory found" >&2
fi
}
while [ $# -gt 0 ]; do
kf=$1
seen_theory=false
seen_endtheory=false
b=$(basename $(dirname "$kf"))
source "$kf"
if ! $seen_theory; then
echo "$kf: error: no theory content found in file!" >&2
exit 1
fi
if ! $seen_endtheory; then
echo "$kf:$lineno: error: no \"endtheory\" declaration found (it is required at the end)" >&2
exit 1
fi
shift
done
check_builtin_theory_seen
## output
text=$(cat "$template")
for var in \
rewriter_includes \
pre_rewrite_get_cache \
post_rewrite_get_cache \
pre_rewrite_set_cache \
post_rewrite_set_cache \
pre_rewrite_attribute_ids \
post_rewrite_attribute_ids \
template \
; do
eval text="\${text//\\\$\\{$var\\}/\${$var}}"
done
error=`expr "$text" : '.*\${\([^}]*\)}.*'`
if [ -n "$error" ]; then
echo "$template:0: error: undefined replacement \${$error}" >&2
exit 1
fi
echo "$text"
© 2015 - 2025 Weber Informatics LLC | Privacy Policy