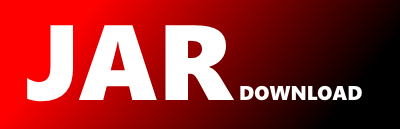
io.github.cvc5.ProofRewriteRule Maven / Gradle / Ivy
Go to download
The WorldWind Kotlin SDK (WWK) includes the library, examples and tutorials for building multiplatform 3D virtual globe applications for Android, Web and Java.
Please wait ...