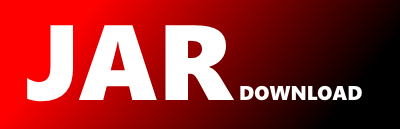
io.github.cvc5.SortKind Maven / Gradle / Ivy
The newest version!
package io.github.cvc5;
import io.github.cvc5.CVC5ApiException;
import java.util.HashMap;
import java.util.Map;
public enum SortKind
{
/**
* Internal kind.
*
* This kind serves as an abstraction for internal kinds that are not exposed
* via the API but may appear in terms returned by API functions, e.g.,
* when querying the simplified form of a term.
*
*
* @api.note Should never be created via the API.
*/
INTERNAL_SORT_KIND(-2),
/**
* Undefined kind.
*
*
* @api.note Should never be exposed or created via the API.
*/
UNDEFINED_SORT_KIND(-1),
/**
* Null kind.
*
* The kind of a null sort ({@link Sort#Sort()}).
*
*
* @api.note May not be explicitly created via API functions other than
* {@link Sort#Sort()}.
*/
NULL_SORT(0),
/**
* An abstract sort.
*
* An abstract sort represents a sort whose parameters or argument sorts are
* unspecified. For example, `mkAbstractSort(BITVECTOR_SORT)` returns a
* sort that represents the sort of bit-vectors whose bit-width is
* unspecified.
*
*
* - Create Sort of this Kind with:
*
*
* - {@link Solver#mkAbstractSort(SortKind)}
*
*
*/
ABSTRACT_SORT(1),
/**
* An array sort, whose argument sorts are the index and element sorts of the
* array.
*
*
* - Create Sort of this Kind with:
*
*
* - {@link Solver#mkArraySort(Sort, Sort)}
*
*
*/
ARRAY_SORT(2),
/**
* A bag sort, whose argument sort is the element sort of the bag.
*
*
* - Create Sort of this Kind with:
*
*
* - {@link Solver#mkBagSort(Sort)}
*
*
*/
BAG_SORT(3),
/**
* The Boolean sort.
*
*
* - Create Sort of this Kind with:
*
*
* - {@link Solver#getBooleanSort()}
*
*
*/
BOOLEAN_SORT(4),
/**
* A bit-vector sort, parameterized by an integer denoting its bit-width.
*
*
* - Create Sort of this Kind with:
*
*
* - {@link Solver#mkBitVectorSort(int)}
*
*
*/
BITVECTOR_SORT(5),
/**
* A datatype sort.
*
*
* - Create Sort of this Kind with:
*
*
* - {@link Solver#mkDatatypeSort(DatatypeDecl)}
*
* - {@link Solver#mkDatatypeSorts(DatatypeDecl[])}
*
*
*/
DATATYPE_SORT(6),
/**
* A finite field sort, parameterized by a size.
*
*
* - Create Sort of this Kind with:
*
*
* - {@link Solver#mkFiniteFieldSort(String, int base)}
*
*
*/
FINITE_FIELD_SORT(7),
/**
* A floating-point sort, parameterized by two integers denoting its
* exponent and significand bit-widths.
*
*
* - Create Sort of this Kind with:
*
*
* - {@link Solver#mkFloatingPointSort(int, int)}
*
*
*/
FLOATINGPOINT_SORT(8),
/**
* A function sort with given domain sorts and codomain sort.
*
*
* - Create Sort of this Kind with:
*
*
* - {@link Solver#mkFunctionSort(Sort[], Sort)}
*
*
*/
FUNCTION_SORT(9),
/**
* The integer sort.
*
*
* - Create Sort of this Kind with:
*
*
* - {@link Solver#getIntegerSort()}
*
*
*/
INTEGER_SORT(10),
/**
* The real sort.
*
*
* - Create Sort of this Kind with:
*
*
* - {@link Solver#getRealSort()}
*
*
*/
REAL_SORT(11),
/**
* The regular language sort.
*
*
* - Create Sort of this Kind with:
*
*
* - {@link Solver#getRegExpSort()}
*
*
*/
REGLAN_SORT(12),
/**
* The rounding mode sort.
*
*
* - Create Sort of this Kind with:
*
*
* - {@link Solver#getRoundingModeSort()}
*
*
*/
ROUNDINGMODE_SORT(13),
/**
* A sequence sort, whose argument sort is the element sort of the sequence.
*
*
* - Create Sort of this Kind with:
*
*
* - {@link Solver#mkSequenceSort(Sort)}
*
*
*/
SEQUENCE_SORT(14),
/**
* A set sort, whose argument sort is the element sort of the set.
*
*
* - Create Sort of this Kind with:
*
*
* - {@link Solver#mkSetSort(Sort)}
*
*
*/
SET_SORT(15),
/**
* The string sort.
*
*
* - Create Sort of this Kind with:
*
*
* - {@link Solver#getStringSort()}
*
*
*/
STRING_SORT(16),
/**
* A tuple sort, whose argument sorts denote the sorts of the direct children
* of the tuple.
*
*
* - Create Sort of this Kind with:
*
*
* - {@link Solver#mkTupleSort(Sort[])}
*
*
*/
TUPLE_SORT(17),
/**
* A nullable sort, whose argument sort denotes the sort of the direct child
* of the nullable.
*
*
* - Create Sort of this Kind with:
*
*
* - {@link Solver#mkNullableSort(Sort)}
*
*
*/
NULLABLE_SORT(18),
/**
* An uninterpreted sort.
*
*
* - Create Sort of this Kind with:
*
*
* - {@link Solver#mkUninterpretedSort(String)}
*
*
*/
UNINTERPRETED_SORT(19),
/**
* Marks the upper-bound of this enumeration.
*/
LAST_SORT_KIND(20),
;
/* the int value of the SortKind */
private int value;
private static int minValue = 0;
private static int maxValue = 0;
private static Map enumMap = new HashMap<>();
private SortKind(int value)
{
this.value = value;
}
static
{
boolean firstValue = true;
for (SortKind enumerator : SortKind.values())
{
int value = enumerator.getValue();
if (firstValue)
{
minValue = value;
maxValue = value;
firstValue = false;
}
minValue = Math.min(minValue, value);
maxValue = Math.max(maxValue, value);
enumMap.put(value, enumerator);
}
}
public static SortKind fromInt(int value) throws CVC5ApiException
{
if (value < minValue || value > maxValue)
{
throw new CVC5ApiException("SortKind value " + value + " is outside the valid range ["
+ minValue + "," + maxValue + "]");
}
return enumMap.get(value);
}
public int getValue()
{
return value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy