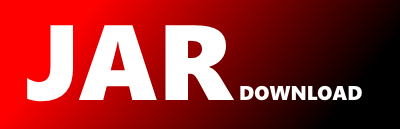
tools.dynamia.commons.DynamicComparator Maven / Gradle / Ivy
/*
* Copyright (C) 2009 - 2019 Dynamia Soluciones IT S.A.S - NIT 900302344-1
* Colombia - South America
* All Rights Reserved.
*
* DynamiaTools is free software: you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License (LGPL v3) as
* published by the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* DynamiaTools is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with DynamiaTools. If not, see .
*/
package tools.dynamia.commons;
import java.util.Comparator;
/**
* Archivo: DynamicComparator.java Fecha Creacion: 4/05/2009
*
* @author Ing. Mario Serrano Leones
* @param the generic type
*/
public class DynamicComparator implements Comparator {
/**
* The field.
*/
private String field;
/**
* The ascending.
*/
private boolean ascending;
/**
* Instantiates a new dynamic comparator.
*/
public DynamicComparator() {
this(null);
}
/**
* Instantiates a new dynamic comparator.
*
* @param field the field
*/
public DynamicComparator(String field) {
setField(field);
}
public DynamicComparator(String field, boolean ascending) {
super();
this.field = field;
this.ascending = ascending;
}
/**
* Checks if is ascending.
*
* @return true, if is ascending
*/
public boolean isAscending() {
return ascending;
}
/**
* Sets the ascending.
*
* @param ascending the new ascending
*/
public void setAscending(boolean ascending) {
this.ascending = ascending;
}
/**
* Gets the field.
*
* @return the field
*/
public String getField() {
return field;
}
/**
* Sets the field.
*
* @param field the new field
*/
public void setField(String field) {
if (field != null && field.isEmpty()) {
throw new IllegalArgumentException("Field name cannot be empty ");
}
this.field = field;
}
/*
* (non-Javadoc)
*
* @see java.util.Comparator#compare(java.lang.Object, java.lang.Object)
*/
@SuppressWarnings({"rawtypes", "unchecked"})
@Override
public int compare(T o1, T o2) {
int result = 0;
Comparable value1 = getValue(o1);
Comparable value2 = getValue(o2);
if (value1 != null && value2 == null) {
result = 1;
} else if (value1 == null && value2 != null) {
result = -1;
} else if (value1 == null && value2 == null) {
result = 0;
} else {
result = value1.compareTo(value2);
}
if (!isAscending()) {
if (result < 0) {
result = 1;
} else if (result > 0) {
result = -1;
}
}
return result;
}
/**
* Gets the value.
*
* @param obj the obj
* @return the value
*/
@SuppressWarnings("rawtypes")
private Comparable getValue(T obj) {
Comparable value = null;
if (obj != null && field != null) {
try {
Object result = BeanUtils.invokeGetMethod(obj, field);
if (result instanceof Comparable) {
value = (Comparable) result;
}
} catch (Exception ex) {
throw new RuntimeException(ex);
}
}
return value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy