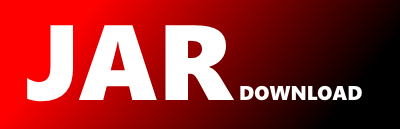
tools.dynamia.ui.UIMessages Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tools.dynamia.ui Show documentation
Show all versions of tools.dynamia.ui Show documentation
Helper classes for module integrations and messages
The newest version!
/*
* Copyright (C) 2023 Dynamia Soluciones IT S.A.S - NIT 900302344-1
* Colombia / South America
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package tools.dynamia.ui;
import tools.dynamia.commons.Callback;
import tools.dynamia.commons.LocalizedMessagesProvider;
import tools.dynamia.commons.Messages;
import tools.dynamia.integration.Containers;
import java.util.ArrayList;
import java.util.Comparator;
import java.util.List;
import java.util.Locale;
import java.util.function.Consumer;
/**
* Show messages and prompts to user *
*/
public class UIMessages {
private static MessageDisplayer currentMessageDisplayer;
private UIMessages() {
}
/**
* Show a normal user message
*/
public static void showMessage(String text) {
showMessage(text, MessageType.NORMAL);
}
/**
* Show a user message with specific {@link MessageType}
*/
public static void showMessage(String text, MessageType type) {
showMessage(text, null, type);
}
/**
* Show a user message with custom title and {@link MessageType}
*/
public static void showMessage(String text, String title, MessageType type) {
showMessage(text, title, type, null);
}
/**
* Show Message with source object
*/
public static void showMessage(String text, String title, MessageType type, Object source) {
MessageDisplayer displayer = getDisplayer();
displayer.showMessage(text, title, type, source);
}
/**
* Show a localized message using default {@link LocalizedMessagesProvider}
*/
public static void showLocalizedMessage(String template, MessageType messageType, Object... vars) {
String localizedMessage = getLocalizedMessage(template, "* UI Messages");
String message = String.format(localizedMessage, vars);
showMessage(message, messageType);
}
public static void showLocalizedMessage(String template, Object... vars) {
showLocalizedMessage(template, MessageType.NORMAL, vars);
}
private static MessageDisplayer getDisplayer() {
if (currentMessageDisplayer == null) {
currentMessageDisplayer = Containers.get().findObject(MessageDisplayer.class);
if (currentMessageDisplayer == null) {
throw new IllegalStateException("MessageDisplayer not found");
}
}
return currentMessageDisplayer;
}
public static void setCurrentMessageDisplayer(MessageDisplayer currentMessageDisplayer) {
UIMessages.currentMessageDisplayer = currentMessageDisplayer;
}
/**
* Show question or confirmation dialog. When user click [Yes] the onYesResponse
* callback is called
*/
public static void showQuestion(String text, Callback onYesResponse) {
showQuestion(text, (String) null, onYesResponse);
}
/**
* Show question or confirmation dialog with custom title. When user click [Yes] the onYesResponse
* callback is called.
*/
public static void showQuestion(String text, String title, Callback onYesResponse) {
getDisplayer().showQuestion(text, title, onYesResponse);
}
/**
* Show question or confirmation dialog. When user click [Yes] the onYesResponse
* callback is called and when [NO] onNoResponse callback is called.
*/
public static void showQuestion(String text, Callback onYesResponse, Callback onNoResponse) {
showQuestion(text, null, onYesResponse, onNoResponse);
}
/**
* Show question or confirmation dialog with custom title. When user click [Yes] the onYesResponse
* callback is called and when [NO] onNoResponse callback is called.
*/
public static void showQuestion(String text, String title, Callback onYesResponse, Callback onNoResponse) {
getDisplayer().showQuestion(text, title, onYesResponse, onNoResponse);
}
/**
* Show Questing with localized message
*/
public static void showLocalizedQuestion(String template, Object[] vars, Callback onYesResponse) {
String localizedMessage = getLocalizedMessage(template, "* UI Messages");
String message = String.format(localizedMessage, vars);
showQuestion(message, onYesResponse);
}
/**
*
*/
public static void showLocalizedQuestion(String template, List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy