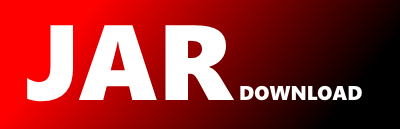
tools.dynamia.ui.UITools Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tools.dynamia.ui Show documentation
Show all versions of tools.dynamia.ui Show documentation
Helper classes for module integrations and messages
The newest version!
package tools.dynamia.ui;
import tools.dynamia.integration.Containers;
import java.util.List;
public class UITools {
private static UIToolsProvider currentProvider;
private static UIToolsProvider getProvider() {
if (currentProvider == null) {
currentProvider = Containers.get().findObject(UIToolsProvider.class);
if (currentProvider == null) {
throw new IllegalStateException("UIToolsProvider not found");
}
}
return currentProvider;
}
public static void setCurrentProvider(UIToolsProvider currentProvider) {
UITools.currentProvider = currentProvider;
}
/**
* Check if current thread is the event thread
*
* @return true if current thread is the event thread
*/
public static boolean isInEventThread() {
return getProvider().isInEventThread();
}
/**
* Create a dialog
*
* @param title the title
* @return the dialog
*/
public static DialogComponent dialog(String title) {
return getProvider().createDialog(title);
}
/**
* Create a dialog
*
* @param title the title
* @param chidlren the children
* @return the dialog
*/
public static DialogComponent dialog(String title, List chidlren) {
var dialog = dialog(title);
if (chidlren != null) {
chidlren.forEach(dialog::add);
}
return dialog;
}
/**
* Show a dialog
*
* @param title the title
* @param content the content
* @return the dialog
*/
public static DialogComponent showDialog(String title, Object content) {
return getProvider().showDialog(title, content);
}
/**
* Show a dialog
*
* @param title the title
* @param content the content
* @param width the width
* @param height the height
* @return the dialog
*/
public static DialogComponent showDialog(String title, Object content, String width, String height) {
return getProvider().showDialog(title, content, width, height);
}
/**
* Show a dialog
*
* @param title the title
* @param content the content
* @param onClose the on close callback
* @return the dialog
*/
public static DialogComponent showDialog(String title, Object content, EventCallback onClose) {
return getProvider().showDialog(title, content, onClose);
}
/**
* Show a dialog
*
* @param title the title
* @param content the content
* @param data the data
* @param width the width
* @param height the height
* @param onClose the on close callback
* @return the dialog
*/
public static DialogComponent showDialog(String title, Object content, Object data, String width, String height, EventCallback onClose) {
return getProvider().showDialog(title, content, data, width, height, onClose);
}
/**
* Create a listbox
*
* @param items the items
* @param the type
* @return the listbox
*/
public static ListboxComponent listbox(List items) {
return getProvider().createListbox(items);
}
/**
* Create a combobox
*
* @param items the items
* @param the type
* @return the combobox
*/
public static ComboboxComponent combobox(List items) {
return getProvider().createCombobox(items);
}
/**
* Show a listbox selector
*
* @param title the title
* @param data the data
* @param onSelect the on select callback
* @param the type
* @return the dialog
*/
public static DialogComponent showListboxSelector(String title, List data, SelectEventCallback onSelect) {
return getProvider().showListboxSelector(title, data, onSelect);
}
/**
* Show a listbox selector
*
* @param title the title
* @param data the data
* @param defaultSelection the default selection
* @param onSelect the on select callback
* @param the type
* @return the dialog
*/
public static DialogComponent showListboxSelector(String title, List data, T defaultSelection, SelectEventCallback onSelect) {
return getProvider().showListboxSelector(title, data, defaultSelection, onSelect);
}
/**
* Show a listbox multi selector
*
* @param title the title
* @param data the data
* @param onSelect the on select callback
* @param the type
* @return the dialog
*/
public static DialogComponent showListboxMultiSelector(String title, String label, List data, SelectionEventCallback onSelect) {
return getProvider().showListboxMultiSelector(title, label, data, onSelect);
}
/**
* Show a listbox multi selector
*
* @param title the title
* @param data the data
* @param defaultSelection the default selection
* @param onSelect the on select callback
* @param the type
* @return the dialog
*/
public static DialogComponent showListboxMultiSelector(String title, String label, List data, List defaultSelection, SelectionEventCallback onSelect) {
return getProvider().showListboxMultiSelector(title, label, data, defaultSelection, onSelect);
}
/**
* Create a button
*
* @param label the label
* @return the button
*/
public static ButtonComponent button(String label) {
return getProvider().createButton(label);
}
/**
* Create a button
*
* @param label the label
* @param onClick the on click callback
* @return the button
*/
public static ButtonComponent button(String label, EventCallback onClick) {
return getProvider().createButton(label, onClick);
}
/**
* Show a table selector
*
* @param title dialog title
* @param columns table field name columns
* @param data selection date
* @param onSelect selected items
* @param data type
* @return dialog
*/
public static DialogComponent showTableSelector(String title, String label, List columns, List data, SelectEventCallback onSelect) {
return getProvider().showTableSelector(title, label, columns, data, onSelect);
}
/**
* Show a table multi select or
*
* @param title dialog title
* @param columns table field name columns
* @param data selection date
* @param onSelect selected items
* @param data type
* @return dialog
*/
public static DialogComponent showTableMultiSelector(String title, String label, List columns, List data, SelectionEventCallback onSelect) {
return getProvider().showTableMultiSelector(title, label, columns, data, onSelect);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy