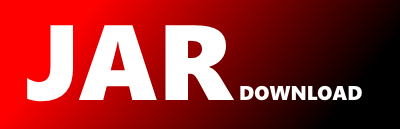
mir.reactions.familiesToPersons.CreatedFatherReaction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tools.vitruv.dsls.demo.familiespersons Show documentation
Show all versions of tools.vitruv.dsls.demo.familiespersons Show documentation
A demo for the Vitruv framework based on the families and persons metamodels
The newest version!
package mir.reactions.familiesToPersons;
import edu.kit.ipd.sdq.metamodels.families.Family;
import edu.kit.ipd.sdq.metamodels.families.Member;
import java.util.function.Function;
import mir.routines.familiesToPersons.FamiliesToPersonsRoutinesFacade;
import org.eclipse.emf.ecore.EObject;
import org.eclipse.emf.ecore.EReference;
import org.eclipse.xtext.xbase.lib.Extension;
import tools.vitruv.change.atomic.EChange;
import tools.vitruv.change.atomic.feature.reference.ReplaceSingleValuedEReference;
import tools.vitruv.dsls.reactions.runtime.reactions.AbstractReaction;
import tools.vitruv.dsls.reactions.runtime.routines.AbstractRoutine;
import tools.vitruv.dsls.reactions.runtime.routines.RoutinesFacade;
import tools.vitruv.dsls.reactions.runtime.state.ReactionExecutionState;
@SuppressWarnings("all")
public class CreatedFatherReaction extends AbstractReaction {
private ReplaceSingleValuedEReference replaceChange;
public CreatedFatherReaction(final Function routinesFacadeGenerator) {
super(routinesFacadeGenerator);
}
private static class Call extends AbstractRoutine.Update {
public Call(final ReactionExecutionState reactionExecutionState) {
super(reactionExecutionState);
}
public void updateModels(final ReplaceSingleValuedEReference replaceChange, final Family affectedEObject, final EReference affectedFeature, final Member oldValue, final Member newValue, @Extension final FamiliesToPersonsRoutinesFacade _routinesFacade) {
_routinesFacade.updateNameAndCorrespondencesAfterMemberBecameFather(newValue, affectedEObject);
_routinesFacade.createMaleFromNewMember(newValue, affectedEObject);
}
}
public boolean isCurrentChangeMatchingTrigger(final EChange change) {
if (!(change instanceof ReplaceSingleValuedEReference>)) {
return false;
}
ReplaceSingleValuedEReference _localTypedChange = (ReplaceSingleValuedEReference) change;
if (!(_localTypedChange.getAffectedElement() instanceof edu.kit.ipd.sdq.metamodels.families.Family)) {
return false;
}
if (!_localTypedChange.getAffectedFeature().getName().equals("father")) {
return false;
}
if (_localTypedChange.isFromNonDefaultValue() && !(_localTypedChange.getOldValue() instanceof edu.kit.ipd.sdq.metamodels.families.Member)) {
return false;
}
if (_localTypedChange.isToNonDefaultValue() && !(_localTypedChange.getNewValue() instanceof edu.kit.ipd.sdq.metamodels.families.Member)) {
return false;
}
this.replaceChange = (ReplaceSingleValuedEReference) change;
return true;
}
private boolean isUserDefinedPreconditionFulfilled(final ReplaceSingleValuedEReference replaceChange, final Family affectedEObject, final EReference affectedFeature, final Member oldValue, final Member newValue) {
return (newValue != null);
}
public void executeReaction(final EChange change, final ReactionExecutionState executionState, final RoutinesFacade routinesFacadeUntyped) {
mir.routines.familiesToPersons.FamiliesToPersonsRoutinesFacade routinesFacade = (mir.routines.familiesToPersons.FamiliesToPersonsRoutinesFacade)routinesFacadeUntyped;
if (!isCurrentChangeMatchingTrigger(change)) {
return;
}
edu.kit.ipd.sdq.metamodels.families.Family affectedEObject = (edu.kit.ipd.sdq.metamodels.families.Family)replaceChange.getAffectedElement();
EReference affectedFeature = replaceChange.getAffectedFeature();
edu.kit.ipd.sdq.metamodels.families.Member oldValue = (edu.kit.ipd.sdq.metamodels.families.Member)replaceChange.getOldValue();
edu.kit.ipd.sdq.metamodels.families.Member newValue = (edu.kit.ipd.sdq.metamodels.families.Member)replaceChange.getNewValue();
if (getLogger().isTraceEnabled()) {
getLogger().trace("Passed change matching of Reaction " + this.getClass().getName());
}
if (!isUserDefinedPreconditionFulfilled(replaceChange, affectedEObject, affectedFeature, oldValue, newValue)) {
return;
}
if (getLogger().isTraceEnabled()) {
getLogger().trace("Passed complete precondition check of Reaction " + this.getClass().getName());
}
new mir.reactions.familiesToPersons.CreatedFatherReaction.Call(executionState).updateModels(replaceChange, affectedEObject, affectedFeature, oldValue, newValue, routinesFacade);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy