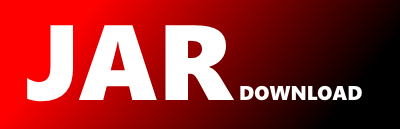
tools.xor.service.XorREST Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xor Show documentation
Show all versions of xor Show documentation
Empowering Model Driven Architecture in J2EE applications
package tools.xor.service;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.InputStream;
import java.util.LinkedList;
import java.util.List;
import org.json.JSONArray;
import org.json.JSONObject;
import org.springframework.core.io.ClassPathResource;
import org.springframework.core.io.Resource;
import tools.xor.AggregateAction;
import tools.xor.EntityType;
import tools.xor.Settings;
import tools.xor.providers.jdbc.JDBCBatchContext;
import tools.xor.util.Constants;
import tools.xor.util.graph.TypeGraph;
/**
* This class represents an implementation of the XOR REST api.
* Provider implementations will extend this class with the appropriate Controller class
* and provide the necessary transaction scaffolding, path/param mapping etc...
*/
public abstract class XorREST
{
/**
* AggregateManager configured with ORM datasource
* @return AggregateManager instance
*/
protected abstract AggregateManager getAM ();
/**
* AggregateManager configured with JDBC datasource
* @return AggregateManager instance
*/
protected abstract AggregateManager getJDBCAM ();
/**
* Return the settings object
* @param jsonString JSON string containing user settings
* @param userData any additional data passed by the user
* @return Settings instance
*/
protected abstract Settings getSettings (String jsonString, Object userData);
/**
* Method used to create an entity.
*
* @param jsonString JSON string of both the settings and the entity object that needs to be created
* @return JSON object of the created entity
*/
protected JSONObject create (String jsonString)
{
JSONObject json = new JSONObject(jsonString);
Object entity = getEntity(json);
DataModel das = getAM().getDataModel();
String settings = json.getJSONObject("settings").toString();
Object persistentObj = getAM().create(entity, das.settings().json(settings).build());
Settings readSettings = das.settings().base(persistentObj.getClass()).build();
JSONObject readJson = (JSONObject)getAM().toExternal(persistentObj, readSettings);
return readJson;
//readJson.toString(3);
}
/**
* Read an entity from the database in JSON format
* @param jsonString JSON string containing the user settings and the entity id/key
* @return JSON representation of the entity
*/
protected JSONObject read (String jsonString)
{
JSONObject json = new JSONObject(jsonString);
Object entity = getEntity(json);
DataModel das = getAM().getDataModel();
String settings = json.getJSONObject("settings").toString();
JSONObject readJson = (JSONObject)getAM().read(
entity,
das.settings().json(settings).build());
return readJson;
}
/**
* Do a partial or a full update of a persistent entity.
* @param jsonString JSON string containing the user settings and the entity values that need to be updated.
*/
protected void update (String jsonString)
{
JSONObject json = new JSONObject(jsonString);
Object entity = getEntity(json);
DataModel das = getAM().getDataModel();
String settings = json.getJSONObject("settings").toString();
getAM().update(entity, das.settings().json(settings).build());
}
/**
* Delete a persistent entity from the database.
* @param jsonString JSON string containing the user settings and the entity id/key to be deleted.
*/
protected void delete (String jsonString)
{
JSONObject json = new JSONObject(jsonString);
Object entity = getEntity(json);
DataModel das = getAM().getDataModel();
String settings = json.getJSONObject("settings").toString();
getAM().delete(entity, das.settings().json(settings).build());
}
/**
* Pass in a json string containing an array of file names containing the generator details.
* Should only contain the file name and not the full path, since the file is assumed to be part
* of the classpath.
*
* @param jsonString JSON string of the generator excel files
*/
protected void initializeGenerators(String jsonString) {
JSONArray array = new JSONArray(jsonString);
try {
// Make sure the files are under src/main/resources
for(int i = 0; i < array.length(); i++) {
Resource resource = new ClassPathResource(array.getString(i));
InputStream inputStream = resource.getInputStream();
initializeGenerators(inputStream);
}
}
catch (FileNotFoundException e) {
e.printStackTrace();
}
catch (IOException e) {
e.printStackTrace();
}
}
/**
* Use this method if the user is sending the excel file directly.
*
* @param inputStream containing the Excel file.
*/
protected void initializeGenerators(InputStream inputStream) {
DataModel das = getAM().getDataModel();
das.initGenerators(inputStream);
}
/**
* Return a Graphviz file in .dot format of the State Graph
* @param settings object
* @return .dot format
*/
protected File getGraphvizDOT(Settings settings) {
TypeGraph sg = settings.getView().getTypeGraph((EntityType)settings.getEntityType());
sg.generateVisual(settings);
File file = new File(settings.getGraphFileName());
if(file.exists()) {
return file;
}
throw new RuntimeException("File not found: " + settings.getGraphFileName());
}
/**
* Should be wrapped by the provider implementation in a transaction.
* Alternatively, the user can wrap the populate method instead but this can cause issues
* if the count is large.
*
* @param settings user settings
* @param userData any user data needed by the provider overridden implementation
*/
protected void populateBatch(Settings settings, Object userData) {
TypeGraph sg = settings.getView().getTypeGraph((EntityType)settings.getEntityType());
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy