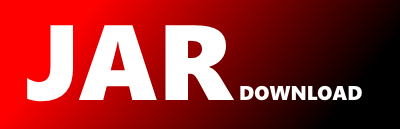
top.doudou.core.util.LogUtil Maven / Gradle / Ivy
package top.doudou.core.util;
import org.apache.commons.lang3.StringUtils;
import java.util.Arrays;
/**
* @Description 日志工具类
* 给日志上色
* 适用于这种日志的格式 %date{yyyy-MM-dd HH:mm:ss} [%-5level] [%thread] %logger{96} [%line] - %msg%n
* @Author 傻男人 <[email protected]>
* @Date 2020-10-22 11:03
* @Version V1.0
*/
public class LogUtil {
private static final String TIME_FORMAT = "[\\d+][\\d+][\\d+][\\d+]-[\\d+][\\d+]-[\\d+][\\d+] [\\d+][\\d+]:[\\d+][\\d+]:[\\d+][\\d+]";
private static final String DEBUG = "";
private static final String INFO = "";
private static final String WARN = "";
private static final String ERROR = "";
private static final String BLACK = "";
private static final String SPACE = " ";
private static final String SHORT_SPACE = " ";
private static final String LONG_SPACE = " ";
private static final String SPAN_OVER = "";
private static final int FIRST_MAX = 400;
private static final String STDOUT_CONSOLE = "StdoutListener$$EnhancerBySpringCGLIB$$";
private static final String STDOUT_CONSOLE_SPLIT = "StdoutListener\\$\\$EnhancerBySpringCGLIB\\$\\$";
private static final String REG_EXP = "\\w+\\s*\\[\\d+\\]\\s*-\\s*";
private static final String SPLIT = " ";
/**
* 将日志上色,方便前端展示时,为不同的颜色的日志
* @param line
* @return
*/
public static String toColor(String line){
if(line.contains(STDOUT_CONSOLE)){
String result = line.split(STDOUT_CONSOLE_SPLIT)[1].replaceFirst(REG_EXP,"");
return "
"+result;
}
//先转义
line = line.replaceAll("&", "&")
.replaceAll("<", "<")
.replaceAll(">", ">")
.replaceAll("\"", """);
//处理等级
line = line.replace("DEBUG", DEBUG + "DEBUG"+SPAN_OVER);
line = line.replace("INFO", INFO + "INFO"+SPAN_OVER);
line = line.replace("WARN", WARN + "WARN"+SPAN_OVER);
line = line.replace("ERROR", ERROR + "ERROR"+SPAN_OVER);
StringBuffer sb = new StringBuffer("
");
//处理类名
String[] split = line.split(SPLIT);
if(split.length >= 5 ){
sb.append(split[0]).append(SHORT_SPACE)
.append(split[1]).append(SHORT_SPACE)
.append(split[2]).append(SHORT_SPACE)
.append(BLACK).append(split[3]).append(SPAN_OVER).append(SHORT_SPACE);
String[] temp = split[4].split("-");
if(temp.length > 1){
sb.append(temp[0]).append(messageColor(sb.toString(),temp,1));
}else {
sb.append(split[4]);
}
if(split.length > 5){
sb.append(messageColor(sb.toString(),split,5));
}
}
if(sb.length() <= 5){
if(line.startsWith("\tat ")){
sb.append(ERROR).append(LONG_SPACE).append(line).append(SPAN_OVER);
}else {
sb.append(line);
}
}
// 匹配日期开头加换行,2019-08-12 14:15:04
// Pattern r = Pattern.compile(TIME_FORMAT);
// Matcher m = r.matcher(line);
// if (m.find( )) {
// //找到下标
// int start = m.start();
// sb.insert(start,"
");
// }
return sb.toString();
}
public static void main(String[] args) {
System.out.println(toColor("2020-10-23 10:37:19 [INFO ] [Thread-34] top.doudou.core.config.StdoutListener$$EnhancerBySpringCGLIB$$633c4e2e [24] - 2020-10-23 10:37:19"));
}
/**
* 将后面的日志上色
* @param line
* @param temp
* @param startLen
* @return
*/
private static String messageColor(String line,String[] temp,int startLen){
if(temp.length <= 1 ){
return "";
}
StringBuilder sb = new StringBuilder();
for (int i = startLen; i < temp.length; i++) {
sb.append(SPLIT+temp[i]);
}
StringBuilder message = new StringBuilder();
message.append(SHORT_SPACE);
if(line.contains(DEBUG)){
message.append(DEBUG);
}else if(line.contains(INFO)){
message.append(INFO);
}else if(line.contains(WARN)){
message.append(WARN);
}else if(line.contains(ERROR)){
message.append(ERROR);
}
message.append(sb);
message.append(SPAN_OVER);
return message.toString();
}
/**
* 日志的处理
* @param lines
* @param first
* @return
*/
public static String handler(Object[] lines,boolean first){
//对日志进行着色,更加美观 PS:注意,这里要根据日志生成规则来操作
for (int i = 0; i < lines.length; i++) {
lines[i] = LogUtil.toColor((String) lines[i]);
}
//第一次如果太大,截取最新的200行就够了,避免传输的数据太大
if(first && lines.length > FIRST_MAX){
lines = Arrays.copyOfRange(lines, lines.length - FIRST_MAX, lines.length);
first = false;
}
return StringUtils.join(lines, "");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy