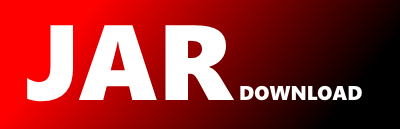
top.cutexingluo.tools.common.opt.OptBundle Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xingtools-core Show documentation
Show all versions of xingtools-core Show documentation
xingtools 核心,包括各种接口,实体类和工具类
package top.cutexingluo.tools.common.opt;
import lombok.Data;
import org.jetbrains.annotations.NotNull;
import top.cutexingluo.tools.common.base.IData;
import java.util.List;
import java.util.function.Consumer;
import java.util.function.Function;
/**
* OptBundle 类
* 类似 Opt 或 Optional
* 可用于执行链策略, 责任链设计模式
*
* @author XingTian
* @version 1.0.0
* @date 2024/3/22 17:04
* @since 1.0.4
*/
@Data
public class OptBundle implements IData {
/**
* 执行函数
*/
public interface OptAction extends Function, OptRes> {
}
/**
* 执行消费者
*/
public interface OptConsumer extends Consumer> {
}
/**
* 执行条件
*
* @since 1.0.5
*/
public interface OptCondition extends Function, Boolean> {
}
/**
* 提供者
*
* @since 1.0.5
*/
public interface OptGetter extends Function, R> {
}
@NotNull
protected OptData data;
/**
* @param data data 不能为 null
*/
public OptBundle(@NotNull OptData data) {
this.data = data;
}
/**
* 获取内部的值
*/
@Override
public T data() {
return data.value;
}
public boolean hasValue() {
return data.value != null;
}
public boolean hasMeta() {
return data.meta != null;
}
public boolean hasType(Class> type) {
return type != null && type.isInstance(data.value);
}
/**
* 比较clazz 变量
*/
public boolean hasClazz(Class> type) {
return data.clazz != null && data.clazz.equals(type);
}
/**
* 比较clazz 变量
* clazz 是否是 type 子类
*/
public boolean isSubClazzFrom(Class> type) {
return type != null && type.isAssignableFrom(data.clazz);
}
/**
* 获取值
*
* @since 1.0.5
*/
public T getValue() {
return data.value;
}
/**
* 获取 Meta
*
* @since 1.0.5
*/
public Meta getMeta() {
return data.meta;
}
public void update(Object obj, boolean updateClazzIfPresent) {
data.update(obj, updateClazzIfPresent);
}
public void updateCheckPresent(Object obj, boolean updateClazzIfPresent) {
data.updateCheckPresent(obj, updateClazzIfPresent);
}
// then
/**
* then 方法, 返回值会赋值
*
* @param updateClazzIfPresent true=> clazz 存在才赋值 clazz 变量
*/
public OptBundle then(boolean updateClazzIfPresent, OptAction action) {
if (action != null) {
OptRes res = action.apply(data);
data.update(res, updateClazzIfPresent);
}
return this;
}
public OptBundle then(OptAction action) {
return then(false, action);
}
/**
* thenCheckPresent 方法 res 不为空才赋值
*
* @param updateClazzIfPresent true=> clazz 存在才赋值 clazz 变量
*/
public OptBundle thenCheckPresent(boolean updateClazzIfPresent, OptAction action) {
if (action != null) {
OptRes res = action.apply(data);
data.updateCheckPresent(res, updateClazzIfPresent);
}
return this;
}
public OptBundle thenCheckPresent(OptAction action) {
return thenCheckPresent(false, action);
}
// pick
/**
* pick 读取数据
*/
public OptBundle pick(OptConsumer consumer) {
if (consumer != null) {
consumer.accept(data);
}
return this;
}
/**
* pick 读取数据
* value 存在才读取数据
*/
public OptBundle filterPick(OptConsumer consumer) {
if (consumer != null && hasValue()) {
consumer.accept(data);
}
return this;
}
/**
* pick 读取数据
* value 不存在才读取数据
*/
public OptBundle emptyPick(OptConsumer consumer) {
if (consumer != null && !hasValue()) {
consumer.accept(data);
}
return this;
}
// then filter
/**
* filterThen 方法
* value 存在才执行函数
* 返回值会赋值
*
* @param updateClazzIfPresent true=> clazz 存在才赋值 clazz 变量
*/
public OptBundle filterThen(boolean updateClazzIfPresent, OptAction action) {
if (action != null && hasValue()) {
OptRes res = action.apply(data);
data.update(res, updateClazzIfPresent);
}
return this;
}
public OptBundle filterThen(OptAction action) {
return filterThen(false, action);
}
/**
* filterThen 方法
* value 存在才执行函数
* 返回值 value 存在才会赋值
*
* @param updateClazzIfPresent true=> clazz 存在才赋值 clazz 变量
*/
public OptBundle filterThenPresent(boolean updateClazzIfPresent, OptAction action) {
if (action != null && hasValue()) {
OptRes res = action.apply(data);
data.updateCheckPresent(res, updateClazzIfPresent);
}
return this;
}
public OptBundle filterThenPresent(OptAction action) {
return filterThenPresent(false, action);
}
/**
* emptyThen 方法
* value 不存在才执行函数
* 返回值会赋值
*
* @param updateClazzIfPresent true=> clazz 存在才赋值 clazz 变量
*/
public OptBundle emptyThen(boolean updateClazzIfPresent, OptAction action) {
if (action != null && !hasValue()) {
OptRes res = action.apply(data);
data.update(res, updateClazzIfPresent);
}
return this;
}
public OptBundle emptyThen(OptAction action) {
return emptyThen(false, action);
}
/**
* emptyThen 方法
* value 不存在才执行函数
* 返回值 value 存在才会赋值
*
* @param updateClazzIfPresent true=> clazz 存在才赋值 clazz 变量
*/
public OptBundle emptyThenPresent(boolean updateClazzIfPresent, OptAction action) {
if (action != null && !hasValue()) {
OptRes res = action.apply(data);
data.updateCheckPresent(res, updateClazzIfPresent);
}
return this;
}
public OptBundle emptyThenPresent(OptAction action) {
return emptyThenPresent(false, action);
}
/**
* 有条件 pick
* 根据条件执行消费者
*
* @param condition 条件
* @param consumer 消费者
* @since 1.0.5
*/
public OptBundle conditionalPick(boolean condition, OptConsumer consumer) {
if (condition && consumer != null) {
consumer.accept(data);
}
return this;
}
/**
* 有条件 pick
* 根据条件执行消费者
*
* @param condition 条件
* @param consumer 消费者
* @since 1.0.5
*/
public OptBundle conditionalPick(OptCondition condition, OptConsumer consumer) {
if (condition != null && Boolean.TRUE.equals(condition.apply(data)) && consumer != null) {
consumer.accept(data);
}
return this;
}
/**
* 有条件 pick
* 根据条件执行消费者
*
* @param getter 目标值获取器
* @param retValCheck 返回值检查 (如果为空,依然会执行消费者)
* @param consumer 消费者
* @since 1.0.5
*/
public OptBundle conditionalPick(OptGetter getter, Function retValCheck, OptConsumer consumer) {
if (getter != null) {
R retVal = getter.apply(data);
if (retValCheck == null || Boolean.TRUE.equals(retValCheck.apply(retVal))) {
consumer.accept(data);
}
}
return this;
}
/**
* 有条件 pick
* 根据条件执行消费者, list 列表需一一对应
*
* @param listGetter 目标值列表获取器
* @param retValCheckList 返回值检查 (如果为空,依然会执行消费者;并且如果list某个元素为空或者越界,则依然会执行对应消费者)
* @param consumerList 消费者列表
* @since 1.0.5
*/
public OptBundle conditionalPick(OptGetter> listGetter, List> retValCheckList, List> consumerList) {
if (listGetter != null) {
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy