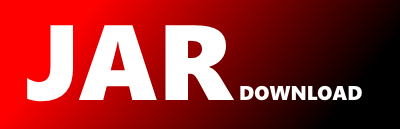
top.cutexingluo.tools.designtools.method.XTObjUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xingtools-core Show documentation
Show all versions of xingtools-core Show documentation
xingtools 核心,包括各种接口,实体类和工具类
package top.cutexingluo.tools.designtools.method;
import org.jetbrains.annotations.NotNull;
import top.cutexingluo.tools.common.Constants;
import top.cutexingluo.tools.exception.ServiceException;
import top.cutexingluo.tools.utils.se.character.XTCharUtil;
import top.cutexingluo.tools.utils.se.map.XTSetUtil;
import java.lang.reflect.Field;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.function.Consumer;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* 反射工具类
* 反射设置属性值
* 于 v1.1.1 合并ruoyi BeanUtils
*
* @author XingTian
* @version 1.0.0
* @date 2023/2/2 21:42
*/
public class XTObjUtil {
/**
* Bean方法名中属性名开始的下标
*/
protected static final int BEAN_METHOD_PROP_INDEX = 3;
/**
* get
*/
public static final String STR_GET = "get";
/**
* set
*/
public static final String STR_SET= "set";
/**
* 匹配getter方法的正则表达式
*/
protected static final Pattern GET_PATTERN = Pattern.compile("get(\\p{javaUpperCase}\\w*)");
/**
* 匹配setter方法的正则表达式
*/
protected static final Pattern SET_PATTERN = Pattern.compile("set(\\p{javaUpperCase}\\w*)");
public static class Unsafe {
public static Unsafe newInstance() {
return new Unsafe();
}
/**
* 设置属性为空
* 含有该属性名的字段设置为null
*
* @param item 对象
* @param properties 属性名
* @return {@link T}
*/
public T setNull(@NotNull T item, @NotNull String... properties) {
return setPropertyContains(item, field -> {
try {
field.set(item, null);
} catch (IllegalAccessException e) {
e.printStackTrace();
}
}, properties);
}
/**
* 设置属性值
* 直接访问属性
*
* @param item 对象
* @param property 属性名
* @param newValue 新值
* @return {@link T}
*/
public T setProperty(@NotNull T item, @NotNull String property, Object newValue) {
try {
Field field = item.getClass().getDeclaredField(property);
field.setAccessible(true);
field.set(item, newValue);
} catch (NoSuchFieldException | IllegalAccessException e) {
e.printStackTrace();
throw new ServiceException(Constants.CODE_400.getCode(), "没有该属性,或者没有该权限");
}
return item;
}
/**
* 遍历字段自行设置属性值
* 直接访问属性
*
* @param item 对象
* @param fieldConsumer 属性遍历操作消费者
* @return {@link T}
*/
public T setPropertyAllFields(@NotNull T item, Consumer fieldConsumer) {
Field[] fields = item.getClass().getDeclaredFields();
for (Field field : fields) {
field.setAccessible(true);
fieldConsumer.accept(field);
}
return item;
}
/**
* 设置属性
* 包含 propertyNames 名称的会进入Consumer
* 直接访问属性
* 遍历字段自行设置属性值
*
* @param item 对象
* @param fieldConsumer 属性遍历操作消费者
* @param propertyNames 满足需要修改的属性名称,会过滤到消费者
* @return {@link T}
*/
public T setPropertyContains(@NotNull T item, @NotNull Consumer fieldConsumer, @NotNull String... propertyNames) {
Field[] fields = item.getClass().getDeclaredFields();
HashSet set = XTSetUtil.hashSet(propertyNames);
for (Field field : fields) {
field.setAccessible(true);
if (set.contains(field.getName())) {
fieldConsumer.accept(field);
}
}
return item;
}
/**
* 设置属性
* propertyNames 名称的所有字段都会进入Consumer
* 没有 propertyNames 的字段就会报错,请使用setPropertyContains
* 直接访问属性
* 遍历字段自行设置属性值
*
* @param item 对象
* @param fieldConsumer 属性遍历操作消费者
* @param propertyNames 需要修改的属性名称,都会过滤到消费者
* @return {@link T}
*/
public T setProperty(@NotNull T item, @NotNull Consumer fieldConsumer, @NotNull String... propertyNames) {
for (String name : propertyNames) {
try {
Field field = item.getClass().getDeclaredField(name);
field.setAccessible(true);
fieldConsumer.accept(field);
} catch (NoSuchFieldException e) {
e.printStackTrace();
}
}
return item;
}
/**
* 获取属性值
* 直接访问属性
*
* @param item 对象
* @param property 属性名
* @return {@link Object}
*/
public Object getProperty(@NotNull T item, @NotNull String property) {
Object result;
try {
Field field = item.getClass().getDeclaredField(property);
field.setAccessible(true);
result = field.get(item);
} catch (NoSuchFieldException | IllegalAccessException e) {
e.printStackTrace();
throw new ServiceException(Constants.CODE_400.getCode(), "没有该属性,或者没有该权限");
}
return result;
}
}
/**
* 利用反射获取对象属性值
* 只获取get和set方法
* 为了安全,不使用getDeclaredFields()
*
* @param item 对象
* @param property 属性名,目标属性必须含有get方法。
* 如果参数未带get,将会自动加上get
* @param isDone 是否不添加get前缀
* @param 对象的类型
* @return 返回Object对象,注意转型
*/
public static Object getProperty(@NotNull T item, @NotNull String property, boolean isDone) {
Object value = null;
property = XTCharUtil.addCheckPrefix(property, STR_GET, isDone);
try {
Method method = item.getClass().getMethod(property);
method.setAccessible(true);
value = method.invoke(item);
} catch (NoSuchMethodException | IllegalAccessException | InvocationTargetException e) {
e.printStackTrace();
throw new ServiceException(Constants.CODE_400.getCode(), "没有该方法,或者没有该权限");
}
return value;
}
public static Object getProperty(@NotNull T item, @NotNull String property) {
return getProperty(item, property, false);
}
/**
* 利用反射设置对象属性值
* 只获取get和set方法
* 为了安全,不使用getDeclaredFields()
*
* @param item 对象
* @param property 属性名,目标属性必须含有set方法。
* 如果参数未带set,将会自动加上set
* @param newValue 设置的新值
* @param isDone 是否不添加set前缀
* @param param 新值的类型,即set参数类型
* @param 对象类型
*/
public static T setProperty(@NotNull T item, @NotNull String property,
Object newValue, boolean isDone, @NotNull Class> param) {
property = XTCharUtil.addCheckPrefix(property, STR_SET, isDone);
try {
Method method;
method = item.getClass().getMethod(property, param);
method.setAccessible(true);
method.invoke(item, newValue);
} catch (NoSuchMethodException | IllegalAccessException | InvocationTargetException e) {
e.printStackTrace();
throw new ServiceException(Constants.CODE_400.getCode(), "没有该方法,或者没有该权限");
}
return item;
}
public static T setProperty(@NotNull T item, @NotNull String property,
Object newValue, @NotNull Class> param) {
return setProperty(item, property, newValue, false, param);
}
/**
* 利用反射获取对象列表单一属性的属性值
* 只获取get和set方法
* 为了安全,不使用getDeclaredFields()
*
* @param items 对象列表
* @param property 属性名,目标属性必须含有get方法。
* 如果参数未带get,将会自动加上get
* @param 对象类型
*/
@NotNull
public static List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy