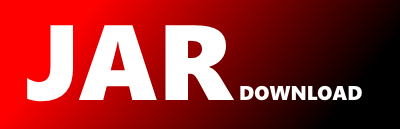
top.hendrixshen.magiclib.language.impl.MagicLanguageResourceManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of magiclib-1_14_4 Show documentation
Show all versions of magiclib-1_14_4 Show documentation
To beat magic with magic :(
package top.hendrixshen.magiclib.language.impl;
import org.jetbrains.annotations.NotNull;
import java.io.File;
import java.io.IOException;
import java.net.JarURLConnection;
import java.net.URISyntaxException;
import java.net.URL;
import java.nio.file.*;
import java.nio.file.attribute.BasicFileAttributes;
import java.util.*;
import java.util.function.Predicate;
import java.util.jar.JarEntry;
import java.util.jar.JarFile;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import java.util.stream.Stream;
import net.minecraft.class_2960;
import net.minecraft.class_3262;
import net.minecraft.class_3298;
import net.minecraft.class_3300;
public class MagicLanguageResourceManager implements class_3300 {
private static final Pattern languageResourcePattern = Pattern.compile("^assets/([\\w-]*)/lang/([a-z\\d-_]*)\\.json$");
private final Set namespaces = new HashSet<>();
private final HashMap> resources = new HashMap<>();
public MagicLanguageResourceManager() {
try {
for (URL resource : Collections.list(this.getClass().getClassLoader().getResources("assets"))) {
if (resource.getProtocol().equalsIgnoreCase("jar")) {
this.addResources(MagicLanguageResourceManager.getJarResources(resource));
} else if (resource.getProtocol().equalsIgnoreCase("file")) {
this.addResources(MagicLanguageResourceManager.getFileResources(resource));
}
}
} catch (IOException e) {
throw new RuntimeException(e);
}
}
private static @NotNull Map getJarResources(@NotNull URL resourceUrl) throws IOException {
JarURLConnection conn = (JarURLConnection) resourceUrl.openConnection();
JarFile jar = conn.getJarFile();
Map ret = new HashMap<>();
for (JarEntry entry : Collections.list(jar.entries())) {
String entryName = entry.getName();
Matcher matcher = languageResourcePattern.matcher(entryName);
if (matcher.find()) {
String namespace = matcher.group(1);
String code = matcher.group(2);
if (!entry.isDirectory()) {
String languagePath = String.format("lang/%s.json", code);
class_2960 resourceLocation = new class_2960(namespace, languagePath);
//#if MC >= 11903
//$$ Resource resource = new MagicLanguageResource(new FilePackResources(namespace, new File(resourceUrl.getPath()), true), resourceLocation, () -> {
//#else
class_3298 resource = new MagicLanguageResource(namespace, resourceLocation, () -> {
//#endif
try {
return jar.getInputStream(entry);
} catch (IOException e) {
throw new RuntimeException(e);
}
});
ret.put(resourceLocation, resource);
}
}
}
return ret;
}
private static @NotNull Map getFileResources(@NotNull URL resourceUrl) throws IOException {
Map ret = new HashMap<>();
try {
Path assetsPath = Paths.get(resourceUrl.toURI());
Files.walkFileTree(assetsPath, new FileVisitor() {
@Override
public FileVisitResult preVisitDirectory(Path dir, BasicFileAttributes attrs) {
return FileVisitResult.CONTINUE;
}
@Override
public FileVisitResult visitFile(Path file, BasicFileAttributes attrs) {
String fileName = "assets/" + assetsPath.relativize(file).toString().replace("\\", "/");
Matcher matcher = languageResourcePattern.matcher(fileName);
if (matcher.find()) {
String namespace = matcher.group(1);
String code = matcher.group(2);
String languagePath = String.format("lang/%s.json", code);
class_2960 resourceLocation = new class_2960(namespace, languagePath);
//#if MC >= 11903
//$$ Resource resource = new MagicLanguageResource(new FilePackResources(namespace, file.toFile(), true), resourceLocation, () -> {
//#else
class_3298 resource = new MagicLanguageResource(namespace, resourceLocation, () -> {
//#endif
try {
return Files.newInputStream(file);
} catch (IOException e) {
throw new RuntimeException(e);
}
});
ret.put(resourceLocation, resource);
}
return FileVisitResult.CONTINUE;
}
@Override
public FileVisitResult visitFileFailed(Path file, IOException exc) {
return FileVisitResult.CONTINUE;
}
@Override
public FileVisitResult postVisitDirectory(Path dir, IOException exc) {
return FileVisitResult.CONTINUE;
}
});
} catch (URISyntaxException e) {
throw new RuntimeException(e);
}
return ret;
}
private void addResources(@NotNull Map resources) {
for (Map.Entry entry : resources.entrySet()) {
class_2960 location = entry.getKey();
namespaces.add(location.method_12836());
Set resourceList = this.resources.computeIfAbsent(location, resourceLocation -> new HashSet<>());
resourceList.add(entry.getValue());
}
}
@Override
public Set method_14487() {
return namespaces;
}
@Override
public List method_14489(@NotNull class_2960 resourceLocation) {
return new ArrayList<>(resources.getOrDefault(resourceLocation, new HashSet<>()));
}
//#if MC > 11802
//$$ @Override
//$$ public Map listResources(@NotNull String string, @NotNull Predicate predicate) {
//$$ return null;
//$$ }
//$$
//$$ @Override
//$$ public Map> listResourceStacks(@NotNull String string, @NotNull Predicate predicate) {
//$$ return null;
//$$ }
//#else
@Override
public Collection method_14488(@NotNull String string, @NotNull Predicate predicate) {
return null;
}
//#endif
//#if MC > 11502
//$$ @Override
//$$ public Stream listPacks() {
//$$ return null;
//$$ }
//#endif
//#if MC <= 11404
@Override
public void method_14475(class_3262 pack) {}
//#endif
//#if MC > 11802
//$$ @Override
//$$ public Optional getResource(ResourceLocation resourceLocation) {
//$$ return Optional.empty();
//$$ }
//#else
@Override
public class_3298 method_14486(@NotNull class_2960 resourceLocation) {
return null;
}
@Override
public boolean method_18234(@NotNull class_2960 resourceLocation) {
return false;
}
//#endif
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy