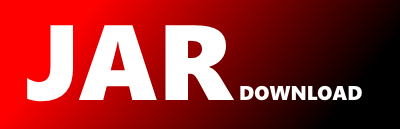
top.hendrixshen.magiclib.util.FabricUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of magiclib-1_14_4 Show documentation
Show all versions of magiclib-1_14_4 Show documentation
To beat magic with magic :(
package top.hendrixshen.magiclib.util;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonToken;
import net.fabricmc.api.EnvType;
import net.fabricmc.loader.api.FabricLoader;
import net.fabricmc.loader.api.ModContainer;
import net.fabricmc.loader.api.Version;
import net.fabricmc.loader.api.metadata.version.VersionPredicate;
import org.jetbrains.annotations.NotNull;
import top.hendrixshen.magiclib.MagicLibReference;
import top.hendrixshen.magiclib.dependency.api.DepCheckException;
import top.hendrixshen.magiclib.dependency.impl.Dependencies;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.net.URL;
import java.nio.charset.StandardCharsets;
import java.util.*;
import java.util.function.BiConsumer;
public class FabricUtil {
// Fabric Loader 0.11 and below support
private static Method fabricLegacyVersionPredicateParser;
private static Method fabricLegacyDisplayCriticalError;
private static Method fabricDisplayCriticalError;
private static Method quiltDisplayCriticalError;
static {
try {
fabricLegacyVersionPredicateParser = Class.forName("net.fabricmc.loader.util.version.VersionPredicateParser").getMethod("matches", Version.class, String.class);
fabricLegacyDisplayCriticalError = Class.forName("net.fabricmc.loader.gui.FabricGuiEntry").getMethod("displayCriticalError", Throwable.class, boolean.class);
} catch (ClassNotFoundException | NoSuchMethodException ignored) {
}
try {
fabricDisplayCriticalError = Class.forName("net.fabricmc.loader.impl.gui.FabricGuiEntry").getMethod("displayCriticalError", Throwable.class, boolean.class);
} catch (ClassNotFoundException | NoSuchMethodException ignored) {
}
try {
quiltDisplayCriticalError = Class.forName("org.quiltmc.loader.impl.gui.QuiltGuiEntry").getMethod("displayError",
String.class, Throwable.class, boolean.class, boolean.class);
} catch (ClassNotFoundException | NoSuchMethodException ignored) {
}
}
/**
* Verify that the Fabric Loader has loaded the qualified mods.
*
* @param version Version provided by the fabric loader.
* @param versionPredicate Semantic versioning expression.
* @return True if the Fabric Loader finds a matching mods from the list of
* loaded mods, false otherwise.
*/
public static boolean isModLoaded(Version version, String versionPredicate) {
try {
if (fabricLegacyVersionPredicateParser != null) {
try {
return (boolean) fabricLegacyVersionPredicateParser.invoke(null, version, versionPredicate);
} catch (InvocationTargetException | IllegalAccessException e) {
MagicLibReference.getLogger().error("Failed to invoke fabricLegacyVersionPredicateParser#matches", e);
throw new RuntimeException(e);
}
} else {
return VersionPredicate.parse(versionPredicate).test(version);
}
} catch (Throwable e) {
MagicLibReference.getLogger().error("Failed to parse version or version predicate {} {}: {}", version.getFriendlyString(), versionPredicate, e);
return false;
}
}
/**
* Verify that the Fabric Loader has loaded the qualified mods.
*
* @param modId Version provided by the fabric loader.
* @return True if the Fabric Loader finds a matching mod from the list of
* loaded mods, false otherwise.
*/
public static boolean isModLoaded(String modId) {
return FabricLoader.getInstance().isModLoaded(modId);
}
/**
* Verify that the Fabric Loader has loaded the qualified mod.
*
* @param modId Version provided by the fabric loader.
* @param versionPredicate Semantic versioning expression.
* @return True if the Fabric Loader finds a matching mod from the list of
* loaded mods, false otherwise.
*/
public static boolean isModLoaded(String modId, String versionPredicate) {
Optional modContainerOptional = FabricLoader.getInstance().getModContainer(modId);
if (modContainerOptional.isPresent()) {
ModContainer modContainer = modContainerOptional.get();
return isModLoaded(modContainer.getMetadata().getVersion(), versionPredicate);
}
return false;
}
public static @NotNull Set getResources(String name) throws IOException {
ClassLoader urlLoader = Thread.currentThread().getContextClassLoader();
HashSet hashSet = new HashSet<>();
Enumeration urlEnumeration = urlLoader.getResources(name);
while (urlEnumeration.hasMoreElements()) {
hashSet.add(urlEnumeration.nextElement());
}
return hashSet;
}
private static @NotNull Map> getModInitDependencies(String entryKey, String entryMethod) {
Map> ret = new HashMap<>();
for (ModMetaData modMetaData : ModMetaData.data.values()) {
for (String entrypointValue : modMetaData.entryPoints.getOrDefault(entryKey, new HashSet<>())) {
Dependencies
© 2015 - 2025 Weber Informatics LLC | Privacy Policy