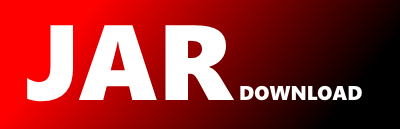
top.hendrixshen.magiclib.impl.i18n.minecraft.translation.MagicTranslation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of magiclib-minecraft-api-1.19.3-fabric Show documentation
Show all versions of magiclib-minecraft-api-1.19.3-fabric Show documentation
Unleash magic into Minecraft, infuse souls, ascend to heaven!
/*
* This file is part of the Carpet TIS Addition project, licensed under the
* GNU Lesser General Public License v3.0
*
* Copyright (C) 2023 Fallen_Breath and contributors
*
* Carpet TIS Addition is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* Carpet TIS Addition is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with Carpet TIS Addition. If not, see .
*/
package top.hendrixshen.magiclib.impl.i18n.minecraft.translation;
import net.minecraft.class_2561;
import net.minecraft.class_2568;
import net.minecraft.class_2588;
import net.minecraft.class_3222;
import net.minecraft.class_5250;
import net.minecraft.network.chat.*;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import top.hendrixshen.magiclib.MagicLib;
import top.hendrixshen.magiclib.api.compat.minecraft.network.chat.MutableComponentCompat;
import top.hendrixshen.magiclib.api.fake.i18n.ServerPlayerLanguage;
import top.hendrixshen.magiclib.api.i18n.I18n;
import top.hendrixshen.magiclib.mixin.minecraft.accessor.StyleAccessor;
import top.hendrixshen.magiclib.util.minecraft.ComponentUtil;
import java.util.List;
/**
* Reference to Carpet-TIS-Addition
*/
public class MagicTranslation {
public static @NotNull MutableComponentCompat translate(MutableComponentCompat text) {
return MagicTranslation.translate(text, I18n.getCurrentLanguageCode());
}
public static @NotNull MutableComponentCompat translate(MutableComponentCompat text, String lang) {
return MagicTranslation.translateComponent(text, lang);
}
public static @NotNull MutableComponentCompat translate(MutableComponentCompat text, class_3222 player) {
return MagicTranslation.translate(text, ((ServerPlayerLanguage) player).magicLib$getLanguage());
}
private static @NotNull MutableComponentCompat translateComponent(@NotNull MutableComponentCompat text,
@NotNull String lang) {
return MutableComponentCompat.of(MagicTranslation.translateComponent(text.get(), lang));
}
private static
//#if MC > 11502
class_5250
//#else
//$$ BaseComponent
//#endif
translateComponent(
//#if MC > 11502
class_5250 text,
//#else
//$$ BaseComponent text,
//#endif
@NotNull String lang
) {
// Quick scan to check if any required translation exists.
boolean[] translationRequired = new boolean[]{false};
MagicTranslation.forEachTranslationComponent(text, lang, (txt, msgKeyString) -> {
translationRequired[0] = true;
return txt;
});
if (!translationRequired[0]) {
return text;
}
// Make a copy of the text, and apply translation.
return MagicTranslation.forEachTranslationComponent(ComponentUtil.copy(text), lang,
(txt, msgKeyString) -> {
//#if MC > 11802
class_2588 content = (class_2588) txt.method_10851();
String txtKey = content.method_11022();
Object[] txtArgs = content.method_11023();
//#else
//$$ String txtKey = txt.getKey();
//$$ Object[] txtArgs = txt.getArgs();
//#endif
if (msgKeyString == null) {
MagicLib.getLogger().warn("MagicTranslation: Unknown translation key {}", txtKey);
return txt;
}
//#if MC > 11502
class_5250 newText;
//#else
//$$ BaseComponent newText;
//#endif
try {
newText = ComponentUtil.format(msgKeyString, txtArgs).get();
} catch (IllegalArgumentException e) {
newText = ComponentUtil.simple(msgKeyString).get();
}
// Migrating text data.
newText.method_10855().addAll(txt.method_10855());
newText.method_10862(txt.method_10866());
return newText;
});
}
private static @NotNull
//#if MC > 11502
class_5250
//#else
//$$ BaseComponent
//#endif
forEachTranslationComponent(
//#if MC > 11502
class_5250 text,
//#else
//$$ BaseComponent text,
//#endif
@NotNull String lang,
ComponentModifier modifier
) {
if (ComponentUtil.getTextContent(text) instanceof
//#if MC > 11802
class_2588
//#else
//$$ TranslatableComponent
//#endif
) {
//#if MC > 11802
class_2588 translatableText = (class_2588) ComponentUtil.getTextContent(text);
//#else
//$$ TranslatableComponent translatableText = (TranslatableComponent) ComponentUtil.getTextContent(text);
//#endif
// Translate arguments
Object[] args = translatableText.method_11023();
for (int i = 0; i < args.length; i++) {
Object arg = args[i];
if (arg instanceof
//#if MC > 11502
class_5250
//#else
//$$ BaseComponent
//#endif
) {
//#if MC > 11502
class_5250 newText = MagicTranslation.forEachTranslationComponent((class_5250) arg,
lang, modifier);
//#else
//$$ BaseComponent newText = MagicTranslation.forEachTranslationComponent((BaseComponent) arg,
//$$ lang, modifier);
//#endif
if (newText != arg) {
args[i] = newText;
}
}
}
// Do translation logic.
if (HookTranslationManager.getInstance().isNamespaceRegistered(translatableText.method_11022())) {
String msgKeyString = I18n.trByCode(lang, translatableText.method_11022());
text = modifier.apply(
//#if MC > 11802
text,
//#else
//$$ translatableText,
//#endif
msgKeyString
);
}
}
// Translate hover text.
class_2568 hoverEvent = ((StyleAccessor) text.method_10866()).getHoverEvent();
if (hoverEvent != null) {
//#if MC > 11502
Object hoverText = hoverEvent.method_10891(hoverEvent.method_10892());
if (hoverEvent.method_10892() == class_2568.class_5247.field_24342 && hoverText instanceof
//#if MC > 11502
class_5250
//#else
//$$ BaseComponent
//#endif
) {
//#if MC > 11502
class_5250 newText = MagicTranslation.forEachTranslationComponent((class_5250) hoverText,
lang, modifier);
//#else
//$$ BaseComponent newText = MagicTranslation.forEachTranslationComponent((BaseComponent) hoverText,
//$$ lang, modifier);
//$$
//#endif
if (newText != hoverText) {
text.method_10862(text.method_10866().method_10949(new class_2568(class_2568.class_5247.field_24342, newText)));
}
}
//#else
//$$ Component hoverText = hoverEvent.getValue();
//$$ BaseComponent newText = MagicTranslation.forEachTranslationComponent((BaseComponent) hoverText,
//$$ lang, modifier);
//$$
//$$ if (newText != hoverText) {
//$$ text.getStyle().setHoverEvent(new HoverEvent(hoverEvent.getAction(), newText));
//$$ }
//#endif
}
// Translate sibling texts.
List siblings = text.method_10855();
for (int i = 0; i < siblings.size(); i++) {
class_2561 sibling = siblings.get(i);
//#if MC > 11502
class_5250 newText = MagicTranslation.forEachTranslationComponent((class_5250) sibling,
lang, modifier);
//#else
//$$ BaseComponent newText = MagicTranslation.forEachTranslationComponent((BaseComponent) sibling,
//$$ lang, modifier);
//#endif
if (newText != sibling) {
siblings.set(i, newText);
}
}
return text;
}
@FunctionalInterface
private interface ComponentModifier {
//#if MC > 11502
class_5250
//#else
//$$ BaseComponent
//#endif
apply(
//#if MC > 11802
class_5250 translatableText,
//#else
//$$ TranslatableComponent translatableText,
//#endif
@Nullable String msgKeyString
);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy