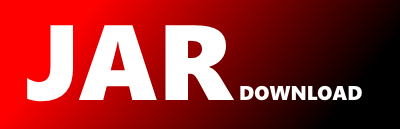
top.hendrixshen.magiclib.util.minecraft.ComponentUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of magiclib-minecraft-api-1.19.3-fabric Show documentation
Show all versions of magiclib-minecraft-api-1.19.3-fabric Show documentation
Unleash magic into Minecraft, infuse souls, ascend to heaven!
/*
* This file is part of the Carpet TIS Addition project, licensed under the
* GNU Lesser General Public License v3.0
*
* Copyright (C) 2023 Fallen_Breath and contributors
*
* Carpet TIS Addition is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* Carpet TIS Addition is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with Carpet TIS Addition. If not, see .
*/
package top.hendrixshen.magiclib.util.minecraft;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.Lists;
import com.google.common.collect.Maps;
import net.minecraft.class_124;
import net.minecraft.class_1297;
import net.minecraft.class_1299;
import net.minecraft.class_1320;
import net.minecraft.class_156;
import net.minecraft.class_1767;
import net.minecraft.class_1792;
import net.minecraft.class_1923;
import net.minecraft.class_2248;
import net.minecraft.class_2382;
import net.minecraft.class_243;
import net.minecraft.class_2558;
import net.minecraft.class_2561;
import net.minecraft.class_2568;
import net.minecraft.class_2586;
import net.minecraft.class_2588;
import net.minecraft.class_2590;
import net.minecraft.class_2680;
import net.minecraft.class_2769;
import net.minecraft.class_2960;
import net.minecraft.class_3610;
import net.minecraft.class_3611;
import net.minecraft.class_5250;
import net.minecraft.class_5251;
import net.minecraft.class_5348;
import net.minecraft.class_7417;
import net.minecraft.network.chat.*;
import org.jetbrains.annotations.Contract;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import top.hendrixshen.magiclib.api.compat.minecraft.network.chat.*;
import top.hendrixshen.magiclib.impl.compat.minecraft.world.level.dimension.DimensionWrapper;
import top.hendrixshen.magiclib.impl.i18n.minecraft.translation.Translator;
import top.hendrixshen.magiclib.mixin.minecraft.accessor.TranslatableComponentAccessor;
import top.hendrixshen.magiclib.util.MiscUtil;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import java.util.function.Consumer;
/**
* Reference to Carpet-TIS-Addition
*/
public class ComponentUtil {
private static final Translator translator = new Translator("magiclib.util.minecraft");
/*
* ----------------------------
* Text Factories - Utils
* ----------------------------
*/
public static
//#if MC > 11802
class_7417
//#elseif MC > 11502
//$$ MutableComponent
//#else
//$$ BaseComponent
//#endif
getTextContent(
//#if MC > 11502
@NotNull class_5250 text
//#else
//$$ @NotNull BaseComponent text
//#endif
) {
//#if MC > 11802
return text.method_10851();
//#else
//$$ return text;
//#endif
}
/*
* ----------------------------
* Component Factories - Utils
* ----------------------------
*/
private static final ImmutableMap> DYE_COLOR_APPLIER = class_156.method_656(() -> {
Map map = Maps.newHashMap();
map.put(class_1767.field_7952, class_124.field_1068);
map.put(class_1767.field_7967, class_124.field_1080);
map.put(class_1767.field_7944, class_124.field_1063);
map.put(class_1767.field_7963, class_124.field_1074);
map.put(class_1767.field_7964, class_124.field_1061);
map.put(class_1767.field_7947, class_124.field_1054);
map.put(class_1767.field_7961, class_124.field_1060);
map.put(class_1767.field_7942, class_124.field_1077);
map.put(class_1767.field_7955, class_124.field_1062);
map.put(class_1767.field_7951, class_124.field_1075);
map.put(class_1767.field_7966, class_124.field_1058);
map.put(class_1767.field_7945, class_124.field_1064);
map.put(class_1767.field_7958, class_124.field_1076);
//#if MC < 11600
//$$ map.put(DyeColor.BROWN, ChatFormatting.DARK_RED);
//$$ map.put(DyeColor.PINK, ChatFormatting.RED);
//$$ map.put(DyeColor.ORANGE, ChatFormatting.GOLD);
//#endif
ImmutableMap.Builder> builder = new ImmutableMap.Builder<>();
map.forEach((dyeColor, fmt) -> builder.put(dyeColor, text -> ComponentUtil.formatting(text, fmt)));
//#if MC > 11502
Arrays.stream(class_1767.values())
.filter(dyeColor -> !map.containsKey(dyeColor))
.forEach(dyeColor -> builder.put(dyeColor, text ->
text.setStyle(text.getStyle().withColor(class_5251.method_27717(dyeColor.method_16357())))));
//#endif
return builder.build();
});
public static @NotNull MutableComponentCompat compose(Object @NotNull ... objects) {
MutableComponentCompat literal = ComponentUtil.empty();
for (Object o : objects) {
if (o instanceof MutableComponentCompat) {
literal.append((MutableComponentCompat) o);
} else if (o instanceof
//#if MC > 11502
class_5250
//#else
//$$ BaseComponent
//#endif
) {
literal.append(MutableComponentCompat.of(MiscUtil.cast(o)));
} else {
literal.append(o.toString());
}
}
return literal;
}
// Simple Component
public static @NotNull MutableComponentCompat simple(@NotNull Object text) {
return ComponentCompat.literal(text.toString());
}
// Simple Component with formatting
public static @NotNull MutableComponentCompat simple(Object text, class_124... chatFormattings) {
return ComponentUtil.formatting(ComponentUtil.simple(text), chatFormattings);
}
public static @NotNull MutableComponentCompat empty() {
return ComponentUtil.simple("");
}
public static @NotNull MutableComponentCompat newLine() {
return ComponentUtil.simple("\n");
}
public static MutableComponentCompat colored(MutableComponentCompat text, class_1767 value) {
Consumer consumer = ComponentUtil.DYE_COLOR_APPLIER.get(value);
if (consumer != null) {
consumer.accept(text);
}
return text;
}
public static MutableComponentCompat colored(MutableComponentCompat text, Object value) {
class_124 color = null;
if (Boolean.TRUE.equals(value)) {
color = class_124.field_1060;
} else if (Boolean.FALSE.equals(value)) {
color = class_124.field_1061;
}
if (value instanceof Number) {
color = class_124.field_1065;
}
if (color != null) {
ComponentUtil.formatting(text, color);
}
return text;
}
public static MutableComponentCompat colored(Object value) {
return ComponentUtil.colored(ComponentUtil.simple(value), value);
}
public static MutableComponentCompat property(class_2769> property, Object value) {
return ComponentUtil.colored(ComponentUtil.simple(TextUtil.property(property, value)), value);
}
public static @NotNull MutableComponentCompat tr(String key, Object... args) {
for (int i = 0; i < args.length; i++) {
if (args[i] instanceof ComponentCompat) {
args[i] = ((ComponentCompat) args[i]).get();
}
}
return ComponentCompat.translatable(key, args);
}
public static @NotNull MutableComponentCompat fancy(MutableComponentCompat displayText,
MutableComponentCompat hoverText,
ClickEventCompat clickEvent) {
MutableComponentCompat text = ComponentUtil.copy(displayText);
if (hoverText != null) {
ComponentUtil.hover(text, hoverText);
}
if (clickEvent != null) {
ComponentUtil.click(text, clickEvent);
}
return text;
}
public static @NotNull MutableComponentCompat join(MutableComponentCompat joiner,
@NotNull Iterable items) {
MutableComponentCompat text = ComponentUtil.empty();
boolean first = true;
for (ComponentCompat item : items) {
if (!first) {
text.append(joiner);
}
first = false;
text.append(item);
}
return text;
}
public static @NotNull MutableComponentCompat join(MutableComponentCompat joiner, ComponentCompat... items) {
return ComponentUtil.join(joiner, Arrays.asList(items));
}
public static @NotNull MutableComponentCompat format(String formatter, Object... args) {
TranslatableComponentAccessor dummy = (TranslatableComponentAccessor) (
ComponentUtil.tr(formatter, args).get()
//#if MC > 11802
.method_10851()
//#endif
);
try {
//#if MC > 11701
List segments = Lists.newArrayList();
dummy.magiclib$invokeDecomposeTemplate(formatter, segments::add);
//#else
//$$ dummy.magiclib$getDecomposedParts().clear();
//$$ dummy.magiclib$invokeDecomposeTemplate(formatter);
//#endif
return ComponentUtil.compose(
//#if MC > 11502
//#if MC > 11701
segments.
//#else
//$$ dummy.magiclib$getDecomposedParts().
//#endif
stream().map(formattedText -> {
if (formattedText instanceof
//#if MC > 11802
class_2561
//#else
//$$ MutableComponent
//#endif
) {
//#if MC > 11802
return (class_2561) formattedText;
//#else
//$$ return formattedText;
//#endif
}
return ComponentUtil.simple(formattedText.getString());
}).toArray()
//#else
//$$ dummy.magiclib$getDecomposedParts().toArray(new Object[0])
//#endif
);
} catch (class_2590 e) {
throw new IllegalArgumentException(formatter);
}
}
/*
* -------------------------------
* Component Factories - Advanced
* -------------------------------
*/
public static @NotNull MutableComponentCompat bool(boolean bool) {
return ComponentUtil.simple(String.valueOf(bool), bool ? class_124.field_1060 : class_124.field_1061);
}
private static MutableComponentCompat getTeleportHint(MutableComponentCompat dest) {
return ComponentUtil.translator.tr("teleport_hint.hover", dest);
}
private static @NotNull MutableComponentCompat coordinate(@Nullable DimensionWrapper dim, String posStr, String command) {
MutableComponentCompat hoverText = ComponentUtil.empty();
hoverText.append(ComponentUtil.getTeleportHint(ComponentUtil.simple(posStr)));
if (dim != null) {
hoverText.append("\n");
hoverText.append(ComponentUtil.translator.tr("teleport_hint.dimension"));
hoverText.append(": ");
hoverText.append(ComponentUtil.dimension(dim));
}
return ComponentUtil.fancy(ComponentUtil.simple(posStr), hoverText, ClickEventCompat.of(class_2558.class_2559.field_11745, command));
}
public static @NotNull MutableComponentCompat coordinate(class_243 pos, DimensionWrapper dim) {
return ComponentUtil.coordinate(dim, TextUtil.coordinate(pos), TextUtil.tp(pos, dim));
}
public static @NotNull MutableComponentCompat coordinate(class_2382 pos, DimensionWrapper dim) {
return ComponentUtil.coordinate(dim, TextUtil.coordinate(pos), TextUtil.tp(pos, dim));
}
public static @NotNull MutableComponentCompat coordinate(class_1923 pos, DimensionWrapper dim) {
return ComponentUtil.coordinate(dim, TextUtil.coordinate(pos), TextUtil.tp(pos, dim));
}
public static @NotNull MutableComponentCompat coordinate(class_243 pos) {
return ComponentUtil.coordinate(null, TextUtil.coordinate(pos), TextUtil.tp(pos));
}
public static @NotNull MutableComponentCompat coordinate(class_2382 pos) {
return ComponentUtil.coordinate(null, TextUtil.coordinate(pos), TextUtil.tp(pos));
}
public static @NotNull MutableComponentCompat coordinate(class_1923 pos) {
return ComponentUtil.coordinate(null, TextUtil.coordinate(pos), TextUtil.tp(pos));
}
private static @NotNull MutableComponentCompat vector(String displayText, String detailedText) {
return ComponentUtil.fancy(ComponentUtil.simple(displayText),
ComponentUtil.simple(detailedText), ClickEventCompat.of(class_2558.class_2559.field_11745, detailedText));
}
public static @NotNull MutableComponentCompat vector(class_243 vec) {
return ComponentUtil.vector(TextUtil.vector(vec), TextUtil.vector(vec, 6));
}
public static @NotNull MutableComponentCompat entityType(@NotNull class_1299> entityType) {
return MutableComponentCompat.of(MiscUtil.cast(entityType.method_5897()));
}
public static @NotNull MutableComponentCompat entityType(@NotNull class_1297 entity) {
return ComponentUtil.entityType(entity.method_5864());
}
public static @NotNull MutableComponentCompat entity(class_1297 entity) {
MutableComponentCompat entityBaseName = ComponentUtil.entityType(entity);
MutableComponentCompat entityDisplayName = MutableComponentCompat.of(MiscUtil.cast(entity.method_5477()));
MutableComponentCompat hoverText = ComponentUtil.compose(ComponentUtil.translator.tr("entity_type", entityBaseName, ComponentUtil.simple(class_1299.method_5890(entity.method_5864()).toString())), ComponentUtil.newLine(), ComponentUtil.getTeleportHint(entityDisplayName));
return ComponentUtil.fancy(entityDisplayName, hoverText, ClickEventCompat.of(class_2558.class_2559.field_11745, TextUtil.tp(entity)));
}
public static @NotNull MutableComponentCompat attribute(@NotNull class_1320 attribute) {
return ComponentUtil.tr(
//#if MC > 11600
attribute.method_26830()
//#else
//$$ "attribute.name." + attribute.getName()
//#endif
);
}
//#if MC > 12004
//$$ public static MutableComponentCompat attribute(Holder attribute) {
//$$ return ComponentUtil.attribute(attribute.value());
//$$ }
//#endif
private static final ImmutableMap DIMENSION_NAME = ImmutableMap.of(
DimensionWrapper.OVERWORLD, ComponentUtil.tr(
//#if MC > 11802
"flat_world_preset.minecraft.overworld"
//#else
//$$ "createWorld.customize.preset.overworld"
//#endif
), DimensionWrapper.NETHER, ComponentUtil.tr("advancements.nether.root.title"),
DimensionWrapper.THE_END, ComponentUtil.tr("advancements.end.root.title"));
public static MutableComponentCompat dimension(DimensionWrapper dim) {
MutableComponentCompat dimText = ComponentUtil.DIMENSION_NAME.get(dim);
return dimText != null ? ComponentUtil.copy(dimText) : ComponentUtil.simple(dim.getResourceLocationString());
}
public static @NotNull MutableComponentCompat getColoredDimensionSymbol(@NotNull DimensionWrapper dimensionType) {
if (dimensionType.equals(DimensionWrapper.OVERWORLD)) {
return ComponentUtil.simple("O", class_124.field_1077);
}
if (dimensionType.equals(DimensionWrapper.NETHER)) {
return ComponentUtil.simple("N", class_124.field_1079);
}
if (dimensionType.equals(DimensionWrapper.THE_END)) {
return ComponentUtil.simple("E", class_124.field_1064);
}
return ComponentUtil.simple(dimensionType.getResourceLocationString().toUpperCase().substring(0, 1));
}
public static @NotNull MutableComponentCompat block(@NotNull class_2248 block) {
return ComponentUtil.hover(ComponentUtil.tr(block.method_9539()), ComponentUtil.simple(TextUtil.block(block)));
}
public static @NotNull MutableComponentCompat block(@NotNull class_2680 blockState) {
List hovers = Lists.newArrayList();
hovers.add(ComponentUtil.simple(TextUtil.block(blockState.method_26204())));
for (class_2769> property : blockState.method_28501()) {
hovers.add(ComponentUtil.compose(ComponentUtil.simple(property.method_11899()), ComponentUtil.simple(" : ").withStyle(style -> style.withColor(class_124.field_1080)), ComponentUtil.property(property, blockState.method_11654(property))));
}
return ComponentUtil.fancy(ComponentUtil.block(blockState.method_26204()), ComponentUtil.join(ComponentUtil.newLine(), hovers.toArray(new MutableComponentCompat[0])), ClickEventCompat.of(class_2558.class_2559.field_11745, TextUtil.block(blockState)));
}
public static @NotNull MutableComponentCompat fluid(@NotNull class_3611 fluid) {
return ComponentUtil.hover(ComponentUtil.block(fluid.method_15785().method_15759().method_26204()), ComponentUtil.simple(ResourceLocationUtil.id(fluid).toString()));
}
public static @NotNull MutableComponentCompat fluid(@NotNull class_3610 fluid) {
return ComponentUtil.fluid(fluid.method_15772());
}
public static @NotNull MutableComponentCompat blockEntity(@NotNull class_2586 blockEntity) {
class_2960 id = ResourceLocationUtil.id(blockEntity.method_11017());
return ComponentUtil.simple(id != null ? id.toString() : // vanilla block entity
blockEntity.getClass().getSimpleName() // modded block entity, assuming the class name is not obfuscated
);
}
public static @NotNull MutableComponentCompat item(@NotNull class_1792 item) {
return ComponentUtil.tr(item.method_7876());
}
public static MutableComponentCompat color(@NotNull class_1767 color) {
return ComponentUtil.translator.tr("color." + color.method_7792().toLowerCase());
}
/*
* --------------------
* Text Modifiers
* --------------------
*/
public static @NotNull MutableComponentCompat hover(@NotNull MutableComponentCompat text, HoverEventCompat hoverEvent) {
text.withStyle(style -> style.withHoverEvent(hoverEvent));
return text;
}
public static @NotNull MutableComponentCompat hover(MutableComponentCompat text, MutableComponentCompat hoverText) {
return ComponentUtil.hover(text, HoverEventCompat.of(class_2568.class_5247.field_24342, hoverText));
}
public static @NotNull MutableComponentCompat click(@NotNull MutableComponentCompat text, ClickEventCompat clickEvent) {
text.withStyle(style -> style.withClickEvent(clickEvent));
return text;
}
public static @NotNull MutableComponentCompat formatting(@NotNull MutableComponentCompat text, class_124... formattings) {
text.withStyle(formattings);
return text;
}
public static @NotNull MutableComponentCompat style(@NotNull MutableComponentCompat text, StyleCompat style) {
text.setStyle(style);
return text;
}
public static @NotNull MutableComponentCompat copy(@NotNull MutableComponentCompat text) {
return MutableComponentCompat.of(ComponentUtil.copy(text.get()));
}
public static @NotNull
//#if MC > 11502
class_5250
//#else
//$$ BaseComponent
//#endif
copy(
//#if MC > 11502
@NotNull class_5250 text
//#else
//$$ @NotNull BaseComponent text
//#endif
) {
//#if MC > 11502
class_5250 copied;
//#else
//$$ BaseComponent copied;
//#endif
//#if MC > 11802
copied = text.method_27661();
//#elseif MC > 11502
//$$ copied = text.copy();
//#else
//$$ copied = (BaseComponent) text.deepCopy();
//#endif
// mc1.16+ doesn't make a copy of args of a TranslatableText,
// so we need to copy that by ourselves.
//#if MC > 11502
if (ComponentUtil.getTextContent(copied) instanceof
//#if MC > 11802
class_2588
//#else
//$$ TranslatableComponent
//#endif
) {
//#if MC > 11802
class_2588 translatableText = (class_2588) ComponentUtil.getTextContent(copied);
//#else
//$$ TranslatableComponent translatableText = (TranslatableComponent) ComponentUtil.getTextContent(copied);
//#endif
Object[] args = translatableText.method_11023().clone();
for (int i = 0; i < args.length; i++) {
if (args[i] instanceof
//#if MC > 11502
class_5250
//#else
//$$ BaseComponent
//#endif
) {
args[i] = ComponentUtil.copy(
//#if MC > 11502
(class_5250) args[i]
//#else
//$$ (BaseComponent) args[i]
//#endif
);
}
}
((TranslatableComponentAccessor) translatableText).magiclib$setArgs(args);
}
//#endif
return copied;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy