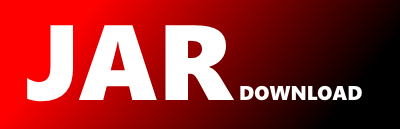
top.jfunc.json.impl.BaseListJSONArray Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Json-Gson Show documentation
Show all versions of Json-Gson Show documentation
common json interface implements by Gson
The newest version!
package top.jfunc.json.impl;
import top.jfunc.json.JsonArray;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.util.*;
/**
* 基于List的JSONArray实现基类
* @author xiongshiyan at 2018/6/11
*/
public abstract class BaseListJSONArray extends BaseJson implements JsonArray {
protected List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy