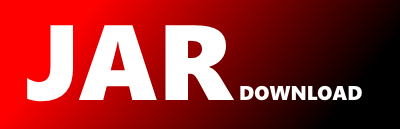
top.jfunc.common.http.basic.OkHttp3Client Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of httpclient Show documentation
Show all versions of httpclient Show documentation
http客户端请求工具类,有多种实现:OkHttp3、ApacheHttpClient、HttpURLConnection、Jodd-Http,可以随意切换http实现
package top.jfunc.common.http.basic;
import okhttp3.Headers;
import okhttp3.MultipartBody;
import top.jfunc.common.http.Method;
import top.jfunc.common.http.ParamUtil;
import top.jfunc.common.http.base.AbstractOkHttp3HttpTemplate;
import top.jfunc.common.http.base.Config;
import top.jfunc.common.utils.ArrayListMultimap;
import top.jfunc.common.utils.IoUtil;
import java.io.File;
import java.io.IOException;
import java.util.Map;
/**
* @author xiongshiyan at 2018/1/11
*/
public class OkHttp3Client extends AbstractOkHttp3HttpTemplate implements HttpClient {
@Override
public OkHttp3Client setConfig(Config config) {
super.setConfig(config);
return this;
}
@Override
public String get(String url, Map params, Map headers, Integer connectTimeout, Integer readTimeout, String resultCharset) throws IOException {
return template(ParamUtil.contactUrlParams(url , params , getDefaultBodyCharset()),Method.GET,null,null,
ArrayListMultimap.fromMap(headers),
connectTimeout,readTimeout,resultCharset,false,(s,b,r,h)-> IoUtil.read(b ,r));
}
/**
* @param bodyCharset 请求体的编码,不支持,需要在contentType中指定
*/
@Override
public String post(String url, String body, String contentType, Map headers, Integer connectTimeout, Integer readTimeout, String bodyCharset, String resultCharset) throws IOException {
return template(url, Method.POST, contentType, d->setRequestBody(d , Method.POST , stringBody(body , contentType)) ,
ArrayListMultimap.fromMap(headers),
connectTimeout,readTimeout,resultCharset,false,
(s,b,r,h)-> IoUtil.read(b ,r));
}
@Override
public byte[] getAsBytes(String url, ArrayListMultimap headers, Integer connectTimeout, Integer readTimeout) throws IOException {
return template(url,Method.GET,null,null, headers ,
connectTimeout,readTimeout,null,false,
(s,b,r,h)-> IoUtil.stream2Bytes(b));
}
@Override
public File getAsFile(String url, ArrayListMultimap headers, File file, Integer connectTimeout, Integer readTimeout) throws IOException {
return template(url,Method.GET,null,null, headers ,
connectTimeout,readTimeout,null,false,
(s,b,r,h)-> IoUtil.copy2File(b, file));
}
@Override
public String upload(String url, ArrayListMultimap headers, Integer connectTimeout, Integer readTimeout, String resultCharset, FormFile... files) throws IOException {
MultipartBody requestBody = getFilesBody(files);
return template(url, Method.POST, null , d->setRequestBody(d, Method.POST , requestBody), headers,
connectTimeout,readTimeout,resultCharset,false,
(s,b,r,h)-> IoUtil.read(b ,r));
}
protected MultipartBody getFilesBody(FormFile... files) {
MultipartBody.Builder builder = new MultipartBody.Builder().setType(MultipartBody.FORM);
for (FormFile formFile : files) {
builder.addPart(Headers.of("Content-Disposition", "form-data; name=\"" + formFile.getParameterName() + "\"; filename=\"" + formFile.getFilName() + "\"") ,
inputStreamBody(formFile.getContentType() , formFile.getInStream() , formFile.getFileLen()));
}
return builder.build();
}
@Override
public String upload(String url, ArrayListMultimap params, ArrayListMultimap headers, Integer connectTimeout, Integer readTimeout, String resultCharset , FormFile... files) throws IOException {
MultipartBody requestBody = getFilesBody(params , files);
return template(url, Method.POST, null , d->setRequestBody(d, Method.POST , requestBody), headers,
connectTimeout,readTimeout,resultCharset,false,
(s,b,r,h)-> IoUtil.read(b ,r));
}
protected MultipartBody getFilesBody(ArrayListMultimap params , FormFile... files) {
MultipartBody.Builder builder = new MultipartBody.Builder().setType(MultipartBody.FORM);
if(null != params){
params.keySet().forEach(key->params.get(key).forEach(value->builder.addFormDataPart(key , value)));
}
for (FormFile formFile : files) {
builder.addPart(Headers.of("Content-Disposition", "form-data; name=\"" + formFile.getParameterName() + "\"; filename=\"" + formFile.getFilName() + "\"") ,
inputStreamBody(formFile.getContentType() , formFile.getInStream() , formFile.getFileLen()));
}
return builder.build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy