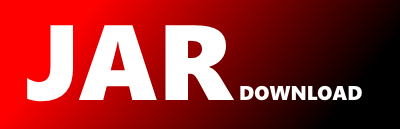
top.lingkang.finalsql.sql.HumpSql Maven / Gradle / Ivy
The newest version!
package top.lingkang.finalsql.sql;
import top.lingkang.finalsql.error.FinalException;
import java.util.List;
import java.util.Map;
/**
* @author lingkang
* 2023/2/27
* 驼峰sql,将驼峰命名自动转化字段为下划线执行
* 例如 select userId from user -> select user_id from user
* 需要注意,若sql的关键词也用大小写混合,将会被转换,可能会执行sql错误:
* select userId fRom user -> select user_id f_rom user
**/
public interface HumpSql {
List nativeHumpSelectForList(String sql, Class resultClass);
List nativeHumpSelectForList(String sql, Class resultClass, Object... param);
List nativeHumpSelectForList(String sql, Class resultClass, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy