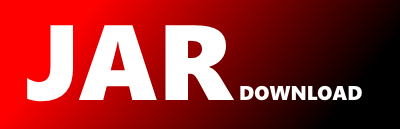
top.lingkang.finalsql.ui.GenerateUtils Maven / Gradle / Ivy
The newest version!
package top.lingkang.finalsql.ui;
import top.lingkang.finalsql.utils.NameUtils;
import java.io.*;
import java.net.URL;
import java.nio.charset.StandardCharsets;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashSet;
import java.util.List;
/**
* @author lingkang
* Created by 2022/11/8
*/
class GenerateUtils {
public static DbColumnTypeToJavaType columnTypeToJavaType = new DefaultDbColumnTypeToJavaType();
public static String getBasePath() {
URL xmlpath = GenerateUtils.class.getResource("/");
String path = xmlpath.getPath().substring(1);
if (path.contains("/target/classes/"))
path = path.substring(0, path.indexOf("/target/classes/"));
return path;
}
public static String getPage(String path) {
path = path.replaceAll("\\\\", ".");
path = path.replaceAll("/", ".");
int index = path.indexOf("main.java");
if (index != -1) {
return path.substring(index + 10);
}
index = path.indexOf("test.java");
if (index != -1) {
return path.substring(index + 10);
}
index = path.indexOf("src");
if (index != -1) {
return path.substring(index + 4);
}
return "";
}
public static String readFile(InputStream inputStream) {
// InputStream in = GenerateBuildMysql.class.getClassLoader().getResourceAsStream("template-mysql5.7.txt");
try {
BufferedReader reader = new BufferedReader(new InputStreamReader(inputStream, StandardCharsets.UTF_8));
String result = "";
for (; ; ) {
String s = reader.readLine();
if (s == null)
break;
result += s + "\n";
}
inputStream.close();
reader.close();
return result;
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
public static String fileRead(File file) {
try {
if (file.exists()) {
// 防止乱码 GB18030/GBK/UTF-8
InputStreamReader isr = new InputStreamReader(new FileInputStream(file), StandardCharsets.UTF_8);
BufferedReader bf = new BufferedReader(isr);
StringBuilder res = new StringBuilder();
String cc = null;
while ((cc = bf.readLine()) != null) {
res.append(cc);
}
bf.close();
isr.close();
return res.toString();
}
} catch (IOException e) {
}
return null;
}
public static List fileReadByLine(File file) {
try {
if (file.exists()) {
// 防止乱码 GB18030/GBK/UTF-8
InputStreamReader isr = new InputStreamReader(new FileInputStream(file), StandardCharsets.UTF_8);
BufferedReader bf = new BufferedReader(isr);
List res = new ArrayList<>();
String cc = null;
while ((cc = bf.readLine()) != null) {
res.add(cc);
}
bf.close();
isr.close();
return res;
}
} catch (IOException e) {
}
return null;
}
public static List stringReadByLine(String str) {
String[] split = str.split("\n");
return new ArrayList<>(Arrays.asList(split));
}
public static boolean isBlank(String str) {
return str == null || str.length() == 0;
}
public static String handlerTableName(String name, String ignoreTablePrefix) {
if (name.length() == 1)
return name.toUpperCase();
if (!isBlank(ignoreTablePrefix)) {
String[] split = ignoreTablePrefix.replace(" ", "").split(",");
for (String ig : split) {
if (isBlank(ig))
continue;
if (name.startsWith(ig)) {
name = name.substring(ig.length());
break;
}
}
}
// f_user_role → fStoreSession
name = NameUtils.toHump(name);
// fStoreSession → FStoreSession
return name.substring(0, 1).toUpperCase() + name.substring(1);
}
public static GenerateColumn columnTypeToJavaType(String columnName, String columnType, String dbType) {
GenerateColumn column = new GenerateColumn();
columnType = columnType.toLowerCase();
DbToJava match = columnTypeToJavaType.match(columnName, columnType, dbType);
column.setType(match.getType());
column.setImportName(match.getImportName());
column.setName(NameUtils.toHump(columnName));
column.setColumnName(columnName);
return column;
}
public static String columnToString(List columns, int keyNumber) {
StringBuffer buffer = new StringBuffer();
for (GenerateColumn column : columns) {
// 注释
if (!isBlank(column.getComment())) {
buffer.append(" // " + column.getComment());
buffer.append("\n");
}
// 主键
if (keyNumber == 1 && column.isKey()) {
buffer.append(" @Id");
} else {
if (column.getName().equals(column.getColumnName()))
buffer.append(" @Column");
else
buffer.append(" @Column(\"" + column.getColumnName() + "\")");
}
buffer.append("\n");
// 字段
buffer.append(" private " + column.getType() + " " + column.getName() + ";");
buffer.append("\n\n");
}
return buffer.toString();
}
public static void writeFile(File file, String content) {
try {
if (!file.exists())
file.createNewFile();
OutputStreamWriter out = new OutputStreamWriter(new FileOutputStream(file), StandardCharsets.UTF_8);
out.write(content);
out.close();
} catch (IOException e) {
throw new RuntimeException(e);
}
}
public static String hasSomeName(String name, HashSet hasName) {
if (hasName.contains(name)) {
int index = name.lastIndexOf("_");
if (index != -1 && index + 1 <= name.length()) {
int i = Integer.parseInt(name.substring(index + 1));
i++;
name = name.substring(0, index + 1) + i;
} else {
name += "_1";
}
return hasSomeName(name, hasName);
}
return name;
}
public static void checkGenerateProperties(GenerateProperties properties) {
if (isBlank(properties.getOutDir()))
throw new RuntimeException("输出的entity目录不能为空!");
System.out.println("输出的实体目录:" + properties.getOutDir());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy