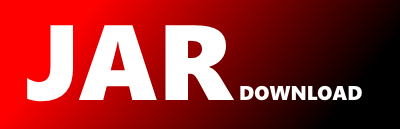
com.memfactory.auth.util.HttpUtil Maven / Gradle / Ivy
package com.memfactory.auth.util;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.NameValuePair;
import org.apache.http.client.HttpClient;
import org.apache.http.client.config.RequestConfig;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.HttpClientBuilder;
import org.apache.http.message.BasicNameValuePair;
import org.apache.http.util.EntityUtils;
public final class HttpUtil {
private HttpUtil() {
}
public static RequestConfig getConfig() {
return RequestConfig.custom().setConnectTimeout(500).setConnectionRequestTimeout(5000).build();
}
public static String post(String url, Map params, Map headers) {
RequestConfig requestConfig = getConfig();
HttpClient httpClient = null;
HttpResponse httpResponse = null;
HttpPost httpPost = new HttpPost(url);
if (headers != null && !headers.isEmpty()) {
for (Map.Entry header : headers.entrySet()) {
httpPost.setHeader(header.getKey(), header.getValue());
}
}
try {
if (params != null && !params.isEmpty()) {
List list = new LinkedList();
for (Map.Entry param : params.entrySet()) {
list.add(new BasicNameValuePair(param.getKey(), param.getValue()));
}
UrlEncodedFormEntity formEntity = new UrlEncodedFormEntity(list, "utf-8");
httpPost.setEntity(formEntity);
}
httpClient = HttpClientBuilder.create().setDefaultRequestConfig(requestConfig).build();
httpResponse = httpClient.execute(httpPost);
HttpEntity httpEntity = httpResponse.getEntity();
return EntityUtils.toString(httpEntity, "utf-8");
} catch (Exception e) {
throw new RuntimeException("got error from http post:" + url, e);
}
}
public static String postBody(String url, String json) {
RequestConfig requestConfig = getConfig();
HttpClient httpClient = null;
HttpResponse httpResponse = null;
HttpPost httpPost = new HttpPost(url);
try {
StringEntity postString = new StringEntity(json);
httpPost.setEntity(postString);
httpPost.setHeader("Content-type", "application/json");
httpClient = HttpClientBuilder.create().setDefaultRequestConfig(requestConfig).build();
httpResponse = httpClient.execute(httpPost);
HttpEntity httpEntity = httpResponse.getEntity();
return EntityUtils.toString(httpEntity, "utf-8");
} catch (Exception e) {
throw new RuntimeException("got error from http post:" + url, e);
}
}
public static String get(String url, Map params, Map headers) {
RequestConfig requestConfig = getConfig();
if (params != null && !params.isEmpty()) {
String paramUrl = "";
for (Map.Entry param : params.entrySet()) {
paramUrl += "&" + param.getKey() + "=" + param.getValue();
}
paramUrl = paramUrl.substring(1);
url += url.contains("?") ? paramUrl : ("?" + paramUrl);
}
HttpGet httpGet = new HttpGet(url);
if (headers != null && !headers.isEmpty()) {
for (Map.Entry header : headers.entrySet()) {
httpGet.setHeader(header.getKey(), header.getValue());
}
}
HttpClient httpClient = null;
HttpResponse httpResponse = null;
try {
httpClient = HttpClientBuilder.create().setDefaultRequestConfig(requestConfig).build();
httpResponse = httpClient.execute(httpGet);
HttpEntity entity = httpResponse.getEntity();
return EntityUtils.toString(entity, "UTF-8");
} catch (Exception e) {
throw new RuntimeException("got error from http get:" + url, e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy