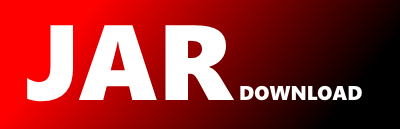
top.netkit.redis.client.command.KeyCommandExecutor Maven / Gradle / Ivy
The newest version!
package top.netkit.redis.client.command;
import top.netkit.redis.client.executor.VoidExecutor;
import java.util.Collection;
/**
* redis key command executor
* @author shixinke
*/
public interface KeyCommandExecutor {
/**
* delete key
* @param key redis key
* @return boolean
*/
boolean del(String key);
/**
* multi delete keys
* @param keys redis keys
* @return boolean
*/
boolean del(Collection keys);
/**
* check the key is exist
* @param key redis key
* @return boolean
*/
boolean exists(String key);
/**
* set expire time of the key
* @param key redis key
* @param seconds expire interval times
* @return boolean
*/
boolean expire(String key, Long seconds);
/**
* set expire time of key
* @param key redis key
* @param timestamp expire time
* @return boolean
*/
boolean expireAt(String key, Integer timestamp);
/**
* set expire time of the key(mills)
* @param key redis key
* @param mills expire time
* @return boolean
*/
boolean pExpire(String key, Long mills);
/**
* set expire time of the key(mills)
* @param key redis key
* @param timestampMills expire time
* @return boolean
*/
boolean pExpireAt(String key, Long timestampMills);
/**
* get the expire time of the key(seconds)
* @param key redis key
* @return long
*/
Long ttl(String key);
/**
* get the expire time of the key(mills)
* @param key redis key
* @return long
*/
Long pTtl(String key);
/**
* set the key is persist(never expired)
* @param key redis key
* @return boolean
*/
boolean persist(String key);
/**
* rename the key
* @param originalKey original key
* @param newKey new key
* @return boolean
*/
boolean rename(String originalKey, String newKey);
/**
* rename the key if the key is not exist
* @param originalKey original key
* @param newKey new key
* @return boolean
*/
boolean renameNx(String originalKey, String newKey);
/**
* get a key by random
* @return String
*/
String randomKey();
/**
* get the type of the key
* @param key redis key
* @return String
*/
String type(String key);
/**
* get keys by key pattern
* @param pattern key pattern
* @return Iterable
*/
Iterable keys(String pattern);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy