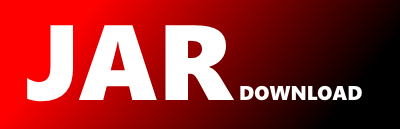
top.netkit.redis.client.configuration.RedisConfig Maven / Gradle / Ivy
package top.netkit.redis.client.configuration;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.core.JsonGenerator;
import com.fasterxml.jackson.core.JsonParser;
import com.fasterxml.jackson.databind.DeserializationFeature;
import com.fasterxml.jackson.databind.MapperFeature;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.SerializationFeature;
import org.redisson.codec.JsonJacksonCodec;
import java.util.List;
/**
* redis configuration
* @author shixinke
*/
public class RedisConfig {
/**
* redis database
*/
private int database;
/**
* redis server hostname
*/
private String host;
/**
* redis server port
*/
private int port;
/**
* redis server auth password
*/
private String password;
/**
* redis server connect timeout
*/
private int connectTimeout;
/**
* redis server response timeout
*/
private int timeout;
/**
* redis max connections
*/
private int maxActiveConn;
/**
* redis min idle pool size
*/
private int minIdle;
/**
* redis poll max wait time
*/
private Integer maxWait;
/**
* redis max idle pool size
*/
private Integer maxIdle;
/**
* keepalive
*/
private boolean keepalive;
/**
* master-slave config
*/
private MasterSlaveConfig masterSlave;
/**
* sentinel config
*/
private SentinelConfig sentinel;
/**
* running mode : single(default),masterSlave,sentinel,cluster
*/
private String mode;
public static class MasterSlaveConfig {
/**
* master address
*/
private String masterAddress;
/**
* slave address
*/
private List slaveAddresses;
public String getMasterAddress() {
return masterAddress;
}
public void setMasterAddress(String masterAddress) {
this.masterAddress = masterAddress;
}
public List getSlaveAddresses() {
return slaveAddresses;
}
public void setSlaveAddresses(List slaveAddresses) {
this.slaveAddresses = slaveAddresses;
}
}
public static class SentinelConfig {
/**
* sentinel address list
*/
private List address;
/**
* sentinel password
*/
private String password;
}
public enum RunningMode {
/**
* single
*/
STANDALONE,
/**
* master-slave
*/
MASTER_SLAVE,
/**
* sentinel
*/
SENTINEL,
/**
* cluster
*/
CLUSTER;
public static RunningMode get(String value) {
if (value != null) {
value = value.replace("-", "_");
}
for (RunningMode mode : values()) {
if (mode.toString().equalsIgnoreCase(value)) {
return mode;
}
}
return RunningMode.STANDALONE;
}
}
public int getDatabase() {
return database;
}
public void setDatabase(int database) {
this.database = database;
}
public String getHost() {
return host;
}
public void setHost(String host) {
this.host = host;
}
public int getPort() {
return port;
}
public void setPort(int port) {
this.port = port;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public int getTimeout() {
return timeout;
}
public void setTimeout(int timeout) {
this.timeout = timeout;
}
public int getMaxActiveConn() {
return maxActiveConn;
}
public void setMaxActiveConn(int maxActiveConn) {
this.maxActiveConn = maxActiveConn;
}
public int getMinIdle() {
return minIdle;
}
public void setMinIdle(int minIdle) {
this.minIdle = minIdle;
}
public Integer getMaxWait() {
return maxWait;
}
public void setMaxWait(Integer maxWait) {
this.maxWait = maxWait;
}
public Integer getMaxIdle() {
return maxIdle;
}
public void setMaxIdle(Integer maxIdle) {
this.maxIdle = maxIdle;
}
public int getConnectTimeout() {
return connectTimeout;
}
public void setConnectTimeout(int connectTimeout) {
this.connectTimeout = connectTimeout;
}
public String getMode() {
return mode;
}
public void setMode(String mode) {
this.mode = mode;
}
public boolean isKeepalive() {
return keepalive;
}
public void setKeepalive(boolean keepalive) {
this.keepalive = keepalive;
}
public MasterSlaveConfig getMasterSlave() {
return masterSlave;
}
public void setMasterSlave(MasterSlaveConfig masterSlave) {
this.masterSlave = masterSlave;
}
public SentinelConfig getSentinel() {
return sentinel;
}
public void setSentinel(SentinelConfig sentinel) {
this.sentinel = sentinel;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy