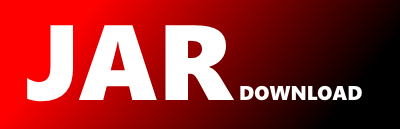
top.osjf.sdk.spring.proxy.ProxyUtils Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2024-? the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package top.osjf.sdk.spring.proxy;
import org.springframework.cglib.proxy.Callback;
import org.springframework.cglib.proxy.Enhancer;
import org.springframework.lang.NonNull;
import java.lang.reflect.InvocationHandler;
import java.lang.reflect.Proxy;
/**
* Proxy related tools.
*
* @author zhangpengfei
* @since 1.0.0
*/
@SuppressWarnings("unchecked")
public abstract class ProxyUtils {
/**
* Using an interface {@code class} and {@code InvocationHandler} build a JDK dynamic proxy object.
*
* @param clazz Proxy type.
* @param invocationHandler Callback interface.
* @param type.
* @return JDK dynamic proxy object
*/
public static T createJdkProxy(Class clazz, InvocationHandler invocationHandler) {
if (!clazz.isInterface()) {
throw new IllegalArgumentException(clazz.getName() + " is not an interface, JDK dynamic " +
"proxy can only proxy interfaces.");
}
return (T) Proxy.newProxyInstance(clazz.getClassLoader(), new Class[]{clazz}, invocationHandler);
}
/**
* Using an interface {@code class} and {@code InvocationHandler} build a JDK dynamic proxy object.
*
* @param args The conditions for creating a proxy object.
* @param type.
* @return JDK dynamic proxy object
*/
public static T createJdkProxy(@NonNull Object... args) {
Class type = null;
InvocationHandler invocationHandler = null;
for (Object arg : args) {
if (type != null && invocationHandler != null) {
break;
}
if (arg instanceof Class && /* is Interface */ ((Class>) arg).isInterface()) {
type = (Class) arg;
}
if (arg instanceof InvocationHandler) {
invocationHandler = (InvocationHandler) arg;
}
}
if (type == null || invocationHandler == null) throw new IllegalArgumentException();
return createJdkProxy(type, invocationHandler);
}
/**
* Using a {@code class} and {@code Callback} build a Cglib dynamic proxy object.
*
* @param type Proxy type.
* @param callback Callback interface.
* @param type.
* @return Cglib dynamic proxy object.
*/
public static T createCglibProxy(Class type, Callback callback) {
final Enhancer enhancer = new Enhancer();
enhancer.setSuperclass(type);
enhancer.setCallback(callback);
return (T) enhancer.create();
}
/**
* Using a {@code class} and {@code Callback} build a Cglib dynamic proxy object.
*
* @param args The conditions for creating a proxy object.
* @param type.
* @return Cglib dynamic proxy object.
*/
public static T createCglibProxy(@NonNull Object... args) {
Class type = null;
Callback callback = null;
for (Object arg : args) {
if (type != null && callback != null) {
break;
}
if (arg instanceof Class) {
type = (Class) arg;
}
if (arg instanceof Callback) {
callback = (Callback) arg;
}
}
if (type == null || callback == null) throw new IllegalArgumentException();
return createCglibProxy(type, callback);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy