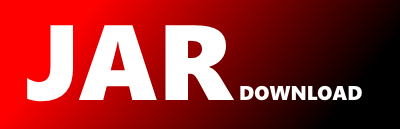
top.zeimao77.product.mysql.MapRepository Maven / Gradle / Ivy
package top.zeimao77.product.mysql;
import top.zeimao77.product.sql.*;
import top.zeimao77.product.util.AssertUtil;
import java.util.*;
public class MapRepository extends AbstractRepository
© 2015 - 2025 Weber Informatics LLC | Privacy Policy