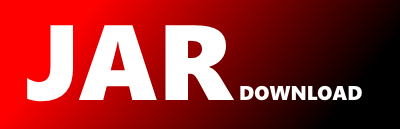
oracle.toplink.essentials.ejb.cmp3.persistence.URLArchive Maven / Gradle / Ivy
/*
* The contents of this file are subject to the terms
* of the Common Development and Distribution License
* (the "License"). You may not use this file except
* in compliance with the License.
*
* You can obtain a copy of the license at
* glassfish/bootstrap/legal/CDDLv1.0.txt or
* https://glassfish.dev.java.net/public/CDDLv1.0.html.
* See the License for the specific language governing
* permissions and limitations under the License.
*
* When distributing Covered Code, include this CDDL
* HEADER in each file and include the License file at
* glassfish/bootstrap/legal/CDDLv1.0.txt. If applicable,
* add the following below this CDDL HEADER, with the
* fields enclosed by brackets "[]" replaced with your
* own identifying information: Portions Copyright [yyyy]
* [name of copyright owner]
*
* Copyright 2006 Sun Microsystems, Inc. All rights reserved.
*/
package oracle.toplink.essentials.ejb.cmp3.persistence;
import java.io.IOException;
import java.io.InputStream;
import java.net.URL;
import java.util.Collections;
import java.util.Iterator;
/**
* This is an implementation of {@link Archive} when container returns a url
* that is not one of the familiar URL types like file or jar URLs. So, we can
* not recurssively walk thru' it's hierarchy. As a result {@link #getEntries()}
* returns an empty collection.
*
* @author [email protected]
*/
public class URLArchive implements Archive {
/*
* Implementation Note: This class does not have any dependency on TopLink
* or GlassFish implementation classes. Please retain this searation.
*/
/**
* The URL representation of this archive.
*/
private URL url;
public URLArchive(URL url) {
this.url = url;
}
public Iterator getEntries() {
return Collections.EMPTY_LIST.iterator();
}
public InputStream getEntry(String entryPath) throws IOException {
URL subEntry = new URL(url, entryPath);
InputStream is = null;
try {
is = subEntry.openStream();
} catch (IOException ioe) {
// we return null when entry does not exist
}
return is;
}
public URL getEntryAsURL(String entryPath) throws IOException {
URL subEntry = new URL(url, entryPath);
try {
InputStream is = subEntry.openStream();
is.close();
} catch (IOException ioe) {
return null; // return null when entry does not exist
}
return subEntry;
}
public URL getRootURL() {
return url;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy