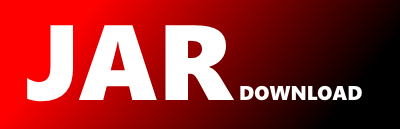
travel.wink.sdk.affiliate.model.BookingContractAffiliate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of affiliate-sdk-java Show documentation
Show all versions of affiliate-sdk-java Show documentation
Java SDK for the wink Affiliate API
/*
* Wink API
* ## APIs Not every integrator needs every APIs. For that reason, we have separated APIs into context. - [Affiliate](/affiliate): All APIs related to selling travel inventory as an affiliate. - [Analytics](/analytics): All APIs related to tracking metrics across a wide variety of data source segments including, more entertaining, leaderboard metrics. - [Booking](/booking): All APIs related to creating platform bookings. - [Channel Manager](/channel-manager): All APIs related to channel managers who want to integrate with our platform. - [Extranet](/extranet): All APIs related to managing travel inventory and suppliers. - [Inventory](/inventory): All APIs related to retrieve known travel inventory as it was found using the Lookup API.. - [Lookup](/lookup): All APIs related to locating inventory by region, locale and property flags. - [Reference](/reference): All APIs related to retrieving platform-supported taxonomies. - [TripPay Acquiring](/payment-acquiring): All APIs related to capture payment details such as a Stripe payment intent. - [TripPay](/payment): All APIs related to TripPay account management, booking, mapping and integration features. ## SDKs We are actively working on supporting the most used languages out there. If you don't see your language here, reach out to us with a request to officially add your language. In the meantime, if you want to roll your own SDK, you can do so by downloading the OpenAPI spec and using one of the many available OpenAPI generators available: [https://openapi-generator.tech/docs/generators](https://openapi-generator.tech/docs/generators). - Java SDK [https://github.com/wink-travel/wink-sdk-java](https://github.com/wink-travel/wink-sdk-java) ## Usage These features are made available to you via a [REST API](https://en.wikipedia.org/wiki/Representational_state_transfer). This API is language agnostic. ## Versioning We chose to version our endpoints in a way that we hope affects your integration with us the least. You request the version of our API you wish to work with via the `Wink-Version` header. When it's time for you to upgrade, you only have to change the version number to get access to our updated endpoints. ## Release history - 2022-10-15: v2.0 - Removed HATEOAS and added Wink-Version header - 2022-05-08: v1 - Exposed channel manager API - 2021-07-01: v1 - Initial release # Affiliate API Welcome to the Affiliate API - A programmer-friendly way to search for and display bespoke travel inventory for your audience. Use this API to help you prepare travel inventory for sale. # Intended Audience Programmers are a requirement to start integrating with wink. You will benefit from an API integration if you are new or existing travel related company that want easy access to great inventory.## Examples: - Hotel brands / chains that want to make their own booking engine - Travel tech companies that want to create the next hot mobile travel app - Destination sites that want to make their own booking engine - Bloggers and influencers who want to sell travel inventory to their audience - OTAs that want access direct relationships with suppliers and better quality hotel inventory
*
* The version of the OpenAPI document: 24.0.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package travel.wink.sdk.affiliate.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.time.OffsetDateTime;
import java.time.LocalDateTime;
import java.util.ArrayList;
import java.util.List;
import java.util.UUID;
import travel.wink.sdk.affiliate.model.BookingContractItemAffiliate;
import travel.wink.sdk.affiliate.model.BookingContractPaymentDetailsAffiliate;
import travel.wink.sdk.affiliate.model.MoneysAffiliate;
import travel.wink.sdk.affiliate.model.QuoteAffiliate;
import travel.wink.sdk.affiliate.model.RefundAffiliate;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import javax.validation.constraints.*;
import javax.validation.Valid;
import org.hibernate.validator.constraints.*;
/**
* Booking contract created by TripPay
*/
@ApiModel(description = "Booking contract created by TripPay")
@JsonPropertyOrder({
BookingContractAffiliate.JSON_PROPERTY_BOOKING_CONTRACT_IDENTIFIER,
BookingContractAffiliate.JSON_PROPERTY_CREATED_DATE,
BookingContractAffiliate.JSON_PROPERTY_LAST_UPDATE,
BookingContractAffiliate.JSON_PROPERTY_IP_ADDRESS,
BookingContractAffiliate.JSON_PROPERTY_TRACE_ID,
BookingContractAffiliate.JSON_PROPERTY_SOURCE_URL,
BookingContractAffiliate.JSON_PROPERTY_IDENTIFIER,
BookingContractAffiliate.JSON_PROPERTY_SUPPLIER_IDENTIFIER,
BookingContractAffiliate.JSON_PROPERTY_SUPPLIER_NAME,
BookingContractAffiliate.JSON_PROPERTY_DISPLAY_PRICE_QUOTE,
BookingContractAffiliate.JSON_PROPERTY_SUPPLIER_PRICE_QUOTE,
BookingContractAffiliate.JSON_PROPERTY_INTERNAL_PRICE_QUOTE,
BookingContractAffiliate.JSON_PROPERTY_CAPTURE_PRICE_QUOTE,
BookingContractAffiliate.JSON_PROPERTY_ITEM_LIST,
BookingContractAffiliate.JSON_PROPERTY_EXTERNAL_SUPPLIER_IDENTIFIER,
BookingContractAffiliate.JSON_PROPERTY_EXTERNAL_SUPPLIER_BOOKING_CODE,
BookingContractAffiliate.JSON_PROPERTY_PAYMENT,
BookingContractAffiliate.JSON_PROPERTY_CANCELLED,
BookingContractAffiliate.JSON_PROPERTY_CANCELLED_ON,
BookingContractAffiliate.JSON_PROPERTY_CANCELLER,
BookingContractAffiliate.JSON_PROPERTY_CANCELLATION_TYPE,
BookingContractAffiliate.JSON_PROPERTY_CANCELLER_USER_IDENTIFIER,
BookingContractAffiliate.JSON_PROPERTY_CANCEL_REASON,
BookingContractAffiliate.JSON_PROPERTY_FUNDS_PROCESSED,
BookingContractAffiliate.JSON_PROPERTY_REFUNDS,
BookingContractAffiliate.JSON_PROPERTY_TOTAL_SOURCE_PRICE,
BookingContractAffiliate.JSON_PROPERTY_TOTAL_DISPLAY_PRICE,
BookingContractAffiliate.JSON_PROPERTY_TOTAL_SUPPLIER_PRICE,
BookingContractAffiliate.JSON_PROPERTY_TOTAL_INTERNAL_PRICE,
BookingContractAffiliate.JSON_PROPERTY_TOTAL_CAPTURE_PRICE,
BookingContractAffiliate.JSON_PROPERTY_TOTAL_SOURCE_PRICE_AFTER_REFUND,
BookingContractAffiliate.JSON_PROPERTY_TOTAL_DISPLAY_PRICE_AFTER_REFUND,
BookingContractAffiliate.JSON_PROPERTY_TOTAL_SUPPLIER_PRICE_AFTER_REFUND,
BookingContractAffiliate.JSON_PROPERTY_TOTAL_INTERNAL_PRICE_AFTER_REFUND,
BookingContractAffiliate.JSON_PROPERTY_TOTAL_CAPTURE_PRICE_AFTER_REFUND,
BookingContractAffiliate.JSON_PROPERTY_REFUNDED_SOURCE_PRICE,
BookingContractAffiliate.JSON_PROPERTY_REFUNDED_DISPLAY_PRICE,
BookingContractAffiliate.JSON_PROPERTY_REFUNDED_SUPPLIER_PRICE,
BookingContractAffiliate.JSON_PROPERTY_REFUNDED_INTERNAL_PRICE,
BookingContractAffiliate.JSON_PROPERTY_REFUNDED_CAPTURE_PRICE,
BookingContractAffiliate.JSON_PROPERTY_TOTAL_TOKENS_EARNED,
BookingContractAffiliate.JSON_PROPERTY_CANCELLABLE_BY_SUPPLIER,
BookingContractAffiliate.JSON_PROPERTY_CANCELLABLE_BY_TRAVELER,
BookingContractAffiliate.JSON_PROPERTY_CANCELLABLE_WITH_NO_CHARGES,
BookingContractAffiliate.JSON_PROPERTY_IS_CANCELLABLE_WITH_POTENTIAL_CHARGES,
BookingContractAffiliate.JSON_PROPERTY_COMMISSIONABLE_TOTAL_SOURCE_AMOUNT,
BookingContractAffiliate.JSON_PROPERTY_COMMISSIONABLE_TOTAL_CAPTURE_AMOUNT,
BookingContractAffiliate.JSON_PROPERTY_COMMISSIONABLE_TOTAL_DISPLAY_AMOUNT,
BookingContractAffiliate.JSON_PROPERTY_COMMISSIONABLE_TOTAL_SUPPLIER_AMOUNT,
BookingContractAffiliate.JSON_PROPERTY_COMMISSIONABLE_TOTAL_INTERNAL_AMOUNT
})
@JsonTypeName("BookingContract_Affiliate")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2023-03-06T16:56:30.815925180+07:00[Asia/Bangkok]")
public class BookingContractAffiliate {
public static final String JSON_PROPERTY_BOOKING_CONTRACT_IDENTIFIER = "bookingContractIdentifier";
private UUID bookingContractIdentifier;
public static final String JSON_PROPERTY_CREATED_DATE = "createdDate";
private java.time.LocalDateTime createdDate;
public static final String JSON_PROPERTY_LAST_UPDATE = "lastUpdate";
private java.time.LocalDateTime lastUpdate;
public static final String JSON_PROPERTY_IP_ADDRESS = "ipAddress";
private String ipAddress;
public static final String JSON_PROPERTY_TRACE_ID = "traceId";
private String traceId;
public static final String JSON_PROPERTY_SOURCE_URL = "sourceUrl";
private String sourceUrl;
public static final String JSON_PROPERTY_IDENTIFIER = "identifier";
private UUID identifier;
public static final String JSON_PROPERTY_SUPPLIER_IDENTIFIER = "supplierIdentifier";
private UUID supplierIdentifier;
public static final String JSON_PROPERTY_SUPPLIER_NAME = "supplierName";
private String supplierName;
public static final String JSON_PROPERTY_DISPLAY_PRICE_QUOTE = "displayPriceQuote";
private QuoteAffiliate displayPriceQuote;
public static final String JSON_PROPERTY_SUPPLIER_PRICE_QUOTE = "supplierPriceQuote";
private QuoteAffiliate supplierPriceQuote;
public static final String JSON_PROPERTY_INTERNAL_PRICE_QUOTE = "internalPriceQuote";
private QuoteAffiliate internalPriceQuote;
public static final String JSON_PROPERTY_CAPTURE_PRICE_QUOTE = "capturePriceQuote";
private QuoteAffiliate capturePriceQuote;
public static final String JSON_PROPERTY_ITEM_LIST = "itemList";
private List itemList = new ArrayList<>();
public static final String JSON_PROPERTY_EXTERNAL_SUPPLIER_IDENTIFIER = "externalSupplierIdentifier";
private String externalSupplierIdentifier;
public static final String JSON_PROPERTY_EXTERNAL_SUPPLIER_BOOKING_CODE = "externalSupplierBookingCode";
private String externalSupplierBookingCode;
public static final String JSON_PROPERTY_PAYMENT = "payment";
private BookingContractPaymentDetailsAffiliate payment;
public static final String JSON_PROPERTY_CANCELLED = "cancelled";
private Boolean cancelled = false;
public static final String JSON_PROPERTY_CANCELLED_ON = "cancelledOn";
private java.time.LocalDateTime cancelledOn;
/**
* Type of entity that cancelled the booking.
*/
public enum CancellerEnum {
SALES_CHANNEL("SALES_CHANNEL"),
SUPPLIER("SUPPLIER"),
TRAVELER("TRAVELER"),
ACQUIRER("ACQUIRER"),
ADMINISTRATOR("ADMINISTRATOR");
private String value;
CancellerEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static CancellerEnum fromValue(String value) {
for (CancellerEnum b : CancellerEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
}
public static final String JSON_PROPERTY_CANCELLER = "canceller";
private CancellerEnum canceller;
/**
* Reason type.
*/
public enum CancellationTypeEnum {
DUPLICATE("DUPLICATE"),
CANCELLATION("CANCELLATION"),
NO_SHOW("NO_SHOW"),
CC_INVALID("CC_INVALID"),
CC_INSUFFICIENT("CC_INSUFFICIENT"),
DISCRETIONARY("DISCRETIONARY");
private String value;
CancellationTypeEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static CancellationTypeEnum fromValue(String value) {
for (CancellationTypeEnum b : CancellationTypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
}
public static final String JSON_PROPERTY_CANCELLATION_TYPE = "cancellationType";
private CancellationTypeEnum cancellationType;
public static final String JSON_PROPERTY_CANCELLER_USER_IDENTIFIER = "cancellerUserIdentifier";
private String cancellerUserIdentifier;
public static final String JSON_PROPERTY_CANCEL_REASON = "cancelReason";
private String cancelReason;
public static final String JSON_PROPERTY_FUNDS_PROCESSED = "fundsProcessed";
private Boolean fundsProcessed;
public static final String JSON_PROPERTY_REFUNDS = "refunds";
private List refunds = null;
public static final String JSON_PROPERTY_TOTAL_SOURCE_PRICE = "totalSourcePrice";
private MoneysAffiliate totalSourcePrice;
public static final String JSON_PROPERTY_TOTAL_DISPLAY_PRICE = "totalDisplayPrice";
private MoneysAffiliate totalDisplayPrice;
public static final String JSON_PROPERTY_TOTAL_SUPPLIER_PRICE = "totalSupplierPrice";
private MoneysAffiliate totalSupplierPrice;
public static final String JSON_PROPERTY_TOTAL_INTERNAL_PRICE = "totalInternalPrice";
private MoneysAffiliate totalInternalPrice;
public static final String JSON_PROPERTY_TOTAL_CAPTURE_PRICE = "totalCapturePrice";
private MoneysAffiliate totalCapturePrice;
public static final String JSON_PROPERTY_TOTAL_SOURCE_PRICE_AFTER_REFUND = "totalSourcePriceAfterRefund";
private MoneysAffiliate totalSourcePriceAfterRefund;
public static final String JSON_PROPERTY_TOTAL_DISPLAY_PRICE_AFTER_REFUND = "totalDisplayPriceAfterRefund";
private MoneysAffiliate totalDisplayPriceAfterRefund;
public static final String JSON_PROPERTY_TOTAL_SUPPLIER_PRICE_AFTER_REFUND = "totalSupplierPriceAfterRefund";
private MoneysAffiliate totalSupplierPriceAfterRefund;
public static final String JSON_PROPERTY_TOTAL_INTERNAL_PRICE_AFTER_REFUND = "totalInternalPriceAfterRefund";
private MoneysAffiliate totalInternalPriceAfterRefund;
public static final String JSON_PROPERTY_TOTAL_CAPTURE_PRICE_AFTER_REFUND = "totalCapturePriceAfterRefund";
private MoneysAffiliate totalCapturePriceAfterRefund;
public static final String JSON_PROPERTY_REFUNDED_SOURCE_PRICE = "refundedSourcePrice";
private MoneysAffiliate refundedSourcePrice;
public static final String JSON_PROPERTY_REFUNDED_DISPLAY_PRICE = "refundedDisplayPrice";
private MoneysAffiliate refundedDisplayPrice;
public static final String JSON_PROPERTY_REFUNDED_SUPPLIER_PRICE = "refundedSupplierPrice";
private MoneysAffiliate refundedSupplierPrice;
public static final String JSON_PROPERTY_REFUNDED_INTERNAL_PRICE = "refundedInternalPrice";
private MoneysAffiliate refundedInternalPrice;
public static final String JSON_PROPERTY_REFUNDED_CAPTURE_PRICE = "refundedCapturePrice";
private MoneysAffiliate refundedCapturePrice;
public static final String JSON_PROPERTY_TOTAL_TOKENS_EARNED = "totalTokensEarned";
private Long totalTokensEarned;
public static final String JSON_PROPERTY_CANCELLABLE_BY_SUPPLIER = "cancellableBySupplier";
private Boolean cancellableBySupplier;
public static final String JSON_PROPERTY_CANCELLABLE_BY_TRAVELER = "cancellableByTraveler";
private Boolean cancellableByTraveler;
public static final String JSON_PROPERTY_CANCELLABLE_WITH_NO_CHARGES = "cancellableWithNoCharges";
private Boolean cancellableWithNoCharges;
public static final String JSON_PROPERTY_IS_CANCELLABLE_WITH_POTENTIAL_CHARGES = "isCancellableWithPotentialCharges";
private Boolean isCancellableWithPotentialCharges;
public static final String JSON_PROPERTY_COMMISSIONABLE_TOTAL_SOURCE_AMOUNT = "commissionableTotalSourceAmount";
private MoneysAffiliate commissionableTotalSourceAmount;
public static final String JSON_PROPERTY_COMMISSIONABLE_TOTAL_CAPTURE_AMOUNT = "commissionableTotalCaptureAmount";
private MoneysAffiliate commissionableTotalCaptureAmount;
public static final String JSON_PROPERTY_COMMISSIONABLE_TOTAL_DISPLAY_AMOUNT = "commissionableTotalDisplayAmount";
private MoneysAffiliate commissionableTotalDisplayAmount;
public static final String JSON_PROPERTY_COMMISSIONABLE_TOTAL_SUPPLIER_AMOUNT = "commissionableTotalSupplierAmount";
private MoneysAffiliate commissionableTotalSupplierAmount;
public static final String JSON_PROPERTY_COMMISSIONABLE_TOTAL_INTERNAL_AMOUNT = "commissionableTotalInternalAmount";
private MoneysAffiliate commissionableTotalInternalAmount;
public BookingContractAffiliate() {
}
public BookingContractAffiliate bookingContractIdentifier(UUID bookingContractIdentifier) {
this.bookingContractIdentifier = bookingContractIdentifier;
return this;
}
/**
* Document UUID
* @return bookingContractIdentifier
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "Document UUID")
@JsonProperty(JSON_PROPERTY_BOOKING_CONTRACT_IDENTIFIER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public UUID getBookingContractIdentifier() {
return bookingContractIdentifier;
}
@JsonProperty(JSON_PROPERTY_BOOKING_CONTRACT_IDENTIFIER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setBookingContractIdentifier(UUID bookingContractIdentifier) {
this.bookingContractIdentifier = bookingContractIdentifier;
}
public BookingContractAffiliate createdDate(java.time.LocalDateTime createdDate) {
this.createdDate = createdDate;
return this;
}
/**
* Datetime this record was first created
* @return createdDate
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "Datetime this record was first created")
@JsonProperty(JSON_PROPERTY_CREATED_DATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public java.time.LocalDateTime getCreatedDate() {
return createdDate;
}
@JsonProperty(JSON_PROPERTY_CREATED_DATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCreatedDate(java.time.LocalDateTime createdDate) {
this.createdDate = createdDate;
}
public BookingContractAffiliate lastUpdate(java.time.LocalDateTime lastUpdate) {
this.lastUpdate = lastUpdate;
return this;
}
/**
* Datetime this record was last updated
* @return lastUpdate
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "Datetime this record was last updated")
@JsonProperty(JSON_PROPERTY_LAST_UPDATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public java.time.LocalDateTime getLastUpdate() {
return lastUpdate;
}
@JsonProperty(JSON_PROPERTY_LAST_UPDATE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setLastUpdate(java.time.LocalDateTime lastUpdate) {
this.lastUpdate = lastUpdate;
}
public BookingContractAffiliate ipAddress(String ipAddress) {
this.ipAddress = ipAddress;
return this;
}
/**
* Caller's IP address
* @return ipAddress
**/
@javax.annotation.Nonnull
@NotNull
@ApiModelProperty(example = "111.222.333.444", required = true, value = "Caller's IP address")
@JsonProperty(JSON_PROPERTY_IP_ADDRESS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getIpAddress() {
return ipAddress;
}
@JsonProperty(JSON_PROPERTY_IP_ADDRESS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setIpAddress(String ipAddress) {
this.ipAddress = ipAddress;
}
public BookingContractAffiliate traceId(String traceId) {
this.traceId = traceId;
return this;
}
/**
* Way to track which booking contracts were made together
* @return traceId
**/
@javax.annotation.Nonnull
@NotNull
@ApiModelProperty(example = "T-123456", required = true, value = "Way to track which booking contracts were made together")
@JsonProperty(JSON_PROPERTY_TRACE_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getTraceId() {
return traceId;
}
@JsonProperty(JSON_PROPERTY_TRACE_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setTraceId(String traceId) {
this.traceId = traceId;
}
public BookingContractAffiliate sourceUrl(String sourceUrl) {
this.sourceUrl = sourceUrl;
return this;
}
/**
* Where did the booking occur
* @return sourceUrl
**/
@javax.annotation.Nonnull
@NotNull
@ApiModelProperty(example = "https://www.traveliko.com", required = true, value = "Where did the booking occur")
@JsonProperty(JSON_PROPERTY_SOURCE_URL)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getSourceUrl() {
return sourceUrl;
}
@JsonProperty(JSON_PROPERTY_SOURCE_URL)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setSourceUrl(String sourceUrl) {
this.sourceUrl = sourceUrl;
}
public BookingContractAffiliate identifier(UUID identifier) {
this.identifier = identifier;
return this;
}
/**
* Unique identifier used to track the contract. Create a UUID for this purpose.
* @return identifier
**/
@javax.annotation.Nonnull
@NotNull
@Valid
@ApiModelProperty(required = true, value = "Unique identifier used to track the contract. Create a UUID for this purpose.")
@JsonProperty(JSON_PROPERTY_IDENTIFIER)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public UUID getIdentifier() {
return identifier;
}
@JsonProperty(JSON_PROPERTY_IDENTIFIER)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setIdentifier(UUID identifier) {
this.identifier = identifier;
}
public BookingContractAffiliate supplierIdentifier(UUID supplierIdentifier) {
this.supplierIdentifier = supplierIdentifier;
return this;
}
/**
* Supplier identifier
* @return supplierIdentifier
**/
@javax.annotation.Nonnull
@NotNull
@Valid
@ApiModelProperty(required = true, value = "Supplier identifier")
@JsonProperty(JSON_PROPERTY_SUPPLIER_IDENTIFIER)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public UUID getSupplierIdentifier() {
return supplierIdentifier;
}
@JsonProperty(JSON_PROPERTY_SUPPLIER_IDENTIFIER)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setSupplierIdentifier(UUID supplierIdentifier) {
this.supplierIdentifier = supplierIdentifier;
}
public BookingContractAffiliate supplierName(String supplierName) {
this.supplierName = supplierName;
return this;
}
/**
* Supplier name
* @return supplierName
**/
@javax.annotation.Nonnull
@NotNull
@ApiModelProperty(example = "Supplier One", required = true, value = "Supplier name")
@JsonProperty(JSON_PROPERTY_SUPPLIER_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getSupplierName() {
return supplierName;
}
@JsonProperty(JSON_PROPERTY_SUPPLIER_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setSupplierName(String supplierName) {
this.supplierName = supplierName;
}
public BookingContractAffiliate displayPriceQuote(QuoteAffiliate displayPriceQuote) {
this.displayPriceQuote = displayPriceQuote;
return this;
}
/**
* Get displayPriceQuote
* @return displayPriceQuote
**/
@javax.annotation.Nonnull
@NotNull
@Valid
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_DISPLAY_PRICE_QUOTE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public QuoteAffiliate getDisplayPriceQuote() {
return displayPriceQuote;
}
@JsonProperty(JSON_PROPERTY_DISPLAY_PRICE_QUOTE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setDisplayPriceQuote(QuoteAffiliate displayPriceQuote) {
this.displayPriceQuote = displayPriceQuote;
}
public BookingContractAffiliate supplierPriceQuote(QuoteAffiliate supplierPriceQuote) {
this.supplierPriceQuote = supplierPriceQuote;
return this;
}
/**
* Get supplierPriceQuote
* @return supplierPriceQuote
**/
@javax.annotation.Nonnull
@NotNull
@Valid
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_SUPPLIER_PRICE_QUOTE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public QuoteAffiliate getSupplierPriceQuote() {
return supplierPriceQuote;
}
@JsonProperty(JSON_PROPERTY_SUPPLIER_PRICE_QUOTE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setSupplierPriceQuote(QuoteAffiliate supplierPriceQuote) {
this.supplierPriceQuote = supplierPriceQuote;
}
public BookingContractAffiliate internalPriceQuote(QuoteAffiliate internalPriceQuote) {
this.internalPriceQuote = internalPriceQuote;
return this;
}
/**
* Get internalPriceQuote
* @return internalPriceQuote
**/
@javax.annotation.Nonnull
@NotNull
@Valid
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_INTERNAL_PRICE_QUOTE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public QuoteAffiliate getInternalPriceQuote() {
return internalPriceQuote;
}
@JsonProperty(JSON_PROPERTY_INTERNAL_PRICE_QUOTE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setInternalPriceQuote(QuoteAffiliate internalPriceQuote) {
this.internalPriceQuote = internalPriceQuote;
}
public BookingContractAffiliate capturePriceQuote(QuoteAffiliate capturePriceQuote) {
this.capturePriceQuote = capturePriceQuote;
return this;
}
/**
* Get capturePriceQuote
* @return capturePriceQuote
**/
@javax.annotation.Nonnull
@NotNull
@Valid
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_CAPTURE_PRICE_QUOTE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public QuoteAffiliate getCapturePriceQuote() {
return capturePriceQuote;
}
@JsonProperty(JSON_PROPERTY_CAPTURE_PRICE_QUOTE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setCapturePriceQuote(QuoteAffiliate capturePriceQuote) {
this.capturePriceQuote = capturePriceQuote;
}
public BookingContractAffiliate itemList(List itemList) {
this.itemList = itemList;
return this;
}
public BookingContractAffiliate addItemListItem(BookingContractItemAffiliate itemListItem) {
this.itemList.add(itemListItem);
return this;
}
/**
* Holds one booking line item for a specific supplier.
* @return itemList
**/
@javax.annotation.Nonnull
@NotNull
@Valid
@Size(min=1,max=2147483647) @ApiModelProperty(required = true, value = "Holds one booking line item for a specific supplier.")
@JsonProperty(JSON_PROPERTY_ITEM_LIST)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getItemList() {
return itemList;
}
@JsonProperty(JSON_PROPERTY_ITEM_LIST)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setItemList(List itemList) {
this.itemList = itemList;
}
public BookingContractAffiliate externalSupplierIdentifier(String externalSupplierIdentifier) {
this.externalSupplierIdentifier = externalSupplierIdentifier;
return this;
}
/**
* Contract creator can choose to geoname this record with her own identifier
* @return externalSupplierIdentifier
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "supplier-a", value = "Contract creator can choose to geoname this record with her own identifier")
@JsonProperty(JSON_PROPERTY_EXTERNAL_SUPPLIER_IDENTIFIER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getExternalSupplierIdentifier() {
return externalSupplierIdentifier;
}
@JsonProperty(JSON_PROPERTY_EXTERNAL_SUPPLIER_IDENTIFIER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setExternalSupplierIdentifier(String externalSupplierIdentifier) {
this.externalSupplierIdentifier = externalSupplierIdentifier;
}
public BookingContractAffiliate externalSupplierBookingCode(String externalSupplierBookingCode) {
this.externalSupplierBookingCode = externalSupplierBookingCode;
return this;
}
/**
* External booking code generated by the affiliate
* @return externalSupplierBookingCode
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "external-booking-code-1", value = "External booking code generated by the affiliate")
@JsonProperty(JSON_PROPERTY_EXTERNAL_SUPPLIER_BOOKING_CODE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getExternalSupplierBookingCode() {
return externalSupplierBookingCode;
}
@JsonProperty(JSON_PROPERTY_EXTERNAL_SUPPLIER_BOOKING_CODE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setExternalSupplierBookingCode(String externalSupplierBookingCode) {
this.externalSupplierBookingCode = externalSupplierBookingCode;
}
public BookingContractAffiliate payment(BookingContractPaymentDetailsAffiliate payment) {
this.payment = payment;
return this;
}
/**
* Get payment
* @return payment
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_PAYMENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public BookingContractPaymentDetailsAffiliate getPayment() {
return payment;
}
@JsonProperty(JSON_PROPERTY_PAYMENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPayment(BookingContractPaymentDetailsAffiliate payment) {
this.payment = payment;
}
public BookingContractAffiliate cancelled(Boolean cancelled) {
this.cancelled = cancelled;
return this;
}
/**
* Optional geoname externalIdentifier to remote inventory.
* @return cancelled
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "false", value = "Optional geoname externalIdentifier to remote inventory.")
@JsonProperty(JSON_PROPERTY_CANCELLED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getCancelled() {
return cancelled;
}
@JsonProperty(JSON_PROPERTY_CANCELLED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCancelled(Boolean cancelled) {
this.cancelled = cancelled;
}
public BookingContractAffiliate cancelledOn(java.time.LocalDateTime cancelledOn) {
this.cancelledOn = cancelledOn;
return this;
}
/**
* When the booking was cancelled.
* @return cancelledOn
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "When the booking was cancelled.")
@JsonProperty(JSON_PROPERTY_CANCELLED_ON)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public java.time.LocalDateTime getCancelledOn() {
return cancelledOn;
}
@JsonProperty(JSON_PROPERTY_CANCELLED_ON)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCancelledOn(java.time.LocalDateTime cancelledOn) {
this.cancelledOn = cancelledOn;
}
public BookingContractAffiliate canceller(CancellerEnum canceller) {
this.canceller = canceller;
return this;
}
/**
* Type of entity that cancelled the booking.
* @return canceller
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Type of entity that cancelled the booking.")
@JsonProperty(JSON_PROPERTY_CANCELLER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public CancellerEnum getCanceller() {
return canceller;
}
@JsonProperty(JSON_PROPERTY_CANCELLER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCanceller(CancellerEnum canceller) {
this.canceller = canceller;
}
public BookingContractAffiliate cancellationType(CancellationTypeEnum cancellationType) {
this.cancellationType = cancellationType;
return this;
}
/**
* Reason type.
* @return cancellationType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Reason type.")
@JsonProperty(JSON_PROPERTY_CANCELLATION_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public CancellationTypeEnum getCancellationType() {
return cancellationType;
}
@JsonProperty(JSON_PROPERTY_CANCELLATION_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCancellationType(CancellationTypeEnum cancellationType) {
this.cancellationType = cancellationType;
}
public BookingContractAffiliate cancellerUserIdentifier(String cancellerUserIdentifier) {
this.cancellerUserIdentifier = cancellerUserIdentifier;
return this;
}
/**
* User identifier that cancelled the entity.
* @return cancellerUserIdentifier
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "User identifier that cancelled the entity.")
@JsonProperty(JSON_PROPERTY_CANCELLER_USER_IDENTIFIER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getCancellerUserIdentifier() {
return cancellerUserIdentifier;
}
@JsonProperty(JSON_PROPERTY_CANCELLER_USER_IDENTIFIER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCancellerUserIdentifier(String cancellerUserIdentifier) {
this.cancellerUserIdentifier = cancellerUserIdentifier;
}
public BookingContractAffiliate cancelReason(String cancelReason) {
this.cancelReason = cancelReason;
return this;
}
/**
* Reason for cancellation.
* @return cancelReason
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Reason for cancellation.")
@JsonProperty(JSON_PROPERTY_CANCEL_REASON)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getCancelReason() {
return cancelReason;
}
@JsonProperty(JSON_PROPERTY_CANCEL_REASON)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCancelReason(String cancelReason) {
this.cancelReason = cancelReason;
}
public BookingContractAffiliate fundsProcessed(Boolean fundsProcessed) {
this.fundsProcessed = fundsProcessed;
return this;
}
/**
* Whether a funds transfer request has been created for this booking.
* @return fundsProcessed
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "false", value = "Whether a funds transfer request has been created for this booking.")
@JsonProperty(JSON_PROPERTY_FUNDS_PROCESSED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getFundsProcessed() {
return fundsProcessed;
}
@JsonProperty(JSON_PROPERTY_FUNDS_PROCESSED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setFundsProcessed(Boolean fundsProcessed) {
this.fundsProcessed = fundsProcessed;
}
public BookingContractAffiliate refunds(List refunds) {
this.refunds = refunds;
return this;
}
public BookingContractAffiliate addRefundsItem(RefundAffiliate refundsItem) {
if (this.refunds == null) {
this.refunds = new ArrayList<>();
}
this.refunds.add(refundsItem);
return this;
}
/**
* An optional list of refunds that occurred with this booking. If the refund amount(s) is the same as the total price, the booking also gets cancelled.
* @return refunds
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "An optional list of refunds that occurred with this booking. If the refund amount(s) is the same as the total price, the booking also gets cancelled.")
@JsonProperty(JSON_PROPERTY_REFUNDS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getRefunds() {
return refunds;
}
@JsonProperty(JSON_PROPERTY_REFUNDS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRefunds(List refunds) {
this.refunds = refunds;
}
public BookingContractAffiliate totalSourcePrice(MoneysAffiliate totalSourcePrice) {
this.totalSourcePrice = totalSourcePrice;
return this;
}
/**
* Get totalSourcePrice
* @return totalSourcePrice
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_TOTAL_SOURCE_PRICE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public MoneysAffiliate getTotalSourcePrice() {
return totalSourcePrice;
}
@JsonProperty(JSON_PROPERTY_TOTAL_SOURCE_PRICE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTotalSourcePrice(MoneysAffiliate totalSourcePrice) {
this.totalSourcePrice = totalSourcePrice;
}
public BookingContractAffiliate totalDisplayPrice(MoneysAffiliate totalDisplayPrice) {
this.totalDisplayPrice = totalDisplayPrice;
return this;
}
/**
* Get totalDisplayPrice
* @return totalDisplayPrice
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_TOTAL_DISPLAY_PRICE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public MoneysAffiliate getTotalDisplayPrice() {
return totalDisplayPrice;
}
@JsonProperty(JSON_PROPERTY_TOTAL_DISPLAY_PRICE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTotalDisplayPrice(MoneysAffiliate totalDisplayPrice) {
this.totalDisplayPrice = totalDisplayPrice;
}
public BookingContractAffiliate totalSupplierPrice(MoneysAffiliate totalSupplierPrice) {
this.totalSupplierPrice = totalSupplierPrice;
return this;
}
/**
* Get totalSupplierPrice
* @return totalSupplierPrice
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_TOTAL_SUPPLIER_PRICE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public MoneysAffiliate getTotalSupplierPrice() {
return totalSupplierPrice;
}
@JsonProperty(JSON_PROPERTY_TOTAL_SUPPLIER_PRICE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTotalSupplierPrice(MoneysAffiliate totalSupplierPrice) {
this.totalSupplierPrice = totalSupplierPrice;
}
public BookingContractAffiliate totalInternalPrice(MoneysAffiliate totalInternalPrice) {
this.totalInternalPrice = totalInternalPrice;
return this;
}
/**
* Get totalInternalPrice
* @return totalInternalPrice
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_TOTAL_INTERNAL_PRICE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public MoneysAffiliate getTotalInternalPrice() {
return totalInternalPrice;
}
@JsonProperty(JSON_PROPERTY_TOTAL_INTERNAL_PRICE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTotalInternalPrice(MoneysAffiliate totalInternalPrice) {
this.totalInternalPrice = totalInternalPrice;
}
public BookingContractAffiliate totalCapturePrice(MoneysAffiliate totalCapturePrice) {
this.totalCapturePrice = totalCapturePrice;
return this;
}
/**
* Get totalCapturePrice
* @return totalCapturePrice
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_TOTAL_CAPTURE_PRICE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public MoneysAffiliate getTotalCapturePrice() {
return totalCapturePrice;
}
@JsonProperty(JSON_PROPERTY_TOTAL_CAPTURE_PRICE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTotalCapturePrice(MoneysAffiliate totalCapturePrice) {
this.totalCapturePrice = totalCapturePrice;
}
public BookingContractAffiliate totalSourcePriceAfterRefund(MoneysAffiliate totalSourcePriceAfterRefund) {
this.totalSourcePriceAfterRefund = totalSourcePriceAfterRefund;
return this;
}
/**
* Get totalSourcePriceAfterRefund
* @return totalSourcePriceAfterRefund
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_TOTAL_SOURCE_PRICE_AFTER_REFUND)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public MoneysAffiliate getTotalSourcePriceAfterRefund() {
return totalSourcePriceAfterRefund;
}
@JsonProperty(JSON_PROPERTY_TOTAL_SOURCE_PRICE_AFTER_REFUND)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTotalSourcePriceAfterRefund(MoneysAffiliate totalSourcePriceAfterRefund) {
this.totalSourcePriceAfterRefund = totalSourcePriceAfterRefund;
}
public BookingContractAffiliate totalDisplayPriceAfterRefund(MoneysAffiliate totalDisplayPriceAfterRefund) {
this.totalDisplayPriceAfterRefund = totalDisplayPriceAfterRefund;
return this;
}
/**
* Get totalDisplayPriceAfterRefund
* @return totalDisplayPriceAfterRefund
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_TOTAL_DISPLAY_PRICE_AFTER_REFUND)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public MoneysAffiliate getTotalDisplayPriceAfterRefund() {
return totalDisplayPriceAfterRefund;
}
@JsonProperty(JSON_PROPERTY_TOTAL_DISPLAY_PRICE_AFTER_REFUND)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTotalDisplayPriceAfterRefund(MoneysAffiliate totalDisplayPriceAfterRefund) {
this.totalDisplayPriceAfterRefund = totalDisplayPriceAfterRefund;
}
public BookingContractAffiliate totalSupplierPriceAfterRefund(MoneysAffiliate totalSupplierPriceAfterRefund) {
this.totalSupplierPriceAfterRefund = totalSupplierPriceAfterRefund;
return this;
}
/**
* Get totalSupplierPriceAfterRefund
* @return totalSupplierPriceAfterRefund
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_TOTAL_SUPPLIER_PRICE_AFTER_REFUND)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public MoneysAffiliate getTotalSupplierPriceAfterRefund() {
return totalSupplierPriceAfterRefund;
}
@JsonProperty(JSON_PROPERTY_TOTAL_SUPPLIER_PRICE_AFTER_REFUND)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTotalSupplierPriceAfterRefund(MoneysAffiliate totalSupplierPriceAfterRefund) {
this.totalSupplierPriceAfterRefund = totalSupplierPriceAfterRefund;
}
public BookingContractAffiliate totalInternalPriceAfterRefund(MoneysAffiliate totalInternalPriceAfterRefund) {
this.totalInternalPriceAfterRefund = totalInternalPriceAfterRefund;
return this;
}
/**
* Get totalInternalPriceAfterRefund
* @return totalInternalPriceAfterRefund
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_TOTAL_INTERNAL_PRICE_AFTER_REFUND)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public MoneysAffiliate getTotalInternalPriceAfterRefund() {
return totalInternalPriceAfterRefund;
}
@JsonProperty(JSON_PROPERTY_TOTAL_INTERNAL_PRICE_AFTER_REFUND)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTotalInternalPriceAfterRefund(MoneysAffiliate totalInternalPriceAfterRefund) {
this.totalInternalPriceAfterRefund = totalInternalPriceAfterRefund;
}
public BookingContractAffiliate totalCapturePriceAfterRefund(MoneysAffiliate totalCapturePriceAfterRefund) {
this.totalCapturePriceAfterRefund = totalCapturePriceAfterRefund;
return this;
}
/**
* Get totalCapturePriceAfterRefund
* @return totalCapturePriceAfterRefund
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_TOTAL_CAPTURE_PRICE_AFTER_REFUND)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public MoneysAffiliate getTotalCapturePriceAfterRefund() {
return totalCapturePriceAfterRefund;
}
@JsonProperty(JSON_PROPERTY_TOTAL_CAPTURE_PRICE_AFTER_REFUND)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTotalCapturePriceAfterRefund(MoneysAffiliate totalCapturePriceAfterRefund) {
this.totalCapturePriceAfterRefund = totalCapturePriceAfterRefund;
}
public BookingContractAffiliate refundedSourcePrice(MoneysAffiliate refundedSourcePrice) {
this.refundedSourcePrice = refundedSourcePrice;
return this;
}
/**
* Get refundedSourcePrice
* @return refundedSourcePrice
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_REFUNDED_SOURCE_PRICE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public MoneysAffiliate getRefundedSourcePrice() {
return refundedSourcePrice;
}
@JsonProperty(JSON_PROPERTY_REFUNDED_SOURCE_PRICE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRefundedSourcePrice(MoneysAffiliate refundedSourcePrice) {
this.refundedSourcePrice = refundedSourcePrice;
}
public BookingContractAffiliate refundedDisplayPrice(MoneysAffiliate refundedDisplayPrice) {
this.refundedDisplayPrice = refundedDisplayPrice;
return this;
}
/**
* Get refundedDisplayPrice
* @return refundedDisplayPrice
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_REFUNDED_DISPLAY_PRICE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public MoneysAffiliate getRefundedDisplayPrice() {
return refundedDisplayPrice;
}
@JsonProperty(JSON_PROPERTY_REFUNDED_DISPLAY_PRICE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRefundedDisplayPrice(MoneysAffiliate refundedDisplayPrice) {
this.refundedDisplayPrice = refundedDisplayPrice;
}
public BookingContractAffiliate refundedSupplierPrice(MoneysAffiliate refundedSupplierPrice) {
this.refundedSupplierPrice = refundedSupplierPrice;
return this;
}
/**
* Get refundedSupplierPrice
* @return refundedSupplierPrice
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_REFUNDED_SUPPLIER_PRICE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public MoneysAffiliate getRefundedSupplierPrice() {
return refundedSupplierPrice;
}
@JsonProperty(JSON_PROPERTY_REFUNDED_SUPPLIER_PRICE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRefundedSupplierPrice(MoneysAffiliate refundedSupplierPrice) {
this.refundedSupplierPrice = refundedSupplierPrice;
}
public BookingContractAffiliate refundedInternalPrice(MoneysAffiliate refundedInternalPrice) {
this.refundedInternalPrice = refundedInternalPrice;
return this;
}
/**
* Get refundedInternalPrice
* @return refundedInternalPrice
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_REFUNDED_INTERNAL_PRICE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public MoneysAffiliate getRefundedInternalPrice() {
return refundedInternalPrice;
}
@JsonProperty(JSON_PROPERTY_REFUNDED_INTERNAL_PRICE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRefundedInternalPrice(MoneysAffiliate refundedInternalPrice) {
this.refundedInternalPrice = refundedInternalPrice;
}
public BookingContractAffiliate refundedCapturePrice(MoneysAffiliate refundedCapturePrice) {
this.refundedCapturePrice = refundedCapturePrice;
return this;
}
/**
* Get refundedCapturePrice
* @return refundedCapturePrice
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_REFUNDED_CAPTURE_PRICE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public MoneysAffiliate getRefundedCapturePrice() {
return refundedCapturePrice;
}
@JsonProperty(JSON_PROPERTY_REFUNDED_CAPTURE_PRICE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRefundedCapturePrice(MoneysAffiliate refundedCapturePrice) {
this.refundedCapturePrice = refundedCapturePrice;
}
public BookingContractAffiliate totalTokensEarned(Long totalTokensEarned) {
this.totalTokensEarned = totalTokensEarned;
return this;
}
/**
* Total amount of tokens minted on this contract.
* @return totalTokensEarned
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Total amount of tokens minted on this contract.")
@JsonProperty(JSON_PROPERTY_TOTAL_TOKENS_EARNED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getTotalTokensEarned() {
return totalTokensEarned;
}
@JsonProperty(JSON_PROPERTY_TOTAL_TOKENS_EARNED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTotalTokensEarned(Long totalTokensEarned) {
this.totalTokensEarned = totalTokensEarned;
}
public BookingContractAffiliate cancellableBySupplier(Boolean cancellableBySupplier) {
this.cancellableBySupplier = cancellableBySupplier;
return this;
}
/**
* Whether the booking can still be cancelled completely by the supplier.
* @return cancellableBySupplier
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Whether the booking can still be cancelled completely by the supplier.")
@JsonProperty(JSON_PROPERTY_CANCELLABLE_BY_SUPPLIER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getCancellableBySupplier() {
return cancellableBySupplier;
}
@JsonProperty(JSON_PROPERTY_CANCELLABLE_BY_SUPPLIER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCancellableBySupplier(Boolean cancellableBySupplier) {
this.cancellableBySupplier = cancellableBySupplier;
}
public BookingContractAffiliate cancellableByTraveler(Boolean cancellableByTraveler) {
this.cancellableByTraveler = cancellableByTraveler;
return this;
}
/**
* Whether the booking can still be cancelled completely by the traveller.
* @return cancellableByTraveler
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Whether the booking can still be cancelled completely by the traveller.")
@JsonProperty(JSON_PROPERTY_CANCELLABLE_BY_TRAVELER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getCancellableByTraveler() {
return cancellableByTraveler;
}
@JsonProperty(JSON_PROPERTY_CANCELLABLE_BY_TRAVELER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCancellableByTraveler(Boolean cancellableByTraveler) {
this.cancellableByTraveler = cancellableByTraveler;
}
public BookingContractAffiliate cancellableWithNoCharges(Boolean cancellableWithNoCharges) {
this.cancellableWithNoCharges = cancellableWithNoCharges;
return this;
}
/**
* Whether the cancellation comes at no cost to the traveler.
* @return cancellableWithNoCharges
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Whether the cancellation comes at no cost to the traveler.")
@JsonProperty(JSON_PROPERTY_CANCELLABLE_WITH_NO_CHARGES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getCancellableWithNoCharges() {
return cancellableWithNoCharges;
}
@JsonProperty(JSON_PROPERTY_CANCELLABLE_WITH_NO_CHARGES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCancellableWithNoCharges(Boolean cancellableWithNoCharges) {
this.cancellableWithNoCharges = cancellableWithNoCharges;
}
public BookingContractAffiliate isCancellableWithPotentialCharges(Boolean isCancellableWithPotentialCharges) {
this.isCancellableWithPotentialCharges = isCancellableWithPotentialCharges;
return this;
}
/**
* Whether a cancellation comes with partial charges. I.e. Only some of the items in contract are not fully refundable.
* @return isCancellableWithPotentialCharges
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Whether a cancellation comes with partial charges. I.e. Only some of the items in contract are not fully refundable.")
@JsonProperty(JSON_PROPERTY_IS_CANCELLABLE_WITH_POTENTIAL_CHARGES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getIsCancellableWithPotentialCharges() {
return isCancellableWithPotentialCharges;
}
@JsonProperty(JSON_PROPERTY_IS_CANCELLABLE_WITH_POTENTIAL_CHARGES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setIsCancellableWithPotentialCharges(Boolean isCancellableWithPotentialCharges) {
this.isCancellableWithPotentialCharges = isCancellableWithPotentialCharges;
}
public BookingContractAffiliate commissionableTotalSourceAmount(MoneysAffiliate commissionableTotalSourceAmount) {
this.commissionableTotalSourceAmount = commissionableTotalSourceAmount;
return this;
}
/**
* Get commissionableTotalSourceAmount
* @return commissionableTotalSourceAmount
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_COMMISSIONABLE_TOTAL_SOURCE_AMOUNT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public MoneysAffiliate getCommissionableTotalSourceAmount() {
return commissionableTotalSourceAmount;
}
@JsonProperty(JSON_PROPERTY_COMMISSIONABLE_TOTAL_SOURCE_AMOUNT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCommissionableTotalSourceAmount(MoneysAffiliate commissionableTotalSourceAmount) {
this.commissionableTotalSourceAmount = commissionableTotalSourceAmount;
}
public BookingContractAffiliate commissionableTotalCaptureAmount(MoneysAffiliate commissionableTotalCaptureAmount) {
this.commissionableTotalCaptureAmount = commissionableTotalCaptureAmount;
return this;
}
/**
* Get commissionableTotalCaptureAmount
* @return commissionableTotalCaptureAmount
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_COMMISSIONABLE_TOTAL_CAPTURE_AMOUNT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public MoneysAffiliate getCommissionableTotalCaptureAmount() {
return commissionableTotalCaptureAmount;
}
@JsonProperty(JSON_PROPERTY_COMMISSIONABLE_TOTAL_CAPTURE_AMOUNT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCommissionableTotalCaptureAmount(MoneysAffiliate commissionableTotalCaptureAmount) {
this.commissionableTotalCaptureAmount = commissionableTotalCaptureAmount;
}
public BookingContractAffiliate commissionableTotalDisplayAmount(MoneysAffiliate commissionableTotalDisplayAmount) {
this.commissionableTotalDisplayAmount = commissionableTotalDisplayAmount;
return this;
}
/**
* Get commissionableTotalDisplayAmount
* @return commissionableTotalDisplayAmount
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_COMMISSIONABLE_TOTAL_DISPLAY_AMOUNT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public MoneysAffiliate getCommissionableTotalDisplayAmount() {
return commissionableTotalDisplayAmount;
}
@JsonProperty(JSON_PROPERTY_COMMISSIONABLE_TOTAL_DISPLAY_AMOUNT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCommissionableTotalDisplayAmount(MoneysAffiliate commissionableTotalDisplayAmount) {
this.commissionableTotalDisplayAmount = commissionableTotalDisplayAmount;
}
public BookingContractAffiliate commissionableTotalSupplierAmount(MoneysAffiliate commissionableTotalSupplierAmount) {
this.commissionableTotalSupplierAmount = commissionableTotalSupplierAmount;
return this;
}
/**
* Get commissionableTotalSupplierAmount
* @return commissionableTotalSupplierAmount
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_COMMISSIONABLE_TOTAL_SUPPLIER_AMOUNT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public MoneysAffiliate getCommissionableTotalSupplierAmount() {
return commissionableTotalSupplierAmount;
}
@JsonProperty(JSON_PROPERTY_COMMISSIONABLE_TOTAL_SUPPLIER_AMOUNT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCommissionableTotalSupplierAmount(MoneysAffiliate commissionableTotalSupplierAmount) {
this.commissionableTotalSupplierAmount = commissionableTotalSupplierAmount;
}
public BookingContractAffiliate commissionableTotalInternalAmount(MoneysAffiliate commissionableTotalInternalAmount) {
this.commissionableTotalInternalAmount = commissionableTotalInternalAmount;
return this;
}
/**
* Get commissionableTotalInternalAmount
* @return commissionableTotalInternalAmount
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_COMMISSIONABLE_TOTAL_INTERNAL_AMOUNT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public MoneysAffiliate getCommissionableTotalInternalAmount() {
return commissionableTotalInternalAmount;
}
@JsonProperty(JSON_PROPERTY_COMMISSIONABLE_TOTAL_INTERNAL_AMOUNT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCommissionableTotalInternalAmount(MoneysAffiliate commissionableTotalInternalAmount) {
this.commissionableTotalInternalAmount = commissionableTotalInternalAmount;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
BookingContractAffiliate bookingContractAffiliate = (BookingContractAffiliate) o;
return Objects.equals(this.bookingContractIdentifier, bookingContractAffiliate.bookingContractIdentifier) &&
Objects.equals(this.createdDate, bookingContractAffiliate.createdDate) &&
Objects.equals(this.lastUpdate, bookingContractAffiliate.lastUpdate) &&
Objects.equals(this.ipAddress, bookingContractAffiliate.ipAddress) &&
Objects.equals(this.traceId, bookingContractAffiliate.traceId) &&
Objects.equals(this.sourceUrl, bookingContractAffiliate.sourceUrl) &&
Objects.equals(this.identifier, bookingContractAffiliate.identifier) &&
Objects.equals(this.supplierIdentifier, bookingContractAffiliate.supplierIdentifier) &&
Objects.equals(this.supplierName, bookingContractAffiliate.supplierName) &&
Objects.equals(this.displayPriceQuote, bookingContractAffiliate.displayPriceQuote) &&
Objects.equals(this.supplierPriceQuote, bookingContractAffiliate.supplierPriceQuote) &&
Objects.equals(this.internalPriceQuote, bookingContractAffiliate.internalPriceQuote) &&
Objects.equals(this.capturePriceQuote, bookingContractAffiliate.capturePriceQuote) &&
Objects.equals(this.itemList, bookingContractAffiliate.itemList) &&
Objects.equals(this.externalSupplierIdentifier, bookingContractAffiliate.externalSupplierIdentifier) &&
Objects.equals(this.externalSupplierBookingCode, bookingContractAffiliate.externalSupplierBookingCode) &&
Objects.equals(this.payment, bookingContractAffiliate.payment) &&
Objects.equals(this.cancelled, bookingContractAffiliate.cancelled) &&
Objects.equals(this.cancelledOn, bookingContractAffiliate.cancelledOn) &&
Objects.equals(this.canceller, bookingContractAffiliate.canceller) &&
Objects.equals(this.cancellationType, bookingContractAffiliate.cancellationType) &&
Objects.equals(this.cancellerUserIdentifier, bookingContractAffiliate.cancellerUserIdentifier) &&
Objects.equals(this.cancelReason, bookingContractAffiliate.cancelReason) &&
Objects.equals(this.fundsProcessed, bookingContractAffiliate.fundsProcessed) &&
Objects.equals(this.refunds, bookingContractAffiliate.refunds) &&
Objects.equals(this.totalSourcePrice, bookingContractAffiliate.totalSourcePrice) &&
Objects.equals(this.totalDisplayPrice, bookingContractAffiliate.totalDisplayPrice) &&
Objects.equals(this.totalSupplierPrice, bookingContractAffiliate.totalSupplierPrice) &&
Objects.equals(this.totalInternalPrice, bookingContractAffiliate.totalInternalPrice) &&
Objects.equals(this.totalCapturePrice, bookingContractAffiliate.totalCapturePrice) &&
Objects.equals(this.totalSourcePriceAfterRefund, bookingContractAffiliate.totalSourcePriceAfterRefund) &&
Objects.equals(this.totalDisplayPriceAfterRefund, bookingContractAffiliate.totalDisplayPriceAfterRefund) &&
Objects.equals(this.totalSupplierPriceAfterRefund, bookingContractAffiliate.totalSupplierPriceAfterRefund) &&
Objects.equals(this.totalInternalPriceAfterRefund, bookingContractAffiliate.totalInternalPriceAfterRefund) &&
Objects.equals(this.totalCapturePriceAfterRefund, bookingContractAffiliate.totalCapturePriceAfterRefund) &&
Objects.equals(this.refundedSourcePrice, bookingContractAffiliate.refundedSourcePrice) &&
Objects.equals(this.refundedDisplayPrice, bookingContractAffiliate.refundedDisplayPrice) &&
Objects.equals(this.refundedSupplierPrice, bookingContractAffiliate.refundedSupplierPrice) &&
Objects.equals(this.refundedInternalPrice, bookingContractAffiliate.refundedInternalPrice) &&
Objects.equals(this.refundedCapturePrice, bookingContractAffiliate.refundedCapturePrice) &&
Objects.equals(this.totalTokensEarned, bookingContractAffiliate.totalTokensEarned) &&
Objects.equals(this.cancellableBySupplier, bookingContractAffiliate.cancellableBySupplier) &&
Objects.equals(this.cancellableByTraveler, bookingContractAffiliate.cancellableByTraveler) &&
Objects.equals(this.cancellableWithNoCharges, bookingContractAffiliate.cancellableWithNoCharges) &&
Objects.equals(this.isCancellableWithPotentialCharges, bookingContractAffiliate.isCancellableWithPotentialCharges) &&
Objects.equals(this.commissionableTotalSourceAmount, bookingContractAffiliate.commissionableTotalSourceAmount) &&
Objects.equals(this.commissionableTotalCaptureAmount, bookingContractAffiliate.commissionableTotalCaptureAmount) &&
Objects.equals(this.commissionableTotalDisplayAmount, bookingContractAffiliate.commissionableTotalDisplayAmount) &&
Objects.equals(this.commissionableTotalSupplierAmount, bookingContractAffiliate.commissionableTotalSupplierAmount) &&
Objects.equals(this.commissionableTotalInternalAmount, bookingContractAffiliate.commissionableTotalInternalAmount);
}
@Override
public int hashCode() {
return Objects.hash(bookingContractIdentifier, createdDate, lastUpdate, ipAddress, traceId, sourceUrl, identifier, supplierIdentifier, supplierName, displayPriceQuote, supplierPriceQuote, internalPriceQuote, capturePriceQuote, itemList, externalSupplierIdentifier, externalSupplierBookingCode, payment, cancelled, cancelledOn, canceller, cancellationType, cancellerUserIdentifier, cancelReason, fundsProcessed, refunds, totalSourcePrice, totalDisplayPrice, totalSupplierPrice, totalInternalPrice, totalCapturePrice, totalSourcePriceAfterRefund, totalDisplayPriceAfterRefund, totalSupplierPriceAfterRefund, totalInternalPriceAfterRefund, totalCapturePriceAfterRefund, refundedSourcePrice, refundedDisplayPrice, refundedSupplierPrice, refundedInternalPrice, refundedCapturePrice, totalTokensEarned, cancellableBySupplier, cancellableByTraveler, cancellableWithNoCharges, isCancellableWithPotentialCharges, commissionableTotalSourceAmount, commissionableTotalCaptureAmount, commissionableTotalDisplayAmount, commissionableTotalSupplierAmount, commissionableTotalInternalAmount);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class BookingContractAffiliate {\n");
sb.append(" bookingContractIdentifier: ").append(toIndentedString(bookingContractIdentifier)).append("\n");
sb.append(" createdDate: ").append(toIndentedString(createdDate)).append("\n");
sb.append(" lastUpdate: ").append(toIndentedString(lastUpdate)).append("\n");
sb.append(" ipAddress: ").append(toIndentedString(ipAddress)).append("\n");
sb.append(" traceId: ").append(toIndentedString(traceId)).append("\n");
sb.append(" sourceUrl: ").append(toIndentedString(sourceUrl)).append("\n");
sb.append(" identifier: ").append(toIndentedString(identifier)).append("\n");
sb.append(" supplierIdentifier: ").append(toIndentedString(supplierIdentifier)).append("\n");
sb.append(" supplierName: ").append(toIndentedString(supplierName)).append("\n");
sb.append(" displayPriceQuote: ").append(toIndentedString(displayPriceQuote)).append("\n");
sb.append(" supplierPriceQuote: ").append(toIndentedString(supplierPriceQuote)).append("\n");
sb.append(" internalPriceQuote: ").append(toIndentedString(internalPriceQuote)).append("\n");
sb.append(" capturePriceQuote: ").append(toIndentedString(capturePriceQuote)).append("\n");
sb.append(" itemList: ").append(toIndentedString(itemList)).append("\n");
sb.append(" externalSupplierIdentifier: ").append(toIndentedString(externalSupplierIdentifier)).append("\n");
sb.append(" externalSupplierBookingCode: ").append(toIndentedString(externalSupplierBookingCode)).append("\n");
sb.append(" payment: ").append(toIndentedString(payment)).append("\n");
sb.append(" cancelled: ").append(toIndentedString(cancelled)).append("\n");
sb.append(" cancelledOn: ").append(toIndentedString(cancelledOn)).append("\n");
sb.append(" canceller: ").append(toIndentedString(canceller)).append("\n");
sb.append(" cancellationType: ").append(toIndentedString(cancellationType)).append("\n");
sb.append(" cancellerUserIdentifier: ").append(toIndentedString(cancellerUserIdentifier)).append("\n");
sb.append(" cancelReason: ").append(toIndentedString(cancelReason)).append("\n");
sb.append(" fundsProcessed: ").append(toIndentedString(fundsProcessed)).append("\n");
sb.append(" refunds: ").append(toIndentedString(refunds)).append("\n");
sb.append(" totalSourcePrice: ").append(toIndentedString(totalSourcePrice)).append("\n");
sb.append(" totalDisplayPrice: ").append(toIndentedString(totalDisplayPrice)).append("\n");
sb.append(" totalSupplierPrice: ").append(toIndentedString(totalSupplierPrice)).append("\n");
sb.append(" totalInternalPrice: ").append(toIndentedString(totalInternalPrice)).append("\n");
sb.append(" totalCapturePrice: ").append(toIndentedString(totalCapturePrice)).append("\n");
sb.append(" totalSourcePriceAfterRefund: ").append(toIndentedString(totalSourcePriceAfterRefund)).append("\n");
sb.append(" totalDisplayPriceAfterRefund: ").append(toIndentedString(totalDisplayPriceAfterRefund)).append("\n");
sb.append(" totalSupplierPriceAfterRefund: ").append(toIndentedString(totalSupplierPriceAfterRefund)).append("\n");
sb.append(" totalInternalPriceAfterRefund: ").append(toIndentedString(totalInternalPriceAfterRefund)).append("\n");
sb.append(" totalCapturePriceAfterRefund: ").append(toIndentedString(totalCapturePriceAfterRefund)).append("\n");
sb.append(" refundedSourcePrice: ").append(toIndentedString(refundedSourcePrice)).append("\n");
sb.append(" refundedDisplayPrice: ").append(toIndentedString(refundedDisplayPrice)).append("\n");
sb.append(" refundedSupplierPrice: ").append(toIndentedString(refundedSupplierPrice)).append("\n");
sb.append(" refundedInternalPrice: ").append(toIndentedString(refundedInternalPrice)).append("\n");
sb.append(" refundedCapturePrice: ").append(toIndentedString(refundedCapturePrice)).append("\n");
sb.append(" totalTokensEarned: ").append(toIndentedString(totalTokensEarned)).append("\n");
sb.append(" cancellableBySupplier: ").append(toIndentedString(cancellableBySupplier)).append("\n");
sb.append(" cancellableByTraveler: ").append(toIndentedString(cancellableByTraveler)).append("\n");
sb.append(" cancellableWithNoCharges: ").append(toIndentedString(cancellableWithNoCharges)).append("\n");
sb.append(" isCancellableWithPotentialCharges: ").append(toIndentedString(isCancellableWithPotentialCharges)).append("\n");
sb.append(" commissionableTotalSourceAmount: ").append(toIndentedString(commissionableTotalSourceAmount)).append("\n");
sb.append(" commissionableTotalCaptureAmount: ").append(toIndentedString(commissionableTotalCaptureAmount)).append("\n");
sb.append(" commissionableTotalDisplayAmount: ").append(toIndentedString(commissionableTotalDisplayAmount)).append("\n");
sb.append(" commissionableTotalSupplierAmount: ").append(toIndentedString(commissionableTotalSupplierAmount)).append("\n");
sb.append(" commissionableTotalInternalAmount: ").append(toIndentedString(commissionableTotalInternalAmount)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy