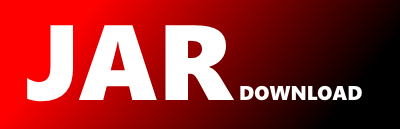
travel.wink.sdk.affiliate.model.BookingContractItemAffiliate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of affiliate-sdk-java Show documentation
Show all versions of affiliate-sdk-java Show documentation
Java SDK for the wink Affiliate API
/*
* Wink API
* ## APIs Not every integrator needs every APIs. For that reason, we have separated APIs into context. - [Affiliate](/affiliate): All APIs related to selling travel inventory as an affiliate. - [Analytics](/analytics): All APIs related to tracking metrics across a wide variety of data source segments including, more entertaining, leaderboard metrics. - [Booking](/booking): All APIs related to creating platform bookings. - [Channel Manager](/channel-manager): All APIs related to channel managers who want to integrate with our platform. - [Extranet](/extranet): All APIs related to managing travel inventory and suppliers. - [Inventory](/inventory): All APIs related to retrieve known travel inventory as it was found using the Lookup API.. - [Lookup](/lookup): All APIs related to locating inventory by region, locale and property flags. - [Reference](/reference): All APIs related to retrieving platform-supported taxonomies. - [TripPay Acquiring](/payment-acquiring): All APIs related to capture payment details such as a Stripe payment intent. - [TripPay](/payment): All APIs related to TripPay account management, booking, mapping and integration features. ## SDKs We are actively working on supporting the most used languages out there. If you don't see your language here, reach out to us with a request to officially add your language. In the meantime, if you want to roll your own SDK, you can do so by downloading the OpenAPI spec and using one of the many available OpenAPI generators available: [https://openapi-generator.tech/docs/generators](https://openapi-generator.tech/docs/generators). - Java SDK [https://github.com/wink-travel/wink-sdk-java](https://github.com/wink-travel/wink-sdk-java) ## Usage These features are made available to you via a [REST API](https://en.wikipedia.org/wiki/Representational_state_transfer). This API is language agnostic. ## Versioning We chose to version our endpoints in a way that we hope affects your integration with us the least. You request the version of our API you wish to work with via the `Wink-Version` header. When it's time for you to upgrade, you only have to change the version number to get access to our updated endpoints. ## Release history - 2022-10-15: v2.0 - Removed HATEOAS and added Wink-Version header - 2022-05-08: v1 - Exposed channel manager API - 2021-07-01: v1 - Initial release # Affiliate API Welcome to the Affiliate API - A programmer-friendly way to search for and display bespoke travel inventory for your audience. Use this API to help you prepare travel inventory for sale. # Intended Audience Programmers are a requirement to start integrating with wink. You will benefit from an API integration if you are new or existing travel related company that want easy access to great inventory.## Examples: - Hotel brands / chains that want to make their own booking engine - Travel tech companies that want to create the next hot mobile travel app - Destination sites that want to make their own booking engine - Bloggers and influencers who want to sell travel inventory to their audience - OTAs that want access direct relationships with suppliers and better quality hotel inventory
*
* The version of the OpenAPI document: 24.0.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package travel.wink.sdk.affiliate.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.util.ArrayList;
import java.util.List;
import travel.wink.sdk.affiliate.model.BeneficiaryAffiliate;
import travel.wink.sdk.affiliate.model.BookingUserAffiliate;
import travel.wink.sdk.affiliate.model.DailyRateAffiliate;
import travel.wink.sdk.affiliate.model.ItineraryAffiliate;
import travel.wink.sdk.affiliate.model.MoneysAffiliate;
import travel.wink.sdk.affiliate.model.SupplierContractItemPolicyAffiliate;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import javax.validation.constraints.*;
import javax.validation.Valid;
import org.hibernate.validator.constraints.*;
/**
* Holds one booking line item for a specific supplier.
*/
@ApiModel(description = "Holds one booking line item for a specific supplier.")
@JsonPropertyOrder({
BookingContractItemAffiliate.JSON_PROPERTY_SUPPLIER_ITEM_BOOKING_CODE,
BookingContractItemAffiliate.JSON_PROPERTY_USER,
BookingContractItemAffiliate.JSON_PROPERTY_NAME_IN_ENGLISH,
BookingContractItemAffiliate.JSON_PROPERTY_DESCRIPTION_IN_ENGLISH,
BookingContractItemAffiliate.JSON_PROPERTY_TOTAL_SOURCE_PRICE,
BookingContractItemAffiliate.JSON_PROPERTY_TOTAL_DISPLAY_PRICE,
BookingContractItemAffiliate.JSON_PROPERTY_TOTAL_SUPPLIER_PRICE,
BookingContractItemAffiliate.JSON_PROPERTY_TOTAL_INTERNAL_PRICE,
BookingContractItemAffiliate.JSON_PROPERTY_TOTAL_CAPTURE_PRICE,
BookingContractItemAffiliate.JSON_PROPERTY_ITINERARY,
BookingContractItemAffiliate.JSON_PROPERTY_PRICING_TYPE,
BookingContractItemAffiliate.JSON_PROPERTY_TYPE,
BookingContractItemAffiliate.JSON_PROPERTY_BENEFICIARY_LIST,
BookingContractItemAffiliate.JSON_PROPERTY_PAYABLE,
BookingContractItemAffiliate.JSON_PROPERTY_POLICY,
BookingContractItemAffiliate.JSON_PROPERTY_EXTERNAL_IDENTIFIER,
BookingContractItemAffiliate.JSON_PROPERTY_TOKENS_EARNED,
BookingContractItemAffiliate.JSON_PROPERTY_DAILY_RATE_LIST,
BookingContractItemAffiliate.JSON_PROPERTY_CANCELLED,
BookingContractItemAffiliate.JSON_PROPERTY_TOTAL_SOURCE_PRICE_AFTER_REFUND,
BookingContractItemAffiliate.JSON_PROPERTY_TOTAL_DISPLAY_PRICE_AFTER_REFUND,
BookingContractItemAffiliate.JSON_PROPERTY_TOTAL_SUPPLIER_PRICE_AFTER_REFUND,
BookingContractItemAffiliate.JSON_PROPERTY_TOTAL_INTERNAL_PRICE_AFTER_REFUND,
BookingContractItemAffiliate.JSON_PROPERTY_TOTAL_CAPTURE_PRICE_AFTER_REFUND,
BookingContractItemAffiliate.JSON_PROPERTY_CANCELLABLE_BY_SUPPLIER,
BookingContractItemAffiliate.JSON_PROPERTY_IS_CANCELLABLE_BY_SUPPLIER,
BookingContractItemAffiliate.JSON_PROPERTY_CANCELLABLE_BY_TRAVELER,
BookingContractItemAffiliate.JSON_PROPERTY_IS_CANCELLABLE_BY_TRAVELER,
BookingContractItemAffiliate.JSON_PROPERTY_CANCELLABLE_WITH_NO_CHARGES,
BookingContractItemAffiliate.JSON_PROPERTY_CANCELLABLE_WITH_POTENTIAL_CHARGES
})
@JsonTypeName("BookingContractItem_Affiliate")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2023-03-06T16:56:30.815925180+07:00[Asia/Bangkok]")
public class BookingContractItemAffiliate {
public static final String JSON_PROPERTY_SUPPLIER_ITEM_BOOKING_CODE = "supplierItemBookingCode";
private String supplierItemBookingCode;
public static final String JSON_PROPERTY_USER = "user";
private BookingUserAffiliate user;
public static final String JSON_PROPERTY_NAME_IN_ENGLISH = "nameInEnglish";
private String nameInEnglish;
public static final String JSON_PROPERTY_DESCRIPTION_IN_ENGLISH = "descriptionInEnglish";
private String descriptionInEnglish;
public static final String JSON_PROPERTY_TOTAL_SOURCE_PRICE = "totalSourcePrice";
private MoneysAffiliate totalSourcePrice;
public static final String JSON_PROPERTY_TOTAL_DISPLAY_PRICE = "totalDisplayPrice";
private MoneysAffiliate totalDisplayPrice;
public static final String JSON_PROPERTY_TOTAL_SUPPLIER_PRICE = "totalSupplierPrice";
private MoneysAffiliate totalSupplierPrice;
public static final String JSON_PROPERTY_TOTAL_INTERNAL_PRICE = "totalInternalPrice";
private MoneysAffiliate totalInternalPrice;
public static final String JSON_PROPERTY_TOTAL_CAPTURE_PRICE = "totalCapturePrice";
private MoneysAffiliate totalCapturePrice;
public static final String JSON_PROPERTY_ITINERARY = "itinerary";
private ItineraryAffiliate itinerary;
/**
* How to calculate the total amount.
*/
public enum PricingTypeEnum {
STAY("PER_STAY"),
DAY("PER_DAY"),
NIGHT("PER_NIGHT"),
USE("PER_USE"),
HOUR("PER_HOUR"),
PERSON("PER_PERSON"),
PERSON_PER_NIGHT("PER_PERSON_PER_NIGHT"),
PERSON_PER_HOUR("PER_PERSON_PER_HOUR"),
ADULT("PER_ADULT"),
ADULT_PER_NIGHT("PER_ADULT_PER_NIGHT"),
ADULT_PER_HOUR("PER_ADULT_PER_HOUR"),
CHILD("PER_CHILD"),
CHILD_PER_NIGHT("PER_CHILD_PER_NIGHT"),
CHILD_PER_HOUR("PER_CHILD_PER_HOUR");
private String value;
PricingTypeEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static PricingTypeEnum fromValue(String value) {
for (PricingTypeEnum b : PricingTypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
}
public static final String JSON_PROPERTY_PRICING_TYPE = "pricingType";
private PricingTypeEnum pricingType;
/**
* Type of item this is.
*/
public enum TypeEnum {
LODGING("LODGING"),
RAIL("RAIL"),
AIR("AIR"),
CAR("CAR"),
CRUISE("CRUISE"),
PACKAGE("PACKAGE"),
ADD_ON("ADD_ON"),
RENTAL("RENTAL"),
EXPERIENCE("EXPERIENCE"),
ANCILLARY_BOOKING("ANCILLARY_BOOKING"),
ANCILLARY_FEE("ANCILLARY_FEE");
private String value;
TypeEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static TypeEnum fromValue(String value) {
for (TypeEnum b : TypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
}
public static final String JSON_PROPERTY_TYPE = "type";
private TypeEnum type;
public static final String JSON_PROPERTY_BENEFICIARY_LIST = "beneficiaryList";
private List beneficiaryList = new ArrayList<>();
/**
* When to charge for this item.
*/
public enum PayableEnum {
IMMEDIATE("IMMEDIATE"),
ARRIVAL("ARRIVAL"),
DEPARTURE("DEPARTURE"),
AGENT("AGENT");
private String value;
PayableEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static PayableEnum fromValue(String value) {
for (PayableEnum b : PayableEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
}
public static final String JSON_PROPERTY_PAYABLE = "payable";
private PayableEnum payable;
public static final String JSON_PROPERTY_POLICY = "policy";
private SupplierContractItemPolicyAffiliate policy;
public static final String JSON_PROPERTY_EXTERNAL_IDENTIFIER = "externalIdentifier";
private String externalIdentifier;
public static final String JSON_PROPERTY_TOKENS_EARNED = "tokensEarned";
private Long tokensEarned;
public static final String JSON_PROPERTY_DAILY_RATE_LIST = "dailyRateList";
private List dailyRateList = null;
public static final String JSON_PROPERTY_CANCELLED = "cancelled";
private Boolean cancelled;
public static final String JSON_PROPERTY_TOTAL_SOURCE_PRICE_AFTER_REFUND = "totalSourcePriceAfterRefund";
private MoneysAffiliate totalSourcePriceAfterRefund;
public static final String JSON_PROPERTY_TOTAL_DISPLAY_PRICE_AFTER_REFUND = "totalDisplayPriceAfterRefund";
private MoneysAffiliate totalDisplayPriceAfterRefund;
public static final String JSON_PROPERTY_TOTAL_SUPPLIER_PRICE_AFTER_REFUND = "totalSupplierPriceAfterRefund";
private MoneysAffiliate totalSupplierPriceAfterRefund;
public static final String JSON_PROPERTY_TOTAL_INTERNAL_PRICE_AFTER_REFUND = "totalInternalPriceAfterRefund";
private MoneysAffiliate totalInternalPriceAfterRefund;
public static final String JSON_PROPERTY_TOTAL_CAPTURE_PRICE_AFTER_REFUND = "totalCapturePriceAfterRefund";
private MoneysAffiliate totalCapturePriceAfterRefund;
public static final String JSON_PROPERTY_CANCELLABLE_BY_SUPPLIER = "cancellableBySupplier";
private Boolean cancellableBySupplier;
public static final String JSON_PROPERTY_IS_CANCELLABLE_BY_SUPPLIER = "isCancellableBySupplier";
private Boolean isCancellableBySupplier;
public static final String JSON_PROPERTY_CANCELLABLE_BY_TRAVELER = "cancellableByTraveler";
private Boolean cancellableByTraveler;
public static final String JSON_PROPERTY_IS_CANCELLABLE_BY_TRAVELER = "isCancellableByTraveler";
private Boolean isCancellableByTraveler;
public static final String JSON_PROPERTY_CANCELLABLE_WITH_NO_CHARGES = "cancellableWithNoCharges";
private Boolean cancellableWithNoCharges;
public static final String JSON_PROPERTY_CANCELLABLE_WITH_POTENTIAL_CHARGES = "cancellableWithPotentialCharges";
private Boolean cancellableWithPotentialCharges;
public BookingContractItemAffiliate() {
}
public BookingContractItemAffiliate supplierItemBookingCode(String supplierItemBookingCode) {
this.supplierItemBookingCode = supplierItemBookingCode;
return this;
}
/**
* Booking code identifying the supplier line item.
* @return supplierItemBookingCode
**/
@javax.annotation.Nonnull
@NotNull
@ApiModelProperty(example = "TP-ASDFG1234", required = true, value = "Booking code identifying the supplier line item.")
@JsonProperty(JSON_PROPERTY_SUPPLIER_ITEM_BOOKING_CODE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getSupplierItemBookingCode() {
return supplierItemBookingCode;
}
@JsonProperty(JSON_PROPERTY_SUPPLIER_ITEM_BOOKING_CODE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setSupplierItemBookingCode(String supplierItemBookingCode) {
this.supplierItemBookingCode = supplierItemBookingCode;
}
public BookingContractItemAffiliate user(BookingUserAffiliate user) {
this.user = user;
return this;
}
/**
* Get user
* @return user
**/
@javax.annotation.Nonnull
@NotNull
@Valid
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_USER)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public BookingUserAffiliate getUser() {
return user;
}
@JsonProperty(JSON_PROPERTY_USER)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setUser(BookingUserAffiliate user) {
this.user = user;
}
public BookingContractItemAffiliate nameInEnglish(String nameInEnglish) {
this.nameInEnglish = nameInEnglish;
return this;
}
/**
* Name of item in English included in booking.
* @return nameInEnglish
**/
@javax.annotation.Nonnull
@NotNull
@ApiModelProperty(example = "Deluxe King", required = true, value = "Name of item in English included in booking.")
@JsonProperty(JSON_PROPERTY_NAME_IN_ENGLISH)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getNameInEnglish() {
return nameInEnglish;
}
@JsonProperty(JSON_PROPERTY_NAME_IN_ENGLISH)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setNameInEnglish(String nameInEnglish) {
this.nameInEnglish = nameInEnglish;
}
public BookingContractItemAffiliate descriptionInEnglish(String descriptionInEnglish) {
this.descriptionInEnglish = descriptionInEnglish;
return this;
}
/**
* Short description in English of item included in booking.
* @return descriptionInEnglish
**/
@javax.annotation.Nonnull
@NotNull
@ApiModelProperty(example = "This is the best deluxe king that money can buy.", required = true, value = "Short description in English of item included in booking.")
@JsonProperty(JSON_PROPERTY_DESCRIPTION_IN_ENGLISH)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getDescriptionInEnglish() {
return descriptionInEnglish;
}
@JsonProperty(JSON_PROPERTY_DESCRIPTION_IN_ENGLISH)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setDescriptionInEnglish(String descriptionInEnglish) {
this.descriptionInEnglish = descriptionInEnglish;
}
public BookingContractItemAffiliate totalSourcePrice(MoneysAffiliate totalSourcePrice) {
this.totalSourcePrice = totalSourcePrice;
return this;
}
/**
* Get totalSourcePrice
* @return totalSourcePrice
**/
@javax.annotation.Nonnull
@NotNull
@Valid
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_TOTAL_SOURCE_PRICE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public MoneysAffiliate getTotalSourcePrice() {
return totalSourcePrice;
}
@JsonProperty(JSON_PROPERTY_TOTAL_SOURCE_PRICE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setTotalSourcePrice(MoneysAffiliate totalSourcePrice) {
this.totalSourcePrice = totalSourcePrice;
}
public BookingContractItemAffiliate totalDisplayPrice(MoneysAffiliate totalDisplayPrice) {
this.totalDisplayPrice = totalDisplayPrice;
return this;
}
/**
* Get totalDisplayPrice
* @return totalDisplayPrice
**/
@javax.annotation.Nonnull
@NotNull
@Valid
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_TOTAL_DISPLAY_PRICE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public MoneysAffiliate getTotalDisplayPrice() {
return totalDisplayPrice;
}
@JsonProperty(JSON_PROPERTY_TOTAL_DISPLAY_PRICE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setTotalDisplayPrice(MoneysAffiliate totalDisplayPrice) {
this.totalDisplayPrice = totalDisplayPrice;
}
public BookingContractItemAffiliate totalSupplierPrice(MoneysAffiliate totalSupplierPrice) {
this.totalSupplierPrice = totalSupplierPrice;
return this;
}
/**
* Get totalSupplierPrice
* @return totalSupplierPrice
**/
@javax.annotation.Nonnull
@NotNull
@Valid
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_TOTAL_SUPPLIER_PRICE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public MoneysAffiliate getTotalSupplierPrice() {
return totalSupplierPrice;
}
@JsonProperty(JSON_PROPERTY_TOTAL_SUPPLIER_PRICE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setTotalSupplierPrice(MoneysAffiliate totalSupplierPrice) {
this.totalSupplierPrice = totalSupplierPrice;
}
public BookingContractItemAffiliate totalInternalPrice(MoneysAffiliate totalInternalPrice) {
this.totalInternalPrice = totalInternalPrice;
return this;
}
/**
* Get totalInternalPrice
* @return totalInternalPrice
**/
@javax.annotation.Nonnull
@NotNull
@Valid
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_TOTAL_INTERNAL_PRICE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public MoneysAffiliate getTotalInternalPrice() {
return totalInternalPrice;
}
@JsonProperty(JSON_PROPERTY_TOTAL_INTERNAL_PRICE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setTotalInternalPrice(MoneysAffiliate totalInternalPrice) {
this.totalInternalPrice = totalInternalPrice;
}
public BookingContractItemAffiliate totalCapturePrice(MoneysAffiliate totalCapturePrice) {
this.totalCapturePrice = totalCapturePrice;
return this;
}
/**
* Get totalCapturePrice
* @return totalCapturePrice
**/
@javax.annotation.Nonnull
@NotNull
@Valid
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_TOTAL_CAPTURE_PRICE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public MoneysAffiliate getTotalCapturePrice() {
return totalCapturePrice;
}
@JsonProperty(JSON_PROPERTY_TOTAL_CAPTURE_PRICE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setTotalCapturePrice(MoneysAffiliate totalCapturePrice) {
this.totalCapturePrice = totalCapturePrice;
}
public BookingContractItemAffiliate itinerary(ItineraryAffiliate itinerary) {
this.itinerary = itinerary;
return this;
}
/**
* Get itinerary
* @return itinerary
**/
@javax.annotation.Nonnull
@NotNull
@Valid
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_ITINERARY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public ItineraryAffiliate getItinerary() {
return itinerary;
}
@JsonProperty(JSON_PROPERTY_ITINERARY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setItinerary(ItineraryAffiliate itinerary) {
this.itinerary = itinerary;
}
public BookingContractItemAffiliate pricingType(PricingTypeEnum pricingType) {
this.pricingType = pricingType;
return this;
}
/**
* How to calculate the total amount.
* @return pricingType
**/
@javax.annotation.Nonnull
@NotNull
@ApiModelProperty(required = true, value = "How to calculate the total amount.")
@JsonProperty(JSON_PROPERTY_PRICING_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public PricingTypeEnum getPricingType() {
return pricingType;
}
@JsonProperty(JSON_PROPERTY_PRICING_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setPricingType(PricingTypeEnum pricingType) {
this.pricingType = pricingType;
}
public BookingContractItemAffiliate type(TypeEnum type) {
this.type = type;
return this;
}
/**
* Type of item this is.
* @return type
**/
@javax.annotation.Nonnull
@NotNull
@ApiModelProperty(example = "LODGING", required = true, value = "Type of item this is.")
@JsonProperty(JSON_PROPERTY_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public TypeEnum getType() {
return type;
}
@JsonProperty(JSON_PROPERTY_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setType(TypeEnum type) {
this.type = type;
}
public BookingContractItemAffiliate beneficiaryList(List beneficiaryList) {
this.beneficiaryList = beneficiaryList;
return this;
}
public BookingContractItemAffiliate addBeneficiaryListItem(BeneficiaryAffiliate beneficiaryListItem) {
this.beneficiaryList.add(beneficiaryListItem);
return this;
}
/**
* Get beneficiaryList
* @return beneficiaryList
**/
@javax.annotation.Nonnull
@NotNull
@Valid
@Size(min=1,max=2147483647) @ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_BENEFICIARY_LIST)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getBeneficiaryList() {
return beneficiaryList;
}
@JsonProperty(JSON_PROPERTY_BENEFICIARY_LIST)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setBeneficiaryList(List beneficiaryList) {
this.beneficiaryList = beneficiaryList;
}
public BookingContractItemAffiliate payable(PayableEnum payable) {
this.payable = payable;
return this;
}
/**
* When to charge for this item.
* @return payable
**/
@javax.annotation.Nonnull
@NotNull
@ApiModelProperty(example = "PREPAY", required = true, value = "When to charge for this item.")
@JsonProperty(JSON_PROPERTY_PAYABLE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public PayableEnum getPayable() {
return payable;
}
@JsonProperty(JSON_PROPERTY_PAYABLE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setPayable(PayableEnum payable) {
this.payable = payable;
}
public BookingContractItemAffiliate policy(SupplierContractItemPolicyAffiliate policy) {
this.policy = policy;
return this;
}
/**
* Get policy
* @return policy
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_POLICY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public SupplierContractItemPolicyAffiliate getPolicy() {
return policy;
}
@JsonProperty(JSON_PROPERTY_POLICY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPolicy(SupplierContractItemPolicyAffiliate policy) {
this.policy = policy;
}
public BookingContractItemAffiliate externalIdentifier(String externalIdentifier) {
this.externalIdentifier = externalIdentifier;
return this;
}
/**
* Optional geoname externalIdentifier to remote inventory.
* @return externalIdentifier
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "room-type-1", value = "Optional geoname externalIdentifier to remote inventory.")
@JsonProperty(JSON_PROPERTY_EXTERNAL_IDENTIFIER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getExternalIdentifier() {
return externalIdentifier;
}
@JsonProperty(JSON_PROPERTY_EXTERNAL_IDENTIFIER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setExternalIdentifier(String externalIdentifier) {
this.externalIdentifier = externalIdentifier;
}
public BookingContractItemAffiliate tokensEarned(Long tokensEarned) {
this.tokensEarned = tokensEarned;
return this;
}
/**
* Tokens earned for this item
* @return tokensEarned
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "12", value = "Tokens earned for this item")
@JsonProperty(JSON_PROPERTY_TOKENS_EARNED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getTokensEarned() {
return tokensEarned;
}
@JsonProperty(JSON_PROPERTY_TOKENS_EARNED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTokensEarned(Long tokensEarned) {
this.tokensEarned = tokensEarned;
}
public BookingContractItemAffiliate dailyRateList(List dailyRateList) {
this.dailyRateList = dailyRateList;
return this;
}
public BookingContractItemAffiliate addDailyRateListItem(DailyRateAffiliate dailyRateListItem) {
if (this.dailyRateList == null) {
this.dailyRateList = new ArrayList<>();
}
this.dailyRateList.add(dailyRateListItem);
return this;
}
/**
* Get dailyRateList
* @return dailyRateList
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_DAILY_RATE_LIST)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getDailyRateList() {
return dailyRateList;
}
@JsonProperty(JSON_PROPERTY_DAILY_RATE_LIST)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDailyRateList(List dailyRateList) {
this.dailyRateList = dailyRateList;
}
public BookingContractItemAffiliate cancelled(Boolean cancelled) {
this.cancelled = cancelled;
return this;
}
/**
* Optional geoname externalIdentifier to remote inventory.
* @return cancelled
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Optional geoname externalIdentifier to remote inventory.")
@JsonProperty(JSON_PROPERTY_CANCELLED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getCancelled() {
return cancelled;
}
@JsonProperty(JSON_PROPERTY_CANCELLED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCancelled(Boolean cancelled) {
this.cancelled = cancelled;
}
public BookingContractItemAffiliate totalSourcePriceAfterRefund(MoneysAffiliate totalSourcePriceAfterRefund) {
this.totalSourcePriceAfterRefund = totalSourcePriceAfterRefund;
return this;
}
/**
* Get totalSourcePriceAfterRefund
* @return totalSourcePriceAfterRefund
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_TOTAL_SOURCE_PRICE_AFTER_REFUND)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public MoneysAffiliate getTotalSourcePriceAfterRefund() {
return totalSourcePriceAfterRefund;
}
@JsonProperty(JSON_PROPERTY_TOTAL_SOURCE_PRICE_AFTER_REFUND)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTotalSourcePriceAfterRefund(MoneysAffiliate totalSourcePriceAfterRefund) {
this.totalSourcePriceAfterRefund = totalSourcePriceAfterRefund;
}
public BookingContractItemAffiliate totalDisplayPriceAfterRefund(MoneysAffiliate totalDisplayPriceAfterRefund) {
this.totalDisplayPriceAfterRefund = totalDisplayPriceAfterRefund;
return this;
}
/**
* Get totalDisplayPriceAfterRefund
* @return totalDisplayPriceAfterRefund
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_TOTAL_DISPLAY_PRICE_AFTER_REFUND)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public MoneysAffiliate getTotalDisplayPriceAfterRefund() {
return totalDisplayPriceAfterRefund;
}
@JsonProperty(JSON_PROPERTY_TOTAL_DISPLAY_PRICE_AFTER_REFUND)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTotalDisplayPriceAfterRefund(MoneysAffiliate totalDisplayPriceAfterRefund) {
this.totalDisplayPriceAfterRefund = totalDisplayPriceAfterRefund;
}
public BookingContractItemAffiliate totalSupplierPriceAfterRefund(MoneysAffiliate totalSupplierPriceAfterRefund) {
this.totalSupplierPriceAfterRefund = totalSupplierPriceAfterRefund;
return this;
}
/**
* Get totalSupplierPriceAfterRefund
* @return totalSupplierPriceAfterRefund
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_TOTAL_SUPPLIER_PRICE_AFTER_REFUND)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public MoneysAffiliate getTotalSupplierPriceAfterRefund() {
return totalSupplierPriceAfterRefund;
}
@JsonProperty(JSON_PROPERTY_TOTAL_SUPPLIER_PRICE_AFTER_REFUND)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTotalSupplierPriceAfterRefund(MoneysAffiliate totalSupplierPriceAfterRefund) {
this.totalSupplierPriceAfterRefund = totalSupplierPriceAfterRefund;
}
public BookingContractItemAffiliate totalInternalPriceAfterRefund(MoneysAffiliate totalInternalPriceAfterRefund) {
this.totalInternalPriceAfterRefund = totalInternalPriceAfterRefund;
return this;
}
/**
* Get totalInternalPriceAfterRefund
* @return totalInternalPriceAfterRefund
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_TOTAL_INTERNAL_PRICE_AFTER_REFUND)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public MoneysAffiliate getTotalInternalPriceAfterRefund() {
return totalInternalPriceAfterRefund;
}
@JsonProperty(JSON_PROPERTY_TOTAL_INTERNAL_PRICE_AFTER_REFUND)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTotalInternalPriceAfterRefund(MoneysAffiliate totalInternalPriceAfterRefund) {
this.totalInternalPriceAfterRefund = totalInternalPriceAfterRefund;
}
public BookingContractItemAffiliate totalCapturePriceAfterRefund(MoneysAffiliate totalCapturePriceAfterRefund) {
this.totalCapturePriceAfterRefund = totalCapturePriceAfterRefund;
return this;
}
/**
* Get totalCapturePriceAfterRefund
* @return totalCapturePriceAfterRefund
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_TOTAL_CAPTURE_PRICE_AFTER_REFUND)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public MoneysAffiliate getTotalCapturePriceAfterRefund() {
return totalCapturePriceAfterRefund;
}
@JsonProperty(JSON_PROPERTY_TOTAL_CAPTURE_PRICE_AFTER_REFUND)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTotalCapturePriceAfterRefund(MoneysAffiliate totalCapturePriceAfterRefund) {
this.totalCapturePriceAfterRefund = totalCapturePriceAfterRefund;
}
public BookingContractItemAffiliate cancellableBySupplier(Boolean cancellableBySupplier) {
this.cancellableBySupplier = cancellableBySupplier;
return this;
}
/**
* Whether the booking can still be cancelled by the supplier. A supplier cancellation overrides the refundable
* @return cancellableBySupplier
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Whether the booking can still be cancelled by the supplier. A supplier cancellation overrides the refundable")
@JsonProperty(JSON_PROPERTY_CANCELLABLE_BY_SUPPLIER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getCancellableBySupplier() {
return cancellableBySupplier;
}
@JsonProperty(JSON_PROPERTY_CANCELLABLE_BY_SUPPLIER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCancellableBySupplier(Boolean cancellableBySupplier) {
this.cancellableBySupplier = cancellableBySupplier;
}
public BookingContractItemAffiliate isCancellableBySupplier(Boolean isCancellableBySupplier) {
this.isCancellableBySupplier = isCancellableBySupplier;
return this;
}
/**
* Get isCancellableBySupplier
* @return isCancellableBySupplier
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_IS_CANCELLABLE_BY_SUPPLIER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getIsCancellableBySupplier() {
return isCancellableBySupplier;
}
@JsonProperty(JSON_PROPERTY_IS_CANCELLABLE_BY_SUPPLIER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setIsCancellableBySupplier(Boolean isCancellableBySupplier) {
this.isCancellableBySupplier = isCancellableBySupplier;
}
public BookingContractItemAffiliate cancellableByTraveler(Boolean cancellableByTraveler) {
this.cancellableByTraveler = cancellableByTraveler;
return this;
}
/**
* Whether the booking can still be cancelled by the traveller.
* @return cancellableByTraveler
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Whether the booking can still be cancelled by the traveller.")
@JsonProperty(JSON_PROPERTY_CANCELLABLE_BY_TRAVELER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getCancellableByTraveler() {
return cancellableByTraveler;
}
@JsonProperty(JSON_PROPERTY_CANCELLABLE_BY_TRAVELER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCancellableByTraveler(Boolean cancellableByTraveler) {
this.cancellableByTraveler = cancellableByTraveler;
}
public BookingContractItemAffiliate isCancellableByTraveler(Boolean isCancellableByTraveler) {
this.isCancellableByTraveler = isCancellableByTraveler;
return this;
}
/**
* Get isCancellableByTraveler
* @return isCancellableByTraveler
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_IS_CANCELLABLE_BY_TRAVELER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getIsCancellableByTraveler() {
return isCancellableByTraveler;
}
@JsonProperty(JSON_PROPERTY_IS_CANCELLABLE_BY_TRAVELER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setIsCancellableByTraveler(Boolean isCancellableByTraveler) {
this.isCancellableByTraveler = isCancellableByTraveler;
}
public BookingContractItemAffiliate cancellableWithNoCharges(Boolean cancellableWithNoCharges) {
this.cancellableWithNoCharges = cancellableWithNoCharges;
return this;
}
/**
* Whether the booking can still be cancelled and whether cancellation charges might still occur.
* @return cancellableWithNoCharges
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Whether the booking can still be cancelled and whether cancellation charges might still occur.")
@JsonProperty(JSON_PROPERTY_CANCELLABLE_WITH_NO_CHARGES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getCancellableWithNoCharges() {
return cancellableWithNoCharges;
}
@JsonProperty(JSON_PROPERTY_CANCELLABLE_WITH_NO_CHARGES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCancellableWithNoCharges(Boolean cancellableWithNoCharges) {
this.cancellableWithNoCharges = cancellableWithNoCharges;
}
public BookingContractItemAffiliate cancellableWithPotentialCharges(Boolean cancellableWithPotentialCharges) {
this.cancellableWithPotentialCharges = cancellableWithPotentialCharges;
return this;
}
/**
* Whether the booking can still be cancelled and whether cancellation charges might still occur.
* @return cancellableWithPotentialCharges
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Whether the booking can still be cancelled and whether cancellation charges might still occur.")
@JsonProperty(JSON_PROPERTY_CANCELLABLE_WITH_POTENTIAL_CHARGES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getCancellableWithPotentialCharges() {
return cancellableWithPotentialCharges;
}
@JsonProperty(JSON_PROPERTY_CANCELLABLE_WITH_POTENTIAL_CHARGES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCancellableWithPotentialCharges(Boolean cancellableWithPotentialCharges) {
this.cancellableWithPotentialCharges = cancellableWithPotentialCharges;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
BookingContractItemAffiliate bookingContractItemAffiliate = (BookingContractItemAffiliate) o;
return Objects.equals(this.supplierItemBookingCode, bookingContractItemAffiliate.supplierItemBookingCode) &&
Objects.equals(this.user, bookingContractItemAffiliate.user) &&
Objects.equals(this.nameInEnglish, bookingContractItemAffiliate.nameInEnglish) &&
Objects.equals(this.descriptionInEnglish, bookingContractItemAffiliate.descriptionInEnglish) &&
Objects.equals(this.totalSourcePrice, bookingContractItemAffiliate.totalSourcePrice) &&
Objects.equals(this.totalDisplayPrice, bookingContractItemAffiliate.totalDisplayPrice) &&
Objects.equals(this.totalSupplierPrice, bookingContractItemAffiliate.totalSupplierPrice) &&
Objects.equals(this.totalInternalPrice, bookingContractItemAffiliate.totalInternalPrice) &&
Objects.equals(this.totalCapturePrice, bookingContractItemAffiliate.totalCapturePrice) &&
Objects.equals(this.itinerary, bookingContractItemAffiliate.itinerary) &&
Objects.equals(this.pricingType, bookingContractItemAffiliate.pricingType) &&
Objects.equals(this.type, bookingContractItemAffiliate.type) &&
Objects.equals(this.beneficiaryList, bookingContractItemAffiliate.beneficiaryList) &&
Objects.equals(this.payable, bookingContractItemAffiliate.payable) &&
Objects.equals(this.policy, bookingContractItemAffiliate.policy) &&
Objects.equals(this.externalIdentifier, bookingContractItemAffiliate.externalIdentifier) &&
Objects.equals(this.tokensEarned, bookingContractItemAffiliate.tokensEarned) &&
Objects.equals(this.dailyRateList, bookingContractItemAffiliate.dailyRateList) &&
Objects.equals(this.cancelled, bookingContractItemAffiliate.cancelled) &&
Objects.equals(this.totalSourcePriceAfterRefund, bookingContractItemAffiliate.totalSourcePriceAfterRefund) &&
Objects.equals(this.totalDisplayPriceAfterRefund, bookingContractItemAffiliate.totalDisplayPriceAfterRefund) &&
Objects.equals(this.totalSupplierPriceAfterRefund, bookingContractItemAffiliate.totalSupplierPriceAfterRefund) &&
Objects.equals(this.totalInternalPriceAfterRefund, bookingContractItemAffiliate.totalInternalPriceAfterRefund) &&
Objects.equals(this.totalCapturePriceAfterRefund, bookingContractItemAffiliate.totalCapturePriceAfterRefund) &&
Objects.equals(this.cancellableBySupplier, bookingContractItemAffiliate.cancellableBySupplier) &&
Objects.equals(this.isCancellableBySupplier, bookingContractItemAffiliate.isCancellableBySupplier) &&
Objects.equals(this.cancellableByTraveler, bookingContractItemAffiliate.cancellableByTraveler) &&
Objects.equals(this.isCancellableByTraveler, bookingContractItemAffiliate.isCancellableByTraveler) &&
Objects.equals(this.cancellableWithNoCharges, bookingContractItemAffiliate.cancellableWithNoCharges) &&
Objects.equals(this.cancellableWithPotentialCharges, bookingContractItemAffiliate.cancellableWithPotentialCharges);
}
@Override
public int hashCode() {
return Objects.hash(supplierItemBookingCode, user, nameInEnglish, descriptionInEnglish, totalSourcePrice, totalDisplayPrice, totalSupplierPrice, totalInternalPrice, totalCapturePrice, itinerary, pricingType, type, beneficiaryList, payable, policy, externalIdentifier, tokensEarned, dailyRateList, cancelled, totalSourcePriceAfterRefund, totalDisplayPriceAfterRefund, totalSupplierPriceAfterRefund, totalInternalPriceAfterRefund, totalCapturePriceAfterRefund, cancellableBySupplier, isCancellableBySupplier, cancellableByTraveler, isCancellableByTraveler, cancellableWithNoCharges, cancellableWithPotentialCharges);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class BookingContractItemAffiliate {\n");
sb.append(" supplierItemBookingCode: ").append(toIndentedString(supplierItemBookingCode)).append("\n");
sb.append(" user: ").append(toIndentedString(user)).append("\n");
sb.append(" nameInEnglish: ").append(toIndentedString(nameInEnglish)).append("\n");
sb.append(" descriptionInEnglish: ").append(toIndentedString(descriptionInEnglish)).append("\n");
sb.append(" totalSourcePrice: ").append(toIndentedString(totalSourcePrice)).append("\n");
sb.append(" totalDisplayPrice: ").append(toIndentedString(totalDisplayPrice)).append("\n");
sb.append(" totalSupplierPrice: ").append(toIndentedString(totalSupplierPrice)).append("\n");
sb.append(" totalInternalPrice: ").append(toIndentedString(totalInternalPrice)).append("\n");
sb.append(" totalCapturePrice: ").append(toIndentedString(totalCapturePrice)).append("\n");
sb.append(" itinerary: ").append(toIndentedString(itinerary)).append("\n");
sb.append(" pricingType: ").append(toIndentedString(pricingType)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" beneficiaryList: ").append(toIndentedString(beneficiaryList)).append("\n");
sb.append(" payable: ").append(toIndentedString(payable)).append("\n");
sb.append(" policy: ").append(toIndentedString(policy)).append("\n");
sb.append(" externalIdentifier: ").append(toIndentedString(externalIdentifier)).append("\n");
sb.append(" tokensEarned: ").append(toIndentedString(tokensEarned)).append("\n");
sb.append(" dailyRateList: ").append(toIndentedString(dailyRateList)).append("\n");
sb.append(" cancelled: ").append(toIndentedString(cancelled)).append("\n");
sb.append(" totalSourcePriceAfterRefund: ").append(toIndentedString(totalSourcePriceAfterRefund)).append("\n");
sb.append(" totalDisplayPriceAfterRefund: ").append(toIndentedString(totalDisplayPriceAfterRefund)).append("\n");
sb.append(" totalSupplierPriceAfterRefund: ").append(toIndentedString(totalSupplierPriceAfterRefund)).append("\n");
sb.append(" totalInternalPriceAfterRefund: ").append(toIndentedString(totalInternalPriceAfterRefund)).append("\n");
sb.append(" totalCapturePriceAfterRefund: ").append(toIndentedString(totalCapturePriceAfterRefund)).append("\n");
sb.append(" cancellableBySupplier: ").append(toIndentedString(cancellableBySupplier)).append("\n");
sb.append(" isCancellableBySupplier: ").append(toIndentedString(isCancellableBySupplier)).append("\n");
sb.append(" cancellableByTraveler: ").append(toIndentedString(cancellableByTraveler)).append("\n");
sb.append(" isCancellableByTraveler: ").append(toIndentedString(isCancellableByTraveler)).append("\n");
sb.append(" cancellableWithNoCharges: ").append(toIndentedString(cancellableWithNoCharges)).append("\n");
sb.append(" cancellableWithPotentialCharges: ").append(toIndentedString(cancellableWithPotentialCharges)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy