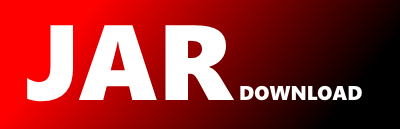
travel.wink.sdk.affiliate.model.DynamicSellerListItemRequestAffiliate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of affiliate-sdk-java Show documentation
Show all versions of affiliate-sdk-java Show documentation
Java SDK for the wink Affiliate API
/*
* Wink API
* ## APIs Not every integrator needs every APIs. For that reason, we have separated APIs into context. - [Affiliate](/affiliate): All APIs related to selling travel inventory as an affiliate. - [Analytics](/analytics): All APIs related to tracking metrics across a wide variety of data source segments including, more entertaining, leaderboard metrics. - [Booking](/booking): All APIs related to creating platform bookings. - [Channel Manager](/channel-manager): All APIs related to channel managers who want to integrate with our platform. - [Extranet](/extranet): All APIs related to managing travel inventory and suppliers. - [Inventory](/inventory): All APIs related to retrieve known travel inventory as it was found using the Lookup API.. - [Lookup](/lookup): All APIs related to locating inventory by region, locale and property flags. - [Reference](/reference): All APIs related to retrieving platform-supported taxonomies. - [TripPay Acquiring](/payment-acquiring): All APIs related to capture payment details such as a Stripe payment intent. - [TripPay](/payment): All APIs related to TripPay account management, booking, mapping and integration features. ## SDKs We are actively working on supporting the most used languages out there. If you don't see your language here, reach out to us with a request to officially add your language. In the meantime, if you want to roll your own SDK, you can do so by downloading the OpenAPI spec and using one of the many available OpenAPI generators available: [https://openapi-generator.tech/docs/generators](https://openapi-generator.tech/docs/generators). - Java SDK [https://github.com/wink-travel/wink-sdk-java](https://github.com/wink-travel/wink-sdk-java) ## Usage These features are made available to you via a [REST API](https://en.wikipedia.org/wiki/Representational_state_transfer). This API is language agnostic. ## Versioning We chose to version our endpoints in a way that we hope affects your integration with us the least. You request the version of our API you wish to work with via the `Wink-Version` header. When it's time for you to upgrade, you only have to change the version number to get access to our updated endpoints. ## Release history - 2022-10-15: v2.0 - Removed HATEOAS and added Wink-Version header - 2022-05-08: v1 - Exposed channel manager API - 2021-07-01: v1 - Initial release # Affiliate API Welcome to the Affiliate API - A programmer-friendly way to search for and display bespoke travel inventory for your audience. Use this API to help you prepare travel inventory for sale. # Intended Audience Programmers are a requirement to start integrating with wink. You will benefit from an API integration if you are new or existing travel related company that want easy access to great inventory.## Examples: - Hotel brands / chains that want to make their own booking engine - Travel tech companies that want to create the next hot mobile travel app - Destination sites that want to make their own booking engine - Bloggers and influencers who want to sell travel inventory to their audience - OTAs that want access direct relationships with suppliers and better quality hotel inventory
*
* The version of the OpenAPI document: 24.0.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package travel.wink.sdk.affiliate.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.util.ArrayList;
import java.util.List;
import travel.wink.sdk.affiliate.model.GeoJsonPointAffiliate;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import javax.validation.constraints.*;
import javax.validation.Valid;
import org.hibernate.validator.constraints.*;
/**
* Contains the caller's search criteria.
*/
@ApiModel(description = "Contains the caller's search criteria.")
@JsonPropertyOrder({
DynamicSellerListItemRequestAffiliate.JSON_PROPERTY_NAME,
DynamicSellerListItemRequestAffiliate.JSON_PROPERTY_PROPERTY_NAME,
DynamicSellerListItemRequestAffiliate.JSON_PROPERTY_INVENTORY_NAME,
DynamicSellerListItemRequestAffiliate.JSON_PROPERTY_CONTINENTS,
DynamicSellerListItemRequestAffiliate.JSON_PROPERTY_COUNTRIES,
DynamicSellerListItemRequestAffiliate.JSON_PROPERTY_CITIES,
DynamicSellerListItemRequestAffiliate.JSON_PROPERTY_SHOW_ECO_FRIENDLY,
DynamicSellerListItemRequestAffiliate.JSON_PROPERTY_SHOW_PET_FRIENDLY,
DynamicSellerListItemRequestAffiliate.JSON_PROPERTY_SHOW_CHILD_FRIENDLY,
DynamicSellerListItemRequestAffiliate.JSON_PROPERTY_SHOW_POPULAR,
DynamicSellerListItemRequestAffiliate.JSON_PROPERTY_SHOW_DIRECT_ONLY,
DynamicSellerListItemRequestAffiliate.JSON_PROPERTY_LIFESTYLES,
DynamicSellerListItemRequestAffiliate.JSON_PROPERTY_HOTEL_STARS,
DynamicSellerListItemRequestAffiliate.JSON_PROPERTY_AGGREGATE_REVIEW_RATING,
DynamicSellerListItemRequestAffiliate.JSON_PROPERTY_NEAR_POINT,
DynamicSellerListItemRequestAffiliate.JSON_PROPERTY_RADIUS_IN_METERS,
DynamicSellerListItemRequestAffiliate.JSON_PROPERTY_INVENTORY_TYPES,
DynamicSellerListItemRequestAffiliate.JSON_PROPERTY_DEFAULT_CURRENCY_CODE,
DynamicSellerListItemRequestAffiliate.JSON_PROPERTY_PRIMARY_ORDER_BY
})
@JsonTypeName("DynamicSellerListItemRequest_Affiliate")
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2023-03-06T16:56:30.815925180+07:00[Asia/Bangkok]")
public class DynamicSellerListItemRequestAffiliate {
public static final String JSON_PROPERTY_NAME = "name";
private String name;
public static final String JSON_PROPERTY_PROPERTY_NAME = "propertyName";
private String propertyName;
public static final String JSON_PROPERTY_INVENTORY_NAME = "inventoryName";
private String inventoryName;
public static final String JSON_PROPERTY_CONTINENTS = "continents";
private List continents = null;
public static final String JSON_PROPERTY_COUNTRIES = "countries";
private List countries = null;
public static final String JSON_PROPERTY_CITIES = "cities";
private List cities = null;
public static final String JSON_PROPERTY_SHOW_ECO_FRIENDLY = "showEcoFriendly";
private Boolean showEcoFriendly = false;
public static final String JSON_PROPERTY_SHOW_PET_FRIENDLY = "showPetFriendly";
private Boolean showPetFriendly = false;
public static final String JSON_PROPERTY_SHOW_CHILD_FRIENDLY = "showChildFriendly";
private Boolean showChildFriendly = false;
public static final String JSON_PROPERTY_SHOW_POPULAR = "showPopular";
private Boolean showPopular = false;
public static final String JSON_PROPERTY_SHOW_DIRECT_ONLY = "showDirectOnly";
private Boolean showDirectOnly = false;
/**
* Filter on lifestyles
*/
public enum LifestylesEnum {
HEALTH_FITNESS("LIFESTYLE_HEALTH_FITNESS"),
RELAX("LIFESTYLE_RELAX"),
ADULT_ONLY("LIFESTYLE_ADULT_ONLY"),
ADVENTURE("LIFESTYLE_ADVENTURE"),
BUSINESS("LIFESTYLE_BUSINESS"),
LGBT("LIFESTYLE_LGBT"),
SINGLE_PARENT("LIFESTYLE_SINGLE_PARENT"),
SOLO_FEMALE("LIFESTYLE_SOLO_FEMALE"),
BEAUTY("LIFESTYLE_BEAUTY"),
FOODIE("LIFESTYLE_FOODIE"),
FAMILY("LIFESTYLE_FAMILY"),
ROMANCE("LIFESTYLE_ROMANCE"),
COUPLE("LIFESTYLE_COUPLE"),
SOLO("LIFESTYLE_SOLO"),
BACKPACKER("LIFESTYLE_BACKPACKER"),
SHOPPING("LIFESTYLE_SHOPPING"),
SPORTS("LIFESTYLE_SPORTS"),
MOUNTAIN("LIFESTYLE_MOUNTAIN"),
BEACH("LIFESTYLE_BEACH"),
CITY("LIFESTYLE_CITY"),
COUNTRY("LIFESTYLE_COUNTRY"),
CULTURE("LIFESTYLE_CULTURE"),
ECO("LIFESTYLE_ECO");
private String value;
LifestylesEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static LifestylesEnum fromValue(String value) {
for (LifestylesEnum b : LifestylesEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
}
public static final String JSON_PROPERTY_LIFESTYLES = "lifestyles";
private List lifestyles = null;
public static final String JSON_PROPERTY_HOTEL_STARS = "hotelStars";
private Integer hotelStars;
public static final String JSON_PROPERTY_AGGREGATE_REVIEW_RATING = "aggregateReviewRating";
private Integer aggregateReviewRating;
public static final String JSON_PROPERTY_NEAR_POINT = "nearPoint";
private GeoJsonPointAffiliate nearPoint;
public static final String JSON_PROPERTY_RADIUS_IN_METERS = "radiusInMeters";
private Long radiusInMeters;
/**
* Gets or Sets inventoryTypes
*/
public enum InventoryTypesEnum {
GUEST_ROOM("GUEST_ROOM"),
ADD_ON("ADD_ON"),
MEETING_ROOM("MEETING_ROOM"),
RESTAURANT("RESTAURANT"),
SPA("SPA"),
ATTRACTION("ATTRACTION"),
PLACE("PLACE"),
ACTIVITY("ACTIVITY");
private String value;
InventoryTypesEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static InventoryTypesEnum fromValue(String value) {
for (InventoryTypesEnum b : InventoryTypesEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
}
public static final String JSON_PROPERTY_INVENTORY_TYPES = "inventoryTypes";
private List inventoryTypes = null;
public static final String JSON_PROPERTY_DEFAULT_CURRENCY_CODE = "defaultCurrencyCode";
private String defaultCurrencyCode = "USD";
public static final String JSON_PROPERTY_PRIMARY_ORDER_BY = "primaryOrderBy";
private String primaryOrderBy;
public DynamicSellerListItemRequestAffiliate() {
}
public DynamicSellerListItemRequestAffiliate name(String name) {
this.name = name;
return this;
}
/**
* Name of dynamic list for when user want to persist it
* @return name
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Name of dynamic list for when user want to persist it")
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getName() {
return name;
}
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setName(String name) {
this.name = name;
}
public DynamicSellerListItemRequestAffiliate propertyName(String propertyName) {
this.propertyName = propertyName;
return this;
}
/**
* Regex expression filter matching on property name.
* @return propertyName
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Shera", value = "Regex expression filter matching on property name.")
@JsonProperty(JSON_PROPERTY_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getPropertyName() {
return propertyName;
}
@JsonProperty(JSON_PROPERTY_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPropertyName(String propertyName) {
this.propertyName = propertyName;
}
public DynamicSellerListItemRequestAffiliate inventoryName(String inventoryName) {
this.inventoryName = inventoryName;
return this;
}
/**
* Regex expression filter matching on inventory name
* @return inventoryName
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "Badmint", value = "Regex expression filter matching on inventory name")
@JsonProperty(JSON_PROPERTY_INVENTORY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getInventoryName() {
return inventoryName;
}
@JsonProperty(JSON_PROPERTY_INVENTORY_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setInventoryName(String inventoryName) {
this.inventoryName = inventoryName;
}
public DynamicSellerListItemRequestAffiliate continents(List continents) {
this.continents = continents;
return this;
}
public DynamicSellerListItemRequestAffiliate addContinentsItem(String continentsItem) {
if (this.continents == null) {
this.continents = new ArrayList<>();
}
this.continents.add(continentsItem);
return this;
}
/**
* Continent filter
* @return continents
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Continent filter")
@JsonProperty(JSON_PROPERTY_CONTINENTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getContinents() {
return continents;
}
@JsonProperty(JSON_PROPERTY_CONTINENTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setContinents(List continents) {
this.continents = continents;
}
public DynamicSellerListItemRequestAffiliate countries(List countries) {
this.countries = countries;
return this;
}
public DynamicSellerListItemRequestAffiliate addCountriesItem(String countriesItem) {
if (this.countries == null) {
this.countries = new ArrayList<>();
}
this.countries.add(countriesItem);
return this;
}
/**
* Country filter
* @return countries
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Country filter")
@JsonProperty(JSON_PROPERTY_COUNTRIES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getCountries() {
return countries;
}
@JsonProperty(JSON_PROPERTY_COUNTRIES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCountries(List countries) {
this.countries = countries;
}
public DynamicSellerListItemRequestAffiliate cities(List cities) {
this.cities = cities;
return this;
}
public DynamicSellerListItemRequestAffiliate addCitiesItem(String citiesItem) {
if (this.cities == null) {
this.cities = new ArrayList<>();
}
this.cities.add(citiesItem);
return this;
}
/**
* City filter
* @return cities
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "City filter")
@JsonProperty(JSON_PROPERTY_CITIES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getCities() {
return cities;
}
@JsonProperty(JSON_PROPERTY_CITIES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCities(List cities) {
this.cities = cities;
}
public DynamicSellerListItemRequestAffiliate showEcoFriendly(Boolean showEcoFriendly) {
this.showEcoFriendly = showEcoFriendly;
return this;
}
/**
* Filter on eco-friendly hotels
* @return showEcoFriendly
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "false", value = "Filter on eco-friendly hotels")
@JsonProperty(JSON_PROPERTY_SHOW_ECO_FRIENDLY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getShowEcoFriendly() {
return showEcoFriendly;
}
@JsonProperty(JSON_PROPERTY_SHOW_ECO_FRIENDLY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setShowEcoFriendly(Boolean showEcoFriendly) {
this.showEcoFriendly = showEcoFriendly;
}
public DynamicSellerListItemRequestAffiliate showPetFriendly(Boolean showPetFriendly) {
this.showPetFriendly = showPetFriendly;
return this;
}
/**
* Filter on pet-friendly hotels
* @return showPetFriendly
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "false", value = "Filter on pet-friendly hotels")
@JsonProperty(JSON_PROPERTY_SHOW_PET_FRIENDLY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getShowPetFriendly() {
return showPetFriendly;
}
@JsonProperty(JSON_PROPERTY_SHOW_PET_FRIENDLY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setShowPetFriendly(Boolean showPetFriendly) {
this.showPetFriendly = showPetFriendly;
}
public DynamicSellerListItemRequestAffiliate showChildFriendly(Boolean showChildFriendly) {
this.showChildFriendly = showChildFriendly;
return this;
}
/**
* Filter on child-friendly hotels
* @return showChildFriendly
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "false", value = "Filter on child-friendly hotels")
@JsonProperty(JSON_PROPERTY_SHOW_CHILD_FRIENDLY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getShowChildFriendly() {
return showChildFriendly;
}
@JsonProperty(JSON_PROPERTY_SHOW_CHILD_FRIENDLY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setShowChildFriendly(Boolean showChildFriendly) {
this.showChildFriendly = showChildFriendly;
}
public DynamicSellerListItemRequestAffiliate showPopular(Boolean showPopular) {
this.showPopular = showPopular;
return this;
}
/**
* Filter on hotel that has had a certain amount of bookings
* @return showPopular
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "false", value = "Filter on hotel that has had a certain amount of bookings")
@JsonProperty(JSON_PROPERTY_SHOW_POPULAR)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getShowPopular() {
return showPopular;
}
@JsonProperty(JSON_PROPERTY_SHOW_POPULAR)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setShowPopular(Boolean showPopular) {
this.showPopular = showPopular;
}
public DynamicSellerListItemRequestAffiliate showDirectOnly(Boolean showDirectOnly) {
this.showDirectOnly = showDirectOnly;
return this;
}
/**
* Filter on direct inventory
* @return showDirectOnly
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "false", value = "Filter on direct inventory")
@JsonProperty(JSON_PROPERTY_SHOW_DIRECT_ONLY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getShowDirectOnly() {
return showDirectOnly;
}
@JsonProperty(JSON_PROPERTY_SHOW_DIRECT_ONLY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setShowDirectOnly(Boolean showDirectOnly) {
this.showDirectOnly = showDirectOnly;
}
public DynamicSellerListItemRequestAffiliate lifestyles(List lifestyles) {
this.lifestyles = lifestyles;
return this;
}
public DynamicSellerListItemRequestAffiliate addLifestylesItem(LifestylesEnum lifestylesItem) {
if (this.lifestyles == null) {
this.lifestyles = new ArrayList<>();
}
this.lifestyles.add(lifestylesItem);
return this;
}
/**
* Filter on lifestyles
* @return lifestyles
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "[\"LIFESTYLE_RELAX\"]", value = "Filter on lifestyles")
@JsonProperty(JSON_PROPERTY_LIFESTYLES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getLifestyles() {
return lifestyles;
}
@JsonProperty(JSON_PROPERTY_LIFESTYLES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setLifestyles(List lifestyles) {
this.lifestyles = lifestyles;
}
public DynamicSellerListItemRequestAffiliate hotelStars(Integer hotelStars) {
this.hotelStars = hotelStars;
return this;
}
/**
* Filter on number of stars the hotel has.
* @return hotelStars
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "4", value = "Filter on number of stars the hotel has.")
@JsonProperty(JSON_PROPERTY_HOTEL_STARS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getHotelStars() {
return hotelStars;
}
@JsonProperty(JSON_PROPERTY_HOTEL_STARS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setHotelStars(Integer hotelStars) {
this.hotelStars = hotelStars;
}
public DynamicSellerListItemRequestAffiliate aggregateReviewRating(Integer aggregateReviewRating) {
this.aggregateReviewRating = aggregateReviewRating;
return this;
}
/**
* Filter on aggregate review score the hotel has
* @return aggregateReviewRating
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "7", value = "Filter on aggregate review score the hotel has")
@JsonProperty(JSON_PROPERTY_AGGREGATE_REVIEW_RATING)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getAggregateReviewRating() {
return aggregateReviewRating;
}
@JsonProperty(JSON_PROPERTY_AGGREGATE_REVIEW_RATING)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAggregateReviewRating(Integer aggregateReviewRating) {
this.aggregateReviewRating = aggregateReviewRating;
}
public DynamicSellerListItemRequestAffiliate nearPoint(GeoJsonPointAffiliate nearPoint) {
this.nearPoint = nearPoint;
return this;
}
/**
* Get nearPoint
* @return nearPoint
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_NEAR_POINT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public GeoJsonPointAffiliate getNearPoint() {
return nearPoint;
}
@JsonProperty(JSON_PROPERTY_NEAR_POINT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setNearPoint(GeoJsonPointAffiliate nearPoint) {
this.nearPoint = nearPoint;
}
public DynamicSellerListItemRequestAffiliate radiusInMeters(Long radiusInMeters) {
this.radiusInMeters = radiusInMeters;
return this;
}
/**
* Use this in conjunction with `nearPoint`. Control the distance from point we are searching for.
* @return radiusInMeters
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "Use this in conjunction with `nearPoint`. Control the distance from point we are searching for.")
@JsonProperty(JSON_PROPERTY_RADIUS_IN_METERS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Long getRadiusInMeters() {
return radiusInMeters;
}
@JsonProperty(JSON_PROPERTY_RADIUS_IN_METERS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRadiusInMeters(Long radiusInMeters) {
this.radiusInMeters = radiusInMeters;
}
public DynamicSellerListItemRequestAffiliate inventoryTypes(List inventoryTypes) {
this.inventoryTypes = inventoryTypes;
return this;
}
public DynamicSellerListItemRequestAffiliate addInventoryTypesItem(InventoryTypesEnum inventoryTypesItem) {
if (this.inventoryTypes == null) {
this.inventoryTypes = new ArrayList<>();
}
this.inventoryTypes.add(inventoryTypesItem);
return this;
}
/**
* Filter on inventory types
* @return inventoryTypes
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "[\"GUEST_ROOM\"]", value = "Filter on inventory types")
@JsonProperty(JSON_PROPERTY_INVENTORY_TYPES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getInventoryTypes() {
return inventoryTypes;
}
@JsonProperty(JSON_PROPERTY_INVENTORY_TYPES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setInventoryTypes(List inventoryTypes) {
this.inventoryTypes = inventoryTypes;
}
public DynamicSellerListItemRequestAffiliate defaultCurrencyCode(String defaultCurrencyCode) {
this.defaultCurrencyCode = defaultCurrencyCode;
return this;
}
/**
* Control which currency you want to see prices in.
* @return defaultCurrencyCode
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "USD", value = "Control which currency you want to see prices in.")
@JsonProperty(JSON_PROPERTY_DEFAULT_CURRENCY_CODE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getDefaultCurrencyCode() {
return defaultCurrencyCode;
}
@JsonProperty(JSON_PROPERTY_DEFAULT_CURRENCY_CODE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDefaultCurrencyCode(String defaultCurrencyCode) {
this.defaultCurrencyCode = defaultCurrencyCode;
}
public DynamicSellerListItemRequestAffiliate primaryOrderBy(String primaryOrderBy) {
this.primaryOrderBy = primaryOrderBy;
return this;
}
/**
* Control how you want the search results sorted. Options are: - 1: Inventory name - 2: Price: High to low - 3: Price: Low to high - 4: Commission: High to low - 5: Commission: Low to high - 6: Discount: High to low - 7: Discount: Low to high
* @return primaryOrderBy
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "4", value = "Control how you want the search results sorted. Options are: - 1: Inventory name - 2: Price: High to low - 3: Price: Low to high - 4: Commission: High to low - 5: Commission: Low to high - 6: Discount: High to low - 7: Discount: Low to high ")
@JsonProperty(JSON_PROPERTY_PRIMARY_ORDER_BY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getPrimaryOrderBy() {
return primaryOrderBy;
}
@JsonProperty(JSON_PROPERTY_PRIMARY_ORDER_BY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPrimaryOrderBy(String primaryOrderBy) {
this.primaryOrderBy = primaryOrderBy;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DynamicSellerListItemRequestAffiliate dynamicSellerListItemRequestAffiliate = (DynamicSellerListItemRequestAffiliate) o;
return Objects.equals(this.name, dynamicSellerListItemRequestAffiliate.name) &&
Objects.equals(this.propertyName, dynamicSellerListItemRequestAffiliate.propertyName) &&
Objects.equals(this.inventoryName, dynamicSellerListItemRequestAffiliate.inventoryName) &&
Objects.equals(this.continents, dynamicSellerListItemRequestAffiliate.continents) &&
Objects.equals(this.countries, dynamicSellerListItemRequestAffiliate.countries) &&
Objects.equals(this.cities, dynamicSellerListItemRequestAffiliate.cities) &&
Objects.equals(this.showEcoFriendly, dynamicSellerListItemRequestAffiliate.showEcoFriendly) &&
Objects.equals(this.showPetFriendly, dynamicSellerListItemRequestAffiliate.showPetFriendly) &&
Objects.equals(this.showChildFriendly, dynamicSellerListItemRequestAffiliate.showChildFriendly) &&
Objects.equals(this.showPopular, dynamicSellerListItemRequestAffiliate.showPopular) &&
Objects.equals(this.showDirectOnly, dynamicSellerListItemRequestAffiliate.showDirectOnly) &&
Objects.equals(this.lifestyles, dynamicSellerListItemRequestAffiliate.lifestyles) &&
Objects.equals(this.hotelStars, dynamicSellerListItemRequestAffiliate.hotelStars) &&
Objects.equals(this.aggregateReviewRating, dynamicSellerListItemRequestAffiliate.aggregateReviewRating) &&
Objects.equals(this.nearPoint, dynamicSellerListItemRequestAffiliate.nearPoint) &&
Objects.equals(this.radiusInMeters, dynamicSellerListItemRequestAffiliate.radiusInMeters) &&
Objects.equals(this.inventoryTypes, dynamicSellerListItemRequestAffiliate.inventoryTypes) &&
Objects.equals(this.defaultCurrencyCode, dynamicSellerListItemRequestAffiliate.defaultCurrencyCode) &&
Objects.equals(this.primaryOrderBy, dynamicSellerListItemRequestAffiliate.primaryOrderBy);
}
@Override
public int hashCode() {
return Objects.hash(name, propertyName, inventoryName, continents, countries, cities, showEcoFriendly, showPetFriendly, showChildFriendly, showPopular, showDirectOnly, lifestyles, hotelStars, aggregateReviewRating, nearPoint, radiusInMeters, inventoryTypes, defaultCurrencyCode, primaryOrderBy);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class DynamicSellerListItemRequestAffiliate {\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" propertyName: ").append(toIndentedString(propertyName)).append("\n");
sb.append(" inventoryName: ").append(toIndentedString(inventoryName)).append("\n");
sb.append(" continents: ").append(toIndentedString(continents)).append("\n");
sb.append(" countries: ").append(toIndentedString(countries)).append("\n");
sb.append(" cities: ").append(toIndentedString(cities)).append("\n");
sb.append(" showEcoFriendly: ").append(toIndentedString(showEcoFriendly)).append("\n");
sb.append(" showPetFriendly: ").append(toIndentedString(showPetFriendly)).append("\n");
sb.append(" showChildFriendly: ").append(toIndentedString(showChildFriendly)).append("\n");
sb.append(" showPopular: ").append(toIndentedString(showPopular)).append("\n");
sb.append(" showDirectOnly: ").append(toIndentedString(showDirectOnly)).append("\n");
sb.append(" lifestyles: ").append(toIndentedString(lifestyles)).append("\n");
sb.append(" hotelStars: ").append(toIndentedString(hotelStars)).append("\n");
sb.append(" aggregateReviewRating: ").append(toIndentedString(aggregateReviewRating)).append("\n");
sb.append(" nearPoint: ").append(toIndentedString(nearPoint)).append("\n");
sb.append(" radiusInMeters: ").append(toIndentedString(radiusInMeters)).append("\n");
sb.append(" inventoryTypes: ").append(toIndentedString(inventoryTypes)).append("\n");
sb.append(" defaultCurrencyCode: ").append(toIndentedString(defaultCurrencyCode)).append("\n");
sb.append(" primaryOrderBy: ").append(toIndentedString(primaryOrderBy)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy