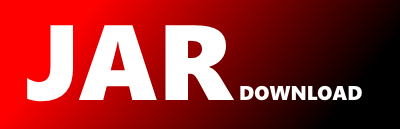
travel.wink.sdk.affiliate.syndication.api.SyndicationConsumerApi Maven / Gradle / Ivy
Show all versions of affiliate-winklinks-sdk-java Show documentation
package travel.wink.sdk.affiliate.syndication.api;
import travel.wink.sdk.affiliate.syndication.invoker.ApiClient;
import travel.wink.sdk.affiliate.syndication.model.BooleanResponseNonAuthenticatedEntity;
import travel.wink.sdk.affiliate.syndication.model.FacebookEmbedNonAuthenticatedEntity;
import travel.wink.sdk.affiliate.syndication.model.GenericErrorMessage;
import travel.wink.sdk.affiliate.syndication.model.InstagramEmbedNonAuthenticatedEntity;
import travel.wink.sdk.affiliate.syndication.model.KeyValuePairNonAuthenticatedEntity;
import travel.wink.sdk.affiliate.syndication.model.PageConsumableSyndicationEntryNonAuthenticatedEntity;
import travel.wink.sdk.affiliate.syndication.model.RedirectViewNonAuthenticatedEntity;
import travel.wink.sdk.affiliate.syndication.model.ShowSyndicationSettings400Response;
import travel.wink.sdk.affiliate.syndication.model.SpotifyEmbedNonAuthenticatedEntity;
import travel.wink.sdk.affiliate.syndication.model.StateNonAuthenticatedEntity;
import travel.wink.sdk.affiliate.syndication.model.SyndicationAccountNonAuthenticatedEntity;
import travel.wink.sdk.affiliate.syndication.model.TwitterEmbedNonAuthenticatedEntity;
import java.util.HashMap;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import java.util.stream.Collectors;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
import org.springframework.core.ParameterizedTypeReference;
import org.springframework.web.reactive.function.client.WebClient.ResponseSpec;
import org.springframework.web.reactive.function.client.WebClientResponseException;
import org.springframework.core.io.FileSystemResource;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpMethod;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import reactor.core.publisher.Mono;
import reactor.core.publisher.Flux;
@jakarta.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2025-01-02T16:41:57.763280625+07:00[Asia/Bangkok]")
public class SyndicationConsumerApi {
private ApiClient apiClient;
public SyndicationConsumerApi() {
this(new ApiClient());
}
@Autowired
public SyndicationConsumerApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Consume Facebook Page URL
* Utility method for reading oEmbed data for Facebook.
* 500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Consume URL for this owner identifier.
* @param url Consume this Facebook page URL.
* @param winkVersion The winkVersion parameter
* @return FacebookEmbedNonAuthenticatedEntity
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec consumeFacebookPageUrlRequestCreation(String companyIdentifier, String url, String winkVersion) throws WebClientResponseException {
Object postBody = null;
// verify the required parameter 'companyIdentifier' is set
if (companyIdentifier == null) {
throw new WebClientResponseException("Missing the required parameter 'companyIdentifier' when calling consumeFacebookPageUrl", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// verify the required parameter 'url' is set
if (url == null) {
throw new WebClientResponseException("Missing the required parameter 'url' when calling consumeFacebookPageUrl", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
pathParams.put("companyIdentifier", companyIdentifier);
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "url", url));
if (winkVersion != null)
headerParams.add("Wink-Version", apiClient.parameterToString(winkVersion));
final String[] localVarAccepts = {
"application/json", "application/xml", "text/xml", "text/plain", "*/*"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { };
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "oauth2ClientCredentials" };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/api/sell/{companyIdentifier}/syndication/facebook/page", HttpMethod.GET, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Consume Facebook Page URL
* Utility method for reading oEmbed data for Facebook.
* 500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Consume URL for this owner identifier.
* @param url Consume this Facebook page URL.
* @param winkVersion The winkVersion parameter
* @return FacebookEmbedNonAuthenticatedEntity
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono consumeFacebookPageUrl(String companyIdentifier, String url, String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return consumeFacebookPageUrlRequestCreation(companyIdentifier, url, winkVersion).bodyToMono(localVarReturnType);
}
/**
* Consume Facebook Page URL
* Utility method for reading oEmbed data for Facebook.
* 500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Consume URL for this owner identifier.
* @param url Consume this Facebook page URL.
* @param winkVersion The winkVersion parameter
* @return ResponseEntity<FacebookEmbedNonAuthenticatedEntity>
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono> consumeFacebookPageUrlWithHttpInfo(String companyIdentifier, String url, String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return consumeFacebookPageUrlRequestCreation(companyIdentifier, url, winkVersion).toEntity(localVarReturnType);
}
/**
* Consume Facebook Page URL
* Utility method for reading oEmbed data for Facebook.
* 500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Consume URL for this owner identifier.
* @param url Consume this Facebook page URL.
* @param winkVersion The winkVersion parameter
* @return ResponseSpec
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public ResponseSpec consumeFacebookPageUrlWithResponseSpec(String companyIdentifier, String url, String winkVersion) throws WebClientResponseException {
return consumeFacebookPageUrlRequestCreation(companyIdentifier, url, winkVersion);
}
/**
* Consume Facebook Post URL
* Utility method for reading oEmbed data for Facebook.
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Consume URL for this owner identifier.
* @param url Consume this Facebook post URL.
* @param winkVersion The winkVersion parameter
* @return FacebookEmbedNonAuthenticatedEntity
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec consumeFacebookPostUrlRequestCreation(String companyIdentifier, String url, String winkVersion) throws WebClientResponseException {
Object postBody = null;
// verify the required parameter 'companyIdentifier' is set
if (companyIdentifier == null) {
throw new WebClientResponseException("Missing the required parameter 'companyIdentifier' when calling consumeFacebookPostUrl", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// verify the required parameter 'url' is set
if (url == null) {
throw new WebClientResponseException("Missing the required parameter 'url' when calling consumeFacebookPostUrl", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
pathParams.put("companyIdentifier", companyIdentifier);
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "url", url));
if (winkVersion != null)
headerParams.add("Wink-Version", apiClient.parameterToString(winkVersion));
final String[] localVarAccepts = {
"application/json", "application/xml", "text/xml", "text/plain", "*/*"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { };
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "oauth2ClientCredentials" };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/api/sell/{companyIdentifier}/syndication/facebook/post", HttpMethod.GET, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Consume Facebook Post URL
* Utility method for reading oEmbed data for Facebook.
* 500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Consume URL for this owner identifier.
* @param url Consume this Facebook post URL.
* @param winkVersion The winkVersion parameter
* @return FacebookEmbedNonAuthenticatedEntity
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono consumeFacebookPostUrl(String companyIdentifier, String url, String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return consumeFacebookPostUrlRequestCreation(companyIdentifier, url, winkVersion).bodyToMono(localVarReturnType);
}
/**
* Consume Facebook Post URL
* Utility method for reading oEmbed data for Facebook.
* 500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Consume URL for this owner identifier.
* @param url Consume this Facebook post URL.
* @param winkVersion The winkVersion parameter
* @return ResponseEntity<FacebookEmbedNonAuthenticatedEntity>
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono> consumeFacebookPostUrlWithHttpInfo(String companyIdentifier, String url, String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return consumeFacebookPostUrlRequestCreation(companyIdentifier, url, winkVersion).toEntity(localVarReturnType);
}
/**
* Consume Facebook Post URL
* Utility method for reading oEmbed data for Facebook.
* 500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Consume URL for this owner identifier.
* @param url Consume this Facebook post URL.
* @param winkVersion The winkVersion parameter
* @return ResponseSpec
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public ResponseSpec consumeFacebookPostUrlWithResponseSpec(String companyIdentifier, String url, String winkVersion) throws WebClientResponseException {
return consumeFacebookPostUrlRequestCreation(companyIdentifier, url, winkVersion);
}
/**
* Consume Facebook Video URL
* Utility method for reading oEmbed data for Facebook.
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Consume URL for this owner identifier.
* @param url Consume this Facebook video URL.
* @param winkVersion The winkVersion parameter
* @return FacebookEmbedNonAuthenticatedEntity
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec consumeFacebookVideoUrlRequestCreation(String companyIdentifier, String url, String winkVersion) throws WebClientResponseException {
Object postBody = null;
// verify the required parameter 'companyIdentifier' is set
if (companyIdentifier == null) {
throw new WebClientResponseException("Missing the required parameter 'companyIdentifier' when calling consumeFacebookVideoUrl", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// verify the required parameter 'url' is set
if (url == null) {
throw new WebClientResponseException("Missing the required parameter 'url' when calling consumeFacebookVideoUrl", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
pathParams.put("companyIdentifier", companyIdentifier);
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "url", url));
if (winkVersion != null)
headerParams.add("Wink-Version", apiClient.parameterToString(winkVersion));
final String[] localVarAccepts = {
"application/json", "application/xml", "text/xml", "text/plain", "*/*"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { };
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "oauth2ClientCredentials" };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/api/sell/{companyIdentifier}/syndication/facebook/video", HttpMethod.GET, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Consume Facebook Video URL
* Utility method for reading oEmbed data for Facebook.
* 500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Consume URL for this owner identifier.
* @param url Consume this Facebook video URL.
* @param winkVersion The winkVersion parameter
* @return FacebookEmbedNonAuthenticatedEntity
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono consumeFacebookVideoUrl(String companyIdentifier, String url, String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return consumeFacebookVideoUrlRequestCreation(companyIdentifier, url, winkVersion).bodyToMono(localVarReturnType);
}
/**
* Consume Facebook Video URL
* Utility method for reading oEmbed data for Facebook.
* 500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Consume URL for this owner identifier.
* @param url Consume this Facebook video URL.
* @param winkVersion The winkVersion parameter
* @return ResponseEntity<FacebookEmbedNonAuthenticatedEntity>
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono> consumeFacebookVideoUrlWithHttpInfo(String companyIdentifier, String url, String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return consumeFacebookVideoUrlRequestCreation(companyIdentifier, url, winkVersion).toEntity(localVarReturnType);
}
/**
* Consume Facebook Video URL
* Utility method for reading oEmbed data for Facebook.
* 500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Consume URL for this owner identifier.
* @param url Consume this Facebook video URL.
* @param winkVersion The winkVersion parameter
* @return ResponseSpec
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public ResponseSpec consumeFacebookVideoUrlWithResponseSpec(String companyIdentifier, String url, String winkVersion) throws WebClientResponseException {
return consumeFacebookVideoUrlRequestCreation(companyIdentifier, url, winkVersion);
}
/**
* Consume Instagram Post URL
* Utility method for reading oEmbed data for Instagram.
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Consume URL for this owner identifier.
* @param url Consume this Instagram post URL.
* @param winkVersion The winkVersion parameter
* @return InstagramEmbedNonAuthenticatedEntity
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec consumeInstagramPostUrlRequestCreation(String companyIdentifier, String url, String winkVersion) throws WebClientResponseException {
Object postBody = null;
// verify the required parameter 'companyIdentifier' is set
if (companyIdentifier == null) {
throw new WebClientResponseException("Missing the required parameter 'companyIdentifier' when calling consumeInstagramPostUrl", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// verify the required parameter 'url' is set
if (url == null) {
throw new WebClientResponseException("Missing the required parameter 'url' when calling consumeInstagramPostUrl", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
pathParams.put("companyIdentifier", companyIdentifier);
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "url", url));
if (winkVersion != null)
headerParams.add("Wink-Version", apiClient.parameterToString(winkVersion));
final String[] localVarAccepts = {
"application/json", "application/xml", "text/xml", "text/plain", "*/*"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { };
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "oauth2ClientCredentials" };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/api/sell/{companyIdentifier}/syndication/instagram/post", HttpMethod.GET, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Consume Instagram Post URL
* Utility method for reading oEmbed data for Instagram.
* 500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Consume URL for this owner identifier.
* @param url Consume this Instagram post URL.
* @param winkVersion The winkVersion parameter
* @return InstagramEmbedNonAuthenticatedEntity
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono consumeInstagramPostUrl(String companyIdentifier, String url, String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return consumeInstagramPostUrlRequestCreation(companyIdentifier, url, winkVersion).bodyToMono(localVarReturnType);
}
/**
* Consume Instagram Post URL
* Utility method for reading oEmbed data for Instagram.
* 500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Consume URL for this owner identifier.
* @param url Consume this Instagram post URL.
* @param winkVersion The winkVersion parameter
* @return ResponseEntity<InstagramEmbedNonAuthenticatedEntity>
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono> consumeInstagramPostUrlWithHttpInfo(String companyIdentifier, String url, String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return consumeInstagramPostUrlRequestCreation(companyIdentifier, url, winkVersion).toEntity(localVarReturnType);
}
/**
* Consume Instagram Post URL
* Utility method for reading oEmbed data for Instagram.
* 500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Consume URL for this owner identifier.
* @param url Consume this Instagram post URL.
* @param winkVersion The winkVersion parameter
* @return ResponseSpec
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public ResponseSpec consumeInstagramPostUrlWithResponseSpec(String companyIdentifier, String url, String winkVersion) throws WebClientResponseException {
return consumeInstagramPostUrlRequestCreation(companyIdentifier, url, winkVersion);
}
/**
* Consume Spotify URL
* Utility method for reading oEmbed data from Spotify.
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Consume URL for this owner identifier.
* @param url Consume this Spotify URL.
* @param winkVersion The winkVersion parameter
* @return SpotifyEmbedNonAuthenticatedEntity
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec consumeSpotifyUrlRequestCreation(String companyIdentifier, String url, String winkVersion) throws WebClientResponseException {
Object postBody = null;
// verify the required parameter 'companyIdentifier' is set
if (companyIdentifier == null) {
throw new WebClientResponseException("Missing the required parameter 'companyIdentifier' when calling consumeSpotifyUrl", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// verify the required parameter 'url' is set
if (url == null) {
throw new WebClientResponseException("Missing the required parameter 'url' when calling consumeSpotifyUrl", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
pathParams.put("companyIdentifier", companyIdentifier);
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "url", url));
if (winkVersion != null)
headerParams.add("Wink-Version", apiClient.parameterToString(winkVersion));
final String[] localVarAccepts = {
"application/json", "application/xml", "text/xml", "text/plain", "*/*"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { };
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "oauth2ClientCredentials" };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/api/sell/{companyIdentifier}/syndication/spotify", HttpMethod.GET, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Consume Spotify URL
* Utility method for reading oEmbed data from Spotify.
* 500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Consume URL for this owner identifier.
* @param url Consume this Spotify URL.
* @param winkVersion The winkVersion parameter
* @return SpotifyEmbedNonAuthenticatedEntity
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono consumeSpotifyUrl(String companyIdentifier, String url, String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return consumeSpotifyUrlRequestCreation(companyIdentifier, url, winkVersion).bodyToMono(localVarReturnType);
}
/**
* Consume Spotify URL
* Utility method for reading oEmbed data from Spotify.
* 500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Consume URL for this owner identifier.
* @param url Consume this Spotify URL.
* @param winkVersion The winkVersion parameter
* @return ResponseEntity<SpotifyEmbedNonAuthenticatedEntity>
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono> consumeSpotifyUrlWithHttpInfo(String companyIdentifier, String url, String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return consumeSpotifyUrlRequestCreation(companyIdentifier, url, winkVersion).toEntity(localVarReturnType);
}
/**
* Consume Spotify URL
* Utility method for reading oEmbed data from Spotify.
* 500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Consume URL for this owner identifier.
* @param url Consume this Spotify URL.
* @param winkVersion The winkVersion parameter
* @return ResponseSpec
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public ResponseSpec consumeSpotifyUrlWithResponseSpec(String companyIdentifier, String url, String winkVersion) throws WebClientResponseException {
return consumeSpotifyUrlRequestCreation(companyIdentifier, url, winkVersion);
}
/**
* Consume Tweet URL
* Utility method for reading oEmbed data for Twitter.
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Consume URL for this owner identifier.
* @param url Consume this Twitter URL.
* @param winkVersion The winkVersion parameter
* @return TwitterEmbedNonAuthenticatedEntity
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec consumeTwitterUrlRequestCreation(String companyIdentifier, String url, String winkVersion) throws WebClientResponseException {
Object postBody = null;
// verify the required parameter 'companyIdentifier' is set
if (companyIdentifier == null) {
throw new WebClientResponseException("Missing the required parameter 'companyIdentifier' when calling consumeTwitterUrl", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// verify the required parameter 'url' is set
if (url == null) {
throw new WebClientResponseException("Missing the required parameter 'url' when calling consumeTwitterUrl", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
pathParams.put("companyIdentifier", companyIdentifier);
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "url", url));
if (winkVersion != null)
headerParams.add("Wink-Version", apiClient.parameterToString(winkVersion));
final String[] localVarAccepts = {
"application/json", "application/xml", "text/xml", "text/plain", "*/*"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { };
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "oauth2ClientCredentials" };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/api/sell/{companyIdentifier}/syndication/tweet", HttpMethod.GET, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Consume Tweet URL
* Utility method for reading oEmbed data for Twitter.
* 500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Consume URL for this owner identifier.
* @param url Consume this Twitter URL.
* @param winkVersion The winkVersion parameter
* @return TwitterEmbedNonAuthenticatedEntity
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono consumeTwitterUrl(String companyIdentifier, String url, String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return consumeTwitterUrlRequestCreation(companyIdentifier, url, winkVersion).bodyToMono(localVarReturnType);
}
/**
* Consume Tweet URL
* Utility method for reading oEmbed data for Twitter.
* 500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Consume URL for this owner identifier.
* @param url Consume this Twitter URL.
* @param winkVersion The winkVersion parameter
* @return ResponseEntity<TwitterEmbedNonAuthenticatedEntity>
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono> consumeTwitterUrlWithHttpInfo(String companyIdentifier, String url, String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return consumeTwitterUrlRequestCreation(companyIdentifier, url, winkVersion).toEntity(localVarReturnType);
}
/**
* Consume Tweet URL
* Utility method for reading oEmbed data for Twitter.
* 500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Consume URL for this owner identifier.
* @param url Consume this Twitter URL.
* @param winkVersion The winkVersion parameter
* @return ResponseSpec
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public ResponseSpec consumeTwitterUrlWithResponseSpec(String companyIdentifier, String url, String winkVersion) throws WebClientResponseException {
return consumeTwitterUrlRequestCreation(companyIdentifier, url, winkVersion);
}
/**
* Redirect WinkLinks link
* Utility method to track external URLs and redirect to it.
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Redirect for this owner identifier.
* @param syndicationEntryIdentifier Redirect to URL for this syndication entry ID.
* @param userAgent User-Agent header.
* @param host Host header.
* @param referer Referrer header.
* @return RedirectViewNonAuthenticatedEntity
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec redirectUrlRequestCreation(String companyIdentifier, String syndicationEntryIdentifier, String userAgent, String host, String referer) throws WebClientResponseException {
Object postBody = null;
// verify the required parameter 'companyIdentifier' is set
if (companyIdentifier == null) {
throw new WebClientResponseException("Missing the required parameter 'companyIdentifier' when calling redirectUrl", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// verify the required parameter 'syndicationEntryIdentifier' is set
if (syndicationEntryIdentifier == null) {
throw new WebClientResponseException("Missing the required parameter 'syndicationEntryIdentifier' when calling redirectUrl", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// verify the required parameter 'userAgent' is set
if (userAgent == null) {
throw new WebClientResponseException("Missing the required parameter 'userAgent' when calling redirectUrl", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// verify the required parameter 'host' is set
if (host == null) {
throw new WebClientResponseException("Missing the required parameter 'host' when calling redirectUrl", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// verify the required parameter 'referer' is set
if (referer == null) {
throw new WebClientResponseException("Missing the required parameter 'referer' when calling redirectUrl", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
pathParams.put("companyIdentifier", companyIdentifier);
pathParams.put("syndicationEntryIdentifier", syndicationEntryIdentifier);
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (userAgent != null)
headerParams.add("User-Agent", apiClient.parameterToString(userAgent));
if (host != null)
headerParams.add("Host", apiClient.parameterToString(host));
if (referer != null)
headerParams.add("Referer", apiClient.parameterToString(referer));
final String[] localVarAccepts = {
"application/json", "application/xml", "text/xml", "text/plain", "*/*"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { };
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "oauth2ClientCredentials" };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/api/sell/{companyIdentifier}/syndication/{syndicationEntryIdentifier}/redirect", HttpMethod.GET, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Redirect WinkLinks link
* Utility method to track external URLs and redirect to it.
* 500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Redirect for this owner identifier.
* @param syndicationEntryIdentifier Redirect to URL for this syndication entry ID.
* @param userAgent User-Agent header.
* @param host Host header.
* @param referer Referrer header.
* @return RedirectViewNonAuthenticatedEntity
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono redirectUrl(String companyIdentifier, String syndicationEntryIdentifier, String userAgent, String host, String referer) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return redirectUrlRequestCreation(companyIdentifier, syndicationEntryIdentifier, userAgent, host, referer).bodyToMono(localVarReturnType);
}
/**
* Redirect WinkLinks link
* Utility method to track external URLs and redirect to it.
* 500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Redirect for this owner identifier.
* @param syndicationEntryIdentifier Redirect to URL for this syndication entry ID.
* @param userAgent User-Agent header.
* @param host Host header.
* @param referer Referrer header.
* @return ResponseEntity<RedirectViewNonAuthenticatedEntity>
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono> redirectUrlWithHttpInfo(String companyIdentifier, String syndicationEntryIdentifier, String userAgent, String host, String referer) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return redirectUrlRequestCreation(companyIdentifier, syndicationEntryIdentifier, userAgent, host, referer).toEntity(localVarReturnType);
}
/**
* Redirect WinkLinks link
* Utility method to track external URLs and redirect to it.
* 500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Redirect for this owner identifier.
* @param syndicationEntryIdentifier Redirect to URL for this syndication entry ID.
* @param userAgent User-Agent header.
* @param host Host header.
* @param referer Referrer header.
* @return ResponseSpec
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public ResponseSpec redirectUrlWithResponseSpec(String companyIdentifier, String syndicationEntryIdentifier, String userAgent, String host, String referer) throws WebClientResponseException {
return redirectUrlRequestCreation(companyIdentifier, syndicationEntryIdentifier, userAgent, host, referer);
}
/**
* Show Syndication Account
* Retrieve syndication account details.
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param urlName Account url name slug
* @param winkVersion The winkVersion parameter
* @param accept The accept parameter
* @return SyndicationAccountNonAuthenticatedEntity
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec showSyndicationAccountRequestCreation(String urlName, String winkVersion, String accept) throws WebClientResponseException {
Object postBody = null;
// verify the required parameter 'urlName' is set
if (urlName == null) {
throw new WebClientResponseException("Missing the required parameter 'urlName' when calling showSyndicationAccount", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
pathParams.put("urlName", urlName);
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (winkVersion != null)
headerParams.add("Wink-Version", apiClient.parameterToString(winkVersion));
if (accept != null)
headerParams.add("Accept", apiClient.parameterToString(accept));
final String[] localVarAccepts = {
"application/json", "application/xml", "text/xml", "text/plain", "*/*"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { };
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "oauth2ClientCredentials" };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/api/sell/syndication/{urlName}", HttpMethod.GET, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Show Syndication Account
* Retrieve syndication account details.
* 500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param urlName Account url name slug
* @param winkVersion The winkVersion parameter
* @param accept The accept parameter
* @return SyndicationAccountNonAuthenticatedEntity
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono showSyndicationAccount(String urlName, String winkVersion, String accept) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return showSyndicationAccountRequestCreation(urlName, winkVersion, accept).bodyToMono(localVarReturnType);
}
/**
* Show Syndication Account
* Retrieve syndication account details.
* 500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param urlName Account url name slug
* @param winkVersion The winkVersion parameter
* @param accept The accept parameter
* @return ResponseEntity<SyndicationAccountNonAuthenticatedEntity>
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono> showSyndicationAccountWithHttpInfo(String urlName, String winkVersion, String accept) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return showSyndicationAccountRequestCreation(urlName, winkVersion, accept).toEntity(localVarReturnType);
}
/**
* Show Syndication Account
* Retrieve syndication account details.
* 500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param urlName Account url name slug
* @param winkVersion The winkVersion parameter
* @param accept The accept parameter
* @return ResponseSpec
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public ResponseSpec showSyndicationAccountWithResponseSpec(String urlName, String winkVersion, String accept) throws WebClientResponseException {
return showSyndicationAccountRequestCreation(urlName, winkVersion, accept);
}
/**
* Show Syndication Categories
* Retrieve syndication category list.
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Account ID
* @param winkVersion The winkVersion parameter
* @param accept The accept parameter
* @return List<KeyValuePairNonAuthenticatedEntity>
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec showSyndicationCategoriesRequestCreation(String companyIdentifier, String winkVersion, String accept) throws WebClientResponseException {
Object postBody = null;
// verify the required parameter 'companyIdentifier' is set
if (companyIdentifier == null) {
throw new WebClientResponseException("Missing the required parameter 'companyIdentifier' when calling showSyndicationCategories", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
pathParams.put("companyIdentifier", companyIdentifier);
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (winkVersion != null)
headerParams.add("Wink-Version", apiClient.parameterToString(winkVersion));
if (accept != null)
headerParams.add("Accept", apiClient.parameterToString(accept));
final String[] localVarAccepts = {
"application/json", "application/xml", "text/xml", "text/plain", "*/*"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { };
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "oauth2ClientCredentials" };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/api/sell/{companyIdentifier}/syndication/category/list", HttpMethod.GET, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Show Syndication Categories
* Retrieve syndication category list.
* 500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Account ID
* @param winkVersion The winkVersion parameter
* @param accept The accept parameter
* @return List<KeyValuePairNonAuthenticatedEntity>
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Flux showSyndicationCategories(String companyIdentifier, String winkVersion, String accept) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return showSyndicationCategoriesRequestCreation(companyIdentifier, winkVersion, accept).bodyToFlux(localVarReturnType);
}
/**
* Show Syndication Categories
* Retrieve syndication category list.
* 500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Account ID
* @param winkVersion The winkVersion parameter
* @param accept The accept parameter
* @return ResponseEntity<List<KeyValuePairNonAuthenticatedEntity>>
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono>> showSyndicationCategoriesWithHttpInfo(String companyIdentifier, String winkVersion, String accept) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return showSyndicationCategoriesRequestCreation(companyIdentifier, winkVersion, accept).toEntityList(localVarReturnType);
}
/**
* Show Syndication Categories
* Retrieve syndication category list.
* 500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Account ID
* @param winkVersion The winkVersion parameter
* @param accept The accept parameter
* @return ResponseSpec
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public ResponseSpec showSyndicationCategoriesWithResponseSpec(String companyIdentifier, String winkVersion, String accept) throws WebClientResponseException {
return showSyndicationCategoriesRequestCreation(companyIdentifier, winkVersion, accept);
}
/**
* Show Syndication Entries
* Retrieve list of syndication entries.
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Account ID
* @param stateNonAuthenticatedEntity Body payload filtering and sorting preferences
* @param winkVersion The winkVersion parameter
* @return PageConsumableSyndicationEntryNonAuthenticatedEntity
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec showSyndicationEntryGridRequestCreation(String companyIdentifier, StateNonAuthenticatedEntity stateNonAuthenticatedEntity, String winkVersion) throws WebClientResponseException {
Object postBody = stateNonAuthenticatedEntity;
// verify the required parameter 'companyIdentifier' is set
if (companyIdentifier == null) {
throw new WebClientResponseException("Missing the required parameter 'companyIdentifier' when calling showSyndicationEntryGrid", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// verify the required parameter 'stateNonAuthenticatedEntity' is set
if (stateNonAuthenticatedEntity == null) {
throw new WebClientResponseException("Missing the required parameter 'stateNonAuthenticatedEntity' when calling showSyndicationEntryGrid", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
pathParams.put("companyIdentifier", companyIdentifier);
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (winkVersion != null)
headerParams.add("Wink-Version", apiClient.parameterToString(winkVersion));
final String[] localVarAccepts = {
"application/json", "application/xml", "text/xml", "text/plain", "*/*"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "oauth2ClientCredentials" };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/api/sell/{companyIdentifier}/syndication/grid", HttpMethod.POST, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Show Syndication Entries
* Retrieve list of syndication entries.
* 500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Account ID
* @param stateNonAuthenticatedEntity Body payload filtering and sorting preferences
* @param winkVersion The winkVersion parameter
* @return PageConsumableSyndicationEntryNonAuthenticatedEntity
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono showSyndicationEntryGrid(String companyIdentifier, StateNonAuthenticatedEntity stateNonAuthenticatedEntity, String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return showSyndicationEntryGridRequestCreation(companyIdentifier, stateNonAuthenticatedEntity, winkVersion).bodyToMono(localVarReturnType);
}
/**
* Show Syndication Entries
* Retrieve list of syndication entries.
* 500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Account ID
* @param stateNonAuthenticatedEntity Body payload filtering and sorting preferences
* @param winkVersion The winkVersion parameter
* @return ResponseEntity<PageConsumableSyndicationEntryNonAuthenticatedEntity>
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono> showSyndicationEntryGridWithHttpInfo(String companyIdentifier, StateNonAuthenticatedEntity stateNonAuthenticatedEntity, String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return showSyndicationEntryGridRequestCreation(companyIdentifier, stateNonAuthenticatedEntity, winkVersion).toEntity(localVarReturnType);
}
/**
* Show Syndication Entries
* Retrieve list of syndication entries.
* 500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Account ID
* @param stateNonAuthenticatedEntity Body payload filtering and sorting preferences
* @param winkVersion The winkVersion parameter
* @return ResponseSpec
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public ResponseSpec showSyndicationEntryGridWithResponseSpec(String companyIdentifier, StateNonAuthenticatedEntity stateNonAuthenticatedEntity, String winkVersion) throws WebClientResponseException {
return showSyndicationEntryGridRequestCreation(companyIdentifier, stateNonAuthenticatedEntity, winkVersion);
}
/**
* Track WinkLinks share
* Utility method to track sharing a url.
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Redirect for this owner identifier.
* @param syndicationEntryIdentifier Redirect to URL for this syndication entry ID.
* @param platform Platform user is sharing on.
* @param userAgent User-Agent header.
* @param host Host header.
* @param referer Referrer header.
* @param winkVersion The winkVersion parameter
* @return BooleanResponseNonAuthenticatedEntity
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec trackShareRequestCreation(String companyIdentifier, String syndicationEntryIdentifier, String platform, String userAgent, String host, String referer, String winkVersion) throws WebClientResponseException {
Object postBody = null;
// verify the required parameter 'companyIdentifier' is set
if (companyIdentifier == null) {
throw new WebClientResponseException("Missing the required parameter 'companyIdentifier' when calling trackShare", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// verify the required parameter 'syndicationEntryIdentifier' is set
if (syndicationEntryIdentifier == null) {
throw new WebClientResponseException("Missing the required parameter 'syndicationEntryIdentifier' when calling trackShare", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// verify the required parameter 'platform' is set
if (platform == null) {
throw new WebClientResponseException("Missing the required parameter 'platform' when calling trackShare", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// verify the required parameter 'userAgent' is set
if (userAgent == null) {
throw new WebClientResponseException("Missing the required parameter 'userAgent' when calling trackShare", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// verify the required parameter 'host' is set
if (host == null) {
throw new WebClientResponseException("Missing the required parameter 'host' when calling trackShare", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// verify the required parameter 'referer' is set
if (referer == null) {
throw new WebClientResponseException("Missing the required parameter 'referer' when calling trackShare", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
pathParams.put("companyIdentifier", companyIdentifier);
pathParams.put("syndicationEntryIdentifier", syndicationEntryIdentifier);
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "platform", platform));
if (userAgent != null)
headerParams.add("User-Agent", apiClient.parameterToString(userAgent));
if (host != null)
headerParams.add("Host", apiClient.parameterToString(host));
if (referer != null)
headerParams.add("Referer", apiClient.parameterToString(referer));
if (winkVersion != null)
headerParams.add("Wink-Version", apiClient.parameterToString(winkVersion));
final String[] localVarAccepts = {
"application/json", "application/xml", "text/xml", "text/plain", "*/*"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { };
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "oauth2ClientCredentials" };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/api/sell/{companyIdentifier}/syndication/{syndicationEntryIdentifier}/share", HttpMethod.GET, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Track WinkLinks share
* Utility method to track sharing a url.
* 500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Redirect for this owner identifier.
* @param syndicationEntryIdentifier Redirect to URL for this syndication entry ID.
* @param platform Platform user is sharing on.
* @param userAgent User-Agent header.
* @param host Host header.
* @param referer Referrer header.
* @param winkVersion The winkVersion parameter
* @return BooleanResponseNonAuthenticatedEntity
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono trackShare(String companyIdentifier, String syndicationEntryIdentifier, String platform, String userAgent, String host, String referer, String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return trackShareRequestCreation(companyIdentifier, syndicationEntryIdentifier, platform, userAgent, host, referer, winkVersion).bodyToMono(localVarReturnType);
}
/**
* Track WinkLinks share
* Utility method to track sharing a url.
* 500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Redirect for this owner identifier.
* @param syndicationEntryIdentifier Redirect to URL for this syndication entry ID.
* @param platform Platform user is sharing on.
* @param userAgent User-Agent header.
* @param host Host header.
* @param referer Referrer header.
* @param winkVersion The winkVersion parameter
* @return ResponseEntity<BooleanResponseNonAuthenticatedEntity>
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono> trackShareWithHttpInfo(String companyIdentifier, String syndicationEntryIdentifier, String platform, String userAgent, String host, String referer, String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return trackShareRequestCreation(companyIdentifier, syndicationEntryIdentifier, platform, userAgent, host, referer, winkVersion).toEntity(localVarReturnType);
}
/**
* Track WinkLinks share
* Utility method to track sharing a url.
* 500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
400 - Bad Request
*
200 - OK
* @param companyIdentifier Redirect for this owner identifier.
* @param syndicationEntryIdentifier Redirect to URL for this syndication entry ID.
* @param platform Platform user is sharing on.
* @param userAgent User-Agent header.
* @param host Host header.
* @param referer Referrer header.
* @param winkVersion The winkVersion parameter
* @return ResponseSpec
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public ResponseSpec trackShareWithResponseSpec(String companyIdentifier, String syndicationEntryIdentifier, String platform, String userAgent, String host, String referer, String winkVersion) throws WebClientResponseException {
return trackShareRequestCreation(companyIdentifier, syndicationEntryIdentifier, platform, userAgent, host, referer, winkVersion);
}
}