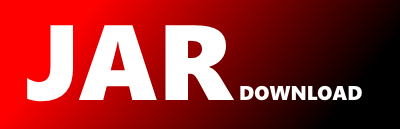
travel.wink.ws.allotz.OTAHotelAvailRS Maven / Gradle / Ivy
package travel.wink.ws.allotz;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.util.ArrayList;
import java.util.List;
import javax.xml.datatype.XMLGregorianCalendar;
import jakarta.xml.bind.annotation.XmlAccessType;
import jakarta.xml.bind.annotation.XmlAccessorType;
import jakarta.xml.bind.annotation.XmlAttribute;
import jakarta.xml.bind.annotation.XmlElement;
import jakarta.xml.bind.annotation.XmlRootElement;
import jakarta.xml.bind.annotation.XmlSchemaType;
import jakarta.xml.bind.annotation.XmlType;
import jakarta.xml.bind.annotation.adapters.CollapsedStringAdapter;
import jakarta.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
{@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"pos",
"success",
"warnings",
"profiles",
"hotelStays",
"roomStays",
"services",
"areas",
"criteria",
"currencyConversions",
"rebatePrograms",
"tpaExtensions",
"errors"
})
@XmlRootElement(name = "OTA_HotelAvailRS")
public class OTAHotelAvailRS {
@XmlElement(name = "POS")
protected POSType pos;
@XmlElement(name = "Success")
protected SuccessType success;
@XmlElement(name = "Warnings")
protected WarningsType warnings;
@XmlElement(name = "Profiles")
protected ProfilesType profiles;
@XmlElement(name = "HotelStays")
protected OTAHotelAvailRS.HotelStays hotelStays;
@XmlElement(name = "RoomStays")
protected OTAHotelAvailRS.RoomStays roomStays;
@XmlElement(name = "Services")
protected ServicesType services;
@XmlElement(name = "Areas")
protected AreasType areas;
@XmlElement(name = "Criteria")
protected OTAHotelAvailRS.Criteria criteria;
@XmlElement(name = "CurrencyConversions")
protected OTAHotelAvailRS.CurrencyConversions currencyConversions;
@XmlElement(name = "RebatePrograms")
protected OTAHotelAvailRS.RebatePrograms rebatePrograms;
@XmlElement(name = "TPA_Extensions")
protected TPAExtensionsType tpaExtensions;
@XmlElement(name = "Errors")
protected ErrorsType errors;
@XmlAttribute(name = "SearchCacheLevel")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
protected String searchCacheLevel;
@XmlAttribute(name = "EchoToken")
protected String echoToken;
@XmlAttribute(name = "TimeStamp")
@XmlSchemaType(name = "dateTime")
protected XMLGregorianCalendar timeStamp;
@XmlAttribute(name = "Target")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
protected String target;
@XmlAttribute(name = "TargetName")
protected String targetName;
@XmlAttribute(name = "Version", required = true)
protected BigDecimal version;
@XmlAttribute(name = "TransactionIdentifier")
protected String transactionIdentifier;
@XmlAttribute(name = "SequenceNmbr")
@XmlSchemaType(name = "nonNegativeInteger")
protected BigInteger sequenceNmbr;
@XmlAttribute(name = "TransactionStatusCode")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
protected String transactionStatusCode;
@XmlAttribute(name = "RetransmissionIndicator")
protected Boolean retransmissionIndicator;
@XmlAttribute(name = "CorrelationID")
protected String correlationID;
@XmlAttribute(name = "AltLangID")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "language")
protected String altLangID;
@XmlAttribute(name = "PrimaryLangID")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "language")
protected String primaryLangID;
/**
* Gets the value of the pos property.
*
* @return
* possible object is
* {@link POSType }
*
*/
public POSType getPOS() {
return pos;
}
/**
* Sets the value of the pos property.
*
* @param value
* allowed object is
* {@link POSType }
*
*/
public void setPOS(POSType value) {
this.pos = value;
}
/**
* Gets the value of the success property.
*
* @return
* possible object is
* {@link SuccessType }
*
*/
public SuccessType getSuccess() {
return success;
}
/**
* Sets the value of the success property.
*
* @param value
* allowed object is
* {@link SuccessType }
*
*/
public void setSuccess(SuccessType value) {
this.success = value;
}
/**
* Gets the value of the warnings property.
*
* @return
* possible object is
* {@link WarningsType }
*
*/
public WarningsType getWarnings() {
return warnings;
}
/**
* Sets the value of the warnings property.
*
* @param value
* allowed object is
* {@link WarningsType }
*
*/
public void setWarnings(WarningsType value) {
this.warnings = value;
}
/**
* Gets the value of the profiles property.
*
* @return
* possible object is
* {@link ProfilesType }
*
*/
public ProfilesType getProfiles() {
return profiles;
}
/**
* Sets the value of the profiles property.
*
* @param value
* allowed object is
* {@link ProfilesType }
*
*/
public void setProfiles(ProfilesType value) {
this.profiles = value;
}
/**
* Gets the value of the hotelStays property.
*
* @return
* possible object is
* {@link OTAHotelAvailRS.HotelStays }
*
*/
public OTAHotelAvailRS.HotelStays getHotelStays() {
return hotelStays;
}
/**
* Sets the value of the hotelStays property.
*
* @param value
* allowed object is
* {@link OTAHotelAvailRS.HotelStays }
*
*/
public void setHotelStays(OTAHotelAvailRS.HotelStays value) {
this.hotelStays = value;
}
/**
* Gets the value of the roomStays property.
*
* @return
* possible object is
* {@link OTAHotelAvailRS.RoomStays }
*
*/
public OTAHotelAvailRS.RoomStays getRoomStays() {
return roomStays;
}
/**
* Sets the value of the roomStays property.
*
* @param value
* allowed object is
* {@link OTAHotelAvailRS.RoomStays }
*
*/
public void setRoomStays(OTAHotelAvailRS.RoomStays value) {
this.roomStays = value;
}
/**
* Gets the value of the services property.
*
* @return
* possible object is
* {@link ServicesType }
*
*/
public ServicesType getServices() {
return services;
}
/**
* Sets the value of the services property.
*
* @param value
* allowed object is
* {@link ServicesType }
*
*/
public void setServices(ServicesType value) {
this.services = value;
}
/**
* Gets the value of the areas property.
*
* @return
* possible object is
* {@link AreasType }
*
*/
public AreasType getAreas() {
return areas;
}
/**
* Sets the value of the areas property.
*
* @param value
* allowed object is
* {@link AreasType }
*
*/
public void setAreas(AreasType value) {
this.areas = value;
}
/**
* Gets the value of the criteria property.
*
* @return
* possible object is
* {@link OTAHotelAvailRS.Criteria }
*
*/
public OTAHotelAvailRS.Criteria getCriteria() {
return criteria;
}
/**
* Sets the value of the criteria property.
*
* @param value
* allowed object is
* {@link OTAHotelAvailRS.Criteria }
*
*/
public void setCriteria(OTAHotelAvailRS.Criteria value) {
this.criteria = value;
}
/**
* Gets the value of the currencyConversions property.
*
* @return
* possible object is
* {@link OTAHotelAvailRS.CurrencyConversions }
*
*/
public OTAHotelAvailRS.CurrencyConversions getCurrencyConversions() {
return currencyConversions;
}
/**
* Sets the value of the currencyConversions property.
*
* @param value
* allowed object is
* {@link OTAHotelAvailRS.CurrencyConversions }
*
*/
public void setCurrencyConversions(OTAHotelAvailRS.CurrencyConversions value) {
this.currencyConversions = value;
}
/**
* Gets the value of the rebatePrograms property.
*
* @return
* possible object is
* {@link OTAHotelAvailRS.RebatePrograms }
*
*/
public OTAHotelAvailRS.RebatePrograms getRebatePrograms() {
return rebatePrograms;
}
/**
* Sets the value of the rebatePrograms property.
*
* @param value
* allowed object is
* {@link OTAHotelAvailRS.RebatePrograms }
*
*/
public void setRebatePrograms(OTAHotelAvailRS.RebatePrograms value) {
this.rebatePrograms = value;
}
/**
* Gets the value of the tpaExtensions property.
*
* @return
* possible object is
* {@link TPAExtensionsType }
*
*/
public TPAExtensionsType getTPAExtensions() {
return tpaExtensions;
}
/**
* Sets the value of the tpaExtensions property.
*
* @param value
* allowed object is
* {@link TPAExtensionsType }
*
*/
public void setTPAExtensions(TPAExtensionsType value) {
this.tpaExtensions = value;
}
/**
* Gets the value of the errors property.
*
* @return
* possible object is
* {@link ErrorsType }
*
*/
public ErrorsType getErrors() {
return errors;
}
/**
* Sets the value of the errors property.
*
* @param value
* allowed object is
* {@link ErrorsType }
*
*/
public void setErrors(ErrorsType value) {
this.errors = value;
}
/**
* Gets the value of the searchCacheLevel property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSearchCacheLevel() {
return searchCacheLevel;
}
/**
* Sets the value of the searchCacheLevel property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSearchCacheLevel(String value) {
this.searchCacheLevel = value;
}
/**
* Gets the value of the echoToken property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getEchoToken() {
return echoToken;
}
/**
* Sets the value of the echoToken property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setEchoToken(String value) {
this.echoToken = value;
}
/**
* Gets the value of the timeStamp property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getTimeStamp() {
return timeStamp;
}
/**
* Sets the value of the timeStamp property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setTimeStamp(XMLGregorianCalendar value) {
this.timeStamp = value;
}
/**
* Gets the value of the target property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTarget() {
return target;
}
/**
* Sets the value of the target property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTarget(String value) {
this.target = value;
}
/**
* Gets the value of the targetName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTargetName() {
return targetName;
}
/**
* Sets the value of the targetName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTargetName(String value) {
this.targetName = value;
}
/**
* Gets the value of the version property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getVersion() {
return version;
}
/**
* Sets the value of the version property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setVersion(BigDecimal value) {
this.version = value;
}
/**
* Gets the value of the transactionIdentifier property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTransactionIdentifier() {
return transactionIdentifier;
}
/**
* Sets the value of the transactionIdentifier property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTransactionIdentifier(String value) {
this.transactionIdentifier = value;
}
/**
* Gets the value of the sequenceNmbr property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getSequenceNmbr() {
return sequenceNmbr;
}
/**
* Sets the value of the sequenceNmbr property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setSequenceNmbr(BigInteger value) {
this.sequenceNmbr = value;
}
/**
* Gets the value of the transactionStatusCode property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTransactionStatusCode() {
return transactionStatusCode;
}
/**
* Sets the value of the transactionStatusCode property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTransactionStatusCode(String value) {
this.transactionStatusCode = value;
}
/**
* Gets the value of the retransmissionIndicator property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isRetransmissionIndicator() {
return retransmissionIndicator;
}
/**
* Sets the value of the retransmissionIndicator property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setRetransmissionIndicator(Boolean value) {
this.retransmissionIndicator = value;
}
/**
* Gets the value of the correlationID property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCorrelationID() {
return correlationID;
}
/**
* Sets the value of the correlationID property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCorrelationID(String value) {
this.correlationID = value;
}
/**
* Gets the value of the altLangID property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAltLangID() {
return altLangID;
}
/**
* Sets the value of the altLangID property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAltLangID(String value) {
this.altLangID = value;
}
/**
* Gets the value of the primaryLangID property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPrimaryLangID() {
return primaryLangID;
}
/**
* Sets the value of the primaryLangID property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPrimaryLangID(String value) {
this.primaryLangID = value;
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
{@code
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"criterion"
})
public static class Criteria {
@XmlElement(name = "Criterion", required = true)
protected List criterion;
/**
* Gets the value of the criterion property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the criterion property.
*
*
* For example, to add a new item, do as follows:
*
* getCriterion().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link HotelSearchCriterionType }
*
*
* @return
* The value of the criterion property.
*/
public List getCriterion() {
if (criterion == null) {
criterion = new ArrayList<>();
}
return this.criterion;
}
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
{@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"currencyConversion"
})
public static class CurrencyConversions {
@XmlElement(name = "CurrencyConversion", required = true)
protected List currencyConversion;
/**
* Gets the value of the currencyConversion property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the currencyConversion property.
*
*
* For example, to add a new item, do as follows:
*
* getCurrencyConversion().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link OTAHotelAvailRS.CurrencyConversions.CurrencyConversion }
*
*
* @return
* The value of the currencyConversion property.
*/
public List getCurrencyConversion() {
if (currencyConversion == null) {
currencyConversion = new ArrayList<>();
}
return this.currencyConversion;
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
{@code
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "")
public static class CurrencyConversion {
@XmlAttribute(name = "RateConversion")
protected BigDecimal rateConversion;
@XmlAttribute(name = "SourceCurrencyCode")
protected String sourceCurrencyCode;
@XmlAttribute(name = "RequestedCurrencyCode")
protected String requestedCurrencyCode;
@XmlAttribute(name = "DecimalPlaces")
@XmlSchemaType(name = "nonNegativeInteger")
protected BigInteger decimalPlaces;
@XmlAttribute(name = "Source")
protected String source;
/**
* Gets the value of the rateConversion property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getRateConversion() {
return rateConversion;
}
/**
* Sets the value of the rateConversion property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setRateConversion(BigDecimal value) {
this.rateConversion = value;
}
/**
* Gets the value of the sourceCurrencyCode property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSourceCurrencyCode() {
return sourceCurrencyCode;
}
/**
* Sets the value of the sourceCurrencyCode property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSourceCurrencyCode(String value) {
this.sourceCurrencyCode = value;
}
/**
* Gets the value of the requestedCurrencyCode property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getRequestedCurrencyCode() {
return requestedCurrencyCode;
}
/**
* Sets the value of the requestedCurrencyCode property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setRequestedCurrencyCode(String value) {
this.requestedCurrencyCode = value;
}
/**
* Gets the value of the decimalPlaces property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getDecimalPlaces() {
return decimalPlaces;
}
/**
* Sets the value of the decimalPlaces property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setDecimalPlaces(BigInteger value) {
this.decimalPlaces = value;
}
/**
* Gets the value of the source property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSource() {
return source;
}
/**
* Sets the value of the source property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSource(String value) {
this.source = value;
}
}
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
{@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"hotelStay"
})
public static class HotelStays {
@XmlElement(name = "HotelStay", required = true)
protected List hotelStay;
/**
* Gets the value of the hotelStay property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the hotelStay property.
*
*
* For example, to add a new item, do as follows:
*
* getHotelStay().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link OTAHotelAvailRS.HotelStays.HotelStay }
*
*
* @return
* The value of the hotelStay property.
*/
public List getHotelStay() {
if (hotelStay == null) {
hotelStay = new ArrayList<>();
}
return this.hotelStay;
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
{@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"availability",
"basicPropertyInfo",
"price"
})
public static class HotelStay {
@XmlElement(name = "Availability")
protected List availability;
@XmlElement(name = "BasicPropertyInfo")
protected BasicPropertyInfoType basicPropertyInfo;
@XmlElement(name = "Price")
protected List price;
@XmlAttribute(name = "RoomStayRPH")
protected List roomStayRPH;
/**
* Gets the value of the availability property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the availability property.
*
*
* For example, to add a new item, do as follows:
*
* getAvailability().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link OTAHotelAvailRS.HotelStays.HotelStay.Availability }
*
*
* @return
* The value of the availability property.
*/
public List getAvailability() {
if (availability == null) {
availability = new ArrayList<>();
}
return this.availability;
}
/**
* Gets the value of the basicPropertyInfo property.
*
* @return
* possible object is
* {@link BasicPropertyInfoType }
*
*/
public BasicPropertyInfoType getBasicPropertyInfo() {
return basicPropertyInfo;
}
/**
* Sets the value of the basicPropertyInfo property.
*
* @param value
* allowed object is
* {@link BasicPropertyInfoType }
*
*/
public void setBasicPropertyInfo(BasicPropertyInfoType value) {
this.basicPropertyInfo = value;
}
/**
* Gets the value of the price property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the price property.
*
*
* For example, to add a new item, do as follows:
*
* getPrice().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link OTAHotelAvailRS.HotelStays.HotelStay.Price }
*
*
* @return
* The value of the price property.
*/
public List getPrice() {
if (price == null) {
price = new ArrayList<>();
}
return this.price;
}
/**
* Gets the value of the roomStayRPH property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the roomStayRPH property.
*
*
* For example, to add a new item, do as follows:
*
* getRoomStayRPH().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
* @return
* The value of the roomStayRPH property.
*/
public List getRoomStayRPH() {
if (roomStayRPH == null) {
roomStayRPH = new ArrayList<>();
}
return this.roomStayRPH;
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
{@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"restriction"
})
public static class Availability {
@XmlElement(name = "Restriction")
protected List restriction;
@XmlAttribute(name = "Status", required = true)
protected List status;
@XmlAttribute(name = "Start")
protected String start;
@XmlAttribute(name = "Duration")
protected String duration;
@XmlAttribute(name = "End")
protected String end;
/**
* Gets the value of the restriction property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the restriction property.
*
*
* For example, to add a new item, do as follows:
*
* getRestriction().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link OTAHotelAvailRS.HotelStays.HotelStay.Availability.Restriction }
*
*
* @return
* The value of the restriction property.
*/
public List getRestriction() {
if (restriction == null) {
restriction = new ArrayList<>();
}
return this.restriction;
}
/**
* Gets the value of the status property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the status property.
*
*
* For example, to add a new item, do as follows:
*
* getStatus().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
* @return
* The value of the status property.
*/
public List getStatus() {
if (status == null) {
status = new ArrayList<>();
}
return this.status;
}
/**
* Gets the value of the start property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getStart() {
return start;
}
/**
* Sets the value of the start property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setStart(String value) {
this.start = value;
}
/**
* Gets the value of the duration property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDuration() {
return duration;
}
/**
* Sets the value of the duration property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDuration(String value) {
this.duration = value;
}
/**
* Gets the value of the end property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getEnd() {
return end;
}
/**
* Sets the value of the end property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setEnd(String value) {
this.end = value;
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
{@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "")
public static class Restriction {
@XmlAttribute(name = "RestrictionType")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
protected String restrictionType;
@XmlAttribute(name = "Time")
protected BigInteger time;
@XmlAttribute(name = "TimeUnit")
protected TimeUnitType timeUnit;
/**
* Gets the value of the restrictionType property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getRestrictionType() {
return restrictionType;
}
/**
* Sets the value of the restrictionType property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setRestrictionType(String value) {
this.restrictionType = value;
}
/**
* Gets the value of the time property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getTime() {
return time;
}
/**
* Sets the value of the time property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setTime(BigInteger value) {
this.time = value;
}
/**
* Gets the value of the timeUnit property.
*
* @return
* possible object is
* {@link TimeUnitType }
*
*/
public TimeUnitType getTimeUnit() {
return timeUnit;
}
/**
* Sets the value of the timeUnit property.
*
* @param value
* allowed object is
* {@link TimeUnitType }
*
*/
public void setTimeUnit(TimeUnitType value) {
this.timeUnit = value;
}
}
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
{@code
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "")
public static class Price {
@XmlAttribute(name = "AmountBeforeTax")
protected BigDecimal amountBeforeTax;
@XmlAttribute(name = "AmountAfterTax")
protected BigDecimal amountAfterTax;
@XmlAttribute(name = "CurrencyCode")
protected String currencyCode;
@XmlAttribute(name = "Decimal")
@XmlSchemaType(name = "nonNegativeInteger")
protected BigInteger decimal;
@XmlAttribute(name = "Start")
protected String start;
@XmlAttribute(name = "Duration")
protected String duration;
@XmlAttribute(name = "End")
protected String end;
/**
* Gets the value of the amountBeforeTax property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getAmountBeforeTax() {
return amountBeforeTax;
}
/**
* Sets the value of the amountBeforeTax property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setAmountBeforeTax(BigDecimal value) {
this.amountBeforeTax = value;
}
/**
* Gets the value of the amountAfterTax property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getAmountAfterTax() {
return amountAfterTax;
}
/**
* Sets the value of the amountAfterTax property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setAmountAfterTax(BigDecimal value) {
this.amountAfterTax = value;
}
/**
* Gets the value of the currencyCode property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCurrencyCode() {
return currencyCode;
}
/**
* Sets the value of the currencyCode property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCurrencyCode(String value) {
this.currencyCode = value;
}
/**
* Gets the value of the decimal property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getDecimal() {
return decimal;
}
/**
* Sets the value of the decimal property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setDecimal(BigInteger value) {
this.decimal = value;
}
/**
* Gets the value of the start property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getStart() {
return start;
}
/**
* Sets the value of the start property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setStart(String value) {
this.start = value;
}
/**
* Gets the value of the duration property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDuration() {
return duration;
}
/**
* Sets the value of the duration property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDuration(String value) {
this.duration = value;
}
/**
* Gets the value of the end property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getEnd() {
return end;
}
/**
* Sets the value of the end property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setEnd(String value) {
this.end = value;
}
}
}
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
{@code
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"rebateProgram"
})
public static class RebatePrograms {
@XmlElement(name = "RebateProgram", required = true)
protected List rebateProgram;
/**
* Gets the value of the rebateProgram property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the rebateProgram property.
*
*
* For example, to add a new item, do as follows:
*
* getRebateProgram().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link RebateType }
*
*
* @return
* The value of the rebateProgram property.
*/
public List getRebateProgram() {
if (rebateProgram == null) {
rebateProgram = new ArrayList<>();
}
return this.rebateProgram;
}
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
{@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"roomStay"
})
public static class RoomStays {
@XmlElement(name = "RoomStay", required = true)
protected List roomStay;
@XmlAttribute(name = "MoreIndicator")
protected String moreIndicator;
@XmlAttribute(name = "SortOrder")
@XmlSchemaType(name = "positiveInteger")
protected BigInteger sortOrder;
/**
* Gets the value of the roomStay property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the Jakarta XML Binding object.
* This is why there is not a {@code set} method for the roomStay property.
*
*
* For example, to add a new item, do as follows:
*
* getRoomStay().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link OTAHotelAvailRS.RoomStays.RoomStay }
*
*
* @return
* The value of the roomStay property.
*/
public List getRoomStay() {
if (roomStay == null) {
roomStay = new ArrayList<>();
}
return this.roomStay;
}
/**
* Gets the value of the moreIndicator property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMoreIndicator() {
return moreIndicator;
}
/**
* Sets the value of the moreIndicator property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMoreIndicator(String value) {
this.moreIndicator = value;
}
/**
* Gets the value of the sortOrder property.
*
* @return
* possible object is
* {@link BigInteger }
*
*/
public BigInteger getSortOrder() {
return sortOrder;
}
/**
* Sets the value of the sortOrder property.
*
* @param value
* allowed object is
* {@link BigInteger }
*
*/
public void setSortOrder(BigInteger value) {
this.sortOrder = value;
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
{@code
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"reference",
"serviceRPHs"
})
public static class RoomStay
extends RoomStayType
{
@XmlElement(name = "Reference")
protected OTAHotelAvailRS.RoomStays.RoomStay.Reference reference;
@XmlElement(name = "ServiceRPHs")
protected ServiceRPHsType serviceRPHs;
@XmlAttribute(name = "IsAlternate")
protected Boolean isAlternate;
@XmlAttribute(name = "AvailabilityStatus")
protected RateIndicatorType availabilityStatus;
@XmlAttribute(name = "RoomStayCandidateRPH")
protected String roomStayCandidateRPH;
@XmlAttribute(name = "MoreDataEchoToken")
protected String moreDataEchoToken;
@XmlAttribute(name = "InfoSource")
protected String infoSource;
@XmlAttribute(name = "RPH")
protected String rph;
@XmlAttribute(name = "AvailableIndicator")
protected Boolean availableIndicator;
@XmlAttribute(name = "ResponseType")
protected String responseType;
/**
* Gets the value of the reference property.
*
* @return
* possible object is
* {@link OTAHotelAvailRS.RoomStays.RoomStay.Reference }
*
*/
public OTAHotelAvailRS.RoomStays.RoomStay.Reference getReference() {
return reference;
}
/**
* Sets the value of the reference property.
*
* @param value
* allowed object is
* {@link OTAHotelAvailRS.RoomStays.RoomStay.Reference }
*
*/
public void setReference(OTAHotelAvailRS.RoomStays.RoomStay.Reference value) {
this.reference = value;
}
/**
* Gets the value of the serviceRPHs property.
*
* @return
* possible object is
* {@link ServiceRPHsType }
*
*/
public ServiceRPHsType getServiceRPHs() {
return serviceRPHs;
}
/**
* Sets the value of the serviceRPHs property.
*
* @param value
* allowed object is
* {@link ServiceRPHsType }
*
*/
public void setServiceRPHs(ServiceRPHsType value) {
this.serviceRPHs = value;
}
/**
* Gets the value of the isAlternate property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isIsAlternate() {
return isAlternate;
}
/**
* Sets the value of the isAlternate property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setIsAlternate(Boolean value) {
this.isAlternate = value;
}
/**
* Gets the value of the availabilityStatus property.
*
* @return
* possible object is
* {@link RateIndicatorType }
*
*/
public RateIndicatorType getAvailabilityStatus() {
return availabilityStatus;
}
/**
* Sets the value of the availabilityStatus property.
*
* @param value
* allowed object is
* {@link RateIndicatorType }
*
*/
public void setAvailabilityStatus(RateIndicatorType value) {
this.availabilityStatus = value;
}
/**
* Gets the value of the roomStayCandidateRPH property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getRoomStayCandidateRPH() {
return roomStayCandidateRPH;
}
/**
* Sets the value of the roomStayCandidateRPH property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setRoomStayCandidateRPH(String value) {
this.roomStayCandidateRPH = value;
}
/**
* Gets the value of the moreDataEchoToken property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMoreDataEchoToken() {
return moreDataEchoToken;
}
/**
* Sets the value of the moreDataEchoToken property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMoreDataEchoToken(String value) {
this.moreDataEchoToken = value;
}
/**
* Gets the value of the infoSource property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getInfoSource() {
return infoSource;
}
/**
* Sets the value of the infoSource property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setInfoSource(String value) {
this.infoSource = value;
}
/**
* Gets the value of the rph property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getRPH() {
return rph;
}
/**
* Sets the value of the rph property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setRPH(String value) {
this.rph = value;
}
/**
* Gets the value of the availableIndicator property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isAvailableIndicator() {
return availableIndicator;
}
/**
* Sets the value of the availableIndicator property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setAvailableIndicator(Boolean value) {
this.availableIndicator = value;
}
/**
* Gets the value of the responseType property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getResponseType() {
return responseType;
}
/**
* Sets the value of the responseType property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setResponseType(String value) {
this.responseType = value;
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
{@code
*
*
*
*
*
*
*
* }
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "")
public static class Reference
extends UniqueIDType
{
@XmlAttribute(name = "DateTime")
@XmlSchemaType(name = "dateTime")
protected XMLGregorianCalendar dateTime;
/**
* Gets the value of the dateTime property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getDateTime() {
return dateTime;
}
/**
* Sets the value of the dateTime property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setDateTime(XMLGregorianCalendar value) {
this.dateTime = value;
}
}
}
}
}