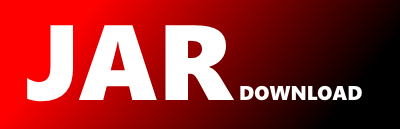
travel.wink.ws.allotz.ObjectFactory Maven / Gradle / Ivy
package travel.wink.ws.allotz;
import javax.xml.namespace.QName;
import jakarta.xml.bind.JAXBElement;
import jakarta.xml.bind.annotation.XmlElementDecl;
import jakarta.xml.bind.annotation.XmlRegistry;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the travel.wink.ws.allotz package.
* An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
*
*/
@XmlRegistry
public class ObjectFactory {
private static final QName _TPAExtensions_QNAME = new QName("http://www.opentravel.org/OTA/2003/05", "TPA_Extensions");
private static final QName _VehReservation_QNAME = new QName("http://www.opentravel.org/OTA/2003/05", "VehReservation");
private static final QName _OTAHotelAvailNotifRS_QNAME = new QName("http://www.opentravel.org/OTA/2003/05", "OTA_HotelAvailNotifRS");
private static final QName _OTAHotelRateAmountNotifRS_QNAME = new QName("http://www.opentravel.org/OTA/2003/05", "OTA_HotelRateAmountNotifRS");
private static final QName _UsernameToken_QNAME = new QName("http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-secext-1.0.xsd", "UsernameToken");
private static final QName _BinarySecurityToken_QNAME = new QName("http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-secext-1.0.xsd", "BinarySecurityToken");
private static final QName _Reference_QNAME = new QName("http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-secext-1.0.xsd", "Reference");
private static final QName _Embedded_QNAME = new QName("http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-secext-1.0.xsd", "Embedded");
private static final QName _KeyIdentifier_QNAME = new QName("http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-secext-1.0.xsd", "KeyIdentifier");
private static final QName _SecurityTokenReference_QNAME = new QName("http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-secext-1.0.xsd", "SecurityTokenReference");
private static final QName _Security_QNAME = new QName("http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-secext-1.0.xsd", "Security");
private static final QName _TransformationParameters_QNAME = new QName("http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-secext-1.0.xsd", "TransformationParameters");
private static final QName _Password_QNAME = new QName("http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-secext-1.0.xsd", "Password");
private static final QName _Nonce_QNAME = new QName("http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-secext-1.0.xsd", "Nonce");
private static final QName _Timestamp_QNAME = new QName("http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-utility-1.0.xsd", "Timestamp");
private static final QName _Expires_QNAME = new QName("http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-utility-1.0.xsd", "Expires");
private static final QName _Created_QNAME = new QName("http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-utility-1.0.xsd", "Created");
private static final QName _ParagraphTypeText_QNAME = new QName("http://www.opentravel.org/OTA/2003/05", "Text");
private static final QName _ParagraphTypeImage_QNAME = new QName("http://www.opentravel.org/OTA/2003/05", "Image");
private static final QName _ParagraphTypeURL_QNAME = new QName("http://www.opentravel.org/OTA/2003/05", "URL");
private static final QName _ParagraphTypeListItem_QNAME = new QName("http://www.opentravel.org/OTA/2003/05", "ListItem");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: travel.wink.ws.allotz
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link OTAHotelAvailRQ }
*
* @return
* the new instance of {@link OTAHotelAvailRQ }
*/
public OTAHotelAvailRQ createOTAHotelAvailRQ() {
return new OTAHotelAvailRQ();
}
/**
* Create an instance of {@link OTAHotelAvailRS }
*
* @return
* the new instance of {@link OTAHotelAvailRS }
*/
public OTAHotelAvailRS createOTAHotelAvailRS() {
return new OTAHotelAvailRS();
}
/**
* Create an instance of {@link OTAHotelAvailNotifRQ }
*
* @return
* the new instance of {@link OTAHotelAvailNotifRQ }
*/
public OTAHotelAvailNotifRQ createOTAHotelAvailNotifRQ() {
return new OTAHotelAvailNotifRQ();
}
/**
* Create an instance of {@link OTAHotelRateAmountNotifRQ }
*
* @return
* the new instance of {@link OTAHotelRateAmountNotifRQ }
*/
public OTAHotelRateAmountNotifRQ createOTAHotelRateAmountNotifRQ() {
return new OTAHotelRateAmountNotifRQ();
}
/**
* Create an instance of {@link OTAHotelAvailGetRQ }
*
* @return
* the new instance of {@link OTAHotelAvailGetRQ }
*/
public OTAHotelAvailGetRQ createOTAHotelAvailGetRQ() {
return new OTAHotelAvailGetRQ();
}
/**
* Create an instance of {@link OTAHotelAvailGetRS }
*
* @return
* the new instance of {@link OTAHotelAvailGetRS }
*/
public OTAHotelAvailGetRS createOTAHotelAvailGetRS() {
return new OTAHotelAvailGetRS();
}
/**
* Create an instance of {@link OTAHotelRatePlanRQ }
*
* @return
* the new instance of {@link OTAHotelRatePlanRQ }
*/
public OTAHotelRatePlanRQ createOTAHotelRatePlanRQ() {
return new OTAHotelRatePlanRQ();
}
/**
* Create an instance of {@link OTAHotelRatePlanRS }
*
* @return
* the new instance of {@link OTAHotelRatePlanRS }
*/
public OTAHotelRatePlanRS createOTAHotelRatePlanRS() {
return new OTAHotelRatePlanRS();
}
/**
* Create an instance of {@link OfferType }
*
* @return
* the new instance of {@link OfferType }
*/
public OfferType createOfferType() {
return new OfferType();
}
/**
* Create an instance of {@link OfferType.Guests }
*
* @return
* the new instance of {@link OfferType.Guests }
*/
public OfferType.Guests createOfferTypeGuests() {
return new OfferType.Guests();
}
/**
* Create an instance of {@link OfferType.Inventories }
*
* @return
* the new instance of {@link OfferType.Inventories }
*/
public OfferType.Inventories createOfferTypeInventories() {
return new OfferType.Inventories();
}
/**
* Create an instance of {@link OfferType.CompatibleOffers }
*
* @return
* the new instance of {@link OfferType.CompatibleOffers }
*/
public OfferType.CompatibleOffers createOfferTypeCompatibleOffers() {
return new OfferType.CompatibleOffers();
}
/**
* Create an instance of {@link OfferType.FreeUpgrade }
*
* @return
* the new instance of {@link OfferType.FreeUpgrade }
*/
public OfferType.FreeUpgrade createOfferTypeFreeUpgrade() {
return new OfferType.FreeUpgrade();
}
/**
* Create an instance of {@link OfferType.OfferRules }
*
* @return
* the new instance of {@link OfferType.OfferRules }
*/
public OfferType.OfferRules createOfferTypeOfferRules() {
return new OfferType.OfferRules();
}
/**
* Create an instance of {@link OfferType.OfferRules.OfferRule }
*
* @return
* the new instance of {@link OfferType.OfferRules.OfferRule }
*/
public OfferType.OfferRules.OfferRule createOfferTypeOfferRulesOfferRule() {
return new OfferType.OfferRules.OfferRule();
}
/**
* Create an instance of {@link OfferType.OfferRules.OfferRule.Inventories }
*
* @return
* the new instance of {@link OfferType.OfferRules.OfferRule.Inventories }
*/
public OfferType.OfferRules.OfferRule.Inventories createOfferTypeOfferRulesOfferRuleInventories() {
return new OfferType.OfferRules.OfferRule.Inventories();
}
/**
* Create an instance of {@link HotelRatePlanType }
*
* @return
* the new instance of {@link HotelRatePlanType }
*/
public HotelRatePlanType createHotelRatePlanType() {
return new HotelRatePlanType();
}
/**
* Create an instance of {@link HotelRatePlanType.RatePlanLevelFee }
*
* @return
* the new instance of {@link HotelRatePlanType.RatePlanLevelFee }
*/
public HotelRatePlanType.RatePlanLevelFee createHotelRatePlanTypeRatePlanLevelFee() {
return new HotelRatePlanType.RatePlanLevelFee();
}
/**
* Create an instance of {@link HotelRatePlanType.RatePlanShoulders }
*
* @return
* the new instance of {@link HotelRatePlanType.RatePlanShoulders }
*/
public HotelRatePlanType.RatePlanShoulders createHotelRatePlanTypeRatePlanShoulders() {
return new HotelRatePlanType.RatePlanShoulders();
}
/**
* Create an instance of {@link HotelRatePlanType.Offers }
*
* @return
* the new instance of {@link HotelRatePlanType.Offers }
*/
public HotelRatePlanType.Offers createHotelRatePlanTypeOffers() {
return new HotelRatePlanType.Offers();
}
/**
* Create an instance of {@link HotelRatePlanType.Supplements }
*
* @return
* the new instance of {@link HotelRatePlanType.Supplements }
*/
public HotelRatePlanType.Supplements createHotelRatePlanTypeSupplements() {
return new HotelRatePlanType.Supplements();
}
/**
* Create an instance of {@link HotelRatePlanType.Supplements.Supplement }
*
* @return
* the new instance of {@link HotelRatePlanType.Supplements.Supplement }
*/
public HotelRatePlanType.Supplements.Supplement createHotelRatePlanTypeSupplementsSupplement() {
return new HotelRatePlanType.Supplements.Supplement();
}
/**
* Create an instance of {@link HotelRatePlanType.Rates }
*
* @return
* the new instance of {@link HotelRatePlanType.Rates }
*/
public HotelRatePlanType.Rates createHotelRatePlanTypeRates() {
return new HotelRatePlanType.Rates();
}
/**
* Create an instance of {@link RateUploadType }
*
* @return
* the new instance of {@link RateUploadType }
*/
public RateUploadType createRateUploadType() {
return new RateUploadType();
}
/**
* Create an instance of {@link RateUploadType.AdditionalGuestAmounts }
*
* @return
* the new instance of {@link RateUploadType.AdditionalGuestAmounts }
*/
public RateUploadType.AdditionalGuestAmounts createRateUploadTypeAdditionalGuestAmounts() {
return new RateUploadType.AdditionalGuestAmounts();
}
/**
* Create an instance of {@link RateUploadType.BaseByGuestAmts }
*
* @return
* the new instance of {@link RateUploadType.BaseByGuestAmts }
*/
public RateUploadType.BaseByGuestAmts createRateUploadTypeBaseByGuestAmts() {
return new RateUploadType.BaseByGuestAmts();
}
/**
* Create an instance of {@link BookingRulesType }
*
* @return
* the new instance of {@link BookingRulesType }
*/
public BookingRulesType createBookingRulesType() {
return new BookingRulesType();
}
/**
* Create an instance of {@link HotelRatePlanType.BookingRules }
*
* @return
* the new instance of {@link HotelRatePlanType.BookingRules }
*/
public HotelRatePlanType.BookingRules createHotelRatePlanTypeBookingRules() {
return new HotelRatePlanType.BookingRules();
}
/**
* Create an instance of {@link BookingRulesType.BookingRule }
*
* @return
* the new instance of {@link BookingRulesType.BookingRule }
*/
public BookingRulesType.BookingRule createBookingRulesTypeBookingRule() {
return new BookingRulesType.BookingRule();
}
/**
* Create an instance of {@link BookingRulesType.BookingRule.AddtionalRules }
*
* @return
* the new instance of {@link BookingRulesType.BookingRule.AddtionalRules }
*/
public BookingRulesType.BookingRule.AddtionalRules createBookingRulesTypeBookingRuleAddtionalRules() {
return new BookingRulesType.BookingRule.AddtionalRules();
}
/**
* Create an instance of {@link BookingRulesType.BookingRule.AcceptableGuarantees }
*
* @return
* the new instance of {@link BookingRulesType.BookingRule.AcceptableGuarantees }
*/
public BookingRulesType.BookingRule.AcceptableGuarantees createBookingRulesTypeBookingRuleAcceptableGuarantees() {
return new BookingRulesType.BookingRule.AcceptableGuarantees();
}
/**
* Create an instance of {@link GuaranteeType }
*
* @return
* the new instance of {@link GuaranteeType }
*/
public GuaranteeType createGuaranteeType() {
return new GuaranteeType();
}
/**
* Create an instance of {@link GuaranteeType.GuaranteesAccepted }
*
* @return
* the new instance of {@link GuaranteeType.GuaranteesAccepted }
*/
public GuaranteeType.GuaranteesAccepted createGuaranteeTypeGuaranteesAccepted() {
return new GuaranteeType.GuaranteesAccepted();
}
/**
* Create an instance of {@link PaymentFormType }
*
* @return
* the new instance of {@link PaymentFormType }
*/
public PaymentFormType createPaymentFormType() {
return new PaymentFormType();
}
/**
* Create an instance of {@link PaymentFormType.Ticket }
*
* @return
* the new instance of {@link PaymentFormType.Ticket }
*/
public PaymentFormType.Ticket createPaymentFormTypeTicket() {
return new PaymentFormType.Ticket();
}
/**
* Create an instance of {@link PaymentFormType.LoyaltyRedemption }
*
* @return
* the new instance of {@link PaymentFormType.LoyaltyRedemption }
*/
public PaymentFormType.LoyaltyRedemption createPaymentFormTypeLoyaltyRedemption() {
return new PaymentFormType.LoyaltyRedemption();
}
/**
* Create an instance of {@link HotelRatePlanType.DestinationSystemsCode }
*
* @return
* the new instance of {@link HotelRatePlanType.DestinationSystemsCode }
*/
public HotelRatePlanType.DestinationSystemsCode createHotelRatePlanTypeDestinationSystemsCode() {
return new HotelRatePlanType.DestinationSystemsCode();
}
/**
* Create an instance of {@link AvailRequestSegmentsType }
*
* @return
* the new instance of {@link AvailRequestSegmentsType }
*/
public AvailRequestSegmentsType createAvailRequestSegmentsType() {
return new AvailRequestSegmentsType();
}
/**
* Create an instance of {@link AvailRequestSegmentsType.AvailRequestSegment }
*
* @return
* the new instance of {@link AvailRequestSegmentsType.AvailRequestSegment }
*/
public AvailRequestSegmentsType.AvailRequestSegment createAvailRequestSegmentsTypeAvailRequestSegment() {
return new AvailRequestSegmentsType.AvailRequestSegment();
}
/**
* Create an instance of {@link HotelSearchCriteriaType }
*
* @return
* the new instance of {@link HotelSearchCriteriaType }
*/
public HotelSearchCriteriaType createHotelSearchCriteriaType() {
return new HotelSearchCriteriaType();
}
/**
* Create an instance of {@link ItemSearchCriterionType }
*
* @return
* the new instance of {@link ItemSearchCriterionType }
*/
public ItemSearchCriterionType createItemSearchCriterionType() {
return new ItemSearchCriterionType();
}
/**
* Create an instance of {@link HotelSearchCriterionType }
*
* @return
* the new instance of {@link HotelSearchCriterionType }
*/
public HotelSearchCriterionType createHotelSearchCriterionType() {
return new HotelSearchCriterionType();
}
/**
* Create an instance of {@link RatePlanCandidatesType }
*
* @return
* the new instance of {@link RatePlanCandidatesType }
*/
public RatePlanCandidatesType createRatePlanCandidatesType() {
return new RatePlanCandidatesType();
}
/**
* Create an instance of {@link RatePlanCandidatesType.RatePlanCandidate }
*
* @return
* the new instance of {@link RatePlanCandidatesType.RatePlanCandidate }
*/
public RatePlanCandidatesType.RatePlanCandidate createRatePlanCandidatesTypeRatePlanCandidate() {
return new RatePlanCandidatesType.RatePlanCandidate();
}
/**
* Create an instance of {@link RatePlanCandidatesType.RatePlanCandidate.HotelRefs }
*
* @return
* the new instance of {@link RatePlanCandidatesType.RatePlanCandidate.HotelRefs }
*/
public RatePlanCandidatesType.RatePlanCandidate.HotelRefs createRatePlanCandidatesTypeRatePlanCandidateHotelRefs() {
return new RatePlanCandidatesType.RatePlanCandidate.HotelRefs();
}
/**
* Create an instance of {@link ItemSearchCriterionType.AdditionalContents }
*
* @return
* the new instance of {@link ItemSearchCriterionType.AdditionalContents }
*/
public ItemSearchCriterionType.AdditionalContents createItemSearchCriterionTypeAdditionalContents() {
return new ItemSearchCriterionType.AdditionalContents();
}
/**
* Create an instance of {@link AddressType }
*
* @return
* the new instance of {@link AddressType }
*/
public AddressType createAddressType() {
return new AddressType();
}
/**
* Create an instance of {@link AvailRequestSegmentsType.AvailRequestSegment.RoomStayCandidates }
*
* @return
* the new instance of {@link AvailRequestSegmentsType.AvailRequestSegment.RoomStayCandidates }
*/
public AvailRequestSegmentsType.AvailRequestSegment.RoomStayCandidates createAvailRequestSegmentsTypeAvailRequestSegmentRoomStayCandidates() {
return new AvailRequestSegmentsType.AvailRequestSegment.RoomStayCandidates();
}
/**
* Create an instance of {@link WeatherInfoType }
*
* @return
* the new instance of {@link WeatherInfoType }
*/
public WeatherInfoType createWeatherInfoType() {
return new WeatherInfoType();
}
/**
* Create an instance of {@link ViewershipsType }
*
* @return
* the new instance of {@link ViewershipsType }
*/
public ViewershipsType createViewershipsType() {
return new ViewershipsType();
}
/**
* Create an instance of {@link ViewershipsType.Viewership }
*
* @return
* the new instance of {@link ViewershipsType.Viewership }
*/
public ViewershipsType.Viewership createViewershipsTypeViewership() {
return new ViewershipsType.Viewership();
}
/**
* Create an instance of {@link ViewershipsType.Viewership.DistributorTypes }
*
* @return
* the new instance of {@link ViewershipsType.Viewership.DistributorTypes }
*/
public ViewershipsType.Viewership.DistributorTypes createViewershipsTypeViewershipDistributorTypes() {
return new ViewershipsType.Viewership.DistributorTypes();
}
/**
* Create an instance of {@link ViewershipsType.Viewership.BookingChannelCodes }
*
* @return
* the new instance of {@link ViewershipsType.Viewership.BookingChannelCodes }
*/
public ViewershipsType.Viewership.BookingChannelCodes createViewershipsTypeViewershipBookingChannelCodes() {
return new ViewershipsType.Viewership.BookingChannelCodes();
}
/**
* Create an instance of {@link ViewershipsType.Viewership.LocationCodes }
*
* @return
* the new instance of {@link ViewershipsType.Viewership.LocationCodes }
*/
public ViewershipsType.Viewership.LocationCodes createViewershipsTypeViewershipLocationCodes() {
return new ViewershipsType.Viewership.LocationCodes();
}
/**
* Create an instance of {@link ViewershipsType.Viewership.ProfileTypes }
*
* @return
* the new instance of {@link ViewershipsType.Viewership.ProfileTypes }
*/
public ViewershipsType.Viewership.ProfileTypes createViewershipsTypeViewershipProfileTypes() {
return new ViewershipsType.Viewership.ProfileTypes();
}
/**
* Create an instance of {@link ViewershipsType.Viewership.SystemCodes }
*
* @return
* the new instance of {@link ViewershipsType.Viewership.SystemCodes }
*/
public ViewershipsType.Viewership.SystemCodes createViewershipsTypeViewershipSystemCodes() {
return new ViewershipsType.Viewership.SystemCodes();
}
/**
* Create an instance of {@link StatisticType }
*
* @return
* the new instance of {@link StatisticType }
*/
public StatisticType createStatisticType() {
return new StatisticType();
}
/**
* Create an instance of {@link StatisticApplicationSetType }
*
* @return
* the new instance of {@link StatisticApplicationSetType }
*/
public StatisticApplicationSetType createStatisticApplicationSetType() {
return new StatisticApplicationSetType();
}
/**
* Create an instance of {@link StatisticApplicationSetType.CountCategorySummaries }
*
* @return
* the new instance of {@link StatisticApplicationSetType.CountCategorySummaries }
*/
public StatisticApplicationSetType.CountCategorySummaries createStatisticApplicationSetTypeCountCategorySummaries() {
return new StatisticApplicationSetType.CountCategorySummaries();
}
/**
* Create an instance of {@link StatisticApplicationSetType.RevenueCategorySummaries }
*
* @return
* the new instance of {@link StatisticApplicationSetType.RevenueCategorySummaries }
*/
public StatisticApplicationSetType.RevenueCategorySummaries createStatisticApplicationSetTypeRevenueCategorySummaries() {
return new StatisticApplicationSetType.RevenueCategorySummaries();
}
/**
* Create an instance of {@link StatisticApplicationSetType.StatisticCodes }
*
* @return
* the new instance of {@link StatisticApplicationSetType.StatisticCodes }
*/
public StatisticApplicationSetType.StatisticCodes createStatisticApplicationSetTypeStatisticCodes() {
return new StatisticApplicationSetType.StatisticCodes();
}
/**
* Create an instance of {@link SellableProductsType }
*
* @return
* the new instance of {@link SellableProductsType }
*/
public SellableProductsType createSellableProductsType() {
return new SellableProductsType();
}
/**
* Create an instance of {@link SellableProductsType.SellableProduct }
*
* @return
* the new instance of {@link SellableProductsType.SellableProduct }
*/
public SellableProductsType.SellableProduct createSellableProductsTypeSellableProduct() {
return new SellableProductsType.SellableProduct();
}
/**
* Create an instance of {@link SellableProductsType.SellableProduct.DestinationSystemCodes }
*
* @return
* the new instance of {@link SellableProductsType.SellableProduct.DestinationSystemCodes }
*/
public SellableProductsType.SellableProduct.DestinationSystemCodes createSellableProductsTypeSellableProductDestinationSystemCodes() {
return new SellableProductsType.SellableProduct.DestinationSystemCodes();
}
/**
* Create an instance of {@link RoutingHopType }
*
* @return
* the new instance of {@link RoutingHopType }
*/
public RoutingHopType createRoutingHopType() {
return new RoutingHopType();
}
/**
* Create an instance of {@link RoomTypeType }
*
* @return
* the new instance of {@link RoomTypeType }
*/
public RoomTypeType createRoomTypeType() {
return new RoomTypeType();
}
/**
* Create an instance of {@link RoomStayType }
*
* @return
* the new instance of {@link RoomStayType }
*/
public RoomStayType createRoomStayType() {
return new RoomStayType();
}
/**
* Create an instance of {@link RoomStayType.RoomRates }
*
* @return
* the new instance of {@link RoomStayType.RoomRates }
*/
public RoomStayType.RoomRates createRoomStayTypeRoomRates() {
return new RoomStayType.RoomRates();
}
/**
* Create an instance of {@link RoomRateType }
*
* @return
* the new instance of {@link RoomRateType }
*/
public RoomRateType createRoomRateType() {
return new RoomRateType();
}
/**
* Create an instance of {@link RoomStayType.RoomRates.RoomRate }
*
* @return
* the new instance of {@link RoomStayType.RoomRates.RoomRate }
*/
public RoomStayType.RoomRates.RoomRate createRoomStayTypeRoomRatesRoomRate() {
return new RoomStayType.RoomRates.RoomRate();
}
/**
* Create an instance of {@link RoomStayType.RoomRates.RoomRate.GuestCounts }
*
* @return
* the new instance of {@link RoomStayType.RoomRates.RoomRate.GuestCounts }
*/
public RoomStayType.RoomRates.RoomRate.GuestCounts createRoomStayTypeRoomRatesRoomRateGuestCounts() {
return new RoomStayType.RoomRates.RoomRate.GuestCounts();
}
/**
* Create an instance of {@link RoomStayType.RoomRates.RoomRate.Restrictions }
*
* @return
* the new instance of {@link RoomStayType.RoomRates.RoomRate.Restrictions }
*/
public RoomStayType.RoomRates.RoomRate.Restrictions createRoomStayTypeRoomRatesRoomRateRestrictions() {
return new RoomStayType.RoomRates.RoomRate.Restrictions();
}
/**
* Create an instance of {@link RoomRateType.Features }
*
* @return
* the new instance of {@link RoomRateType.Features }
*/
public RoomRateType.Features createRoomRateTypeFeatures() {
return new RoomRateType.Features();
}
/**
* Create an instance of {@link RoomSharesType }
*
* @return
* the new instance of {@link RoomSharesType }
*/
public RoomSharesType createRoomSharesType() {
return new RoomSharesType();
}
/**
* Create an instance of {@link RoomSharesType.RoomShare }
*
* @return
* the new instance of {@link RoomSharesType.RoomShare }
*/
public RoomSharesType.RoomShare createRoomSharesTypeRoomShare() {
return new RoomSharesType.RoomShare();
}
/**
* Create an instance of {@link RoomSharesType.RoomShare.GuestRPHs }
*
* @return
* the new instance of {@link RoomSharesType.RoomShare.GuestRPHs }
*/
public RoomSharesType.RoomShare.GuestRPHs createRoomSharesTypeRoomShareGuestRPHs() {
return new RoomSharesType.RoomShare.GuestRPHs();
}
/**
* Create an instance of {@link RoomStayLiteType }
*
* @return
* the new instance of {@link RoomStayLiteType }
*/
public RoomStayLiteType createRoomStayLiteType() {
return new RoomStayLiteType();
}
/**
* Create an instance of {@link RFPResponseDetailType }
*
* @return
* the new instance of {@link RFPResponseDetailType }
*/
public RFPResponseDetailType createRFPResponseDetailType() {
return new RFPResponseDetailType();
}
/**
* Create an instance of {@link RequiredPaymentsType }
*
* @return
* the new instance of {@link RequiredPaymentsType }
*/
public RequiredPaymentsType createRequiredPaymentsType() {
return new RequiredPaymentsType();
}
/**
* Create an instance of {@link RequiredPaymentsType.GuaranteePayment }
*
* @return
* the new instance of {@link RequiredPaymentsType.GuaranteePayment }
*/
public RequiredPaymentsType.GuaranteePayment createRequiredPaymentsTypeGuaranteePayment() {
return new RequiredPaymentsType.GuaranteePayment();
}
/**
* Create an instance of {@link RateType }
*
* @return
* the new instance of {@link RateType }
*/
public RateType createRateType() {
return new RateType();
}
/**
* Create an instance of {@link AmountType }
*
* @return
* the new instance of {@link AmountType }
*/
public AmountType createAmountType() {
return new AmountType();
}
/**
* Create an instance of {@link RatePlanType }
*
* @return
* the new instance of {@link RatePlanType }
*/
public RatePlanType createRatePlanType() {
return new RatePlanType();
}
/**
* Create an instance of {@link RateAmountMessageType }
*
* @return
* the new instance of {@link RateAmountMessageType }
*/
public RateAmountMessageType createRateAmountMessageType() {
return new RateAmountMessageType();
}
/**
* Create an instance of {@link RateAmountMessageType.Rates }
*
* @return
* the new instance of {@link RateAmountMessageType.Rates }
*/
public RateAmountMessageType.Rates createRateAmountMessageTypeRates() {
return new RateAmountMessageType.Rates();
}
/**
* Create an instance of {@link BasicPropertyInfoType }
*
* @return
* the new instance of {@link BasicPropertyInfoType }
*/
public BasicPropertyInfoType createBasicPropertyInfoType() {
return new BasicPropertyInfoType();
}
/**
* Create an instance of {@link PropertyValueMatchType }
*
* @return
* the new instance of {@link PropertyValueMatchType }
*/
public PropertyValueMatchType createPropertyValueMatchType() {
return new PropertyValueMatchType();
}
/**
* Create an instance of {@link PropertyValueMatchType.Amenities }
*
* @return
* the new instance of {@link PropertyValueMatchType.Amenities }
*/
public PropertyValueMatchType.Amenities createPropertyValueMatchTypeAmenities() {
return new PropertyValueMatchType.Amenities();
}
/**
* Create an instance of {@link BasicPropertyInfoType.ContactNumbers }
*
* @return
* the new instance of {@link BasicPropertyInfoType.ContactNumbers }
*/
public BasicPropertyInfoType.ContactNumbers createBasicPropertyInfoTypeContactNumbers() {
return new BasicPropertyInfoType.ContactNumbers();
}
/**
* Create an instance of {@link ProductDescriptionsType }
*
* @return
* the new instance of {@link ProductDescriptionsType }
*/
public ProductDescriptionsType createProductDescriptionsType() {
return new ProductDescriptionsType();
}
/**
* Create an instance of {@link MessageType }
*
* @return
* the new instance of {@link MessageType }
*/
public MessageType createMessageType() {
return new MessageType();
}
/**
* Create an instance of {@link MembershipType }
*
* @return
* the new instance of {@link MembershipType }
*/
public MembershipType createMembershipType() {
return new MembershipType();
}
/**
* Create an instance of {@link MeetingRoomsType }
*
* @return
* the new instance of {@link MeetingRoomsType }
*/
public MeetingRoomsType createMeetingRoomsType() {
return new MeetingRoomsType();
}
/**
* Create an instance of {@link MeetingRoomsType.MeetingRoom }
*
* @return
* the new instance of {@link MeetingRoomsType.MeetingRoom }
*/
public MeetingRoomsType.MeetingRoom createMeetingRoomsTypeMeetingRoom() {
return new MeetingRoomsType.MeetingRoom();
}
/**
* Create an instance of {@link MeetingRoomCapacityType }
*
* @return
* the new instance of {@link MeetingRoomCapacityType }
*/
public MeetingRoomCapacityType createMeetingRoomCapacityType() {
return new MeetingRoomCapacityType();
}
/**
* Create an instance of {@link LengthsOfStayType }
*
* @return
* the new instance of {@link LengthsOfStayType }
*/
public LengthsOfStayType createLengthsOfStayType() {
return new LengthsOfStayType();
}
/**
* Create an instance of {@link LengthsOfStayType.LengthOfStay }
*
* @return
* the new instance of {@link LengthsOfStayType.LengthOfStay }
*/
public LengthsOfStayType.LengthOfStay createLengthsOfStayTypeLengthOfStay() {
return new LengthsOfStayType.LengthOfStay();
}
/**
* Create an instance of {@link InvBlockType }
*
* @return
* the new instance of {@link InvBlockType }
*/
public InvBlockType createInvBlockType() {
return new InvBlockType();
}
/**
* Create an instance of {@link InvBlockType.BlockDescriptions }
*
* @return
* the new instance of {@link InvBlockType.BlockDescriptions }
*/
public InvBlockType.BlockDescriptions createInvBlockTypeBlockDescriptions() {
return new InvBlockType.BlockDescriptions();
}
/**
* Create an instance of {@link ParagraphType }
*
* @return
* the new instance of {@link ParagraphType }
*/
public ParagraphType createParagraphType() {
return new ParagraphType();
}
/**
* Create an instance of {@link InvBlockRoomType }
*
* @return
* the new instance of {@link InvBlockRoomType }
*/
public InvBlockRoomType createInvBlockRoomType() {
return new InvBlockRoomType();
}
/**
* Create an instance of {@link InvBlockRoomType.RatePlans }
*
* @return
* the new instance of {@link InvBlockRoomType.RatePlans }
*/
public InvBlockRoomType.RatePlans createInvBlockRoomTypeRatePlans() {
return new InvBlockRoomType.RatePlans();
}
/**
* Create an instance of {@link InvBlockRoomType.RatePlans.RatePlan }
*
* @return
* the new instance of {@link InvBlockRoomType.RatePlans.RatePlan }
*/
public InvBlockRoomType.RatePlans.RatePlan createInvBlockRoomTypeRatePlansRatePlan() {
return new InvBlockRoomType.RatePlans.RatePlan();
}
/**
* Create an instance of {@link InvBlockRoomType.RoomTypeAllocations }
*
* @return
* the new instance of {@link InvBlockRoomType.RoomTypeAllocations }
*/
public InvBlockRoomType.RoomTypeAllocations createInvBlockRoomTypeRoomTypeAllocations() {
return new InvBlockRoomType.RoomTypeAllocations();
}
/**
* Create an instance of {@link HotelRoomListType }
*
* @return
* the new instance of {@link HotelRoomListType }
*/
public HotelRoomListType createHotelRoomListType() {
return new HotelRoomListType();
}
/**
* Create an instance of {@link HotelRoomListType.RoomStays }
*
* @return
* the new instance of {@link HotelRoomListType.RoomStays }
*/
public HotelRoomListType.RoomStays createHotelRoomListTypeRoomStays() {
return new HotelRoomListType.RoomStays();
}
/**
* Create an instance of {@link DirectBillType }
*
* @return
* the new instance of {@link DirectBillType }
*/
public DirectBillType createDirectBillType() {
return new DirectBillType();
}
/**
* Create an instance of {@link ContactPersonType }
*
* @return
* the new instance of {@link ContactPersonType }
*/
public ContactPersonType createContactPersonType() {
return new ContactPersonType();
}
/**
* Create an instance of {@link HotelRoomListType.MasterContact }
*
* @return
* the new instance of {@link HotelRoomListType.MasterContact }
*/
public HotelRoomListType.MasterContact createHotelRoomListTypeMasterContact() {
return new HotelRoomListType.MasterContact();
}
/**
* Create an instance of {@link HotelRoomListType.Guests }
*
* @return
* the new instance of {@link HotelRoomListType.Guests }
*/
public HotelRoomListType.Guests createHotelRoomListTypeGuests() {
return new HotelRoomListType.Guests();
}
/**
* Create an instance of {@link HotelRoomListType.Guests.Guest }
*
* @return
* the new instance of {@link HotelRoomListType.Guests.Guest }
*/
public HotelRoomListType.Guests.Guest createHotelRoomListTypeGuestsGuest() {
return new HotelRoomListType.Guests.Guest();
}
/**
* Create an instance of {@link HotelPaymentFormType }
*
* @return
* the new instance of {@link HotelPaymentFormType }
*/
public HotelPaymentFormType createHotelPaymentFormType() {
return new HotelPaymentFormType();
}
/**
* Create an instance of {@link HotelAdditionalChargesType }
*
* @return
* the new instance of {@link HotelAdditionalChargesType }
*/
public HotelAdditionalChargesType createHotelAdditionalChargesType() {
return new HotelAdditionalChargesType();
}
/**
* Create an instance of {@link GuestRoomType }
*
* @return
* the new instance of {@link GuestRoomType }
*/
public GuestRoomType createGuestRoomType() {
return new GuestRoomType();
}
/**
* Create an instance of {@link GuestRoomType.Amenities }
*
* @return
* the new instance of {@link GuestRoomType.Amenities }
*/
public GuestRoomType.Amenities createGuestRoomTypeAmenities() {
return new GuestRoomType.Amenities();
}
/**
* Create an instance of {@link GuestCountType }
*
* @return
* the new instance of {@link GuestCountType }
*/
public GuestCountType createGuestCountType() {
return new GuestCountType();
}
/**
* Create an instance of {@link GDSInfoType }
*
* @return
* the new instance of {@link GDSInfoType }
*/
public GDSInfoType createGDSInfoType() {
return new GDSInfoType();
}
/**
* Create an instance of {@link GDSInfoType.GDSCodes }
*
* @return
* the new instance of {@link GDSInfoType.GDSCodes }
*/
public GDSInfoType.GDSCodes createGDSInfoTypeGDSCodes() {
return new GDSInfoType.GDSCodes();
}
/**
* Create an instance of {@link GDSInfoType.GDSCodes.GDSCode }
*
* @return
* the new instance of {@link GDSInfoType.GDSCodes.GDSCode }
*/
public GDSInfoType.GDSCodes.GDSCode createGDSInfoTypeGDSCodesGDSCode() {
return new GDSInfoType.GDSCodes.GDSCode();
}
/**
* Create an instance of {@link GDSInfoType.GDSCodes.GDSCode.GDSCodeDetails }
*
* @return
* the new instance of {@link GDSInfoType.GDSCodes.GDSCode.GDSCodeDetails }
*/
public GDSInfoType.GDSCodes.GDSCode.GDSCodeDetails createGDSInfoTypeGDSCodesGDSCodeGDSCodeDetails() {
return new GDSInfoType.GDSCodes.GDSCode.GDSCodeDetails();
}
/**
* Create an instance of {@link FeaturesType }
*
* @return
* the new instance of {@link FeaturesType }
*/
public FeaturesType createFeaturesType() {
return new FeaturesType();
}
/**
* Create an instance of {@link FeaturesType.Feature }
*
* @return
* the new instance of {@link FeaturesType.Feature }
*/
public FeaturesType.Feature createFeaturesTypeFeature() {
return new FeaturesType.Feature();
}
/**
* Create an instance of {@link DOWRestrictionsType }
*
* @return
* the new instance of {@link DOWRestrictionsType }
*/
public DOWRestrictionsType createDOWRestrictionsType() {
return new DOWRestrictionsType();
}
/**
* Create an instance of {@link DestinationSystemCodesType }
*
* @return
* the new instance of {@link DestinationSystemCodesType }
*/
public DestinationSystemCodesType createDestinationSystemCodesType() {
return new DestinationSystemCodesType();
}
/**
* Create an instance of {@link CancelPenaltyType }
*
* @return
* the new instance of {@link CancelPenaltyType }
*/
public CancelPenaltyType createCancelPenaltyType() {
return new CancelPenaltyType();
}
/**
* Create an instance of {@link BaseInvCountType }
*
* @return
* the new instance of {@link BaseInvCountType }
*/
public BaseInvCountType createBaseInvCountType() {
return new BaseInvCountType();
}
/**
* Create an instance of {@link BaseInvCountType.InvCounts }
*
* @return
* the new instance of {@link BaseInvCountType.InvCounts }
*/
public BaseInvCountType.InvCounts createBaseInvCountTypeInvCounts() {
return new BaseInvCountType.InvCounts();
}
/**
* Create an instance of {@link BaseInvCountType.InvCounts.InvCount }
*
* @return
* the new instance of {@link BaseInvCountType.InvCounts.InvCount }
*/
public BaseInvCountType.InvCounts.InvCount createBaseInvCountTypeInvCountsInvCount() {
return new BaseInvCountType.InvCounts.InvCount();
}
/**
* Create an instance of {@link AvailStatusMessageType }
*
* @return
* the new instance of {@link AvailStatusMessageType }
*/
public AvailStatusMessageType createAvailStatusMessageType() {
return new AvailStatusMessageType();
}
/**
* Create an instance of {@link AvailStatusMessageType.BestAvailableRates }
*
* @return
* the new instance of {@link AvailStatusMessageType.BestAvailableRates }
*/
public AvailStatusMessageType.BestAvailableRates createAvailStatusMessageTypeBestAvailableRates() {
return new AvailStatusMessageType.BestAvailableRates();
}
/**
* Create an instance of {@link AdjustmentsType }
*
* @return
* the new instance of {@link AdjustmentsType }
*/
public AdjustmentsType createAdjustmentsType() {
return new AdjustmentsType();
}
/**
* Create an instance of {@link AddressesType }
*
* @return
* the new instance of {@link AddressesType }
*/
public AddressesType createAddressesType() {
return new AddressesType();
}
/**
* Create an instance of {@link TransportInfoType }
*
* @return
* the new instance of {@link TransportInfoType }
*/
public TransportInfoType createTransportInfoType() {
return new TransportInfoType();
}
/**
* Create an instance of {@link ServiceRPHsType }
*
* @return
* the new instance of {@link ServiceRPHsType }
*/
public ServiceRPHsType createServiceRPHsType() {
return new ServiceRPHsType();
}
/**
* Create an instance of {@link RoomStaysType }
*
* @return
* the new instance of {@link RoomStaysType }
*/
public RoomStaysType createRoomStaysType() {
return new RoomStaysType();
}
/**
* Create an instance of {@link RoomStaysType.RoomStay }
*
* @return
* the new instance of {@link RoomStaysType.RoomStay }
*/
public RoomStaysType.RoomStay createRoomStaysTypeRoomStay() {
return new RoomStaysType.RoomStay();
}
/**
* Create an instance of {@link RevenueCategoryType }
*
* @return
* the new instance of {@link RevenueCategoryType }
*/
public RevenueCategoryType createRevenueCategoryType() {
return new RevenueCategoryType();
}
/**
* Create an instance of {@link ResGuestType }
*
* @return
* the new instance of {@link ResGuestType }
*/
public ResGuestType createResGuestType() {
return new ResGuestType();
}
/**
* Create an instance of {@link ResGuestType.ProfileRPHs }
*
* @return
* the new instance of {@link ResGuestType.ProfileRPHs }
*/
public ResGuestType.ProfileRPHs createResGuestTypeProfileRPHs() {
return new ResGuestType.ProfileRPHs();
}
/**
* Create an instance of {@link ResCommonDetailType }
*
* @return
* the new instance of {@link ResCommonDetailType }
*/
public ResCommonDetailType createResCommonDetailType() {
return new ResCommonDetailType();
}
/**
* Create an instance of {@link DateTimeSpanType }
*
* @return
* the new instance of {@link DateTimeSpanType }
*/
public DateTimeSpanType createDateTimeSpanType() {
return new DateTimeSpanType();
}
/**
* Create an instance of {@link LoyaltyPointsAccrualsType }
*
* @return
* the new instance of {@link LoyaltyPointsAccrualsType }
*/
public LoyaltyPointsAccrualsType createLoyaltyPointsAccrualsType() {
return new LoyaltyPointsAccrualsType();
}
/**
* Create an instance of {@link HotelReservationType }
*
* @return
* the new instance of {@link HotelReservationType }
*/
public HotelReservationType createHotelReservationType() {
return new HotelReservationType();
}
/**
* Create an instance of {@link HotelReservationType.BillingInstructionCode }
*
* @return
* the new instance of {@link HotelReservationType.BillingInstructionCode }
*/
public HotelReservationType.BillingInstructionCode createHotelReservationTypeBillingInstructionCode() {
return new HotelReservationType.BillingInstructionCode();
}
/**
* Create an instance of {@link HotelResModifyType }
*
* @return
* the new instance of {@link HotelResModifyType }
*/
public HotelResModifyType createHotelResModifyType() {
return new HotelResModifyType();
}
/**
* Create an instance of {@link HotelResModifyType.HotelResModify }
*
* @return
* the new instance of {@link HotelResModifyType.HotelResModify }
*/
public HotelResModifyType.HotelResModify createHotelResModifyTypeHotelResModify() {
return new HotelResModifyType.HotelResModify();
}
/**
* Create an instance of {@link VerificationType }
*
* @return
* the new instance of {@link VerificationType }
*/
public VerificationType createVerificationType() {
return new VerificationType();
}
/**
* Create an instance of {@link PersonNameType }
*
* @return
* the new instance of {@link PersonNameType }
*/
public PersonNameType createPersonNameType() {
return new PersonNameType();
}
/**
* Create an instance of {@link TravelClubType }
*
* @return
* the new instance of {@link TravelClubType }
*/
public TravelClubType createTravelClubType() {
return new TravelClubType();
}
/**
* Create an instance of {@link travel.wink.ws.allotz.ProfileType }
*
* @return
* the new instance of {@link travel.wink.ws.allotz.ProfileType }
*/
public travel.wink.ws.allotz.ProfileType createProfileType() {
return new travel.wink.ws.allotz.ProfileType();
}
/**
* Create an instance of {@link travel.wink.ws.allotz.ProfileType.Comments }
*
* @return
* the new instance of {@link travel.wink.ws.allotz.ProfileType.Comments }
*/
public travel.wink.ws.allotz.ProfileType.Comments createProfileTypeComments() {
return new travel.wink.ws.allotz.ProfileType.Comments();
}
/**
* Create an instance of {@link travel.wink.ws.allotz.ProfileType.Comments.Comment }
*
* @return
* the new instance of {@link travel.wink.ws.allotz.ProfileType.Comments.Comment }
*/
public travel.wink.ws.allotz.ProfileType.Comments.Comment createProfileTypeCommentsComment() {
return new travel.wink.ws.allotz.ProfileType.Comments.Comment();
}
/**
* Create an instance of {@link PreferencesType }
*
* @return
* the new instance of {@link PreferencesType }
*/
public PreferencesType createPreferencesType() {
return new PreferencesType();
}
/**
* Create an instance of {@link OrganizationType }
*
* @return
* the new instance of {@link OrganizationType }
*/
public OrganizationType createOrganizationType() {
return new OrganizationType();
}
/**
* Create an instance of {@link CompanyInfoType }
*
* @return
* the new instance of {@link CompanyInfoType }
*/
public CompanyInfoType createCompanyInfoType() {
return new CompanyInfoType();
}
/**
* Create an instance of {@link AllianceConsortiumType }
*
* @return
* the new instance of {@link AllianceConsortiumType }
*/
public AllianceConsortiumType createAllianceConsortiumType() {
return new AllianceConsortiumType();
}
/**
* Create an instance of {@link AgreementsType }
*
* @return
* the new instance of {@link AgreementsType }
*/
public AgreementsType createAgreementsType() {
return new AgreementsType();
}
/**
* Create an instance of {@link AccessesType }
*
* @return
* the new instance of {@link AccessesType }
*/
public AccessesType createAccessesType() {
return new AccessesType();
}
/**
* Create an instance of {@link VehicleVendorAvailabilityType }
*
* @return
* the new instance of {@link VehicleVendorAvailabilityType }
*/
public VehicleVendorAvailabilityType createVehicleVendorAvailabilityType() {
return new VehicleVendorAvailabilityType();
}
/**
* Create an instance of {@link VehicleVendorAvailabilityType.VehAvails }
*
* @return
* the new instance of {@link VehicleVendorAvailabilityType.VehAvails }
*/
public VehicleVendorAvailabilityType.VehAvails createVehicleVendorAvailabilityTypeVehAvails() {
return new VehicleVendorAvailabilityType.VehAvails();
}
/**
* Create an instance of {@link VehicleVendorAvailabilityType.VehAvails.VehAvail }
*
* @return
* the new instance of {@link VehicleVendorAvailabilityType.VehAvails.VehAvail }
*/
public VehicleVendorAvailabilityType.VehAvails.VehAvail createVehicleVendorAvailabilityTypeVehAvailsVehAvail() {
return new VehicleVendorAvailabilityType.VehAvails.VehAvail();
}
/**
* Create an instance of {@link VehicleCoreType }
*
* @return
* the new instance of {@link VehicleCoreType }
*/
public VehicleCoreType createVehicleCoreType() {
return new VehicleCoreType();
}
/**
* Create an instance of {@link VehicleType }
*
* @return
* the new instance of {@link VehicleType }
*/
public VehicleType createVehicleType() {
return new VehicleType();
}
/**
* Create an instance of {@link VehicleSegmentCoreType }
*
* @return
* the new instance of {@link VehicleSegmentCoreType }
*/
public VehicleSegmentCoreType createVehicleSegmentCoreType() {
return new VehicleSegmentCoreType();
}
/**
* Create an instance of {@link VehicleSegmentAdditionalInfoType }
*
* @return
* the new instance of {@link VehicleSegmentAdditionalInfoType }
*/
public VehicleSegmentAdditionalInfoType createVehicleSegmentAdditionalInfoType() {
return new VehicleSegmentAdditionalInfoType();
}
/**
* Create an instance of {@link VehicleReservationSummaryType }
*
* @return
* the new instance of {@link VehicleReservationSummaryType }
*/
public VehicleReservationSummaryType createVehicleReservationSummaryType() {
return new VehicleReservationSummaryType();
}
/**
* Create an instance of {@link VehicleReservationRQCoreType }
*
* @return
* the new instance of {@link VehicleReservationRQCoreType }
*/
public VehicleReservationRQCoreType createVehicleReservationRQCoreType() {
return new VehicleReservationRQCoreType();
}
/**
* Create an instance of {@link VehicleReservationRQCoreType.SpecialEquipPrefs }
*
* @return
* the new instance of {@link VehicleReservationRQCoreType.SpecialEquipPrefs }
*/
public VehicleReservationRQCoreType.SpecialEquipPrefs createVehicleReservationRQCoreTypeSpecialEquipPrefs() {
return new VehicleReservationRQCoreType.SpecialEquipPrefs();
}
/**
* Create an instance of {@link VehicleReservationRQAdditionalInfoType }
*
* @return
* the new instance of {@link VehicleReservationRQAdditionalInfoType }
*/
public VehicleReservationRQAdditionalInfoType createVehicleReservationRQAdditionalInfoType() {
return new VehicleReservationRQAdditionalInfoType();
}
/**
* Create an instance of {@link PaymentDetailType }
*
* @return
* the new instance of {@link PaymentDetailType }
*/
public PaymentDetailType createPaymentDetailType() {
return new PaymentDetailType();
}
/**
* Create an instance of {@link VehicleReservationRQAdditionalInfoType.CoveragePrefs }
*
* @return
* the new instance of {@link VehicleReservationRQAdditionalInfoType.CoveragePrefs }
*/
public VehicleReservationRQAdditionalInfoType.CoveragePrefs createVehicleReservationRQAdditionalInfoTypeCoveragePrefs() {
return new VehicleReservationRQAdditionalInfoType.CoveragePrefs();
}
/**
* Create an instance of {@link VehicleRentalTransactionType }
*
* @return
* the new instance of {@link VehicleRentalTransactionType }
*/
public VehicleRentalTransactionType createVehicleRentalTransactionType() {
return new VehicleRentalTransactionType();
}
/**
* Create an instance of {@link VehicleRentalTransactionType.PricedEquips }
*
* @return
* the new instance of {@link VehicleRentalTransactionType.PricedEquips }
*/
public VehicleRentalTransactionType.PricedEquips createVehicleRentalTransactionTypePricedEquips() {
return new VehicleRentalTransactionType.PricedEquips();
}
/**
* Create an instance of {@link VehicleRentalTransactionType.PricedEquips.PricedEquip }
*
* @return
* the new instance of {@link VehicleRentalTransactionType.PricedEquips.PricedEquip }
*/
public VehicleRentalTransactionType.PricedEquips.PricedEquip createVehicleRentalTransactionTypePricedEquipsPricedEquip() {
return new VehicleRentalTransactionType.PricedEquips.PricedEquip();
}
/**
* Create an instance of {@link VehicleRentalCoreType }
*
* @return
* the new instance of {@link VehicleRentalCoreType }
*/
public VehicleRentalCoreType createVehicleRentalCoreType() {
return new VehicleRentalCoreType();
}
/**
* Create an instance of {@link VehicleRentalRateType }
*
* @return
* the new instance of {@link VehicleRentalRateType }
*/
public VehicleRentalRateType createVehicleRentalRateType() {
return new VehicleRentalRateType();
}
/**
* Create an instance of {@link RateQualifierType }
*
* @return
* the new instance of {@link RateQualifierType }
*/
public RateQualifierType createRateQualifierType() {
return new RateQualifierType();
}
/**
* Create an instance of {@link RateQualifierType.RateComments }
*
* @return
* the new instance of {@link RateQualifierType.RateComments }
*/
public RateQualifierType.RateComments createRateQualifierTypeRateComments() {
return new RateQualifierType.RateComments();
}
/**
* Create an instance of {@link VehicleRentalDetailsType }
*
* @return
* the new instance of {@link VehicleRentalDetailsType }
*/
public VehicleRentalDetailsType createVehicleRentalDetailsType() {
return new VehicleRentalDetailsType();
}
/**
* Create an instance of {@link VehicleProfileRentalPrefType }
*
* @return
* the new instance of {@link VehicleProfileRentalPrefType }
*/
public VehicleProfileRentalPrefType createVehicleProfileRentalPrefType() {
return new VehicleProfileRentalPrefType();
}
/**
* Create an instance of {@link VehiclePrefType }
*
* @return
* the new instance of {@link VehiclePrefType }
*/
public VehiclePrefType createVehiclePrefType() {
return new VehiclePrefType();
}
/**
* Create an instance of {@link VehicleLocationVehiclesType }
*
* @return
* the new instance of {@link VehicleLocationVehiclesType }
*/
public VehicleLocationVehiclesType createVehicleLocationVehiclesType() {
return new VehicleLocationVehiclesType();
}
/**
* Create an instance of {@link VehicleLocationVehiclesType.VehicleInfos }
*
* @return
* the new instance of {@link VehicleLocationVehiclesType.VehicleInfos }
*/
public VehicleLocationVehiclesType.VehicleInfos createVehicleLocationVehiclesTypeVehicleInfos() {
return new VehicleLocationVehiclesType.VehicleInfos();
}
/**
* Create an instance of {@link VehicleLocationLiabilitiesType }
*
* @return
* the new instance of {@link VehicleLocationLiabilitiesType }
*/
public VehicleLocationLiabilitiesType createVehicleLocationLiabilitiesType() {
return new VehicleLocationLiabilitiesType();
}
/**
* Create an instance of {@link VehicleLocationLiabilitiesType.Coverages }
*
* @return
* the new instance of {@link VehicleLocationLiabilitiesType.Coverages }
*/
public VehicleLocationLiabilitiesType.Coverages createVehicleLocationLiabilitiesTypeCoverages() {
return new VehicleLocationLiabilitiesType.Coverages();
}
/**
* Create an instance of {@link VehicleLocationLiabilitiesType.Coverages.Coverage }
*
* @return
* the new instance of {@link VehicleLocationLiabilitiesType.Coverages.Coverage }
*/
public VehicleLocationLiabilitiesType.Coverages.Coverage createVehicleLocationLiabilitiesTypeCoveragesCoverage() {
return new VehicleLocationLiabilitiesType.Coverages.Coverage();
}
/**
* Create an instance of {@link VehicleLocationLiabilitiesType.Coverages.Coverage.CoverageFees }
*
* @return
* the new instance of {@link VehicleLocationLiabilitiesType.Coverages.Coverage.CoverageFees }
*/
public VehicleLocationLiabilitiesType.Coverages.Coverage.CoverageFees createVehicleLocationLiabilitiesTypeCoveragesCoverageCoverageFees() {
return new VehicleLocationLiabilitiesType.Coverages.Coverage.CoverageFees();
}
/**
* Create an instance of {@link VehicleLocationLiabilitiesType.Coverages.Coverage.CoverageFees.CoverageFee }
*
* @return
* the new instance of {@link VehicleLocationLiabilitiesType.Coverages.Coverage.CoverageFees.CoverageFee }
*/
public VehicleLocationLiabilitiesType.Coverages.Coverage.CoverageFees.CoverageFee createVehicleLocationLiabilitiesTypeCoveragesCoverageCoverageFeesCoverageFee() {
return new VehicleLocationLiabilitiesType.Coverages.Coverage.CoverageFees.CoverageFee();
}
/**
* Create an instance of {@link VehicleLocationLiabilitiesType.Coverages.Coverage.CoverageFees.CoverageFee.Vehicles }
*
* @return
* the new instance of {@link VehicleLocationLiabilitiesType.Coverages.Coverage.CoverageFees.CoverageFee.Vehicles }
*/
public VehicleLocationLiabilitiesType.Coverages.Coverage.CoverageFees.CoverageFee.Vehicles createVehicleLocationLiabilitiesTypeCoveragesCoverageCoverageFeesCoverageFeeVehicles() {
return new VehicleLocationLiabilitiesType.Coverages.Coverage.CoverageFees.CoverageFee.Vehicles();
}
/**
* Create an instance of {@link VehicleLocationDetailsType }
*
* @return
* the new instance of {@link VehicleLocationDetailsType }
*/
public VehicleLocationDetailsType createVehicleLocationDetailsType() {
return new VehicleLocationDetailsType();
}
/**
* Create an instance of {@link VehicleLocationAdditionalFeesType }
*
* @return
* the new instance of {@link VehicleLocationAdditionalFeesType }
*/
public VehicleLocationAdditionalFeesType createVehicleLocationAdditionalFeesType() {
return new VehicleLocationAdditionalFeesType();
}
/**
* Create an instance of {@link VehicleLocationAdditionalFeesType.MiscellaneousCharges }
*
* @return
* the new instance of {@link VehicleLocationAdditionalFeesType.MiscellaneousCharges }
*/
public VehicleLocationAdditionalFeesType.MiscellaneousCharges createVehicleLocationAdditionalFeesTypeMiscellaneousCharges() {
return new VehicleLocationAdditionalFeesType.MiscellaneousCharges();
}
/**
* Create an instance of {@link VehicleChargeType }
*
* @return
* the new instance of {@link VehicleChargeType }
*/
public VehicleChargeType createVehicleChargeType() {
return new VehicleChargeType();
}
/**
* Create an instance of {@link VehicleChargeType.TaxAmounts }
*
* @return
* the new instance of {@link VehicleChargeType.TaxAmounts }
*/
public VehicleChargeType.TaxAmounts createVehicleChargeTypeTaxAmounts() {
return new VehicleChargeType.TaxAmounts();
}
/**
* Create an instance of {@link VehicleLocationAdditionalFeesType.Surcharges }
*
* @return
* the new instance of {@link VehicleLocationAdditionalFeesType.Surcharges }
*/
public VehicleLocationAdditionalFeesType.Surcharges createVehicleLocationAdditionalFeesTypeSurcharges() {
return new VehicleLocationAdditionalFeesType.Surcharges();
}
/**
* Create an instance of {@link VehicleLocationAdditionalFeesType.Fees }
*
* @return
* the new instance of {@link VehicleLocationAdditionalFeesType.Fees }
*/
public VehicleLocationAdditionalFeesType.Fees createVehicleLocationAdditionalFeesTypeFees() {
return new VehicleLocationAdditionalFeesType.Fees();
}
/**
* Create an instance of {@link VehicleLocationAdditionalFeesType.Taxes }
*
* @return
* the new instance of {@link VehicleLocationAdditionalFeesType.Taxes }
*/
public VehicleLocationAdditionalFeesType.Taxes createVehicleLocationAdditionalFeesTypeTaxes() {
return new VehicleLocationAdditionalFeesType.Taxes();
}
/**
* Create an instance of {@link VehicleLocationAdditionalDetailsType }
*
* @return
* the new instance of {@link VehicleLocationAdditionalDetailsType }
*/
public VehicleLocationAdditionalDetailsType createVehicleLocationAdditionalDetailsType() {
return new VehicleLocationAdditionalDetailsType();
}
/**
* Create an instance of {@link VehicleLocationAdditionalDetailsType.OneWayDropLocations }
*
* @return
* the new instance of {@link VehicleLocationAdditionalDetailsType.OneWayDropLocations }
*/
public VehicleLocationAdditionalDetailsType.OneWayDropLocations createVehicleLocationAdditionalDetailsTypeOneWayDropLocations() {
return new VehicleLocationAdditionalDetailsType.OneWayDropLocations();
}
/**
* Create an instance of {@link VehicleLocationAdditionalDetailsType.Shuttle }
*
* @return
* the new instance of {@link VehicleLocationAdditionalDetailsType.Shuttle }
*/
public VehicleLocationAdditionalDetailsType.Shuttle createVehicleLocationAdditionalDetailsTypeShuttle() {
return new VehicleLocationAdditionalDetailsType.Shuttle();
}
/**
* Create an instance of {@link VehicleLocationAdditionalDetailsType.Shuttle.ShuttleInfos }
*
* @return
* the new instance of {@link VehicleLocationAdditionalDetailsType.Shuttle.ShuttleInfos }
*/
public VehicleLocationAdditionalDetailsType.Shuttle.ShuttleInfos createVehicleLocationAdditionalDetailsTypeShuttleShuttleInfos() {
return new VehicleLocationAdditionalDetailsType.Shuttle.ShuttleInfos();
}
/**
* Create an instance of {@link VehicleAvailVendorInfoType }
*
* @return
* the new instance of {@link VehicleAvailVendorInfoType }
*/
public VehicleAvailVendorInfoType createVehicleAvailVendorInfoType() {
return new VehicleAvailVendorInfoType();
}
/**
* Create an instance of {@link VehicleAvailRSCoreType }
*
* @return
* the new instance of {@link VehicleAvailRSCoreType }
*/
public VehicleAvailRSCoreType createVehicleAvailRSCoreType() {
return new VehicleAvailRSCoreType();
}
/**
* Create an instance of {@link VehicleAvailRQCoreType }
*
* @return
* the new instance of {@link VehicleAvailRQCoreType }
*/
public VehicleAvailRQCoreType createVehicleAvailRQCoreType() {
return new VehicleAvailRQCoreType();
}
/**
* Create an instance of {@link VehicleAvailRQCoreType.SpecialEquipPrefs }
*
* @return
* the new instance of {@link VehicleAvailRQCoreType.SpecialEquipPrefs }
*/
public VehicleAvailRQCoreType.SpecialEquipPrefs createVehicleAvailRQCoreTypeSpecialEquipPrefs() {
return new VehicleAvailRQCoreType.SpecialEquipPrefs();
}
/**
* Create an instance of {@link VehicleAvailRQCoreType.VehPrefs }
*
* @return
* the new instance of {@link VehicleAvailRQCoreType.VehPrefs }
*/
public VehicleAvailRQCoreType.VehPrefs createVehicleAvailRQCoreTypeVehPrefs() {
return new VehicleAvailRQCoreType.VehPrefs();
}
/**
* Create an instance of {@link VehicleAvailRQCoreType.VendorPrefs }
*
* @return
* the new instance of {@link VehicleAvailRQCoreType.VendorPrefs }
*/
public VehicleAvailRQCoreType.VendorPrefs createVehicleAvailRQCoreTypeVendorPrefs() {
return new VehicleAvailRQCoreType.VendorPrefs();
}
/**
* Create an instance of {@link VehicleAvailRQAdditionalInfoType }
*
* @return
* the new instance of {@link VehicleAvailRQAdditionalInfoType }
*/
public VehicleAvailRQAdditionalInfoType createVehicleAvailRQAdditionalInfoType() {
return new VehicleAvailRQAdditionalInfoType();
}
/**
* Create an instance of {@link VehicleAvailRQAdditionalInfoType.CoveragePrefs }
*
* @return
* the new instance of {@link VehicleAvailRQAdditionalInfoType.CoveragePrefs }
*/
public VehicleAvailRQAdditionalInfoType.CoveragePrefs createVehicleAvailRQAdditionalInfoTypeCoveragePrefs() {
return new VehicleAvailRQAdditionalInfoType.CoveragePrefs();
}
/**
* Create an instance of {@link VehicleAvailCoreType }
*
* @return
* the new instance of {@link VehicleAvailCoreType }
*/
public VehicleAvailCoreType createVehicleAvailCoreType() {
return new VehicleAvailCoreType();
}
/**
* Create an instance of {@link VehicleAvailAdditionalInfoType }
*
* @return
* the new instance of {@link VehicleAvailAdditionalInfoType }
*/
public VehicleAvailAdditionalInfoType createVehicleAvailAdditionalInfoType() {
return new VehicleAvailAdditionalInfoType();
}
/**
* Create an instance of {@link VehicleAgeRequirementsType }
*
* @return
* the new instance of {@link VehicleAgeRequirementsType }
*/
public VehicleAgeRequirementsType createVehicleAgeRequirementsType() {
return new VehicleAgeRequirementsType();
}
/**
* Create an instance of {@link VehicleAgeRequirementsType.Age }
*
* @return
* the new instance of {@link VehicleAgeRequirementsType.Age }
*/
public VehicleAgeRequirementsType.Age createVehicleAgeRequirementsTypeAge() {
return new VehicleAgeRequirementsType.Age();
}
/**
* Create an instance of {@link VehicleAgeRequirementsType.Age.Vehicles }
*
* @return
* the new instance of {@link VehicleAgeRequirementsType.Age.Vehicles }
*/
public VehicleAgeRequirementsType.Age.Vehicles createVehicleAgeRequirementsTypeAgeVehicles() {
return new VehicleAgeRequirementsType.Age.Vehicles();
}
/**
* Create an instance of {@link VehicleAgeRequirementsType.Age.AgeInfos }
*
* @return
* the new instance of {@link VehicleAgeRequirementsType.Age.AgeInfos }
*/
public VehicleAgeRequirementsType.Age.AgeInfos createVehicleAgeRequirementsTypeAgeAgeInfos() {
return new VehicleAgeRequirementsType.Age.AgeInfos();
}
/**
* Create an instance of {@link VehicleAdditionalDriverRequirementsType }
*
* @return
* the new instance of {@link VehicleAdditionalDriverRequirementsType }
*/
public VehicleAdditionalDriverRequirementsType createVehicleAdditionalDriverRequirementsType() {
return new VehicleAdditionalDriverRequirementsType();
}
/**
* Create an instance of {@link VehicleAdditionalDriverRequirementsType.AddlDriverInfos }
*
* @return
* the new instance of {@link VehicleAdditionalDriverRequirementsType.AddlDriverInfos }
*/
public VehicleAdditionalDriverRequirementsType.AddlDriverInfos createVehicleAdditionalDriverRequirementsTypeAddlDriverInfos() {
return new VehicleAdditionalDriverRequirementsType.AddlDriverInfos();
}
/**
* Create an instance of {@link VehicleAdditionalDriverRequirementsType.AddlDriverInfos.Vehicles }
*
* @return
* the new instance of {@link VehicleAdditionalDriverRequirementsType.AddlDriverInfos.Vehicles }
*/
public VehicleAdditionalDriverRequirementsType.AddlDriverInfos.Vehicles createVehicleAdditionalDriverRequirementsTypeAddlDriverInfosVehicles() {
return new VehicleAdditionalDriverRequirementsType.AddlDriverInfos.Vehicles();
}
/**
* Create an instance of {@link RateRulesType }
*
* @return
* the new instance of {@link RateRulesType }
*/
public RateRulesType createRateRulesType() {
return new RateRulesType();
}
/**
* Create an instance of {@link RateRulesType.CancelPenaltyInfo }
*
* @return
* the new instance of {@link RateRulesType.CancelPenaltyInfo }
*/
public RateRulesType.CancelPenaltyInfo createRateRulesTypeCancelPenaltyInfo() {
return new RateRulesType.CancelPenaltyInfo();
}
/**
* Create an instance of {@link RateRulesType.PaymentRules }
*
* @return
* the new instance of {@link RateRulesType.PaymentRules }
*/
public RateRulesType.PaymentRules createRateRulesTypePaymentRules() {
return new RateRulesType.PaymentRules();
}
/**
* Create an instance of {@link RateRulesType.PaymentRules.AcceptablePayments }
*
* @return
* the new instance of {@link RateRulesType.PaymentRules.AcceptablePayments }
*/
public RateRulesType.PaymentRules.AcceptablePayments createRateRulesTypePaymentRulesAcceptablePayments() {
return new RateRulesType.PaymentRules.AcceptablePayments();
}
/**
* Create an instance of {@link RateRulesType.PickupReturnRules }
*
* @return
* the new instance of {@link RateRulesType.PickupReturnRules }
*/
public RateRulesType.PickupReturnRules createRateRulesTypePickupReturnRules() {
return new RateRulesType.PickupReturnRules();
}
/**
* Create an instance of {@link OffLocationServiceCoreType }
*
* @return
* the new instance of {@link OffLocationServiceCoreType }
*/
public OffLocationServiceCoreType createOffLocationServiceCoreType() {
return new OffLocationServiceCoreType();
}
/**
* Create an instance of {@link OffLocationServiceType }
*
* @return
* the new instance of {@link OffLocationServiceType }
*/
public OffLocationServiceType createOffLocationServiceType() {
return new OffLocationServiceType();
}
/**
* Create an instance of {@link NoShowFeeType }
*
* @return
* the new instance of {@link NoShowFeeType }
*/
public NoShowFeeType createNoShowFeeType() {
return new NoShowFeeType();
}
/**
* Create an instance of {@link CustomerPrimaryAdditionalType }
*
* @return
* the new instance of {@link CustomerPrimaryAdditionalType }
*/
public CustomerPrimaryAdditionalType createCustomerPrimaryAdditionalType() {
return new CustomerPrimaryAdditionalType();
}
/**
* Create an instance of {@link CustomerType }
*
* @return
* the new instance of {@link CustomerType }
*/
public CustomerType createCustomerType() {
return new CustomerType();
}
/**
* Create an instance of {@link CustomerType.CustLoyalty }
*
* @return
* the new instance of {@link CustomerType.CustLoyalty }
*/
public CustomerType.CustLoyalty createCustomerTypeCustLoyalty() {
return new CustomerType.CustLoyalty();
}
/**
* Create an instance of {@link CustomerType.CustLoyalty.SecurityInfo }
*
* @return
* the new instance of {@link CustomerType.CustLoyalty.SecurityInfo }
*/
public CustomerType.CustLoyalty.SecurityInfo createCustomerTypeCustLoyaltySecurityInfo() {
return new CustomerType.CustLoyalty.SecurityInfo();
}
/**
* Create an instance of {@link CustomerType.CustLoyalty.MemberPreferences }
*
* @return
* the new instance of {@link CustomerType.CustLoyalty.MemberPreferences }
*/
public CustomerType.CustLoyalty.MemberPreferences createCustomerTypeCustLoyaltyMemberPreferences() {
return new CustomerType.CustLoyalty.MemberPreferences();
}
/**
* Create an instance of {@link CustomerType.CustLoyalty.MemberPreferences.Offer }
*
* @return
* the new instance of {@link CustomerType.CustLoyalty.MemberPreferences.Offer }
*/
public CustomerType.CustLoyalty.MemberPreferences.Offer createCustomerTypeCustLoyaltyMemberPreferencesOffer() {
return new CustomerType.CustLoyalty.MemberPreferences.Offer();
}
/**
* Create an instance of {@link CustomerType.PaymentForm }
*
* @return
* the new instance of {@link CustomerType.PaymentForm }
*/
public CustomerType.PaymentForm createCustomerTypePaymentForm() {
return new CustomerType.PaymentForm();
}
/**
* Create an instance of {@link AirSearchPrefsType }
*
* @return
* the new instance of {@link AirSearchPrefsType }
*/
public AirSearchPrefsType createAirSearchPrefsType() {
return new AirSearchPrefsType();
}
/**
* Create an instance of {@link AirlinePrefType }
*
* @return
* the new instance of {@link AirlinePrefType }
*/
public AirlinePrefType createAirlinePrefType() {
return new AirlinePrefType();
}
/**
* Create an instance of {@link AirlinePrefType.AccountInformation }
*
* @return
* the new instance of {@link AirlinePrefType.AccountInformation }
*/
public AirlinePrefType.AccountInformation createAirlinePrefTypeAccountInformation() {
return new AirlinePrefType.AccountInformation();
}
/**
* Create an instance of {@link AirlinePrefType.TourCodePref }
*
* @return
* the new instance of {@link AirlinePrefType.TourCodePref }
*/
public AirlinePrefType.TourCodePref createAirlinePrefTypeTourCodePref() {
return new AirlinePrefType.TourCodePref();
}
/**
* Create an instance of {@link PhonePrefType }
*
* @return
* the new instance of {@link PhonePrefType }
*/
public PhonePrefType createPhonePrefType() {
return new PhonePrefType();
}
/**
* Create an instance of {@link CommonPrefType }
*
* @return
* the new instance of {@link CommonPrefType }
*/
public CommonPrefType createCommonPrefType() {
return new CommonPrefType();
}
/**
* Create an instance of {@link VoluntaryChangesType }
*
* @return
* the new instance of {@link VoluntaryChangesType }
*/
public VoluntaryChangesType createVoluntaryChangesType() {
return new VoluntaryChangesType();
}
/**
* Create an instance of {@link TravelerInfoType }
*
* @return
* the new instance of {@link TravelerInfoType }
*/
public TravelerInfoType createTravelerInfoType() {
return new TravelerInfoType();
}
/**
* Create an instance of {@link AirTravelerType }
*
* @return
* the new instance of {@link AirTravelerType }
*/
public AirTravelerType createAirTravelerType() {
return new AirTravelerType();
}
/**
* Create an instance of {@link TravelerInfoType.AirTraveler }
*
* @return
* the new instance of {@link TravelerInfoType.AirTraveler }
*/
public TravelerInfoType.AirTraveler createTravelerInfoTypeAirTraveler() {
return new TravelerInfoType.AirTraveler();
}
/**
* Create an instance of {@link DocumentType }
*
* @return
* the new instance of {@link DocumentType }
*/
public DocumentType createDocumentType() {
return new DocumentType();
}
/**
* Create an instance of {@link TravelerInfoSummaryType }
*
* @return
* the new instance of {@link TravelerInfoSummaryType }
*/
public TravelerInfoSummaryType createTravelerInfoSummaryType() {
return new TravelerInfoSummaryType();
}
/**
* Create an instance of {@link PriceRequestInformationType }
*
* @return
* the new instance of {@link PriceRequestInformationType }
*/
public PriceRequestInformationType createPriceRequestInformationType() {
return new PriceRequestInformationType();
}
/**
* Create an instance of {@link TravelerInfoSummaryType.PriceRequestInformation }
*
* @return
* the new instance of {@link TravelerInfoSummaryType.PriceRequestInformation }
*/
public TravelerInfoSummaryType.PriceRequestInformation createTravelerInfoSummaryTypePriceRequestInformation() {
return new TravelerInfoSummaryType.PriceRequestInformation();
}
/**
* Create an instance of {@link TravelerInfoSummaryType.PriceRequestInformation.DiscountPricing }
*
* @return
* the new instance of {@link TravelerInfoSummaryType.PriceRequestInformation.DiscountPricing }
*/
public TravelerInfoSummaryType.PriceRequestInformation.DiscountPricing createTravelerInfoSummaryTypePriceRequestInformationDiscountPricing() {
return new TravelerInfoSummaryType.PriceRequestInformation.DiscountPricing();
}
/**
* Create an instance of {@link TicketingInfoType }
*
* @return
* the new instance of {@link TicketingInfoType }
*/
public TicketingInfoType createTicketingInfoType() {
return new TicketingInfoType();
}
/**
* Create an instance of {@link StayRestrictionsType }
*
* @return
* the new instance of {@link StayRestrictionsType }
*/
public StayRestrictionsType createStayRestrictionsType() {
return new StayRestrictionsType();
}
/**
* Create an instance of {@link SpecificFlightInfoType }
*
* @return
* the new instance of {@link SpecificFlightInfoType }
*/
public SpecificFlightInfoType createSpecificFlightInfoType() {
return new SpecificFlightInfoType();
}
/**
* Create an instance of {@link SpecialReqDetailsType }
*
* @return
* the new instance of {@link SpecialReqDetailsType }
*/
public SpecialReqDetailsType createSpecialReqDetailsType() {
return new SpecialReqDetailsType();
}
/**
* Create an instance of {@link SpecialReqDetailsType.SpecialRemarks }
*
* @return
* the new instance of {@link SpecialReqDetailsType.SpecialRemarks }
*/
public SpecialReqDetailsType.SpecialRemarks createSpecialReqDetailsTypeSpecialRemarks() {
return new SpecialReqDetailsType.SpecialRemarks();
}
/**
* Create an instance of {@link SpecialRemarkType }
*
* @return
* the new instance of {@link SpecialRemarkType }
*/
public SpecialRemarkType createSpecialRemarkType() {
return new SpecialRemarkType();
}
/**
* Create an instance of {@link SpecialRemarkType.AuthorizedViewers }
*
* @return
* the new instance of {@link SpecialRemarkType.AuthorizedViewers }
*/
public SpecialRemarkType.AuthorizedViewers createSpecialRemarkTypeAuthorizedViewers() {
return new SpecialRemarkType.AuthorizedViewers();
}
/**
* Create an instance of {@link SpecialReqDetailsType.Remarks }
*
* @return
* the new instance of {@link SpecialReqDetailsType.Remarks }
*/
public SpecialReqDetailsType.Remarks createSpecialReqDetailsTypeRemarks() {
return new SpecialReqDetailsType.Remarks();
}
/**
* Create an instance of {@link SpecialReqDetailsType.OtherServiceInformations }
*
* @return
* the new instance of {@link SpecialReqDetailsType.OtherServiceInformations }
*/
public SpecialReqDetailsType.OtherServiceInformations createSpecialReqDetailsTypeOtherServiceInformations() {
return new SpecialReqDetailsType.OtherServiceInformations();
}
/**
* Create an instance of {@link OtherServiceInfoType }
*
* @return
* the new instance of {@link OtherServiceInfoType }
*/
public OtherServiceInfoType createOtherServiceInfoType() {
return new OtherServiceInfoType();
}
/**
* Create an instance of {@link SpecialReqDetailsType.SpecialServiceRequests }
*
* @return
* the new instance of {@link SpecialReqDetailsType.SpecialServiceRequests }
*/
public SpecialReqDetailsType.SpecialServiceRequests createSpecialReqDetailsTypeSpecialServiceRequests() {
return new SpecialReqDetailsType.SpecialServiceRequests();
}
/**
* Create an instance of {@link SpecialReqDetailsType.SeatRequests }
*
* @return
* the new instance of {@link SpecialReqDetailsType.SeatRequests }
*/
public SpecialReqDetailsType.SeatRequests createSpecialReqDetailsTypeSeatRequests() {
return new SpecialReqDetailsType.SeatRequests();
}
/**
* Create an instance of {@link SeatMapDetailsType }
*
* @return
* the new instance of {@link SeatMapDetailsType }
*/
public SeatMapDetailsType createSeatMapDetailsType() {
return new SeatMapDetailsType();
}
/**
* Create an instance of {@link CabinClassType }
*
* @return
* the new instance of {@link CabinClassType }
*/
public CabinClassType createCabinClassType() {
return new CabinClassType();
}
/**
* Create an instance of {@link RuleInfoType }
*
* @return
* the new instance of {@link RuleInfoType }
*/
public RuleInfoType createRuleInfoType() {
return new RuleInfoType();
}
/**
* Create an instance of {@link RuleInfoType.ResTicketingRules }
*
* @return
* the new instance of {@link RuleInfoType.ResTicketingRules }
*/
public RuleInfoType.ResTicketingRules createRuleInfoTypeResTicketingRules() {
return new RuleInfoType.ResTicketingRules();
}
/**
* Create an instance of {@link AdvResTicketingType }
*
* @return
* the new instance of {@link AdvResTicketingType }
*/
public AdvResTicketingType createAdvResTicketingType() {
return new AdvResTicketingType();
}
/**
* Create an instance of {@link RowDetailsType }
*
* @return
* the new instance of {@link RowDetailsType }
*/
public RowDetailsType createRowDetailsType() {
return new RowDetailsType();
}
/**
* Create an instance of {@link RowDetailsType.AirSeats }
*
* @return
* the new instance of {@link RowDetailsType.AirSeats }
*/
public RowDetailsType.AirSeats createRowDetailsTypeAirSeats() {
return new RowDetailsType.AirSeats();
}
/**
* Create an instance of {@link PTCFareBreakdownType }
*
* @return
* the new instance of {@link PTCFareBreakdownType }
*/
public PTCFareBreakdownType createPTCFareBreakdownType() {
return new PTCFareBreakdownType();
}
/**
* Create an instance of {@link PTCFareBreakdownType.PricingUnit }
*
* @return
* the new instance of {@link PTCFareBreakdownType.PricingUnit }
*/
public PTCFareBreakdownType.PricingUnit createPTCFareBreakdownTypePricingUnit() {
return new PTCFareBreakdownType.PricingUnit();
}
/**
* Create an instance of {@link PTCFareBreakdownType.PricingUnit.FareComponent }
*
* @return
* the new instance of {@link PTCFareBreakdownType.PricingUnit.FareComponent }
*/
public PTCFareBreakdownType.PricingUnit.FareComponent createPTCFareBreakdownTypePricingUnitFareComponent() {
return new PTCFareBreakdownType.PricingUnit.FareComponent();
}
/**
* Create an instance of {@link FlightSegmentBaseType }
*
* @return
* the new instance of {@link FlightSegmentBaseType }
*/
public FlightSegmentBaseType createFlightSegmentBaseType() {
return new FlightSegmentBaseType();
}
/**
* Create an instance of {@link FlightSegmentType }
*
* @return
* the new instance of {@link FlightSegmentType }
*/
public FlightSegmentType createFlightSegmentType() {
return new FlightSegmentType();
}
/**
* Create an instance of {@link BookFlightSegmentType }
*
* @return
* the new instance of {@link BookFlightSegmentType }
*/
public BookFlightSegmentType createBookFlightSegmentType() {
return new BookFlightSegmentType();
}
/**
* Create an instance of {@link BookFlightSegmentType.BookingClassAvails }
*
* @return
* the new instance of {@link BookFlightSegmentType.BookingClassAvails }
*/
public BookFlightSegmentType.BookingClassAvails createBookFlightSegmentTypeBookingClassAvails() {
return new BookFlightSegmentType.BookingClassAvails();
}
/**
* Create an instance of {@link FareInfoType }
*
* @return
* the new instance of {@link FareInfoType }
*/
public FareInfoType createFareInfoType() {
return new FareInfoType();
}
/**
* Create an instance of {@link FareInfoType.FareInfo }
*
* @return
* the new instance of {@link FareInfoType.FareInfo }
*/
public FareInfoType.FareInfo createFareInfoTypeFareInfo() {
return new FareInfoType.FareInfo();
}
/**
* Create an instance of {@link PTCFareBreakdownType.Endorsements }
*
* @return
* the new instance of {@link PTCFareBreakdownType.Endorsements }
*/
public PTCFareBreakdownType.Endorsements createPTCFareBreakdownTypeEndorsements() {
return new PTCFareBreakdownType.Endorsements();
}
/**
* Create an instance of {@link PTCFareBreakdownType.TicketDesignators }
*
* @return
* the new instance of {@link PTCFareBreakdownType.TicketDesignators }
*/
public PTCFareBreakdownType.TicketDesignators createPTCFareBreakdownTypeTicketDesignators() {
return new PTCFareBreakdownType.TicketDesignators();
}
/**
* Create an instance of {@link FareType }
*
* @return
* the new instance of {@link FareType }
*/
public FareType createFareType() {
return new FareType();
}
/**
* Create an instance of {@link PTCFareBreakdownType.PassengerFare }
*
* @return
* the new instance of {@link PTCFareBreakdownType.PassengerFare }
*/
public PTCFareBreakdownType.PassengerFare createPTCFareBreakdownTypePassengerFare() {
return new PTCFareBreakdownType.PassengerFare();
}
/**
* Create an instance of {@link PTCFareBreakdownType.PassengerFare.TicketFeeDetail }
*
* @return
* the new instance of {@link PTCFareBreakdownType.PassengerFare.TicketFeeDetail }
*/
public PTCFareBreakdownType.PassengerFare.TicketFeeDetail createPTCFareBreakdownTypePassengerFareTicketFeeDetail() {
return new PTCFareBreakdownType.PassengerFare.TicketFeeDetail();
}
/**
* Create an instance of {@link PTCFareBreakdownType.PassengerFare.TicketFeeDetail.Fee }
*
* @return
* the new instance of {@link PTCFareBreakdownType.PassengerFare.TicketFeeDetail.Fee }
*/
public PTCFareBreakdownType.PassengerFare.TicketFeeDetail.Fee createPTCFareBreakdownTypePassengerFareTicketFeeDetailFee() {
return new PTCFareBreakdownType.PassengerFare.TicketFeeDetail.Fee();
}
/**
* Create an instance of {@link FareType.Discounts }
*
* @return
* the new instance of {@link FareType.Discounts }
*/
public FareType.Discounts createFareTypeDiscounts() {
return new FareType.Discounts();
}
/**
* Create an instance of {@link FareType.ExchangeInfo }
*
* @return
* the new instance of {@link FareType.ExchangeInfo }
*/
public FareType.ExchangeInfo createFareTypeExchangeInfo() {
return new FareType.ExchangeInfo();
}
/**
* Create an instance of {@link PricedItineraryType }
*
* @return
* the new instance of {@link PricedItineraryType }
*/
public PricedItineraryType createPricedItineraryType() {
return new PricedItineraryType();
}
/**
* Create an instance of {@link PricedItineraryType.TicketingInfo }
*
* @return
* the new instance of {@link PricedItineraryType.TicketingInfo }
*/
public PricedItineraryType.TicketingInfo createPricedItineraryTypeTicketingInfo() {
return new PricedItineraryType.TicketingInfo();
}
/**
* Create an instance of {@link AirItineraryPricingInfoType }
*
* @return
* the new instance of {@link AirItineraryPricingInfoType }
*/
public AirItineraryPricingInfoType createAirItineraryPricingInfoType() {
return new AirItineraryPricingInfoType();
}
/**
* Create an instance of {@link AirItineraryPricingInfoType.FareInfos }
*
* @return
* the new instance of {@link AirItineraryPricingInfoType.FareInfos }
*/
public AirItineraryPricingInfoType.FareInfos createAirItineraryPricingInfoTypeFareInfos() {
return new AirItineraryPricingInfoType.FareInfos();
}
/**
* Create an instance of {@link PricedItinerariesType }
*
* @return
* the new instance of {@link PricedItinerariesType }
*/
public PricedItinerariesType createPricedItinerariesType() {
return new PricedItinerariesType();
}
/**
* Create an instance of {@link OriginDestinationOptionType }
*
* @return
* the new instance of {@link OriginDestinationOptionType }
*/
public OriginDestinationOptionType createOriginDestinationOptionType() {
return new OriginDestinationOptionType();
}
/**
* Create an instance of {@link CabinAvailabilityType }
*
* @return
* the new instance of {@link CabinAvailabilityType }
*/
public CabinAvailabilityType createCabinAvailabilityType() {
return new CabinAvailabilityType();
}
/**
* Create an instance of {@link FulfillmentType }
*
* @return
* the new instance of {@link FulfillmentType }
*/
public FulfillmentType createFulfillmentType() {
return new FulfillmentType();
}
/**
* Create an instance of {@link FulfillmentType.PaymentDetails }
*
* @return
* the new instance of {@link FulfillmentType.PaymentDetails }
*/
public FulfillmentType.PaymentDetails createFulfillmentTypePaymentDetails() {
return new FulfillmentType.PaymentDetails();
}
/**
* Create an instance of {@link FlightLegType }
*
* @return
* the new instance of {@link FlightLegType }
*/
public FlightLegType createFlightLegType() {
return new FlightLegType();
}
/**
* Create an instance of {@link FareComponentType }
*
* @return
* the new instance of {@link FareComponentType }
*/
public FareComponentType createFareComponentType() {
return new FareComponentType();
}
/**
* Create an instance of {@link FareComponentType.PriceableUnit }
*
* @return
* the new instance of {@link FareComponentType.PriceableUnit }
*/
public FareComponentType.PriceableUnit createFareComponentTypePriceableUnit() {
return new FareComponentType.PriceableUnit();
}
/**
* Create an instance of {@link FareComponentType.PriceableUnit.FareComponentDetail }
*
* @return
* the new instance of {@link FareComponentType.PriceableUnit.FareComponentDetail }
*/
public FareComponentType.PriceableUnit.FareComponentDetail createFareComponentTypePriceableUnitFareComponentDetail() {
return new FareComponentType.PriceableUnit.FareComponentDetail();
}
/**
* Create an instance of {@link ETFareInfo }
*
* @return
* the new instance of {@link ETFareInfo }
*/
public ETFareInfo createETFareInfo() {
return new ETFareInfo();
}
/**
* Create an instance of {@link EMDType }
*
* @return
* the new instance of {@link EMDType }
*/
public EMDType createEMDType() {
return new EMDType();
}
/**
* Create an instance of {@link EMDType.TaxCouponInformation }
*
* @return
* the new instance of {@link EMDType.TaxCouponInformation }
*/
public EMDType.TaxCouponInformation createEMDTypeTaxCouponInformation() {
return new EMDType.TaxCouponInformation();
}
/**
* Create an instance of {@link EMDType.TaxCouponInformation.TicketDocument }
*
* @return
* the new instance of {@link EMDType.TaxCouponInformation.TicketDocument }
*/
public EMDType.TaxCouponInformation.TicketDocument createEMDTypeTaxCouponInformationTicketDocument() {
return new EMDType.TaxCouponInformation.TicketDocument();
}
/**
* Create an instance of {@link EMDType.TaxCouponInformation.TicketDocument.CouponNumber }
*
* @return
* the new instance of {@link EMDType.TaxCouponInformation.TicketDocument.CouponNumber }
*/
public EMDType.TaxCouponInformation.TicketDocument.CouponNumber createEMDTypeTaxCouponInformationTicketDocumentCouponNumber() {
return new EMDType.TaxCouponInformation.TicketDocument.CouponNumber();
}
/**
* Create an instance of {@link EMDType.ExchResidualFareComponent }
*
* @return
* the new instance of {@link EMDType.ExchResidualFareComponent }
*/
public EMDType.ExchResidualFareComponent createEMDTypeExchResidualFareComponent() {
return new EMDType.ExchResidualFareComponent();
}
/**
* Create an instance of {@link EMDType.CarrierFeeInfo }
*
* @return
* the new instance of {@link EMDType.CarrierFeeInfo }
*/
public EMDType.CarrierFeeInfo createEMDTypeCarrierFeeInfo() {
return new EMDType.CarrierFeeInfo();
}
/**
* Create an instance of {@link EMDType.CarrierFeeInfo.CarrierFee }
*
* @return
* the new instance of {@link EMDType.CarrierFeeInfo.CarrierFee }
*/
public EMDType.CarrierFeeInfo.CarrierFee createEMDTypeCarrierFeeInfoCarrierFee() {
return new EMDType.CarrierFeeInfo.CarrierFee();
}
/**
* Create an instance of {@link EMDType.TicketDocument }
*
* @return
* the new instance of {@link EMDType.TicketDocument }
*/
public EMDType.TicketDocument createEMDTypeTicketDocument() {
return new EMDType.TicketDocument();
}
/**
* Create an instance of {@link EMDType.TicketDocument.CouponInfo }
*
* @return
* the new instance of {@link EMDType.TicketDocument.CouponInfo }
*/
public EMDType.TicketDocument.CouponInfo createEMDTypeTicketDocumentCouponInfo() {
return new EMDType.TicketDocument.CouponInfo();
}
/**
* Create an instance of {@link EMDType.Taxes }
*
* @return
* the new instance of {@link EMDType.Taxes }
*/
public EMDType.Taxes createEMDTypeTaxes() {
return new EMDType.Taxes();
}
/**
* Create an instance of {@link AuthorizationType }
*
* @return
* the new instance of {@link AuthorizationType }
*/
public AuthorizationType createAuthorizationType() {
return new AuthorizationType();
}
/**
* Create an instance of {@link AuthorizationType.AccountAuthorization }
*
* @return
* the new instance of {@link AuthorizationType.AccountAuthorization }
*/
public AuthorizationType.AccountAuthorization createAuthorizationTypeAccountAuthorization() {
return new AuthorizationType.AccountAuthorization();
}
/**
* Create an instance of {@link AirReservationType }
*
* @return
* the new instance of {@link AirReservationType }
*/
public AirReservationType createAirReservationType() {
return new AirReservationType();
}
/**
* Create an instance of {@link AirReservationType.PricingOverview }
*
* @return
* the new instance of {@link AirReservationType.PricingOverview }
*/
public AirReservationType.PricingOverview createAirReservationTypePricingOverview() {
return new AirReservationType.PricingOverview();
}
/**
* Create an instance of {@link AirReservationType.Queues }
*
* @return
* the new instance of {@link AirReservationType.Queues }
*/
public AirReservationType.Queues createAirReservationTypeQueues() {
return new AirReservationType.Queues();
}
/**
* Create an instance of {@link AirItineraryType }
*
* @return
* the new instance of {@link AirItineraryType }
*/
public AirItineraryType createAirItineraryType() {
return new AirItineraryType();
}
/**
* Create an instance of {@link AirItineraryType.OriginDestinationOptions }
*
* @return
* the new instance of {@link AirItineraryType.OriginDestinationOptions }
*/
public AirItineraryType.OriginDestinationOptions createAirItineraryTypeOriginDestinationOptions() {
return new AirItineraryType.OriginDestinationOptions();
}
/**
* Create an instance of {@link VideoItemsType }
*
* @return
* the new instance of {@link VideoItemsType }
*/
public VideoItemsType createVideoItemsType() {
return new VideoItemsType();
}
/**
* Create an instance of {@link VideoDescriptionType }
*
* @return
* the new instance of {@link VideoDescriptionType }
*/
public VideoDescriptionType createVideoDescriptionType() {
return new VideoDescriptionType();
}
/**
* Create an instance of {@link TravelerRPHs }
*
* @return
* the new instance of {@link TravelerRPHs }
*/
public TravelerRPHs createTravelerRPHs() {
return new TravelerRPHs();
}
/**
* Create an instance of {@link TransportationType }
*
* @return
* the new instance of {@link TransportationType }
*/
public TransportationType createTransportationType() {
return new TransportationType();
}
/**
* Create an instance of {@link TextItemsType }
*
* @return
* the new instance of {@link TextItemsType }
*/
public TextItemsType createTextItemsType() {
return new TextItemsType();
}
/**
* Create an instance of {@link TextDescriptionType }
*
* @return
* the new instance of {@link TextDescriptionType }
*/
public TextDescriptionType createTextDescriptionType() {
return new TextDescriptionType();
}
/**
* Create an instance of {@link SpecialRequestType }
*
* @return
* the new instance of {@link SpecialRequestType }
*/
public SpecialRequestType createSpecialRequestType() {
return new SpecialRequestType();
}
/**
* Create an instance of {@link SourceType }
*
* @return
* the new instance of {@link SourceType }
*/
public SourceType createSourceType() {
return new SourceType();
}
/**
* Create an instance of {@link RestaurantType }
*
* @return
* the new instance of {@link RestaurantType }
*/
public RestaurantType createRestaurantType() {
return new RestaurantType();
}
/**
* Create an instance of {@link RestaurantType.CuisineCodes }
*
* @return
* the new instance of {@link RestaurantType.CuisineCodes }
*/
public RestaurantType.CuisineCodes createRestaurantTypeCuisineCodes() {
return new RestaurantType.CuisineCodes();
}
/**
* Create an instance of {@link RestaurantType.InfoCodes }
*
* @return
* the new instance of {@link RestaurantType.InfoCodes }
*/
public RestaurantType.InfoCodes createRestaurantTypeInfoCodes() {
return new RestaurantType.InfoCodes();
}
/**
* Create an instance of {@link RecipientInfosType }
*
* @return
* the new instance of {@link RecipientInfosType }
*/
public RecipientInfosType createRecipientInfosType() {
return new RecipientInfosType();
}
/**
* Create an instance of {@link RecipientInfosType.RecipientInfo }
*
* @return
* the new instance of {@link RecipientInfosType.RecipientInfo }
*/
public RecipientInfosType.RecipientInfo createRecipientInfosTypeRecipientInfo() {
return new RecipientInfosType.RecipientInfo();
}
/**
* Create an instance of {@link PaymentCardType }
*
* @return
* the new instance of {@link PaymentCardType }
*/
public PaymentCardType createPaymentCardType() {
return new PaymentCardType();
}
/**
* Create an instance of {@link OriginDestinationInformationType }
*
* @return
* the new instance of {@link OriginDestinationInformationType }
*/
public OriginDestinationInformationType createOriginDestinationInformationType() {
return new OriginDestinationInformationType();
}
/**
* Create an instance of {@link OrdersType }
*
* @return
* the new instance of {@link OrdersType }
*/
public OrdersType createOrdersType() {
return new OrdersType();
}
/**
* Create an instance of {@link OrdersType.Order }
*
* @return
* the new instance of {@link OrdersType.Order }
*/
public OrdersType.Order createOrdersTypeOrder() {
return new OrdersType.Order();
}
/**
* Create an instance of {@link OrdersType.Order.Products }
*
* @return
* the new instance of {@link OrdersType.Order.Products }
*/
public OrdersType.Order.Products createOrdersTypeOrderProducts() {
return new OrdersType.Order.Products();
}
/**
* Create an instance of {@link OperationSchedulesPlusChargeType }
*
* @return
* the new instance of {@link OperationSchedulesPlusChargeType }
*/
public OperationSchedulesPlusChargeType createOperationSchedulesPlusChargeType() {
return new OperationSchedulesPlusChargeType();
}
/**
* Create an instance of {@link OperationScheduleType }
*
* @return
* the new instance of {@link OperationScheduleType }
*/
public OperationScheduleType createOperationScheduleType() {
return new OperationScheduleType();
}
/**
* Create an instance of {@link OperationScheduleType.OperationTimes }
*
* @return
* the new instance of {@link OperationScheduleType.OperationTimes }
*/
public OperationScheduleType.OperationTimes createOperationScheduleTypeOperationTimes() {
return new OperationScheduleType.OperationTimes();
}
/**
* Create an instance of {@link ImageItemsType }
*
* @return
* the new instance of {@link ImageItemsType }
*/
public ImageItemsType createImageItemsType() {
return new ImageItemsType();
}
/**
* Create an instance of {@link ImageDescriptionType }
*
* @return
* the new instance of {@link ImageDescriptionType }
*/
public ImageDescriptionType createImageDescriptionType() {
return new ImageDescriptionType();
}
/**
* Create an instance of {@link DonationType }
*
* @return
* the new instance of {@link DonationType }
*/
public DonationType createDonationType() {
return new DonationType();
}
/**
* Create an instance of {@link DonationType.DonorInfo }
*
* @return
* the new instance of {@link DonationType.DonorInfo }
*/
public DonationType.DonorInfo createDonationTypeDonorInfo() {
return new DonationType.DonorInfo();
}
/**
* Create an instance of {@link ConnectionType }
*
* @return
* the new instance of {@link ConnectionType }
*/
public ConnectionType createConnectionType() {
return new ConnectionType();
}
/**
* Create an instance of {@link CommissionType }
*
* @return
* the new instance of {@link CommissionType }
*/
public CommissionType createCommissionType() {
return new CommissionType();
}
/**
* Create an instance of {@link CommentType }
*
* @return
* the new instance of {@link CommentType }
*/
public CommentType createCommentType() {
return new CommentType();
}
/**
* Create an instance of {@link CancelInfoRSType }
*
* @return
* the new instance of {@link CancelInfoRSType }
*/
public CancelInfoRSType createCancelInfoRSType() {
return new CancelInfoRSType();
}
/**
* Create an instance of {@link AcceptablePaymentCardsInfoType }
*
* @return
* the new instance of {@link AcceptablePaymentCardsInfoType }
*/
public AcceptablePaymentCardsInfoType createAcceptablePaymentCardsInfoType() {
return new AcceptablePaymentCardsInfoType();
}
/**
* Create an instance of {@link AcceptablePaymentCardsInfoType.AcceptablePaymentCards }
*
* @return
* the new instance of {@link AcceptablePaymentCardsInfoType.AcceptablePaymentCards }
*/
public AcceptablePaymentCardsInfoType.AcceptablePaymentCards createAcceptablePaymentCardsInfoTypeAcceptablePaymentCards() {
return new AcceptablePaymentCardsInfoType.AcceptablePaymentCards();
}
/**
* Create an instance of {@link OTAHotelRatePlanRQ.RatePlans }
*
* @return
* the new instance of {@link OTAHotelRatePlanRQ.RatePlans }
*/
public OTAHotelRatePlanRQ.RatePlans createOTAHotelRatePlanRQRatePlans() {
return new OTAHotelRatePlanRQ.RatePlans();
}
/**
* Create an instance of {@link OTAHotelRatePlanRQ.RatePlans.RatePlan }
*
* @return
* the new instance of {@link OTAHotelRatePlanRQ.RatePlans.RatePlan }
*/
public OTAHotelRatePlanRQ.RatePlans.RatePlan createOTAHotelRatePlanRQRatePlansRatePlan() {
return new OTAHotelRatePlanRQ.RatePlans.RatePlan();
}
/**
* Create an instance of {@link OTAHotelRatePlanRQ.RatePlans.RatePlan.Offers }
*
* @return
* the new instance of {@link OTAHotelRatePlanRQ.RatePlans.RatePlan.Offers }
*/
public OTAHotelRatePlanRQ.RatePlans.RatePlan.Offers createOTAHotelRatePlanRQRatePlansRatePlanOffers() {
return new OTAHotelRatePlanRQ.RatePlans.RatePlan.Offers();
}
/**
* Create an instance of {@link OTAHotelAvailGetRQ.HotelAvailRequests }
*
* @return
* the new instance of {@link OTAHotelAvailGetRQ.HotelAvailRequests }
*/
public OTAHotelAvailGetRQ.HotelAvailRequests createOTAHotelAvailGetRQHotelAvailRequests() {
return new OTAHotelAvailGetRQ.HotelAvailRequests();
}
/**
* Create an instance of {@link OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest }
*
* @return
* the new instance of {@link OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest }
*/
public OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest createOTAHotelAvailGetRQHotelAvailRequestsHotelAvailRequest() {
return new OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest();
}
/**
* Create an instance of {@link OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.LengthsOfStayCandidates }
*
* @return
* the new instance of {@link OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.LengthsOfStayCandidates }
*/
public OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.LengthsOfStayCandidates createOTAHotelAvailGetRQHotelAvailRequestsHotelAvailRequestLengthsOfStayCandidates() {
return new OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.LengthsOfStayCandidates();
}
/**
* Create an instance of {@link OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.RestrictionStatusCandidates }
*
* @return
* the new instance of {@link OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.RestrictionStatusCandidates }
*/
public OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.RestrictionStatusCandidates createOTAHotelAvailGetRQHotelAvailRequestsHotelAvailRequestRestrictionStatusCandidates() {
return new OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.RestrictionStatusCandidates();
}
/**
* Create an instance of {@link OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.Offers }
*
* @return
* the new instance of {@link OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.Offers }
*/
public OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.Offers createOTAHotelAvailGetRQHotelAvailRequestsHotelAvailRequestOffers() {
return new OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.Offers();
}
/**
* Create an instance of {@link OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.RoomTypeCandidates }
*
* @return
* the new instance of {@link OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.RoomTypeCandidates }
*/
public OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.RoomTypeCandidates createOTAHotelAvailGetRQHotelAvailRequestsHotelAvailRequestRoomTypeCandidates() {
return new OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.RoomTypeCandidates();
}
/**
* Create an instance of {@link HotelReservationsType }
*
* @return
* the new instance of {@link HotelReservationsType }
*/
public HotelReservationsType createHotelReservationsType() {
return new HotelReservationsType();
}
/**
* Create an instance of {@link HotelReservationsType.HotelReservation }
*
* @return
* the new instance of {@link HotelReservationsType.HotelReservation }
*/
public HotelReservationsType.HotelReservation createHotelReservationsTypeHotelReservation() {
return new HotelReservationsType.HotelReservation();
}
/**
* Create an instance of {@link OTAHotelAvailRS.CurrencyConversions }
*
* @return
* the new instance of {@link OTAHotelAvailRS.CurrencyConversions }
*/
public OTAHotelAvailRS.CurrencyConversions createOTAHotelAvailRSCurrencyConversions() {
return new OTAHotelAvailRS.CurrencyConversions();
}
/**
* Create an instance of {@link AreasType }
*
* @return
* the new instance of {@link AreasType }
*/
public AreasType createAreasType() {
return new AreasType();
}
/**
* Create an instance of {@link OTAHotelAvailRS.RoomStays }
*
* @return
* the new instance of {@link OTAHotelAvailRS.RoomStays }
*/
public OTAHotelAvailRS.RoomStays createOTAHotelAvailRSRoomStays() {
return new OTAHotelAvailRS.RoomStays();
}
/**
* Create an instance of {@link OTAHotelAvailRS.RoomStays.RoomStay }
*
* @return
* the new instance of {@link OTAHotelAvailRS.RoomStays.RoomStay }
*/
public OTAHotelAvailRS.RoomStays.RoomStay createOTAHotelAvailRSRoomStaysRoomStay() {
return new OTAHotelAvailRS.RoomStays.RoomStay();
}
/**
* Create an instance of {@link OTAHotelAvailRS.HotelStays }
*
* @return
* the new instance of {@link OTAHotelAvailRS.HotelStays }
*/
public OTAHotelAvailRS.HotelStays createOTAHotelAvailRSHotelStays() {
return new OTAHotelAvailRS.HotelStays();
}
/**
* Create an instance of {@link OTAHotelAvailRS.HotelStays.HotelStay }
*
* @return
* the new instance of {@link OTAHotelAvailRS.HotelStays.HotelStay }
*/
public OTAHotelAvailRS.HotelStays.HotelStay createOTAHotelAvailRSHotelStaysHotelStay() {
return new OTAHotelAvailRS.HotelStays.HotelStay();
}
/**
* Create an instance of {@link OTAHotelAvailRS.HotelStays.HotelStay.Availability }
*
* @return
* the new instance of {@link OTAHotelAvailRS.HotelStays.HotelStay.Availability }
*/
public OTAHotelAvailRS.HotelStays.HotelStay.Availability createOTAHotelAvailRSHotelStaysHotelStayAvailability() {
return new OTAHotelAvailRS.HotelStays.HotelStay.Availability();
}
/**
* Create an instance of {@link ProfilesType }
*
* @return
* the new instance of {@link ProfilesType }
*/
public ProfilesType createProfilesType() {
return new ProfilesType();
}
/**
* Create an instance of {@link HotelReservationIDsType }
*
* @return
* the new instance of {@link HotelReservationIDsType }
*/
public HotelReservationIDsType createHotelReservationIDsType() {
return new HotelReservationIDsType();
}
/**
* Create an instance of {@link VehicleReservationType }
*
* @return
* the new instance of {@link VehicleReservationType }
*/
public VehicleReservationType createVehicleReservationType() {
return new VehicleReservationType();
}
/**
* Create an instance of {@link TPAExtensionsType }
*
* @return
* the new instance of {@link TPAExtensionsType }
*/
public TPAExtensionsType createTPAExtensionsType() {
return new TPAExtensionsType();
}
/**
* Create an instance of {@link POSType }
*
* @return
* the new instance of {@link POSType }
*/
public POSType createPOSType() {
return new POSType();
}
/**
* Create an instance of {@link OTAHotelAvailRQ.AvailRequestSegments }
*
* @return
* the new instance of {@link OTAHotelAvailRQ.AvailRequestSegments }
*/
public OTAHotelAvailRQ.AvailRequestSegments createOTAHotelAvailRQAvailRequestSegments() {
return new OTAHotelAvailRQ.AvailRequestSegments();
}
/**
* Create an instance of {@link SuccessType }
*
* @return
* the new instance of {@link SuccessType }
*/
public SuccessType createSuccessType() {
return new SuccessType();
}
/**
* Create an instance of {@link WarningsType }
*
* @return
* the new instance of {@link WarningsType }
*/
public WarningsType createWarningsType() {
return new WarningsType();
}
/**
* Create an instance of {@link ServicesType }
*
* @return
* the new instance of {@link ServicesType }
*/
public ServicesType createServicesType() {
return new ServicesType();
}
/**
* Create an instance of {@link OTAHotelAvailRS.Criteria }
*
* @return
* the new instance of {@link OTAHotelAvailRS.Criteria }
*/
public OTAHotelAvailRS.Criteria createOTAHotelAvailRSCriteria() {
return new OTAHotelAvailRS.Criteria();
}
/**
* Create an instance of {@link OTAHotelAvailRS.RebatePrograms }
*
* @return
* the new instance of {@link OTAHotelAvailRS.RebatePrograms }
*/
public OTAHotelAvailRS.RebatePrograms createOTAHotelAvailRSRebatePrograms() {
return new OTAHotelAvailRS.RebatePrograms();
}
/**
* Create an instance of {@link ErrorsType }
*
* @return
* the new instance of {@link ErrorsType }
*/
public ErrorsType createErrorsType() {
return new ErrorsType();
}
/**
* Create an instance of {@link UniqueIDType }
*
* @return
* the new instance of {@link UniqueIDType }
*/
public UniqueIDType createUniqueIDType() {
return new UniqueIDType();
}
/**
* Create an instance of {@link OTAHotelAvailNotifRQ.AvailStatusMessages }
*
* @return
* the new instance of {@link OTAHotelAvailNotifRQ.AvailStatusMessages }
*/
public OTAHotelAvailNotifRQ.AvailStatusMessages createOTAHotelAvailNotifRQAvailStatusMessages() {
return new OTAHotelAvailNotifRQ.AvailStatusMessages();
}
/**
* Create an instance of {@link MessageAcknowledgementType }
*
* @return
* the new instance of {@link MessageAcknowledgementType }
*/
public MessageAcknowledgementType createMessageAcknowledgementType() {
return new MessageAcknowledgementType();
}
/**
* Create an instance of {@link OTAHotelRateAmountNotifRQ.RateAmountMessages }
*
* @return
* the new instance of {@link OTAHotelRateAmountNotifRQ.RateAmountMessages }
*/
public OTAHotelRateAmountNotifRQ.RateAmountMessages createOTAHotelRateAmountNotifRQRateAmountMessages() {
return new OTAHotelRateAmountNotifRQ.RateAmountMessages();
}
/**
* Create an instance of {@link OTAHotelResNotifRQ }
*
* @return
* the new instance of {@link OTAHotelResNotifRQ }
*/
public OTAHotelResNotifRQ createOTAHotelResNotifRQ() {
return new OTAHotelResNotifRQ();
}
/**
* Create an instance of {@link OTAHotelResNotifRS }
*
* @return
* the new instance of {@link OTAHotelResNotifRS }
*/
public OTAHotelResNotifRS createOTAHotelResNotifRS() {
return new OTAHotelResNotifRS();
}
/**
* Create an instance of {@link OTAPingRQ }
*
* @return
* the new instance of {@link OTAPingRQ }
*/
public OTAPingRQ createOTAPingRQ() {
return new OTAPingRQ();
}
/**
* Create an instance of {@link OTAPingRS }
*
* @return
* the new instance of {@link OTAPingRS }
*/
public OTAPingRS createOTAPingRS() {
return new OTAPingRS();
}
/**
* Create an instance of {@link OTAHotelAvailGetRS.AvailStatusMessages }
*
* @return
* the new instance of {@link OTAHotelAvailGetRS.AvailStatusMessages }
*/
public OTAHotelAvailGetRS.AvailStatusMessages createOTAHotelAvailGetRSAvailStatusMessages() {
return new OTAHotelAvailGetRS.AvailStatusMessages();
}
/**
* Create an instance of {@link OTAHotelRatePlanRS.RatePlans }
*
* @return
* the new instance of {@link OTAHotelRatePlanRS.RatePlans }
*/
public OTAHotelRatePlanRS.RatePlans createOTAHotelRatePlanRSRatePlans() {
return new OTAHotelRatePlanRS.RatePlans();
}
/**
* Create an instance of {@link OTAHotelRatePlanRS.Offers }
*
* @return
* the new instance of {@link OTAHotelRatePlanRS.Offers }
*/
public OTAHotelRatePlanRS.Offers createOTAHotelRatePlanRSOffers() {
return new OTAHotelRatePlanRS.Offers();
}
/**
* Create an instance of {@link AcceptedPaymentsType }
*
* @return
* the new instance of {@link AcceptedPaymentsType }
*/
public AcceptedPaymentsType createAcceptedPaymentsType() {
return new AcceptedPaymentsType();
}
/**
* Create an instance of {@link AddressInfoType }
*
* @return
* the new instance of {@link AddressInfoType }
*/
public AddressInfoType createAddressInfoType() {
return new AddressInfoType();
}
/**
* Create an instance of {@link BankAcctType }
*
* @return
* the new instance of {@link BankAcctType }
*/
public BankAcctType createBankAcctType() {
return new BankAcctType();
}
/**
* Create an instance of {@link BlackoutDateType }
*
* @return
* the new instance of {@link BlackoutDateType }
*/
public BlackoutDateType createBlackoutDateType() {
return new BlackoutDateType();
}
/**
* Create an instance of {@link CancelInfoRQType }
*
* @return
* the new instance of {@link CancelInfoRQType }
*/
public CancelInfoRQType createCancelInfoRQType() {
return new CancelInfoRQType();
}
/**
* Create an instance of {@link CancelRuleType }
*
* @return
* the new instance of {@link CancelRuleType }
*/
public CancelRuleType createCancelRuleType() {
return new CancelRuleType();
}
/**
* Create an instance of {@link CompanyNameType }
*
* @return
* the new instance of {@link CompanyNameType }
*/
public CompanyNameType createCompanyNameType() {
return new CompanyNameType();
}
/**
* Create an instance of {@link CountryNameType }
*
* @return
* the new instance of {@link CountryNameType }
*/
public CountryNameType createCountryNameType() {
return new CountryNameType();
}
/**
* Create an instance of {@link EmailType }
*
* @return
* the new instance of {@link EmailType }
*/
public EmailType createEmailType() {
return new EmailType();
}
/**
* Create an instance of {@link EmployeeInfoType }
*
* @return
* the new instance of {@link EmployeeInfoType }
*/
public EmployeeInfoType createEmployeeInfoType() {
return new EmployeeInfoType();
}
/**
* Create an instance of {@link EquipmentType }
*
* @return
* the new instance of {@link EquipmentType }
*/
public EquipmentType createEquipmentType() {
return new EquipmentType();
}
/**
* Create an instance of {@link ErrorType }
*
* @return
* the new instance of {@link ErrorType }
*/
public ErrorType createErrorType() {
return new ErrorType();
}
/**
* Create an instance of {@link FeeType }
*
* @return
* the new instance of {@link FeeType }
*/
public FeeType createFeeType() {
return new FeeType();
}
/**
* Create an instance of {@link FeesType }
*
* @return
* the new instance of {@link FeesType }
*/
public FeesType createFeesType() {
return new FeesType();
}
/**
* Create an instance of {@link FormattedTextSubSectionType }
*
* @return
* the new instance of {@link FormattedTextSubSectionType }
*/
public FormattedTextSubSectionType createFormattedTextSubSectionType() {
return new FormattedTextSubSectionType();
}
/**
* Create an instance of {@link FormattedTextTextType }
*
* @return
* the new instance of {@link FormattedTextTextType }
*/
public FormattedTextTextType createFormattedTextTextType() {
return new FormattedTextTextType();
}
/**
* Create an instance of {@link FormattedTextType }
*
* @return
* the new instance of {@link FormattedTextType }
*/
public FormattedTextType createFormattedTextType() {
return new FormattedTextType();
}
/**
* Create an instance of {@link FreeTextType }
*
* @return
* the new instance of {@link FreeTextType }
*/
public FreeTextType createFreeTextType() {
return new FreeTextType();
}
/**
* Create an instance of {@link ImageItemType }
*
* @return
* the new instance of {@link ImageItemType }
*/
public ImageItemType createImageItemType() {
return new ImageItemType();
}
/**
* Create an instance of {@link LocationGeneralType }
*
* @return
* the new instance of {@link LocationGeneralType }
*/
public LocationGeneralType createLocationGeneralType() {
return new LocationGeneralType();
}
/**
* Create an instance of {@link LocationType }
*
* @return
* the new instance of {@link LocationType }
*/
public LocationType createLocationType() {
return new LocationType();
}
/**
* Create an instance of {@link MonetaryRuleType }
*
* @return
* the new instance of {@link MonetaryRuleType }
*/
public MonetaryRuleType createMonetaryRuleType() {
return new MonetaryRuleType();
}
/**
* Create an instance of {@link MultimediaDescriptionsType }
*
* @return
* the new instance of {@link MultimediaDescriptionsType }
*/
public MultimediaDescriptionsType createMultimediaDescriptionsType() {
return new MultimediaDescriptionsType();
}
/**
* Create an instance of {@link MultimediaDescriptionType }
*
* @return
* the new instance of {@link MultimediaDescriptionType }
*/
public MultimediaDescriptionType createMultimediaDescriptionType() {
return new MultimediaDescriptionType();
}
/**
* Create an instance of {@link OperatingAirlineType }
*
* @return
* the new instance of {@link OperatingAirlineType }
*/
public OperatingAirlineType createOperatingAirlineType() {
return new OperatingAirlineType();
}
/**
* Create an instance of {@link OperationSchedulesType }
*
* @return
* the new instance of {@link OperationSchedulesType }
*/
public OperationSchedulesType createOperationSchedulesType() {
return new OperationSchedulesType();
}
/**
* Create an instance of {@link OperationSchedulePlusChargeType }
*
* @return
* the new instance of {@link OperationSchedulePlusChargeType }
*/
public OperationSchedulePlusChargeType createOperationSchedulePlusChargeType() {
return new OperationSchedulePlusChargeType();
}
/**
* Create an instance of {@link PaymentRulesType }
*
* @return
* the new instance of {@link PaymentRulesType }
*/
public PaymentRulesType createPaymentRulesType() {
return new PaymentRulesType();
}
/**
* Create an instance of {@link RebateType }
*
* @return
* the new instance of {@link RebateType }
*/
public RebateType createRebateType() {
return new RebateType();
}
/**
* Create an instance of {@link ReferencePlaceHolderType }
*
* @return
* the new instance of {@link ReferencePlaceHolderType }
*/
public ReferencePlaceHolderType createReferencePlaceHolderType() {
return new ReferencePlaceHolderType();
}
/**
* Create an instance of {@link RelatedTravelerType }
*
* @return
* the new instance of {@link RelatedTravelerType }
*/
public RelatedTravelerType createRelatedTravelerType() {
return new RelatedTravelerType();
}
/**
* Create an instance of {@link RelativePositionType }
*
* @return
* the new instance of {@link RelativePositionType }
*/
public RelativePositionType createRelativePositionType() {
return new RelativePositionType();
}
/**
* Create an instance of {@link StateProvType }
*
* @return
* the new instance of {@link StateProvType }
*/
public StateProvType createStateProvType() {
return new StateProvType();
}
/**
* Create an instance of {@link StreetNmbrType }
*
* @return
* the new instance of {@link StreetNmbrType }
*/
public StreetNmbrType createStreetNmbrType() {
return new StreetNmbrType();
}
/**
* Create an instance of {@link TaxType }
*
* @return
* the new instance of {@link TaxType }
*/
public TaxType createTaxType() {
return new TaxType();
}
/**
* Create an instance of {@link TaxesType }
*
* @return
* the new instance of {@link TaxesType }
*/
public TaxesType createTaxesType() {
return new TaxesType();
}
/**
* Create an instance of {@link TimeDurationType }
*
* @return
* the new instance of {@link TimeDurationType }
*/
public TimeDurationType createTimeDurationType() {
return new TimeDurationType();
}
/**
* Create an instance of {@link TimeInstantType }
*
* @return
* the new instance of {@link TimeInstantType }
*/
public TimeInstantType createTimeInstantType() {
return new TimeInstantType();
}
/**
* Create an instance of {@link TotalType }
*
* @return
* the new instance of {@link TotalType }
*/
public TotalType createTotalType() {
return new TotalType();
}
/**
* Create an instance of {@link TransportationsType }
*
* @return
* the new instance of {@link TransportationsType }
*/
public TransportationsType createTransportationsType() {
return new TransportationsType();
}
/**
* Create an instance of {@link TravelDateTimeType }
*
* @return
* the new instance of {@link TravelDateTimeType }
*/
public TravelDateTimeType createTravelDateTimeType() {
return new TravelDateTimeType();
}
/**
* Create an instance of {@link URLType }
*
* @return
* the new instance of {@link URLType }
*/
public URLType createURLType() {
return new URLType();
}
/**
* Create an instance of {@link VendorMessageType }
*
* @return
* the new instance of {@link VendorMessageType }
*/
public VendorMessageType createVendorMessageType() {
return new VendorMessageType();
}
/**
* Create an instance of {@link VendorMessagesType }
*
* @return
* the new instance of {@link VendorMessagesType }
*/
public VendorMessagesType createVendorMessagesType() {
return new VendorMessagesType();
}
/**
* Create an instance of {@link VideoItemType }
*
* @return
* the new instance of {@link VideoItemType }
*/
public VideoItemType createVideoItemType() {
return new VideoItemType();
}
/**
* Create an instance of {@link WarningType }
*
* @return
* the new instance of {@link WarningType }
*/
public WarningType createWarningType() {
return new WarningType();
}
/**
* Create an instance of {@link WrittenConfInstType }
*
* @return
* the new instance of {@link WrittenConfInstType }
*/
public WrittenConfInstType createWrittenConfInstType() {
return new WrittenConfInstType();
}
/**
* Create an instance of {@link AirFeeType }
*
* @return
* the new instance of {@link AirFeeType }
*/
public AirFeeType createAirFeeType() {
return new AirFeeType();
}
/**
* Create an instance of {@link AirTaxType }
*
* @return
* the new instance of {@link AirTaxType }
*/
public AirTaxType createAirTaxType() {
return new AirTaxType();
}
/**
* Create an instance of {@link BookingPriceInfoType }
*
* @return
* the new instance of {@link BookingPriceInfoType }
*/
public BookingPriceInfoType createBookingPriceInfoType() {
return new BookingPriceInfoType();
}
/**
* Create an instance of {@link FareBasisCodeType }
*
* @return
* the new instance of {@link FareBasisCodeType }
*/
public FareBasisCodeType createFareBasisCodeType() {
return new FareBasisCodeType();
}
/**
* Create an instance of {@link MarketingCabinType }
*
* @return
* the new instance of {@link MarketingCabinType }
*/
public MarketingCabinType createMarketingCabinType() {
return new MarketingCabinType();
}
/**
* Create an instance of {@link PassengerTypeQuantityType }
*
* @return
* the new instance of {@link PassengerTypeQuantityType }
*/
public PassengerTypeQuantityType createPassengerTypeQuantityType() {
return new PassengerTypeQuantityType();
}
/**
* Create an instance of {@link SeatRequestType }
*
* @return
* the new instance of {@link SeatRequestType }
*/
public SeatRequestType createSeatRequestType() {
return new SeatRequestType();
}
/**
* Create an instance of {@link SpecialServiceRequestType }
*
* @return
* the new instance of {@link SpecialServiceRequestType }
*/
public SpecialServiceRequestType createSpecialServiceRequestType() {
return new SpecialServiceRequestType();
}
/**
* Create an instance of {@link TicketingInfoRSType }
*
* @return
* the new instance of {@link TicketingInfoRSType }
*/
public TicketingInfoRSType createTicketingInfoRSType() {
return new TicketingInfoRSType();
}
/**
* Create an instance of {@link TravelerInformationType }
*
* @return
* the new instance of {@link TravelerInformationType }
*/
public TravelerInformationType createTravelerInformationType() {
return new TravelerInformationType();
}
/**
* Create an instance of {@link AddressPrefType }
*
* @return
* the new instance of {@link AddressPrefType }
*/
public AddressPrefType createAddressPrefType() {
return new AddressPrefType();
}
/**
* Create an instance of {@link CompanyNamePrefType }
*
* @return
* the new instance of {@link CompanyNamePrefType }
*/
public CompanyNamePrefType createCompanyNamePrefType() {
return new CompanyNamePrefType();
}
/**
* Create an instance of {@link InsurancePrefType }
*
* @return
* the new instance of {@link InsurancePrefType }
*/
public InsurancePrefType createInsurancePrefType() {
return new InsurancePrefType();
}
/**
* Create an instance of {@link InterestPrefType }
*
* @return
* the new instance of {@link InterestPrefType }
*/
public InterestPrefType createInterestPrefType() {
return new InterestPrefType();
}
/**
* Create an instance of {@link LoyaltyPrefType }
*
* @return
* the new instance of {@link LoyaltyPrefType }
*/
public LoyaltyPrefType createLoyaltyPrefType() {
return new LoyaltyPrefType();
}
/**
* Create an instance of {@link MealPrefType }
*
* @return
* the new instance of {@link MealPrefType }
*/
public MealPrefType createMealPrefType() {
return new MealPrefType();
}
/**
* Create an instance of {@link MediaEntertainPrefType }
*
* @return
* the new instance of {@link MediaEntertainPrefType }
*/
public MediaEntertainPrefType createMediaEntertainPrefType() {
return new MediaEntertainPrefType();
}
/**
* Create an instance of {@link NamePrefType }
*
* @return
* the new instance of {@link NamePrefType }
*/
public NamePrefType createNamePrefType() {
return new NamePrefType();
}
/**
* Create an instance of {@link OtherSrvcPrefType }
*
* @return
* the new instance of {@link OtherSrvcPrefType }
*/
public OtherSrvcPrefType createOtherSrvcPrefType() {
return new OtherSrvcPrefType();
}
/**
* Create an instance of {@link PaymentFormPrefType }
*
* @return
* the new instance of {@link PaymentFormPrefType }
*/
public PaymentFormPrefType createPaymentFormPrefType() {
return new PaymentFormPrefType();
}
/**
* Create an instance of {@link PetInfoPrefType }
*
* @return
* the new instance of {@link PetInfoPrefType }
*/
public PetInfoPrefType createPetInfoPrefType() {
return new PetInfoPrefType();
}
/**
* Create an instance of {@link RelatedTravelerPrefType }
*
* @return
* the new instance of {@link RelatedTravelerPrefType }
*/
public RelatedTravelerPrefType createRelatedTravelerPrefType() {
return new RelatedTravelerPrefType();
}
/**
* Create an instance of {@link SeatingPrefType }
*
* @return
* the new instance of {@link SeatingPrefType }
*/
public SeatingPrefType createSeatingPrefType() {
return new SeatingPrefType();
}
/**
* Create an instance of {@link SpecRequestPrefType }
*
* @return
* the new instance of {@link SpecRequestPrefType }
*/
public SpecRequestPrefType createSpecRequestPrefType() {
return new SpecRequestPrefType();
}
/**
* Create an instance of {@link TicketDistribPrefType }
*
* @return
* the new instance of {@link TicketDistribPrefType }
*/
public TicketDistribPrefType createTicketDistribPrefType() {
return new TicketDistribPrefType();
}
/**
* Create an instance of {@link AirportPrefType }
*
* @return
* the new instance of {@link AirportPrefType }
*/
public AirportPrefType createAirportPrefType() {
return new AirportPrefType();
}
/**
* Create an instance of {@link EquipmentTypePref }
*
* @return
* the new instance of {@link EquipmentTypePref }
*/
public EquipmentTypePref createEquipmentTypePref() {
return new EquipmentTypePref();
}
/**
* Create an instance of {@link BedTypePrefType }
*
* @return
* the new instance of {@link BedTypePrefType }
*/
public BedTypePrefType createBedTypePrefType() {
return new BedTypePrefType();
}
/**
* Create an instance of {@link BusinessSrvcPrefType }
*
* @return
* the new instance of {@link BusinessSrvcPrefType }
*/
public BusinessSrvcPrefType createBusinessSrvcPrefType() {
return new BusinessSrvcPrefType();
}
/**
* Create an instance of {@link FoodSrvcPrefType }
*
* @return
* the new instance of {@link FoodSrvcPrefType }
*/
public FoodSrvcPrefType createFoodSrvcPrefType() {
return new FoodSrvcPrefType();
}
/**
* Create an instance of {@link HotelPrefType }
*
* @return
* the new instance of {@link HotelPrefType }
*/
public HotelPrefType createHotelPrefType() {
return new HotelPrefType();
}
/**
* Create an instance of {@link PersonalSrvcPrefType }
*
* @return
* the new instance of {@link PersonalSrvcPrefType }
*/
public PersonalSrvcPrefType createPersonalSrvcPrefType() {
return new PersonalSrvcPrefType();
}
/**
* Create an instance of {@link PhysChallFeaturePrefType }
*
* @return
* the new instance of {@link PhysChallFeaturePrefType }
*/
public PhysChallFeaturePrefType createPhysChallFeaturePrefType() {
return new PhysChallFeaturePrefType();
}
/**
* Create an instance of {@link PropertyAmenityPrefType }
*
* @return
* the new instance of {@link PropertyAmenityPrefType }
*/
public PropertyAmenityPrefType createPropertyAmenityPrefType() {
return new PropertyAmenityPrefType();
}
/**
* Create an instance of {@link PropertyClassPrefType }
*
* @return
* the new instance of {@link PropertyClassPrefType }
*/
public PropertyClassPrefType createPropertyClassPrefType() {
return new PropertyClassPrefType();
}
/**
* Create an instance of {@link PropertyLocationPrefType }
*
* @return
* the new instance of {@link PropertyLocationPrefType }
*/
public PropertyLocationPrefType createPropertyLocationPrefType() {
return new PropertyLocationPrefType();
}
/**
* Create an instance of {@link PropertyNamePrefType }
*
* @return
* the new instance of {@link PropertyNamePrefType }
*/
public PropertyNamePrefType createPropertyNamePrefType() {
return new PropertyNamePrefType();
}
/**
* Create an instance of {@link PropertyTypePrefType }
*
* @return
* the new instance of {@link PropertyTypePrefType }
*/
public PropertyTypePrefType createPropertyTypePrefType() {
return new PropertyTypePrefType();
}
/**
* Create an instance of {@link RecreationSrvcPrefType }
*
* @return
* the new instance of {@link RecreationSrvcPrefType }
*/
public RecreationSrvcPrefType createRecreationSrvcPrefType() {
return new RecreationSrvcPrefType();
}
/**
* Create an instance of {@link RoomAmenityPrefType }
*
* @return
* the new instance of {@link RoomAmenityPrefType }
*/
public RoomAmenityPrefType createRoomAmenityPrefType() {
return new RoomAmenityPrefType();
}
/**
* Create an instance of {@link RoomLocationPrefType }
*
* @return
* the new instance of {@link RoomLocationPrefType }
*/
public RoomLocationPrefType createRoomLocationPrefType() {
return new RoomLocationPrefType();
}
/**
* Create an instance of {@link SecurityFeaturePrefType }
*
* @return
* the new instance of {@link SecurityFeaturePrefType }
*/
public SecurityFeaturePrefType createSecurityFeaturePrefType() {
return new SecurityFeaturePrefType();
}
/**
* Create an instance of {@link CoverageDetailsType }
*
* @return
* the new instance of {@link CoverageDetailsType }
*/
public CoverageDetailsType createCoverageDetailsType() {
return new CoverageDetailsType();
}
/**
* Create an instance of {@link CoveragePricedType }
*
* @return
* the new instance of {@link CoveragePricedType }
*/
public CoveragePricedType createCoveragePricedType() {
return new CoveragePricedType();
}
/**
* Create an instance of {@link CoverageType }
*
* @return
* the new instance of {@link CoverageType }
*/
public CoverageType createCoverageType() {
return new CoverageType();
}
/**
* Create an instance of {@link DeductibleType }
*
* @return
* the new instance of {@link DeductibleType }
*/
public DeductibleType createDeductibleType() {
return new DeductibleType();
}
/**
* Create an instance of {@link OffLocationServicePricedType }
*
* @return
* the new instance of {@link OffLocationServicePricedType }
*/
public OffLocationServicePricedType createOffLocationServicePricedType() {
return new OffLocationServicePricedType();
}
/**
* Create an instance of {@link VehicleArrivalDetailsType }
*
* @return
* the new instance of {@link VehicleArrivalDetailsType }
*/
public VehicleArrivalDetailsType createVehicleArrivalDetailsType() {
return new VehicleArrivalDetailsType();
}
/**
* Create an instance of {@link VehicleAvailRSAdditionalInfoType }
*
* @return
* the new instance of {@link VehicleAvailRSAdditionalInfoType }
*/
public VehicleAvailRSAdditionalInfoType createVehicleAvailRSAdditionalInfoType() {
return new VehicleAvailRSAdditionalInfoType();
}
/**
* Create an instance of {@link VehicleChargePurposeType }
*
* @return
* the new instance of {@link VehicleChargePurposeType }
*/
public VehicleChargePurposeType createVehicleChargePurposeType() {
return new VehicleChargePurposeType();
}
/**
* Create an instance of {@link VehicleEquipmentPricedType }
*
* @return
* the new instance of {@link VehicleEquipmentPricedType }
*/
public VehicleEquipmentPricedType createVehicleEquipmentPricedType() {
return new VehicleEquipmentPricedType();
}
/**
* Create an instance of {@link VehicleEquipmentType }
*
* @return
* the new instance of {@link VehicleEquipmentType }
*/
public VehicleEquipmentType createVehicleEquipmentType() {
return new VehicleEquipmentType();
}
/**
* Create an instance of {@link VehicleLocationInformationType }
*
* @return
* the new instance of {@link VehicleLocationInformationType }
*/
public VehicleLocationInformationType createVehicleLocationInformationType() {
return new VehicleLocationInformationType();
}
/**
* Create an instance of {@link VehicleSpecialReqPrefType }
*
* @return
* the new instance of {@link VehicleSpecialReqPrefType }
*/
public VehicleSpecialReqPrefType createVehicleSpecialReqPrefType() {
return new VehicleSpecialReqPrefType();
}
/**
* Create an instance of {@link VehicleTourInfoType }
*
* @return
* the new instance of {@link VehicleTourInfoType }
*/
public VehicleTourInfoType createVehicleTourInfoType() {
return new VehicleTourInfoType();
}
/**
* Create an instance of {@link VehicleWhereAtFacilityType }
*
* @return
* the new instance of {@link VehicleWhereAtFacilityType }
*/
public VehicleWhereAtFacilityType createVehicleWhereAtFacilityType() {
return new VehicleWhereAtFacilityType();
}
/**
* Create an instance of {@link VehicleResRSAdditionalInfoType }
*
* @return
* the new instance of {@link VehicleResRSAdditionalInfoType }
*/
public VehicleResRSAdditionalInfoType createVehicleResRSAdditionalInfoType() {
return new VehicleResRSAdditionalInfoType();
}
/**
* Create an instance of {@link VehicleResRSCoreType }
*
* @return
* the new instance of {@link VehicleResRSCoreType }
*/
public VehicleResRSCoreType createVehicleResRSCoreType() {
return new VehicleResRSCoreType();
}
/**
* Create an instance of {@link AffiliationsType }
*
* @return
* the new instance of {@link AffiliationsType }
*/
public AffiliationsType createAffiliationsType() {
return new AffiliationsType();
}
/**
* Create an instance of {@link CertificationType }
*
* @return
* the new instance of {@link CertificationType }
*/
public CertificationType createCertificationType() {
return new CertificationType();
}
/**
* Create an instance of {@link CommissionInfoType }
*
* @return
* the new instance of {@link CommissionInfoType }
*/
public CommissionInfoType createCommissionInfoType() {
return new CommissionInfoType();
}
/**
* Create an instance of {@link EmployerType }
*
* @return
* the new instance of {@link EmployerType }
*/
public EmployerType createEmployerType() {
return new EmployerType();
}
/**
* Create an instance of {@link InsuranceType }
*
* @return
* the new instance of {@link InsuranceType }
*/
public InsuranceType createInsuranceType() {
return new InsuranceType();
}
/**
* Create an instance of {@link LoyaltyProgramType }
*
* @return
* the new instance of {@link LoyaltyProgramType }
*/
public LoyaltyProgramType createLoyaltyProgramType() {
return new LoyaltyProgramType();
}
/**
* Create an instance of {@link TravelArrangerType }
*
* @return
* the new instance of {@link TravelArrangerType }
*/
public TravelArrangerType createTravelArrangerType() {
return new TravelArrangerType();
}
/**
* Create an instance of {@link FolioIDsType }
*
* @return
* the new instance of {@link FolioIDsType }
*/
public FolioIDsType createFolioIDsType() {
return new FolioIDsType();
}
/**
* Create an instance of {@link HotelResModifyRequestType }
*
* @return
* the new instance of {@link HotelResModifyRequestType }
*/
public HotelResModifyRequestType createHotelResModifyRequestType() {
return new HotelResModifyRequestType();
}
/**
* Create an instance of {@link HotelResModifyResponseType }
*
* @return
* the new instance of {@link HotelResModifyResponseType }
*/
public HotelResModifyResponseType createHotelResModifyResponseType() {
return new HotelResModifyResponseType();
}
/**
* Create an instance of {@link HotelResRequestType }
*
* @return
* the new instance of {@link HotelResRequestType }
*/
public HotelResRequestType createHotelResRequestType() {
return new HotelResRequestType();
}
/**
* Create an instance of {@link HotelResResponseType }
*
* @return
* the new instance of {@link HotelResResponseType }
*/
public HotelResResponseType createHotelResResponseType() {
return new HotelResResponseType();
}
/**
* Create an instance of {@link ResGlobalInfoType }
*
* @return
* the new instance of {@link ResGlobalInfoType }
*/
public ResGlobalInfoType createResGlobalInfoType() {
return new ResGlobalInfoType();
}
/**
* Create an instance of {@link ResGuestRPHsType }
*
* @return
* the new instance of {@link ResGuestRPHsType }
*/
public ResGuestRPHsType createResGuestRPHsType() {
return new ResGuestRPHsType();
}
/**
* Create an instance of {@link ResGuestsType }
*
* @return
* the new instance of {@link ResGuestsType }
*/
public ResGuestsType createResGuestsType() {
return new ResGuestsType();
}
/**
* Create an instance of {@link RevenueCategoriesType }
*
* @return
* the new instance of {@link RevenueCategoriesType }
*/
public RevenueCategoriesType createRevenueCategoriesType() {
return new RevenueCategoriesType();
}
/**
* Create an instance of {@link RevenueDetailsType }
*
* @return
* the new instance of {@link RevenueDetailsType }
*/
public RevenueDetailsType createRevenueDetailsType() {
return new RevenueDetailsType();
}
/**
* Create an instance of {@link RevenueDetailType }
*
* @return
* the new instance of {@link RevenueDetailType }
*/
public RevenueDetailType createRevenueDetailType() {
return new RevenueDetailType();
}
/**
* Create an instance of {@link ServiceType }
*
* @return
* the new instance of {@link ServiceType }
*/
public ServiceType createServiceType() {
return new ServiceType();
}
/**
* Create an instance of {@link StayInfosType }
*
* @return
* the new instance of {@link StayInfosType }
*/
public StayInfosType createStayInfosType() {
return new StayInfosType();
}
/**
* Create an instance of {@link StayInfoType }
*
* @return
* the new instance of {@link StayInfoType }
*/
public StayInfoType createStayInfoType() {
return new StayInfoType();
}
/**
* Create an instance of {@link AdditionalDetailType }
*
* @return
* the new instance of {@link AdditionalDetailType }
*/
public AdditionalDetailType createAdditionalDetailType() {
return new AdditionalDetailType();
}
/**
* Create an instance of {@link AdditionalDetailsType }
*
* @return
* the new instance of {@link AdditionalDetailsType }
*/
public AdditionalDetailsType createAdditionalDetailsType() {
return new AdditionalDetailsType();
}
/**
* Create an instance of {@link AdditionalGuestAmountType }
*
* @return
* the new instance of {@link AdditionalGuestAmountType }
*/
public AdditionalGuestAmountType createAdditionalGuestAmountType() {
return new AdditionalGuestAmountType();
}
/**
* Create an instance of {@link AmountLiteType }
*
* @return
* the new instance of {@link AmountLiteType }
*/
public AmountLiteType createAmountLiteType() {
return new AmountLiteType();
}
/**
* Create an instance of {@link AmountPercentType }
*
* @return
* the new instance of {@link AmountPercentType }
*/
public AmountPercentType createAmountPercentType() {
return new AmountPercentType();
}
/**
* Create an instance of {@link CancelPenaltiesType }
*
* @return
* the new instance of {@link CancelPenaltiesType }
*/
public CancelPenaltiesType createCancelPenaltiesType() {
return new CancelPenaltiesType();
}
/**
* Create an instance of {@link DiscountType }
*
* @return
* the new instance of {@link DiscountType }
*/
public DiscountType createDiscountType() {
return new DiscountType();
}
/**
* Create an instance of {@link DOWRulesType }
*
* @return
* the new instance of {@link DOWRulesType }
*/
public DOWRulesType createDOWRulesType() {
return new DOWRulesType();
}
/**
* Create an instance of {@link InvCountType }
*
* @return
* the new instance of {@link InvCountType }
*/
public InvCountType createInvCountType() {
return new InvCountType();
}
/**
* Create an instance of {@link MeetingRoomCodeType }
*
* @return
* the new instance of {@link MeetingRoomCodeType }
*/
public MeetingRoomCodeType createMeetingRoomCodeType() {
return new MeetingRoomCodeType();
}
/**
* Create an instance of {@link RateLiteType }
*
* @return
* the new instance of {@link RateLiteType }
*/
public RateLiteType createRateLiteType() {
return new RateLiteType();
}
/**
* Create an instance of {@link RatePlanLiteType }
*
* @return
* the new instance of {@link RatePlanLiteType }
*/
public RatePlanLiteType createRatePlanLiteType() {
return new RatePlanLiteType();
}
/**
* Create an instance of {@link RequiredPaymentLiteType }
*
* @return
* the new instance of {@link RequiredPaymentLiteType }
*/
public RequiredPaymentLiteType createRequiredPaymentLiteType() {
return new RequiredPaymentLiteType();
}
/**
* Create an instance of {@link RoomRateLiteType }
*
* @return
* the new instance of {@link RoomRateLiteType }
*/
public RoomRateLiteType createRoomRateLiteType() {
return new RoomRateLiteType();
}
/**
* Create an instance of {@link RoomStayCandidateType }
*
* @return
* the new instance of {@link RoomStayCandidateType }
*/
public RoomStayCandidateType createRoomStayCandidateType() {
return new RoomStayCandidateType();
}
/**
* Create an instance of {@link RoomTypeLiteType }
*
* @return
* the new instance of {@link RoomTypeLiteType }
*/
public RoomTypeLiteType createRoomTypeLiteType() {
return new RoomTypeLiteType();
}
/**
* Create an instance of {@link StatisticsType }
*
* @return
* the new instance of {@link StatisticsType }
*/
public StatisticsType createStatisticsType() {
return new StatisticsType();
}
/**
* Create an instance of {@link StatusApplicationControlType }
*
* @return
* the new instance of {@link StatusApplicationControlType }
*/
public StatusApplicationControlType createStatusApplicationControlType() {
return new StatusApplicationControlType();
}
/**
* Create an instance of {@link UsernameTokenType }
*
* @return
* the new instance of {@link UsernameTokenType }
*/
public UsernameTokenType createUsernameTokenType() {
return new UsernameTokenType();
}
/**
* Create an instance of {@link BinarySecurityTokenType }
*
* @return
* the new instance of {@link BinarySecurityTokenType }
*/
public BinarySecurityTokenType createBinarySecurityTokenType() {
return new BinarySecurityTokenType();
}
/**
* Create an instance of {@link ReferenceType }
*
* @return
* the new instance of {@link ReferenceType }
*/
public ReferenceType createReferenceType() {
return new ReferenceType();
}
/**
* Create an instance of {@link EmbeddedType }
*
* @return
* the new instance of {@link EmbeddedType }
*/
public EmbeddedType createEmbeddedType() {
return new EmbeddedType();
}
/**
* Create an instance of {@link KeyIdentifierType }
*
* @return
* the new instance of {@link KeyIdentifierType }
*/
public KeyIdentifierType createKeyIdentifierType() {
return new KeyIdentifierType();
}
/**
* Create an instance of {@link SecurityTokenReferenceType }
*
* @return
* the new instance of {@link SecurityTokenReferenceType }
*/
public SecurityTokenReferenceType createSecurityTokenReferenceType() {
return new SecurityTokenReferenceType();
}
/**
* Create an instance of {@link SecurityHeaderType }
*
* @return
* the new instance of {@link SecurityHeaderType }
*/
public SecurityHeaderType createSecurityHeaderType() {
return new SecurityHeaderType();
}
/**
* Create an instance of {@link TransformationParametersType }
*
* @return
* the new instance of {@link TransformationParametersType }
*/
public TransformationParametersType createTransformationParametersType() {
return new TransformationParametersType();
}
/**
* Create an instance of {@link PasswordString }
*
* @return
* the new instance of {@link PasswordString }
*/
public PasswordString createPasswordString() {
return new PasswordString();
}
/**
* Create an instance of {@link EncodedString }
*
* @return
* the new instance of {@link EncodedString }
*/
public EncodedString createEncodedString() {
return new EncodedString();
}
/**
* Create an instance of {@link AttributedString }
*
* @return
* the new instance of {@link AttributedString }
*/
public AttributedString createAttributedString() {
return new AttributedString();
}
/**
* Create an instance of {@link TimestampType }
*
* @return
* the new instance of {@link TimestampType }
*/
public TimestampType createTimestampType() {
return new TimestampType();
}
/**
* Create an instance of {@link AttributedDateTime }
*
* @return
* the new instance of {@link AttributedDateTime }
*/
public AttributedDateTime createAttributedDateTime() {
return new AttributedDateTime();
}
/**
* Create an instance of {@link AttributedURI }
*
* @return
* the new instance of {@link AttributedURI }
*/
public AttributedURI createAttributedURI() {
return new AttributedURI();
}
/**
* Create an instance of {@link OfferType.Discount }
*
* @return
* the new instance of {@link OfferType.Discount }
*/
public OfferType.Discount createOfferTypeDiscount() {
return new OfferType.Discount();
}
/**
* Create an instance of {@link OfferType.Guests.Guest }
*
* @return
* the new instance of {@link OfferType.Guests.Guest }
*/
public OfferType.Guests.Guest createOfferTypeGuestsGuest() {
return new OfferType.Guests.Guest();
}
/**
* Create an instance of {@link OfferType.Inventories.Inventory }
*
* @return
* the new instance of {@link OfferType.Inventories.Inventory }
*/
public OfferType.Inventories.Inventory createOfferTypeInventoriesInventory() {
return new OfferType.Inventories.Inventory();
}
/**
* Create an instance of {@link OfferType.CompatibleOffers.CompatibleOffer }
*
* @return
* the new instance of {@link OfferType.CompatibleOffers.CompatibleOffer }
*/
public OfferType.CompatibleOffers.CompatibleOffer createOfferTypeCompatibleOffersCompatibleOffer() {
return new OfferType.CompatibleOffers.CompatibleOffer();
}
/**
* Create an instance of {@link OfferType.FreeUpgrade.UpgradeFrom }
*
* @return
* the new instance of {@link OfferType.FreeUpgrade.UpgradeFrom }
*/
public OfferType.FreeUpgrade.UpgradeFrom createOfferTypeFreeUpgradeUpgradeFrom() {
return new OfferType.FreeUpgrade.UpgradeFrom();
}
/**
* Create an instance of {@link OfferType.FreeUpgrade.UpgradeTo }
*
* @return
* the new instance of {@link OfferType.FreeUpgrade.UpgradeTo }
*/
public OfferType.FreeUpgrade.UpgradeTo createOfferTypeFreeUpgradeUpgradeTo() {
return new OfferType.FreeUpgrade.UpgradeTo();
}
/**
* Create an instance of {@link OfferType.OfferRules.OfferRule.DateRestriction }
*
* @return
* the new instance of {@link OfferType.OfferRules.OfferRule.DateRestriction }
*/
public OfferType.OfferRules.OfferRule.DateRestriction createOfferTypeOfferRulesOfferRuleDateRestriction() {
return new OfferType.OfferRules.OfferRule.DateRestriction();
}
/**
* Create an instance of {@link OfferType.OfferRules.OfferRule.Occupancy }
*
* @return
* the new instance of {@link OfferType.OfferRules.OfferRule.Occupancy }
*/
public OfferType.OfferRules.OfferRule.Occupancy createOfferTypeOfferRulesOfferRuleOccupancy() {
return new OfferType.OfferRules.OfferRule.Occupancy();
}
/**
* Create an instance of {@link OfferType.OfferRules.OfferRule.Inventories.Inventory }
*
* @return
* the new instance of {@link OfferType.OfferRules.OfferRule.Inventories.Inventory }
*/
public OfferType.OfferRules.OfferRule.Inventories.Inventory createOfferTypeOfferRulesOfferRuleInventoriesInventory() {
return new OfferType.OfferRules.OfferRule.Inventories.Inventory();
}
/**
* Create an instance of {@link HotelRatePlanType.HotelRef }
*
* @return
* the new instance of {@link HotelRatePlanType.HotelRef }
*/
public HotelRatePlanType.HotelRef createHotelRatePlanTypeHotelRef() {
return new HotelRatePlanType.HotelRef();
}
/**
* Create an instance of {@link HotelRatePlanType.RatePlanLevelFee.Fee }
*
* @return
* the new instance of {@link HotelRatePlanType.RatePlanLevelFee.Fee }
*/
public HotelRatePlanType.RatePlanLevelFee.Fee createHotelRatePlanTypeRatePlanLevelFeeFee() {
return new HotelRatePlanType.RatePlanLevelFee.Fee();
}
/**
* Create an instance of {@link HotelRatePlanType.RatePlanShoulders.RatePlanShoulder }
*
* @return
* the new instance of {@link HotelRatePlanType.RatePlanShoulders.RatePlanShoulder }
*/
public HotelRatePlanType.RatePlanShoulders.RatePlanShoulder createHotelRatePlanTypeRatePlanShouldersRatePlanShoulder() {
return new HotelRatePlanType.RatePlanShoulders.RatePlanShoulder();
}
/**
* Create an instance of {@link HotelRatePlanType.Offers.Offer }
*
* @return
* the new instance of {@link HotelRatePlanType.Offers.Offer }
*/
public HotelRatePlanType.Offers.Offer createHotelRatePlanTypeOffersOffer() {
return new HotelRatePlanType.Offers.Offer();
}
/**
* Create an instance of {@link HotelRatePlanType.Supplements.Supplement.RoomCompanions }
*
* @return
* the new instance of {@link HotelRatePlanType.Supplements.Supplement.RoomCompanions }
*/
public HotelRatePlanType.Supplements.Supplement.RoomCompanions createHotelRatePlanTypeSupplementsSupplementRoomCompanions() {
return new HotelRatePlanType.Supplements.Supplement.RoomCompanions();
}
/**
* Create an instance of {@link HotelRatePlanType.Supplements.Supplement.PrerequisiteInventory }
*
* @return
* the new instance of {@link HotelRatePlanType.Supplements.Supplement.PrerequisiteInventory }
*/
public HotelRatePlanType.Supplements.Supplement.PrerequisiteInventory createHotelRatePlanTypeSupplementsSupplementPrerequisiteInventory() {
return new HotelRatePlanType.Supplements.Supplement.PrerequisiteInventory();
}
/**
* Create an instance of {@link HotelRatePlanType.Rates.Rate }
*
* @return
* the new instance of {@link HotelRatePlanType.Rates.Rate }
*/
public HotelRatePlanType.Rates.Rate createHotelRatePlanTypeRatesRate() {
return new HotelRatePlanType.Rates.Rate();
}
/**
* Create an instance of {@link RateUploadType.GuaranteePolicies }
*
* @return
* the new instance of {@link RateUploadType.GuaranteePolicies }
*/
public RateUploadType.GuaranteePolicies createRateUploadTypeGuaranteePolicies() {
return new RateUploadType.GuaranteePolicies();
}
/**
* Create an instance of {@link RateUploadType.MealsIncluded }
*
* @return
* the new instance of {@link RateUploadType.MealsIncluded }
*/
public RateUploadType.MealsIncluded createRateUploadTypeMealsIncluded() {
return new RateUploadType.MealsIncluded();
}
/**
* Create an instance of {@link RateUploadType.AdditionalGuestAmounts.AdditionalGuestAmount }
*
* @return
* the new instance of {@link RateUploadType.AdditionalGuestAmounts.AdditionalGuestAmount }
*/
public RateUploadType.AdditionalGuestAmounts.AdditionalGuestAmount createRateUploadTypeAdditionalGuestAmountsAdditionalGuestAmount() {
return new RateUploadType.AdditionalGuestAmounts.AdditionalGuestAmount();
}
/**
* Create an instance of {@link RateUploadType.BaseByGuestAmts.BaseByGuestAmt }
*
* @return
* the new instance of {@link RateUploadType.BaseByGuestAmts.BaseByGuestAmt }
*/
public RateUploadType.BaseByGuestAmts.BaseByGuestAmt createRateUploadTypeBaseByGuestAmtsBaseByGuestAmt() {
return new RateUploadType.BaseByGuestAmts.BaseByGuestAmt();
}
/**
* Create an instance of {@link HotelRatePlanType.BookingRules.InventoryInfo }
*
* @return
* the new instance of {@link HotelRatePlanType.BookingRules.InventoryInfo }
*/
public HotelRatePlanType.BookingRules.InventoryInfo createHotelRatePlanTypeBookingRulesInventoryInfo() {
return new HotelRatePlanType.BookingRules.InventoryInfo();
}
/**
* Create an instance of {@link BookingRulesType.BookingRule.RestrictionStatus }
*
* @return
* the new instance of {@link BookingRulesType.BookingRule.RestrictionStatus }
*/
public BookingRulesType.BookingRule.RestrictionStatus createBookingRulesTypeBookingRuleRestrictionStatus() {
return new BookingRulesType.BookingRule.RestrictionStatus();
}
/**
* Create an instance of {@link BookingRulesType.BookingRule.CheckoutCharge }
*
* @return
* the new instance of {@link BookingRulesType.BookingRule.CheckoutCharge }
*/
public BookingRulesType.BookingRule.CheckoutCharge createBookingRulesTypeBookingRuleCheckoutCharge() {
return new BookingRulesType.BookingRule.CheckoutCharge();
}
/**
* Create an instance of {@link BookingRulesType.BookingRule.AddtionalRules.AdditionalRule }
*
* @return
* the new instance of {@link BookingRulesType.BookingRule.AddtionalRules.AdditionalRule }
*/
public BookingRulesType.BookingRule.AddtionalRules.AdditionalRule createBookingRulesTypeBookingRuleAddtionalRulesAdditionalRule() {
return new BookingRulesType.BookingRule.AddtionalRules.AdditionalRule();
}
/**
* Create an instance of {@link BookingRulesType.BookingRule.AcceptableGuarantees.AcceptableGuarantee }
*
* @return
* the new instance of {@link BookingRulesType.BookingRule.AcceptableGuarantees.AcceptableGuarantee }
*/
public BookingRulesType.BookingRule.AcceptableGuarantees.AcceptableGuarantee createBookingRulesTypeBookingRuleAcceptableGuaranteesAcceptableGuarantee() {
return new BookingRulesType.BookingRule.AcceptableGuarantees.AcceptableGuarantee();
}
/**
* Create an instance of {@link GuaranteeType.Deadline }
*
* @return
* the new instance of {@link GuaranteeType.Deadline }
*/
public GuaranteeType.Deadline createGuaranteeTypeDeadline() {
return new GuaranteeType.Deadline();
}
/**
* Create an instance of {@link GuaranteeType.GuaranteesAccepted.GuaranteeAccepted }
*
* @return
* the new instance of {@link GuaranteeType.GuaranteesAccepted.GuaranteeAccepted }
*/
public GuaranteeType.GuaranteesAccepted.GuaranteeAccepted createGuaranteeTypeGuaranteesAcceptedGuaranteeAccepted() {
return new GuaranteeType.GuaranteesAccepted.GuaranteeAccepted();
}
/**
* Create an instance of {@link PaymentFormType.Voucher }
*
* @return
* the new instance of {@link PaymentFormType.Voucher }
*/
public PaymentFormType.Voucher createPaymentFormTypeVoucher() {
return new PaymentFormType.Voucher();
}
/**
* Create an instance of {@link PaymentFormType.MiscChargeOrder }
*
* @return
* the new instance of {@link PaymentFormType.MiscChargeOrder }
*/
public PaymentFormType.MiscChargeOrder createPaymentFormTypeMiscChargeOrder() {
return new PaymentFormType.MiscChargeOrder();
}
/**
* Create an instance of {@link PaymentFormType.Cash }
*
* @return
* the new instance of {@link PaymentFormType.Cash }
*/
public PaymentFormType.Cash createPaymentFormTypeCash() {
return new PaymentFormType.Cash();
}
/**
* Create an instance of {@link PaymentFormType.Ticket.ConjunctionTicketNbr }
*
* @return
* the new instance of {@link PaymentFormType.Ticket.ConjunctionTicketNbr }
*/
public PaymentFormType.Ticket.ConjunctionTicketNbr createPaymentFormTypeTicketConjunctionTicketNbr() {
return new PaymentFormType.Ticket.ConjunctionTicketNbr();
}
/**
* Create an instance of {@link PaymentFormType.LoyaltyRedemption.LoyaltyCertificate }
*
* @return
* the new instance of {@link PaymentFormType.LoyaltyRedemption.LoyaltyCertificate }
*/
public PaymentFormType.LoyaltyRedemption.LoyaltyCertificate createPaymentFormTypeLoyaltyRedemptionLoyaltyCertificate() {
return new PaymentFormType.LoyaltyRedemption.LoyaltyCertificate();
}
/**
* Create an instance of {@link HotelRatePlanType.DestinationSystemsCode.DestinationSystemCode }
*
* @return
* the new instance of {@link HotelRatePlanType.DestinationSystemsCode.DestinationSystemCode }
*/
public HotelRatePlanType.DestinationSystemsCode.DestinationSystemCode createHotelRatePlanTypeDestinationSystemsCodeDestinationSystemCode() {
return new HotelRatePlanType.DestinationSystemsCode.DestinationSystemCode();
}
/**
* Create an instance of {@link AvailRequestSegmentsType.AvailRequestSegment.RateRange }
*
* @return
* the new instance of {@link AvailRequestSegmentsType.AvailRequestSegment.RateRange }
*/
public AvailRequestSegmentsType.AvailRequestSegment.RateRange createAvailRequestSegmentsTypeAvailRequestSegmentRateRange() {
return new AvailRequestSegmentsType.AvailRequestSegment.RateRange();
}
/**
* Create an instance of {@link AvailRequestSegmentsType.AvailRequestSegment.HotelSearchCriteria }
*
* @return
* the new instance of {@link AvailRequestSegmentsType.AvailRequestSegment.HotelSearchCriteria }
*/
public AvailRequestSegmentsType.AvailRequestSegment.HotelSearchCriteria createAvailRequestSegmentsTypeAvailRequestSegmentHotelSearchCriteria() {
return new AvailRequestSegmentsType.AvailRequestSegment.HotelSearchCriteria();
}
/**
* Create an instance of {@link HotelSearchCriteriaType.Criterion }
*
* @return
* the new instance of {@link HotelSearchCriteriaType.Criterion }
*/
public HotelSearchCriteriaType.Criterion createHotelSearchCriteriaTypeCriterion() {
return new HotelSearchCriteriaType.Criterion();
}
/**
* Create an instance of {@link ItemSearchCriterionType.Position }
*
* @return
* the new instance of {@link ItemSearchCriterionType.Position }
*/
public ItemSearchCriterionType.Position createItemSearchCriterionTypePosition() {
return new ItemSearchCriterionType.Position();
}
/**
* Create an instance of {@link ItemSearchCriterionType.Address }
*
* @return
* the new instance of {@link ItemSearchCriterionType.Address }
*/
public ItemSearchCriterionType.Address createItemSearchCriterionTypeAddress() {
return new ItemSearchCriterionType.Address();
}
/**
* Create an instance of {@link ItemSearchCriterionType.Telephone }
*
* @return
* the new instance of {@link ItemSearchCriterionType.Telephone }
*/
public ItemSearchCriterionType.Telephone createItemSearchCriterionTypeTelephone() {
return new ItemSearchCriterionType.Telephone();
}
/**
* Create an instance of {@link ItemSearchCriterionType.RefPoint }
*
* @return
* the new instance of {@link ItemSearchCriterionType.RefPoint }
*/
public ItemSearchCriterionType.RefPoint createItemSearchCriterionTypeRefPoint() {
return new ItemSearchCriterionType.RefPoint();
}
/**
* Create an instance of {@link ItemSearchCriterionType.CodeRef }
*
* @return
* the new instance of {@link ItemSearchCriterionType.CodeRef }
*/
public ItemSearchCriterionType.CodeRef createItemSearchCriterionTypeCodeRef() {
return new ItemSearchCriterionType.CodeRef();
}
/**
* Create an instance of {@link ItemSearchCriterionType.HotelRef }
*
* @return
* the new instance of {@link ItemSearchCriterionType.HotelRef }
*/
public ItemSearchCriterionType.HotelRef createItemSearchCriterionTypeHotelRef() {
return new ItemSearchCriterionType.HotelRef();
}
/**
* Create an instance of {@link ItemSearchCriterionType.Radius }
*
* @return
* the new instance of {@link ItemSearchCriterionType.Radius }
*/
public ItemSearchCriterionType.Radius createItemSearchCriterionTypeRadius() {
return new ItemSearchCriterionType.Radius();
}
/**
* Create an instance of {@link ItemSearchCriterionType.MapArea }
*
* @return
* the new instance of {@link ItemSearchCriterionType.MapArea }
*/
public ItemSearchCriterionType.MapArea createItemSearchCriterionTypeMapArea() {
return new ItemSearchCriterionType.MapArea();
}
/**
* Create an instance of {@link HotelSearchCriterionType.HotelAmenity }
*
* @return
* the new instance of {@link HotelSearchCriterionType.HotelAmenity }
*/
public HotelSearchCriterionType.HotelAmenity createHotelSearchCriterionTypeHotelAmenity() {
return new HotelSearchCriterionType.HotelAmenity();
}
/**
* Create an instance of {@link HotelSearchCriterionType.HotelFeature }
*
* @return
* the new instance of {@link HotelSearchCriterionType.HotelFeature }
*/
public HotelSearchCriterionType.HotelFeature createHotelSearchCriterionTypeHotelFeature() {
return new HotelSearchCriterionType.HotelFeature();
}
/**
* Create an instance of {@link HotelSearchCriterionType.Award }
*
* @return
* the new instance of {@link HotelSearchCriterionType.Award }
*/
public HotelSearchCriterionType.Award createHotelSearchCriterionTypeAward() {
return new HotelSearchCriterionType.Award();
}
/**
* Create an instance of {@link HotelSearchCriterionType.Recreation }
*
* @return
* the new instance of {@link HotelSearchCriterionType.Recreation }
*/
public HotelSearchCriterionType.Recreation createHotelSearchCriterionTypeRecreation() {
return new HotelSearchCriterionType.Recreation();
}
/**
* Create an instance of {@link HotelSearchCriterionType.Service }
*
* @return
* the new instance of {@link HotelSearchCriterionType.Service }
*/
public HotelSearchCriterionType.Service createHotelSearchCriterionTypeService() {
return new HotelSearchCriterionType.Service();
}
/**
* Create an instance of {@link HotelSearchCriterionType.Transportation }
*
* @return
* the new instance of {@link HotelSearchCriterionType.Transportation }
*/
public HotelSearchCriterionType.Transportation createHotelSearchCriterionTypeTransportation() {
return new HotelSearchCriterionType.Transportation();
}
/**
* Create an instance of {@link HotelSearchCriterionType.RateRange }
*
* @return
* the new instance of {@link HotelSearchCriterionType.RateRange }
*/
public HotelSearchCriterionType.RateRange createHotelSearchCriterionTypeRateRange() {
return new HotelSearchCriterionType.RateRange();
}
/**
* Create an instance of {@link HotelSearchCriterionType.RatePlanCandidates }
*
* @return
* the new instance of {@link HotelSearchCriterionType.RatePlanCandidates }
*/
public HotelSearchCriterionType.RatePlanCandidates createHotelSearchCriterionTypeRatePlanCandidates() {
return new HotelSearchCriterionType.RatePlanCandidates();
}
/**
* Create an instance of {@link HotelSearchCriterionType.RoomStayCandidates }
*
* @return
* the new instance of {@link HotelSearchCriterionType.RoomStayCandidates }
*/
public HotelSearchCriterionType.RoomStayCandidates createHotelSearchCriterionTypeRoomStayCandidates() {
return new HotelSearchCriterionType.RoomStayCandidates();
}
/**
* Create an instance of {@link HotelSearchCriterionType.Media }
*
* @return
* the new instance of {@link HotelSearchCriterionType.Media }
*/
public HotelSearchCriterionType.Media createHotelSearchCriterionTypeMedia() {
return new HotelSearchCriterionType.Media();
}
/**
* Create an instance of {@link HotelSearchCriterionType.HotelMeetingFacility }
*
* @return
* the new instance of {@link HotelSearchCriterionType.HotelMeetingFacility }
*/
public HotelSearchCriterionType.HotelMeetingFacility createHotelSearchCriterionTypeHotelMeetingFacility() {
return new HotelSearchCriterionType.HotelMeetingFacility();
}
/**
* Create an instance of {@link HotelSearchCriterionType.MealPlan }
*
* @return
* the new instance of {@link HotelSearchCriterionType.MealPlan }
*/
public HotelSearchCriterionType.MealPlan createHotelSearchCriterionTypeMealPlan() {
return new HotelSearchCriterionType.MealPlan();
}
/**
* Create an instance of {@link HotelSearchCriterionType.RebatePrograms }
*
* @return
* the new instance of {@link HotelSearchCriterionType.RebatePrograms }
*/
public HotelSearchCriterionType.RebatePrograms createHotelSearchCriterionTypeRebatePrograms() {
return new HotelSearchCriterionType.RebatePrograms();
}
/**
* Create an instance of {@link RatePlanCandidatesType.RatePlanCandidate.MealsIncluded }
*
* @return
* the new instance of {@link RatePlanCandidatesType.RatePlanCandidate.MealsIncluded }
*/
public RatePlanCandidatesType.RatePlanCandidate.MealsIncluded createRatePlanCandidatesTypeRatePlanCandidateMealsIncluded() {
return new RatePlanCandidatesType.RatePlanCandidate.MealsIncluded();
}
/**
* Create an instance of {@link RatePlanCandidatesType.RatePlanCandidate.ArrivalPolicy }
*
* @return
* the new instance of {@link RatePlanCandidatesType.RatePlanCandidate.ArrivalPolicy }
*/
public RatePlanCandidatesType.RatePlanCandidate.ArrivalPolicy createRatePlanCandidatesTypeRatePlanCandidateArrivalPolicy() {
return new RatePlanCandidatesType.RatePlanCandidate.ArrivalPolicy();
}
/**
* Create an instance of {@link RatePlanCandidatesType.RatePlanCandidate.RatePlanCommission }
*
* @return
* the new instance of {@link RatePlanCandidatesType.RatePlanCandidate.RatePlanCommission }
*/
public RatePlanCandidatesType.RatePlanCandidate.RatePlanCommission createRatePlanCandidatesTypeRatePlanCandidateRatePlanCommission() {
return new RatePlanCandidatesType.RatePlanCandidate.RatePlanCommission();
}
/**
* Create an instance of {@link RatePlanCandidatesType.RatePlanCandidate.HotelRefs.HotelRef }
*
* @return
* the new instance of {@link RatePlanCandidatesType.RatePlanCandidate.HotelRefs.HotelRef }
*/
public RatePlanCandidatesType.RatePlanCandidate.HotelRefs.HotelRef createRatePlanCandidatesTypeRatePlanCandidateHotelRefsHotelRef() {
return new RatePlanCandidatesType.RatePlanCandidate.HotelRefs.HotelRef();
}
/**
* Create an instance of {@link ItemSearchCriterionType.AdditionalContents.AdditionalContent }
*
* @return
* the new instance of {@link ItemSearchCriterionType.AdditionalContents.AdditionalContent }
*/
public ItemSearchCriterionType.AdditionalContents.AdditionalContent createItemSearchCriterionTypeAdditionalContentsAdditionalContent() {
return new ItemSearchCriterionType.AdditionalContents.AdditionalContent();
}
/**
* Create an instance of {@link AddressType.StreetNmbr }
*
* @return
* the new instance of {@link AddressType.StreetNmbr }
*/
public AddressType.StreetNmbr createAddressTypeStreetNmbr() {
return new AddressType.StreetNmbr();
}
/**
* Create an instance of {@link AddressType.BldgRoom }
*
* @return
* the new instance of {@link AddressType.BldgRoom }
*/
public AddressType.BldgRoom createAddressTypeBldgRoom() {
return new AddressType.BldgRoom();
}
/**
* Create an instance of {@link AvailRequestSegmentsType.AvailRequestSegment.RoomStayCandidates.RoomStayCandidate }
*
* @return
* the new instance of {@link AvailRequestSegmentsType.AvailRequestSegment.RoomStayCandidates.RoomStayCandidate }
*/
public AvailRequestSegmentsType.AvailRequestSegment.RoomStayCandidates.RoomStayCandidate createAvailRequestSegmentsTypeAvailRequestSegmentRoomStayCandidatesRoomStayCandidate() {
return new AvailRequestSegmentsType.AvailRequestSegment.RoomStayCandidates.RoomStayCandidate();
}
/**
* Create an instance of {@link WeatherInfoType.Precipitation }
*
* @return
* the new instance of {@link WeatherInfoType.Precipitation }
*/
public WeatherInfoType.Precipitation createWeatherInfoTypePrecipitation() {
return new WeatherInfoType.Precipitation();
}
/**
* Create an instance of {@link WeatherInfoType.Temperature }
*
* @return
* the new instance of {@link WeatherInfoType.Temperature }
*/
public WeatherInfoType.Temperature createWeatherInfoTypeTemperature() {
return new WeatherInfoType.Temperature();
}
/**
* Create an instance of {@link ViewershipsType.Viewership.ViewershipCodes }
*
* @return
* the new instance of {@link ViewershipsType.Viewership.ViewershipCodes }
*/
public ViewershipsType.Viewership.ViewershipCodes createViewershipsTypeViewershipViewershipCodes() {
return new ViewershipsType.Viewership.ViewershipCodes();
}
/**
* Create an instance of {@link ViewershipsType.Viewership.ProfileRefs }
*
* @return
* the new instance of {@link ViewershipsType.Viewership.ProfileRefs }
*/
public ViewershipsType.Viewership.ProfileRefs createViewershipsTypeViewershipProfileRefs() {
return new ViewershipsType.Viewership.ProfileRefs();
}
/**
* Create an instance of {@link ViewershipsType.Viewership.Profiles }
*
* @return
* the new instance of {@link ViewershipsType.Viewership.Profiles }
*/
public ViewershipsType.Viewership.Profiles createViewershipsTypeViewershipProfiles() {
return new ViewershipsType.Viewership.Profiles();
}
/**
* Create an instance of {@link ViewershipsType.Viewership.DistributorTypes.DistributorType }
*
* @return
* the new instance of {@link ViewershipsType.Viewership.DistributorTypes.DistributorType }
*/
public ViewershipsType.Viewership.DistributorTypes.DistributorType createViewershipsTypeViewershipDistributorTypesDistributorType() {
return new ViewershipsType.Viewership.DistributorTypes.DistributorType();
}
/**
* Create an instance of {@link ViewershipsType.Viewership.BookingChannelCodes.BookingChannelCode }
*
* @return
* the new instance of {@link ViewershipsType.Viewership.BookingChannelCodes.BookingChannelCode }
*/
public ViewershipsType.Viewership.BookingChannelCodes.BookingChannelCode createViewershipsTypeViewershipBookingChannelCodesBookingChannelCode() {
return new ViewershipsType.Viewership.BookingChannelCodes.BookingChannelCode();
}
/**
* Create an instance of {@link ViewershipsType.Viewership.LocationCodes.LocationCode }
*
* @return
* the new instance of {@link ViewershipsType.Viewership.LocationCodes.LocationCode }
*/
public ViewershipsType.Viewership.LocationCodes.LocationCode createViewershipsTypeViewershipLocationCodesLocationCode() {
return new ViewershipsType.Viewership.LocationCodes.LocationCode();
}
/**
* Create an instance of {@link ViewershipsType.Viewership.ProfileTypes.ProfileType }
*
* @return
* the new instance of {@link ViewershipsType.Viewership.ProfileTypes.ProfileType }
*/
public ViewershipsType.Viewership.ProfileTypes.ProfileType createViewershipsTypeViewershipProfileTypesProfileType() {
return new ViewershipsType.Viewership.ProfileTypes.ProfileType();
}
/**
* Create an instance of {@link ViewershipsType.Viewership.SystemCodes.SystemCode }
*
* @return
* the new instance of {@link ViewershipsType.Viewership.SystemCodes.SystemCode }
*/
public ViewershipsType.Viewership.SystemCodes.SystemCode createViewershipsTypeViewershipSystemCodesSystemCode() {
return new ViewershipsType.Viewership.SystemCodes.SystemCode();
}
/**
* Create an instance of {@link StatisticType.StatisticApplicationSets }
*
* @return
* the new instance of {@link StatisticType.StatisticApplicationSets }
*/
public StatisticType.StatisticApplicationSets createStatisticTypeStatisticApplicationSets() {
return new StatisticType.StatisticApplicationSets();
}
/**
* Create an instance of {@link StatisticApplicationSetType.ReportSummaries }
*
* @return
* the new instance of {@link StatisticApplicationSetType.ReportSummaries }
*/
public StatisticApplicationSetType.ReportSummaries createStatisticApplicationSetTypeReportSummaries() {
return new StatisticApplicationSetType.ReportSummaries();
}
/**
* Create an instance of {@link StatisticApplicationSetType.CountCategorySummaries.CountCategorySummary }
*
* @return
* the new instance of {@link StatisticApplicationSetType.CountCategorySummaries.CountCategorySummary }
*/
public StatisticApplicationSetType.CountCategorySummaries.CountCategorySummary createStatisticApplicationSetTypeCountCategorySummariesCountCategorySummary() {
return new StatisticApplicationSetType.CountCategorySummaries.CountCategorySummary();
}
/**
* Create an instance of {@link StatisticApplicationSetType.RevenueCategorySummaries.RevenueCategorySummary }
*
* @return
* the new instance of {@link StatisticApplicationSetType.RevenueCategorySummaries.RevenueCategorySummary }
*/
public StatisticApplicationSetType.RevenueCategorySummaries.RevenueCategorySummary createStatisticApplicationSetTypeRevenueCategorySummariesRevenueCategorySummary() {
return new StatisticApplicationSetType.RevenueCategorySummaries.RevenueCategorySummary();
}
/**
* Create an instance of {@link StatisticApplicationSetType.StatisticCodes.StatisticCode }
*
* @return
* the new instance of {@link StatisticApplicationSetType.StatisticCodes.StatisticCode }
*/
public StatisticApplicationSetType.StatisticCodes.StatisticCode createStatisticApplicationSetTypeStatisticCodesStatisticCode() {
return new StatisticApplicationSetType.StatisticCodes.StatisticCode();
}
/**
* Create an instance of {@link SellableProductsType.SellableProduct.InventoryBlock }
*
* @return
* the new instance of {@link SellableProductsType.SellableProduct.InventoryBlock }
*/
public SellableProductsType.SellableProduct.InventoryBlock createSellableProductsTypeSellableProductInventoryBlock() {
return new SellableProductsType.SellableProduct.InventoryBlock();
}
/**
* Create an instance of {@link SellableProductsType.SellableProduct.DestinationSystemCodes.DestinationSystemCode }
*
* @return
* the new instance of {@link SellableProductsType.SellableProduct.DestinationSystemCodes.DestinationSystemCode }
*/
public SellableProductsType.SellableProduct.DestinationSystemCodes.DestinationSystemCode createSellableProductsTypeSellableProductDestinationSystemCodesDestinationSystemCode() {
return new SellableProductsType.SellableProduct.DestinationSystemCodes.DestinationSystemCode();
}
/**
* Create an instance of {@link RoutingHopType.RoutingHop }
*
* @return
* the new instance of {@link RoutingHopType.RoutingHop }
*/
public RoutingHopType.RoutingHop createRoutingHopTypeRoutingHop() {
return new RoutingHopType.RoutingHop();
}
/**
* Create an instance of {@link RoomTypeType.Amenities }
*
* @return
* the new instance of {@link RoomTypeType.Amenities }
*/
public RoomTypeType.Amenities createRoomTypeTypeAmenities() {
return new RoomTypeType.Amenities();
}
/**
* Create an instance of {@link RoomTypeType.Occupancy }
*
* @return
* the new instance of {@link RoomTypeType.Occupancy }
*/
public RoomTypeType.Occupancy createRoomTypeTypeOccupancy() {
return new RoomTypeType.Occupancy();
}
/**
* Create an instance of {@link RoomStayType.RoomTypes }
*
* @return
* the new instance of {@link RoomStayType.RoomTypes }
*/
public RoomStayType.RoomTypes createRoomStayTypeRoomTypes() {
return new RoomStayType.RoomTypes();
}
/**
* Create an instance of {@link RoomStayType.RatePlans }
*
* @return
* the new instance of {@link RoomStayType.RatePlans }
*/
public RoomStayType.RatePlans createRoomStayTypeRatePlans() {
return new RoomStayType.RatePlans();
}
/**
* Create an instance of {@link RoomStayType.MapURL }
*
* @return
* the new instance of {@link RoomStayType.MapURL }
*/
public RoomStayType.MapURL createRoomStayTypeMapURL() {
return new RoomStayType.MapURL();
}
/**
* Create an instance of {@link RoomRateType.Availability }
*
* @return
* the new instance of {@link RoomRateType.Availability }
*/
public RoomRateType.Availability createRoomRateTypeAvailability() {
return new RoomRateType.Availability();
}
/**
* Create an instance of {@link RoomStayType.RoomRates.RoomRate.AdvanceBookingRestriction }
*
* @return
* the new instance of {@link RoomStayType.RoomRates.RoomRate.AdvanceBookingRestriction }
*/
public RoomStayType.RoomRates.RoomRate.AdvanceBookingRestriction createRoomStayTypeRoomRatesRoomRateAdvanceBookingRestriction() {
return new RoomStayType.RoomRates.RoomRate.AdvanceBookingRestriction();
}
/**
* Create an instance of {@link RoomStayType.RoomRates.RoomRate.GuestCounts.GuestCount }
*
* @return
* the new instance of {@link RoomStayType.RoomRates.RoomRate.GuestCounts.GuestCount }
*/
public RoomStayType.RoomRates.RoomRate.GuestCounts.GuestCount createRoomStayTypeRoomRatesRoomRateGuestCountsGuestCount() {
return new RoomStayType.RoomRates.RoomRate.GuestCounts.GuestCount();
}
/**
* Create an instance of {@link RoomStayType.RoomRates.RoomRate.Restrictions.Restriction }
*
* @return
* the new instance of {@link RoomStayType.RoomRates.RoomRate.Restrictions.Restriction }
*/
public RoomStayType.RoomRates.RoomRate.Restrictions.Restriction createRoomStayTypeRoomRatesRoomRateRestrictionsRestriction() {
return new RoomStayType.RoomRates.RoomRate.Restrictions.Restriction();
}
/**
* Create an instance of {@link RoomRateType.Features.Feature }
*
* @return
* the new instance of {@link RoomRateType.Features.Feature }
*/
public RoomRateType.Features.Feature createRoomRateTypeFeaturesFeature() {
return new RoomRateType.Features.Feature();
}
/**
* Create an instance of {@link RoomSharesType.RoomShare.GuestRPHs.GuestRPH }
*
* @return
* the new instance of {@link RoomSharesType.RoomShare.GuestRPHs.GuestRPH }
*/
public RoomSharesType.RoomShare.GuestRPHs.GuestRPH createRoomSharesTypeRoomShareGuestRPHsGuestRPH() {
return new RoomSharesType.RoomShare.GuestRPHs.GuestRPH();
}
/**
* Create an instance of {@link RoomStayLiteType.RoomTypes }
*
* @return
* the new instance of {@link RoomStayLiteType.RoomTypes }
*/
public RoomStayLiteType.RoomTypes createRoomStayLiteTypeRoomTypes() {
return new RoomStayLiteType.RoomTypes();
}
/**
* Create an instance of {@link RoomStayLiteType.RatePlans }
*
* @return
* the new instance of {@link RoomStayLiteType.RatePlans }
*/
public RoomStayLiteType.RatePlans createRoomStayLiteTypeRatePlans() {
return new RoomStayLiteType.RatePlans();
}
/**
* Create an instance of {@link RoomStayLiteType.RoomRates }
*
* @return
* the new instance of {@link RoomStayLiteType.RoomRates }
*/
public RoomStayLiteType.RoomRates createRoomStayLiteTypeRoomRates() {
return new RoomStayLiteType.RoomRates();
}
/**
* Create an instance of {@link RoomStayLiteType.BasicPropertyInfo }
*
* @return
* the new instance of {@link RoomStayLiteType.BasicPropertyInfo }
*/
public RoomStayLiteType.BasicPropertyInfo createRoomStayLiteTypeBasicPropertyInfo() {
return new RoomStayLiteType.BasicPropertyInfo();
}
/**
* Create an instance of {@link RFPResponseDetailType.Comments }
*
* @return
* the new instance of {@link RFPResponseDetailType.Comments }
*/
public RFPResponseDetailType.Comments createRFPResponseDetailTypeComments() {
return new RFPResponseDetailType.Comments();
}
/**
* Create an instance of {@link RequiredPaymentsType.GuaranteePayment.AmountPercent }
*
* @return
* the new instance of {@link RequiredPaymentsType.GuaranteePayment.AmountPercent }
*/
public RequiredPaymentsType.GuaranteePayment.AmountPercent createRequiredPaymentsTypeGuaranteePaymentAmountPercent() {
return new RequiredPaymentsType.GuaranteePayment.AmountPercent();
}
/**
* Create an instance of {@link RequiredPaymentsType.GuaranteePayment.Deadline }
*
* @return
* the new instance of {@link RequiredPaymentsType.GuaranteePayment.Deadline }
*/
public RequiredPaymentsType.GuaranteePayment.Deadline createRequiredPaymentsTypeGuaranteePaymentDeadline() {
return new RequiredPaymentsType.GuaranteePayment.Deadline();
}
/**
* Create an instance of {@link RequiredPaymentsType.GuaranteePayment.Address }
*
* @return
* the new instance of {@link RequiredPaymentsType.GuaranteePayment.Address }
*/
public RequiredPaymentsType.GuaranteePayment.Address createRequiredPaymentsTypeGuaranteePaymentAddress() {
return new RequiredPaymentsType.GuaranteePayment.Address();
}
/**
* Create an instance of {@link RateType.Rate }
*
* @return
* the new instance of {@link RateType.Rate }
*/
public RateType.Rate createRateTypeRate() {
return new RateType.Rate();
}
/**
* Create an instance of {@link AmountType.AdditionalGuestAmounts }
*
* @return
* the new instance of {@link AmountType.AdditionalGuestAmounts }
*/
public AmountType.AdditionalGuestAmounts createAmountTypeAdditionalGuestAmounts() {
return new AmountType.AdditionalGuestAmounts();
}
/**
* Create an instance of {@link AmountType.Discount }
*
* @return
* the new instance of {@link AmountType.Discount }
*/
public AmountType.Discount createAmountTypeDiscount() {
return new AmountType.Discount();
}
/**
* Create an instance of {@link RatePlanType.RatePlanInclusions }
*
* @return
* the new instance of {@link RatePlanType.RatePlanInclusions }
*/
public RatePlanType.RatePlanInclusions createRatePlanTypeRatePlanInclusions() {
return new RatePlanType.RatePlanInclusions();
}
/**
* Create an instance of {@link RatePlanType.MealsIncluded }
*
* @return
* the new instance of {@link RatePlanType.MealsIncluded }
*/
public RatePlanType.MealsIncluded createRatePlanTypeMealsIncluded() {
return new RatePlanType.MealsIncluded();
}
/**
* Create an instance of {@link RatePlanType.RestrictionStatus }
*
* @return
* the new instance of {@link RatePlanType.RestrictionStatus }
*/
public RatePlanType.RestrictionStatus createRatePlanTypeRestrictionStatus() {
return new RatePlanType.RestrictionStatus();
}
/**
* Create an instance of {@link RateAmountMessageType.Rates.Rate }
*
* @return
* the new instance of {@link RateAmountMessageType.Rates.Rate }
*/
public RateAmountMessageType.Rates.Rate createRateAmountMessageTypeRatesRate() {
return new RateAmountMessageType.Rates.Rate();
}
/**
* Create an instance of {@link BasicPropertyInfoType.Position }
*
* @return
* the new instance of {@link BasicPropertyInfoType.Position }
*/
public BasicPropertyInfoType.Position createBasicPropertyInfoTypePosition() {
return new BasicPropertyInfoType.Position();
}
/**
* Create an instance of {@link BasicPropertyInfoType.Award }
*
* @return
* the new instance of {@link BasicPropertyInfoType.Award }
*/
public BasicPropertyInfoType.Award createBasicPropertyInfoTypeAward() {
return new BasicPropertyInfoType.Award();
}
/**
* Create an instance of {@link BasicPropertyInfoType.HotelAmenity }
*
* @return
* the new instance of {@link BasicPropertyInfoType.HotelAmenity }
*/
public BasicPropertyInfoType.HotelAmenity createBasicPropertyInfoTypeHotelAmenity() {
return new BasicPropertyInfoType.HotelAmenity();
}
/**
* Create an instance of {@link BasicPropertyInfoType.Recreation }
*
* @return
* the new instance of {@link BasicPropertyInfoType.Recreation }
*/
public BasicPropertyInfoType.Recreation createBasicPropertyInfoTypeRecreation() {
return new BasicPropertyInfoType.Recreation();
}
/**
* Create an instance of {@link BasicPropertyInfoType.Service }
*
* @return
* the new instance of {@link BasicPropertyInfoType.Service }
*/
public BasicPropertyInfoType.Service createBasicPropertyInfoTypeService() {
return new BasicPropertyInfoType.Service();
}
/**
* Create an instance of {@link BasicPropertyInfoType.Policy }
*
* @return
* the new instance of {@link BasicPropertyInfoType.Policy }
*/
public BasicPropertyInfoType.Policy createBasicPropertyInfoTypePolicy() {
return new BasicPropertyInfoType.Policy();
}
/**
* Create an instance of {@link PropertyValueMatchType.SearchValueMatch }
*
* @return
* the new instance of {@link PropertyValueMatchType.SearchValueMatch }
*/
public PropertyValueMatchType.SearchValueMatch createPropertyValueMatchTypeSearchValueMatch() {
return new PropertyValueMatchType.SearchValueMatch();
}
/**
* Create an instance of {@link PropertyValueMatchType.RateRange }
*
* @return
* the new instance of {@link PropertyValueMatchType.RateRange }
*/
public PropertyValueMatchType.RateRange createPropertyValueMatchTypeRateRange() {
return new PropertyValueMatchType.RateRange();
}
/**
* Create an instance of {@link PropertyValueMatchType.Amenities.Amenity }
*
* @return
* the new instance of {@link PropertyValueMatchType.Amenities.Amenity }
*/
public PropertyValueMatchType.Amenities.Amenity createPropertyValueMatchTypeAmenitiesAmenity() {
return new PropertyValueMatchType.Amenities.Amenity();
}
/**
* Create an instance of {@link BasicPropertyInfoType.ContactNumbers.ContactNumber }
*
* @return
* the new instance of {@link BasicPropertyInfoType.ContactNumbers.ContactNumber }
*/
public BasicPropertyInfoType.ContactNumbers.ContactNumber createBasicPropertyInfoTypeContactNumbersContactNumber() {
return new BasicPropertyInfoType.ContactNumbers.ContactNumber();
}
/**
* Create an instance of {@link ProductDescriptionsType.ProductDescription }
*
* @return
* the new instance of {@link ProductDescriptionsType.ProductDescription }
*/
public ProductDescriptionsType.ProductDescription createProductDescriptionsTypeProductDescription() {
return new ProductDescriptionsType.ProductDescription();
}
/**
* Create an instance of {@link MessageType.OriginalPayloadStdAttributes }
*
* @return
* the new instance of {@link MessageType.OriginalPayloadStdAttributes }
*/
public MessageType.OriginalPayloadStdAttributes createMessageTypeOriginalPayloadStdAttributes() {
return new MessageType.OriginalPayloadStdAttributes();
}
/**
* Create an instance of {@link MembershipType.Membership }
*
* @return
* the new instance of {@link MembershipType.Membership }
*/
public MembershipType.Membership createMembershipTypeMembership() {
return new MembershipType.Membership();
}
/**
* Create an instance of {@link MeetingRoomsType.MeetingRoom.Codes }
*
* @return
* the new instance of {@link MeetingRoomsType.MeetingRoom.Codes }
*/
public MeetingRoomsType.MeetingRoom.Codes createMeetingRoomsTypeMeetingRoomCodes() {
return new MeetingRoomsType.MeetingRoom.Codes();
}
/**
* Create an instance of {@link MeetingRoomsType.MeetingRoom.Dimension }
*
* @return
* the new instance of {@link MeetingRoomsType.MeetingRoom.Dimension }
*/
public MeetingRoomsType.MeetingRoom.Dimension createMeetingRoomsTypeMeetingRoomDimension() {
return new MeetingRoomsType.MeetingRoom.Dimension();
}
/**
* Create an instance of {@link MeetingRoomsType.MeetingRoom.AvailableCapacities }
*
* @return
* the new instance of {@link MeetingRoomsType.MeetingRoom.AvailableCapacities }
*/
public MeetingRoomsType.MeetingRoom.AvailableCapacities createMeetingRoomsTypeMeetingRoomAvailableCapacities() {
return new MeetingRoomsType.MeetingRoom.AvailableCapacities();
}
/**
* Create an instance of {@link MeetingRoomCapacityType.Occupancy }
*
* @return
* the new instance of {@link MeetingRoomCapacityType.Occupancy }
*/
public MeetingRoomCapacityType.Occupancy createMeetingRoomCapacityTypeOccupancy() {
return new MeetingRoomCapacityType.Occupancy();
}
/**
* Create an instance of {@link LengthsOfStayType.LengthOfStay.LOSPattern }
*
* @return
* the new instance of {@link LengthsOfStayType.LengthOfStay.LOSPattern }
*/
public LengthsOfStayType.LengthOfStay.LOSPattern createLengthsOfStayTypeLengthOfStayLOSPattern() {
return new LengthsOfStayType.LengthOfStay.LOSPattern();
}
/**
* Create an instance of {@link InvBlockType.HotelRef }
*
* @return
* the new instance of {@link InvBlockType.HotelRef }
*/
public InvBlockType.HotelRef createInvBlockTypeHotelRef() {
return new InvBlockType.HotelRef();
}
/**
* Create an instance of {@link InvBlockType.InvBlockDates }
*
* @return
* the new instance of {@link InvBlockType.InvBlockDates }
*/
public InvBlockType.InvBlockDates createInvBlockTypeInvBlockDates() {
return new InvBlockType.InvBlockDates();
}
/**
* Create an instance of {@link InvBlockType.RoomTypes }
*
* @return
* the new instance of {@link InvBlockType.RoomTypes }
*/
public InvBlockType.RoomTypes createInvBlockTypeRoomTypes() {
return new InvBlockType.RoomTypes();
}
/**
* Create an instance of {@link InvBlockType.MethodInfo }
*
* @return
* the new instance of {@link InvBlockType.MethodInfo }
*/
public InvBlockType.MethodInfo createInvBlockTypeMethodInfo() {
return new InvBlockType.MethodInfo();
}
/**
* Create an instance of {@link InvBlockType.Contacts }
*
* @return
* the new instance of {@link InvBlockType.Contacts }
*/
public InvBlockType.Contacts createInvBlockTypeContacts() {
return new InvBlockType.Contacts();
}
/**
* Create an instance of {@link InvBlockType.BlockDescriptions.BlockDescription }
*
* @return
* the new instance of {@link InvBlockType.BlockDescriptions.BlockDescription }
*/
public InvBlockType.BlockDescriptions.BlockDescription createInvBlockTypeBlockDescriptionsBlockDescription() {
return new InvBlockType.BlockDescriptions.BlockDescription();
}
/**
* Create an instance of {@link ParagraphType.ListItem }
*
* @return
* the new instance of {@link ParagraphType.ListItem }
*/
public ParagraphType.ListItem createParagraphTypeListItem() {
return new ParagraphType.ListItem();
}
/**
* Create an instance of {@link InvBlockRoomType.DaysOfWeeks }
*
* @return
* the new instance of {@link InvBlockRoomType.DaysOfWeeks }
*/
public InvBlockRoomType.DaysOfWeeks createInvBlockRoomTypeDaysOfWeeks() {
return new InvBlockRoomType.DaysOfWeeks();
}
/**
* Create an instance of {@link InvBlockRoomType.RatePlans.RatePlan.MarketCode }
*
* @return
* the new instance of {@link InvBlockRoomType.RatePlans.RatePlan.MarketCode }
*/
public InvBlockRoomType.RatePlans.RatePlan.MarketCode createInvBlockRoomTypeRatePlansRatePlanMarketCode() {
return new InvBlockRoomType.RatePlans.RatePlan.MarketCode();
}
/**
* Create an instance of {@link InvBlockRoomType.RatePlans.RatePlan.MethodInfo }
*
* @return
* the new instance of {@link InvBlockRoomType.RatePlans.RatePlan.MethodInfo }
*/
public InvBlockRoomType.RatePlans.RatePlan.MethodInfo createInvBlockRoomTypeRatePlansRatePlanMethodInfo() {
return new InvBlockRoomType.RatePlans.RatePlan.MethodInfo();
}
/**
* Create an instance of {@link InvBlockRoomType.RatePlans.RatePlan.DaysOfWeeks }
*
* @return
* the new instance of {@link InvBlockRoomType.RatePlans.RatePlan.DaysOfWeeks }
*/
public InvBlockRoomType.RatePlans.RatePlan.DaysOfWeeks createInvBlockRoomTypeRatePlansRatePlanDaysOfWeeks() {
return new InvBlockRoomType.RatePlans.RatePlan.DaysOfWeeks();
}
/**
* Create an instance of {@link InvBlockRoomType.RoomTypeAllocations.RoomTypeAllocation }
*
* @return
* the new instance of {@link InvBlockRoomType.RoomTypeAllocations.RoomTypeAllocation }
*/
public InvBlockRoomType.RoomTypeAllocations.RoomTypeAllocation createInvBlockRoomTypeRoomTypeAllocationsRoomTypeAllocation() {
return new InvBlockRoomType.RoomTypeAllocations.RoomTypeAllocation();
}
/**
* Create an instance of {@link HotelRoomListType.MasterAccount }
*
* @return
* the new instance of {@link HotelRoomListType.MasterAccount }
*/
public HotelRoomListType.MasterAccount createHotelRoomListTypeMasterAccount() {
return new HotelRoomListType.MasterAccount();
}
/**
* Create an instance of {@link HotelRoomListType.Event }
*
* @return
* the new instance of {@link HotelRoomListType.Event }
*/
public HotelRoomListType.Event createHotelRoomListTypeEvent() {
return new HotelRoomListType.Event();
}
/**
* Create an instance of {@link HotelRoomListType.RoomStays.RoomStay }
*
* @return
* the new instance of {@link HotelRoomListType.RoomStays.RoomStay }
*/
public HotelRoomListType.RoomStays.RoomStay createHotelRoomListTypeRoomStaysRoomStay() {
return new HotelRoomListType.RoomStays.RoomStay();
}
/**
* Create an instance of {@link DirectBillType.CompanyName }
*
* @return
* the new instance of {@link DirectBillType.CompanyName }
*/
public DirectBillType.CompanyName createDirectBillTypeCompanyName() {
return new DirectBillType.CompanyName();
}
/**
* Create an instance of {@link DirectBillType.Telephone }
*
* @return
* the new instance of {@link DirectBillType.Telephone }
*/
public DirectBillType.Telephone createDirectBillTypeTelephone() {
return new DirectBillType.Telephone();
}
/**
* Create an instance of {@link ContactPersonType.Telephone }
*
* @return
* the new instance of {@link ContactPersonType.Telephone }
*/
public ContactPersonType.Telephone createContactPersonTypeTelephone() {
return new ContactPersonType.Telephone();
}
/**
* Create an instance of {@link HotelRoomListType.MasterContact.UniqueIDs }
*
* @return
* the new instance of {@link HotelRoomListType.MasterContact.UniqueIDs }
*/
public HotelRoomListType.MasterContact.UniqueIDs createHotelRoomListTypeMasterContactUniqueIDs() {
return new HotelRoomListType.MasterContact.UniqueIDs();
}
/**
* Create an instance of {@link HotelRoomListType.MasterContact.Loyalty }
*
* @return
* the new instance of {@link HotelRoomListType.MasterContact.Loyalty }
*/
public HotelRoomListType.MasterContact.Loyalty createHotelRoomListTypeMasterContactLoyalty() {
return new HotelRoomListType.MasterContact.Loyalty();
}
/**
* Create an instance of {@link HotelRoomListType.Guests.Guest.Loyalty }
*
* @return
* the new instance of {@link HotelRoomListType.Guests.Guest.Loyalty }
*/
public HotelRoomListType.Guests.Guest.Loyalty createHotelRoomListTypeGuestsGuestLoyalty() {
return new HotelRoomListType.Guests.Guest.Loyalty();
}
/**
* Create an instance of {@link HotelRoomListType.Guests.Guest.GuaranteePayment }
*
* @return
* the new instance of {@link HotelRoomListType.Guests.Guest.GuaranteePayment }
*/
public HotelRoomListType.Guests.Guest.GuaranteePayment createHotelRoomListTypeGuestsGuestGuaranteePayment() {
return new HotelRoomListType.Guests.Guest.GuaranteePayment();
}
/**
* Create an instance of {@link HotelPaymentFormType.MasterAccountUsage }
*
* @return
* the new instance of {@link HotelPaymentFormType.MasterAccountUsage }
*/
public HotelPaymentFormType.MasterAccountUsage createHotelPaymentFormTypeMasterAccountUsage() {
return new HotelPaymentFormType.MasterAccountUsage();
}
/**
* Create an instance of {@link HotelAdditionalChargesType.AdditionalCharge }
*
* @return
* the new instance of {@link HotelAdditionalChargesType.AdditionalCharge }
*/
public HotelAdditionalChargesType.AdditionalCharge createHotelAdditionalChargesTypeAdditionalCharge() {
return new HotelAdditionalChargesType.AdditionalCharge();
}
/**
* Create an instance of {@link GuestRoomType.Quantities }
*
* @return
* the new instance of {@link GuestRoomType.Quantities }
*/
public GuestRoomType.Quantities createGuestRoomTypeQuantities() {
return new GuestRoomType.Quantities();
}
/**
* Create an instance of {@link GuestRoomType.Occupancy }
*
* @return
* the new instance of {@link GuestRoomType.Occupancy }
*/
public GuestRoomType.Occupancy createGuestRoomTypeOccupancy() {
return new GuestRoomType.Occupancy();
}
/**
* Create an instance of {@link GuestRoomType.Room }
*
* @return
* the new instance of {@link GuestRoomType.Room }
*/
public GuestRoomType.Room createGuestRoomTypeRoom() {
return new GuestRoomType.Room();
}
/**
* Create an instance of {@link GuestRoomType.RoomLevelFees }
*
* @return
* the new instance of {@link GuestRoomType.RoomLevelFees }
*/
public GuestRoomType.RoomLevelFees createGuestRoomTypeRoomLevelFees() {
return new GuestRoomType.RoomLevelFees();
}
/**
* Create an instance of {@link GuestRoomType.Amenities.Amenity }
*
* @return
* the new instance of {@link GuestRoomType.Amenities.Amenity }
*/
public GuestRoomType.Amenities.Amenity createGuestRoomTypeAmenitiesAmenity() {
return new GuestRoomType.Amenities.Amenity();
}
/**
* Create an instance of {@link GuestCountType.GuestCount }
*
* @return
* the new instance of {@link GuestCountType.GuestCount }
*/
public GuestCountType.GuestCount createGuestCountTypeGuestCount() {
return new GuestCountType.GuestCount();
}
/**
* Create an instance of {@link GDSInfoType.GDSCodes.GDSCode.GDSCodeDetails.GDSCodeDetail }
*
* @return
* the new instance of {@link GDSInfoType.GDSCodes.GDSCode.GDSCodeDetails.GDSCodeDetail }
*/
public GDSInfoType.GDSCodes.GDSCode.GDSCodeDetails.GDSCodeDetail createGDSInfoTypeGDSCodesGDSCodeGDSCodeDetailsGDSCodeDetail() {
return new GDSInfoType.GDSCodes.GDSCode.GDSCodeDetails.GDSCodeDetail();
}
/**
* Create an instance of {@link FeaturesType.Feature.Charge }
*
* @return
* the new instance of {@link FeaturesType.Feature.Charge }
*/
public FeaturesType.Feature.Charge createFeaturesTypeFeatureCharge() {
return new FeaturesType.Feature.Charge();
}
/**
* Create an instance of {@link DOWRestrictionsType.AvailableDaysOfWeek }
*
* @return
* the new instance of {@link DOWRestrictionsType.AvailableDaysOfWeek }
*/
public DOWRestrictionsType.AvailableDaysOfWeek createDOWRestrictionsTypeAvailableDaysOfWeek() {
return new DOWRestrictionsType.AvailableDaysOfWeek();
}
/**
* Create an instance of {@link DOWRestrictionsType.ArrivalDaysOfWeek }
*
* @return
* the new instance of {@link DOWRestrictionsType.ArrivalDaysOfWeek }
*/
public DOWRestrictionsType.ArrivalDaysOfWeek createDOWRestrictionsTypeArrivalDaysOfWeek() {
return new DOWRestrictionsType.ArrivalDaysOfWeek();
}
/**
* Create an instance of {@link DOWRestrictionsType.DepartureDaysOfWeek }
*
* @return
* the new instance of {@link DOWRestrictionsType.DepartureDaysOfWeek }
*/
public DOWRestrictionsType.DepartureDaysOfWeek createDOWRestrictionsTypeDepartureDaysOfWeek() {
return new DOWRestrictionsType.DepartureDaysOfWeek();
}
/**
* Create an instance of {@link DOWRestrictionsType.RequiredDaysOfWeek }
*
* @return
* the new instance of {@link DOWRestrictionsType.RequiredDaysOfWeek }
*/
public DOWRestrictionsType.RequiredDaysOfWeek createDOWRestrictionsTypeRequiredDaysOfWeek() {
return new DOWRestrictionsType.RequiredDaysOfWeek();
}
/**
* Create an instance of {@link DestinationSystemCodesType.DestinationSystemCode }
*
* @return
* the new instance of {@link DestinationSystemCodesType.DestinationSystemCode }
*/
public DestinationSystemCodesType.DestinationSystemCode createDestinationSystemCodesTypeDestinationSystemCode() {
return new DestinationSystemCodesType.DestinationSystemCode();
}
/**
* Create an instance of {@link CancelPenaltyType.Deadline }
*
* @return
* the new instance of {@link CancelPenaltyType.Deadline }
*/
public CancelPenaltyType.Deadline createCancelPenaltyTypeDeadline() {
return new CancelPenaltyType.Deadline();
}
/**
* Create an instance of {@link BaseInvCountType.OffSell }
*
* @return
* the new instance of {@link BaseInvCountType.OffSell }
*/
public BaseInvCountType.OffSell createBaseInvCountTypeOffSell() {
return new BaseInvCountType.OffSell();
}
/**
* Create an instance of {@link BaseInvCountType.InvCounts.InvCount.InvBlockCutoff }
*
* @return
* the new instance of {@link BaseInvCountType.InvCounts.InvCount.InvBlockCutoff }
*/
public BaseInvCountType.InvCounts.InvCount.InvBlockCutoff createBaseInvCountTypeInvCountsInvCountInvBlockCutoff() {
return new BaseInvCountType.InvCounts.InvCount.InvBlockCutoff();
}
/**
* Create an instance of {@link AvailStatusMessageType.HurdleRate }
*
* @return
* the new instance of {@link AvailStatusMessageType.HurdleRate }
*/
public AvailStatusMessageType.HurdleRate createAvailStatusMessageTypeHurdleRate() {
return new AvailStatusMessageType.HurdleRate();
}
/**
* Create an instance of {@link AvailStatusMessageType.Delta }
*
* @return
* the new instance of {@link AvailStatusMessageType.Delta }
*/
public AvailStatusMessageType.Delta createAvailStatusMessageTypeDelta() {
return new AvailStatusMessageType.Delta();
}
/**
* Create an instance of {@link AvailStatusMessageType.RestrictionStatus }
*
* @return
* the new instance of {@link AvailStatusMessageType.RestrictionStatus }
*/
public AvailStatusMessageType.RestrictionStatus createAvailStatusMessageTypeRestrictionStatus() {
return new AvailStatusMessageType.RestrictionStatus();
}
/**
* Create an instance of {@link AvailStatusMessageType.BestAvailableRates.BestAvailableRate }
*
* @return
* the new instance of {@link AvailStatusMessageType.BestAvailableRates.BestAvailableRate }
*/
public AvailStatusMessageType.BestAvailableRates.BestAvailableRate createAvailStatusMessageTypeBestAvailableRatesBestAvailableRate() {
return new AvailStatusMessageType.BestAvailableRates.BestAvailableRate();
}
/**
* Create an instance of {@link AdjustmentsType.Adjustment }
*
* @return
* the new instance of {@link AdjustmentsType.Adjustment }
*/
public AdjustmentsType.Adjustment createAdjustmentsTypeAdjustment() {
return new AdjustmentsType.Adjustment();
}
/**
* Create an instance of {@link AddressesType.Address }
*
* @return
* the new instance of {@link AddressesType.Address }
*/
public AddressesType.Address createAddressesTypeAddress() {
return new AddressesType.Address();
}
/**
* Create an instance of {@link TransportInfoType.TransportInfo }
*
* @return
* the new instance of {@link TransportInfoType.TransportInfo }
*/
public TransportInfoType.TransportInfo createTransportInfoTypeTransportInfo() {
return new TransportInfoType.TransportInfo();
}
/**
* Create an instance of {@link ServiceRPHsType.ServiceRPH }
*
* @return
* the new instance of {@link ServiceRPHsType.ServiceRPH }
*/
public ServiceRPHsType.ServiceRPH createServiceRPHsTypeServiceRPH() {
return new ServiceRPHsType.ServiceRPH();
}
/**
* Create an instance of {@link RoomStaysType.RoomStay.Reference }
*
* @return
* the new instance of {@link RoomStaysType.RoomStay.Reference }
*/
public RoomStaysType.RoomStay.Reference createRoomStaysTypeRoomStayReference() {
return new RoomStaysType.RoomStay.Reference();
}
/**
* Create an instance of {@link RevenueCategoryType.SummaryAmount }
*
* @return
* the new instance of {@link RevenueCategoryType.SummaryAmount }
*/
public RevenueCategoryType.SummaryAmount createRevenueCategoryTypeSummaryAmount() {
return new RevenueCategoryType.SummaryAmount();
}
/**
* Create an instance of {@link ResGuestType.ProfileRPHs.ProfileRPH }
*
* @return
* the new instance of {@link ResGuestType.ProfileRPHs.ProfileRPH }
*/
public ResGuestType.ProfileRPHs.ProfileRPH createResGuestTypeProfileRPHsProfileRPH() {
return new ResGuestType.ProfileRPHs.ProfileRPH();
}
/**
* Create an instance of {@link ResCommonDetailType.TimeSpan }
*
* @return
* the new instance of {@link ResCommonDetailType.TimeSpan }
*/
public ResCommonDetailType.TimeSpan createResCommonDetailTypeTimeSpan() {
return new ResCommonDetailType.TimeSpan();
}
/**
* Create an instance of {@link DateTimeSpanType.StartDateWindow }
*
* @return
* the new instance of {@link DateTimeSpanType.StartDateWindow }
*/
public DateTimeSpanType.StartDateWindow createDateTimeSpanTypeStartDateWindow() {
return new DateTimeSpanType.StartDateWindow();
}
/**
* Create an instance of {@link DateTimeSpanType.EndDateWindow }
*
* @return
* the new instance of {@link DateTimeSpanType.EndDateWindow }
*/
public DateTimeSpanType.EndDateWindow createDateTimeSpanTypeEndDateWindow() {
return new DateTimeSpanType.EndDateWindow();
}
/**
* Create an instance of {@link LoyaltyPointsAccrualsType.SelectedLoyalty }
*
* @return
* the new instance of {@link LoyaltyPointsAccrualsType.SelectedLoyalty }
*/
public LoyaltyPointsAccrualsType.SelectedLoyalty createLoyaltyPointsAccrualsTypeSelectedLoyalty() {
return new LoyaltyPointsAccrualsType.SelectedLoyalty();
}
/**
* Create an instance of {@link HotelReservationType.Queue }
*
* @return
* the new instance of {@link HotelReservationType.Queue }
*/
public HotelReservationType.Queue createHotelReservationTypeQueue() {
return new HotelReservationType.Queue();
}
/**
* Create an instance of {@link HotelReservationType.BillingInstructionCode.ResGuestRPH }
*
* @return
* the new instance of {@link HotelReservationType.BillingInstructionCode.ResGuestRPH }
*/
public HotelReservationType.BillingInstructionCode.ResGuestRPH createHotelReservationTypeBillingInstructionCodeResGuestRPH() {
return new HotelReservationType.BillingInstructionCode.ResGuestRPH();
}
/**
* Create an instance of {@link HotelResModifyType.HotelResModify.Verification }
*
* @return
* the new instance of {@link HotelResModifyType.HotelResModify.Verification }
*/
public HotelResModifyType.HotelResModify.Verification createHotelResModifyTypeHotelResModifyVerification() {
return new HotelResModifyType.HotelResModify.Verification();
}
/**
* Create an instance of {@link VerificationType.PersonName }
*
* @return
* the new instance of {@link VerificationType.PersonName }
*/
public VerificationType.PersonName createVerificationTypePersonName() {
return new VerificationType.PersonName();
}
/**
* Create an instance of {@link VerificationType.TelephoneInfo }
*
* @return
* the new instance of {@link VerificationType.TelephoneInfo }
*/
public VerificationType.TelephoneInfo createVerificationTypeTelephoneInfo() {
return new VerificationType.TelephoneInfo();
}
/**
* Create an instance of {@link VerificationType.CustLoyalty }
*
* @return
* the new instance of {@link VerificationType.CustLoyalty }
*/
public VerificationType.CustLoyalty createVerificationTypeCustLoyalty() {
return new VerificationType.CustLoyalty();
}
/**
* Create an instance of {@link VerificationType.ReservationTimeSpan }
*
* @return
* the new instance of {@link VerificationType.ReservationTimeSpan }
*/
public VerificationType.ReservationTimeSpan createVerificationTypeReservationTimeSpan() {
return new VerificationType.ReservationTimeSpan();
}
/**
* Create an instance of {@link VerificationType.AssociatedQuantity }
*
* @return
* the new instance of {@link VerificationType.AssociatedQuantity }
*/
public VerificationType.AssociatedQuantity createVerificationTypeAssociatedQuantity() {
return new VerificationType.AssociatedQuantity();
}
/**
* Create an instance of {@link VerificationType.StartLocation }
*
* @return
* the new instance of {@link VerificationType.StartLocation }
*/
public VerificationType.StartLocation createVerificationTypeStartLocation() {
return new VerificationType.StartLocation();
}
/**
* Create an instance of {@link VerificationType.EndLocation }
*
* @return
* the new instance of {@link VerificationType.EndLocation }
*/
public VerificationType.EndLocation createVerificationTypeEndLocation() {
return new VerificationType.EndLocation();
}
/**
* Create an instance of {@link PersonNameType.Document }
*
* @return
* the new instance of {@link PersonNameType.Document }
*/
public PersonNameType.Document createPersonNameTypeDocument() {
return new PersonNameType.Document();
}
/**
* Create an instance of {@link TravelClubType.ClubMemberName }
*
* @return
* the new instance of {@link TravelClubType.ClubMemberName }
*/
public TravelClubType.ClubMemberName createTravelClubTypeClubMemberName() {
return new TravelClubType.ClubMemberName();
}
/**
* Create an instance of {@link travel.wink.ws.allotz.ProfileType.UserID }
*
* @return
* the new instance of {@link travel.wink.ws.allotz.ProfileType.UserID }
*/
public travel.wink.ws.allotz.ProfileType.UserID createProfileTypeUserID() {
return new travel.wink.ws.allotz.ProfileType.UserID();
}
/**
* Create an instance of {@link travel.wink.ws.allotz.ProfileType.Comments.Comment.AuthorizedViewer }
*
* @return
* the new instance of {@link travel.wink.ws.allotz.ProfileType.Comments.Comment.AuthorizedViewer }
*/
public travel.wink.ws.allotz.ProfileType.Comments.Comment.AuthorizedViewer createProfileTypeCommentsCommentAuthorizedViewer() {
return new travel.wink.ws.allotz.ProfileType.Comments.Comment.AuthorizedViewer();
}
/**
* Create an instance of {@link PreferencesType.PrefCollection }
*
* @return
* the new instance of {@link PreferencesType.PrefCollection }
*/
public PreferencesType.PrefCollection createPreferencesTypePrefCollection() {
return new PreferencesType.PrefCollection();
}
/**
* Create an instance of {@link OrganizationType.OrgMemberName }
*
* @return
* the new instance of {@link OrganizationType.OrgMemberName }
*/
public OrganizationType.OrgMemberName createOrganizationTypeOrgMemberName() {
return new OrganizationType.OrgMemberName();
}
/**
* Create an instance of {@link CompanyInfoType.AddressInfo }
*
* @return
* the new instance of {@link CompanyInfoType.AddressInfo }
*/
public CompanyInfoType.AddressInfo createCompanyInfoTypeAddressInfo() {
return new CompanyInfoType.AddressInfo();
}
/**
* Create an instance of {@link CompanyInfoType.TelephoneInfo }
*
* @return
* the new instance of {@link CompanyInfoType.TelephoneInfo }
*/
public CompanyInfoType.TelephoneInfo createCompanyInfoTypeTelephoneInfo() {
return new CompanyInfoType.TelephoneInfo();
}
/**
* Create an instance of {@link CompanyInfoType.Email }
*
* @return
* the new instance of {@link CompanyInfoType.Email }
*/
public CompanyInfoType.Email createCompanyInfoTypeEmail() {
return new CompanyInfoType.Email();
}
/**
* Create an instance of {@link CompanyInfoType.PaymentForm }
*
* @return
* the new instance of {@link CompanyInfoType.PaymentForm }
*/
public CompanyInfoType.PaymentForm createCompanyInfoTypePaymentForm() {
return new CompanyInfoType.PaymentForm();
}
/**
* Create an instance of {@link CompanyInfoType.TripPurpose }
*
* @return
* the new instance of {@link CompanyInfoType.TripPurpose }
*/
public CompanyInfoType.TripPurpose createCompanyInfoTypeTripPurpose() {
return new CompanyInfoType.TripPurpose();
}
/**
* Create an instance of {@link AllianceConsortiumType.AllianceMember }
*
* @return
* the new instance of {@link AllianceConsortiumType.AllianceMember }
*/
public AllianceConsortiumType.AllianceMember createAllianceConsortiumTypeAllianceMember() {
return new AllianceConsortiumType.AllianceMember();
}
/**
* Create an instance of {@link AgreementsType.ProfileSecurity }
*
* @return
* the new instance of {@link AgreementsType.ProfileSecurity }
*/
public AgreementsType.ProfileSecurity createAgreementsTypeProfileSecurity() {
return new AgreementsType.ProfileSecurity();
}
/**
* Create an instance of {@link AccessesType.Access }
*
* @return
* the new instance of {@link AccessesType.Access }
*/
public AccessesType.Access createAccessesTypeAccess() {
return new AccessesType.Access();
}
/**
* Create an instance of {@link VehicleVendorAvailabilityType.VehAvails.VehAvail.AdvanceBooking }
*
* @return
* the new instance of {@link VehicleVendorAvailabilityType.VehAvails.VehAvail.AdvanceBooking }
*/
public VehicleVendorAvailabilityType.VehAvails.VehAvail.AdvanceBooking createVehicleVendorAvailabilityTypeVehAvailsVehAvailAdvanceBooking() {
return new VehicleVendorAvailabilityType.VehAvails.VehAvail.AdvanceBooking();
}
/**
* Create an instance of {@link VehicleCoreType.VehType }
*
* @return
* the new instance of {@link VehicleCoreType.VehType }
*/
public VehicleCoreType.VehType createVehicleCoreTypeVehType() {
return new VehicleCoreType.VehType();
}
/**
* Create an instance of {@link VehicleCoreType.VehClass }
*
* @return
* the new instance of {@link VehicleCoreType.VehClass }
*/
public VehicleCoreType.VehClass createVehicleCoreTypeVehClass() {
return new VehicleCoreType.VehClass();
}
/**
* Create an instance of {@link VehicleType.VehMakeModel }
*
* @return
* the new instance of {@link VehicleType.VehMakeModel }
*/
public VehicleType.VehMakeModel createVehicleTypeVehMakeModel() {
return new VehicleType.VehMakeModel();
}
/**
* Create an instance of {@link VehicleType.VehIdentity }
*
* @return
* the new instance of {@link VehicleType.VehIdentity }
*/
public VehicleType.VehIdentity createVehicleTypeVehIdentity() {
return new VehicleType.VehIdentity();
}
/**
* Create an instance of {@link VehicleSegmentCoreType.ConfID }
*
* @return
* the new instance of {@link VehicleSegmentCoreType.ConfID }
*/
public VehicleSegmentCoreType.ConfID createVehicleSegmentCoreTypeConfID() {
return new VehicleSegmentCoreType.ConfID();
}
/**
* Create an instance of {@link VehicleSegmentCoreType.PricedEquips }
*
* @return
* the new instance of {@link VehicleSegmentCoreType.PricedEquips }
*/
public VehicleSegmentCoreType.PricedEquips createVehicleSegmentCoreTypePricedEquips() {
return new VehicleSegmentCoreType.PricedEquips();
}
/**
* Create an instance of {@link VehicleSegmentCoreType.Fees }
*
* @return
* the new instance of {@link VehicleSegmentCoreType.Fees }
*/
public VehicleSegmentCoreType.Fees createVehicleSegmentCoreTypeFees() {
return new VehicleSegmentCoreType.Fees();
}
/**
* Create an instance of {@link VehicleSegmentCoreType.TotalCharge }
*
* @return
* the new instance of {@link VehicleSegmentCoreType.TotalCharge }
*/
public VehicleSegmentCoreType.TotalCharge createVehicleSegmentCoreTypeTotalCharge() {
return new VehicleSegmentCoreType.TotalCharge();
}
/**
* Create an instance of {@link VehicleSegmentAdditionalInfoType.PaymentRules }
*
* @return
* the new instance of {@link VehicleSegmentAdditionalInfoType.PaymentRules }
*/
public VehicleSegmentAdditionalInfoType.PaymentRules createVehicleSegmentAdditionalInfoTypePaymentRules() {
return new VehicleSegmentAdditionalInfoType.PaymentRules();
}
/**
* Create an instance of {@link VehicleSegmentAdditionalInfoType.PricedCoverages }
*
* @return
* the new instance of {@link VehicleSegmentAdditionalInfoType.PricedCoverages }
*/
public VehicleSegmentAdditionalInfoType.PricedCoverages createVehicleSegmentAdditionalInfoTypePricedCoverages() {
return new VehicleSegmentAdditionalInfoType.PricedCoverages();
}
/**
* Create an instance of {@link VehicleSegmentAdditionalInfoType.VendorMessages }
*
* @return
* the new instance of {@link VehicleSegmentAdditionalInfoType.VendorMessages }
*/
public VehicleSegmentAdditionalInfoType.VendorMessages createVehicleSegmentAdditionalInfoTypeVendorMessages() {
return new VehicleSegmentAdditionalInfoType.VendorMessages();
}
/**
* Create an instance of {@link VehicleReservationSummaryType.ConfID }
*
* @return
* the new instance of {@link VehicleReservationSummaryType.ConfID }
*/
public VehicleReservationSummaryType.ConfID createVehicleReservationSummaryTypeConfID() {
return new VehicleReservationSummaryType.ConfID();
}
/**
* Create an instance of {@link VehicleReservationRQCoreType.DriverType }
*
* @return
* the new instance of {@link VehicleReservationRQCoreType.DriverType }
*/
public VehicleReservationRQCoreType.DriverType createVehicleReservationRQCoreTypeDriverType() {
return new VehicleReservationRQCoreType.DriverType();
}
/**
* Create an instance of {@link VehicleReservationRQCoreType.Fees }
*
* @return
* the new instance of {@link VehicleReservationRQCoreType.Fees }
*/
public VehicleReservationRQCoreType.Fees createVehicleReservationRQCoreTypeFees() {
return new VehicleReservationRQCoreType.Fees();
}
/**
* Create an instance of {@link VehicleReservationRQCoreType.VehicleCharges }
*
* @return
* the new instance of {@link VehicleReservationRQCoreType.VehicleCharges }
*/
public VehicleReservationRQCoreType.VehicleCharges createVehicleReservationRQCoreTypeVehicleCharges() {
return new VehicleReservationRQCoreType.VehicleCharges();
}
/**
* Create an instance of {@link VehicleReservationRQCoreType.RateDistance }
*
* @return
* the new instance of {@link VehicleReservationRQCoreType.RateDistance }
*/
public VehicleReservationRQCoreType.RateDistance createVehicleReservationRQCoreTypeRateDistance() {
return new VehicleReservationRQCoreType.RateDistance();
}
/**
* Create an instance of {@link VehicleReservationRQCoreType.TotalCharge }
*
* @return
* the new instance of {@link VehicleReservationRQCoreType.TotalCharge }
*/
public VehicleReservationRQCoreType.TotalCharge createVehicleReservationRQCoreTypeTotalCharge() {
return new VehicleReservationRQCoreType.TotalCharge();
}
/**
* Create an instance of {@link VehicleReservationRQCoreType.Queue }
*
* @return
* the new instance of {@link VehicleReservationRQCoreType.Queue }
*/
public VehicleReservationRQCoreType.Queue createVehicleReservationRQCoreTypeQueue() {
return new VehicleReservationRQCoreType.Queue();
}
/**
* Create an instance of {@link VehicleReservationRQCoreType.SpecialEquipPrefs.SpecialEquipPref }
*
* @return
* the new instance of {@link VehicleReservationRQCoreType.SpecialEquipPrefs.SpecialEquipPref }
*/
public VehicleReservationRQCoreType.SpecialEquipPrefs.SpecialEquipPref createVehicleReservationRQCoreTypeSpecialEquipPrefsSpecialEquipPref() {
return new VehicleReservationRQCoreType.SpecialEquipPrefs.SpecialEquipPref();
}
/**
* Create an instance of {@link VehicleReservationRQAdditionalInfoType.RentalPaymentPref }
*
* @return
* the new instance of {@link VehicleReservationRQAdditionalInfoType.RentalPaymentPref }
*/
public VehicleReservationRQAdditionalInfoType.RentalPaymentPref createVehicleReservationRQAdditionalInfoTypeRentalPaymentPref() {
return new VehicleReservationRQAdditionalInfoType.RentalPaymentPref();
}
/**
* Create an instance of {@link VehicleReservationRQAdditionalInfoType.Reference }
*
* @return
* the new instance of {@link VehicleReservationRQAdditionalInfoType.Reference }
*/
public VehicleReservationRQAdditionalInfoType.Reference createVehicleReservationRQAdditionalInfoTypeReference() {
return new VehicleReservationRQAdditionalInfoType.Reference();
}
/**
* Create an instance of {@link PaymentDetailType.PaymentAmount }
*
* @return
* the new instance of {@link PaymentDetailType.PaymentAmount }
*/
public PaymentDetailType.PaymentAmount createPaymentDetailTypePaymentAmount() {
return new PaymentDetailType.PaymentAmount();
}
/**
* Create an instance of {@link VehicleReservationRQAdditionalInfoType.CoveragePrefs.CoveragePref }
*
* @return
* the new instance of {@link VehicleReservationRQAdditionalInfoType.CoveragePrefs.CoveragePref }
*/
public VehicleReservationRQAdditionalInfoType.CoveragePrefs.CoveragePref createVehicleReservationRQAdditionalInfoTypeCoveragePrefsCoveragePref() {
return new VehicleReservationRQAdditionalInfoType.CoveragePrefs.CoveragePref();
}
/**
* Create an instance of {@link VehicleRentalTransactionType.PickUpReturnDetails }
*
* @return
* the new instance of {@link VehicleRentalTransactionType.PickUpReturnDetails }
*/
public VehicleRentalTransactionType.PickUpReturnDetails createVehicleRentalTransactionTypePickUpReturnDetails() {
return new VehicleRentalTransactionType.PickUpReturnDetails();
}
/**
* Create an instance of {@link VehicleRentalTransactionType.Vehicle }
*
* @return
* the new instance of {@link VehicleRentalTransactionType.Vehicle }
*/
public VehicleRentalTransactionType.Vehicle createVehicleRentalTransactionTypeVehicle() {
return new VehicleRentalTransactionType.Vehicle();
}
/**
* Create an instance of {@link VehicleRentalTransactionType.Fees }
*
* @return
* the new instance of {@link VehicleRentalTransactionType.Fees }
*/
public VehicleRentalTransactionType.Fees createVehicleRentalTransactionTypeFees() {
return new VehicleRentalTransactionType.Fees();
}
/**
* Create an instance of {@link VehicleRentalTransactionType.TotalCharge }
*
* @return
* the new instance of {@link VehicleRentalTransactionType.TotalCharge }
*/
public VehicleRentalTransactionType.TotalCharge createVehicleRentalTransactionTypeTotalCharge() {
return new VehicleRentalTransactionType.TotalCharge();
}
/**
* Create an instance of {@link VehicleRentalTransactionType.PricedEquips.PricedEquip.Equipment }
*
* @return
* the new instance of {@link VehicleRentalTransactionType.PricedEquips.PricedEquip.Equipment }
*/
public VehicleRentalTransactionType.PricedEquips.PricedEquip.Equipment createVehicleRentalTransactionTypePricedEquipsPricedEquipEquipment() {
return new VehicleRentalTransactionType.PricedEquips.PricedEquip.Equipment();
}
/**
* Create an instance of {@link VehicleRentalCoreType.PickUpLocation }
*
* @return
* the new instance of {@link VehicleRentalCoreType.PickUpLocation }
*/
public VehicleRentalCoreType.PickUpLocation createVehicleRentalCoreTypePickUpLocation() {
return new VehicleRentalCoreType.PickUpLocation();
}
/**
* Create an instance of {@link VehicleRentalCoreType.ReturnLocation }
*
* @return
* the new instance of {@link VehicleRentalCoreType.ReturnLocation }
*/
public VehicleRentalCoreType.ReturnLocation createVehicleRentalCoreTypeReturnLocation() {
return new VehicleRentalCoreType.ReturnLocation();
}
/**
* Create an instance of {@link VehicleRentalRateType.RateDistance }
*
* @return
* the new instance of {@link VehicleRentalRateType.RateDistance }
*/
public VehicleRentalRateType.RateDistance createVehicleRentalRateTypeRateDistance() {
return new VehicleRentalRateType.RateDistance();
}
/**
* Create an instance of {@link VehicleRentalRateType.VehicleCharges }
*
* @return
* the new instance of {@link VehicleRentalRateType.VehicleCharges }
*/
public VehicleRentalRateType.VehicleCharges createVehicleRentalRateTypeVehicleCharges() {
return new VehicleRentalRateType.VehicleCharges();
}
/**
* Create an instance of {@link VehicleRentalRateType.RateQualifier }
*
* @return
* the new instance of {@link VehicleRentalRateType.RateQualifier }
*/
public VehicleRentalRateType.RateQualifier createVehicleRentalRateTypeRateQualifier() {
return new VehicleRentalRateType.RateQualifier();
}
/**
* Create an instance of {@link VehicleRentalRateType.RateRestrictions }
*
* @return
* the new instance of {@link VehicleRentalRateType.RateRestrictions }
*/
public VehicleRentalRateType.RateRestrictions createVehicleRentalRateTypeRateRestrictions() {
return new VehicleRentalRateType.RateRestrictions();
}
/**
* Create an instance of {@link VehicleRentalRateType.RateGuarantee }
*
* @return
* the new instance of {@link VehicleRentalRateType.RateGuarantee }
*/
public VehicleRentalRateType.RateGuarantee createVehicleRentalRateTypeRateGuarantee() {
return new VehicleRentalRateType.RateGuarantee();
}
/**
* Create an instance of {@link VehicleRentalRateType.PickupReturnRule }
*
* @return
* the new instance of {@link VehicleRentalRateType.PickupReturnRule }
*/
public VehicleRentalRateType.PickupReturnRule createVehicleRentalRateTypePickupReturnRule() {
return new VehicleRentalRateType.PickupReturnRule();
}
/**
* Create an instance of {@link RateQualifierType.RateComments.RateComment }
*
* @return
* the new instance of {@link RateQualifierType.RateComments.RateComment }
*/
public RateQualifierType.RateComments.RateComment createRateQualifierTypeRateCommentsRateComment() {
return new RateQualifierType.RateComments.RateComment();
}
/**
* Create an instance of {@link VehicleRentalDetailsType.FuelLevelDetails }
*
* @return
* the new instance of {@link VehicleRentalDetailsType.FuelLevelDetails }
*/
public VehicleRentalDetailsType.FuelLevelDetails createVehicleRentalDetailsTypeFuelLevelDetails() {
return new VehicleRentalDetailsType.FuelLevelDetails();
}
/**
* Create an instance of {@link VehicleRentalDetailsType.OdometerReading }
*
* @return
* the new instance of {@link VehicleRentalDetailsType.OdometerReading }
*/
public VehicleRentalDetailsType.OdometerReading createVehicleRentalDetailsTypeOdometerReading() {
return new VehicleRentalDetailsType.OdometerReading();
}
/**
* Create an instance of {@link VehicleRentalDetailsType.ConditionReport }
*
* @return
* the new instance of {@link VehicleRentalDetailsType.ConditionReport }
*/
public VehicleRentalDetailsType.ConditionReport createVehicleRentalDetailsTypeConditionReport() {
return new VehicleRentalDetailsType.ConditionReport();
}
/**
* Create an instance of {@link VehicleProfileRentalPrefType.LoyaltyPref }
*
* @return
* the new instance of {@link VehicleProfileRentalPrefType.LoyaltyPref }
*/
public VehicleProfileRentalPrefType.LoyaltyPref createVehicleProfileRentalPrefTypeLoyaltyPref() {
return new VehicleProfileRentalPrefType.LoyaltyPref();
}
/**
* Create an instance of {@link VehicleProfileRentalPrefType.PaymentFormPref }
*
* @return
* the new instance of {@link VehicleProfileRentalPrefType.PaymentFormPref }
*/
public VehicleProfileRentalPrefType.PaymentFormPref createVehicleProfileRentalPrefTypePaymentFormPref() {
return new VehicleProfileRentalPrefType.PaymentFormPref();
}
/**
* Create an instance of {@link VehicleProfileRentalPrefType.CoveragePref }
*
* @return
* the new instance of {@link VehicleProfileRentalPrefType.CoveragePref }
*/
public VehicleProfileRentalPrefType.CoveragePref createVehicleProfileRentalPrefTypeCoveragePref() {
return new VehicleProfileRentalPrefType.CoveragePref();
}
/**
* Create an instance of {@link VehicleProfileRentalPrefType.SpecialEquipPref }
*
* @return
* the new instance of {@link VehicleProfileRentalPrefType.SpecialEquipPref }
*/
public VehicleProfileRentalPrefType.SpecialEquipPref createVehicleProfileRentalPrefTypeSpecialEquipPref() {
return new VehicleProfileRentalPrefType.SpecialEquipPref();
}
/**
* Create an instance of {@link VehiclePrefType.VehMakeModel }
*
* @return
* the new instance of {@link VehiclePrefType.VehMakeModel }
*/
public VehiclePrefType.VehMakeModel createVehiclePrefTypeVehMakeModel() {
return new VehiclePrefType.VehMakeModel();
}
/**
* Create an instance of {@link VehicleLocationVehiclesType.Vehicle }
*
* @return
* the new instance of {@link VehicleLocationVehiclesType.Vehicle }
*/
public VehicleLocationVehiclesType.Vehicle createVehicleLocationVehiclesTypeVehicle() {
return new VehicleLocationVehiclesType.Vehicle();
}
/**
* Create an instance of {@link VehicleLocationVehiclesType.VehicleInfos.VehicleInfo }
*
* @return
* the new instance of {@link VehicleLocationVehiclesType.VehicleInfos.VehicleInfo }
*/
public VehicleLocationVehiclesType.VehicleInfos.VehicleInfo createVehicleLocationVehiclesTypeVehicleInfosVehicleInfo() {
return new VehicleLocationVehiclesType.VehicleInfos.VehicleInfo();
}
/**
* Create an instance of {@link VehicleLocationLiabilitiesType.Coverages.Coverage.CoverageFees.CoverageFee.Vehicles.Vehicle }
*
* @return
* the new instance of {@link VehicleLocationLiabilitiesType.Coverages.Coverage.CoverageFees.CoverageFee.Vehicles.Vehicle }
*/
public VehicleLocationLiabilitiesType.Coverages.Coverage.CoverageFees.CoverageFee.Vehicles.Vehicle createVehicleLocationLiabilitiesTypeCoveragesCoverageCoverageFeesCoverageFeeVehiclesVehicle() {
return new VehicleLocationLiabilitiesType.Coverages.Coverage.CoverageFees.CoverageFee.Vehicles.Vehicle();
}
/**
* Create an instance of {@link VehicleLocationDetailsType.Telephone }
*
* @return
* the new instance of {@link VehicleLocationDetailsType.Telephone }
*/
public VehicleLocationDetailsType.Telephone createVehicleLocationDetailsTypeTelephone() {
return new VehicleLocationDetailsType.Telephone();
}
/**
* Create an instance of {@link VehicleLocationAdditionalFeesType.MiscellaneousCharges.MiscellaneousCharge }
*
* @return
* the new instance of {@link VehicleLocationAdditionalFeesType.MiscellaneousCharges.MiscellaneousCharge }
*/
public VehicleLocationAdditionalFeesType.MiscellaneousCharges.MiscellaneousCharge createVehicleLocationAdditionalFeesTypeMiscellaneousChargesMiscellaneousCharge() {
return new VehicleLocationAdditionalFeesType.MiscellaneousCharges.MiscellaneousCharge();
}
/**
* Create an instance of {@link VehicleChargeType.MinMax }
*
* @return
* the new instance of {@link VehicleChargeType.MinMax }
*/
public VehicleChargeType.MinMax createVehicleChargeTypeMinMax() {
return new VehicleChargeType.MinMax();
}
/**
* Create an instance of {@link VehicleChargeType.Calculation }
*
* @return
* the new instance of {@link VehicleChargeType.Calculation }
*/
public VehicleChargeType.Calculation createVehicleChargeTypeCalculation() {
return new VehicleChargeType.Calculation();
}
/**
* Create an instance of {@link VehicleChargeType.TaxAmounts.TaxAmount }
*
* @return
* the new instance of {@link VehicleChargeType.TaxAmounts.TaxAmount }
*/
public VehicleChargeType.TaxAmounts.TaxAmount createVehicleChargeTypeTaxAmountsTaxAmount() {
return new VehicleChargeType.TaxAmounts.TaxAmount();
}
/**
* Create an instance of {@link VehicleLocationAdditionalFeesType.Surcharges.Surcharge }
*
* @return
* the new instance of {@link VehicleLocationAdditionalFeesType.Surcharges.Surcharge }
*/
public VehicleLocationAdditionalFeesType.Surcharges.Surcharge createVehicleLocationAdditionalFeesTypeSurchargesSurcharge() {
return new VehicleLocationAdditionalFeesType.Surcharges.Surcharge();
}
/**
* Create an instance of {@link VehicleLocationAdditionalFeesType.Fees.Fee }
*
* @return
* the new instance of {@link VehicleLocationAdditionalFeesType.Fees.Fee }
*/
public VehicleLocationAdditionalFeesType.Fees.Fee createVehicleLocationAdditionalFeesTypeFeesFee() {
return new VehicleLocationAdditionalFeesType.Fees.Fee();
}
/**
* Create an instance of {@link VehicleLocationAdditionalFeesType.Taxes.Tax }
*
* @return
* the new instance of {@link VehicleLocationAdditionalFeesType.Taxes.Tax }
*/
public VehicleLocationAdditionalFeesType.Taxes.Tax createVehicleLocationAdditionalFeesTypeTaxesTax() {
return new VehicleLocationAdditionalFeesType.Taxes.Tax();
}
/**
* Create an instance of {@link VehicleLocationAdditionalDetailsType.VehRentLocInfos }
*
* @return
* the new instance of {@link VehicleLocationAdditionalDetailsType.VehRentLocInfos }
*/
public VehicleLocationAdditionalDetailsType.VehRentLocInfos createVehicleLocationAdditionalDetailsTypeVehRentLocInfos() {
return new VehicleLocationAdditionalDetailsType.VehRentLocInfos();
}
/**
* Create an instance of {@link VehicleLocationAdditionalDetailsType.OneWayDropLocations.OneWayDropLocation }
*
* @return
* the new instance of {@link VehicleLocationAdditionalDetailsType.OneWayDropLocations.OneWayDropLocation }
*/
public VehicleLocationAdditionalDetailsType.OneWayDropLocations.OneWayDropLocation createVehicleLocationAdditionalDetailsTypeOneWayDropLocationsOneWayDropLocation() {
return new VehicleLocationAdditionalDetailsType.OneWayDropLocations.OneWayDropLocation();
}
/**
* Create an instance of {@link VehicleLocationAdditionalDetailsType.Shuttle.ShuttleInfos.ShuttleInfo }
*
* @return
* the new instance of {@link VehicleLocationAdditionalDetailsType.Shuttle.ShuttleInfos.ShuttleInfo }
*/
public VehicleLocationAdditionalDetailsType.Shuttle.ShuttleInfos.ShuttleInfo createVehicleLocationAdditionalDetailsTypeShuttleShuttleInfosShuttleInfo() {
return new VehicleLocationAdditionalDetailsType.Shuttle.ShuttleInfos.ShuttleInfo();
}
/**
* Create an instance of {@link VehicleAvailVendorInfoType.TourInfo }
*
* @return
* the new instance of {@link VehicleAvailVendorInfoType.TourInfo }
*/
public VehicleAvailVendorInfoType.TourInfo createVehicleAvailVendorInfoTypeTourInfo() {
return new VehicleAvailVendorInfoType.TourInfo();
}
/**
* Create an instance of {@link VehicleAvailRSCoreType.VehVendorAvails }
*
* @return
* the new instance of {@link VehicleAvailRSCoreType.VehVendorAvails }
*/
public VehicleAvailRSCoreType.VehVendorAvails createVehicleAvailRSCoreTypeVehVendorAvails() {
return new VehicleAvailRSCoreType.VehVendorAvails();
}
/**
* Create an instance of {@link VehicleAvailRQCoreType.DriverType }
*
* @return
* the new instance of {@link VehicleAvailRQCoreType.DriverType }
*/
public VehicleAvailRQCoreType.DriverType createVehicleAvailRQCoreTypeDriverType() {
return new VehicleAvailRQCoreType.DriverType();
}
/**
* Create an instance of {@link VehicleAvailRQCoreType.RateQualifier }
*
* @return
* the new instance of {@link VehicleAvailRQCoreType.RateQualifier }
*/
public VehicleAvailRQCoreType.RateQualifier createVehicleAvailRQCoreTypeRateQualifier() {
return new VehicleAvailRQCoreType.RateQualifier();
}
/**
* Create an instance of {@link VehicleAvailRQCoreType.RateRange }
*
* @return
* the new instance of {@link VehicleAvailRQCoreType.RateRange }
*/
public VehicleAvailRQCoreType.RateRange createVehicleAvailRQCoreTypeRateRange() {
return new VehicleAvailRQCoreType.RateRange();
}
/**
* Create an instance of {@link VehicleAvailRQCoreType.SpecialEquipPrefs.SpecialEquipPref }
*
* @return
* the new instance of {@link VehicleAvailRQCoreType.SpecialEquipPrefs.SpecialEquipPref }
*/
public VehicleAvailRQCoreType.SpecialEquipPrefs.SpecialEquipPref createVehicleAvailRQCoreTypeSpecialEquipPrefsSpecialEquipPref() {
return new VehicleAvailRQCoreType.SpecialEquipPrefs.SpecialEquipPref();
}
/**
* Create an instance of {@link VehicleAvailRQCoreType.VehPrefs.VehPref }
*
* @return
* the new instance of {@link VehicleAvailRQCoreType.VehPrefs.VehPref }
*/
public VehicleAvailRQCoreType.VehPrefs.VehPref createVehicleAvailRQCoreTypeVehPrefsVehPref() {
return new VehicleAvailRQCoreType.VehPrefs.VehPref();
}
/**
* Create an instance of {@link VehicleAvailRQCoreType.VendorPrefs.VendorPref }
*
* @return
* the new instance of {@link VehicleAvailRQCoreType.VendorPrefs.VendorPref }
*/
public VehicleAvailRQCoreType.VendorPrefs.VendorPref createVehicleAvailRQCoreTypeVendorPrefsVendorPref() {
return new VehicleAvailRQCoreType.VendorPrefs.VendorPref();
}
/**
* Create an instance of {@link VehicleAvailRQAdditionalInfoType.CoveragePrefs.CoveragePref }
*
* @return
* the new instance of {@link VehicleAvailRQAdditionalInfoType.CoveragePrefs.CoveragePref }
*/
public VehicleAvailRQAdditionalInfoType.CoveragePrefs.CoveragePref createVehicleAvailRQAdditionalInfoTypeCoveragePrefsCoveragePref() {
return new VehicleAvailRQAdditionalInfoType.CoveragePrefs.CoveragePref();
}
/**
* Create an instance of {@link VehicleAvailCoreType.TotalCharge }
*
* @return
* the new instance of {@link VehicleAvailCoreType.TotalCharge }
*/
public VehicleAvailCoreType.TotalCharge createVehicleAvailCoreTypeTotalCharge() {
return new VehicleAvailCoreType.TotalCharge();
}
/**
* Create an instance of {@link VehicleAvailCoreType.PricedEquips }
*
* @return
* the new instance of {@link VehicleAvailCoreType.PricedEquips }
*/
public VehicleAvailCoreType.PricedEquips createVehicleAvailCoreTypePricedEquips() {
return new VehicleAvailCoreType.PricedEquips();
}
/**
* Create an instance of {@link VehicleAvailCoreType.Fees }
*
* @return
* the new instance of {@link VehicleAvailCoreType.Fees }
*/
public VehicleAvailCoreType.Fees createVehicleAvailCoreTypeFees() {
return new VehicleAvailCoreType.Fees();
}
/**
* Create an instance of {@link VehicleAvailCoreType.Reference }
*
* @return
* the new instance of {@link VehicleAvailCoreType.Reference }
*/
public VehicleAvailCoreType.Reference createVehicleAvailCoreTypeReference() {
return new VehicleAvailCoreType.Reference();
}
/**
* Create an instance of {@link VehicleAvailCoreType.Vendor }
*
* @return
* the new instance of {@link VehicleAvailCoreType.Vendor }
*/
public VehicleAvailCoreType.Vendor createVehicleAvailCoreTypeVendor() {
return new VehicleAvailCoreType.Vendor();
}
/**
* Create an instance of {@link VehicleAvailCoreType.VendorLocation }
*
* @return
* the new instance of {@link VehicleAvailCoreType.VendorLocation }
*/
public VehicleAvailCoreType.VendorLocation createVehicleAvailCoreTypeVendorLocation() {
return new VehicleAvailCoreType.VendorLocation();
}
/**
* Create an instance of {@link VehicleAvailCoreType.DropOffLocation }
*
* @return
* the new instance of {@link VehicleAvailCoreType.DropOffLocation }
*/
public VehicleAvailCoreType.DropOffLocation createVehicleAvailCoreTypeDropOffLocation() {
return new VehicleAvailCoreType.DropOffLocation();
}
/**
* Create an instance of {@link VehicleAvailCoreType.Discount }
*
* @return
* the new instance of {@link VehicleAvailCoreType.Discount }
*/
public VehicleAvailCoreType.Discount createVehicleAvailCoreTypeDiscount() {
return new VehicleAvailCoreType.Discount();
}
/**
* Create an instance of {@link VehicleAvailAdditionalInfoType.PricedCoverages }
*
* @return
* the new instance of {@link VehicleAvailAdditionalInfoType.PricedCoverages }
*/
public VehicleAvailAdditionalInfoType.PricedCoverages createVehicleAvailAdditionalInfoTypePricedCoverages() {
return new VehicleAvailAdditionalInfoType.PricedCoverages();
}
/**
* Create an instance of {@link VehicleAgeRequirementsType.Age.AgeSurcharge }
*
* @return
* the new instance of {@link VehicleAgeRequirementsType.Age.AgeSurcharge }
*/
public VehicleAgeRequirementsType.Age.AgeSurcharge createVehicleAgeRequirementsTypeAgeAgeSurcharge() {
return new VehicleAgeRequirementsType.Age.AgeSurcharge();
}
/**
* Create an instance of {@link VehicleAgeRequirementsType.Age.Vehicles.Vehicle }
*
* @return
* the new instance of {@link VehicleAgeRequirementsType.Age.Vehicles.Vehicle }
*/
public VehicleAgeRequirementsType.Age.Vehicles.Vehicle createVehicleAgeRequirementsTypeAgeVehiclesVehicle() {
return new VehicleAgeRequirementsType.Age.Vehicles.Vehicle();
}
/**
* Create an instance of {@link VehicleAgeRequirementsType.Age.AgeInfos.AgeInfo }
*
* @return
* the new instance of {@link VehicleAgeRequirementsType.Age.AgeInfos.AgeInfo }
*/
public VehicleAgeRequirementsType.Age.AgeInfos.AgeInfo createVehicleAgeRequirementsTypeAgeAgeInfosAgeInfo() {
return new VehicleAgeRequirementsType.Age.AgeInfos.AgeInfo();
}
/**
* Create an instance of {@link VehicleAdditionalDriverRequirementsType.AddlDriverInfos.AddlDriverInfo }
*
* @return
* the new instance of {@link VehicleAdditionalDriverRequirementsType.AddlDriverInfos.AddlDriverInfo }
*/
public VehicleAdditionalDriverRequirementsType.AddlDriverInfos.AddlDriverInfo createVehicleAdditionalDriverRequirementsTypeAddlDriverInfosAddlDriverInfo() {
return new VehicleAdditionalDriverRequirementsType.AddlDriverInfos.AddlDriverInfo();
}
/**
* Create an instance of {@link VehicleAdditionalDriverRequirementsType.AddlDriverInfos.Vehicles.Vehicle }
*
* @return
* the new instance of {@link VehicleAdditionalDriverRequirementsType.AddlDriverInfos.Vehicles.Vehicle }
*/
public VehicleAdditionalDriverRequirementsType.AddlDriverInfos.Vehicles.Vehicle createVehicleAdditionalDriverRequirementsTypeAddlDriverInfosVehiclesVehicle() {
return new VehicleAdditionalDriverRequirementsType.AddlDriverInfos.Vehicles.Vehicle();
}
/**
* Create an instance of {@link RateRulesType.AdvanceBooking }
*
* @return
* the new instance of {@link RateRulesType.AdvanceBooking }
*/
public RateRulesType.AdvanceBooking createRateRulesTypeAdvanceBooking() {
return new RateRulesType.AdvanceBooking();
}
/**
* Create an instance of {@link RateRulesType.RateGuarantee }
*
* @return
* the new instance of {@link RateRulesType.RateGuarantee }
*/
public RateRulesType.RateGuarantee createRateRulesTypeRateGuarantee() {
return new RateRulesType.RateGuarantee();
}
/**
* Create an instance of {@link RateRulesType.RateDeposit }
*
* @return
* the new instance of {@link RateRulesType.RateDeposit }
*/
public RateRulesType.RateDeposit createRateRulesTypeRateDeposit() {
return new RateRulesType.RateDeposit();
}
/**
* Create an instance of {@link RateRulesType.CancelPenaltyInfo.Deadline }
*
* @return
* the new instance of {@link RateRulesType.CancelPenaltyInfo.Deadline }
*/
public RateRulesType.CancelPenaltyInfo.Deadline createRateRulesTypeCancelPenaltyInfoDeadline() {
return new RateRulesType.CancelPenaltyInfo.Deadline();
}
/**
* Create an instance of {@link RateRulesType.CancelPenaltyInfo.PenaltyFee }
*
* @return
* the new instance of {@link RateRulesType.CancelPenaltyInfo.PenaltyFee }
*/
public RateRulesType.CancelPenaltyInfo.PenaltyFee createRateRulesTypeCancelPenaltyInfoPenaltyFee() {
return new RateRulesType.CancelPenaltyInfo.PenaltyFee();
}
/**
* Create an instance of {@link RateRulesType.PaymentRules.AcceptablePayments.AcceptablePayment }
*
* @return
* the new instance of {@link RateRulesType.PaymentRules.AcceptablePayments.AcceptablePayment }
*/
public RateRulesType.PaymentRules.AcceptablePayments.AcceptablePayment createRateRulesTypePaymentRulesAcceptablePaymentsAcceptablePayment() {
return new RateRulesType.PaymentRules.AcceptablePayments.AcceptablePayment();
}
/**
* Create an instance of {@link RateRulesType.PickupReturnRules.EarliestPickup }
*
* @return
* the new instance of {@link RateRulesType.PickupReturnRules.EarliestPickup }
*/
public RateRulesType.PickupReturnRules.EarliestPickup createRateRulesTypePickupReturnRulesEarliestPickup() {
return new RateRulesType.PickupReturnRules.EarliestPickup();
}
/**
* Create an instance of {@link RateRulesType.PickupReturnRules.LatestPickup }
*
* @return
* the new instance of {@link RateRulesType.PickupReturnRules.LatestPickup }
*/
public RateRulesType.PickupReturnRules.LatestPickup createRateRulesTypePickupReturnRulesLatestPickup() {
return new RateRulesType.PickupReturnRules.LatestPickup();
}
/**
* Create an instance of {@link RateRulesType.PickupReturnRules.LatestReturn }
*
* @return
* the new instance of {@link RateRulesType.PickupReturnRules.LatestReturn }
*/
public RateRulesType.PickupReturnRules.LatestReturn createRateRulesTypePickupReturnRulesLatestReturn() {
return new RateRulesType.PickupReturnRules.LatestReturn();
}
/**
* Create an instance of {@link RateRulesType.PickupReturnRules.EarliestReturn }
*
* @return
* the new instance of {@link RateRulesType.PickupReturnRules.EarliestReturn }
*/
public RateRulesType.PickupReturnRules.EarliestReturn createRateRulesTypePickupReturnRulesEarliestReturn() {
return new RateRulesType.PickupReturnRules.EarliestReturn();
}
/**
* Create an instance of {@link OffLocationServiceCoreType.Address }
*
* @return
* the new instance of {@link OffLocationServiceCoreType.Address }
*/
public OffLocationServiceCoreType.Address createOffLocationServiceCoreTypeAddress() {
return new OffLocationServiceCoreType.Address();
}
/**
* Create an instance of {@link OffLocationServiceType.Telephone }
*
* @return
* the new instance of {@link OffLocationServiceType.Telephone }
*/
public OffLocationServiceType.Telephone createOffLocationServiceTypeTelephone() {
return new OffLocationServiceType.Telephone();
}
/**
* Create an instance of {@link NoShowFeeType.Deadline }
*
* @return
* the new instance of {@link NoShowFeeType.Deadline }
*/
public NoShowFeeType.Deadline createNoShowFeeTypeDeadline() {
return new NoShowFeeType.Deadline();
}
/**
* Create an instance of {@link NoShowFeeType.GracePeriod }
*
* @return
* the new instance of {@link NoShowFeeType.GracePeriod }
*/
public NoShowFeeType.GracePeriod createNoShowFeeTypeGracePeriod() {
return new NoShowFeeType.GracePeriod();
}
/**
* Create an instance of {@link NoShowFeeType.FeeAmount }
*
* @return
* the new instance of {@link NoShowFeeType.FeeAmount }
*/
public NoShowFeeType.FeeAmount createNoShowFeeTypeFeeAmount() {
return new NoShowFeeType.FeeAmount();
}
/**
* Create an instance of {@link CustomerPrimaryAdditionalType.Primary }
*
* @return
* the new instance of {@link CustomerPrimaryAdditionalType.Primary }
*/
public CustomerPrimaryAdditionalType.Primary createCustomerPrimaryAdditionalTypePrimary() {
return new CustomerPrimaryAdditionalType.Primary();
}
/**
* Create an instance of {@link CustomerPrimaryAdditionalType.Additional }
*
* @return
* the new instance of {@link CustomerPrimaryAdditionalType.Additional }
*/
public CustomerPrimaryAdditionalType.Additional createCustomerPrimaryAdditionalTypeAdditional() {
return new CustomerPrimaryAdditionalType.Additional();
}
/**
* Create an instance of {@link CustomerType.Telephone }
*
* @return
* the new instance of {@link CustomerType.Telephone }
*/
public CustomerType.Telephone createCustomerTypeTelephone() {
return new CustomerType.Telephone();
}
/**
* Create an instance of {@link CustomerType.Email }
*
* @return
* the new instance of {@link CustomerType.Email }
*/
public CustomerType.Email createCustomerTypeEmail() {
return new CustomerType.Email();
}
/**
* Create an instance of {@link CustomerType.Address }
*
* @return
* the new instance of {@link CustomerType.Address }
*/
public CustomerType.Address createCustomerTypeAddress() {
return new CustomerType.Address();
}
/**
* Create an instance of {@link CustomerType.URL }
*
* @return
* the new instance of {@link CustomerType.URL }
*/
public CustomerType.URL createCustomerTypeURL() {
return new CustomerType.URL();
}
/**
* Create an instance of {@link CustomerType.CitizenCountryName }
*
* @return
* the new instance of {@link CustomerType.CitizenCountryName }
*/
public CustomerType.CitizenCountryName createCustomerTypeCitizenCountryName() {
return new CustomerType.CitizenCountryName();
}
/**
* Create an instance of {@link CustomerType.PhysChallName }
*
* @return
* the new instance of {@link CustomerType.PhysChallName }
*/
public CustomerType.PhysChallName createCustomerTypePhysChallName() {
return new CustomerType.PhysChallName();
}
/**
* Create an instance of {@link CustomerType.AdditionalLanguage }
*
* @return
* the new instance of {@link CustomerType.AdditionalLanguage }
*/
public CustomerType.AdditionalLanguage createCustomerTypeAdditionalLanguage() {
return new CustomerType.AdditionalLanguage();
}
/**
* Create an instance of {@link CustomerType.CustLoyalty.SubAccountBalance }
*
* @return
* the new instance of {@link CustomerType.CustLoyalty.SubAccountBalance }
*/
public CustomerType.CustLoyalty.SubAccountBalance createCustomerTypeCustLoyaltySubAccountBalance() {
return new CustomerType.CustLoyalty.SubAccountBalance();
}
/**
* Create an instance of {@link CustomerType.CustLoyalty.SecurityInfo.PasswordHint }
*
* @return
* the new instance of {@link CustomerType.CustLoyalty.SecurityInfo.PasswordHint }
*/
public CustomerType.CustLoyalty.SecurityInfo.PasswordHint createCustomerTypeCustLoyaltySecurityInfoPasswordHint() {
return new CustomerType.CustLoyalty.SecurityInfo.PasswordHint();
}
/**
* Create an instance of {@link CustomerType.CustLoyalty.MemberPreferences.AdditionalReward }
*
* @return
* the new instance of {@link CustomerType.CustLoyalty.MemberPreferences.AdditionalReward }
*/
public CustomerType.CustLoyalty.MemberPreferences.AdditionalReward createCustomerTypeCustLoyaltyMemberPreferencesAdditionalReward() {
return new CustomerType.CustLoyalty.MemberPreferences.AdditionalReward();
}
/**
* Create an instance of {@link CustomerType.CustLoyalty.MemberPreferences.Offer.Communication }
*
* @return
* the new instance of {@link CustomerType.CustLoyalty.MemberPreferences.Offer.Communication }
*/
public CustomerType.CustLoyalty.MemberPreferences.Offer.Communication createCustomerTypeCustLoyaltyMemberPreferencesOfferCommunication() {
return new CustomerType.CustLoyalty.MemberPreferences.Offer.Communication();
}
/**
* Create an instance of {@link CustomerType.PaymentForm.AssociatedSupplier }
*
* @return
* the new instance of {@link CustomerType.PaymentForm.AssociatedSupplier }
*/
public CustomerType.PaymentForm.AssociatedSupplier createCustomerTypePaymentFormAssociatedSupplier() {
return new CustomerType.PaymentForm.AssociatedSupplier();
}
/**
* Create an instance of {@link AirSearchPrefsType.VendorPref }
*
* @return
* the new instance of {@link AirSearchPrefsType.VendorPref }
*/
public AirSearchPrefsType.VendorPref createAirSearchPrefsTypeVendorPref() {
return new AirSearchPrefsType.VendorPref();
}
/**
* Create an instance of {@link AirSearchPrefsType.FlightTypePref }
*
* @return
* the new instance of {@link AirSearchPrefsType.FlightTypePref }
*/
public AirSearchPrefsType.FlightTypePref createAirSearchPrefsTypeFlightTypePref() {
return new AirSearchPrefsType.FlightTypePref();
}
/**
* Create an instance of {@link AirSearchPrefsType.FareRestrictPref }
*
* @return
* the new instance of {@link AirSearchPrefsType.FareRestrictPref }
*/
public AirSearchPrefsType.FareRestrictPref createAirSearchPrefsTypeFareRestrictPref() {
return new AirSearchPrefsType.FareRestrictPref();
}
/**
* Create an instance of {@link AirSearchPrefsType.CabinPref }
*
* @return
* the new instance of {@link AirSearchPrefsType.CabinPref }
*/
public AirSearchPrefsType.CabinPref createAirSearchPrefsTypeCabinPref() {
return new AirSearchPrefsType.CabinPref();
}
/**
* Create an instance of {@link AirSearchPrefsType.TicketDistribPref }
*
* @return
* the new instance of {@link AirSearchPrefsType.TicketDistribPref }
*/
public AirSearchPrefsType.TicketDistribPref createAirSearchPrefsTypeTicketDistribPref() {
return new AirSearchPrefsType.TicketDistribPref();
}
/**
* Create an instance of {@link AirSearchPrefsType.BookingSeatPref }
*
* @return
* the new instance of {@link AirSearchPrefsType.BookingSeatPref }
*/
public AirSearchPrefsType.BookingSeatPref createAirSearchPrefsTypeBookingSeatPref() {
return new AirSearchPrefsType.BookingSeatPref();
}
/**
* Create an instance of {@link AirlinePrefType.VendorPref }
*
* @return
* the new instance of {@link AirlinePrefType.VendorPref }
*/
public AirlinePrefType.VendorPref createAirlinePrefTypeVendorPref() {
return new AirlinePrefType.VendorPref();
}
/**
* Create an instance of {@link AirlinePrefType.FareRestrictPref }
*
* @return
* the new instance of {@link AirlinePrefType.FareRestrictPref }
*/
public AirlinePrefType.FareRestrictPref createAirlinePrefTypeFareRestrictPref() {
return new AirlinePrefType.FareRestrictPref();
}
/**
* Create an instance of {@link AirlinePrefType.FarePref }
*
* @return
* the new instance of {@link AirlinePrefType.FarePref }
*/
public AirlinePrefType.FarePref createAirlinePrefTypeFarePref() {
return new AirlinePrefType.FarePref();
}
/**
* Create an instance of {@link AirlinePrefType.FlightTypePref }
*
* @return
* the new instance of {@link AirlinePrefType.FlightTypePref }
*/
public AirlinePrefType.FlightTypePref createAirlinePrefTypeFlightTypePref() {
return new AirlinePrefType.FlightTypePref();
}
/**
* Create an instance of {@link AirlinePrefType.CabinPref }
*
* @return
* the new instance of {@link AirlinePrefType.CabinPref }
*/
public AirlinePrefType.CabinPref createAirlinePrefTypeCabinPref() {
return new AirlinePrefType.CabinPref();
}
/**
* Create an instance of {@link AirlinePrefType.SeatPref }
*
* @return
* the new instance of {@link AirlinePrefType.SeatPref }
*/
public AirlinePrefType.SeatPref createAirlinePrefTypeSeatPref() {
return new AirlinePrefType.SeatPref();
}
/**
* Create an instance of {@link AirlinePrefType.SSRPref }
*
* @return
* the new instance of {@link AirlinePrefType.SSRPref }
*/
public AirlinePrefType.SSRPref createAirlinePrefTypeSSRPref() {
return new AirlinePrefType.SSRPref();
}
/**
* Create an instance of {@link AirlinePrefType.KeywordPref }
*
* @return
* the new instance of {@link AirlinePrefType.KeywordPref }
*/
public AirlinePrefType.KeywordPref createAirlinePrefTypeKeywordPref() {
return new AirlinePrefType.KeywordPref();
}
/**
* Create an instance of {@link AirlinePrefType.AccountInformation.TaxRegistrationDetails }
*
* @return
* the new instance of {@link AirlinePrefType.AccountInformation.TaxRegistrationDetails }
*/
public AirlinePrefType.AccountInformation.TaxRegistrationDetails createAirlinePrefTypeAccountInformationTaxRegistrationDetails() {
return new AirlinePrefType.AccountInformation.TaxRegistrationDetails();
}
/**
* Create an instance of {@link AirlinePrefType.TourCodePref.TourCodeInfo }
*
* @return
* the new instance of {@link AirlinePrefType.TourCodePref.TourCodeInfo }
*/
public AirlinePrefType.TourCodePref.TourCodeInfo createAirlinePrefTypeTourCodePrefTourCodeInfo() {
return new AirlinePrefType.TourCodePref.TourCodeInfo();
}
/**
* Create an instance of {@link AirlinePrefType.TourCodePref.StaffTourCodeInfo }
*
* @return
* the new instance of {@link AirlinePrefType.TourCodePref.StaffTourCodeInfo }
*/
public AirlinePrefType.TourCodePref.StaffTourCodeInfo createAirlinePrefTypeTourCodePrefStaffTourCodeInfo() {
return new AirlinePrefType.TourCodePref.StaffTourCodeInfo();
}
/**
* Create an instance of {@link PhonePrefType.Telephone }
*
* @return
* the new instance of {@link PhonePrefType.Telephone }
*/
public PhonePrefType.Telephone createPhonePrefTypeTelephone() {
return new PhonePrefType.Telephone();
}
/**
* Create an instance of {@link CommonPrefType.ContactPref }
*
* @return
* the new instance of {@link CommonPrefType.ContactPref }
*/
public CommonPrefType.ContactPref createCommonPrefTypeContactPref() {
return new CommonPrefType.ContactPref();
}
/**
* Create an instance of {@link VoluntaryChangesType.Penalty }
*
* @return
* the new instance of {@link VoluntaryChangesType.Penalty }
*/
public VoluntaryChangesType.Penalty createVoluntaryChangesTypePenalty() {
return new VoluntaryChangesType.Penalty();
}
/**
* Create an instance of {@link AirTravelerType.ProfileRef }
*
* @return
* the new instance of {@link AirTravelerType.ProfileRef }
*/
public AirTravelerType.ProfileRef createAirTravelerTypeProfileRef() {
return new AirTravelerType.ProfileRef();
}
/**
* Create an instance of {@link AirTravelerType.Telephone }
*
* @return
* the new instance of {@link AirTravelerType.Telephone }
*/
public AirTravelerType.Telephone createAirTravelerTypeTelephone() {
return new AirTravelerType.Telephone();
}
/**
* Create an instance of {@link AirTravelerType.Email }
*
* @return
* the new instance of {@link AirTravelerType.Email }
*/
public AirTravelerType.Email createAirTravelerTypeEmail() {
return new AirTravelerType.Email();
}
/**
* Create an instance of {@link AirTravelerType.Address }
*
* @return
* the new instance of {@link AirTravelerType.Address }
*/
public AirTravelerType.Address createAirTravelerTypeAddress() {
return new AirTravelerType.Address();
}
/**
* Create an instance of {@link AirTravelerType.CustLoyalty }
*
* @return
* the new instance of {@link AirTravelerType.CustLoyalty }
*/
public AirTravelerType.CustLoyalty createAirTravelerTypeCustLoyalty() {
return new AirTravelerType.CustLoyalty();
}
/**
* Create an instance of {@link AirTravelerType.Document }
*
* @return
* the new instance of {@link AirTravelerType.Document }
*/
public AirTravelerType.Document createAirTravelerTypeDocument() {
return new AirTravelerType.Document();
}
/**
* Create an instance of {@link AirTravelerType.TravelerRefNumber }
*
* @return
* the new instance of {@link AirTravelerType.TravelerRefNumber }
*/
public AirTravelerType.TravelerRefNumber createAirTravelerTypeTravelerRefNumber() {
return new AirTravelerType.TravelerRefNumber();
}
/**
* Create an instance of {@link AirTravelerType.FlightSegmentRPHs }
*
* @return
* the new instance of {@link AirTravelerType.FlightSegmentRPHs }
*/
public AirTravelerType.FlightSegmentRPHs createAirTravelerTypeFlightSegmentRPHs() {
return new AirTravelerType.FlightSegmentRPHs();
}
/**
* Create an instance of {@link TravelerInfoType.AirTraveler.Comment }
*
* @return
* the new instance of {@link TravelerInfoType.AirTraveler.Comment }
*/
public TravelerInfoType.AirTraveler.Comment createTravelerInfoTypeAirTravelerComment() {
return new TravelerInfoType.AirTraveler.Comment();
}
/**
* Create an instance of {@link DocumentType.AdditionalPersonNames }
*
* @return
* the new instance of {@link DocumentType.AdditionalPersonNames }
*/
public DocumentType.AdditionalPersonNames createDocumentTypeAdditionalPersonNames() {
return new DocumentType.AdditionalPersonNames();
}
/**
* Create an instance of {@link PriceRequestInformationType.NegotiatedFareCode }
*
* @return
* the new instance of {@link PriceRequestInformationType.NegotiatedFareCode }
*/
public PriceRequestInformationType.NegotiatedFareCode createPriceRequestInformationTypeNegotiatedFareCode() {
return new PriceRequestInformationType.NegotiatedFareCode();
}
/**
* Create an instance of {@link PriceRequestInformationType.RebookOption }
*
* @return
* the new instance of {@link PriceRequestInformationType.RebookOption }
*/
public PriceRequestInformationType.RebookOption createPriceRequestInformationTypeRebookOption() {
return new PriceRequestInformationType.RebookOption();
}
/**
* Create an instance of {@link TravelerInfoSummaryType.PriceRequestInformation.FareRestrictionPref }
*
* @return
* the new instance of {@link TravelerInfoSummaryType.PriceRequestInformation.FareRestrictionPref }
*/
public TravelerInfoSummaryType.PriceRequestInformation.FareRestrictionPref createTravelerInfoSummaryTypePriceRequestInformationFareRestrictionPref() {
return new TravelerInfoSummaryType.PriceRequestInformation.FareRestrictionPref();
}
/**
* Create an instance of {@link TravelerInfoSummaryType.PriceRequestInformation.SegmentOverride }
*
* @return
* the new instance of {@link TravelerInfoSummaryType.PriceRequestInformation.SegmentOverride }
*/
public TravelerInfoSummaryType.PriceRequestInformation.SegmentOverride createTravelerInfoSummaryTypePriceRequestInformationSegmentOverride() {
return new TravelerInfoSummaryType.PriceRequestInformation.SegmentOverride();
}
/**
* Create an instance of {@link TravelerInfoSummaryType.PriceRequestInformation.Account }
*
* @return
* the new instance of {@link TravelerInfoSummaryType.PriceRequestInformation.Account }
*/
public TravelerInfoSummaryType.PriceRequestInformation.Account createTravelerInfoSummaryTypePriceRequestInformationAccount() {
return new TravelerInfoSummaryType.PriceRequestInformation.Account();
}
/**
* Create an instance of {@link TravelerInfoSummaryType.PriceRequestInformation.LocationRequirement }
*
* @return
* the new instance of {@link TravelerInfoSummaryType.PriceRequestInformation.LocationRequirement }
*/
public TravelerInfoSummaryType.PriceRequestInformation.LocationRequirement createTravelerInfoSummaryTypePriceRequestInformationLocationRequirement() {
return new TravelerInfoSummaryType.PriceRequestInformation.LocationRequirement();
}
/**
* Create an instance of {@link TravelerInfoSummaryType.PriceRequestInformation.DiscountPricing.FlightReference }
*
* @return
* the new instance of {@link TravelerInfoSummaryType.PriceRequestInformation.DiscountPricing.FlightReference }
*/
public TravelerInfoSummaryType.PriceRequestInformation.DiscountPricing.FlightReference createTravelerInfoSummaryTypePriceRequestInformationDiscountPricingFlightReference() {
return new TravelerInfoSummaryType.PriceRequestInformation.DiscountPricing.FlightReference();
}
/**
* Create an instance of {@link TicketingInfoType.TicketAdvisory }
*
* @return
* the new instance of {@link TicketingInfoType.TicketAdvisory }
*/
public TicketingInfoType.TicketAdvisory createTicketingInfoTypeTicketAdvisory() {
return new TicketingInfoType.TicketAdvisory();
}
/**
* Create an instance of {@link TicketingInfoType.TicketingVendor }
*
* @return
* the new instance of {@link TicketingInfoType.TicketingVendor }
*/
public TicketingInfoType.TicketingVendor createTicketingInfoTypeTicketingVendor() {
return new TicketingInfoType.TicketingVendor();
}
/**
* Create an instance of {@link TicketingInfoType.PricingSystem }
*
* @return
* the new instance of {@link TicketingInfoType.PricingSystem }
*/
public TicketingInfoType.PricingSystem createTicketingInfoTypePricingSystem() {
return new TicketingInfoType.PricingSystem();
}
/**
* Create an instance of {@link TicketingInfoType.TotalFare }
*
* @return
* the new instance of {@link TicketingInfoType.TotalFare }
*/
public TicketingInfoType.TotalFare createTicketingInfoTypeTotalFare() {
return new TicketingInfoType.TotalFare();
}
/**
* Create an instance of {@link StayRestrictionsType.MinimumStay }
*
* @return
* the new instance of {@link StayRestrictionsType.MinimumStay }
*/
public StayRestrictionsType.MinimumStay createStayRestrictionsTypeMinimumStay() {
return new StayRestrictionsType.MinimumStay();
}
/**
* Create an instance of {@link StayRestrictionsType.MaximumStay }
*
* @return
* the new instance of {@link StayRestrictionsType.MaximumStay }
*/
public StayRestrictionsType.MaximumStay createStayRestrictionsTypeMaximumStay() {
return new StayRestrictionsType.MaximumStay();
}
/**
* Create an instance of {@link SpecificFlightInfoType.BookingClassPref }
*
* @return
* the new instance of {@link SpecificFlightInfoType.BookingClassPref }
*/
public SpecificFlightInfoType.BookingClassPref createSpecificFlightInfoTypeBookingClassPref() {
return new SpecificFlightInfoType.BookingClassPref();
}
/**
* Create an instance of {@link SpecialReqDetailsType.SpecialRemarks.SpecialRemark }
*
* @return
* the new instance of {@link SpecialReqDetailsType.SpecialRemarks.SpecialRemark }
*/
public SpecialReqDetailsType.SpecialRemarks.SpecialRemark createSpecialReqDetailsTypeSpecialRemarksSpecialRemark() {
return new SpecialReqDetailsType.SpecialRemarks.SpecialRemark();
}
/**
* Create an instance of {@link SpecialRemarkType.TravelerRefNumber }
*
* @return
* the new instance of {@link SpecialRemarkType.TravelerRefNumber }
*/
public SpecialRemarkType.TravelerRefNumber createSpecialRemarkTypeTravelerRefNumber() {
return new SpecialRemarkType.TravelerRefNumber();
}
/**
* Create an instance of {@link SpecialRemarkType.FlightRefNumber }
*
* @return
* the new instance of {@link SpecialRemarkType.FlightRefNumber }
*/
public SpecialRemarkType.FlightRefNumber createSpecialRemarkTypeFlightRefNumber() {
return new SpecialRemarkType.FlightRefNumber();
}
/**
* Create an instance of {@link SpecialRemarkType.AuthorizedViewers.AuthorizedViewer }
*
* @return
* the new instance of {@link SpecialRemarkType.AuthorizedViewers.AuthorizedViewer }
*/
public SpecialRemarkType.AuthorizedViewers.AuthorizedViewer createSpecialRemarkTypeAuthorizedViewersAuthorizedViewer() {
return new SpecialRemarkType.AuthorizedViewers.AuthorizedViewer();
}
/**
* Create an instance of {@link SpecialReqDetailsType.Remarks.Remark }
*
* @return
* the new instance of {@link SpecialReqDetailsType.Remarks.Remark }
*/
public SpecialReqDetailsType.Remarks.Remark createSpecialReqDetailsTypeRemarksRemark() {
return new SpecialReqDetailsType.Remarks.Remark();
}
/**
* Create an instance of {@link SpecialReqDetailsType.OtherServiceInformations.OtherServiceInformation }
*
* @return
* the new instance of {@link SpecialReqDetailsType.OtherServiceInformations.OtherServiceInformation }
*/
public SpecialReqDetailsType.OtherServiceInformations.OtherServiceInformation createSpecialReqDetailsTypeOtherServiceInformationsOtherServiceInformation() {
return new SpecialReqDetailsType.OtherServiceInformations.OtherServiceInformation();
}
/**
* Create an instance of {@link OtherServiceInfoType.TravelerRefNumber }
*
* @return
* the new instance of {@link OtherServiceInfoType.TravelerRefNumber }
*/
public OtherServiceInfoType.TravelerRefNumber createOtherServiceInfoTypeTravelerRefNumber() {
return new OtherServiceInfoType.TravelerRefNumber();
}
/**
* Create an instance of {@link SpecialReqDetailsType.SpecialServiceRequests.SpecialServiceRequest }
*
* @return
* the new instance of {@link SpecialReqDetailsType.SpecialServiceRequests.SpecialServiceRequest }
*/
public SpecialReqDetailsType.SpecialServiceRequests.SpecialServiceRequest createSpecialReqDetailsTypeSpecialServiceRequestsSpecialServiceRequest() {
return new SpecialReqDetailsType.SpecialServiceRequests.SpecialServiceRequest();
}
/**
* Create an instance of {@link SpecialReqDetailsType.SeatRequests.SeatRequest }
*
* @return
* the new instance of {@link SpecialReqDetailsType.SeatRequests.SeatRequest }
*/
public SpecialReqDetailsType.SeatRequests.SeatRequest createSpecialReqDetailsTypeSeatRequestsSeatRequest() {
return new SpecialReqDetailsType.SeatRequests.SeatRequest();
}
/**
* Create an instance of {@link SeatMapDetailsType.CabinClass }
*
* @return
* the new instance of {@link SeatMapDetailsType.CabinClass }
*/
public SeatMapDetailsType.CabinClass createSeatMapDetailsTypeCabinClass() {
return new SeatMapDetailsType.CabinClass();
}
/**
* Create an instance of {@link CabinClassType.AirRows }
*
* @return
* the new instance of {@link CabinClassType.AirRows }
*/
public CabinClassType.AirRows createCabinClassTypeAirRows() {
return new CabinClassType.AirRows();
}
/**
* Create an instance of {@link RuleInfoType.ChargesRules }
*
* @return
* the new instance of {@link RuleInfoType.ChargesRules }
*/
public RuleInfoType.ChargesRules createRuleInfoTypeChargesRules() {
return new RuleInfoType.ChargesRules();
}
/**
* Create an instance of {@link RuleInfoType.ResTicketingRules.AdvResTicketing }
*
* @return
* the new instance of {@link RuleInfoType.ResTicketingRules.AdvResTicketing }
*/
public RuleInfoType.ResTicketingRules.AdvResTicketing createRuleInfoTypeResTicketingRulesAdvResTicketing() {
return new RuleInfoType.ResTicketingRules.AdvResTicketing();
}
/**
* Create an instance of {@link AdvResTicketingType.AdvReservation }
*
* @return
* the new instance of {@link AdvResTicketingType.AdvReservation }
*/
public AdvResTicketingType.AdvReservation createAdvResTicketingTypeAdvReservation() {
return new AdvResTicketingType.AdvReservation();
}
/**
* Create an instance of {@link AdvResTicketingType.AdvTicketing }
*
* @return
* the new instance of {@link AdvResTicketingType.AdvTicketing }
*/
public AdvResTicketingType.AdvTicketing createAdvResTicketingTypeAdvTicketing() {
return new AdvResTicketingType.AdvTicketing();
}
/**
* Create an instance of {@link RowDetailsType.AirRowCharacteristics }
*
* @return
* the new instance of {@link RowDetailsType.AirRowCharacteristics }
*/
public RowDetailsType.AirRowCharacteristics createRowDetailsTypeAirRowCharacteristics() {
return new RowDetailsType.AirRowCharacteristics();
}
/**
* Create an instance of {@link RowDetailsType.AirSeats.AirSeat }
*
* @return
* the new instance of {@link RowDetailsType.AirSeats.AirSeat }
*/
public RowDetailsType.AirSeats.AirSeat createRowDetailsTypeAirSeatsAirSeat() {
return new RowDetailsType.AirSeats.AirSeat();
}
/**
* Create an instance of {@link PTCFareBreakdownType.FareBasisCodes }
*
* @return
* the new instance of {@link PTCFareBreakdownType.FareBasisCodes }
*/
public PTCFareBreakdownType.FareBasisCodes createPTCFareBreakdownTypeFareBasisCodes() {
return new PTCFareBreakdownType.FareBasisCodes();
}
/**
* Create an instance of {@link PTCFareBreakdownType.TravelerRefNumber }
*
* @return
* the new instance of {@link PTCFareBreakdownType.TravelerRefNumber }
*/
public PTCFareBreakdownType.TravelerRefNumber createPTCFareBreakdownTypeTravelerRefNumber() {
return new PTCFareBreakdownType.TravelerRefNumber();
}
/**
* Create an instance of {@link PTCFareBreakdownType.FareInfo }
*
* @return
* the new instance of {@link PTCFareBreakdownType.FareInfo }
*/
public PTCFareBreakdownType.FareInfo createPTCFareBreakdownTypeFareInfo() {
return new PTCFareBreakdownType.FareInfo();
}
/**
* Create an instance of {@link PTCFareBreakdownType.PricingUnit.FareComponent.FlightLeg }
*
* @return
* the new instance of {@link PTCFareBreakdownType.PricingUnit.FareComponent.FlightLeg }
*/
public PTCFareBreakdownType.PricingUnit.FareComponent.FlightLeg createPTCFareBreakdownTypePricingUnitFareComponentFlightLeg() {
return new PTCFareBreakdownType.PricingUnit.FareComponent.FlightLeg();
}
/**
* Create an instance of {@link FlightSegmentBaseType.DepartureAirport }
*
* @return
* the new instance of {@link FlightSegmentBaseType.DepartureAirport }
*/
public FlightSegmentBaseType.DepartureAirport createFlightSegmentBaseTypeDepartureAirport() {
return new FlightSegmentBaseType.DepartureAirport();
}
/**
* Create an instance of {@link FlightSegmentBaseType.ArrivalAirport }
*
* @return
* the new instance of {@link FlightSegmentBaseType.ArrivalAirport }
*/
public FlightSegmentBaseType.ArrivalAirport createFlightSegmentBaseTypeArrivalAirport() {
return new FlightSegmentBaseType.ArrivalAirport();
}
/**
* Create an instance of {@link FlightSegmentType.MarketingAirline }
*
* @return
* the new instance of {@link FlightSegmentType.MarketingAirline }
*/
public FlightSegmentType.MarketingAirline createFlightSegmentTypeMarketingAirline() {
return new FlightSegmentType.MarketingAirline();
}
/**
* Create an instance of {@link BookFlightSegmentType.StopLocation }
*
* @return
* the new instance of {@link BookFlightSegmentType.StopLocation }
*/
public BookFlightSegmentType.StopLocation createBookFlightSegmentTypeStopLocation() {
return new BookFlightSegmentType.StopLocation();
}
/**
* Create an instance of {@link BookFlightSegmentType.BookingClassAvails.BookingClassAvail }
*
* @return
* the new instance of {@link BookFlightSegmentType.BookingClassAvails.BookingClassAvail }
*/
public BookFlightSegmentType.BookingClassAvails.BookingClassAvail createBookFlightSegmentTypeBookingClassAvailsBookingClassAvail() {
return new BookFlightSegmentType.BookingClassAvails.BookingClassAvail();
}
/**
* Create an instance of {@link FareInfoType.FareReference }
*
* @return
* the new instance of {@link FareInfoType.FareReference }
*/
public FareInfoType.FareReference createFareInfoTypeFareReference() {
return new FareInfoType.FareReference();
}
/**
* Create an instance of {@link FareInfoType.RuleInfo }
*
* @return
* the new instance of {@link FareInfoType.RuleInfo }
*/
public FareInfoType.RuleInfo createFareInfoTypeRuleInfo() {
return new FareInfoType.RuleInfo();
}
/**
* Create an instance of {@link FareInfoType.Date }
*
* @return
* the new instance of {@link FareInfoType.Date }
*/
public FareInfoType.Date createFareInfoTypeDate() {
return new FareInfoType.Date();
}
/**
* Create an instance of {@link FareInfoType.DiscountPricing }
*
* @return
* the new instance of {@link FareInfoType.DiscountPricing }
*/
public FareInfoType.DiscountPricing createFareInfoTypeDiscountPricing() {
return new FareInfoType.DiscountPricing();
}
/**
* Create an instance of {@link FareInfoType.City }
*
* @return
* the new instance of {@link FareInfoType.City }
*/
public FareInfoType.City createFareInfoTypeCity() {
return new FareInfoType.City();
}
/**
* Create an instance of {@link FareInfoType.Airport }
*
* @return
* the new instance of {@link FareInfoType.Airport }
*/
public FareInfoType.Airport createFareInfoTypeAirport() {
return new FareInfoType.Airport();
}
/**
* Create an instance of {@link FareInfoType.FareInfo.Date }
*
* @return
* the new instance of {@link FareInfoType.FareInfo.Date }
*/
public FareInfoType.FareInfo.Date createFareInfoTypeFareInfoDate() {
return new FareInfoType.FareInfo.Date();
}
/**
* Create an instance of {@link FareInfoType.FareInfo.Fare }
*
* @return
* the new instance of {@link FareInfoType.FareInfo.Fare }
*/
public FareInfoType.FareInfo.Fare createFareInfoTypeFareInfoFare() {
return new FareInfoType.FareInfo.Fare();
}
/**
* Create an instance of {@link FareInfoType.FareInfo.PTC }
*
* @return
* the new instance of {@link FareInfoType.FareInfo.PTC }
*/
public FareInfoType.FareInfo.PTC createFareInfoTypeFareInfoPTC() {
return new FareInfoType.FareInfo.PTC();
}
/**
* Create an instance of {@link PTCFareBreakdownType.Endorsements.Endorsement }
*
* @return
* the new instance of {@link PTCFareBreakdownType.Endorsements.Endorsement }
*/
public PTCFareBreakdownType.Endorsements.Endorsement createPTCFareBreakdownTypeEndorsementsEndorsement() {
return new PTCFareBreakdownType.Endorsements.Endorsement();
}
/**
* Create an instance of {@link PTCFareBreakdownType.TicketDesignators.TicketDesignator }
*
* @return
* the new instance of {@link PTCFareBreakdownType.TicketDesignators.TicketDesignator }
*/
public PTCFareBreakdownType.TicketDesignators.TicketDesignator createPTCFareBreakdownTypeTicketDesignatorsTicketDesignator() {
return new PTCFareBreakdownType.TicketDesignators.TicketDesignator();
}
/**
* Create an instance of {@link FareType.BaseFare }
*
* @return
* the new instance of {@link FareType.BaseFare }
*/
public FareType.BaseFare createFareTypeBaseFare() {
return new FareType.BaseFare();
}
/**
* Create an instance of {@link FareType.EquivFare }
*
* @return
* the new instance of {@link FareType.EquivFare }
*/
public FareType.EquivFare createFareTypeEquivFare() {
return new FareType.EquivFare();
}
/**
* Create an instance of {@link FareType.Taxes }
*
* @return
* the new instance of {@link FareType.Taxes }
*/
public FareType.Taxes createFareTypeTaxes() {
return new FareType.Taxes();
}
/**
* Create an instance of {@link FareType.Fees }
*
* @return
* the new instance of {@link FareType.Fees }
*/
public FareType.Fees createFareTypeFees() {
return new FareType.Fees();
}
/**
* Create an instance of {@link FareType.TotalFare }
*
* @return
* the new instance of {@link FareType.TotalFare }
*/
public FareType.TotalFare createFareTypeTotalFare() {
return new FareType.TotalFare();
}
/**
* Create an instance of {@link FareType.FareConstruction }
*
* @return
* the new instance of {@link FareType.FareConstruction }
*/
public FareType.FareConstruction createFareTypeFareConstruction() {
return new FareType.FareConstruction();
}
/**
* Create an instance of {@link FareType.UnstructuredFareCalc }
*
* @return
* the new instance of {@link FareType.UnstructuredFareCalc }
*/
public FareType.UnstructuredFareCalc createFareTypeUnstructuredFareCalc() {
return new FareType.UnstructuredFareCalc();
}
/**
* Create an instance of {@link FareType.FareBaggageAllowance }
*
* @return
* the new instance of {@link FareType.FareBaggageAllowance }
*/
public FareType.FareBaggageAllowance createFareTypeFareBaggageAllowance() {
return new FareType.FareBaggageAllowance();
}
/**
* Create an instance of {@link FareType.TourCode }
*
* @return
* the new instance of {@link FareType.TourCode }
*/
public FareType.TourCode createFareTypeTourCode() {
return new FareType.TourCode();
}
/**
* Create an instance of {@link FareType.Remark }
*
* @return
* the new instance of {@link FareType.Remark }
*/
public FareType.Remark createFareTypeRemark() {
return new FareType.Remark();
}
/**
* Create an instance of {@link FareType.OriginalIssueInfo }
*
* @return
* the new instance of {@link FareType.OriginalIssueInfo }
*/
public FareType.OriginalIssueInfo createFareTypeOriginalIssueInfo() {
return new FareType.OriginalIssueInfo();
}
/**
* Create an instance of {@link PTCFareBreakdownType.PassengerFare.TicketFeeDetail.Total }
*
* @return
* the new instance of {@link PTCFareBreakdownType.PassengerFare.TicketFeeDetail.Total }
*/
public PTCFareBreakdownType.PassengerFare.TicketFeeDetail.Total createPTCFareBreakdownTypePassengerFareTicketFeeDetailTotal() {
return new PTCFareBreakdownType.PassengerFare.TicketFeeDetail.Total();
}
/**
* Create an instance of {@link PTCFareBreakdownType.PassengerFare.TicketFeeDetail.Fee.BaseFee }
*
* @return
* the new instance of {@link PTCFareBreakdownType.PassengerFare.TicketFeeDetail.Fee.BaseFee }
*/
public PTCFareBreakdownType.PassengerFare.TicketFeeDetail.Fee.BaseFee createPTCFareBreakdownTypePassengerFareTicketFeeDetailFeeBaseFee() {
return new PTCFareBreakdownType.PassengerFare.TicketFeeDetail.Fee.BaseFee();
}
/**
* Create an instance of {@link PTCFareBreakdownType.PassengerFare.TicketFeeDetail.Fee.Taxes }
*
* @return
* the new instance of {@link PTCFareBreakdownType.PassengerFare.TicketFeeDetail.Fee.Taxes }
*/
public PTCFareBreakdownType.PassengerFare.TicketFeeDetail.Fee.Taxes createPTCFareBreakdownTypePassengerFareTicketFeeDetailFeeTaxes() {
return new PTCFareBreakdownType.PassengerFare.TicketFeeDetail.Fee.Taxes();
}
/**
* Create an instance of {@link PTCFareBreakdownType.PassengerFare.TicketFeeDetail.Fee.Total }
*
* @return
* the new instance of {@link PTCFareBreakdownType.PassengerFare.TicketFeeDetail.Fee.Total }
*/
public PTCFareBreakdownType.PassengerFare.TicketFeeDetail.Fee.Total createPTCFareBreakdownTypePassengerFareTicketFeeDetailFeeTotal() {
return new PTCFareBreakdownType.PassengerFare.TicketFeeDetail.Fee.Total();
}
/**
* Create an instance of {@link FareType.Discounts.Discount }
*
* @return
* the new instance of {@link FareType.Discounts.Discount }
*/
public FareType.Discounts.Discount createFareTypeDiscountsDiscount() {
return new FareType.Discounts.Discount();
}
/**
* Create an instance of {@link FareType.ExchangeInfo.CouponInfo }
*
* @return
* the new instance of {@link FareType.ExchangeInfo.CouponInfo }
*/
public FareType.ExchangeInfo.CouponInfo createFareTypeExchangeInfoCouponInfo() {
return new FareType.ExchangeInfo.CouponInfo();
}
/**
* Create an instance of {@link FareType.ExchangeInfo.OriginalOriginDestination }
*
* @return
* the new instance of {@link FareType.ExchangeInfo.OriginalOriginDestination }
*/
public FareType.ExchangeInfo.OriginalOriginDestination createFareTypeExchangeInfoOriginalOriginDestination() {
return new FareType.ExchangeInfo.OriginalOriginDestination();
}
/**
* Create an instance of {@link PricedItineraryType.AirItineraryPricingInfo }
*
* @return
* the new instance of {@link PricedItineraryType.AirItineraryPricingInfo }
*/
public PricedItineraryType.AirItineraryPricingInfo createPricedItineraryTypeAirItineraryPricingInfo() {
return new PricedItineraryType.AirItineraryPricingInfo();
}
/**
* Create an instance of {@link PricedItineraryType.TicketingInfo.DeliveryInfo }
*
* @return
* the new instance of {@link PricedItineraryType.TicketingInfo.DeliveryInfo }
*/
public PricedItineraryType.TicketingInfo.DeliveryInfo createPricedItineraryTypeTicketingInfoDeliveryInfo() {
return new PricedItineraryType.TicketingInfo.DeliveryInfo();
}
/**
* Create an instance of {@link AirItineraryPricingInfoType.ItinTotalFare }
*
* @return
* the new instance of {@link AirItineraryPricingInfoType.ItinTotalFare }
*/
public AirItineraryPricingInfoType.ItinTotalFare createAirItineraryPricingInfoTypeItinTotalFare() {
return new AirItineraryPricingInfoType.ItinTotalFare();
}
/**
* Create an instance of {@link AirItineraryPricingInfoType.PTCFareBreakdowns }
*
* @return
* the new instance of {@link AirItineraryPricingInfoType.PTCFareBreakdowns }
*/
public AirItineraryPricingInfoType.PTCFareBreakdowns createAirItineraryPricingInfoTypePTCFareBreakdowns() {
return new AirItineraryPricingInfoType.PTCFareBreakdowns();
}
/**
* Create an instance of {@link AirItineraryPricingInfoType.FareInfos.FareInfo }
*
* @return
* the new instance of {@link AirItineraryPricingInfoType.FareInfos.FareInfo }
*/
public AirItineraryPricingInfoType.FareInfos.FareInfo createAirItineraryPricingInfoTypeFareInfosFareInfo() {
return new AirItineraryPricingInfoType.FareInfos.FareInfo();
}
/**
* Create an instance of {@link PricedItinerariesType.PricedItinerary }
*
* @return
* the new instance of {@link PricedItinerariesType.PricedItinerary }
*/
public PricedItinerariesType.PricedItinerary createPricedItinerariesTypePricedItinerary() {
return new PricedItinerariesType.PricedItinerary();
}
/**
* Create an instance of {@link OriginDestinationOptionType.FlightSegment }
*
* @return
* the new instance of {@link OriginDestinationOptionType.FlightSegment }
*/
public OriginDestinationOptionType.FlightSegment createOriginDestinationOptionTypeFlightSegment() {
return new OriginDestinationOptionType.FlightSegment();
}
/**
* Create an instance of {@link CabinAvailabilityType.Meal }
*
* @return
* the new instance of {@link CabinAvailabilityType.Meal }
*/
public CabinAvailabilityType.Meal createCabinAvailabilityTypeMeal() {
return new CabinAvailabilityType.Meal();
}
/**
* Create an instance of {@link CabinAvailabilityType.BaggageAllowance }
*
* @return
* the new instance of {@link CabinAvailabilityType.BaggageAllowance }
*/
public CabinAvailabilityType.BaggageAllowance createCabinAvailabilityTypeBaggageAllowance() {
return new CabinAvailabilityType.BaggageAllowance();
}
/**
* Create an instance of {@link CabinAvailabilityType.Entertainment }
*
* @return
* the new instance of {@link CabinAvailabilityType.Entertainment }
*/
public CabinAvailabilityType.Entertainment createCabinAvailabilityTypeEntertainment() {
return new CabinAvailabilityType.Entertainment();
}
/**
* Create an instance of {@link CabinAvailabilityType.FlightLoadInfo }
*
* @return
* the new instance of {@link CabinAvailabilityType.FlightLoadInfo }
*/
public CabinAvailabilityType.FlightLoadInfo createCabinAvailabilityTypeFlightLoadInfo() {
return new CabinAvailabilityType.FlightLoadInfo();
}
/**
* Create an instance of {@link FulfillmentType.Receipt }
*
* @return
* the new instance of {@link FulfillmentType.Receipt }
*/
public FulfillmentType.Receipt createFulfillmentTypeReceipt() {
return new FulfillmentType.Receipt();
}
/**
* Create an instance of {@link FulfillmentType.PaymentText }
*
* @return
* the new instance of {@link FulfillmentType.PaymentText }
*/
public FulfillmentType.PaymentText createFulfillmentTypePaymentText() {
return new FulfillmentType.PaymentText();
}
/**
* Create an instance of {@link FulfillmentType.PaymentDetails.PaymentDetail }
*
* @return
* the new instance of {@link FulfillmentType.PaymentDetails.PaymentDetail }
*/
public FulfillmentType.PaymentDetails.PaymentDetail createFulfillmentTypePaymentDetailsPaymentDetail() {
return new FulfillmentType.PaymentDetails.PaymentDetail();
}
/**
* Create an instance of {@link FlightLegType.DepartureAirport }
*
* @return
* the new instance of {@link FlightLegType.DepartureAirport }
*/
public FlightLegType.DepartureAirport createFlightLegTypeDepartureAirport() {
return new FlightLegType.DepartureAirport();
}
/**
* Create an instance of {@link FlightLegType.ArrivalAirport }
*
* @return
* the new instance of {@link FlightLegType.ArrivalAirport }
*/
public FlightLegType.ArrivalAirport createFlightLegTypeArrivalAirport() {
return new FlightLegType.ArrivalAirport();
}
/**
* Create an instance of {@link FareComponentType.TotalConstructionAmount }
*
* @return
* the new instance of {@link FareComponentType.TotalConstructionAmount }
*/
public FareComponentType.TotalConstructionAmount createFareComponentTypeTotalConstructionAmount() {
return new FareComponentType.TotalConstructionAmount();
}
/**
* Create an instance of {@link FareComponentType.PriceableUnit.FareComponentDetail.CouponSequence }
*
* @return
* the new instance of {@link FareComponentType.PriceableUnit.FareComponentDetail.CouponSequence }
*/
public FareComponentType.PriceableUnit.FareComponentDetail.CouponSequence createFareComponentTypePriceableUnitFareComponentDetailCouponSequence() {
return new FareComponentType.PriceableUnit.FareComponentDetail.CouponSequence();
}
/**
* Create an instance of {@link FareComponentType.PriceableUnit.FareComponentDetail.ConstructionPrinciple }
*
* @return
* the new instance of {@link FareComponentType.PriceableUnit.FareComponentDetail.ConstructionPrinciple }
*/
public FareComponentType.PriceableUnit.FareComponentDetail.ConstructionPrinciple createFareComponentTypePriceableUnitFareComponentDetailConstructionPrinciple() {
return new FareComponentType.PriceableUnit.FareComponentDetail.ConstructionPrinciple();
}
/**
* Create an instance of {@link FareComponentType.PriceableUnit.FareComponentDetail.BaseAmount }
*
* @return
* the new instance of {@link FareComponentType.PriceableUnit.FareComponentDetail.BaseAmount }
*/
public FareComponentType.PriceableUnit.FareComponentDetail.BaseAmount createFareComponentTypePriceableUnitFareComponentDetailBaseAmount() {
return new FareComponentType.PriceableUnit.FareComponentDetail.BaseAmount();
}
/**
* Create an instance of {@link FareComponentType.PriceableUnit.FareComponentDetail.TicketDesignator }
*
* @return
* the new instance of {@link FareComponentType.PriceableUnit.FareComponentDetail.TicketDesignator }
*/
public FareComponentType.PriceableUnit.FareComponentDetail.TicketDesignator createFareComponentTypePriceableUnitFareComponentDetailTicketDesignator() {
return new FareComponentType.PriceableUnit.FareComponentDetail.TicketDesignator();
}
/**
* Create an instance of {@link ETFareInfo.Waiver }
*
* @return
* the new instance of {@link ETFareInfo.Waiver }
*/
public ETFareInfo.Waiver createETFareInfoWaiver() {
return new ETFareInfo.Waiver();
}
/**
* Create an instance of {@link ETFareInfo.RuleIndicator }
*
* @return
* the new instance of {@link ETFareInfo.RuleIndicator }
*/
public ETFareInfo.RuleIndicator createETFareInfoRuleIndicator() {
return new ETFareInfo.RuleIndicator();
}
/**
* Create an instance of {@link EMDType.TravelerRefNumber }
*
* @return
* the new instance of {@link EMDType.TravelerRefNumber }
*/
public EMDType.TravelerRefNumber createEMDTypeTravelerRefNumber() {
return new EMDType.TravelerRefNumber();
}
/**
* Create an instance of {@link EMDType.OriginDestination }
*
* @return
* the new instance of {@link EMDType.OriginDestination }
*/
public EMDType.OriginDestination createEMDTypeOriginDestination() {
return new EMDType.OriginDestination();
}
/**
* Create an instance of {@link EMDType.CustLoyalty }
*
* @return
* the new instance of {@link EMDType.CustLoyalty }
*/
public EMDType.CustLoyalty createEMDTypeCustLoyalty() {
return new EMDType.CustLoyalty();
}
/**
* Create an instance of {@link EMDType.Endorsement }
*
* @return
* the new instance of {@link EMDType.Endorsement }
*/
public EMDType.Endorsement createEMDTypeEndorsement() {
return new EMDType.Endorsement();
}
/**
* Create an instance of {@link EMDType.BaseFare }
*
* @return
* the new instance of {@link EMDType.BaseFare }
*/
public EMDType.BaseFare createEMDTypeBaseFare() {
return new EMDType.BaseFare();
}
/**
* Create an instance of {@link EMDType.EquivFare }
*
* @return
* the new instance of {@link EMDType.EquivFare }
*/
public EMDType.EquivFare createEMDTypeEquivFare() {
return new EMDType.EquivFare();
}
/**
* Create an instance of {@link EMDType.TotalFare }
*
* @return
* the new instance of {@link EMDType.TotalFare }
*/
public EMDType.TotalFare createEMDTypeTotalFare() {
return new EMDType.TotalFare();
}
/**
* Create an instance of {@link EMDType.UnstructuredFareCalc }
*
* @return
* the new instance of {@link EMDType.UnstructuredFareCalc }
*/
public EMDType.UnstructuredFareCalc createEMDTypeUnstructuredFareCalc() {
return new EMDType.UnstructuredFareCalc();
}
/**
* Create an instance of {@link EMDType.FareInfo }
*
* @return
* the new instance of {@link EMDType.FareInfo }
*/
public EMDType.FareInfo createEMDTypeFareInfo() {
return new EMDType.FareInfo();
}
/**
* Create an instance of {@link EMDType.Commission }
*
* @return
* the new instance of {@link EMDType.Commission }
*/
public EMDType.Commission createEMDTypeCommission() {
return new EMDType.Commission();
}
/**
* Create an instance of {@link EMDType.OriginalIssueInfo }
*
* @return
* the new instance of {@link EMDType.OriginalIssueInfo }
*/
public EMDType.OriginalIssueInfo createEMDTypeOriginalIssueInfo() {
return new EMDType.OriginalIssueInfo();
}
/**
* Create an instance of {@link EMDType.ReissuedFlown }
*
* @return
* the new instance of {@link EMDType.ReissuedFlown }
*/
public EMDType.ReissuedFlown createEMDTypeReissuedFlown() {
return new EMDType.ReissuedFlown();
}
/**
* Create an instance of {@link EMDType.PresentInfo }
*
* @return
* the new instance of {@link EMDType.PresentInfo }
*/
public EMDType.PresentInfo createEMDTypePresentInfo() {
return new EMDType.PresentInfo();
}
/**
* Create an instance of {@link EMDType.ReasonForIssuance }
*
* @return
* the new instance of {@link EMDType.ReasonForIssuance }
*/
public EMDType.ReasonForIssuance createEMDTypeReasonForIssuance() {
return new EMDType.ReasonForIssuance();
}
/**
* Create an instance of {@link EMDType.ValidatingAirline }
*
* @return
* the new instance of {@link EMDType.ValidatingAirline }
*/
public EMDType.ValidatingAirline createEMDTypeValidatingAirline() {
return new EMDType.ValidatingAirline();
}
/**
* Create an instance of {@link EMDType.TaxCouponInformation.TicketDocument.CouponNumber.TaxCouponInfo }
*
* @return
* the new instance of {@link EMDType.TaxCouponInformation.TicketDocument.CouponNumber.TaxCouponInfo }
*/
public EMDType.TaxCouponInformation.TicketDocument.CouponNumber.TaxCouponInfo createEMDTypeTaxCouponInformationTicketDocumentCouponNumberTaxCouponInfo() {
return new EMDType.TaxCouponInformation.TicketDocument.CouponNumber.TaxCouponInfo();
}
/**
* Create an instance of {@link EMDType.TaxCouponInformation.TicketDocument.CouponNumber.UnticketedPointInfo }
*
* @return
* the new instance of {@link EMDType.TaxCouponInformation.TicketDocument.CouponNumber.UnticketedPointInfo }
*/
public EMDType.TaxCouponInformation.TicketDocument.CouponNumber.UnticketedPointInfo createEMDTypeTaxCouponInformationTicketDocumentCouponNumberUnticketedPointInfo() {
return new EMDType.TaxCouponInformation.TicketDocument.CouponNumber.UnticketedPointInfo();
}
/**
* Create an instance of {@link EMDType.ExchResidualFareComponent.Taxes }
*
* @return
* the new instance of {@link EMDType.ExchResidualFareComponent.Taxes }
*/
public EMDType.ExchResidualFareComponent.Taxes createEMDTypeExchResidualFareComponentTaxes() {
return new EMDType.ExchResidualFareComponent.Taxes();
}
/**
* Create an instance of {@link EMDType.ExchResidualFareComponent.TotalAmount }
*
* @return
* the new instance of {@link EMDType.ExchResidualFareComponent.TotalAmount }
*/
public EMDType.ExchResidualFareComponent.TotalAmount createEMDTypeExchResidualFareComponentTotalAmount() {
return new EMDType.ExchResidualFareComponent.TotalAmount();
}
/**
* Create an instance of {@link EMDType.CarrierFeeInfo.Taxes }
*
* @return
* the new instance of {@link EMDType.CarrierFeeInfo.Taxes }
*/
public EMDType.CarrierFeeInfo.Taxes createEMDTypeCarrierFeeInfoTaxes() {
return new EMDType.CarrierFeeInfo.Taxes();
}
/**
* Create an instance of {@link EMDType.CarrierFeeInfo.CarrierFee.FeeAmount }
*
* @return
* the new instance of {@link EMDType.CarrierFeeInfo.CarrierFee.FeeAmount }
*/
public EMDType.CarrierFeeInfo.CarrierFee.FeeAmount createEMDTypeCarrierFeeInfoCarrierFeeFeeAmount() {
return new EMDType.CarrierFeeInfo.CarrierFee.FeeAmount();
}
/**
* Create an instance of {@link EMDType.TicketDocument.CouponInfo.ExcessBaggage }
*
* @return
* the new instance of {@link EMDType.TicketDocument.CouponInfo.ExcessBaggage }
*/
public EMDType.TicketDocument.CouponInfo.ExcessBaggage createEMDTypeTicketDocumentCouponInfoExcessBaggage() {
return new EMDType.TicketDocument.CouponInfo.ExcessBaggage();
}
/**
* Create an instance of {@link EMDType.TicketDocument.CouponInfo.PresentInfo }
*
* @return
* the new instance of {@link EMDType.TicketDocument.CouponInfo.PresentInfo }
*/
public EMDType.TicketDocument.CouponInfo.PresentInfo createEMDTypeTicketDocumentCouponInfoPresentInfo() {
return new EMDType.TicketDocument.CouponInfo.PresentInfo();
}
/**
* Create an instance of {@link EMDType.TicketDocument.CouponInfo.ReasonForIssuance }
*
* @return
* the new instance of {@link EMDType.TicketDocument.CouponInfo.ReasonForIssuance }
*/
public EMDType.TicketDocument.CouponInfo.ReasonForIssuance createEMDTypeTicketDocumentCouponInfoReasonForIssuance() {
return new EMDType.TicketDocument.CouponInfo.ReasonForIssuance();
}
/**
* Create an instance of {@link EMDType.TicketDocument.CouponInfo.ValidatingAirline }
*
* @return
* the new instance of {@link EMDType.TicketDocument.CouponInfo.ValidatingAirline }
*/
public EMDType.TicketDocument.CouponInfo.ValidatingAirline createEMDTypeTicketDocumentCouponInfoValidatingAirline() {
return new EMDType.TicketDocument.CouponInfo.ValidatingAirline();
}
/**
* Create an instance of {@link EMDType.TicketDocument.CouponInfo.FiledFeeInfo }
*
* @return
* the new instance of {@link EMDType.TicketDocument.CouponInfo.FiledFeeInfo }
*/
public EMDType.TicketDocument.CouponInfo.FiledFeeInfo createEMDTypeTicketDocumentCouponInfoFiledFeeInfo() {
return new EMDType.TicketDocument.CouponInfo.FiledFeeInfo();
}
/**
* Create an instance of {@link EMDType.Taxes.Tax }
*
* @return
* the new instance of {@link EMDType.Taxes.Tax }
*/
public EMDType.Taxes.Tax createEMDTypeTaxesTax() {
return new EMDType.Taxes.Tax();
}
/**
* Create an instance of {@link AuthorizationType.CheckAuthorization }
*
* @return
* the new instance of {@link AuthorizationType.CheckAuthorization }
*/
public AuthorizationType.CheckAuthorization createAuthorizationTypeCheckAuthorization() {
return new AuthorizationType.CheckAuthorization();
}
/**
* Create an instance of {@link AuthorizationType.CreditCardAuthorization }
*
* @return
* the new instance of {@link AuthorizationType.CreditCardAuthorization }
*/
public AuthorizationType.CreditCardAuthorization createAuthorizationTypeCreditCardAuthorization() {
return new AuthorizationType.CreditCardAuthorization();
}
/**
* Create an instance of {@link AuthorizationType.BookingReferenceID }
*
* @return
* the new instance of {@link AuthorizationType.BookingReferenceID }
*/
public AuthorizationType.BookingReferenceID createAuthorizationTypeBookingReferenceID() {
return new AuthorizationType.BookingReferenceID();
}
/**
* Create an instance of {@link AuthorizationType.AccountAuthorization.AccountInfo }
*
* @return
* the new instance of {@link AuthorizationType.AccountAuthorization.AccountInfo }
*/
public AuthorizationType.AccountAuthorization.AccountInfo createAuthorizationTypeAccountAuthorizationAccountInfo() {
return new AuthorizationType.AccountAuthorization.AccountInfo();
}
/**
* Create an instance of {@link AirReservationType.BookingReferenceID }
*
* @return
* the new instance of {@link AirReservationType.BookingReferenceID }
*/
public AirReservationType.BookingReferenceID createAirReservationTypeBookingReferenceID() {
return new AirReservationType.BookingReferenceID();
}
/**
* Create an instance of {@link AirReservationType.PricingOverview.PricingIndicator }
*
* @return
* the new instance of {@link AirReservationType.PricingOverview.PricingIndicator }
*/
public AirReservationType.PricingOverview.PricingIndicator createAirReservationTypePricingOverviewPricingIndicator() {
return new AirReservationType.PricingOverview.PricingIndicator();
}
/**
* Create an instance of {@link AirReservationType.PricingOverview.Account }
*
* @return
* the new instance of {@link AirReservationType.PricingOverview.Account }
*/
public AirReservationType.PricingOverview.Account createAirReservationTypePricingOverviewAccount() {
return new AirReservationType.PricingOverview.Account();
}
/**
* Create an instance of {@link AirReservationType.Queues.Queue }
*
* @return
* the new instance of {@link AirReservationType.Queues.Queue }
*/
public AirReservationType.Queues.Queue createAirReservationTypeQueuesQueue() {
return new AirReservationType.Queues.Queue();
}
/**
* Create an instance of {@link AirItineraryType.OriginDestinationOptions.OriginDestinationOption }
*
* @return
* the new instance of {@link AirItineraryType.OriginDestinationOptions.OriginDestinationOption }
*/
public AirItineraryType.OriginDestinationOptions.OriginDestinationOption createAirItineraryTypeOriginDestinationOptionsOriginDestinationOption() {
return new AirItineraryType.OriginDestinationOptions.OriginDestinationOption();
}
/**
* Create an instance of {@link VideoItemsType.VideoItem }
*
* @return
* the new instance of {@link VideoItemsType.VideoItem }
*/
public VideoItemsType.VideoItem createVideoItemsTypeVideoItem() {
return new VideoItemsType.VideoItem();
}
/**
* Create an instance of {@link VideoDescriptionType.VideoFormat }
*
* @return
* the new instance of {@link VideoDescriptionType.VideoFormat }
*/
public VideoDescriptionType.VideoFormat createVideoDescriptionTypeVideoFormat() {
return new VideoDescriptionType.VideoFormat();
}
/**
* Create an instance of {@link TravelerRPHs.TravelerRPH }
*
* @return
* the new instance of {@link TravelerRPHs.TravelerRPH }
*/
public TravelerRPHs.TravelerRPH createTravelerRPHsTravelerRPH() {
return new TravelerRPHs.TravelerRPH();
}
/**
* Create an instance of {@link TransportationType.Transportation }
*
* @return
* the new instance of {@link TransportationType.Transportation }
*/
public TransportationType.Transportation createTransportationTypeTransportation() {
return new TransportationType.Transportation();
}
/**
* Create an instance of {@link TextItemsType.TextItem }
*
* @return
* the new instance of {@link TextItemsType.TextItem }
*/
public TextItemsType.TextItem createTextItemsTypeTextItem() {
return new TextItemsType.TextItem();
}
/**
* Create an instance of {@link TextDescriptionType.Description }
*
* @return
* the new instance of {@link TextDescriptionType.Description }
*/
public TextDescriptionType.Description createTextDescriptionTypeDescription() {
return new TextDescriptionType.Description();
}
/**
* Create an instance of {@link SpecialRequestType.SpecialRequest }
*
* @return
* the new instance of {@link SpecialRequestType.SpecialRequest }
*/
public SpecialRequestType.SpecialRequest createSpecialRequestTypeSpecialRequest() {
return new SpecialRequestType.SpecialRequest();
}
/**
* Create an instance of {@link SourceType.RequestorID }
*
* @return
* the new instance of {@link SourceType.RequestorID }
*/
public SourceType.RequestorID createSourceTypeRequestorID() {
return new SourceType.RequestorID();
}
/**
* Create an instance of {@link SourceType.Position }
*
* @return
* the new instance of {@link SourceType.Position }
*/
public SourceType.Position createSourceTypePosition() {
return new SourceType.Position();
}
/**
* Create an instance of {@link SourceType.BookingChannel }
*
* @return
* the new instance of {@link SourceType.BookingChannel }
*/
public SourceType.BookingChannel createSourceTypeBookingChannel() {
return new SourceType.BookingChannel();
}
/**
* Create an instance of {@link RestaurantType.MultimediaDescriptions }
*
* @return
* the new instance of {@link RestaurantType.MultimediaDescriptions }
*/
public RestaurantType.MultimediaDescriptions createRestaurantTypeMultimediaDescriptions() {
return new RestaurantType.MultimediaDescriptions();
}
/**
* Create an instance of {@link RestaurantType.CuisineCodes.CuisineCode }
*
* @return
* the new instance of {@link RestaurantType.CuisineCodes.CuisineCode }
*/
public RestaurantType.CuisineCodes.CuisineCode createRestaurantTypeCuisineCodesCuisineCode() {
return new RestaurantType.CuisineCodes.CuisineCode();
}
/**
* Create an instance of {@link RestaurantType.InfoCodes.InfoCode }
*
* @return
* the new instance of {@link RestaurantType.InfoCodes.InfoCode }
*/
public RestaurantType.InfoCodes.InfoCode createRestaurantTypeInfoCodesInfoCode() {
return new RestaurantType.InfoCodes.InfoCode();
}
/**
* Create an instance of {@link RecipientInfosType.RecipientInfo.ShippingInfo }
*
* @return
* the new instance of {@link RecipientInfosType.RecipientInfo.ShippingInfo }
*/
public RecipientInfosType.RecipientInfo.ShippingInfo createRecipientInfosTypeRecipientInfoShippingInfo() {
return new RecipientInfosType.RecipientInfo.ShippingInfo();
}
/**
* Create an instance of {@link RecipientInfosType.RecipientInfo.Comments }
*
* @return
* the new instance of {@link RecipientInfosType.RecipientInfo.Comments }
*/
public RecipientInfosType.RecipientInfo.Comments createRecipientInfosTypeRecipientInfoComments() {
return new RecipientInfosType.RecipientInfo.Comments();
}
/**
* Create an instance of {@link PaymentCardType.CardIssuerName }
*
* @return
* the new instance of {@link PaymentCardType.CardIssuerName }
*/
public PaymentCardType.CardIssuerName createPaymentCardTypeCardIssuerName() {
return new PaymentCardType.CardIssuerName();
}
/**
* Create an instance of {@link PaymentCardType.Telephone }
*
* @return
* the new instance of {@link PaymentCardType.Telephone }
*/
public PaymentCardType.Telephone createPaymentCardTypeTelephone() {
return new PaymentCardType.Telephone();
}
/**
* Create an instance of {@link PaymentCardType.CustLoyalty }
*
* @return
* the new instance of {@link PaymentCardType.CustLoyalty }
*/
public PaymentCardType.CustLoyalty createPaymentCardTypeCustLoyalty() {
return new PaymentCardType.CustLoyalty();
}
/**
* Create an instance of {@link PaymentCardType.SignatureOnFile }
*
* @return
* the new instance of {@link PaymentCardType.SignatureOnFile }
*/
public PaymentCardType.SignatureOnFile createPaymentCardTypeSignatureOnFile() {
return new PaymentCardType.SignatureOnFile();
}
/**
* Create an instance of {@link PaymentCardType.MagneticStripe }
*
* @return
* the new instance of {@link PaymentCardType.MagneticStripe }
*/
public PaymentCardType.MagneticStripe createPaymentCardTypeMagneticStripe() {
return new PaymentCardType.MagneticStripe();
}
/**
* Create an instance of {@link OriginDestinationInformationType.OriginLocation }
*
* @return
* the new instance of {@link OriginDestinationInformationType.OriginLocation }
*/
public OriginDestinationInformationType.OriginLocation createOriginDestinationInformationTypeOriginLocation() {
return new OriginDestinationInformationType.OriginLocation();
}
/**
* Create an instance of {@link OriginDestinationInformationType.DestinationLocation }
*
* @return
* the new instance of {@link OriginDestinationInformationType.DestinationLocation }
*/
public OriginDestinationInformationType.DestinationLocation createOriginDestinationInformationTypeDestinationLocation() {
return new OriginDestinationInformationType.DestinationLocation();
}
/**
* Create an instance of {@link OrdersType.Order.Products.Product }
*
* @return
* the new instance of {@link OrdersType.Order.Products.Product }
*/
public OrdersType.Order.Products.Product createOrdersTypeOrderProductsProduct() {
return new OrdersType.Order.Products.Product();
}
/**
* Create an instance of {@link OperationSchedulesPlusChargeType.OperationSchedule }
*
* @return
* the new instance of {@link OperationSchedulesPlusChargeType.OperationSchedule }
*/
public OperationSchedulesPlusChargeType.OperationSchedule createOperationSchedulesPlusChargeTypeOperationSchedule() {
return new OperationSchedulesPlusChargeType.OperationSchedule();
}
/**
* Create an instance of {@link OperationScheduleType.OperationTimes.OperationTime }
*
* @return
* the new instance of {@link OperationScheduleType.OperationTimes.OperationTime }
*/
public OperationScheduleType.OperationTimes.OperationTime createOperationScheduleTypeOperationTimesOperationTime() {
return new OperationScheduleType.OperationTimes.OperationTime();
}
/**
* Create an instance of {@link ImageItemsType.ImageItem }
*
* @return
* the new instance of {@link ImageItemsType.ImageItem }
*/
public ImageItemsType.ImageItem createImageItemsTypeImageItem() {
return new ImageItemsType.ImageItem();
}
/**
* Create an instance of {@link ImageDescriptionType.ImageFormat }
*
* @return
* the new instance of {@link ImageDescriptionType.ImageFormat }
*/
public ImageDescriptionType.ImageFormat createImageDescriptionTypeImageFormat() {
return new ImageDescriptionType.ImageFormat();
}
/**
* Create an instance of {@link ImageDescriptionType.Description }
*
* @return
* the new instance of {@link ImageDescriptionType.Description }
*/
public ImageDescriptionType.Description createImageDescriptionTypeDescription() {
return new ImageDescriptionType.Description();
}
/**
* Create an instance of {@link DonationType.FrontOfficeInfo }
*
* @return
* the new instance of {@link DonationType.FrontOfficeInfo }
*/
public DonationType.FrontOfficeInfo createDonationTypeFrontOfficeInfo() {
return new DonationType.FrontOfficeInfo();
}
/**
* Create an instance of {@link DonationType.CreditCardInfo }
*
* @return
* the new instance of {@link DonationType.CreditCardInfo }
*/
public DonationType.CreditCardInfo createDonationTypeCreditCardInfo() {
return new DonationType.CreditCardInfo();
}
/**
* Create an instance of {@link DonationType.DonorInfo.Name }
*
* @return
* the new instance of {@link DonationType.DonorInfo.Name }
*/
public DonationType.DonorInfo.Name createDonationTypeDonorInfoName() {
return new DonationType.DonorInfo.Name();
}
/**
* Create an instance of {@link DonationType.DonorInfo.ContactInfo }
*
* @return
* the new instance of {@link DonationType.DonorInfo.ContactInfo }
*/
public DonationType.DonorInfo.ContactInfo createDonationTypeDonorInfoContactInfo() {
return new DonationType.DonorInfo.ContactInfo();
}
/**
* Create an instance of {@link ConnectionType.ConnectionLocation }
*
* @return
* the new instance of {@link ConnectionType.ConnectionLocation }
*/
public ConnectionType.ConnectionLocation createConnectionTypeConnectionLocation() {
return new ConnectionType.ConnectionLocation();
}
/**
* Create an instance of {@link CommissionType.CommissionableAmount }
*
* @return
* the new instance of {@link CommissionType.CommissionableAmount }
*/
public CommissionType.CommissionableAmount createCommissionTypeCommissionableAmount() {
return new CommissionType.CommissionableAmount();
}
/**
* Create an instance of {@link CommissionType.PrepaidAmount }
*
* @return
* the new instance of {@link CommissionType.PrepaidAmount }
*/
public CommissionType.PrepaidAmount createCommissionTypePrepaidAmount() {
return new CommissionType.PrepaidAmount();
}
/**
* Create an instance of {@link CommissionType.FlatCommission }
*
* @return
* the new instance of {@link CommissionType.FlatCommission }
*/
public CommissionType.FlatCommission createCommissionTypeFlatCommission() {
return new CommissionType.FlatCommission();
}
/**
* Create an instance of {@link CommissionType.CommissionPayableAmount }
*
* @return
* the new instance of {@link CommissionType.CommissionPayableAmount }
*/
public CommissionType.CommissionPayableAmount createCommissionTypeCommissionPayableAmount() {
return new CommissionType.CommissionPayableAmount();
}
/**
* Create an instance of {@link CommentType.Comment }
*
* @return
* the new instance of {@link CommentType.Comment }
*/
public CommentType.Comment createCommentTypeComment() {
return new CommentType.Comment();
}
/**
* Create an instance of {@link CancelInfoRSType.CancelRules }
*
* @return
* the new instance of {@link CancelInfoRSType.CancelRules }
*/
public CancelInfoRSType.CancelRules createCancelInfoRSTypeCancelRules() {
return new CancelInfoRSType.CancelRules();
}
/**
* Create an instance of {@link AcceptablePaymentCardsInfoType.AcceptablePaymentCards.AcceptablePaymentCard }
*
* @return
* the new instance of {@link AcceptablePaymentCardsInfoType.AcceptablePaymentCards.AcceptablePaymentCard }
*/
public AcceptablePaymentCardsInfoType.AcceptablePaymentCards.AcceptablePaymentCard createAcceptablePaymentCardsInfoTypeAcceptablePaymentCardsAcceptablePaymentCard() {
return new AcceptablePaymentCardsInfoType.AcceptablePaymentCards.AcceptablePaymentCard();
}
/**
* Create an instance of {@link OTAHotelRatePlanRQ.RatePlans.RatePlan.DateRange }
*
* @return
* the new instance of {@link OTAHotelRatePlanRQ.RatePlans.RatePlan.DateRange }
*/
public OTAHotelRatePlanRQ.RatePlans.RatePlan.DateRange createOTAHotelRatePlanRQRatePlansRatePlanDateRange() {
return new OTAHotelRatePlanRQ.RatePlans.RatePlan.DateRange();
}
/**
* Create an instance of {@link OTAHotelRatePlanRQ.RatePlans.RatePlan.HotelRef }
*
* @return
* the new instance of {@link OTAHotelRatePlanRQ.RatePlans.RatePlan.HotelRef }
*/
public OTAHotelRatePlanRQ.RatePlans.RatePlan.HotelRef createOTAHotelRatePlanRQRatePlansRatePlanHotelRef() {
return new OTAHotelRatePlanRQ.RatePlans.RatePlan.HotelRef();
}
/**
* Create an instance of {@link OTAHotelRatePlanRQ.RatePlans.RatePlan.Offers.Offer }
*
* @return
* the new instance of {@link OTAHotelRatePlanRQ.RatePlans.RatePlan.Offers.Offer }
*/
public OTAHotelRatePlanRQ.RatePlans.RatePlan.Offers.Offer createOTAHotelRatePlanRQRatePlansRatePlanOffersOffer() {
return new OTAHotelRatePlanRQ.RatePlans.RatePlan.Offers.Offer();
}
/**
* Create an instance of {@link OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.DateRange }
*
* @return
* the new instance of {@link OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.DateRange }
*/
public OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.DateRange createOTAHotelAvailGetRQHotelAvailRequestsHotelAvailRequestDateRange() {
return new OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.DateRange();
}
/**
* Create an instance of {@link OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.BestAvailableRateCandidate }
*
* @return
* the new instance of {@link OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.BestAvailableRateCandidate }
*/
public OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.BestAvailableRateCandidate createOTAHotelAvailGetRQHotelAvailRequestsHotelAvailRequestBestAvailableRateCandidate() {
return new OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.BestAvailableRateCandidate();
}
/**
* Create an instance of {@link OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.HurdleRateCandidate }
*
* @return
* the new instance of {@link OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.HurdleRateCandidate }
*/
public OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.HurdleRateCandidate createOTAHotelAvailGetRQHotelAvailRequestsHotelAvailRequestHurdleRateCandidate() {
return new OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.HurdleRateCandidate();
}
/**
* Create an instance of {@link OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.DeltaCandidate }
*
* @return
* the new instance of {@link OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.DeltaCandidate }
*/
public OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.DeltaCandidate createOTAHotelAvailGetRQHotelAvailRequestsHotelAvailRequestDeltaCandidate() {
return new OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.DeltaCandidate();
}
/**
* Create an instance of {@link OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.HotelRef }
*
* @return
* the new instance of {@link OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.HotelRef }
*/
public OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.HotelRef createOTAHotelAvailGetRQHotelAvailRequestsHotelAvailRequestHotelRef() {
return new OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.HotelRef();
}
/**
* Create an instance of {@link OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.RebatePrograms }
*
* @return
* the new instance of {@link OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.RebatePrograms }
*/
public OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.RebatePrograms createOTAHotelAvailGetRQHotelAvailRequestsHotelAvailRequestRebatePrograms() {
return new OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.RebatePrograms();
}
/**
* Create an instance of {@link OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.LengthsOfStayCandidates.LengthOfStayCandidate }
*
* @return
* the new instance of {@link OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.LengthsOfStayCandidates.LengthOfStayCandidate }
*/
public OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.LengthsOfStayCandidates.LengthOfStayCandidate createOTAHotelAvailGetRQHotelAvailRequestsHotelAvailRequestLengthsOfStayCandidatesLengthOfStayCandidate() {
return new OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.LengthsOfStayCandidates.LengthOfStayCandidate();
}
/**
* Create an instance of {@link OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.RestrictionStatusCandidates.RestrictionStatusCandidate }
*
* @return
* the new instance of {@link OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.RestrictionStatusCandidates.RestrictionStatusCandidate }
*/
public OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.RestrictionStatusCandidates.RestrictionStatusCandidate createOTAHotelAvailGetRQHotelAvailRequestsHotelAvailRequestRestrictionStatusCandidatesRestrictionStatusCandidate() {
return new OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.RestrictionStatusCandidates.RestrictionStatusCandidate();
}
/**
* Create an instance of {@link OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.Offers.Offer }
*
* @return
* the new instance of {@link OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.Offers.Offer }
*/
public OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.Offers.Offer createOTAHotelAvailGetRQHotelAvailRequestsHotelAvailRequestOffersOffer() {
return new OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.Offers.Offer();
}
/**
* Create an instance of {@link OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.RoomTypeCandidates.RoomTypeCandidate }
*
* @return
* the new instance of {@link OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.RoomTypeCandidates.RoomTypeCandidate }
*/
public OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.RoomTypeCandidates.RoomTypeCandidate createOTAHotelAvailGetRQHotelAvailRequestsHotelAvailRequestRoomTypeCandidatesRoomTypeCandidate() {
return new OTAHotelAvailGetRQ.HotelAvailRequests.HotelAvailRequest.RoomTypeCandidates.RoomTypeCandidate();
}
/**
* Create an instance of {@link HotelReservationsType.HotelReservation.RebatePrograms }
*
* @return
* the new instance of {@link HotelReservationsType.HotelReservation.RebatePrograms }
*/
public HotelReservationsType.HotelReservation.RebatePrograms createHotelReservationsTypeHotelReservationRebatePrograms() {
return new HotelReservationsType.HotelReservation.RebatePrograms();
}
/**
* Create an instance of {@link OTAHotelAvailRS.CurrencyConversions.CurrencyConversion }
*
* @return
* the new instance of {@link OTAHotelAvailRS.CurrencyConversions.CurrencyConversion }
*/
public OTAHotelAvailRS.CurrencyConversions.CurrencyConversion createOTAHotelAvailRSCurrencyConversionsCurrencyConversion() {
return new OTAHotelAvailRS.CurrencyConversions.CurrencyConversion();
}
/**
* Create an instance of {@link AreasType.Area }
*
* @return
* the new instance of {@link AreasType.Area }
*/
public AreasType.Area createAreasTypeArea() {
return new AreasType.Area();
}
/**
* Create an instance of {@link OTAHotelAvailRS.RoomStays.RoomStay.Reference }
*
* @return
* the new instance of {@link OTAHotelAvailRS.RoomStays.RoomStay.Reference }
*/
public OTAHotelAvailRS.RoomStays.RoomStay.Reference createOTAHotelAvailRSRoomStaysRoomStayReference() {
return new OTAHotelAvailRS.RoomStays.RoomStay.Reference();
}
/**
* Create an instance of {@link OTAHotelAvailRS.HotelStays.HotelStay.Price }
*
* @return
* the new instance of {@link OTAHotelAvailRS.HotelStays.HotelStay.Price }
*/
public OTAHotelAvailRS.HotelStays.HotelStay.Price createOTAHotelAvailRSHotelStaysHotelStayPrice() {
return new OTAHotelAvailRS.HotelStays.HotelStay.Price();
}
/**
* Create an instance of {@link OTAHotelAvailRS.HotelStays.HotelStay.Availability.Restriction }
*
* @return
* the new instance of {@link OTAHotelAvailRS.HotelStays.HotelStay.Availability.Restriction }
*/
public OTAHotelAvailRS.HotelStays.HotelStay.Availability.Restriction createOTAHotelAvailRSHotelStaysHotelStayAvailabilityRestriction() {
return new OTAHotelAvailRS.HotelStays.HotelStay.Availability.Restriction();
}
/**
* Create an instance of {@link ProfilesType.ProfileInfo }
*
* @return
* the new instance of {@link ProfilesType.ProfileInfo }
*/
public ProfilesType.ProfileInfo createProfilesTypeProfileInfo() {
return new ProfilesType.ProfileInfo();
}
/**
* Create an instance of {@link HotelReservationIDsType.HotelReservationID }
*
* @return
* the new instance of {@link HotelReservationIDsType.HotelReservationID }
*/
public HotelReservationIDsType.HotelReservationID createHotelReservationIDsTypeHotelReservationID() {
return new HotelReservationIDsType.HotelReservationID();
}
/**
* Create an instance of {@link VehicleReservationType.VehSegmentCore }
*
* @return
* the new instance of {@link VehicleReservationType.VehSegmentCore }
*/
public VehicleReservationType.VehSegmentCore createVehicleReservationTypeVehSegmentCore() {
return new VehicleReservationType.VehSegmentCore();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link TPAExtensionsType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link TPAExtensionsType }{@code >}
*/
@XmlElementDecl(namespace = "http://www.opentravel.org/OTA/2003/05", name = "TPA_Extensions")
public JAXBElement createTPAExtensions(TPAExtensionsType value) {
return new JAXBElement<>(_TPAExtensions_QNAME, TPAExtensionsType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link VehicleReservationType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link VehicleReservationType }{@code >}
*/
@XmlElementDecl(namespace = "http://www.opentravel.org/OTA/2003/05", name = "VehReservation")
public JAXBElement createVehReservation(VehicleReservationType value) {
return new JAXBElement<>(_VehReservation_QNAME, VehicleReservationType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link MessageAcknowledgementType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link MessageAcknowledgementType }{@code >}
*/
@XmlElementDecl(namespace = "http://www.opentravel.org/OTA/2003/05", name = "OTA_HotelAvailNotifRS")
public JAXBElement createOTAHotelAvailNotifRS(MessageAcknowledgementType value) {
return new JAXBElement<>(_OTAHotelAvailNotifRS_QNAME, MessageAcknowledgementType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link MessageAcknowledgementType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link MessageAcknowledgementType }{@code >}
*/
@XmlElementDecl(namespace = "http://www.opentravel.org/OTA/2003/05", name = "OTA_HotelRateAmountNotifRS")
public JAXBElement createOTAHotelRateAmountNotifRS(MessageAcknowledgementType value) {
return new JAXBElement<>(_OTAHotelRateAmountNotifRS_QNAME, MessageAcknowledgementType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link UsernameTokenType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link UsernameTokenType }{@code >}
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-secext-1.0.xsd", name = "UsernameToken")
public JAXBElement createUsernameToken(UsernameTokenType value) {
return new JAXBElement<>(_UsernameToken_QNAME, UsernameTokenType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link BinarySecurityTokenType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link BinarySecurityTokenType }{@code >}
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-secext-1.0.xsd", name = "BinarySecurityToken")
public JAXBElement createBinarySecurityToken(BinarySecurityTokenType value) {
return new JAXBElement<>(_BinarySecurityToken_QNAME, BinarySecurityTokenType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ReferenceType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ReferenceType }{@code >}
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-secext-1.0.xsd", name = "Reference")
public JAXBElement createReference(ReferenceType value) {
return new JAXBElement<>(_Reference_QNAME, ReferenceType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link EmbeddedType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link EmbeddedType }{@code >}
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-secext-1.0.xsd", name = "Embedded")
public JAXBElement createEmbedded(EmbeddedType value) {
return new JAXBElement<>(_Embedded_QNAME, EmbeddedType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link KeyIdentifierType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link KeyIdentifierType }{@code >}
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-secext-1.0.xsd", name = "KeyIdentifier")
public JAXBElement createKeyIdentifier(KeyIdentifierType value) {
return new JAXBElement<>(_KeyIdentifier_QNAME, KeyIdentifierType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link SecurityTokenReferenceType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link SecurityTokenReferenceType }{@code >}
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-secext-1.0.xsd", name = "SecurityTokenReference")
public JAXBElement createSecurityTokenReference(SecurityTokenReferenceType value) {
return new JAXBElement<>(_SecurityTokenReference_QNAME, SecurityTokenReferenceType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link SecurityHeaderType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link SecurityHeaderType }{@code >}
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-secext-1.0.xsd", name = "Security")
public JAXBElement createSecurity(SecurityHeaderType value) {
return new JAXBElement<>(_Security_QNAME, SecurityHeaderType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link TransformationParametersType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link TransformationParametersType }{@code >}
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-secext-1.0.xsd", name = "TransformationParameters")
public JAXBElement createTransformationParameters(TransformationParametersType value) {
return new JAXBElement<>(_TransformationParameters_QNAME, TransformationParametersType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link PasswordString }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link PasswordString }{@code >}
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-secext-1.0.xsd", name = "Password")
public JAXBElement createPassword(PasswordString value) {
return new JAXBElement<>(_Password_QNAME, PasswordString.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link EncodedString }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link EncodedString }{@code >}
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-secext-1.0.xsd", name = "Nonce")
public JAXBElement createNonce(EncodedString value) {
return new JAXBElement<>(_Nonce_QNAME, EncodedString.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link TimestampType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link TimestampType }{@code >}
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-utility-1.0.xsd", name = "Timestamp")
public JAXBElement createTimestamp(TimestampType value) {
return new JAXBElement<>(_Timestamp_QNAME, TimestampType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AttributedDateTime }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link AttributedDateTime }{@code >}
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-utility-1.0.xsd", name = "Expires")
public JAXBElement createExpires(AttributedDateTime value) {
return new JAXBElement<>(_Expires_QNAME, AttributedDateTime.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link AttributedDateTime }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link AttributedDateTime }{@code >}
*/
@XmlElementDecl(namespace = "http://docs.oasis-open.org/wss/2004/01/oasis-200401-wss-wssecurity-utility-1.0.xsd", name = "Created")
public JAXBElement createCreated(AttributedDateTime value) {
return new JAXBElement<>(_Created_QNAME, AttributedDateTime.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link FormattedTextTextType }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link FormattedTextTextType }{@code >}
*/
@XmlElementDecl(namespace = "http://www.opentravel.org/OTA/2003/05", name = "Text", scope = ParagraphType.class)
public JAXBElement createParagraphTypeText(FormattedTextTextType value) {
return new JAXBElement<>(_ParagraphTypeText_QNAME, FormattedTextTextType.class, ParagraphType.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*/
@XmlElementDecl(namespace = "http://www.opentravel.org/OTA/2003/05", name = "Image", scope = ParagraphType.class)
public JAXBElement createParagraphTypeImage(String value) {
return new JAXBElement<>(_ParagraphTypeImage_QNAME, String.class, ParagraphType.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link String }{@code >}
*/
@XmlElementDecl(namespace = "http://www.opentravel.org/OTA/2003/05", name = "URL", scope = ParagraphType.class)
public JAXBElement createParagraphTypeURL(String value) {
return new JAXBElement<>(_ParagraphTypeURL_QNAME, String.class, ParagraphType.class, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link ParagraphType.ListItem }{@code >}
*
* @param value
* Java instance representing xml element's value.
* @return
* the new instance of {@link JAXBElement }{@code <}{@link ParagraphType.ListItem }{@code >}
*/
@XmlElementDecl(namespace = "http://www.opentravel.org/OTA/2003/05", name = "ListItem", scope = ParagraphType.class)
public JAXBElement createParagraphTypeListItem(ParagraphType.ListItem value) {
return new JAXBElement<>(_ParagraphTypeListItem_QNAME, ParagraphType.ListItem.class, ParagraphType.class, value);
}
}