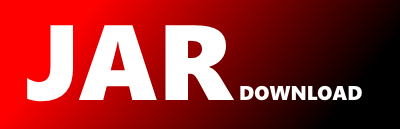
travel.wink.sdk.booking.engine.api.InventoryApi Maven / Gradle / Ivy
Show all versions of booking-engine-sdk-java Show documentation
package travel.wink.sdk.booking.engine.api;
import travel.wink.sdk.booking.engine.invoker.ApiClient;
import travel.wink.sdk.booking.engine.model.AggregateActivityRequest;
import travel.wink.sdk.booking.engine.model.AggregateAddOnRequest;
import travel.wink.sdk.booking.engine.model.AggregateAttractionRequest;
import travel.wink.sdk.booking.engine.model.AggregateGuestRoomRequest;
import travel.wink.sdk.booking.engine.model.AggregateHotelRequest;
import travel.wink.sdk.booking.engine.model.AggregateInventoryGridItemRequest;
import travel.wink.sdk.booking.engine.model.AggregateMeetingRoomRequest;
import travel.wink.sdk.booking.engine.model.AggregatePackageRequest;
import travel.wink.sdk.booking.engine.model.AggregatePlaceRequest;
import travel.wink.sdk.booking.engine.model.AggregateRestaurantRequest;
import travel.wink.sdk.booking.engine.model.AggregateSellerInventoryListRequest;
import travel.wink.sdk.booking.engine.model.AggregateSpaRequest;
import travel.wink.sdk.booking.engine.model.CityScoreRequest;
import travel.wink.sdk.booking.engine.model.CitySearchRequest;
import travel.wink.sdk.booking.engine.model.CountryScoreRequest;
import travel.wink.sdk.booking.engine.model.GenericErrorMessage;
import travel.wink.sdk.booking.engine.model.GlobalScoreRequest;
import travel.wink.sdk.booking.engine.model.HotelInventoryListRequest;
import travel.wink.sdk.booking.engine.model.HotelInventoryListResponse;
import travel.wink.sdk.booking.engine.model.HotelInventoryRequest;
import travel.wink.sdk.booking.engine.model.HotelInventoryResponse;
import travel.wink.sdk.booking.engine.model.InventoryGridItem;
import travel.wink.sdk.booking.engine.model.MapRequest;
import travel.wink.sdk.booking.engine.model.Oauth2SearchByGeoLocation400Response;
import travel.wink.sdk.booking.engine.model.PageHotelWithBestPrice;
import travel.wink.sdk.booking.engine.model.SellerInventoryActivity;
import travel.wink.sdk.booking.engine.model.SellerInventoryAddOn;
import travel.wink.sdk.booking.engine.model.SellerInventoryAttraction;
import travel.wink.sdk.booking.engine.model.SellerInventoryGuestRoom;
import travel.wink.sdk.booking.engine.model.SellerInventoryHotel;
import travel.wink.sdk.booking.engine.model.SellerInventoryListResponse;
import travel.wink.sdk.booking.engine.model.SellerInventoryMeetingRoom;
import travel.wink.sdk.booking.engine.model.SellerInventoryPackage;
import travel.wink.sdk.booking.engine.model.SellerInventoryPlace;
import travel.wink.sdk.booking.engine.model.SellerInventoryRankedListResponse;
import travel.wink.sdk.booking.engine.model.SellerInventoryRestaurant;
import travel.wink.sdk.booking.engine.model.SellerInventorySpa;
import java.util.HashMap;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import java.util.stream.Collectors;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
import org.springframework.core.ParameterizedTypeReference;
import org.springframework.web.reactive.function.client.WebClient.ResponseSpec;
import org.springframework.web.reactive.function.client.WebClientResponseException;
import org.springframework.core.io.FileSystemResource;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpMethod;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import reactor.core.publisher.Mono;
import reactor.core.publisher.Flux;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2022-06-29T13:20:36.800596+07:00[Asia/Bangkok]")
public class InventoryApi {
private ApiClient apiClient;
public InventoryApi() {
this(new ApiClient());
}
@Autowired
public InventoryApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Ranked City Search
* Search for hotels in / around a city and order by scoring type
* 405 - Method Not Allowed
*
415 - Unsupported Media Type
*
400 - Bad Request
*
403 - Forbidden
*
401 - Unauthorized
*
500 - Internal Server Error
*
404 - Not Found
*
503 - Service Unavailable
*
200 - OK
* @param cityScoreRequest The cityScoreRequest parameter
* @param engineConfigurationIdentifier Engine configuration identifier
* @param accept The accept parameter
* @return PageHotelWithBestPrice
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec oauth2ScoreInventoryByCityRequestCreation(CityScoreRequest cityScoreRequest, String engineConfigurationIdentifier, String accept) throws WebClientResponseException {
Object postBody = cityScoreRequest;
// verify the required parameter 'cityScoreRequest' is set
if (cityScoreRequest == null) {
throw new WebClientResponseException("Missing the required parameter 'cityScoreRequest' when calling oauth2ScoreInventoryByCity", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "engineConfigurationIdentifier", engineConfigurationIdentifier));
if (accept != null)
headerParams.add("Accept", apiClient.parameterToString(accept));
final String[] localVarAccepts = {
"application/json", "application/vnd.platform-v1+json", "application/xml", "text/xml", "text/plain", "*/*"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "oauth2_client_credentials" };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/api/ranked/search/city", HttpMethod.POST, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Ranked City Search
* Search for hotels in / around a city and order by scoring type
* 405 - Method Not Allowed
*
415 - Unsupported Media Type
*
400 - Bad Request
*
403 - Forbidden
*
401 - Unauthorized
*
500 - Internal Server Error
*
404 - Not Found
*
503 - Service Unavailable
*
200 - OK
* @param cityScoreRequest The cityScoreRequest parameter
* @param engineConfigurationIdentifier Engine configuration identifier
* @param accept The accept parameter
* @return PageHotelWithBestPrice
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono oauth2ScoreInventoryByCity(CityScoreRequest cityScoreRequest, String engineConfigurationIdentifier, String accept) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return oauth2ScoreInventoryByCityRequestCreation(cityScoreRequest, engineConfigurationIdentifier, accept).bodyToMono(localVarReturnType);
}
public Mono> oauth2ScoreInventoryByCityWithHttpInfo(CityScoreRequest cityScoreRequest, String engineConfigurationIdentifier, String accept) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return oauth2ScoreInventoryByCityRequestCreation(cityScoreRequest, engineConfigurationIdentifier, accept).toEntity(localVarReturnType);
}
/**
* By Geo-Location
* Search for properties near a point or within bounds. Populate either `request.userSession.location` OR `request.userSession.bounds`.
* 405 - Method Not Allowed
*
415 - Unsupported Media Type
*
400 - Bad Request
*
403 - Forbidden
*
401 - Unauthorized
*
500 - Internal Server Error
*
404 - Not Found
*
503 - Service Unavailable
*
200 - OK
* @param mapRequest The mapRequest parameter
* @param engineConfigurationIdentifier Engine configuration identifier
* @param accept The accept parameter
* @return PageHotelWithBestPrice
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec oauth2SearchByGeoLocationRequestCreation(MapRequest mapRequest, String engineConfigurationIdentifier, String accept) throws WebClientResponseException {
Object postBody = mapRequest;
// verify the required parameter 'mapRequest' is set
if (mapRequest == null) {
throw new WebClientResponseException("Missing the required parameter 'mapRequest' when calling oauth2SearchByGeoLocation", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "engineConfigurationIdentifier", engineConfigurationIdentifier));
if (accept != null)
headerParams.add("Accept", apiClient.parameterToString(accept));
final String[] localVarAccepts = {
"application/json", "application/vnd.platform-v1+json", "application/xml", "text/xml", "text/plain", "*/*"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "oauth2_client_credentials" };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/api/search/geo", HttpMethod.POST, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* By Geo-Location
* Search for properties near a point or within bounds. Populate either `request.userSession.location` OR `request.userSession.bounds`.
* 405 - Method Not Allowed
*
415 - Unsupported Media Type
*
400 - Bad Request
*
403 - Forbidden
*
401 - Unauthorized
*
500 - Internal Server Error
*
404 - Not Found
*
503 - Service Unavailable
*
200 - OK
* @param mapRequest The mapRequest parameter
* @param engineConfigurationIdentifier Engine configuration identifier
* @param accept The accept parameter
* @return PageHotelWithBestPrice
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono oauth2SearchByGeoLocation(MapRequest mapRequest, String engineConfigurationIdentifier, String accept) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return oauth2SearchByGeoLocationRequestCreation(mapRequest, engineConfigurationIdentifier, accept).bodyToMono(localVarReturnType);
}
public Mono> oauth2SearchByGeoLocationWithHttpInfo(MapRequest mapRequest, String engineConfigurationIdentifier, String accept) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return oauth2SearchByGeoLocationRequestCreation(mapRequest, engineConfigurationIdentifier, accept).toEntity(localVarReturnType);
}
/**
* City Search
* Search for hotels in / around a city
* 405 - Method Not Allowed
*
415 - Unsupported Media Type
*
400 - Bad Request
*
403 - Forbidden
*
401 - Unauthorized
*
500 - Internal Server Error
*
404 - Not Found
*
503 - Service Unavailable
*
200 - OK
* @param citySearchRequest The citySearchRequest parameter
* @param engineConfigurationIdentifier Engine configuration identifier
* @param accept The accept parameter
* @return PageHotelWithBestPrice
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec oauth2SearchInventoryByCityRequestCreation(CitySearchRequest citySearchRequest, String engineConfigurationIdentifier, String accept) throws WebClientResponseException {
Object postBody = citySearchRequest;
// verify the required parameter 'citySearchRequest' is set
if (citySearchRequest == null) {
throw new WebClientResponseException("Missing the required parameter 'citySearchRequest' when calling oauth2SearchInventoryByCity", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "engineConfigurationIdentifier", engineConfigurationIdentifier));
if (accept != null)
headerParams.add("Accept", apiClient.parameterToString(accept));
final String[] localVarAccepts = {
"application/json", "application/vnd.platform-v1+json", "application/xml", "text/xml", "text/plain", "*/*"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "oauth2_client_credentials" };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/api/search/city", HttpMethod.POST, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* City Search
* Search for hotels in / around a city
* 405 - Method Not Allowed
*
415 - Unsupported Media Type
*
400 - Bad Request
*
403 - Forbidden
*
401 - Unauthorized
*
500 - Internal Server Error
*
404 - Not Found
*
503 - Service Unavailable
*
200 - OK
* @param citySearchRequest The citySearchRequest parameter
* @param engineConfigurationIdentifier Engine configuration identifier
* @param accept The accept parameter
* @return PageHotelWithBestPrice
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono oauth2SearchInventoryByCity(CitySearchRequest citySearchRequest, String engineConfigurationIdentifier, String accept) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return oauth2SearchInventoryByCityRequestCreation(citySearchRequest, engineConfigurationIdentifier, accept).bodyToMono(localVarReturnType);
}
public Mono> oauth2SearchInventoryByCityWithHttpInfo(CitySearchRequest citySearchRequest, String engineConfigurationIdentifier, String accept) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return oauth2SearchInventoryByCityRequestCreation(citySearchRequest, engineConfigurationIdentifier, accept).toEntity(localVarReturnType);
}
/**
* Ranked Country Search
* Search for hotels in a country and order by scoring type
* 405 - Method Not Allowed
*
415 - Unsupported Media Type
*
400 - Bad Request
*
403 - Forbidden
*
401 - Unauthorized
*
500 - Internal Server Error
*
404 - Not Found
*
503 - Service Unavailable
*
200 - OK
* @param countryScoreRequest The countryScoreRequest parameter
* @param engineConfigurationIdentifier Engine configuration identifier
* @param accept The accept parameter
* @return PageHotelWithBestPrice
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec oauth2SearchScoreByCountryRequestCreation(CountryScoreRequest countryScoreRequest, String engineConfigurationIdentifier, String accept) throws WebClientResponseException {
Object postBody = countryScoreRequest;
// verify the required parameter 'countryScoreRequest' is set
if (countryScoreRequest == null) {
throw new WebClientResponseException("Missing the required parameter 'countryScoreRequest' when calling oauth2SearchScoreByCountry", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "engineConfigurationIdentifier", engineConfigurationIdentifier));
if (accept != null)
headerParams.add("Accept", apiClient.parameterToString(accept));
final String[] localVarAccepts = {
"application/json", "application/vnd.platform-v1+json", "application/xml", "text/xml", "text/plain", "*/*"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "oauth2_client_credentials" };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/api/ranked/search/country", HttpMethod.POST, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Ranked Country Search
* Search for hotels in a country and order by scoring type
* 405 - Method Not Allowed
*
415 - Unsupported Media Type
*
400 - Bad Request
*
403 - Forbidden
*
401 - Unauthorized
*
500 - Internal Server Error
*
404 - Not Found
*
503 - Service Unavailable
*
200 - OK
* @param countryScoreRequest The countryScoreRequest parameter
* @param engineConfigurationIdentifier Engine configuration identifier
* @param accept The accept parameter
* @return PageHotelWithBestPrice
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono oauth2SearchScoreByCountry(CountryScoreRequest countryScoreRequest, String engineConfigurationIdentifier, String accept) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return oauth2SearchScoreByCountryRequestCreation(countryScoreRequest, engineConfigurationIdentifier, accept).bodyToMono(localVarReturnType);
}
public Mono> oauth2SearchScoreByCountryWithHttpInfo(CountryScoreRequest countryScoreRequest, String engineConfigurationIdentifier, String accept) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return oauth2SearchScoreByCountryRequestCreation(countryScoreRequest, engineConfigurationIdentifier, accept).toEntity(localVarReturnType);
}
/**
* Ranked Global Search
* Search best scoring hotels in the world and order by scoring type
* 405 - Method Not Allowed
*
415 - Unsupported Media Type
*
400 - Bad Request
*
403 - Forbidden
*
401 - Unauthorized
*
500 - Internal Server Error
*
404 - Not Found
*
503 - Service Unavailable
*
200 - OK
* @param globalScoreRequest The globalScoreRequest parameter
* @param engineConfigurationIdentifier Engine configuration identifier
* @param accept The accept parameter
* @return PageHotelWithBestPrice
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec oauth2SearchScoreByGlobalRequestCreation(GlobalScoreRequest globalScoreRequest, String engineConfigurationIdentifier, String accept) throws WebClientResponseException {
Object postBody = globalScoreRequest;
// verify the required parameter 'globalScoreRequest' is set
if (globalScoreRequest == null) {
throw new WebClientResponseException("Missing the required parameter 'globalScoreRequest' when calling oauth2SearchScoreByGlobal", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "engineConfigurationIdentifier", engineConfigurationIdentifier));
if (accept != null)
headerParams.add("Accept", apiClient.parameterToString(accept));
final String[] localVarAccepts = {
"application/json", "application/vnd.platform-v1+json", "application/xml", "text/xml", "text/plain", "*/*"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "oauth2_client_credentials" };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/api/ranked/search/global", HttpMethod.POST, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Ranked Global Search
* Search best scoring hotels in the world and order by scoring type
* 405 - Method Not Allowed
*
415 - Unsupported Media Type
*
400 - Bad Request
*
403 - Forbidden
*
401 - Unauthorized
*
500 - Internal Server Error
*
404 - Not Found
*
503 - Service Unavailable
*
200 - OK
* @param globalScoreRequest The globalScoreRequest parameter
* @param engineConfigurationIdentifier Engine configuration identifier
* @param accept The accept parameter
* @return PageHotelWithBestPrice
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono oauth2SearchScoreByGlobal(GlobalScoreRequest globalScoreRequest, String engineConfigurationIdentifier, String accept) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return oauth2SearchScoreByGlobalRequestCreation(globalScoreRequest, engineConfigurationIdentifier, accept).bodyToMono(localVarReturnType);
}
public Mono> oauth2SearchScoreByGlobalWithHttpInfo(GlobalScoreRequest globalScoreRequest, String engineConfigurationIdentifier, String accept) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return oauth2SearchScoreByGlobalRequestCreation(globalScoreRequest, engineConfigurationIdentifier, accept).toEntity(localVarReturnType);
}
/**
* Show Inventory
* Shows single grid based on channel inventory identifier
* 405 - Method Not Allowed
*
415 - Unsupported Media Type
*
400 - Bad Request
*
403 - Forbidden
*
401 - Unauthorized
*
500 - Internal Server Error
*
404 - Not Found
*
503 - Service Unavailable
*
200 - OK
* @param aggregateInventoryGridItemRequest The aggregateInventoryGridItemRequest parameter
* @param engineConfigurationIdentifier Engine configuration identifier
* @param accept The accept parameter
* @return InventoryGridItem
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec oauth2ShowInventoryGridItemRequestCreation(AggregateInventoryGridItemRequest aggregateInventoryGridItemRequest, String engineConfigurationIdentifier, String accept) throws WebClientResponseException {
Object postBody = aggregateInventoryGridItemRequest;
// verify the required parameter 'aggregateInventoryGridItemRequest' is set
if (aggregateInventoryGridItemRequest == null) {
throw new WebClientResponseException("Missing the required parameter 'aggregateInventoryGridItemRequest' when calling oauth2ShowInventoryGridItem", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "engineConfigurationIdentifier", engineConfigurationIdentifier));
if (accept != null)
headerParams.add("Accept", apiClient.parameterToString(accept));
final String[] localVarAccepts = {
"application/json", "application/vnd.platform-v1+json", "application/xml", "text/xml", "text/plain", "*/*"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "oauth2_client_credentials" };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/api/inventory/grid/item", HttpMethod.POST, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Show Inventory
* Shows single grid based on channel inventory identifier
* 405 - Method Not Allowed
*
415 - Unsupported Media Type
*
400 - Bad Request
*
403 - Forbidden
*
401 - Unauthorized
*
500 - Internal Server Error
*
404 - Not Found
*
503 - Service Unavailable
*
200 - OK
* @param aggregateInventoryGridItemRequest The aggregateInventoryGridItemRequest parameter
* @param engineConfigurationIdentifier Engine configuration identifier
* @param accept The accept parameter
* @return InventoryGridItem
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono oauth2ShowInventoryGridItem(AggregateInventoryGridItemRequest aggregateInventoryGridItemRequest, String engineConfigurationIdentifier, String accept) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return oauth2ShowInventoryGridItemRequestCreation(aggregateInventoryGridItemRequest, engineConfigurationIdentifier, accept).bodyToMono(localVarReturnType);
}
public Mono> oauth2ShowInventoryGridItemWithHttpInfo(AggregateInventoryGridItemRequest aggregateInventoryGridItemRequest, String engineConfigurationIdentifier, String accept) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return oauth2ShowInventoryGridItemRequestCreation(aggregateInventoryGridItemRequest, engineConfigurationIdentifier, accept).toEntity(localVarReturnType);
}
/**
* Show Add-On
* Show single add-on based on a channel inventory identifier
* 405 - Method Not Allowed
*
415 - Unsupported Media Type
*
400 - Bad Request
*
403 - Forbidden
*
401 - Unauthorized
*
500 - Internal Server Error
*
404 - Not Found
*
503 - Service Unavailable
*
200 - OK
* @param aggregateAddOnRequest The aggregateAddOnRequest parameter
* @param engineConfigurationIdentifier Engine configuration identifier
* @param accept The accept parameter
* @return SellerInventoryAddOn
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec oauth2ShowPropertyAddOnRequestCreation(AggregateAddOnRequest aggregateAddOnRequest, String engineConfigurationIdentifier, String accept) throws WebClientResponseException {
Object postBody = aggregateAddOnRequest;
// verify the required parameter 'aggregateAddOnRequest' is set
if (aggregateAddOnRequest == null) {
throw new WebClientResponseException("Missing the required parameter 'aggregateAddOnRequest' when calling oauth2ShowPropertyAddOn", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "engineConfigurationIdentifier", engineConfigurationIdentifier));
if (accept != null)
headerParams.add("Accept", apiClient.parameterToString(accept));
final String[] localVarAccepts = {
"application/json", "application/vnd.platform-v1+json", "application/xml", "text/xml", "text/plain", "*/*"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "oauth2_client_credentials" };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/api/inventory/upgrade", HttpMethod.POST, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Show Add-On
* Show single add-on based on a channel inventory identifier
* 405 - Method Not Allowed
*
415 - Unsupported Media Type
*
400 - Bad Request
*
403 - Forbidden
*
401 - Unauthorized
*
500 - Internal Server Error
*
404 - Not Found
*
503 - Service Unavailable
*
200 - OK
* @param aggregateAddOnRequest The aggregateAddOnRequest parameter
* @param engineConfigurationIdentifier Engine configuration identifier
* @param accept The accept parameter
* @return SellerInventoryAddOn
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono oauth2ShowPropertyAddOn(AggregateAddOnRequest aggregateAddOnRequest, String engineConfigurationIdentifier, String accept) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return oauth2ShowPropertyAddOnRequestCreation(aggregateAddOnRequest, engineConfigurationIdentifier, accept).bodyToMono(localVarReturnType);
}
public Mono> oauth2ShowPropertyAddOnWithHttpInfo(AggregateAddOnRequest aggregateAddOnRequest, String engineConfigurationIdentifier, String accept) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return oauth2ShowPropertyAddOnRequestCreation(aggregateAddOnRequest, engineConfigurationIdentifier, accept).toEntity(localVarReturnType);
}
/**
* Show Property
* Show single hotel card with price based on a given record identifier
* 405 - Method Not Allowed
*
415 - Unsupported Media Type
*
400 - Bad Request
*
403 - Forbidden
*
401 - Unauthorized
*
500 - Internal Server Error
*
404 - Not Found
*
503 - Service Unavailable
*
200 - OK
* @param aggregateHotelRequest The aggregateHotelRequest parameter
* @param engineConfigurationIdentifier Engine configuration identifier
* @param accept The accept parameter
* @return SellerInventoryHotel
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec oauth2ShowPropertyAggregateRequestCreation(AggregateHotelRequest aggregateHotelRequest, String engineConfigurationIdentifier, String accept) throws WebClientResponseException {
Object postBody = aggregateHotelRequest;
// verify the required parameter 'aggregateHotelRequest' is set
if (aggregateHotelRequest == null) {
throw new WebClientResponseException("Missing the required parameter 'aggregateHotelRequest' when calling oauth2ShowPropertyAggregate", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "engineConfigurationIdentifier", engineConfigurationIdentifier));
if (accept != null)
headerParams.add("Accept", apiClient.parameterToString(accept));
final String[] localVarAccepts = {
"application/json", "application/vnd.platform-v1+json", "application/xml", "text/xml", "text/plain", "*/*"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "oauth2_client_credentials" };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/api/inventory/property", HttpMethod.POST, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Show Property
* Show single hotel card with price based on a given record identifier
* 405 - Method Not Allowed
*
415 - Unsupported Media Type
*
400 - Bad Request
*
403 - Forbidden
*
401 - Unauthorized
*
500 - Internal Server Error
*
404 - Not Found
*
503 - Service Unavailable
*
200 - OK
* @param aggregateHotelRequest The aggregateHotelRequest parameter
* @param engineConfigurationIdentifier Engine configuration identifier
* @param accept The accept parameter
* @return SellerInventoryHotel
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono oauth2ShowPropertyAggregate(AggregateHotelRequest aggregateHotelRequest, String engineConfigurationIdentifier, String accept) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return oauth2ShowPropertyAggregateRequestCreation(aggregateHotelRequest, engineConfigurationIdentifier, accept).bodyToMono(localVarReturnType);
}
public Mono> oauth2ShowPropertyAggregateWithHttpInfo(AggregateHotelRequest aggregateHotelRequest, String engineConfigurationIdentifier, String accept) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return oauth2ShowPropertyAggregateRequestCreation(aggregateHotelRequest, engineConfigurationIdentifier, accept).toEntity(localVarReturnType);
}
/**
* Show Attraction
* Show single attraction based on a channel inventory identifier
* 405 - Method Not Allowed
*
415 - Unsupported Media Type
*
400 - Bad Request
*
403 - Forbidden
*
401 - Unauthorized
*
500 - Internal Server Error
*
404 - Not Found
*
503 - Service Unavailable
*
200 - OK
* @param aggregateAttractionRequest The aggregateAttractionRequest parameter
* @param engineConfigurationIdentifier Engine configuration identifier
* @param accept The accept parameter
* @return SellerInventoryAttraction
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec oauth2ShowPropertyAttractionRequestCreation(AggregateAttractionRequest aggregateAttractionRequest, String engineConfigurationIdentifier, String accept) throws WebClientResponseException {
Object postBody = aggregateAttractionRequest;
// verify the required parameter 'aggregateAttractionRequest' is set
if (aggregateAttractionRequest == null) {
throw new WebClientResponseException("Missing the required parameter 'aggregateAttractionRequest' when calling oauth2ShowPropertyAttraction", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "engineConfigurationIdentifier", engineConfigurationIdentifier));
if (accept != null)
headerParams.add("Accept", apiClient.parameterToString(accept));
final String[] localVarAccepts = {
"application/json", "application/vnd.platform-v1+json", "application/xml", "text/xml", "text/plain", "*/*"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "oauth2_client_credentials" };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/api/inventory/attraction", HttpMethod.POST, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Show Attraction
* Show single attraction based on a channel inventory identifier
* 405 - Method Not Allowed
*
415 - Unsupported Media Type
*
400 - Bad Request
*
403 - Forbidden
*
401 - Unauthorized
*
500 - Internal Server Error
*
404 - Not Found
*
503 - Service Unavailable
*
200 - OK
* @param aggregateAttractionRequest The aggregateAttractionRequest parameter
* @param engineConfigurationIdentifier Engine configuration identifier
* @param accept The accept parameter
* @return SellerInventoryAttraction
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono oauth2ShowPropertyAttraction(AggregateAttractionRequest aggregateAttractionRequest, String engineConfigurationIdentifier, String accept) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return oauth2ShowPropertyAttractionRequestCreation(aggregateAttractionRequest, engineConfigurationIdentifier, accept).bodyToMono(localVarReturnType);
}
public Mono> oauth2ShowPropertyAttractionWithHttpInfo(AggregateAttractionRequest aggregateAttractionRequest, String engineConfigurationIdentifier, String accept) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return oauth2ShowPropertyAttractionRequestCreation(aggregateAttractionRequest, engineConfigurationIdentifier, accept).toEntity(localVarReturnType);
}
/**
* Show property
* Show property content / availability / rate details.
* 405 - Method Not Allowed
*
415 - Unsupported Media Type
*
400 - Bad Request
*
403 - Forbidden
*
401 - Unauthorized
*
500 - Internal Server Error
*
404 - Not Found
*
503 - Service Unavailable
*
200 - OK
* @param hotelInventoryRequest The hotelInventoryRequest parameter
* @param engineConfigurationIdentifier Engine configuration identifier
* @return HotelInventoryResponse
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec oauth2ShowPropertyInventoryRequestCreation(HotelInventoryRequest hotelInventoryRequest, String engineConfigurationIdentifier) throws WebClientResponseException {
Object postBody = hotelInventoryRequest;
// verify the required parameter 'hotelInventoryRequest' is set
if (hotelInventoryRequest == null) {
throw new WebClientResponseException("Missing the required parameter 'hotelInventoryRequest' when calling oauth2ShowPropertyInventory", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "engineConfigurationIdentifier", engineConfigurationIdentifier));
final String[] localVarAccepts = {
"application/json", "application/vnd.platform-v1+json", "application/xml", "text/xml", "text/plain", "*/*"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/vnd.platform-v1+json", "application/json"
};
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "oauth2_client_credentials" };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/api/inventory", HttpMethod.POST, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Show property
* Show property content / availability / rate details.
* 405 - Method Not Allowed
*
415 - Unsupported Media Type
*
400 - Bad Request
*
403 - Forbidden
*
401 - Unauthorized
*
500 - Internal Server Error
*
404 - Not Found
*
503 - Service Unavailable
*
200 - OK
* @param hotelInventoryRequest The hotelInventoryRequest parameter
* @param engineConfigurationIdentifier Engine configuration identifier
* @return HotelInventoryResponse
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono oauth2ShowPropertyInventory(HotelInventoryRequest hotelInventoryRequest, String engineConfigurationIdentifier) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return oauth2ShowPropertyInventoryRequestCreation(hotelInventoryRequest, engineConfigurationIdentifier).bodyToMono(localVarReturnType);
}
public Mono> oauth2ShowPropertyInventoryWithHttpInfo(HotelInventoryRequest hotelInventoryRequest, String engineConfigurationIdentifier) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return oauth2ShowPropertyInventoryRequestCreation(hotelInventoryRequest, engineConfigurationIdentifier).toEntity(localVarReturnType);
}
/**
* Show Property List
* Show property content / availability / rate details.
* 405 - Method Not Allowed
*
415 - Unsupported Media Type
*
400 - Bad Request
*
403 - Forbidden
*
401 - Unauthorized
*
500 - Internal Server Error
*
404 - Not Found
*
503 - Service Unavailable
*
200 - OK
* @param hotelInventoryListRequest The hotelInventoryListRequest parameter
* @param engineConfigurationIdentifier Engine configuration identifier
* @return HotelInventoryListResponse
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec oauth2ShowPropertyListRequestCreation(HotelInventoryListRequest hotelInventoryListRequest, String engineConfigurationIdentifier) throws WebClientResponseException {
Object postBody = hotelInventoryListRequest;
// verify the required parameter 'hotelInventoryListRequest' is set
if (hotelInventoryListRequest == null) {
throw new WebClientResponseException("Missing the required parameter 'hotelInventoryListRequest' when calling oauth2ShowPropertyList", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "engineConfigurationIdentifier", engineConfigurationIdentifier));
final String[] localVarAccepts = {
"application/json", "application/vnd.platform-v1+json", "application/xml", "text/xml", "text/plain", "*/*"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/vnd.platform-v1+json", "application/json"
};
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "oauth2_client_credentials" };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/api/inventory/list", HttpMethod.POST, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Show Property List
* Show property content / availability / rate details.
* 405 - Method Not Allowed
*
415 - Unsupported Media Type
*
400 - Bad Request
*
403 - Forbidden
*
401 - Unauthorized
*
500 - Internal Server Error
*
404 - Not Found
*
503 - Service Unavailable
*
200 - OK
* @param hotelInventoryListRequest The hotelInventoryListRequest parameter
* @param engineConfigurationIdentifier Engine configuration identifier
* @return HotelInventoryListResponse
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono oauth2ShowPropertyList(HotelInventoryListRequest hotelInventoryListRequest, String engineConfigurationIdentifier) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return oauth2ShowPropertyListRequestCreation(hotelInventoryListRequest, engineConfigurationIdentifier).bodyToMono(localVarReturnType);
}
public Mono> oauth2ShowPropertyListWithHttpInfo(HotelInventoryListRequest hotelInventoryListRequest, String engineConfigurationIdentifier) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return oauth2ShowPropertyListRequestCreation(hotelInventoryListRequest, engineConfigurationIdentifier).toEntity(localVarReturnType);
}
/**
* Show Meeting Room
* Show single meeting room based on a channel inventory identifier
* 405 - Method Not Allowed
*
415 - Unsupported Media Type
*
400 - Bad Request
*
403 - Forbidden
*
401 - Unauthorized
*
500 - Internal Server Error
*
404 - Not Found
*
503 - Service Unavailable
*
200 - OK
* @param aggregateMeetingRoomRequest The aggregateMeetingRoomRequest parameter
* @param engineConfigurationIdentifier Engine configuration identifier
* @param accept The accept parameter
* @return SellerInventoryMeetingRoom
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec oauth2ShowPropertyMeetingRoomRequestCreation(AggregateMeetingRoomRequest aggregateMeetingRoomRequest, String engineConfigurationIdentifier, String accept) throws WebClientResponseException {
Object postBody = aggregateMeetingRoomRequest;
// verify the required parameter 'aggregateMeetingRoomRequest' is set
if (aggregateMeetingRoomRequest == null) {
throw new WebClientResponseException("Missing the required parameter 'aggregateMeetingRoomRequest' when calling oauth2ShowPropertyMeetingRoom", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "engineConfigurationIdentifier", engineConfigurationIdentifier));
if (accept != null)
headerParams.add("Accept", apiClient.parameterToString(accept));
final String[] localVarAccepts = {
"application/json", "application/vnd.platform-v1+json", "application/xml", "text/xml", "text/plain", "*/*"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "oauth2_client_credentials" };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/api/inventory/meetingroom", HttpMethod.POST, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Show Meeting Room
* Show single meeting room based on a channel inventory identifier
* 405 - Method Not Allowed
*
415 - Unsupported Media Type
*
400 - Bad Request
*
403 - Forbidden
*
401 - Unauthorized
*
500 - Internal Server Error
*
404 - Not Found
*
503 - Service Unavailable
*
200 - OK
* @param aggregateMeetingRoomRequest The aggregateMeetingRoomRequest parameter
* @param engineConfigurationIdentifier Engine configuration identifier
* @param accept The accept parameter
* @return SellerInventoryMeetingRoom
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono oauth2ShowPropertyMeetingRoom(AggregateMeetingRoomRequest aggregateMeetingRoomRequest, String engineConfigurationIdentifier, String accept) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return oauth2ShowPropertyMeetingRoomRequestCreation(aggregateMeetingRoomRequest, engineConfigurationIdentifier, accept).bodyToMono(localVarReturnType);
}
public Mono> oauth2ShowPropertyMeetingRoomWithHttpInfo(AggregateMeetingRoomRequest aggregateMeetingRoomRequest, String engineConfigurationIdentifier, String accept) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return oauth2ShowPropertyMeetingRoomRequestCreation(aggregateMeetingRoomRequest, engineConfigurationIdentifier, accept).toEntity(localVarReturnType);
}
/**
* Show Package
* Show single package based on a channel inventory identifier
* 405 - Method Not Allowed
*
415 - Unsupported Media Type
*
400 - Bad Request
*
403 - Forbidden
*
401 - Unauthorized
*
500 - Internal Server Error
*
404 - Not Found
*
503 - Service Unavailable
*
200 - OK
* @param aggregatePackageRequest The aggregatePackageRequest parameter
* @param engineConfigurationIdentifier Engine configuration identifier
* @param accept The accept parameter
* @return SellerInventoryPackage
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec oauth2ShowPropertyPackageRequestCreation(AggregatePackageRequest aggregatePackageRequest, String engineConfigurationIdentifier, String accept) throws WebClientResponseException {
Object postBody = aggregatePackageRequest;
// verify the required parameter 'aggregatePackageRequest' is set
if (aggregatePackageRequest == null) {
throw new WebClientResponseException("Missing the required parameter 'aggregatePackageRequest' when calling oauth2ShowPropertyPackage", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "engineConfigurationIdentifier", engineConfigurationIdentifier));
if (accept != null)
headerParams.add("Accept", apiClient.parameterToString(accept));
final String[] localVarAccepts = {
"application/json", "application/vnd.platform-v1+json", "application/xml", "text/xml", "text/plain", "*/*"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "oauth2_client_credentials" };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/api/inventory/bundle", HttpMethod.POST, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Show Package
* Show single package based on a channel inventory identifier
* 405 - Method Not Allowed
*
415 - Unsupported Media Type
*
400 - Bad Request
*
403 - Forbidden
*
401 - Unauthorized
*
500 - Internal Server Error
*
404 - Not Found
*
503 - Service Unavailable
*
200 - OK
* @param aggregatePackageRequest The aggregatePackageRequest parameter
* @param engineConfigurationIdentifier Engine configuration identifier
* @param accept The accept parameter
* @return SellerInventoryPackage
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono oauth2ShowPropertyPackage(AggregatePackageRequest aggregatePackageRequest, String engineConfigurationIdentifier, String accept) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return oauth2ShowPropertyPackageRequestCreation(aggregatePackageRequest, engineConfigurationIdentifier, accept).bodyToMono(localVarReturnType);
}
public Mono> oauth2ShowPropertyPackageWithHttpInfo(AggregatePackageRequest aggregatePackageRequest, String engineConfigurationIdentifier, String accept) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return oauth2ShowPropertyPackageRequestCreation(aggregatePackageRequest, engineConfigurationIdentifier, accept).toEntity(localVarReturnType);
}
/**
* Show Place
* Show single place based on a channel inventory identifier
* 405 - Method Not Allowed
*
415 - Unsupported Media Type
*
400 - Bad Request
*
403 - Forbidden
*
401 - Unauthorized
*
500 - Internal Server Error
*
404 - Not Found
*
503 - Service Unavailable
*
200 - OK
* @param aggregatePlaceRequest The aggregatePlaceRequest parameter
* @param engineConfigurationIdentifier Engine configuration identifier
* @param accept The accept parameter
* @return SellerInventoryPlace
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec oauth2ShowPropertyPlaceRequestCreation(AggregatePlaceRequest aggregatePlaceRequest, String engineConfigurationIdentifier, String accept) throws WebClientResponseException {
Object postBody = aggregatePlaceRequest;
// verify the required parameter 'aggregatePlaceRequest' is set
if (aggregatePlaceRequest == null) {
throw new WebClientResponseException("Missing the required parameter 'aggregatePlaceRequest' when calling oauth2ShowPropertyPlace", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
final MultiValueMap