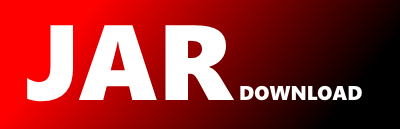
travel.wink.sdk.booking.engine.model.HotelInventoryResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of booking-engine-sdk-java Show documentation
Show all versions of booking-engine-sdk-java Show documentation
Java SDK for the wink Booking Engine API
/*
* Wink API
* ## APIs Not every integrator needs every APIs. For that reason, we have separated APIs into context. - [Affiliate](/docs?api=affiliate): All APIs related to selling travel inventory as an affiliate. - [Extranet](/docs?api=extranet): All APIs related to managing travel inventory and suppliers. - [Booking Engine](/docs?api=booking-engine): All APIs related to searching for travel inventory and creating bookings. - [Channel Manager](https://integration.wink.travel/docs?api=channel-manager): All APIs related to channel managers who want to integrate with our payment. ## SDKs We are actively working on supporting the most used languages out there. If you don't see your language here, reach out to us with a request to officially add your language. In the meantime, if you want to roll your own SDK, you can do so by downloading the OpenAPI spec and using one of the many available OpenAPI generators available: [https://openapi-generator.tech/docs/generators](https://openapi-generator.tech/docs/generators). - Java SDK [https://github.com/wink-travel/wink-sdk-java](https://github.com/wink-travel/wink-sdk-java) ## Usage These features are made available to you via a [REST API](https://en.wikipedia.org/wiki/Representational_state_transfer). This API is language agnostic. ## Versioning We chose to version our endpoints in a way that we hope affects your integration with us the least. You request the version of our API you wish to work with via the `Accept` header and by using our custom JSON mime type. When it's time for you to upgrade, you only have to change the version number to get access to our updated endpoints. You can also choose to always work with the latest major version release by accepting application/json. We recommend the former. Example: application/vnd.payment-v`1`+json. ## Release history - 2022-05-08: v1 - Exposed channel manager API - 2021-07-01: v1 - Initial release # Booking Engine API Welcome to the Booking Engine API - A programmer-friendly way to search for bespoke travel inventory and create bookings for you or your customers. We take great care in only working with properties that have quality, curated content and ways to bundle and cross sell customers with ancillary products and experiences. Suppliers have the ability to make their inventory look great and be searchable in a wide variety of ways. You can work with suppliers directly or receive payment-level pricing available to all our integration partners. # Intended Audience Programmers are a requirement to start integrating with wink. You will benefit from an API integration if you are new or existing travel related company that want easy access to great inventory. Examples: - Hotel brands / chains that want to make their own booking engine - Travel tech companies that want to create the next hot mobile travel app - Destination sites that want to make their own booking engine - OTAs that want access direct relationships with suppliers and better quality hotel inventory
*
* The version of the OpenAPI document: 20.3.2-SNAPSHOT
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package travel.wink.sdk.booking.engine.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.util.ArrayList;
import java.util.List;
import travel.wink.sdk.booking.engine.model.ActivityHotelInventory;
import travel.wink.sdk.booking.engine.model.AggregateGreendexAnswers;
import travel.wink.sdk.booking.engine.model.Announcement;
import travel.wink.sdk.booking.engine.model.AttractionHotelInventory;
import travel.wink.sdk.booking.engine.model.HotelOnMap;
import travel.wink.sdk.booking.engine.model.MeetingRoomHotelInventory;
import travel.wink.sdk.booking.engine.model.MetaData;
import travel.wink.sdk.booking.engine.model.Multimedia;
import travel.wink.sdk.booking.engine.model.PlaceHotelInventory;
import travel.wink.sdk.booking.engine.model.Recognition;
import travel.wink.sdk.booking.engine.model.RestaurantHotelInventory;
import travel.wink.sdk.booking.engine.model.Review;
import travel.wink.sdk.booking.engine.model.RoomTypeWithPriceConfiguration;
import travel.wink.sdk.booking.engine.model.RoomTypeWithPriceConfigurations;
import travel.wink.sdk.booking.engine.model.SpaHotelInventory;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import javax.validation.constraints.*;
import javax.validation.Valid;
import org.hibernate.validator.constraints.*;
/**
* HotelInventoryResponse
*/
@JsonPropertyOrder({
HotelInventoryResponse.JSON_PROPERTY_HOTEL_IDENTIFIER,
HotelInventoryResponse.JSON_PROPERTY_URL_NAME,
HotelInventoryResponse.JSON_PROPERTY_HOTEL,
HotelInventoryResponse.JSON_PROPERTY_GREEN_INDEX_SCORES,
HotelInventoryResponse.JSON_PROPERTY_ROOM_TYPES,
HotelInventoryResponse.JSON_PROPERTY_MEETING_ROOMS,
HotelInventoryResponse.JSON_PROPERTY_RESTAURANTS,
HotelInventoryResponse.JSON_PROPERTY_SPAS,
HotelInventoryResponse.JSON_PROPERTY_ACTIVITIES,
HotelInventoryResponse.JSON_PROPERTY_ATTRACTIONS,
HotelInventoryResponse.JSON_PROPERTY_PLACES,
HotelInventoryResponse.JSON_PROPERTY_METADATA,
HotelInventoryResponse.JSON_PROPERTY_IMAGES,
HotelInventoryResponse.JSON_PROPERTY_VIDEOS,
HotelInventoryResponse.JSON_PROPERTY_RECOGNITIONS,
HotelInventoryResponse.JSON_PROPERTY_ANNOUNCEMENTS,
HotelInventoryResponse.JSON_PROPERTY_REVIEWS,
HotelInventoryResponse.JSON_PROPERTY_CHANNEL_DISCOUNT_PERCENT,
HotelInventoryResponse.JSON_PROPERTY_AVAILABLE,
HotelInventoryResponse.JSON_PROPERTY_CHEAPEST_ROOM_TYPES,
HotelInventoryResponse.JSON_PROPERTY_BEST_PRICE,
HotelInventoryResponse.JSON_PROPERTY_MULTIMEDIAS
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2022-06-29T13:20:36.800596+07:00[Asia/Bangkok]")
public class HotelInventoryResponse {
public static final String JSON_PROPERTY_HOTEL_IDENTIFIER = "hotelIdentifier";
private String hotelIdentifier;
public static final String JSON_PROPERTY_URL_NAME = "urlName";
private String urlName;
public static final String JSON_PROPERTY_HOTEL = "hotel";
private HotelOnMap hotel;
public static final String JSON_PROPERTY_GREEN_INDEX_SCORES = "greenIndexScores";
private AggregateGreendexAnswers greenIndexScores;
public static final String JSON_PROPERTY_ROOM_TYPES = "roomTypes";
private List roomTypes = null;
public static final String JSON_PROPERTY_MEETING_ROOMS = "meetingRooms";
private List meetingRooms = null;
public static final String JSON_PROPERTY_RESTAURANTS = "restaurants";
private List restaurants = null;
public static final String JSON_PROPERTY_SPAS = "spas";
private List spas = null;
public static final String JSON_PROPERTY_ACTIVITIES = "activities";
private List activities = null;
public static final String JSON_PROPERTY_ATTRACTIONS = "attractions";
private List attractions = null;
public static final String JSON_PROPERTY_PLACES = "places";
private List places = null;
public static final String JSON_PROPERTY_METADATA = "metadata";
private List metadata = null;
public static final String JSON_PROPERTY_IMAGES = "images";
private List images = null;
public static final String JSON_PROPERTY_VIDEOS = "videos";
private List videos = null;
public static final String JSON_PROPERTY_RECOGNITIONS = "recognitions";
private List recognitions = null;
public static final String JSON_PROPERTY_ANNOUNCEMENTS = "announcements";
private List announcements = null;
public static final String JSON_PROPERTY_REVIEWS = "reviews";
private List reviews = null;
public static final String JSON_PROPERTY_CHANNEL_DISCOUNT_PERCENT = "channelDiscountPercent";
private Float channelDiscountPercent;
public static final String JSON_PROPERTY_AVAILABLE = "available";
private Boolean available;
public static final String JSON_PROPERTY_CHEAPEST_ROOM_TYPES = "cheapestRoomTypes";
private List cheapestRoomTypes = null;
public static final String JSON_PROPERTY_BEST_PRICE = "bestPrice";
private RoomTypeWithPriceConfiguration bestPrice;
public static final String JSON_PROPERTY_MULTIMEDIAS = "multimedias";
private List multimedias = null;
public HotelInventoryResponse() {
}
public HotelInventoryResponse hotelIdentifier(String hotelIdentifier) {
this.hotelIdentifier = hotelIdentifier;
return this;
}
/**
* Unique property identifier to retrieve inventory for.
* @return hotelIdentifier
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "hotel-1", value = "Unique property identifier to retrieve inventory for.")
@JsonProperty(JSON_PROPERTY_HOTEL_IDENTIFIER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getHotelIdentifier() {
return hotelIdentifier;
}
@JsonProperty(JSON_PROPERTY_HOTEL_IDENTIFIER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setHotelIdentifier(String hotelIdentifier) {
this.hotelIdentifier = hotelIdentifier;
}
public HotelInventoryResponse urlName(String urlName) {
this.urlName = urlName;
return this;
}
/**
* Unique url-friendly record identifier of property.
* @return urlName
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "the-most-fantastic-hotel", value = "Unique url-friendly record identifier of property.")
@JsonProperty(JSON_PROPERTY_URL_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getUrlName() {
return urlName;
}
@JsonProperty(JSON_PROPERTY_URL_NAME)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setUrlName(String urlName) {
this.urlName = urlName;
}
public HotelInventoryResponse hotel(HotelOnMap hotel) {
this.hotel = hotel;
return this;
}
/**
* Get hotel
* @return hotel
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_HOTEL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public HotelOnMap getHotel() {
return hotel;
}
@JsonProperty(JSON_PROPERTY_HOTEL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setHotel(HotelOnMap hotel) {
this.hotel = hotel;
}
public HotelInventoryResponse greenIndexScores(AggregateGreendexAnswers greenIndexScores) {
this.greenIndexScores = greenIndexScores;
return this;
}
/**
* Get greenIndexScores
* @return greenIndexScores
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_GREEN_INDEX_SCORES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public AggregateGreendexAnswers getGreenIndexScores() {
return greenIndexScores;
}
@JsonProperty(JSON_PROPERTY_GREEN_INDEX_SCORES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setGreenIndexScores(AggregateGreendexAnswers greenIndexScores) {
this.greenIndexScores = greenIndexScores;
}
public HotelInventoryResponse roomTypes(List roomTypes) {
this.roomTypes = roomTypes;
return this;
}
public HotelInventoryResponse addRoomTypesItem(RoomTypeWithPriceConfigurations roomTypesItem) {
if (this.roomTypes == null) {
this.roomTypes = new ArrayList<>();
}
this.roomTypes.add(roomTypesItem);
return this;
}
/**
* List of room types with price configurations based on the itinerary that was passed on the user session.
* @return roomTypes
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "List of room types with price configurations based on the itinerary that was passed on the user session.")
@JsonProperty(JSON_PROPERTY_ROOM_TYPES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getRoomTypes() {
return roomTypes;
}
@JsonProperty(JSON_PROPERTY_ROOM_TYPES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRoomTypes(List roomTypes) {
this.roomTypes = roomTypes;
}
public HotelInventoryResponse meetingRooms(List meetingRooms) {
this.meetingRooms = meetingRooms;
return this;
}
public HotelInventoryResponse addMeetingRoomsItem(MeetingRoomHotelInventory meetingRoomsItem) {
if (this.meetingRooms == null) {
this.meetingRooms = new ArrayList<>();
}
this.meetingRooms.add(meetingRoomsItem);
return this;
}
/**
* List of property meeting rooms on and off the premises.
* @return meetingRooms
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "List of property meeting rooms on and off the premises.")
@JsonProperty(JSON_PROPERTY_MEETING_ROOMS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getMeetingRooms() {
return meetingRooms;
}
@JsonProperty(JSON_PROPERTY_MEETING_ROOMS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMeetingRooms(List meetingRooms) {
this.meetingRooms = meetingRooms;
}
public HotelInventoryResponse restaurants(List restaurants) {
this.restaurants = restaurants;
return this;
}
public HotelInventoryResponse addRestaurantsItem(RestaurantHotelInventory restaurantsItem) {
if (this.restaurants == null) {
this.restaurants = new ArrayList<>();
}
this.restaurants.add(restaurantsItem);
return this;
}
/**
* List of property restaurants on and off the premises.
* @return restaurants
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "List of property restaurants on and off the premises.")
@JsonProperty(JSON_PROPERTY_RESTAURANTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getRestaurants() {
return restaurants;
}
@JsonProperty(JSON_PROPERTY_RESTAURANTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRestaurants(List restaurants) {
this.restaurants = restaurants;
}
public HotelInventoryResponse spas(List spas) {
this.spas = spas;
return this;
}
public HotelInventoryResponse addSpasItem(SpaHotelInventory spasItem) {
if (this.spas == null) {
this.spas = new ArrayList<>();
}
this.spas.add(spasItem);
return this;
}
/**
* List of property spas on and off the premises.
* @return spas
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "List of property spas on and off the premises.")
@JsonProperty(JSON_PROPERTY_SPAS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getSpas() {
return spas;
}
@JsonProperty(JSON_PROPERTY_SPAS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSpas(List spas) {
this.spas = spas;
}
public HotelInventoryResponse activities(List activities) {
this.activities = activities;
return this;
}
public HotelInventoryResponse addActivitiesItem(ActivityHotelInventory activitiesItem) {
if (this.activities == null) {
this.activities = new ArrayList<>();
}
this.activities.add(activitiesItem);
return this;
}
/**
* List of property activities on and off the premises.
* @return activities
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "List of property activities on and off the premises.")
@JsonProperty(JSON_PROPERTY_ACTIVITIES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getActivities() {
return activities;
}
@JsonProperty(JSON_PROPERTY_ACTIVITIES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setActivities(List activities) {
this.activities = activities;
}
public HotelInventoryResponse attractions(List attractions) {
this.attractions = attractions;
return this;
}
public HotelInventoryResponse addAttractionsItem(AttractionHotelInventory attractionsItem) {
if (this.attractions == null) {
this.attractions = new ArrayList<>();
}
this.attractions.add(attractionsItem);
return this;
}
/**
* List of property attractions on and off the premises.
* @return attractions
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "List of property attractions on and off the premises.")
@JsonProperty(JSON_PROPERTY_ATTRACTIONS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getAttractions() {
return attractions;
}
@JsonProperty(JSON_PROPERTY_ATTRACTIONS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAttractions(List attractions) {
this.attractions = attractions;
}
public HotelInventoryResponse places(List places) {
this.places = places;
return this;
}
public HotelInventoryResponse addPlacesItem(PlaceHotelInventory placesItem) {
if (this.places == null) {
this.places = new ArrayList<>();
}
this.places.add(placesItem);
return this;
}
/**
* List of property places on and off the premises.
* @return places
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "List of property places on and off the premises.")
@JsonProperty(JSON_PROPERTY_PLACES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getPlaces() {
return places;
}
@JsonProperty(JSON_PROPERTY_PLACES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPlaces(List places) {
this.places = places;
}
public HotelInventoryResponse metadata(List metadata) {
this.metadata = metadata;
return this;
}
public HotelInventoryResponse addMetadataItem(MetaData metadataItem) {
if (this.metadata == null) {
this.metadata = new ArrayList<>();
}
this.metadata.add(metadataItem);
return this;
}
/**
* List of property meta data.
* @return metadata
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "List of property meta data.")
@JsonProperty(JSON_PROPERTY_METADATA)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getMetadata() {
return metadata;
}
@JsonProperty(JSON_PROPERTY_METADATA)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMetadata(List metadata) {
this.metadata = metadata;
}
public HotelInventoryResponse images(List images) {
this.images = images;
return this;
}
public HotelInventoryResponse addImagesItem(Multimedia imagesItem) {
if (this.images == null) {
this.images = new ArrayList<>();
}
this.images.add(imagesItem);
return this;
}
/**
* List of property images
* @return images
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "List of property images")
@JsonProperty(JSON_PROPERTY_IMAGES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getImages() {
return images;
}
@JsonProperty(JSON_PROPERTY_IMAGES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setImages(List images) {
this.images = images;
}
public HotelInventoryResponse videos(List videos) {
this.videos = videos;
return this;
}
public HotelInventoryResponse addVideosItem(Multimedia videosItem) {
if (this.videos == null) {
this.videos = new ArrayList<>();
}
this.videos.add(videosItem);
return this;
}
/**
* List of property videos
* @return videos
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "List of property videos")
@JsonProperty(JSON_PROPERTY_VIDEOS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getVideos() {
return videos;
}
@JsonProperty(JSON_PROPERTY_VIDEOS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setVideos(List videos) {
this.videos = videos;
}
public HotelInventoryResponse recognitions(List recognitions) {
this.recognitions = recognitions;
return this;
}
public HotelInventoryResponse addRecognitionsItem(Recognition recognitionsItem) {
if (this.recognitions == null) {
this.recognitions = new ArrayList<>();
}
this.recognitions.add(recognitionsItem);
return this;
}
/**
* List of property recognitions
* @return recognitions
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "List of property recognitions")
@JsonProperty(JSON_PROPERTY_RECOGNITIONS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getRecognitions() {
return recognitions;
}
@JsonProperty(JSON_PROPERTY_RECOGNITIONS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRecognitions(List recognitions) {
this.recognitions = recognitions;
}
public HotelInventoryResponse announcements(List announcements) {
this.announcements = announcements;
return this;
}
public HotelInventoryResponse addAnnouncementsItem(Announcement announcementsItem) {
if (this.announcements == null) {
this.announcements = new ArrayList<>();
}
this.announcements.add(announcementsItem);
return this;
}
/**
* List of property announcements
* @return announcements
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "List of property announcements")
@JsonProperty(JSON_PROPERTY_ANNOUNCEMENTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getAnnouncements() {
return announcements;
}
@JsonProperty(JSON_PROPERTY_ANNOUNCEMENTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAnnouncements(List announcements) {
this.announcements = announcements;
}
public HotelInventoryResponse reviews(List reviews) {
this.reviews = reviews;
return this;
}
public HotelInventoryResponse addReviewsItem(Review reviewsItem) {
if (this.reviews == null) {
this.reviews = new ArrayList<>();
}
this.reviews.add(reviewsItem);
return this;
}
/**
* List of property reviews
* @return reviews
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "List of property reviews")
@JsonProperty(JSON_PROPERTY_REVIEWS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getReviews() {
return reviews;
}
@JsonProperty(JSON_PROPERTY_REVIEWS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setReviews(List reviews) {
this.reviews = reviews;
}
public HotelInventoryResponse channelDiscountPercent(Float channelDiscountPercent) {
this.channelDiscountPercent = channelDiscountPercent;
return this;
}
/**
* A percent discount the property gave the caller. Value between 0.0 - 1.0.
* @return channelDiscountPercent
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "0.2", value = "A percent discount the property gave the caller. Value between 0.0 - 1.0.")
@JsonProperty(JSON_PROPERTY_CHANNEL_DISCOUNT_PERCENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Float getChannelDiscountPercent() {
return channelDiscountPercent;
}
@JsonProperty(JSON_PROPERTY_CHANNEL_DISCOUNT_PERCENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setChannelDiscountPercent(Float channelDiscountPercent) {
this.channelDiscountPercent = channelDiscountPercent;
}
public HotelInventoryResponse available(Boolean available) {
this.available = available;
return this;
}
/**
* Get available
* @return available
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_AVAILABLE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getAvailable() {
return available;
}
@JsonProperty(JSON_PROPERTY_AVAILABLE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAvailable(Boolean available) {
this.available = available;
}
public HotelInventoryResponse cheapestRoomTypes(List cheapestRoomTypes) {
this.cheapestRoomTypes = cheapestRoomTypes;
return this;
}
public HotelInventoryResponse addCheapestRoomTypesItem(RoomTypeWithPriceConfiguration cheapestRoomTypesItem) {
if (this.cheapestRoomTypes == null) {
this.cheapestRoomTypes = new ArrayList<>();
}
this.cheapestRoomTypes.add(cheapestRoomTypesItem);
return this;
}
/**
* Get cheapestRoomTypes
* @return cheapestRoomTypes
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_CHEAPEST_ROOM_TYPES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getCheapestRoomTypes() {
return cheapestRoomTypes;
}
@JsonProperty(JSON_PROPERTY_CHEAPEST_ROOM_TYPES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCheapestRoomTypes(List cheapestRoomTypes) {
this.cheapestRoomTypes = cheapestRoomTypes;
}
public HotelInventoryResponse bestPrice(RoomTypeWithPriceConfiguration bestPrice) {
this.bestPrice = bestPrice;
return this;
}
/**
* Get bestPrice
* @return bestPrice
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_BEST_PRICE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public RoomTypeWithPriceConfiguration getBestPrice() {
return bestPrice;
}
@JsonProperty(JSON_PROPERTY_BEST_PRICE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setBestPrice(RoomTypeWithPriceConfiguration bestPrice) {
this.bestPrice = bestPrice;
}
public HotelInventoryResponse multimedias(List multimedias) {
this.multimedias = multimedias;
return this;
}
public HotelInventoryResponse addMultimediasItem(Multimedia multimediasItem) {
if (this.multimedias == null) {
this.multimedias = new ArrayList<>();
}
this.multimedias.add(multimediasItem);
return this;
}
/**
* Get multimedias
* @return multimedias
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_MULTIMEDIAS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getMultimedias() {
return multimedias;
}
@JsonProperty(JSON_PROPERTY_MULTIMEDIAS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMultimedias(List multimedias) {
this.multimedias = multimedias;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
HotelInventoryResponse hotelInventoryResponse = (HotelInventoryResponse) o;
return Objects.equals(this.hotelIdentifier, hotelInventoryResponse.hotelIdentifier) &&
Objects.equals(this.urlName, hotelInventoryResponse.urlName) &&
Objects.equals(this.hotel, hotelInventoryResponse.hotel) &&
Objects.equals(this.greenIndexScores, hotelInventoryResponse.greenIndexScores) &&
Objects.equals(this.roomTypes, hotelInventoryResponse.roomTypes) &&
Objects.equals(this.meetingRooms, hotelInventoryResponse.meetingRooms) &&
Objects.equals(this.restaurants, hotelInventoryResponse.restaurants) &&
Objects.equals(this.spas, hotelInventoryResponse.spas) &&
Objects.equals(this.activities, hotelInventoryResponse.activities) &&
Objects.equals(this.attractions, hotelInventoryResponse.attractions) &&
Objects.equals(this.places, hotelInventoryResponse.places) &&
Objects.equals(this.metadata, hotelInventoryResponse.metadata) &&
Objects.equals(this.images, hotelInventoryResponse.images) &&
Objects.equals(this.videos, hotelInventoryResponse.videos) &&
Objects.equals(this.recognitions, hotelInventoryResponse.recognitions) &&
Objects.equals(this.announcements, hotelInventoryResponse.announcements) &&
Objects.equals(this.reviews, hotelInventoryResponse.reviews) &&
Objects.equals(this.channelDiscountPercent, hotelInventoryResponse.channelDiscountPercent) &&
Objects.equals(this.available, hotelInventoryResponse.available) &&
Objects.equals(this.cheapestRoomTypes, hotelInventoryResponse.cheapestRoomTypes) &&
Objects.equals(this.bestPrice, hotelInventoryResponse.bestPrice) &&
Objects.equals(this.multimedias, hotelInventoryResponse.multimedias);
}
@Override
public int hashCode() {
return Objects.hash(hotelIdentifier, urlName, hotel, greenIndexScores, roomTypes, meetingRooms, restaurants, spas, activities, attractions, places, metadata, images, videos, recognitions, announcements, reviews, channelDiscountPercent, available, cheapestRoomTypes, bestPrice, multimedias);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class HotelInventoryResponse {\n");
sb.append(" hotelIdentifier: ").append(toIndentedString(hotelIdentifier)).append("\n");
sb.append(" urlName: ").append(toIndentedString(urlName)).append("\n");
sb.append(" hotel: ").append(toIndentedString(hotel)).append("\n");
sb.append(" greenIndexScores: ").append(toIndentedString(greenIndexScores)).append("\n");
sb.append(" roomTypes: ").append(toIndentedString(roomTypes)).append("\n");
sb.append(" meetingRooms: ").append(toIndentedString(meetingRooms)).append("\n");
sb.append(" restaurants: ").append(toIndentedString(restaurants)).append("\n");
sb.append(" spas: ").append(toIndentedString(spas)).append("\n");
sb.append(" activities: ").append(toIndentedString(activities)).append("\n");
sb.append(" attractions: ").append(toIndentedString(attractions)).append("\n");
sb.append(" places: ").append(toIndentedString(places)).append("\n");
sb.append(" metadata: ").append(toIndentedString(metadata)).append("\n");
sb.append(" images: ").append(toIndentedString(images)).append("\n");
sb.append(" videos: ").append(toIndentedString(videos)).append("\n");
sb.append(" recognitions: ").append(toIndentedString(recognitions)).append("\n");
sb.append(" announcements: ").append(toIndentedString(announcements)).append("\n");
sb.append(" reviews: ").append(toIndentedString(reviews)).append("\n");
sb.append(" channelDiscountPercent: ").append(toIndentedString(channelDiscountPercent)).append("\n");
sb.append(" available: ").append(toIndentedString(available)).append("\n");
sb.append(" cheapestRoomTypes: ").append(toIndentedString(cheapestRoomTypes)).append("\n");
sb.append(" bestPrice: ").append(toIndentedString(bestPrice)).append("\n");
sb.append(" multimedias: ").append(toIndentedString(multimedias)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy