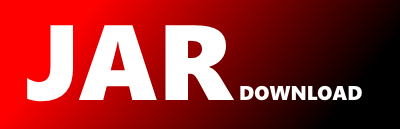
travel.wink.sdk.booking.engine.model.Recreation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of booking-engine-sdk-java Show documentation
Show all versions of booking-engine-sdk-java Show documentation
Java SDK for the wink Booking Engine API
/*
* Wink API
* ## APIs Not every integrator needs every APIs. For that reason, we have separated APIs into context. - [Affiliate](/docs?api=affiliate): All APIs related to selling travel inventory as an affiliate. - [Extranet](/docs?api=extranet): All APIs related to managing travel inventory and suppliers. - [Booking Engine](/docs?api=booking-engine): All APIs related to searching for travel inventory and creating bookings. - [Channel Manager](https://integration.wink.travel/docs?api=channel-manager): All APIs related to channel managers who want to integrate with our payment. ## SDKs We are actively working on supporting the most used languages out there. If you don't see your language here, reach out to us with a request to officially add your language. In the meantime, if you want to roll your own SDK, you can do so by downloading the OpenAPI spec and using one of the many available OpenAPI generators available: [https://openapi-generator.tech/docs/generators](https://openapi-generator.tech/docs/generators). - Java SDK [https://github.com/wink-travel/wink-sdk-java](https://github.com/wink-travel/wink-sdk-java) ## Usage These features are made available to you via a [REST API](https://en.wikipedia.org/wiki/Representational_state_transfer). This API is language agnostic. ## Versioning We chose to version our endpoints in a way that we hope affects your integration with us the least. You request the version of our API you wish to work with via the `Accept` header and by using our custom JSON mime type. When it's time for you to upgrade, you only have to change the version number to get access to our updated endpoints. You can also choose to always work with the latest major version release by accepting application/json. We recommend the former. Example: application/vnd.payment-v`1`+json. ## Release history - 2022-05-08: v1 - Exposed channel manager API - 2021-07-01: v1 - Initial release # Booking Engine API Welcome to the Booking Engine API - A programmer-friendly way to search for bespoke travel inventory and create bookings for you or your customers. We take great care in only working with properties that have quality, curated content and ways to bundle and cross sell customers with ancillary products and experiences. Suppliers have the ability to make their inventory look great and be searchable in a wide variety of ways. You can work with suppliers directly or receive payment-level pricing available to all our integration partners. # Intended Audience Programmers are a requirement to start integrating with wink. You will benefit from an API integration if you are new or existing travel related company that want easy access to great inventory. Examples: - Hotel brands / chains that want to make their own booking engine - Travel tech companies that want to create the next hot mobile travel app - Destination sites that want to make their own booking engine - OTAs that want access direct relationships with suppliers and better quality hotel inventory
*
* The version of the OpenAPI document: 20.3.2-SNAPSHOT
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package travel.wink.sdk.booking.engine.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.List;
import travel.wink.sdk.booking.engine.model.Address;
import travel.wink.sdk.booking.engine.model.Contact;
import travel.wink.sdk.booking.engine.model.DowPatternGroup;
import travel.wink.sdk.booking.engine.model.GeoJsonPoint;
import travel.wink.sdk.booking.engine.model.Moneys;
import travel.wink.sdk.booking.engine.model.Multimedia;
import travel.wink.sdk.booking.engine.model.SimpleDescription;
import travel.wink.sdk.booking.engine.model.Social;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import javax.validation.constraints.*;
import javax.validation.Valid;
import org.hibernate.validator.constraints.*;
/**
* Inventory data object
*/
@ApiModel(description = "Inventory data object")
@JsonPropertyOrder({
Recreation.JSON_PROPERTY_IDENTIFIER,
Recreation.JSON_PROPERTY_HOTEL_IDENTIFIER,
Recreation.JSON_PROPERTY_FEATURED_IND,
Recreation.JSON_PROPERTY_LIFESTYLE_TYPE,
Recreation.JSON_PROPERTY_LOCATION,
Recreation.JSON_PROPERTY_DESCRIPTIONS,
Recreation.JSON_PROPERTY_MULTIMEDIAS,
Recreation.JSON_PROPERTY_CONTACT,
Recreation.JSON_PROPERTY_ADDRESS,
Recreation.JSON_PROPERTY_COMMISSIONABLE,
Recreation.JSON_PROPERTY_NAME,
Recreation.JSON_PROPERTY_PROXIMITY_CODE,
Recreation.JSON_PROPERTY_SORT,
Recreation.JSON_PROPERTY_MIN_AGE_APPROPRIATE_CODE,
Recreation.JSON_PROPERTY_BOOKABLE,
Recreation.JSON_PROPERTY_ACTIVE,
Recreation.JSON_PROPERTY_DISABILITY_FEATURES,
Recreation.JSON_PROPERTY_SECURITY_FEATURES,
Recreation.JSON_PROPERTY_SOCIALS,
Recreation.JSON_PROPERTY_APPLICABLE_START,
Recreation.JSON_PROPERTY_APPLICABLE_END,
Recreation.JSON_PROPERTY_RESERVATION_REQUIRED_IND,
Recreation.JSON_PROPERTY_OPENS,
Recreation.JSON_PROPERTY_CLOSES,
Recreation.JSON_PROPERTY_FEE,
Recreation.JSON_PROPERTY_PERCENT_DISCOUNT,
Recreation.JSON_PROPERTY_DAYS_OF_WEEK,
Recreation.JSON_PROPERTY_RECREATION_CATEGORY_CODE,
Recreation.JSON_PROPERTY_AMENITIES,
Recreation.JSON_PROPERTY_PRICING_TYPE
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2022-06-29T13:20:36.800596+07:00[Asia/Bangkok]")
public class Recreation {
public static final String JSON_PROPERTY_IDENTIFIER = "identifier";
private String identifier;
public static final String JSON_PROPERTY_HOTEL_IDENTIFIER = "hotelIdentifier";
private String hotelIdentifier;
public static final String JSON_PROPERTY_FEATURED_IND = "featuredInd";
private Boolean featuredInd;
/**
* Indicate the type of lifestyle this inventory should be associated with.
*/
public enum LifestyleTypeEnum {
HEALTH_FITNESS("LIFESTYLE_HEALTH_FITNESS"),
RELAX("LIFESTYLE_RELAX"),
ADULT_ONLY("LIFESTYLE_ADULT_ONLY"),
ADVENTURE("LIFESTYLE_ADVENTURE"),
BUSINESS("LIFESTYLE_BUSINESS"),
LGBT("LIFESTYLE_LGBT"),
SINGLE_PARENT("LIFESTYLE_SINGLE_PARENT"),
SOLO_FEMALE("LIFESTYLE_SOLO_FEMALE"),
BEAUTY("LIFESTYLE_BEAUTY"),
FOODIE("LIFESTYLE_FOODIE"),
FAMILY("LIFESTYLE_FAMILY"),
ROMANCE("LIFESTYLE_ROMANCE"),
COUPLE("LIFESTYLE_COUPLE"),
SOLO("LIFESTYLE_SOLO"),
BACKPACKER("LIFESTYLE_BACKPACKER"),
SHOPPING("LIFESTYLE_SHOPPING"),
SPORTS("LIFESTYLE_SPORTS"),
MOUNTAIN("LIFESTYLE_MOUNTAIN"),
BEACH("LIFESTYLE_BEACH"),
CITY("LIFESTYLE_CITY"),
COUNTRY("LIFESTYLE_COUNTRY"),
CULTURE("LIFESTYLE_CULTURE"),
ECO("LIFESTYLE_ECO");
private String value;
LifestyleTypeEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static LifestyleTypeEnum fromValue(String value) {
for (LifestyleTypeEnum b : LifestyleTypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
}
public static final String JSON_PROPERTY_LIFESTYLE_TYPE = "lifestyleType";
private LifestyleTypeEnum lifestyleType;
public static final String JSON_PROPERTY_LOCATION = "location";
private GeoJsonPoint location;
public static final String JSON_PROPERTY_DESCRIPTIONS = "descriptions";
private List descriptions = new ArrayList<>();
public static final String JSON_PROPERTY_MULTIMEDIAS = "multimedias";
private List multimedias = new ArrayList<>();
public static final String JSON_PROPERTY_CONTACT = "contact";
private Contact contact;
public static final String JSON_PROPERTY_ADDRESS = "address";
private Address address;
public static final String JSON_PROPERTY_COMMISSIONABLE = "commissionable";
private Boolean commissionable = true;
public static final String JSON_PROPERTY_NAME = "name";
private String name;
public static final String JSON_PROPERTY_PROXIMITY_CODE = "proximityCode";
private String proximityCode;
public static final String JSON_PROPERTY_SORT = "sort";
private Integer sort;
public static final String JSON_PROPERTY_MIN_AGE_APPROPRIATE_CODE = "minAgeAppropriateCode";
private String minAgeAppropriateCode;
public static final String JSON_PROPERTY_BOOKABLE = "bookable";
private Boolean bookable = true;
public static final String JSON_PROPERTY_ACTIVE = "active";
private Boolean active = true;
public static final String JSON_PROPERTY_DISABILITY_FEATURES = "disabilityFeatures";
private List disabilityFeatures = null;
public static final String JSON_PROPERTY_SECURITY_FEATURES = "securityFeatures";
private List securityFeatures = null;
public static final String JSON_PROPERTY_SOCIALS = "socials";
private List socials = null;
public static final String JSON_PROPERTY_APPLICABLE_START = "applicableStart";
private LocalDate applicableStart;
public static final String JSON_PROPERTY_APPLICABLE_END = "applicableEnd";
private LocalDate applicableEnd;
public static final String JSON_PROPERTY_RESERVATION_REQUIRED_IND = "reservationRequiredInd";
private Boolean reservationRequiredInd;
public static final String JSON_PROPERTY_OPENS = "opens";
private String opens;
public static final String JSON_PROPERTY_CLOSES = "closes";
private String closes;
public static final String JSON_PROPERTY_FEE = "fee";
private Moneys fee;
public static final String JSON_PROPERTY_PERCENT_DISCOUNT = "percentDiscount";
private Double percentDiscount;
public static final String JSON_PROPERTY_DAYS_OF_WEEK = "daysOfWeek";
private DowPatternGroup daysOfWeek;
public static final String JSON_PROPERTY_RECREATION_CATEGORY_CODE = "recreationCategoryCode";
private String recreationCategoryCode;
public static final String JSON_PROPERTY_AMENITIES = "amenities";
private List amenities = null;
/**
* How this inventory item should be priced.
*/
public enum PricingTypeEnum {
PERSON_PER_USE("PER_PERSON_PER_USE"),
USE("PER_USE"),
PERSON_PER_HOUR("PER_PERSON_PER_HOUR"),
HOUR("PER_HOUR");
private String value;
PricingTypeEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static PricingTypeEnum fromValue(String value) {
for (PricingTypeEnum b : PricingTypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + value + "'");
}
}
public static final String JSON_PROPERTY_PRICING_TYPE = "pricingType";
private PricingTypeEnum pricingType;
public Recreation() {
}
public Recreation identifier(String identifier) {
this.identifier = identifier;
return this;
}
/**
* Unique record identifier
* @return identifier
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "document-1", value = "Unique record identifier")
@JsonProperty(JSON_PROPERTY_IDENTIFIER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getIdentifier() {
return identifier;
}
@JsonProperty(JSON_PROPERTY_IDENTIFIER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setIdentifier(String identifier) {
this.identifier = identifier;
}
public Recreation hotelIdentifier(String hotelIdentifier) {
this.hotelIdentifier = hotelIdentifier;
return this;
}
/**
* Hotel identifier.
* @return hotelIdentifier
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "hotel-1", value = "Hotel identifier.")
@JsonProperty(JSON_PROPERTY_HOTEL_IDENTIFIER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getHotelIdentifier() {
return hotelIdentifier;
}
@JsonProperty(JSON_PROPERTY_HOTEL_IDENTIFIER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setHotelIdentifier(String hotelIdentifier) {
this.hotelIdentifier = hotelIdentifier;
}
public Recreation featuredInd(Boolean featuredInd) {
this.featuredInd = featuredInd;
return this;
}
/**
* Indicates whether this inventory is featured. Use this flag as a way to signify that this inventory is special.
* @return featuredInd
**/
@javax.annotation.Nonnull
@NotNull
@ApiModelProperty(example = "false", required = true, value = "Indicates whether this inventory is featured. Use this flag as a way to signify that this inventory is special.")
@JsonProperty(JSON_PROPERTY_FEATURED_IND)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Boolean getFeaturedInd() {
return featuredInd;
}
@JsonProperty(JSON_PROPERTY_FEATURED_IND)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setFeaturedInd(Boolean featuredInd) {
this.featuredInd = featuredInd;
}
public Recreation lifestyleType(LifestyleTypeEnum lifestyleType) {
this.lifestyleType = lifestyleType;
return this;
}
/**
* Indicate the type of lifestyle this inventory should be associated with.
* @return lifestyleType
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "LIFESTYLE_HEALTH_FITNESS", value = "Indicate the type of lifestyle this inventory should be associated with.")
@JsonProperty(JSON_PROPERTY_LIFESTYLE_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public LifestyleTypeEnum getLifestyleType() {
return lifestyleType;
}
@JsonProperty(JSON_PROPERTY_LIFESTYLE_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setLifestyleType(LifestyleTypeEnum lifestyleType) {
this.lifestyleType = lifestyleType;
}
public Recreation location(GeoJsonPoint location) {
this.location = location;
return this;
}
/**
* Get location
* @return location
**/
@javax.annotation.Nonnull
@NotNull
@Valid
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_LOCATION)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public GeoJsonPoint getLocation() {
return location;
}
@JsonProperty(JSON_PROPERTY_LOCATION)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setLocation(GeoJsonPoint location) {
this.location = location;
}
public Recreation descriptions(List descriptions) {
this.descriptions = descriptions;
return this;
}
public Recreation addDescriptionsItem(SimpleDescription descriptionsItem) {
this.descriptions.add(descriptionsItem);
return this;
}
/**
* Localized descriptions describing inventory.
* @return descriptions
**/
@javax.annotation.Nonnull
@NotNull
@Valid
@ApiModelProperty(required = true, value = "Localized descriptions describing inventory.")
@JsonProperty(JSON_PROPERTY_DESCRIPTIONS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getDescriptions() {
return descriptions;
}
@JsonProperty(JSON_PROPERTY_DESCRIPTIONS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setDescriptions(List descriptions) {
this.descriptions = descriptions;
}
public Recreation multimedias(List multimedias) {
this.multimedias = multimedias;
return this;
}
public Recreation addMultimediasItem(Multimedia multimediasItem) {
this.multimedias.add(multimediasItem);
return this;
}
/**
* List of images / videos of inventory.
* @return multimedias
**/
@javax.annotation.Nonnull
@NotNull
@Valid
@ApiModelProperty(required = true, value = "List of images / videos of inventory.")
@JsonProperty(JSON_PROPERTY_MULTIMEDIAS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getMultimedias() {
return multimedias;
}
@JsonProperty(JSON_PROPERTY_MULTIMEDIAS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setMultimedias(List multimedias) {
this.multimedias = multimedias;
}
public Recreation contact(Contact contact) {
this.contact = contact;
return this;
}
/**
* Get contact
* @return contact
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_CONTACT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Contact getContact() {
return contact;
}
@JsonProperty(JSON_PROPERTY_CONTACT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setContact(Contact contact) {
this.contact = contact;
}
public Recreation address(Address address) {
this.address = address;
return this;
}
/**
* Get address
* @return address
**/
@javax.annotation.Nonnull
@NotNull
@Valid
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_ADDRESS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Address getAddress() {
return address;
}
@JsonProperty(JSON_PROPERTY_ADDRESS)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setAddress(Address address) {
this.address = address;
}
public Recreation commissionable(Boolean commissionable) {
this.commissionable = commissionable;
return this;
}
/**
* Indicate whether sales channels receive commission for selling this inventory.
* @return commissionable
**/
@javax.annotation.Nonnull
@NotNull
@ApiModelProperty(example = "true", required = true, value = "Indicate whether sales channels receive commission for selling this inventory.")
@JsonProperty(JSON_PROPERTY_COMMISSIONABLE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Boolean getCommissionable() {
return commissionable;
}
@JsonProperty(JSON_PROPERTY_COMMISSIONABLE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setCommissionable(Boolean commissionable) {
this.commissionable = commissionable;
}
public Recreation name(String name) {
this.name = name;
return this;
}
/**
* Internal name of inventory.
* @return name
**/
@javax.annotation.Nonnull
@NotNull
@ApiModelProperty(example = "Archery lesson", required = true, value = "Internal name of inventory.")
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getName() {
return name;
}
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setName(String name) {
this.name = name;
}
public Recreation proximityCode(String proximityCode) {
this.proximityCode = proximityCode;
return this;
}
/**
* Supported OTA specification `PRX` code. See [OTA geoname data](#operation/showAvailableCodesForCategory)
* @return proximityCode
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "1", value = "Supported OTA specification `PRX` code. See [OTA geoname data](#operation/showAvailableCodesForCategory)")
@JsonProperty(JSON_PROPERTY_PROXIMITY_CODE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getProximityCode() {
return proximityCode;
}
@JsonProperty(JSON_PROPERTY_PROXIMITY_CODE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setProximityCode(String proximityCode) {
this.proximityCode = proximityCode;
}
public Recreation sort(Integer sort) {
this.sort = sort;
return this;
}
/**
* Use this property to sort an inventory in a list of activities.
* @return sort
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "1", value = "Use this property to sort an inventory in a list of activities.")
@JsonProperty(JSON_PROPERTY_SORT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getSort() {
return sort;
}
@JsonProperty(JSON_PROPERTY_SORT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSort(Integer sort) {
this.sort = sort;
}
public Recreation minAgeAppropriateCode(String minAgeAppropriateCode) {
this.minAgeAppropriateCode = minAgeAppropriateCode;
return this;
}
/**
* Supported OTA specification `AQC` code. See [OTA geoname data](#operation/showAvailableCodesForCategory)
* @return minAgeAppropriateCode
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "1", value = "Supported OTA specification `AQC` code. See [OTA geoname data](#operation/showAvailableCodesForCategory)")
@JsonProperty(JSON_PROPERTY_MIN_AGE_APPROPRIATE_CODE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getMinAgeAppropriateCode() {
return minAgeAppropriateCode;
}
@JsonProperty(JSON_PROPERTY_MIN_AGE_APPROPRIATE_CODE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMinAgeAppropriateCode(String minAgeAppropriateCode) {
this.minAgeAppropriateCode = minAgeAppropriateCode;
}
public Recreation bookable(Boolean bookable) {
this.bookable = bookable;
return this;
}
/**
* Indicates if this inventory can be added to a booking or if it is read-only marketing material only.
* @return bookable
**/
@javax.annotation.Nonnull
@NotNull
@ApiModelProperty(example = "true", required = true, value = "Indicates if this inventory can be added to a booking or if it is read-only marketing material only.")
@JsonProperty(JSON_PROPERTY_BOOKABLE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Boolean getBookable() {
return bookable;
}
@JsonProperty(JSON_PROPERTY_BOOKABLE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setBookable(Boolean bookable) {
this.bookable = bookable;
}
public Recreation active(Boolean active) {
this.active = active;
return this;
}
/**
* Modify inventory availability with this flag.
* @return active
**/
@javax.annotation.Nonnull
@NotNull
@ApiModelProperty(example = "true", required = true, value = "Modify inventory availability with this flag.")
@JsonProperty(JSON_PROPERTY_ACTIVE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Boolean getActive() {
return active;
}
@JsonProperty(JSON_PROPERTY_ACTIVE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setActive(Boolean active) {
this.active = active;
}
public Recreation disabilityFeatures(List disabilityFeatures) {
this.disabilityFeatures = disabilityFeatures;
return this;
}
public Recreation addDisabilityFeaturesItem(String disabilityFeaturesItem) {
if (this.disabilityFeatures == null) {
this.disabilityFeatures = new ArrayList<>();
}
this.disabilityFeatures.add(disabilityFeaturesItem);
return this;
}
/**
* Supported OTA specification `PHY` code. See [OTA geoname data](#operation/showAvailableCodesForCategory)
* @return disabilityFeatures
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "[\"1\"]", value = "Supported OTA specification `PHY` code. See [OTA geoname data](#operation/showAvailableCodesForCategory)")
@JsonProperty(JSON_PROPERTY_DISABILITY_FEATURES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getDisabilityFeatures() {
return disabilityFeatures;
}
@JsonProperty(JSON_PROPERTY_DISABILITY_FEATURES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDisabilityFeatures(List disabilityFeatures) {
this.disabilityFeatures = disabilityFeatures;
}
public Recreation securityFeatures(List securityFeatures) {
this.securityFeatures = securityFeatures;
return this;
}
public Recreation addSecurityFeaturesItem(String securityFeaturesItem) {
if (this.securityFeatures == null) {
this.securityFeatures = new ArrayList<>();
}
this.securityFeatures.add(securityFeaturesItem);
return this;
}
/**
* Supported OTA specification `SEC` code. See [OTA geoname data](#operation/showAvailableCodesForCategory)
* @return securityFeatures
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "[\"1\"]", value = "Supported OTA specification `SEC` code. See [OTA geoname data](#operation/showAvailableCodesForCategory)")
@JsonProperty(JSON_PROPERTY_SECURITY_FEATURES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getSecurityFeatures() {
return securityFeatures;
}
@JsonProperty(JSON_PROPERTY_SECURITY_FEATURES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSecurityFeatures(List securityFeatures) {
this.securityFeatures = securityFeatures;
}
public Recreation socials(List socials) {
this.socials = socials;
return this;
}
public Recreation addSocialsItem(Social socialsItem) {
if (this.socials == null) {
this.socials = new ArrayList<>();
}
this.socials.add(socialsItem);
return this;
}
/**
* Social network accounts for inventory (if applicable).
* @return socials
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "Social network accounts for inventory (if applicable).")
@JsonProperty(JSON_PROPERTY_SOCIALS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getSocials() {
return socials;
}
@JsonProperty(JSON_PROPERTY_SOCIALS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSocials(List socials) {
this.socials = socials;
}
public Recreation applicableStart(LocalDate applicableStart) {
this.applicableStart = applicableStart;
return this;
}
/**
* Start month and day or date for which the attraction (e.g. the start of a season) is available. This date property signifies that the inventory is recurring and / or seasonal. If the date is in the past, only day and month will be used to infer seasonality. If the date is a future date, it will be interpreted as a starting date.
* @return applicableStart
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(example = "Thu Jan 01 07:00:00 ICT 1970", value = "Start month and day or date for which the attraction (e.g. the start of a season) is available. This date property signifies that the inventory is recurring and / or seasonal. If the date is in the past, only day and month will be used to infer seasonality. If the date is a future date, it will be interpreted as a starting date.")
@JsonProperty(JSON_PROPERTY_APPLICABLE_START)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public LocalDate getApplicableStart() {
return applicableStart;
}
@JsonProperty(JSON_PROPERTY_APPLICABLE_START)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setApplicableStart(LocalDate applicableStart) {
this.applicableStart = applicableStart;
}
public Recreation applicableEnd(LocalDate applicableEnd) {
this.applicableEnd = applicableEnd;
return this;
}
/**
* End month and day or date for which the attraction (e.g. the start of a season) is available. This date property signifies that the inventory is recurring and / or seasonal. If the date is in the past, only day and month will be used to infer seasonality. If the date is a future date, it will be interpreted as a ending date.
* @return applicableEnd
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(example = "Tue Dec 01 07:00:00 ICT 1970", value = "End month and day or date for which the attraction (e.g. the start of a season) is available. This date property signifies that the inventory is recurring and / or seasonal. If the date is in the past, only day and month will be used to infer seasonality. If the date is a future date, it will be interpreted as a ending date.")
@JsonProperty(JSON_PROPERTY_APPLICABLE_END)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public LocalDate getApplicableEnd() {
return applicableEnd;
}
@JsonProperty(JSON_PROPERTY_APPLICABLE_END)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setApplicableEnd(LocalDate applicableEnd) {
this.applicableEnd = applicableEnd;
}
public Recreation reservationRequiredInd(Boolean reservationRequiredInd) {
this.reservationRequiredInd = reservationRequiredInd;
return this;
}
/**
* Indicates whether a reservation is required to participate in this inventory.
* @return reservationRequiredInd
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "false", value = "Indicates whether a reservation is required to participate in this inventory.")
@JsonProperty(JSON_PROPERTY_RESERVATION_REQUIRED_IND)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getReservationRequiredInd() {
return reservationRequiredInd;
}
@JsonProperty(JSON_PROPERTY_RESERVATION_REQUIRED_IND)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setReservationRequiredInd(Boolean reservationRequiredInd) {
this.reservationRequiredInd = reservationRequiredInd;
}
public Recreation opens(String opens) {
this.opens = opens;
return this;
}
/**
* Opening time of inventory (if applicable). Leave empty if inventory is always available.
* @return opens
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "09:00", value = "Opening time of inventory (if applicable). Leave empty if inventory is always available.")
@JsonProperty(JSON_PROPERTY_OPENS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getOpens() {
return opens;
}
@JsonProperty(JSON_PROPERTY_OPENS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setOpens(String opens) {
this.opens = opens;
}
public Recreation closes(String closes) {
this.closes = closes;
return this;
}
/**
* Closing time of inventory (if applicable). Leave empty if inventory is always available.
* @return closes
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "17:30", value = "Closing time of inventory (if applicable). Leave empty if inventory is always available.")
@JsonProperty(JSON_PROPERTY_CLOSES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getCloses() {
return closes;
}
@JsonProperty(JSON_PROPERTY_CLOSES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCloses(String closes) {
this.closes = closes;
}
public Recreation fee(Moneys fee) {
this.fee = fee;
return this;
}
/**
* Get fee
* @return fee
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_FEE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Moneys getFee() {
return fee;
}
@JsonProperty(JSON_PROPERTY_FEE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setFee(Moneys fee) {
this.fee = fee;
}
public Recreation percentDiscount(Double percentDiscount) {
this.percentDiscount = percentDiscount;
return this;
}
/**
* Expected savings. Indicate how much the traveler is [usually] saving by booking it through this payment. Example: 20% discount.
* minimum: 0.0
* maximum: 1.0
* @return percentDiscount
**/
@javax.annotation.Nonnull
@NotNull
@DecimalMin("0.0") @DecimalMax("1.0") @ApiModelProperty(example = "0.2", required = true, value = "Expected savings. Indicate how much the traveler is [usually] saving by booking it through this payment. Example: 20% discount.")
@JsonProperty(JSON_PROPERTY_PERCENT_DISCOUNT)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Double getPercentDiscount() {
return percentDiscount;
}
@JsonProperty(JSON_PROPERTY_PERCENT_DISCOUNT)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setPercentDiscount(Double percentDiscount) {
this.percentDiscount = percentDiscount;
}
public Recreation daysOfWeek(DowPatternGroup daysOfWeek) {
this.daysOfWeek = daysOfWeek;
return this;
}
/**
* Get daysOfWeek
* @return daysOfWeek
**/
@javax.annotation.Nullable
@Valid
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_DAYS_OF_WEEK)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DowPatternGroup getDaysOfWeek() {
return daysOfWeek;
}
@JsonProperty(JSON_PROPERTY_DAYS_OF_WEEK)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDaysOfWeek(DowPatternGroup daysOfWeek) {
this.daysOfWeek = daysOfWeek;
}
public Recreation recreationCategoryCode(String recreationCategoryCode) {
this.recreationCategoryCode = recreationCategoryCode;
return this;
}
/**
* Supported OTA specification `RST` code. See [OTA geoname data](#operation/showAvailableCodesForCategory)
* @return recreationCategoryCode
**/
@javax.annotation.Nonnull
@NotNull
@ApiModelProperty(example = "1", required = true, value = "Supported OTA specification `RST` code. See [OTA geoname data](#operation/showAvailableCodesForCategory)")
@JsonProperty(JSON_PROPERTY_RECREATION_CATEGORY_CODE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getRecreationCategoryCode() {
return recreationCategoryCode;
}
@JsonProperty(JSON_PROPERTY_RECREATION_CATEGORY_CODE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setRecreationCategoryCode(String recreationCategoryCode) {
this.recreationCategoryCode = recreationCategoryCode;
}
public Recreation amenities(List amenities) {
this.amenities = amenities;
return this;
}
public Recreation addAmenitiesItem(String amenitiesItem) {
if (this.amenities == null) {
this.amenities = new ArrayList<>();
}
this.amenities.add(amenitiesItem);
return this;
}
/**
* Supported OTA specification `REC` code. See [OTA geoname data](#operation/showAvailableCodesForCategory).
* @return amenities
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "[\"1\"]", value = "Supported OTA specification `REC` code. See [OTA geoname data](#operation/showAvailableCodesForCategory).")
@JsonProperty(JSON_PROPERTY_AMENITIES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getAmenities() {
return amenities;
}
@JsonProperty(JSON_PROPERTY_AMENITIES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAmenities(List amenities) {
this.amenities = amenities;
}
public Recreation pricingType(PricingTypeEnum pricingType) {
this.pricingType = pricingType;
return this;
}
/**
* How this inventory item should be priced.
* @return pricingType
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "How this inventory item should be priced.")
@JsonProperty(JSON_PROPERTY_PRICING_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public PricingTypeEnum getPricingType() {
return pricingType;
}
@JsonProperty(JSON_PROPERTY_PRICING_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPricingType(PricingTypeEnum pricingType) {
this.pricingType = pricingType;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Recreation recreation = (Recreation) o;
return Objects.equals(this.identifier, recreation.identifier) &&
Objects.equals(this.hotelIdentifier, recreation.hotelIdentifier) &&
Objects.equals(this.featuredInd, recreation.featuredInd) &&
Objects.equals(this.lifestyleType, recreation.lifestyleType) &&
Objects.equals(this.location, recreation.location) &&
Objects.equals(this.descriptions, recreation.descriptions) &&
Objects.equals(this.multimedias, recreation.multimedias) &&
Objects.equals(this.contact, recreation.contact) &&
Objects.equals(this.address, recreation.address) &&
Objects.equals(this.commissionable, recreation.commissionable) &&
Objects.equals(this.name, recreation.name) &&
Objects.equals(this.proximityCode, recreation.proximityCode) &&
Objects.equals(this.sort, recreation.sort) &&
Objects.equals(this.minAgeAppropriateCode, recreation.minAgeAppropriateCode) &&
Objects.equals(this.bookable, recreation.bookable) &&
Objects.equals(this.active, recreation.active) &&
Objects.equals(this.disabilityFeatures, recreation.disabilityFeatures) &&
Objects.equals(this.securityFeatures, recreation.securityFeatures) &&
Objects.equals(this.socials, recreation.socials) &&
Objects.equals(this.applicableStart, recreation.applicableStart) &&
Objects.equals(this.applicableEnd, recreation.applicableEnd) &&
Objects.equals(this.reservationRequiredInd, recreation.reservationRequiredInd) &&
Objects.equals(this.opens, recreation.opens) &&
Objects.equals(this.closes, recreation.closes) &&
Objects.equals(this.fee, recreation.fee) &&
Objects.equals(this.percentDiscount, recreation.percentDiscount) &&
Objects.equals(this.daysOfWeek, recreation.daysOfWeek) &&
Objects.equals(this.recreationCategoryCode, recreation.recreationCategoryCode) &&
Objects.equals(this.amenities, recreation.amenities) &&
Objects.equals(this.pricingType, recreation.pricingType);
}
@Override
public int hashCode() {
return Objects.hash(identifier, hotelIdentifier, featuredInd, lifestyleType, location, descriptions, multimedias, contact, address, commissionable, name, proximityCode, sort, minAgeAppropriateCode, bookable, active, disabilityFeatures, securityFeatures, socials, applicableStart, applicableEnd, reservationRequiredInd, opens, closes, fee, percentDiscount, daysOfWeek, recreationCategoryCode, amenities, pricingType);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Recreation {\n");
sb.append(" identifier: ").append(toIndentedString(identifier)).append("\n");
sb.append(" hotelIdentifier: ").append(toIndentedString(hotelIdentifier)).append("\n");
sb.append(" featuredInd: ").append(toIndentedString(featuredInd)).append("\n");
sb.append(" lifestyleType: ").append(toIndentedString(lifestyleType)).append("\n");
sb.append(" location: ").append(toIndentedString(location)).append("\n");
sb.append(" descriptions: ").append(toIndentedString(descriptions)).append("\n");
sb.append(" multimedias: ").append(toIndentedString(multimedias)).append("\n");
sb.append(" contact: ").append(toIndentedString(contact)).append("\n");
sb.append(" address: ").append(toIndentedString(address)).append("\n");
sb.append(" commissionable: ").append(toIndentedString(commissionable)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" proximityCode: ").append(toIndentedString(proximityCode)).append("\n");
sb.append(" sort: ").append(toIndentedString(sort)).append("\n");
sb.append(" minAgeAppropriateCode: ").append(toIndentedString(minAgeAppropriateCode)).append("\n");
sb.append(" bookable: ").append(toIndentedString(bookable)).append("\n");
sb.append(" active: ").append(toIndentedString(active)).append("\n");
sb.append(" disabilityFeatures: ").append(toIndentedString(disabilityFeatures)).append("\n");
sb.append(" securityFeatures: ").append(toIndentedString(securityFeatures)).append("\n");
sb.append(" socials: ").append(toIndentedString(socials)).append("\n");
sb.append(" applicableStart: ").append(toIndentedString(applicableStart)).append("\n");
sb.append(" applicableEnd: ").append(toIndentedString(applicableEnd)).append("\n");
sb.append(" reservationRequiredInd: ").append(toIndentedString(reservationRequiredInd)).append("\n");
sb.append(" opens: ").append(toIndentedString(opens)).append("\n");
sb.append(" closes: ").append(toIndentedString(closes)).append("\n");
sb.append(" fee: ").append(toIndentedString(fee)).append("\n");
sb.append(" percentDiscount: ").append(toIndentedString(percentDiscount)).append("\n");
sb.append(" daysOfWeek: ").append(toIndentedString(daysOfWeek)).append("\n");
sb.append(" recreationCategoryCode: ").append(toIndentedString(recreationCategoryCode)).append("\n");
sb.append(" amenities: ").append(toIndentedString(amenities)).append("\n");
sb.append(" pricingType: ").append(toIndentedString(pricingType)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy