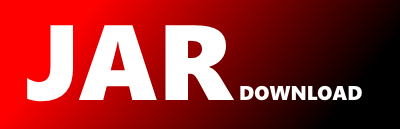
travel.wink.sdk.extranet.api.PropertyApi Maven / Gradle / Ivy
Show all versions of extranet-sdk-java Show documentation
package travel.wink.sdk.extranet.api;
import travel.wink.sdk.extranet.invoker.ApiClient;
import travel.wink.sdk.extranet.model.ChangePropertyNameRequestSupplier;
import java.io.File;
import travel.wink.sdk.extranet.model.GeneralManagerSupplier;
import travel.wink.sdk.extranet.model.GenericErrorMessage;
import travel.wink.sdk.extranet.model.HotelViewSupplier;
import travel.wink.sdk.extranet.model.ImproveWelcomeTextRequestSupplier;
import travel.wink.sdk.extranet.model.KeyValuePairSupplier;
import travel.wink.sdk.extranet.model.ShowPropertyPolicy400Response;
import travel.wink.sdk.extranet.model.SimpleDescriptionSupplier;
import travel.wink.sdk.extranet.model.SuggestAmenitiesRequestSupplier;
import travel.wink.sdk.extranet.model.SuggestProfileRequestSupplier;
import travel.wink.sdk.extranet.model.SuggestProfileResponseSupplier;
import travel.wink.sdk.extranet.model.SuggestWelcomeTextRequestSupplier;
import travel.wink.sdk.extranet.model.UniqueResultSupplier;
import travel.wink.sdk.extranet.model.UpdateExternalHotelStatusRequestSupplier;
import travel.wink.sdk.extranet.model.UpdatePropertyAmenitiesAndServicesRequestSupplier;
import travel.wink.sdk.extranet.model.UpsertPropertyAddressRequestSupplier;
import travel.wink.sdk.extranet.model.UpsertPropertyProfileRequestSupplier;
import travel.wink.sdk.extranet.model.UpsertReservationsDeskRequestSupplier;
import travel.wink.sdk.extranet.model.UpsertWelcomeTextRequestSupplier;
import java.util.HashMap;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import java.util.stream.Collectors;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
import org.springframework.core.ParameterizedTypeReference;
import org.springframework.web.reactive.function.client.WebClient.ResponseSpec;
import org.springframework.web.reactive.function.client.WebClientResponseException;
import org.springframework.core.io.FileSystemResource;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpMethod;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import reactor.core.publisher.Mono;
import reactor.core.publisher.Flux;
@jakarta.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-06-04T22:29:13.908295668+07:00[Asia/Bangkok]")
public class PropertyApi {
private ApiClient apiClient;
public PropertyApi() {
this(new ApiClient());
}
@Autowired
public PropertyApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Change property name
* Gives property owners a way to change the property name.
* 503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Update basic information for this hotel identifier
* @param changePropertyNameRequestSupplier The changePropertyNameRequestSupplier parameter
* @param winkVersion The winkVersion parameter
* @return HotelViewSupplier
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec changePropertyNameRequestCreation(String hotelIdentifier, ChangePropertyNameRequestSupplier changePropertyNameRequestSupplier, String winkVersion) throws WebClientResponseException {
Object postBody = changePropertyNameRequestSupplier;
// verify the required parameter 'hotelIdentifier' is set
if (hotelIdentifier == null) {
throw new WebClientResponseException("Missing the required parameter 'hotelIdentifier' when calling changePropertyName", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// verify the required parameter 'changePropertyNameRequestSupplier' is set
if (changePropertyNameRequestSupplier == null) {
throw new WebClientResponseException("Missing the required parameter 'changePropertyNameRequestSupplier' when calling changePropertyName", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
pathParams.put("hotelIdentifier", hotelIdentifier);
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (winkVersion != null)
headerParams.add("Wink-Version", apiClient.parameterToString(winkVersion));
final String[] localVarAccepts = {
"*/*", "application/json", "application/xml", "text/xml", "text/plain"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "oauth2ClientCredentials" };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/api/hotel/{hotelIdentifier}/change-name", HttpMethod.PATCH, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Change property name
* Gives property owners a way to change the property name.
* 503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Update basic information for this hotel identifier
* @param changePropertyNameRequestSupplier The changePropertyNameRequestSupplier parameter
* @param winkVersion The winkVersion parameter
* @return HotelViewSupplier
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono changePropertyName(String hotelIdentifier, ChangePropertyNameRequestSupplier changePropertyNameRequestSupplier, String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return changePropertyNameRequestCreation(hotelIdentifier, changePropertyNameRequestSupplier, winkVersion).bodyToMono(localVarReturnType);
}
/**
* Change property name
* Gives property owners a way to change the property name.
* 503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Update basic information for this hotel identifier
* @param changePropertyNameRequestSupplier The changePropertyNameRequestSupplier parameter
* @param winkVersion The winkVersion parameter
* @return ResponseEntity<HotelViewSupplier>
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono> changePropertyNameWithHttpInfo(String hotelIdentifier, ChangePropertyNameRequestSupplier changePropertyNameRequestSupplier, String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return changePropertyNameRequestCreation(hotelIdentifier, changePropertyNameRequestSupplier, winkVersion).toEntity(localVarReturnType);
}
/**
* Change property name
* Gives property owners a way to change the property name.
* 503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Update basic information for this hotel identifier
* @param changePropertyNameRequestSupplier The changePropertyNameRequestSupplier parameter
* @param winkVersion The winkVersion parameter
* @return ResponseSpec
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public ResponseSpec changePropertyNameWithResponseSpec(String hotelIdentifier, ChangePropertyNameRequestSupplier changePropertyNameRequestSupplier, String winkVersion) throws WebClientResponseException {
return changePropertyNameRequestCreation(hotelIdentifier, changePropertyNameRequestSupplier, winkVersion);
}
/**
* Improve welcome text
* Let AI improve existing property descriptions.
*
503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Update basic information for this hotel identifier
* @param improveWelcomeTextRequestSupplier The improveWelcomeTextRequestSupplier parameter
* @param winkVersion The winkVersion parameter
* @return SimpleDescriptionSupplier
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec improveWelcomeTextRequestCreation(String hotelIdentifier, ImproveWelcomeTextRequestSupplier improveWelcomeTextRequestSupplier, String winkVersion) throws WebClientResponseException {
Object postBody = improveWelcomeTextRequestSupplier;
// verify the required parameter 'hotelIdentifier' is set
if (hotelIdentifier == null) {
throw new WebClientResponseException("Missing the required parameter 'hotelIdentifier' when calling improveWelcomeText", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// verify the required parameter 'improveWelcomeTextRequestSupplier' is set
if (improveWelcomeTextRequestSupplier == null) {
throw new WebClientResponseException("Missing the required parameter 'improveWelcomeTextRequestSupplier' when calling improveWelcomeText", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
pathParams.put("hotelIdentifier", hotelIdentifier);
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (winkVersion != null)
headerParams.add("Wink-Version", apiClient.parameterToString(winkVersion));
final String[] localVarAccepts = {
"*/*", "application/json", "application/xml", "text/xml", "text/plain"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "oauth2ClientCredentials" };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/api/hotel/{hotelIdentifier}/welcome-text/improve", HttpMethod.POST, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Improve welcome text
* Let AI improve existing property descriptions.
* 503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Update basic information for this hotel identifier
* @param improveWelcomeTextRequestSupplier The improveWelcomeTextRequestSupplier parameter
* @param winkVersion The winkVersion parameter
* @return SimpleDescriptionSupplier
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono improveWelcomeText(String hotelIdentifier, ImproveWelcomeTextRequestSupplier improveWelcomeTextRequestSupplier, String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return improveWelcomeTextRequestCreation(hotelIdentifier, improveWelcomeTextRequestSupplier, winkVersion).bodyToMono(localVarReturnType);
}
/**
* Improve welcome text
* Let AI improve existing property descriptions.
* 503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Update basic information for this hotel identifier
* @param improveWelcomeTextRequestSupplier The improveWelcomeTextRequestSupplier parameter
* @param winkVersion The winkVersion parameter
* @return ResponseEntity<SimpleDescriptionSupplier>
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono> improveWelcomeTextWithHttpInfo(String hotelIdentifier, ImproveWelcomeTextRequestSupplier improveWelcomeTextRequestSupplier, String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return improveWelcomeTextRequestCreation(hotelIdentifier, improveWelcomeTextRequestSupplier, winkVersion).toEntity(localVarReturnType);
}
/**
* Improve welcome text
* Let AI improve existing property descriptions.
* 503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Update basic information for this hotel identifier
* @param improveWelcomeTextRequestSupplier The improveWelcomeTextRequestSupplier parameter
* @param winkVersion The winkVersion parameter
* @return ResponseSpec
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public ResponseSpec improveWelcomeTextWithResponseSpec(String hotelIdentifier, ImproveWelcomeTextRequestSupplier improveWelcomeTextRequestSupplier, String winkVersion) throws WebClientResponseException {
return improveWelcomeTextRequestCreation(hotelIdentifier, improveWelcomeTextRequestSupplier, winkVersion);
}
/**
* Check hotel name uniqueness
* Check if hotel name is a unique.
*
503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param key Search for uniqueness for this hotel name.
* @param hotelIdentifier Optional, existing hotel identifier
* @param winkVersion The winkVersion parameter
* @return UniqueResultSupplier
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec isHotelNameUniqueRequestCreation(String key, String hotelIdentifier, String winkVersion) throws WebClientResponseException {
Object postBody = null;
// verify the required parameter 'key' is set
if (key == null) {
throw new WebClientResponseException("Missing the required parameter 'key' when calling isHotelNameUnique", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "key", key));
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "hotelIdentifier", hotelIdentifier));
if (winkVersion != null)
headerParams.add("Wink-Version", apiClient.parameterToString(winkVersion));
final String[] localVarAccepts = {
"*/*", "application/json", "application/xml", "text/xml", "text/plain"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { };
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "oauth2ClientCredentials" };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/api/hotel/uniquename", HttpMethod.GET, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Check hotel name uniqueness
* Check if hotel name is a unique.
* 503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param key Search for uniqueness for this hotel name.
* @param hotelIdentifier Optional, existing hotel identifier
* @param winkVersion The winkVersion parameter
* @return UniqueResultSupplier
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono isHotelNameUnique(String key, String hotelIdentifier, String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return isHotelNameUniqueRequestCreation(key, hotelIdentifier, winkVersion).bodyToMono(localVarReturnType);
}
/**
* Check hotel name uniqueness
* Check if hotel name is a unique.
* 503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param key Search for uniqueness for this hotel name.
* @param hotelIdentifier Optional, existing hotel identifier
* @param winkVersion The winkVersion parameter
* @return ResponseEntity<UniqueResultSupplier>
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono> isHotelNameUniqueWithHttpInfo(String key, String hotelIdentifier, String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return isHotelNameUniqueRequestCreation(key, hotelIdentifier, winkVersion).toEntity(localVarReturnType);
}
/**
* Check hotel name uniqueness
* Check if hotel name is a unique.
* 503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param key Search for uniqueness for this hotel name.
* @param hotelIdentifier Optional, existing hotel identifier
* @param winkVersion The winkVersion parameter
* @return ResponseSpec
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public ResponseSpec isHotelNameUniqueWithResponseSpec(String key, String hotelIdentifier, String winkVersion) throws WebClientResponseException {
return isHotelNameUniqueRequestCreation(key, hotelIdentifier, winkVersion);
}
/**
* Show hotel
* Retrieve hotel by hotel identifier
*
503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Retrieve property record for this hotel identifier
* @param winkVersion The winkVersion parameter
* @return HotelViewSupplier
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec showHotelByManagerRequestCreation(String hotelIdentifier, String winkVersion) throws WebClientResponseException {
Object postBody = null;
// verify the required parameter 'hotelIdentifier' is set
if (hotelIdentifier == null) {
throw new WebClientResponseException("Missing the required parameter 'hotelIdentifier' when calling showHotelByManager", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
pathParams.put("hotelIdentifier", hotelIdentifier);
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (winkVersion != null)
headerParams.add("Wink-Version", apiClient.parameterToString(winkVersion));
final String[] localVarAccepts = {
"*/*", "application/json", "application/xml", "text/xml", "text/plain"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { };
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "oauth2ClientCredentials" };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/api/hotel/{hotelIdentifier}", HttpMethod.GET, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Show hotel
* Retrieve hotel by hotel identifier
* 503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Retrieve property record for this hotel identifier
* @param winkVersion The winkVersion parameter
* @return HotelViewSupplier
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono showHotelByManager(String hotelIdentifier, String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return showHotelByManagerRequestCreation(hotelIdentifier, winkVersion).bodyToMono(localVarReturnType);
}
/**
* Show hotel
* Retrieve hotel by hotel identifier
* 503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Retrieve property record for this hotel identifier
* @param winkVersion The winkVersion parameter
* @return ResponseEntity<HotelViewSupplier>
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono> showHotelByManagerWithHttpInfo(String hotelIdentifier, String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return showHotelByManagerRequestCreation(hotelIdentifier, winkVersion).toEntity(localVarReturnType);
}
/**
* Show hotel
* Retrieve hotel by hotel identifier
* 503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Retrieve property record for this hotel identifier
* @param winkVersion The winkVersion parameter
* @return ResponseSpec
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public ResponseSpec showHotelByManagerWithResponseSpec(String hotelIdentifier, String winkVersion) throws WebClientResponseException {
return showHotelByManagerRequestCreation(hotelIdentifier, winkVersion);
}
/**
* Show hotel status
* Retrieve hotel status
*
503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Show property status for this hotel identifier
* @param winkVersion The winkVersion parameter
* @return UpdateExternalHotelStatusRequestSupplier
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec showHotelStatusRequestCreation(String hotelIdentifier, String winkVersion) throws WebClientResponseException {
Object postBody = null;
// verify the required parameter 'hotelIdentifier' is set
if (hotelIdentifier == null) {
throw new WebClientResponseException("Missing the required parameter 'hotelIdentifier' when calling showHotelStatus", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
pathParams.put("hotelIdentifier", hotelIdentifier);
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (winkVersion != null)
headerParams.add("Wink-Version", apiClient.parameterToString(winkVersion));
final String[] localVarAccepts = {
"*/*", "application/json", "application/xml", "text/xml", "text/plain"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { };
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "oauth2ClientCredentials" };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/api/hotel/{hotelIdentifier}/status", HttpMethod.GET, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Show hotel status
* Retrieve hotel status
* 503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Show property status for this hotel identifier
* @param winkVersion The winkVersion parameter
* @return UpdateExternalHotelStatusRequestSupplier
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono showHotelStatus(String hotelIdentifier, String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return showHotelStatusRequestCreation(hotelIdentifier, winkVersion).bodyToMono(localVarReturnType);
}
/**
* Show hotel status
* Retrieve hotel status
* 503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Show property status for this hotel identifier
* @param winkVersion The winkVersion parameter
* @return ResponseEntity<UpdateExternalHotelStatusRequestSupplier>
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono> showHotelStatusWithHttpInfo(String hotelIdentifier, String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return showHotelStatusRequestCreation(hotelIdentifier, winkVersion).toEntity(localVarReturnType);
}
/**
* Show hotel status
* Retrieve hotel status
* 503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Show property status for this hotel identifier
* @param winkVersion The winkVersion parameter
* @return ResponseSpec
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public ResponseSpec showHotelStatusWithResponseSpec(String hotelIdentifier, String winkVersion) throws WebClientResponseException {
return showHotelStatusRequestCreation(hotelIdentifier, winkVersion);
}
/**
* Show hotels
* Retrieve a list of all your hotels
*
503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param winkVersion The winkVersion parameter
* @return List<HotelViewSupplier>
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec showHotelsByManagerRequestCreation(String winkVersion) throws WebClientResponseException {
Object postBody = null;
// create path and map variables
final Map pathParams = new HashMap();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (winkVersion != null)
headerParams.add("Wink-Version", apiClient.parameterToString(winkVersion));
final String[] localVarAccepts = {
"*/*", "application/json", "application/xml", "text/xml", "text/plain"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { };
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "oauth2ClientCredentials" };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/api/hotel/list", HttpMethod.GET, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Show hotels
* Retrieve a list of all your hotels
* 503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param winkVersion The winkVersion parameter
* @return List<HotelViewSupplier>
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Flux showHotelsByManager(String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return showHotelsByManagerRequestCreation(winkVersion).bodyToFlux(localVarReturnType);
}
/**
* Show hotels
* Retrieve a list of all your hotels
* 503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param winkVersion The winkVersion parameter
* @return ResponseEntity<List<HotelViewSupplier>>
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono>> showHotelsByManagerWithHttpInfo(String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return showHotelsByManagerRequestCreation(winkVersion).toEntityList(localVarReturnType);
}
/**
* Show hotels
* Retrieve a list of all your hotels
* 503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param winkVersion The winkVersion parameter
* @return ResponseSpec
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public ResponseSpec showHotelsByManagerWithResponseSpec(String winkVersion) throws WebClientResponseException {
return showHotelsByManagerRequestCreation(winkVersion);
}
/**
* Suggest property profile
* Let AI suggest property profile.
*
503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Suggest for this hotel identifier
* @param suggestProfileRequestSupplier The suggestProfileRequestSupplier parameter
* @param winkVersion The winkVersion parameter
* @return SuggestProfileResponseSupplier
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec suggestPropertyProfileRequestCreation(String hotelIdentifier, SuggestProfileRequestSupplier suggestProfileRequestSupplier, String winkVersion) throws WebClientResponseException {
Object postBody = suggestProfileRequestSupplier;
// verify the required parameter 'hotelIdentifier' is set
if (hotelIdentifier == null) {
throw new WebClientResponseException("Missing the required parameter 'hotelIdentifier' when calling suggestPropertyProfile", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// verify the required parameter 'suggestProfileRequestSupplier' is set
if (suggestProfileRequestSupplier == null) {
throw new WebClientResponseException("Missing the required parameter 'suggestProfileRequestSupplier' when calling suggestPropertyProfile", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
pathParams.put("hotelIdentifier", hotelIdentifier);
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (winkVersion != null)
headerParams.add("Wink-Version", apiClient.parameterToString(winkVersion));
final String[] localVarAccepts = {
"*/*", "application/json", "application/xml", "text/xml", "text/plain"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "oauth2ClientCredentials" };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/api/hotel/{hotelIdentifier}/profile/suggest", HttpMethod.POST, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Suggest property profile
* Let AI suggest property profile.
* 503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Suggest for this hotel identifier
* @param suggestProfileRequestSupplier The suggestProfileRequestSupplier parameter
* @param winkVersion The winkVersion parameter
* @return SuggestProfileResponseSupplier
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono suggestPropertyProfile(String hotelIdentifier, SuggestProfileRequestSupplier suggestProfileRequestSupplier, String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return suggestPropertyProfileRequestCreation(hotelIdentifier, suggestProfileRequestSupplier, winkVersion).bodyToMono(localVarReturnType);
}
/**
* Suggest property profile
* Let AI suggest property profile.
* 503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Suggest for this hotel identifier
* @param suggestProfileRequestSupplier The suggestProfileRequestSupplier parameter
* @param winkVersion The winkVersion parameter
* @return ResponseEntity<SuggestProfileResponseSupplier>
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono> suggestPropertyProfileWithHttpInfo(String hotelIdentifier, SuggestProfileRequestSupplier suggestProfileRequestSupplier, String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return suggestPropertyProfileRequestCreation(hotelIdentifier, suggestProfileRequestSupplier, winkVersion).toEntity(localVarReturnType);
}
/**
* Suggest property profile
* Let AI suggest property profile.
* 503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Suggest for this hotel identifier
* @param suggestProfileRequestSupplier The suggestProfileRequestSupplier parameter
* @param winkVersion The winkVersion parameter
* @return ResponseSpec
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public ResponseSpec suggestPropertyProfileWithResponseSpec(String hotelIdentifier, SuggestProfileRequestSupplier suggestProfileRequestSupplier, String winkVersion) throws WebClientResponseException {
return suggestPropertyProfileRequestCreation(hotelIdentifier, suggestProfileRequestSupplier, winkVersion);
}
/**
* Suggest property welcome text
* Let AI suggest property descriptions.
*
503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Suggest for this hotel identifier
* @param suggestWelcomeTextRequestSupplier The suggestWelcomeTextRequestSupplier parameter
* @param winkVersion The winkVersion parameter
* @return SimpleDescriptionSupplier
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec suggestPropertyWelcomeTextRequestCreation(String hotelIdentifier, SuggestWelcomeTextRequestSupplier suggestWelcomeTextRequestSupplier, String winkVersion) throws WebClientResponseException {
Object postBody = suggestWelcomeTextRequestSupplier;
// verify the required parameter 'hotelIdentifier' is set
if (hotelIdentifier == null) {
throw new WebClientResponseException("Missing the required parameter 'hotelIdentifier' when calling suggestPropertyWelcomeText", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// verify the required parameter 'suggestWelcomeTextRequestSupplier' is set
if (suggestWelcomeTextRequestSupplier == null) {
throw new WebClientResponseException("Missing the required parameter 'suggestWelcomeTextRequestSupplier' when calling suggestPropertyWelcomeText", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
pathParams.put("hotelIdentifier", hotelIdentifier);
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (winkVersion != null)
headerParams.add("Wink-Version", apiClient.parameterToString(winkVersion));
final String[] localVarAccepts = {
"*/*", "application/json", "application/xml", "text/xml", "text/plain"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "oauth2ClientCredentials" };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/api/hotel/{hotelIdentifier}/welcome-text/suggest", HttpMethod.POST, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Suggest property welcome text
* Let AI suggest property descriptions.
* 503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Suggest for this hotel identifier
* @param suggestWelcomeTextRequestSupplier The suggestWelcomeTextRequestSupplier parameter
* @param winkVersion The winkVersion parameter
* @return SimpleDescriptionSupplier
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono suggestPropertyWelcomeText(String hotelIdentifier, SuggestWelcomeTextRequestSupplier suggestWelcomeTextRequestSupplier, String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return suggestPropertyWelcomeTextRequestCreation(hotelIdentifier, suggestWelcomeTextRequestSupplier, winkVersion).bodyToMono(localVarReturnType);
}
/**
* Suggest property welcome text
* Let AI suggest property descriptions.
* 503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Suggest for this hotel identifier
* @param suggestWelcomeTextRequestSupplier The suggestWelcomeTextRequestSupplier parameter
* @param winkVersion The winkVersion parameter
* @return ResponseEntity<SimpleDescriptionSupplier>
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono> suggestPropertyWelcomeTextWithHttpInfo(String hotelIdentifier, SuggestWelcomeTextRequestSupplier suggestWelcomeTextRequestSupplier, String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return suggestPropertyWelcomeTextRequestCreation(hotelIdentifier, suggestWelcomeTextRequestSupplier, winkVersion).toEntity(localVarReturnType);
}
/**
* Suggest property welcome text
* Let AI suggest property descriptions.
* 503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Suggest for this hotel identifier
* @param suggestWelcomeTextRequestSupplier The suggestWelcomeTextRequestSupplier parameter
* @param winkVersion The winkVersion parameter
* @return ResponseSpec
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public ResponseSpec suggestPropertyWelcomeTextWithResponseSpec(String hotelIdentifier, SuggestWelcomeTextRequestSupplier suggestWelcomeTextRequestSupplier, String winkVersion) throws WebClientResponseException {
return suggestPropertyWelcomeTextRequestCreation(hotelIdentifier, suggestWelcomeTextRequestSupplier, winkVersion);
}
/**
* Suggest property amenities
* Let AI suggest property amenities.
*
503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Suggest for this hotel identifier
* @param suggestAmenitiesRequestSupplier The suggestAmenitiesRequestSupplier parameter
* @param winkVersion The winkVersion parameter
* @return List<KeyValuePairSupplier>
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec suggestPropertyWelcomeText1RequestCreation(String hotelIdentifier, SuggestAmenitiesRequestSupplier suggestAmenitiesRequestSupplier, String winkVersion) throws WebClientResponseException {
Object postBody = suggestAmenitiesRequestSupplier;
// verify the required parameter 'hotelIdentifier' is set
if (hotelIdentifier == null) {
throw new WebClientResponseException("Missing the required parameter 'hotelIdentifier' when calling suggestPropertyWelcomeText1", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// verify the required parameter 'suggestAmenitiesRequestSupplier' is set
if (suggestAmenitiesRequestSupplier == null) {
throw new WebClientResponseException("Missing the required parameter 'suggestAmenitiesRequestSupplier' when calling suggestPropertyWelcomeText1", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
pathParams.put("hotelIdentifier", hotelIdentifier);
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (winkVersion != null)
headerParams.add("Wink-Version", apiClient.parameterToString(winkVersion));
final String[] localVarAccepts = {
"*/*", "application/json", "application/xml", "text/xml", "text/plain"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "oauth2ClientCredentials" };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/api/hotel/{hotelIdentifier}/services/suggest", HttpMethod.POST, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Suggest property amenities
* Let AI suggest property amenities.
* 503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Suggest for this hotel identifier
* @param suggestAmenitiesRequestSupplier The suggestAmenitiesRequestSupplier parameter
* @param winkVersion The winkVersion parameter
* @return List<KeyValuePairSupplier>
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Flux suggestPropertyWelcomeText1(String hotelIdentifier, SuggestAmenitiesRequestSupplier suggestAmenitiesRequestSupplier, String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return suggestPropertyWelcomeText1RequestCreation(hotelIdentifier, suggestAmenitiesRequestSupplier, winkVersion).bodyToFlux(localVarReturnType);
}
/**
* Suggest property amenities
* Let AI suggest property amenities.
* 503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Suggest for this hotel identifier
* @param suggestAmenitiesRequestSupplier The suggestAmenitiesRequestSupplier parameter
* @param winkVersion The winkVersion parameter
* @return ResponseEntity<List<KeyValuePairSupplier>>
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono>> suggestPropertyWelcomeText1WithHttpInfo(String hotelIdentifier, SuggestAmenitiesRequestSupplier suggestAmenitiesRequestSupplier, String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return suggestPropertyWelcomeText1RequestCreation(hotelIdentifier, suggestAmenitiesRequestSupplier, winkVersion).toEntityList(localVarReturnType);
}
/**
* Suggest property amenities
* Let AI suggest property amenities.
* 503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Suggest for this hotel identifier
* @param suggestAmenitiesRequestSupplier The suggestAmenitiesRequestSupplier parameter
* @param winkVersion The winkVersion parameter
* @return ResponseSpec
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public ResponseSpec suggestPropertyWelcomeText1WithResponseSpec(String hotelIdentifier, SuggestAmenitiesRequestSupplier suggestAmenitiesRequestSupplier, String winkVersion) throws WebClientResponseException {
return suggestPropertyWelcomeText1RequestCreation(hotelIdentifier, suggestAmenitiesRequestSupplier, winkVersion);
}
/**
* Update Address
* Update address
*
503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Update basic information for this hotel identifier
* @param upsertPropertyAddressRequestSupplier The upsertPropertyAddressRequestSupplier parameter
* @param winkVersion The winkVersion parameter
* @return HotelViewSupplier
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec updateAddressRequestCreation(String hotelIdentifier, UpsertPropertyAddressRequestSupplier upsertPropertyAddressRequestSupplier, String winkVersion) throws WebClientResponseException {
Object postBody = upsertPropertyAddressRequestSupplier;
// verify the required parameter 'hotelIdentifier' is set
if (hotelIdentifier == null) {
throw new WebClientResponseException("Missing the required parameter 'hotelIdentifier' when calling updateAddress", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// verify the required parameter 'upsertPropertyAddressRequestSupplier' is set
if (upsertPropertyAddressRequestSupplier == null) {
throw new WebClientResponseException("Missing the required parameter 'upsertPropertyAddressRequestSupplier' when calling updateAddress", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
pathParams.put("hotelIdentifier", hotelIdentifier);
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (winkVersion != null)
headerParams.add("Wink-Version", apiClient.parameterToString(winkVersion));
final String[] localVarAccepts = {
"*/*", "application/json", "application/xml", "text/xml", "text/plain"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "oauth2ClientCredentials" };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/api/hotel/{hotelIdentifier}/address", HttpMethod.PATCH, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Update Address
* Update address
* 503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Update basic information for this hotel identifier
* @param upsertPropertyAddressRequestSupplier The upsertPropertyAddressRequestSupplier parameter
* @param winkVersion The winkVersion parameter
* @return HotelViewSupplier
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono updateAddress(String hotelIdentifier, UpsertPropertyAddressRequestSupplier upsertPropertyAddressRequestSupplier, String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return updateAddressRequestCreation(hotelIdentifier, upsertPropertyAddressRequestSupplier, winkVersion).bodyToMono(localVarReturnType);
}
/**
* Update Address
* Update address
* 503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Update basic information for this hotel identifier
* @param upsertPropertyAddressRequestSupplier The upsertPropertyAddressRequestSupplier parameter
* @param winkVersion The winkVersion parameter
* @return ResponseEntity<HotelViewSupplier>
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono> updateAddressWithHttpInfo(String hotelIdentifier, UpsertPropertyAddressRequestSupplier upsertPropertyAddressRequestSupplier, String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return updateAddressRequestCreation(hotelIdentifier, upsertPropertyAddressRequestSupplier, winkVersion).toEntity(localVarReturnType);
}
/**
* Update Address
* Update address
* 503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Update basic information for this hotel identifier
* @param upsertPropertyAddressRequestSupplier The upsertPropertyAddressRequestSupplier parameter
* @param winkVersion The winkVersion parameter
* @return ResponseSpec
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public ResponseSpec updateAddressWithResponseSpec(String hotelIdentifier, UpsertPropertyAddressRequestSupplier upsertPropertyAddressRequestSupplier, String winkVersion) throws WebClientResponseException {
return updateAddressRequestCreation(hotelIdentifier, upsertPropertyAddressRequestSupplier, winkVersion);
}
/**
* Update General Manager
* Update general manager information
*
503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Update basic information for this hotel identifier
* @param generalManagerSupplier The generalManagerSupplier parameter
* @param winkVersion The winkVersion parameter
* @return HotelViewSupplier
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec updateGeneralManagerRequestCreation(String hotelIdentifier, GeneralManagerSupplier generalManagerSupplier, String winkVersion) throws WebClientResponseException {
Object postBody = generalManagerSupplier;
// verify the required parameter 'hotelIdentifier' is set
if (hotelIdentifier == null) {
throw new WebClientResponseException("Missing the required parameter 'hotelIdentifier' when calling updateGeneralManager", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// verify the required parameter 'generalManagerSupplier' is set
if (generalManagerSupplier == null) {
throw new WebClientResponseException("Missing the required parameter 'generalManagerSupplier' when calling updateGeneralManager", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
pathParams.put("hotelIdentifier", hotelIdentifier);
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (winkVersion != null)
headerParams.add("Wink-Version", apiClient.parameterToString(winkVersion));
final String[] localVarAccepts = {
"*/*", "application/json", "application/xml", "text/xml", "text/plain"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "oauth2ClientCredentials" };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/api/hotel/{hotelIdentifier}/general-manager", HttpMethod.PATCH, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Update General Manager
* Update general manager information
* 503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Update basic information for this hotel identifier
* @param generalManagerSupplier The generalManagerSupplier parameter
* @param winkVersion The winkVersion parameter
* @return HotelViewSupplier
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono updateGeneralManager(String hotelIdentifier, GeneralManagerSupplier generalManagerSupplier, String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return updateGeneralManagerRequestCreation(hotelIdentifier, generalManagerSupplier, winkVersion).bodyToMono(localVarReturnType);
}
/**
* Update General Manager
* Update general manager information
* 503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Update basic information for this hotel identifier
* @param generalManagerSupplier The generalManagerSupplier parameter
* @param winkVersion The winkVersion parameter
* @return ResponseEntity<HotelViewSupplier>
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono> updateGeneralManagerWithHttpInfo(String hotelIdentifier, GeneralManagerSupplier generalManagerSupplier, String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return updateGeneralManagerRequestCreation(hotelIdentifier, generalManagerSupplier, winkVersion).toEntity(localVarReturnType);
}
/**
* Update General Manager
* Update general manager information
* 503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Update basic information for this hotel identifier
* @param generalManagerSupplier The generalManagerSupplier parameter
* @param winkVersion The winkVersion parameter
* @return ResponseSpec
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public ResponseSpec updateGeneralManagerWithResponseSpec(String hotelIdentifier, GeneralManagerSupplier generalManagerSupplier, String winkVersion) throws WebClientResponseException {
return updateGeneralManagerRequestCreation(hotelIdentifier, generalManagerSupplier, winkVersion);
}
/**
* Update hotel status
* Update hotel status
*
503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Change status for this hotel identifier
* @param updateExternalHotelStatusRequestSupplier The updateExternalHotelStatusRequestSupplier parameter
* @param winkVersion The winkVersion parameter
* @return HotelViewSupplier
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec updateHotelStatusRequestCreation(String hotelIdentifier, UpdateExternalHotelStatusRequestSupplier updateExternalHotelStatusRequestSupplier, String winkVersion) throws WebClientResponseException {
Object postBody = updateExternalHotelStatusRequestSupplier;
// verify the required parameter 'hotelIdentifier' is set
if (hotelIdentifier == null) {
throw new WebClientResponseException("Missing the required parameter 'hotelIdentifier' when calling updateHotelStatus", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// verify the required parameter 'updateExternalHotelStatusRequestSupplier' is set
if (updateExternalHotelStatusRequestSupplier == null) {
throw new WebClientResponseException("Missing the required parameter 'updateExternalHotelStatusRequestSupplier' when calling updateHotelStatus", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
pathParams.put("hotelIdentifier", hotelIdentifier);
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (winkVersion != null)
headerParams.add("Wink-Version", apiClient.parameterToString(winkVersion));
final String[] localVarAccepts = {
"*/*", "application/json", "application/xml", "text/xml", "text/plain"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "oauth2ClientCredentials" };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/api/hotel/{hotelIdentifier}/status", HttpMethod.PATCH, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Update hotel status
* Update hotel status
* 503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Change status for this hotel identifier
* @param updateExternalHotelStatusRequestSupplier The updateExternalHotelStatusRequestSupplier parameter
* @param winkVersion The winkVersion parameter
* @return HotelViewSupplier
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono updateHotelStatus(String hotelIdentifier, UpdateExternalHotelStatusRequestSupplier updateExternalHotelStatusRequestSupplier, String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return updateHotelStatusRequestCreation(hotelIdentifier, updateExternalHotelStatusRequestSupplier, winkVersion).bodyToMono(localVarReturnType);
}
/**
* Update hotel status
* Update hotel status
* 503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Change status for this hotel identifier
* @param updateExternalHotelStatusRequestSupplier The updateExternalHotelStatusRequestSupplier parameter
* @param winkVersion The winkVersion parameter
* @return ResponseEntity<HotelViewSupplier>
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono> updateHotelStatusWithHttpInfo(String hotelIdentifier, UpdateExternalHotelStatusRequestSupplier updateExternalHotelStatusRequestSupplier, String winkVersion) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return updateHotelStatusRequestCreation(hotelIdentifier, updateExternalHotelStatusRequestSupplier, winkVersion).toEntity(localVarReturnType);
}
/**
* Update hotel status
* Update hotel status
* 503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Change status for this hotel identifier
* @param updateExternalHotelStatusRequestSupplier The updateExternalHotelStatusRequestSupplier parameter
* @param winkVersion The winkVersion parameter
* @return ResponseSpec
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public ResponseSpec updateHotelStatusWithResponseSpec(String hotelIdentifier, UpdateExternalHotelStatusRequestSupplier updateExternalHotelStatusRequestSupplier, String winkVersion) throws WebClientResponseException {
return updateHotelStatusRequestCreation(hotelIdentifier, updateExternalHotelStatusRequestSupplier, winkVersion);
}
/**
* Update property profile
* Update basic property information
*
503 - Service Unavailable
*
400 - Bad Request
*
405 - Method Not Allowed
*
415 - Unsupported Media Type
*
500 - Internal Server Error
*
403 - Forbidden
*
401 - Unauthorized
*
200 - OK
* @param hotelIdentifier Update basic information for this hotel identifier
* @param upsertPropertyProfileRequestSupplier The upsertPropertyProfileRequestSupplier parameter
* @param winkVersion The winkVersion parameter
* @return HotelViewSupplier
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec updatePropertyProfileRequestCreation(String hotelIdentifier, UpsertPropertyProfileRequestSupplier upsertPropertyProfileRequestSupplier, String winkVersion) throws WebClientResponseException {
Object postBody = upsertPropertyProfileRequestSupplier;
// verify the required parameter 'hotelIdentifier' is set
if (hotelIdentifier == null) {
throw new WebClientResponseException("Missing the required parameter 'hotelIdentifier' when calling updatePropertyProfile", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// verify the required parameter 'upsertPropertyProfileRequestSupplier' is set
if (upsertPropertyProfileRequestSupplier == null) {
throw new WebClientResponseException("Missing the required parameter 'upsertPropertyProfileRequestSupplier' when calling updatePropertyProfile", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
pathParams.put("hotelIdentifier", hotelIdentifier);
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
if (winkVersion != null)
headerParams.add("Wink-Version", apiClient.parameterToString(winkVersion));
final String[] localVarAccepts = {
"*/*", "application/json", "application/xml", "text/xml", "text/plain"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "oauth2ClientCredentials" };
ParameterizedTypeReference