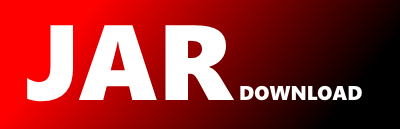
travel.wink.sdk.extranet.model.RateSupplier Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of extranet-sdk-java Show documentation
Show all versions of extranet-sdk-java Show documentation
Java SDK for the wink Extranet API
/*
* Wink API
* ## APIs Not every integrator needs every APIs. For that reason, we have separated APIs into context. - [Affiliate](/affiliate): All APIs related to selling travel inventory as an affiliate. - [Analytics](/analytics): All APIs related to tracking metrics across a wide variety of data source segments including, more entertaining, leaderboard metrics. - [Booking](/booking): All APIs related to creating platform bookings. - [Channel Manager](/channel-manager): All APIs related to channel managers who want to integrate with our platform. - [Extranet](/extranet): All APIs related to managing travel inventory and suppliers. - [Inventory](/inventory): All APIs related to retrieve known travel inventory as it was found using the Lookup API.. - [Lookup](/lookup): All APIs related to locating inventory by region, locale and property flags. - [Reference](/reference): All APIs related to retrieving platform-supported taxonomies. - [TripPay](/payment): All APIs related to TripPay account management, booking, mapping and integration features. ## SDKs We are actively working on supporting the most used languages out there. If you don't see your language here, reach out to us with a request to officially add your language. In the meantime, if you want to roll your own SDK, you can do so by downloading the OpenAPI spec and using one of the many available OpenAPI generators available: [https://openapi-generator.tech/docs/generators](https://openapi-generator.tech/docs/generators). - Java SDK [https://github.com/wink-travel/wink-sdk-java](https://github.com/wink-travel/wink-sdk-java) ## Usage These features are made available to you via a [REST API](https://en.wikipedia.org/wiki/Representational_state_transfer). This API is language agnostic. ## Versioning We chose to version our endpoints in a way that we hope affects your integration with us the least. You request the version of our API you wish to work with via the `Wink-Version` header. When it's time for you to upgrade, you only have to change the version number to get access to our updated endpoints. ## Release history - 2022-10-15: v2.0 - Removed HATEOAS and added Wink-Version header - 2022-05-08: v1 - Exposed channel manager API - 2021-07-01: v1 - Initial release # Extranet API Welcome to the Extranet API - A programmer-friendly way to manage your travel inventory on the wink payment. This API offers a superset of the features you can find at [https://extranet.wink.travel](https://extranet.wink.travel) and gives you all the tools you need to ready your properties and inventory for sale across 10000s of our unique sales channels. What differentiates us from existing Extranets is 1. we make it available for everyone to use and 2. the care we take in only working with properties that have quality, curated content and ways to bundle and cross sell customers with ancillary products and experiences. Content creators have the ability to make their inventory look great and be searchable in a wide variety of ways. You won't find properties with generic inventory, low resolution pictures and little ancillary content that can easily be found everywhere else on the internet. # Integrations We have already integrated with the most well-known channel managers so you don't have to. Once your properties are set up, you can finish the setup by mapping your property to wink using your channel manager partner portal. If your properties don't have a channel manager, you can easily manage rates and availability with this API. - Allotz - CloudBeds / MyAllocator - Comanche - d-edge - FastBooking - HotelLink - HoteliersGuru - Omnibees - RateGain - Rate Tiger - ResAvenue - Siteminder - Sabre SynXis - Travelclick - Yieldplanet # Intended Audience Programmers are [most likely] a requirement to start integrating with wink. Companies and organizations that would most benefit from integrating with us are new and existing travel companies that have relationships with suppliers and that need an advanced system from which to manage their travel inventory and get that same inventory out to as many eyeballs as possible at the lowest price possible. - Hotel chains - Hotel brands - Travel tech companies - Destination sites - Integrators - Aggregators - Destination management companies - Travel agencies - OTAs
*
* The version of the OpenAPI document: 29.61.2
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package travel.wink.sdk.extranet.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.time.LocalDate;
import java.util.UUID;
import travel.wink.sdk.extranet.model.CustomMonetaryAmount;
import travel.wink.sdk.extranet.model.VariableChargeSupplier;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
import jakarta.validation.constraints.*;
import jakarta.validation.Valid;
import org.hibernate.validator.constraints.*;
/**
* RateSupplier
*/
@JsonPropertyOrder({
RateSupplier.JSON_PROPERTY_IDENTIFIER,
RateSupplier.JSON_PROPERTY_HOTEL_IDENTIFIER,
RateSupplier.JSON_PROPERTY_RATE_SOURCE,
RateSupplier.JSON_PROPERTY_MASTER_RATE_IDENTIFIER,
RateSupplier.JSON_PROPERTY_RATE_PLAN_IDENTIFIER,
RateSupplier.JSON_PROPERTY_GUEST_ROOM_IDENTIFIER,
RateSupplier.JSON_PROPERTY_RATE,
RateSupplier.JSON_PROPERTY_MASTER,
RateSupplier.JSON_PROPERTY_CLOSED_ON_ARRIVAL,
RateSupplier.JSON_PROPERTY_CLOSED_ON_DEPARTURE,
RateSupplier.JSON_PROPERTY_DATE,
RateSupplier.JSON_PROPERTY_QUANTITY,
RateSupplier.JSON_PROPERTY_MIN_OCCUPANCY,
RateSupplier.JSON_PROPERTY_MAX_OCCUPANCY,
RateSupplier.JSON_PROPERTY_INCLUDED_ADULT_OCCUPANCY,
RateSupplier.JSON_PROPERTY_INCLUDED_CHILD_OCCUPANCY,
RateSupplier.JSON_PROPERTY_MAX_ADULT_OCCUPANCY,
RateSupplier.JSON_PROPERTY_MAX_CHILD_OCCUPANCY,
RateSupplier.JSON_PROPERTY_MIN_LENGTH_OF_STAY,
RateSupplier.JSON_PROPERTY_MAX_LENGTH_OF_STAY,
RateSupplier.JSON_PROPERTY_SINGLE_OCCUPANCY_RATE_MODIFIER,
RateSupplier.JSON_PROPERTY_EXTRA_PAX_RATE_MODIFIER,
RateSupplier.JSON_PROPERTY_EXTRA_CHILD_RATE_MODIFIER,
RateSupplier.JSON_PROPERTY_AVAILABLE
})
@JsonTypeName("Rate_Supplier")
@jakarta.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-06-04T22:29:13.908295668+07:00[Asia/Bangkok]")
public class RateSupplier {
public static final String JSON_PROPERTY_IDENTIFIER = "identifier";
private UUID identifier;
public static final String JSON_PROPERTY_HOTEL_IDENTIFIER = "hotelIdentifier";
private UUID hotelIdentifier;
public static final String JSON_PROPERTY_RATE_SOURCE = "rateSource";
private String rateSource = "TRAVELIKO";
public static final String JSON_PROPERTY_MASTER_RATE_IDENTIFIER = "masterRateIdentifier";
private UUID masterRateIdentifier;
public static final String JSON_PROPERTY_RATE_PLAN_IDENTIFIER = "ratePlanIdentifier";
private UUID ratePlanIdentifier;
public static final String JSON_PROPERTY_GUEST_ROOM_IDENTIFIER = "guestRoomIdentifier";
private UUID guestRoomIdentifier;
public static final String JSON_PROPERTY_RATE = "rate";
private CustomMonetaryAmount rate;
public static final String JSON_PROPERTY_MASTER = "master";
private Boolean master = true;
public static final String JSON_PROPERTY_CLOSED_ON_ARRIVAL = "closedOnArrival";
private Boolean closedOnArrival = false;
public static final String JSON_PROPERTY_CLOSED_ON_DEPARTURE = "closedOnDeparture";
private Boolean closedOnDeparture = false;
public static final String JSON_PROPERTY_DATE = "date";
private LocalDate date;
public static final String JSON_PROPERTY_QUANTITY = "quantity";
private Integer quantity = 0;
public static final String JSON_PROPERTY_MIN_OCCUPANCY = "minOccupancy";
private Integer minOccupancy = 1;
public static final String JSON_PROPERTY_MAX_OCCUPANCY = "maxOccupancy";
private Integer maxOccupancy = 2;
public static final String JSON_PROPERTY_INCLUDED_ADULT_OCCUPANCY = "includedAdultOccupancy";
private Integer includedAdultOccupancy = 2;
public static final String JSON_PROPERTY_INCLUDED_CHILD_OCCUPANCY = "includedChildOccupancy";
private Integer includedChildOccupancy = 0;
public static final String JSON_PROPERTY_MAX_ADULT_OCCUPANCY = "maxAdultOccupancy";
private Integer maxAdultOccupancy = 2;
public static final String JSON_PROPERTY_MAX_CHILD_OCCUPANCY = "maxChildOccupancy";
private Integer maxChildOccupancy = 0;
public static final String JSON_PROPERTY_MIN_LENGTH_OF_STAY = "minLengthOfStay";
private Integer minLengthOfStay = -1;
public static final String JSON_PROPERTY_MAX_LENGTH_OF_STAY = "maxLengthOfStay";
private Integer maxLengthOfStay = -1;
public static final String JSON_PROPERTY_SINGLE_OCCUPANCY_RATE_MODIFIER = "singleOccupancyRateModifier";
private VariableChargeSupplier singleOccupancyRateModifier;
public static final String JSON_PROPERTY_EXTRA_PAX_RATE_MODIFIER = "extraPaxRateModifier";
private VariableChargeSupplier extraPaxRateModifier;
public static final String JSON_PROPERTY_EXTRA_CHILD_RATE_MODIFIER = "extraChildRateModifier";
private VariableChargeSupplier extraChildRateModifier;
public static final String JSON_PROPERTY_AVAILABLE = "available";
private Boolean available;
public RateSupplier() {
}
public RateSupplier identifier(UUID identifier) {
this.identifier = identifier;
return this;
}
/**
* Unique record identifier.
* @return identifier
**/
@jakarta.annotation.Nonnull
@NotNull
@Valid
@JsonProperty(JSON_PROPERTY_IDENTIFIER)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public UUID getIdentifier() {
return identifier;
}
@JsonProperty(JSON_PROPERTY_IDENTIFIER)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setIdentifier(UUID identifier) {
this.identifier = identifier;
}
public RateSupplier hotelIdentifier(UUID hotelIdentifier) {
this.hotelIdentifier = hotelIdentifier;
return this;
}
/**
* Owner of daily rate.
* @return hotelIdentifier
**/
@jakarta.annotation.Nonnull
@NotNull
@Valid
@JsonProperty(JSON_PROPERTY_HOTEL_IDENTIFIER)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public UUID getHotelIdentifier() {
return hotelIdentifier;
}
@JsonProperty(JSON_PROPERTY_HOTEL_IDENTIFIER)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setHotelIdentifier(UUID hotelIdentifier) {
this.hotelIdentifier = hotelIdentifier;
}
public RateSupplier rateSource(String rateSource) {
this.rateSource = rateSource;
return this;
}
/**
* Indicate where this rate originated from. Leave as TRAVELIKO unless you are a channel manager and responsible for the property's rates externally of this payment.
* @return rateSource
**/
@jakarta.annotation.Nonnull
@NotNull
@JsonProperty(JSON_PROPERTY_RATE_SOURCE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getRateSource() {
return rateSource;
}
@JsonProperty(JSON_PROPERTY_RATE_SOURCE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setRateSource(String rateSource) {
this.rateSource = rateSource;
}
public RateSupplier masterRateIdentifier(UUID masterRateIdentifier) {
this.masterRateIdentifier = masterRateIdentifier;
return this;
}
/**
* Master rate associated with this daily rate.
* @return masterRateIdentifier
**/
@jakarta.annotation.Nonnull
@NotNull
@Valid
@JsonProperty(JSON_PROPERTY_MASTER_RATE_IDENTIFIER)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public UUID getMasterRateIdentifier() {
return masterRateIdentifier;
}
@JsonProperty(JSON_PROPERTY_MASTER_RATE_IDENTIFIER)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setMasterRateIdentifier(UUID masterRateIdentifier) {
this.masterRateIdentifier = masterRateIdentifier;
}
public RateSupplier ratePlanIdentifier(UUID ratePlanIdentifier) {
this.ratePlanIdentifier = ratePlanIdentifier;
return this;
}
/**
* Rate plan associated with this daily rate.
* @return ratePlanIdentifier
**/
@jakarta.annotation.Nonnull
@NotNull
@Valid
@JsonProperty(JSON_PROPERTY_RATE_PLAN_IDENTIFIER)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public UUID getRatePlanIdentifier() {
return ratePlanIdentifier;
}
@JsonProperty(JSON_PROPERTY_RATE_PLAN_IDENTIFIER)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setRatePlanIdentifier(UUID ratePlanIdentifier) {
this.ratePlanIdentifier = ratePlanIdentifier;
}
public RateSupplier guestRoomIdentifier(UUID guestRoomIdentifier) {
this.guestRoomIdentifier = guestRoomIdentifier;
return this;
}
/**
* Guest room associated with this daily rate.
* @return guestRoomIdentifier
**/
@jakarta.annotation.Nonnull
@NotNull
@Valid
@JsonProperty(JSON_PROPERTY_GUEST_ROOM_IDENTIFIER)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public UUID getGuestRoomIdentifier() {
return guestRoomIdentifier;
}
@JsonProperty(JSON_PROPERTY_GUEST_ROOM_IDENTIFIER)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setGuestRoomIdentifier(UUID guestRoomIdentifier) {
this.guestRoomIdentifier = guestRoomIdentifier;
}
public RateSupplier rate(CustomMonetaryAmount rate) {
this.rate = rate;
return this;
}
/**
* Get rate
* @return rate
**/
@jakarta.annotation.Nonnull
@NotNull
@Valid
@JsonProperty(JSON_PROPERTY_RATE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public CustomMonetaryAmount getRate() {
return rate;
}
@JsonProperty(JSON_PROPERTY_RATE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setRate(CustomMonetaryAmount rate) {
this.rate = rate;
}
public RateSupplier master(Boolean master) {
this.master = master;
return this;
}
/**
* This flag indicates whether this rate is available for this date.
* @return master
**/
@jakarta.annotation.Nonnull
@NotNull
@JsonProperty(JSON_PROPERTY_MASTER)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Boolean getMaster() {
return master;
}
@JsonProperty(JSON_PROPERTY_MASTER)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setMaster(Boolean master) {
this.master = master;
}
public RateSupplier closedOnArrival(Boolean closedOnArrival) {
this.closedOnArrival = closedOnArrival;
return this;
}
/**
* This flag indicates whether a guest can arrive at the property on this date.
* @return closedOnArrival
**/
@jakarta.annotation.Nonnull
@NotNull
@JsonProperty(JSON_PROPERTY_CLOSED_ON_ARRIVAL)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Boolean getClosedOnArrival() {
return closedOnArrival;
}
@JsonProperty(JSON_PROPERTY_CLOSED_ON_ARRIVAL)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setClosedOnArrival(Boolean closedOnArrival) {
this.closedOnArrival = closedOnArrival;
}
public RateSupplier closedOnDeparture(Boolean closedOnDeparture) {
this.closedOnDeparture = closedOnDeparture;
return this;
}
/**
* This flag indicates whether a guest can leave the property on this date.
* @return closedOnDeparture
**/
@jakarta.annotation.Nonnull
@NotNull
@JsonProperty(JSON_PROPERTY_CLOSED_ON_DEPARTURE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Boolean getClosedOnDeparture() {
return closedOnDeparture;
}
@JsonProperty(JSON_PROPERTY_CLOSED_ON_DEPARTURE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setClosedOnDeparture(Boolean closedOnDeparture) {
this.closedOnDeparture = closedOnDeparture;
}
public RateSupplier date(LocalDate date) {
this.date = date;
return this;
}
/**
* The date this rate is applicable for.
* @return date
**/
@jakarta.annotation.Nonnull
@NotNull
@Valid
@JsonProperty(JSON_PROPERTY_DATE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public LocalDate getDate() {
return date;
}
@JsonProperty(JSON_PROPERTY_DATE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setDate(LocalDate date) {
this.date = date;
}
public RateSupplier quantity(Integer quantity) {
this.quantity = quantity;
return this;
}
/**
* Amount of rooms available for this date.
* @return quantity
**/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_QUANTITY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getQuantity() {
return quantity;
}
@JsonProperty(JSON_PROPERTY_QUANTITY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setQuantity(Integer quantity) {
this.quantity = quantity;
}
public RateSupplier minOccupancy(Integer minOccupancy) {
this.minOccupancy = minOccupancy;
return this;
}
/**
* Minimum number of guests allowed in a room type.
* minimum: 1
* @return minOccupancy
**/
@jakarta.annotation.Nonnull
@NotNull
@Min(1)
@JsonProperty(JSON_PROPERTY_MIN_OCCUPANCY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Integer getMinOccupancy() {
return minOccupancy;
}
@JsonProperty(JSON_PROPERTY_MIN_OCCUPANCY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setMinOccupancy(Integer minOccupancy) {
this.minOccupancy = minOccupancy;
}
public RateSupplier maxOccupancy(Integer maxOccupancy) {
this.maxOccupancy = maxOccupancy;
return this;
}
/**
* Maximum number of guest allowed in a room type.
* minimum: 1
* @return maxOccupancy
**/
@jakarta.annotation.Nonnull
@NotNull
@Min(1)
@JsonProperty(JSON_PROPERTY_MAX_OCCUPANCY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Integer getMaxOccupancy() {
return maxOccupancy;
}
@JsonProperty(JSON_PROPERTY_MAX_OCCUPANCY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setMaxOccupancy(Integer maxOccupancy) {
this.maxOccupancy = maxOccupancy;
}
public RateSupplier includedAdultOccupancy(Integer includedAdultOccupancy) {
this.includedAdultOccupancy = includedAdultOccupancy;
return this;
}
/**
* The number of pax the room price was meant for
* minimum: 0
* @return includedAdultOccupancy
**/
@jakarta.annotation.Nonnull
@NotNull
@Min(0)
@JsonProperty(JSON_PROPERTY_INCLUDED_ADULT_OCCUPANCY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Integer getIncludedAdultOccupancy() {
return includedAdultOccupancy;
}
@JsonProperty(JSON_PROPERTY_INCLUDED_ADULT_OCCUPANCY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setIncludedAdultOccupancy(Integer includedAdultOccupancy) {
this.includedAdultOccupancy = includedAdultOccupancy;
}
public RateSupplier includedChildOccupancy(Integer includedChildOccupancy) {
this.includedChildOccupancy = includedChildOccupancy;
return this;
}
/**
* The number of children the room price was meant for
* minimum: 0
* @return includedChildOccupancy
**/
@jakarta.annotation.Nonnull
@NotNull
@Min(0)
@JsonProperty(JSON_PROPERTY_INCLUDED_CHILD_OCCUPANCY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Integer getIncludedChildOccupancy() {
return includedChildOccupancy;
}
@JsonProperty(JSON_PROPERTY_INCLUDED_CHILD_OCCUPANCY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setIncludedChildOccupancy(Integer includedChildOccupancy) {
this.includedChildOccupancy = includedChildOccupancy;
}
public RateSupplier maxAdultOccupancy(Integer maxAdultOccupancy) {
this.maxAdultOccupancy = maxAdultOccupancy;
return this;
}
/**
* Maximum number of adults allowed in a room type.
* minimum: 1
* @return maxAdultOccupancy
**/
@jakarta.annotation.Nonnull
@NotNull
@Min(1)
@JsonProperty(JSON_PROPERTY_MAX_ADULT_OCCUPANCY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Integer getMaxAdultOccupancy() {
return maxAdultOccupancy;
}
@JsonProperty(JSON_PROPERTY_MAX_ADULT_OCCUPANCY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setMaxAdultOccupancy(Integer maxAdultOccupancy) {
this.maxAdultOccupancy = maxAdultOccupancy;
}
public RateSupplier maxChildOccupancy(Integer maxChildOccupancy) {
this.maxChildOccupancy = maxChildOccupancy;
return this;
}
/**
* Maximum number of children allowed in a room type.
* minimum: 0
* @return maxChildOccupancy
**/
@jakarta.annotation.Nonnull
@NotNull
@Min(0)
@JsonProperty(JSON_PROPERTY_MAX_CHILD_OCCUPANCY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public Integer getMaxChildOccupancy() {
return maxChildOccupancy;
}
@JsonProperty(JSON_PROPERTY_MAX_CHILD_OCCUPANCY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setMaxChildOccupancy(Integer maxChildOccupancy) {
this.maxChildOccupancy = maxChildOccupancy;
}
public RateSupplier minLengthOfStay(Integer minLengthOfStay) {
this.minLengthOfStay = minLengthOfStay;
return this;
}
/**
* Control the minimum length of stay at the day-level. This means that a guest arriving within this date range is required to stay at least these number of days in order to get this rate. Leave empty if you don't want to update this property.
* @return minLengthOfStay
**/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_MIN_LENGTH_OF_STAY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getMinLengthOfStay() {
return minLengthOfStay;
}
@JsonProperty(JSON_PROPERTY_MIN_LENGTH_OF_STAY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMinLengthOfStay(Integer minLengthOfStay) {
this.minLengthOfStay = minLengthOfStay;
}
public RateSupplier maxLengthOfStay(Integer maxLengthOfStay) {
this.maxLengthOfStay = maxLengthOfStay;
return this;
}
/**
* Control the maximum length of stay at the day-level. This means that a guest arriving within this date range is required to stay no longer than these number of days in order to get this rate. Leave empty if you don't want to update this property.
* @return maxLengthOfStay
**/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_MAX_LENGTH_OF_STAY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getMaxLengthOfStay() {
return maxLengthOfStay;
}
@JsonProperty(JSON_PROPERTY_MAX_LENGTH_OF_STAY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMaxLengthOfStay(Integer maxLengthOfStay) {
this.maxLengthOfStay = maxLengthOfStay;
}
public RateSupplier singleOccupancyRateModifier(VariableChargeSupplier singleOccupancyRateModifier) {
this.singleOccupancyRateModifier = singleOccupancyRateModifier;
return this;
}
/**
* Get singleOccupancyRateModifier
* @return singleOccupancyRateModifier
**/
@jakarta.annotation.Nullable
@Valid
@JsonProperty(JSON_PROPERTY_SINGLE_OCCUPANCY_RATE_MODIFIER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public VariableChargeSupplier getSingleOccupancyRateModifier() {
return singleOccupancyRateModifier;
}
@JsonProperty(JSON_PROPERTY_SINGLE_OCCUPANCY_RATE_MODIFIER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSingleOccupancyRateModifier(VariableChargeSupplier singleOccupancyRateModifier) {
this.singleOccupancyRateModifier = singleOccupancyRateModifier;
}
public RateSupplier extraPaxRateModifier(VariableChargeSupplier extraPaxRateModifier) {
this.extraPaxRateModifier = extraPaxRateModifier;
return this;
}
/**
* Get extraPaxRateModifier
* @return extraPaxRateModifier
**/
@jakarta.annotation.Nullable
@Valid
@JsonProperty(JSON_PROPERTY_EXTRA_PAX_RATE_MODIFIER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public VariableChargeSupplier getExtraPaxRateModifier() {
return extraPaxRateModifier;
}
@JsonProperty(JSON_PROPERTY_EXTRA_PAX_RATE_MODIFIER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setExtraPaxRateModifier(VariableChargeSupplier extraPaxRateModifier) {
this.extraPaxRateModifier = extraPaxRateModifier;
}
public RateSupplier extraChildRateModifier(VariableChargeSupplier extraChildRateModifier) {
this.extraChildRateModifier = extraChildRateModifier;
return this;
}
/**
* Get extraChildRateModifier
* @return extraChildRateModifier
**/
@jakarta.annotation.Nullable
@Valid
@JsonProperty(JSON_PROPERTY_EXTRA_CHILD_RATE_MODIFIER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public VariableChargeSupplier getExtraChildRateModifier() {
return extraChildRateModifier;
}
@JsonProperty(JSON_PROPERTY_EXTRA_CHILD_RATE_MODIFIER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setExtraChildRateModifier(VariableChargeSupplier extraChildRateModifier) {
this.extraChildRateModifier = extraChildRateModifier;
}
public RateSupplier available(Boolean available) {
this.available = available;
return this;
}
/**
* Convenience data point that tells you if there is availability on this day. It tests that quantity is greater than 0, rate amount is valid and master flag is true.
* @return available
**/
@jakarta.annotation.Nullable
@JsonProperty(JSON_PROPERTY_AVAILABLE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getAvailable() {
return available;
}
@JsonProperty(JSON_PROPERTY_AVAILABLE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAvailable(Boolean available) {
this.available = available;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
RateSupplier rateSupplier = (RateSupplier) o;
return Objects.equals(this.identifier, rateSupplier.identifier) &&
Objects.equals(this.hotelIdentifier, rateSupplier.hotelIdentifier) &&
Objects.equals(this.rateSource, rateSupplier.rateSource) &&
Objects.equals(this.masterRateIdentifier, rateSupplier.masterRateIdentifier) &&
Objects.equals(this.ratePlanIdentifier, rateSupplier.ratePlanIdentifier) &&
Objects.equals(this.guestRoomIdentifier, rateSupplier.guestRoomIdentifier) &&
Objects.equals(this.rate, rateSupplier.rate) &&
Objects.equals(this.master, rateSupplier.master) &&
Objects.equals(this.closedOnArrival, rateSupplier.closedOnArrival) &&
Objects.equals(this.closedOnDeparture, rateSupplier.closedOnDeparture) &&
Objects.equals(this.date, rateSupplier.date) &&
Objects.equals(this.quantity, rateSupplier.quantity) &&
Objects.equals(this.minOccupancy, rateSupplier.minOccupancy) &&
Objects.equals(this.maxOccupancy, rateSupplier.maxOccupancy) &&
Objects.equals(this.includedAdultOccupancy, rateSupplier.includedAdultOccupancy) &&
Objects.equals(this.includedChildOccupancy, rateSupplier.includedChildOccupancy) &&
Objects.equals(this.maxAdultOccupancy, rateSupplier.maxAdultOccupancy) &&
Objects.equals(this.maxChildOccupancy, rateSupplier.maxChildOccupancy) &&
Objects.equals(this.minLengthOfStay, rateSupplier.minLengthOfStay) &&
Objects.equals(this.maxLengthOfStay, rateSupplier.maxLengthOfStay) &&
Objects.equals(this.singleOccupancyRateModifier, rateSupplier.singleOccupancyRateModifier) &&
Objects.equals(this.extraPaxRateModifier, rateSupplier.extraPaxRateModifier) &&
Objects.equals(this.extraChildRateModifier, rateSupplier.extraChildRateModifier) &&
Objects.equals(this.available, rateSupplier.available);
}
@Override
public int hashCode() {
return Objects.hash(identifier, hotelIdentifier, rateSource, masterRateIdentifier, ratePlanIdentifier, guestRoomIdentifier, rate, master, closedOnArrival, closedOnDeparture, date, quantity, minOccupancy, maxOccupancy, includedAdultOccupancy, includedChildOccupancy, maxAdultOccupancy, maxChildOccupancy, minLengthOfStay, maxLengthOfStay, singleOccupancyRateModifier, extraPaxRateModifier, extraChildRateModifier, available);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class RateSupplier {\n");
sb.append(" identifier: ").append(toIndentedString(identifier)).append("\n");
sb.append(" hotelIdentifier: ").append(toIndentedString(hotelIdentifier)).append("\n");
sb.append(" rateSource: ").append(toIndentedString(rateSource)).append("\n");
sb.append(" masterRateIdentifier: ").append(toIndentedString(masterRateIdentifier)).append("\n");
sb.append(" ratePlanIdentifier: ").append(toIndentedString(ratePlanIdentifier)).append("\n");
sb.append(" guestRoomIdentifier: ").append(toIndentedString(guestRoomIdentifier)).append("\n");
sb.append(" rate: ").append(toIndentedString(rate)).append("\n");
sb.append(" master: ").append(toIndentedString(master)).append("\n");
sb.append(" closedOnArrival: ").append(toIndentedString(closedOnArrival)).append("\n");
sb.append(" closedOnDeparture: ").append(toIndentedString(closedOnDeparture)).append("\n");
sb.append(" date: ").append(toIndentedString(date)).append("\n");
sb.append(" quantity: ").append(toIndentedString(quantity)).append("\n");
sb.append(" minOccupancy: ").append(toIndentedString(minOccupancy)).append("\n");
sb.append(" maxOccupancy: ").append(toIndentedString(maxOccupancy)).append("\n");
sb.append(" includedAdultOccupancy: ").append(toIndentedString(includedAdultOccupancy)).append("\n");
sb.append(" includedChildOccupancy: ").append(toIndentedString(includedChildOccupancy)).append("\n");
sb.append(" maxAdultOccupancy: ").append(toIndentedString(maxAdultOccupancy)).append("\n");
sb.append(" maxChildOccupancy: ").append(toIndentedString(maxChildOccupancy)).append("\n");
sb.append(" minLengthOfStay: ").append(toIndentedString(minLengthOfStay)).append("\n");
sb.append(" maxLengthOfStay: ").append(toIndentedString(maxLengthOfStay)).append("\n");
sb.append(" singleOccupancyRateModifier: ").append(toIndentedString(singleOccupancyRateModifier)).append("\n");
sb.append(" extraPaxRateModifier: ").append(toIndentedString(extraPaxRateModifier)).append("\n");
sb.append(" extraChildRateModifier: ").append(toIndentedString(extraChildRateModifier)).append("\n");
sb.append(" available: ").append(toIndentedString(available)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy